[ad_1]
Introduction
The sphere of knowledge science is ever evolving. New instruments and strategies preserve rising daily. Within the present job state of affairs, significantly in 2024, professionals are anticipated to maintain themselves up to date on these adjustments. All kinds of companies are looking for expert knowledge scientists who will help them decipher their knowledge sensibly and preserve tempo with others. No matter whether or not you’re skilled or a novice, acing these coding interview questions performs a significant function in securing that dream knowledge science job. We’re right here that will help you get by way of these new-age interviews of 2024, with this complete information of knowledge science coding questions and solutions.
Additionally Learn: Find out how to Put together for Information Science Interview in 2024?
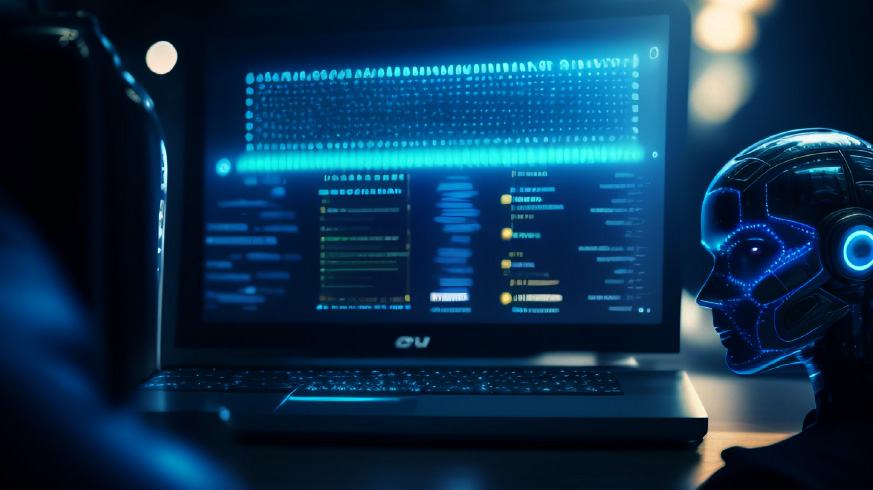
Information Science Coding Questions and Solutions
The intention behind in the present day’s knowledge science coding interviews is to guage your problem-solving capabilities. Additionally they check your effectivity in coding, in addition to your grasp of assorted algorithms and knowledge buildings. The questions sometimes mirror real-life eventualities which permit the evaluators to check extra than simply your technical abilities. Additionally they assess your capability for important pondering and the way virtually you’ll be able to apply your information in real-life conditions.
We’ve compiled a listing of the 40 most-asked and most academic knowledge science coding questions and solutions that you could be come throughout in interviews in 2024. For those who’re preparing for an interview or just trying to improve your skills, this listing will offer you a robust base to strategy the hurdles of knowledge science coding.
In case you’re questioning how realizing these coding questions and coaching on them will enable you to, let me clarify. Firstly, it helps you put together for troublesome interviews with main tech corporations, throughout which you’ll be able to stand out if you understand widespread issues and patterns, effectively prematurely. Secondly, going by way of such issues improves your analytical abilities, serving to you develop into a simpler knowledge scientist in your day-to-day work. Thirdly, these coding questions will enhance the cleanliness and effectivity of your code writing — an essential benefit in any data-related place.
So let’s get began — let’s start writing our code in direction of triumph within the area of knowledge science!
Additionally Learn: Prime 100 Information Science Interview Questions & Solutions 2024
Python Coding Questions
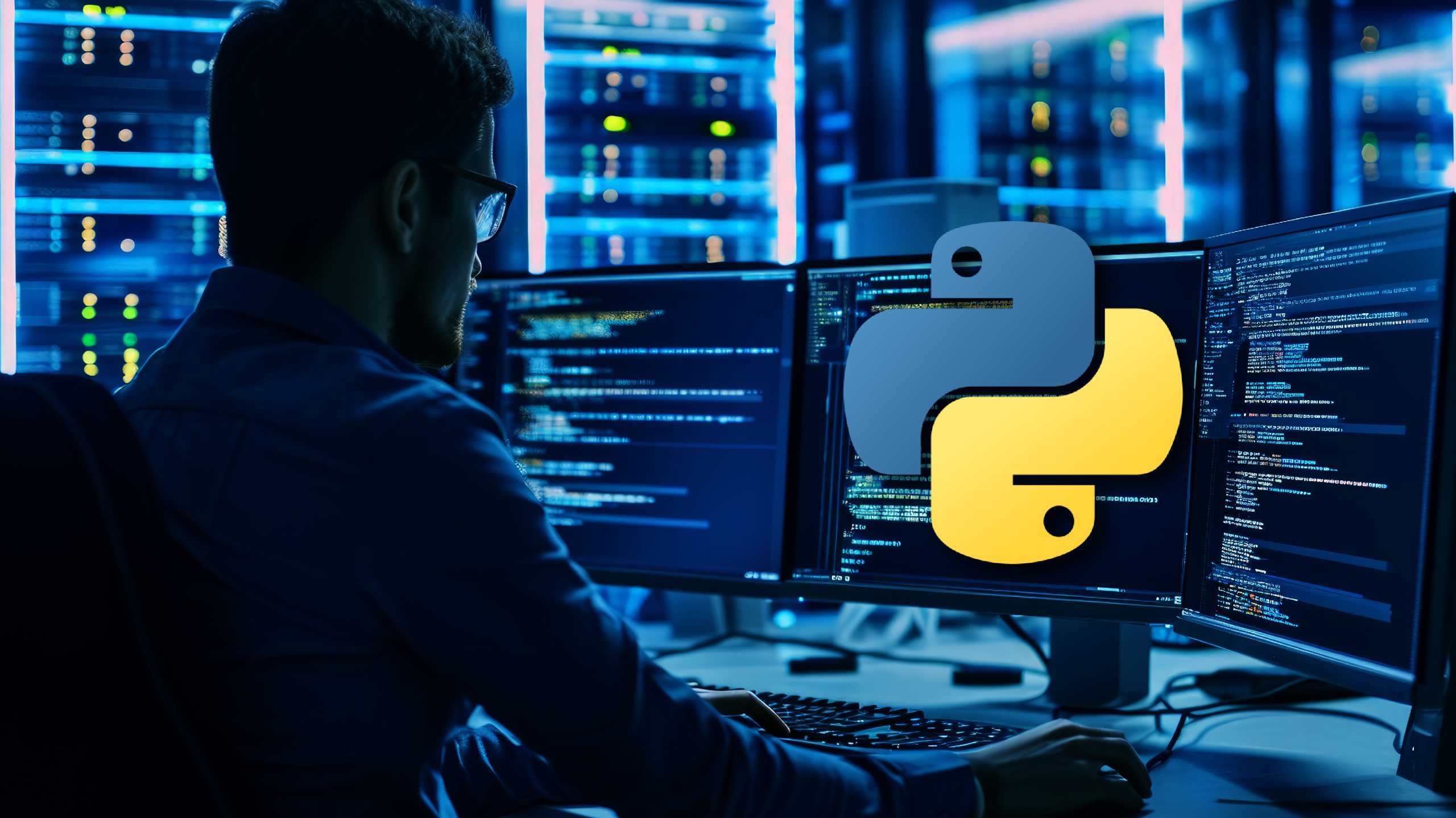
Q1. Write a Python perform to reverse a string.
Ans. To reverse a string in Python, you should use slicing. Right here’s how you are able to do it:
def reverse_string(s):
return s[::-1]
The slicing notation s[::-1] begins from the tip of the string and strikes to the start, successfully reversing it. It’s a concise and environment friendly option to obtain this.
Q2. Clarify the distinction between a listing and a tuple in Python.
Ans. The principle distinction between a listing and a tuple in Python is mutability. An inventory is mutable, that means you’ll be able to change its content material after it’s created. You possibly can add, take away, or modify parts. Right here’s an instance:
my_list = [1, 2, 3]
my_list.append(4) # Now my_list is [1, 2, 3, 4]
Then again, a tuple is immutable. As soon as it’s created, you’ll be able to’t change its content material. Tuples are outlined utilizing parentheses. Right here’s an instance:
my_tuple = (1, 2, 3)
# my_tuple.append(4) would elevate an error as a result of tuples don’t help merchandise task
Selecting between a listing and a tuple relies on whether or not it’s worthwhile to modify the information. Tuples can be barely quicker and are sometimes used when the information mustn’t change.
Q3. Write a Python perform to examine if a given quantity is prime.
Ans. To examine if a quantity is prime, it’s worthwhile to check if it’s solely divisible by 1 and itself. Right here’s a easy perform to try this:
def is_prime(n):
if n <= 1:
return False
for i in vary(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
This perform first checks if the quantity is lower than or equal to 1, which aren’t prime numbers. Then it checks divisibility from 2 as much as the sq. root of the quantity. If any quantity divides evenly, it’s not prime.
Q4. Clarify the distinction between == and is in Python.
Ans. In Python, == checks for worth equality. That means, it checks if the values of two variables are the identical. For instance:
a = [1, 2, 3]
b = [1, 2, 3]print(a == b) # True, as a result of the values are the identical
print(a == b) # True, as a result of the values are the identical
Then again, it checks for identification, that means it checks if two variables level to the identical object in reminiscence. For instance:
a = [1, 2, 3]
b = [1, 2, 3]
print(a is b) # False, as a result of they're totally different objects in reminiscence
c = a
print(a is c) # True, as a result of c factors to the identical object as a
This distinction is essential when coping with mutable objects like lists.
Q5. Write a Python perform to calculate the factorial of a quantity.
Ans. Calculating the factorial of a quantity may be finished utilizing both a loop or recursion. Right here’s an instance utilizing a loop:
def factorial(n):
if n < 0:
return "Invalid enter"
end result = 1
for i in vary(1, n + 1):
end result *= I
return end result
This perform initializes the end result to 1 and multiplies it by every integer as much as n. It’s simple and avoids the chance of stack overflow with giant numbers that recursion may encounter.
Q6. What’s a generator in Python? Present an instance.
Ans. Turbines are a particular sort of iterator in Python that means that you can iterate by way of a sequence of values lazily, that means they generate values on the fly and save reminiscence. You create a generator utilizing a perform and the yield key phrase. Right here’s a easy instance:
def my_generator():
for i in vary(1, 4):
yield I
gen = my_generator()
print(subsequent(gen)) # 1
print(subsequent(gen)) # 2
print(subsequent(gen)) # 3
Utilizing yield as an alternative of return permits the perform to provide a sequence of values over time, pausing and resuming as wanted. That is very helpful for dealing with giant datasets or streams of knowledge.
Q7. Clarify the distinction between map and filter features in Python.
Ans. Each map and filter are built-in features in Python used for practical programming, however they serve totally different functions. The map perform applies a given perform to all gadgets in an enter listing (or any iterable) and returns a brand new listing of outcomes. For instance:
def sq.(x):
return x * x
numbers = [1, 2, 3, 4]
squared = map(sq., numbers)
print(listing(squared)) # [1, 4, 9, 16]
Then again, the filter perform applies a given perform to all gadgets in an enter listing and returns solely the gadgets for which the perform returns True. Right here’s an instance:
def is_even(x):
return x % 2 == 0
numbers = [1, 2, 3, 4]
evens = filter(is_even, numbers)
print(listing(evens)) # [2, 4]
So, map transforms every merchandise, whereas filter selects gadgets based mostly on a situation. Each are very highly effective instruments for processing knowledge effectively.
Try extra Python interview questions.
Information Buildings and Algorithms Coding Questions
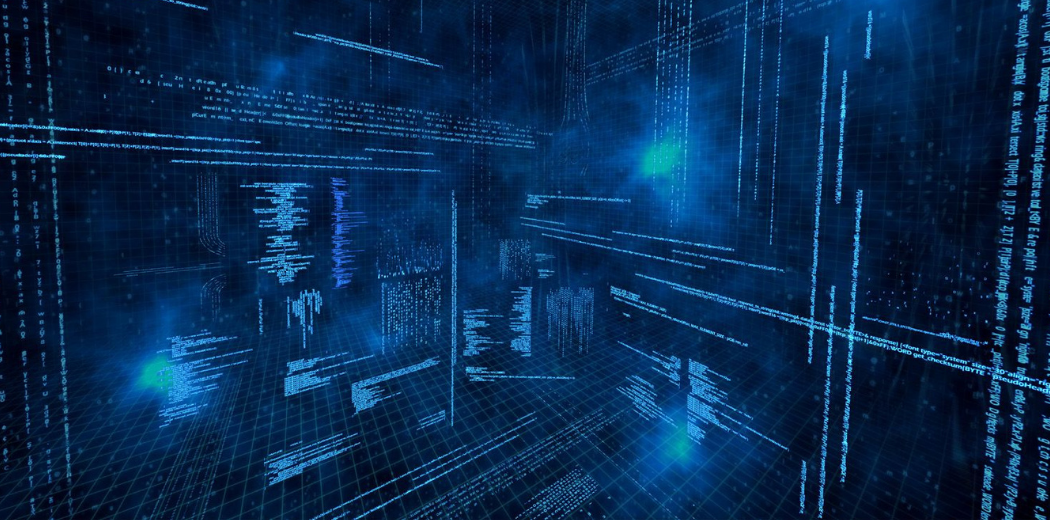
Q8. Implement a binary search algorithm in Python.
Ans. Binary search is an environment friendly algorithm for locating an merchandise from a sorted listing of things. It really works by repeatedly dividing the search interval in half. If the worth of the search secret is lower than the merchandise in the midst of the interval, slim the interval to the decrease half. In any other case, slim it to the higher half. Right here’s how one can implement it in Python:
def binary_search(arr, goal):
left, proper = 0, len(arr) - 1
whereas left <= proper:
mid = (left + proper) // 2
if arr[mid] == goal:
return mid
elif arr[mid] < goal:
left = mid + 1
else:
proper = mid - 1
return -1 # Goal not discovered
On this perform, we initialize two pointers, left and proper, to the beginning and finish of the listing, respectively. We then repeatedly examine the center component and alter the pointers based mostly on the comparability with the goal worth.
Q9. Clarify how a hash desk works. Present an instance.
Ans. A hash desk is a knowledge construction that shops key-value pairs. It makes use of a hash perform to compute an index into an array of buckets or slots, from which the specified worth may be discovered. The principle benefit of hash tables is their environment friendly knowledge retrieval, as they permit for average-case constant-time complexity, O(1), for lookups, insertions, and deletions.
Right here’s a easy instance in Python utilizing a dictionary, which is actually a hash desk:
# Making a hash desk (dictionary)
hash_table = {}
# Including key-value pairs
hash_table["name"] = "Alice"
hash_table["age"] = 25
hash_table["city"] = "New York"
# Retrieving values
print(hash_table["name"]) # Output: Alice
print(hash_table["age"]) # Output: 25
print(hash_table["city"]) # Output: New York
On this instance, the hash perform is implicitly dealt with by Python’s dictionary implementation. Keys are hashed to provide a singular index the place the corresponding worth is saved.
Q10. Implement a bubble type algorithm in Python.
Ans. Bubble type is an easy sorting algorithm that repeatedly steps by way of the listing, compares adjoining parts, and swaps them if they’re within the flawed order. The move by way of the listing is repeated till the listing is sorted. Right here’s a Python implementation:
def bubble_sort(arr):
n = len(arr)
for i in vary(n):
for j in vary(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
# Instance utilization
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
print("Sorted array:", arr)
On this perform, now we have two nested loops. The inside loop performs the comparisons and swaps, and the outer loop ensures that the method is repeated till your complete listing is sorted.
Q11. Clarify the distinction between depth-first search (DFS) and breadth-first search (BFS).
Ans. Depth-first search (DFS) and breadth-first search (BFS) are two basic algorithms for traversing or looking out by way of a graph or tree knowledge construction.
DFS (Depth-First Search): This algorithm begins on the root (or an arbitrary node) and explores so far as attainable alongside every department earlier than backtracking. It makes use of a stack knowledge construction, both implicitly with recursion or explicitly with an iterative strategy.
def dfs(graph, begin, visited=None):
if visited is None:
visited = set()
visited.add(begin)
for subsequent in graph[start] - visited:
dfs(graph, subsequent, visited)
return visited
BFS (Breadth-First Search): This algorithm begins on the root (or an arbitrary node) and explores the neighbor nodes at the moment depth previous to transferring on to nodes on the subsequent depth stage. It makes use of a queue knowledge construction.
from collections import deque
def bfs(graph, begin):
visited = set()
queue = deque([start])
whereas queue:
vertex = queue.popleft()
if vertex not in visited:
visited.add(vertex)
queue.prolong(graph[vertex] - visited)
return visited
The first distinction is of their strategy: DFS goes deep into the graph first, whereas BFS explores all neighbors on the present depth earlier than going deeper. DFS may be helpful for pathfinding and connectivity checking, whereas BFS is usually used for locating the shortest path in an unweighted graph.
Q12. Implement a linked listing in Python.
Ans. A linked listing is a knowledge construction wherein parts are saved in nodes, and every node factors to the following node within the sequence. Right here’s how one can implement a easy singly linked listing in Python:
class Node:
def __init__(self, knowledge):
self.knowledge = knowledge
self.subsequent = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, knowledge):
new_node = Node(knowledge)
if not self.head:
self.head = new_node
return
last_node = self.head
whereas last_node.subsequent:
last_node = last_node.subsequent
last_node.subsequent = new_node
def print_list(self):
present = self.head
whereas present:
print(present.knowledge, finish=" -> ")
present = present.subsequent
print("None")
# Instance utilization
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
ll.print_list() # Output: 1 -> 2 -> 3 -> None
On this implementation, now we have a Node class to characterize every component within the listing and a LinkedList class to handle the nodes. The append methodology provides a brand new node to the tip of the listing, and the print_list methodology prints all parts.
Q13. Write a perform to seek out the nth Fibonacci quantity utilizing recursion.
Ans. The Fibonacci sequence is a sequence of numbers the place every quantity is the sum of the 2 previous ones, often beginning with 0 and 1. Right here’s a recursive perform to seek out the nth Fibonacci quantity:
def fibonacci(n):
if n <= 0:
return "Invalid enter"
elif n == 1:
return 0
elif n == 2:
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
# Instance utilization
print(fibonacci(10)) # Output: 34
This perform makes use of recursion to compute the Fibonacci quantity. The bottom circumstances deal with the primary two Fibonacci numbers (0 and 1), and the recursive case sums the earlier two Fibonacci numbers.
Q14. Clarify time complexity and area complexity.
Ans. Time complexity and area complexity are used to explain the effectivity of an algorithm.
Time Complexity: This measures the period of time an algorithm takes to finish as a perform of the size of the enter. It’s sometimes expressed utilizing Huge O notation, which describes the higher sure of the operating time. For instance, a linear search has a time complexity of O(n), that means its operating time will increase linearly with the dimensions of the enter.
# Instance of O(n) time complexity
def linear_search(arr, goal):
for i in vary(len(arr)):
if arr[i] == goal:
return I
return -1
House Complexity: This measures the quantity of reminiscence an algorithm makes use of as a perform of the size of the enter. It’s additionally expressed utilizing Huge O notation. For instance, the area complexity of an algorithm that makes use of a continuing quantity of additional reminiscence is O(1).
# Instance of O(1) area complexity
def example_function(arr):
complete = 0
for i in arr:
complete += I
return complete
Understanding these ideas helps you select essentially the most environment friendly algorithm for a given drawback, particularly when coping with giant datasets or constrained assets.
Try extra interview questions on knowledge buildings.
Pandas Coding Questions
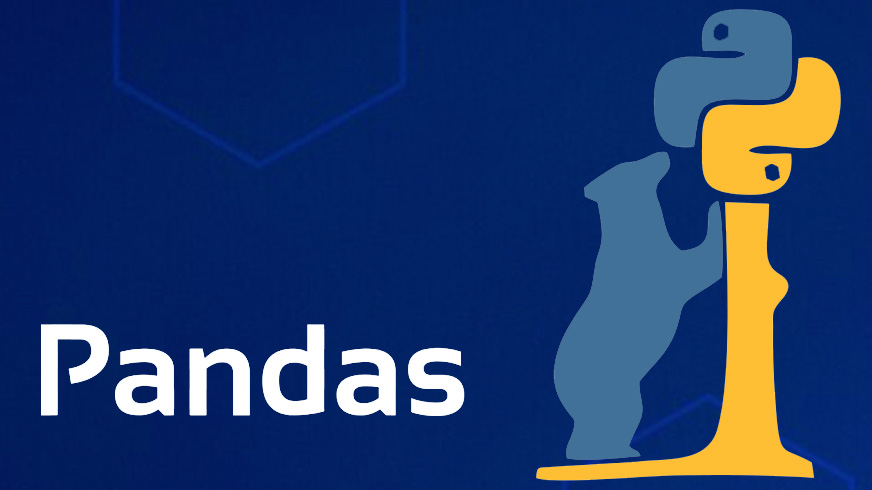
Q15. Given a dataset of retail transactions, write a Pandas script to carry out the next duties:
- Load the dataset from a CSV file named retail_data.csv.
- Show the primary 5 rows of the dataset.
- Clear the information by eradicating any rows with lacking values.
- Create a brand new column named TotalPrice that’s the product of Amount and UnitPrice.
- Group the information by Nation and calculate the overall TotalPrice for every nation.
- Kind the ensuing grouped knowledge by TotalPrice in descending order and show the highest 10 nations.
Assume the dataset has the next columns: InvoiceNo, StockCode, Description, Amount, InvoiceDate, UnitPrice, CustomerID, Nation
Ans. Right here’s how you are able to do it:
import pandas as pd
# Step 1: Load the dataset from a CSV file named 'retail_data.csv'
df = pd.read_csv('retail_data.csv')
# Step 2: Show the primary 5 rows of the dataset
print("First 5 rows of the dataset:")
print(df.head())
# Step 3: Clear the information by eradicating any rows with lacking values
df_cleaned = df.dropna()
# Step 4: Create a brand new column named 'TotalPrice' that's the product of 'Amount' and 'UnitPrice'
df_cleaned['TotalPrice'] = df_cleaned['Quantity'] * df_cleaned['UnitPrice']
# Step 5: Group the information by 'Nation' and calculate the overall 'TotalPrice' for every nation
country_totals = df_cleaned.groupby('Nation')['TotalPrice'].sum().reset_index()
# Step 6: Kind the ensuing grouped knowledge by 'TotalPrice' in descending order and show the highest 10 nations
top_countries = country_totals.sort_values(by='TotalPrice', ascending=False).head(10)
print("Prime 10 nations by complete gross sales:")
print(top_countries)
Q16. How do you learn a CSV file right into a DataFrame in Pandas?
Ans. Studying a CSV file right into a DataFrame is easy with Pandas. You employ the read_csv perform. Right here’s how you are able to do it:
import pandas as pd
# Studying a CSV file right into a DataFrame
df = pd.read_csv('path_to_file.csv')
# Displaying the primary few rows of the DataFrame
print(df.head())
This perform reads the CSV file from the desired path and hundreds it right into a DataFrame, which is a robust knowledge construction for knowledge manipulation and evaluation.
Q17. How do you choose particular rows and columns in a DataFrame?
Ans. Choosing particular rows and columns in a DataFrame may be finished utilizing varied strategies. Listed here are a number of examples:
1. Choosing columns:
# Choose a single column
column = df['column_name']
# Choose a number of columns
columns = df[['column1', 'column2']]
2. Choosing rows:
# Choose rows by index
rows = df[0:5] # First 5 rows
3. Choosing rows and columns:
# Choose particular rows and columns
subset = df.loc[0:5, ['column1', 'column2']] # Utilizing labels
subset_iloc = df.iloc[0:5, [0, 1]] # Utilizing integer positions
These strategies let you entry and manipulate particular elements of your knowledge effectively.
Q18. What’s the distinction between loc and iloc in Pandas?
Ans. The principle distinction between loc and iloc lies in how you choose knowledge from a DataFrame:
loc: Makes use of labels or boolean arrays to pick knowledge. It’s label-based.
# Choose rows and columns by label
df.loc[0:5, ['column1', 'column2']]
iloc: Makes use of integer positions to pick knowledge. It’s position-based.
# Choose rows and columns by integer place
df.iloc[0:5, [0, 1]]
Basically, loc is used when you understand the labels of your knowledge, and iloc is used when you understand the index positions.
Q19. How do you deal with lacking values in a DataFrame?
Ans. Dealing with lacking values is essential for knowledge evaluation. Pandas supplies a number of strategies to take care of lacking knowledge.
Detecting lacking values:
# Detect lacking values
missing_values = df.isnull()
Dropping lacking values:
# Drop rows with lacking values
df_cleaned = df.dropna()
# Drop columns with lacking values
df_cleaned = df.dropna(axis=1)
Filling lacking values:
# Fill lacking values with a particular worth
df_filled = df.fillna(0)
# Fill lacking values with the imply of the column
df_filled = df.fillna(df.imply())
These strategies let you clear your knowledge, making it prepared for evaluation.
Q20. How do you merge two DataFrames in Pandas?
Ans. To merge two DataFrames, you should use the merge perform, which is analogous to SQL joins. Right here’s an instance:
# Creating two DataFrames
df1 = pd.DataFrame({'key': ['A', 'B', 'C'], 'value1': [1, 2, 3]})
df2 = pd.DataFrame({'key': ['A', 'B', 'D'], 'value2': [4, 5, 6]})
# Merging DataFrames on the 'key' column
merged_df = pd.merge(df1, df2, on='key', how='inside')
# Displaying the merged DataFrame
print(merged_df)
On this instance, how=’inside’ specifies an inside be part of. You too can use ‘left’, ‘proper’, or ‘outer’ for various kinds of joins.
Q21. What’s groupby in Pandas? Present an instance.
Ans. The groupby perform in Pandas is used to separate the information into teams based mostly on some standards, apply a perform to every group, after which mix the outcomes. Right here’s a easy instance:
# Making a DataFrame
knowledge = {'Class': ['A', 'B', 'A', 'B'], 'Values': [10, 20, 30, 40]}
df = pd.DataFrame(knowledge)
# Grouping by 'Class' and calculating the sum of 'Values'
grouped = df.groupby('Class').sum()
# Displaying the grouped DataFrame
print(grouped)
On this instance, the DataFrame is grouped by the ‘Class’ column, and the sum of the ‘Values’ column is calculated for every group. Grouping knowledge may be very highly effective for aggregation and abstract statistics.
Be taught extra about Pandas with this complete course from Analytics Vidhya.
NumPy Coding Questions
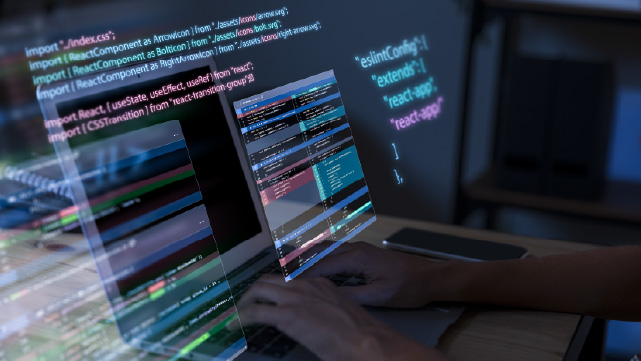
Q22. Given a 2D array, write a NumPy script to carry out the next duties:
- Create a 5×5 matrix with values starting from 1 to 25.
- Reshape the matrix to 1×25 after which again to five×5.
- Compute the sum of all parts within the matrix.
- Calculate the imply of every row.
- Change all values higher than 10 with 10.
- Transpose of the matrix.
Ans. Right here’s how you are able to do it:
import numpy as np
# Step 1: Create a 5x5 matrix with values starting from 1 to 25
matrix = np.arange(1, 26).reshape(5, 5)
print("Unique 5x5 matrix:")
print(matrix)
# Step 2: Reshape the matrix to 1x25 after which again to 5x5
matrix_reshaped = matrix.reshape(1, 25)
print("Reshaped to 1x25:")
print(matrix_reshaped)
matrix_back_to_5x5 = matrix_reshaped.reshape(5, 5)
print("Reshaped again to 5x5:")
print(matrix_back_to_5x5)
# Step 3: Compute the sum of all parts within the matrix
sum_of_elements = np.sum(matrix)
print("Sum of all parts:")
print(sum_of_elements)
# Step 4: Calculate the imply of every row
mean_of_rows = np.imply(matrix, axis=1)
print("Imply of every row:")
print(mean_of_rows)
# Step 5: Change all values higher than 10 with 10
matrix_clipped = np.clip(matrix, None, 10)
print("Matrix with values higher than 10 changed with 10:")
print(matrix_clipped)
# Step 6: Transpose the matrix
matrix_transposed = np.transpose(matrix)
print("Transposed matrix:")
print(matrix_transposed)
Q23. How do you create a NumPy array?
Ans. Making a NumPy array is easy. You should utilize the array perform from the NumPy library. Right here’s an instance:
import numpy as np
# Making a NumPy array from a listing
my_array = np.array([1, 2, 3, 4, 5])
# Displaying the array
print(my_array)
This code converts a Python listing right into a NumPy array. You too can create arrays with particular shapes and values utilizing features like np.zeros, np.ones, and np.arange.
Q24. Clarify the distinction between a Python listing and a NumPy array with an instance.
Ans. Whereas each Python lists and NumPy arrays can retailer collections of things, there are key variations between them:
- Homogeneity: NumPy arrays require all parts to be of the identical knowledge sort, which makes them extra environment friendly for numerical operations. Python lists can include parts of various knowledge varieties.
- Efficiency: NumPy arrays are extra reminiscence environment friendly and quicker resulting from their homogeneous nature and the underlying implementation in C.
- Performance: NumPy supplies an enormous array of features and strategies for mathematical and statistical operations which can be optimized for arrays, which aren’t obtainable with Python lists.
Right here’s an instance evaluating a Python listing and a NumPy array:
import numpy as np
# Python listing
py_list = [1, 2, 3, 4, 5]
# NumPy array
np_array = np.array([1, 2, 3, 4, 5])
# Factor-wise addition
np_array += 1
# Python listing requires a loop or comprehension for a similar operation
py_list = [x + 1 for x in py_list]
NumPy arrays are the go-to for performance-critical purposes, particularly in knowledge science and numerical computing.
Q25. How do you carry out element-wise operations in NumPy?
Ans. Factor-wise operations in NumPy are simple and environment friendly. NumPy means that you can carry out operations instantly on arrays with out the necessity for specific loops. Right here’s an instance:
import numpy as np
# Creating two NumPy arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Factor-wise addition
result_add = array1 + array2
# Factor-wise multiplication
result_mul = array1 * array2
# Displaying the outcomes
print("Addition:", result_add) # [5, 7, 9]
print("Multiplication:", result_mul) # [4, 10, 18]
On this instance, addition and multiplication are carried out element-wise, that means every component of array1 is added to the corresponding component of array2, and the identical for multiplication.
Q26. What’s broadcasting in NumPy? Present an instance.
Ans. Broadcasting is a robust characteristic in NumPy that means that you can carry out operations on arrays of various shapes. NumPy robotically expands the smaller array to match the form of the bigger array with out making copies of knowledge. Right here’s an instance:
import numpy as np
# Making a 1D array
array1 = np.array([1, 2, 3])
# Making a 2D array
array2 = np.array([[4], [5], [6]])
# Broadcasting array1 throughout array2
end result = array1 + array2
# Displaying the end result
print(end result)
The output will probably be:
[[5 6 7]
[6 7 8]
[7 8 9]]
On this instance, array1 is broadcasted throughout array2 to carry out element-wise addition. Broadcasting simplifies code and improves effectivity.
Q27. How do you transpose a NumPy array?
Ans. Transposing an array means swapping its rows and columns. You should utilize the transpose methodology or the .T attribute. Right here’s how you are able to do it:
import numpy as np
# Making a 2D array
array = np.array([[1, 2, 3], [4, 5, 6]])
# Transposing the array
transposed_array = array.T
# Displaying the transposed array
print(transposed_array)
The output will probably be:
[[1 4]
[2 5]
[3 6]]
This operation is especially helpful in linear algebra and knowledge manipulation.
Q28. How do you carry out matrix multiplication in NumPy?
Ans. Matrix multiplication in NumPy may be carried out utilizing the dot perform or the @ operator. Right here’s an instance:
import numpy as np
# Creating two matrices
matrix1 = np.array([[1, 2], [3, 4]])
matrix2 = np.array([[5, 6], [7, 8]])
# Performing matrix multiplication
end result = np.dot(matrix1, matrix2)
# Alternatively, utilizing the @ operator
result_alt = matrix1 @ matrix2
# Displaying the end result
print(end result)
The output will probably be:
[[19 22]
[43 50]]
Matrix multiplication combines rows of the primary matrix with columns of the second matrix, which is a typical operation in varied numerical and machine-learning purposes.
SQL Coding Questions
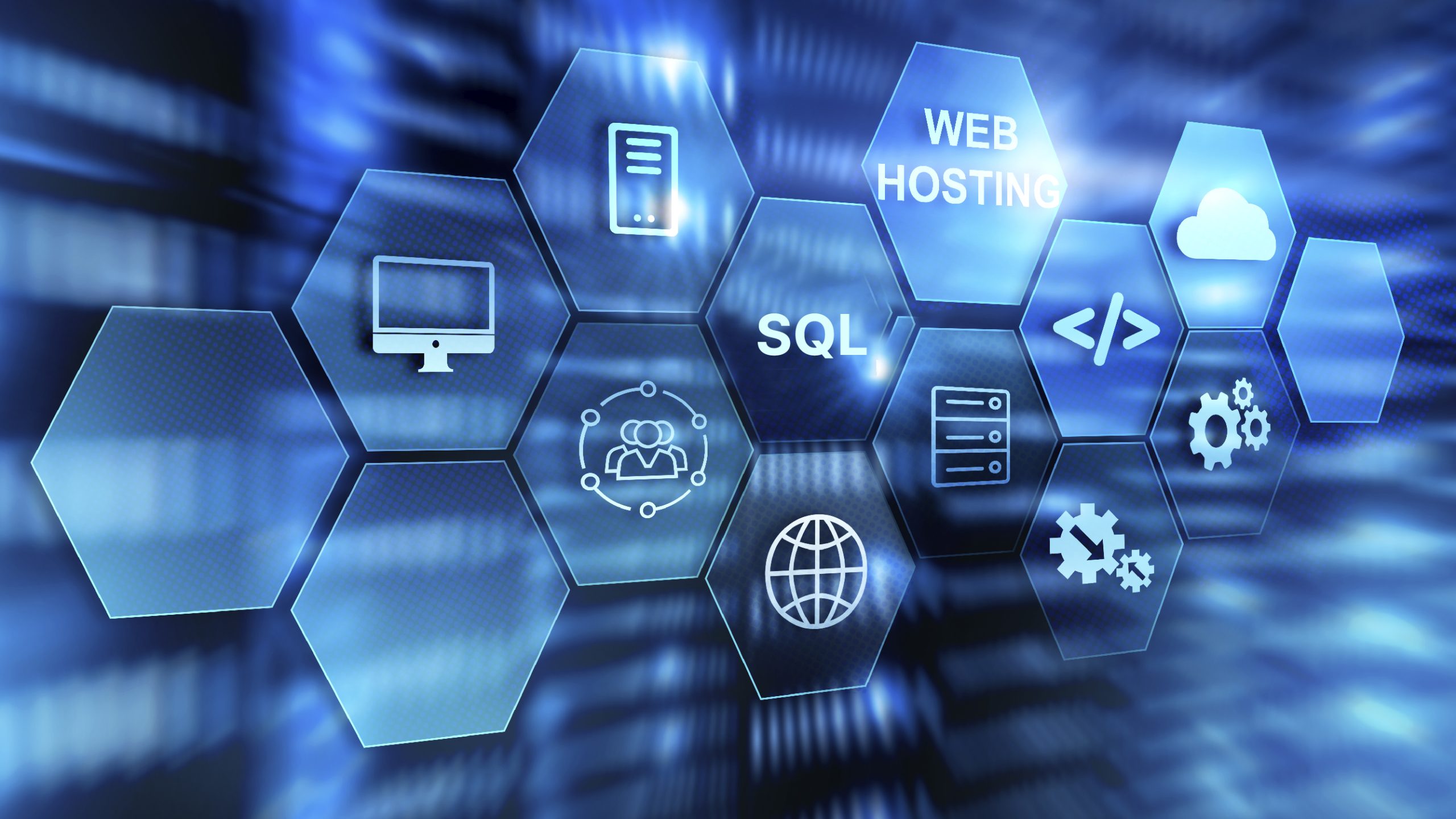
Q29. Write a SQL question that finds all prospects who positioned an order with a complete quantity higher than $100 within the final month (from in the present day’s date). Assume the database has the next tables:
- prospects: Accommodates buyer info like customer_id, identify, e-mail
- orders: Accommodates order particulars like order_id, customer_id, order_date, total_amount
Ans: Right here’s the way you write the question for it:
SELECT prospects.identify, orders.order_date, orders.total_amount
FROM prospects
INNER JOIN orders ON prospects.customer_id = orders.customer_id
WHERE orders.order_date >= DATE_SUB(CURDATE(), INTERVAL 1 MONTH)
AND orders.total_amount > 100;
Q30. Write an SQL question to pick all data from a desk.
Ans. To pick out all data from a desk, you utilize the SELECT assertion with the asterisk (*) wildcard, which suggests ‘all columns’. Right here’s the syntax:
SELECT * FROM table_name;
For instance, when you have a desk named workers, the question can be:
SELECT * FROM workers;
This question retrieves all columns and rows from the staff desk.
Q31. Clarify the distinction between GROUP BY and HAVING clauses in SQL.
Ans. Each GROUP BY and HAVING are utilized in SQL to arrange and filter knowledge, however they serve totally different functions:
GROUP BY: This clause is used to group rows which have the identical values in specified columns into aggregated knowledge. It’s typically used with combination features like COUNT, SUM, AVG, and so forth.
SELECT division, COUNT(*)
FROM workers
GROUP BY division;
HAVING: This clause is used to filter teams created by the GROUP BY clause. It acts like a WHERE clause, however is used after the aggregation.
SELECT division, COUNT(*)
FROM workers
GROUP BY division
HAVING COUNT(*) > 10;
In abstract, GROUP BY creates the teams, and HAVING filters these teams based mostly on a situation.
Q32. Write an SQL question to seek out the second-highest wage from an Worker desk.
Ans. To seek out the second-highest wage, you should use the LIMIT clause together with a subquery. Right here’s one option to do it:
SELECT MAX(wage)
FROM workers
WHERE wage < (SELECT MAX(wage) FROM workers);
This question first finds the very best wage after which makes use of it to seek out the utmost wage that’s lower than this highest wage, successfully supplying you with the second-highest wage.
Q33. Clarify the distinction between INNER JOIN, LEFT JOIN, and RIGHT JOIN.
Ans. These JOIN operations are used to mix rows from two or extra tables based mostly on a associated column between them:
INNER JOIN: Returns solely the rows which have matching values in each tables.
SELECT a.column1, b.column2
FROM table1 a
INNER JOIN table2 b ON a.common_column = b.common_column;
LEFT JOIN (or LEFT OUTER JOIN): Returns all rows from the left desk, and the matched rows from the best desk. If no match is discovered, NULL values are returned for columns from the best desk.
SELECT a.column1, b.column2
FROM table1 a
LEFT JOIN table2 b ON a.common_column = b.common_column;
RIGHT JOIN (or RIGHT OUTER JOIN): Returns all rows from the best desk, and the matched rows from the left desk. If no match is discovered, NULL values are returned for columns from the left desk.
SELECT a.column1, b.column2
FROM table1 a
RIGHT JOIN table2 b ON a.common_column = b.common_column;
These totally different JOIN varieties assist in retrieving the information as per the particular wants of the question.
Q34. Write an SQL question to rely the variety of workers in every division.
Ans. To rely the variety of workers in every division, you should use the GROUP BY clause together with the COUNT perform. Right here’s how:
SELECT division, COUNT(*) AS employee_count
FROM workers
GROUP BY division;
This question teams the staff by their division and counts the variety of workers in every group.
Q35. What’s a subquery in SQL? Present an instance.
Ans. A subquery, or inside question, is a question nested inside one other question. It may be utilized in varied locations just like the SELECT, INSERT, UPDATE, and DELETE statements, or inside different subqueries. Right here’s an instance:
SELECT identify, wage
FROM workers
WHERE wage > (SELECT AVG(wage) FROM workers);
On this instance, the subquery (SELECT AVG(wage) FROM workers) calculates the common wage of all workers. The outer question then selects the names and salaries of workers who earn greater than this common wage.
Try extra SQL coding questions.
Machine Studying Coding Questions
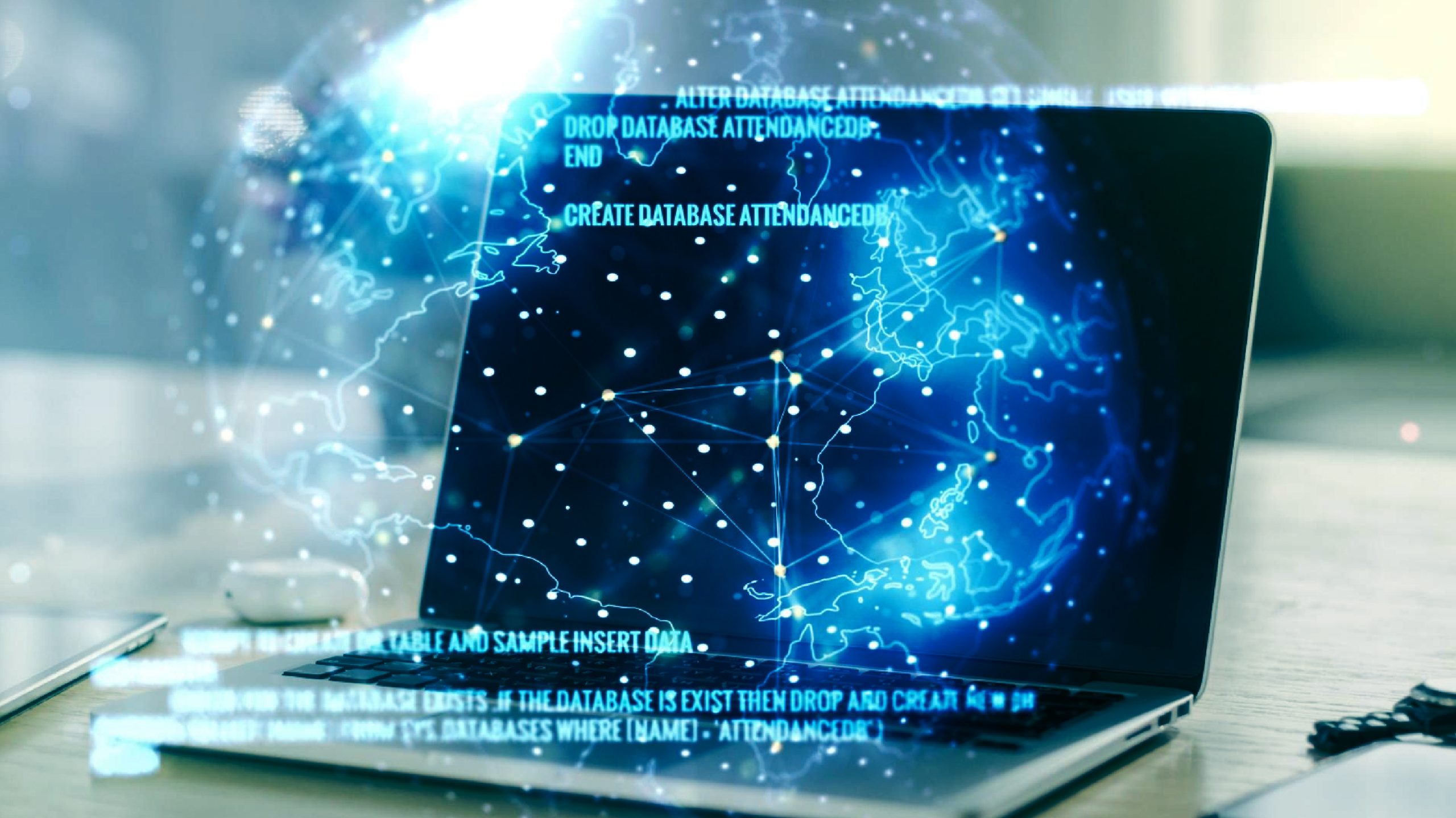
Q36. What’s overfitting? How do you forestall it?
Ans. Overfitting happens when a machine studying mannequin learns not solely the underlying patterns within the coaching knowledge but in addition the noise and outliers. This ends in glorious efficiency on the coaching knowledge however poor generalization to new, unseen knowledge. Listed here are a number of methods to forestall overfitting:
- Cross-Validation: Use strategies like k-fold cross-validation to make sure the mannequin performs effectively on totally different subsets of the information.
- Regularization: Add a penalty for bigger coefficients (L1 or L2 regularization) to simplify the mannequin.
from sklearn.linear_model import Ridge
mannequin = Ridge(alpha=1.0)
- Pruning (for choice timber): Trim the branches of a tree which have little significance.
- Early Stopping: Cease coaching when the mannequin efficiency on a validation set begins to degrade.
- Dropout (for neural networks): Randomly drop neurons throughout coaching to forestall co-adaptation.
from tensorflow.keras.layers import Dropout
mannequin.add(Dropout(0.5))
- Extra Information: Rising the dimensions of the coaching dataset will help the mannequin generalize higher.
Stopping overfitting is essential for constructing strong fashions that carry out effectively on new knowledge.
Q37. Clarify the distinction between supervised and unsupervised studying. Give an instance.
Ans. Supervised and unsupervised studying are two basic kinds of machine studying.
Supervised Studying: On this strategy, the mannequin is skilled on labeled knowledge, that means that every coaching instance comes with an related output label. The purpose is to be taught a mapping from inputs to outputs. Frequent duties embody classification and regression.
# Instance: Supervised studying with a classifier
from sklearn.ensemble import RandomForestClassifier
mannequin = RandomForestClassifier()
mannequin.match(X_train, y_train)
Unsupervised Studying: On this strategy, the mannequin is skilled on knowledge with out labeled responses. The purpose is to seek out hidden patterns or intrinsic buildings within the enter knowledge. Frequent duties embody clustering and dimensionality discount.
# Instance: Unsupervised studying with a clustering algorithm
from sklearn.cluster import KMeans
mannequin = KMeans(n_clusters=3)
mannequin.match(X_train)
The principle distinction lies within the presence or absence of labeled outputs throughout coaching. Supervised studying is used when the purpose is prediction, whereas unsupervised studying is used for locating patterns.
Q38. What’s the distinction between classification and regression?
Ans. Classification and regression are each kinds of supervised studying duties, however they serve totally different functions.
Classification: This entails predicting a categorical end result. The purpose is to assign inputs to one among a set of predefined lessons.
# Instance: Classification
from sklearn.linear_model import LogisticRegression
mannequin = LogisticRegression()
mannequin.match(X_train, y_train)
Regression: This entails predicting a steady end result. The purpose is to foretell a numeric worth based mostly on enter options.
# Instance: Regression
from sklearn.linear_model import LinearRegression
mannequin = LinearRegression()
mannequin.match(X_train, y_train)
In abstract, classification predicts discrete labels, whereas regression predicts steady values.
Q39. Write a Python script to carry out Principal Part Evaluation (PCA) on a dataset and plot the primary two principal elements.
Ans. I used an instance DataFrame df with three options. Carried out PCA to cut back the dimensionality to 2 elements utilizing PCA from sklearn and plotted the primary two principal elements utilizing matplotlib. Right here’s how you are able to do it:
import pandas as pd
from sklearn.decomposition import PCA
import matplotlib.pyplot as plt
# Instance DataFrame
df = pd.DataFrame({
'feature1': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10],
'feature2': [2, 3, 4, 5, 6, 7, 8, 9, 10, 11],
'feature3': [3, 4, 5, 6, 7, 8, 9, 10, 11, 12] })
X = df[['feature1', 'feature2', 'feature3']]
# Step 1: Apply PCA
pca = PCA(n_components=2)
principal_components = pca.fit_transform(X)
principal_df = pd.DataFrame(knowledge=principal_components, columns=['PC1', 'PC2'])
# Step 2: Plot the primary two principal elements
plt.scatter(principal_df['PC1'], principal_df['PC2'])
plt.xlabel('Principal Part 1')
plt.ylabel('Principal Part 2')
plt.title('PCA of Dataset')
plt.present()
Q40. How do you consider a machine studying mannequin?
Ans. Evaluating a machine studying mannequin entails a number of metrics and strategies to make sure its efficiency. Listed here are some widespread strategies:
Prepare-Take a look at Break up: Divide the dataset right into a coaching set and a check set to guage how effectively the mannequin generalizes to unseen knowledge.
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
Cross-Validation: Use k-fold cross-validation to evaluate the mannequin’s efficiency on totally different subsets of the information.
from sklearn.model_selection import cross_val_score
scores = cross_val_score(mannequin, X, y, cv=5)
Confusion Matrix: For classification issues, a confusion matrix helps visualize the efficiency by displaying true vs. predicted values.
from sklearn.metrics import confusion_matrix
y_pred = mannequin.predict(X_test)
cm = confusion_matrix(y_test, y_pred)
ROC-AUC Curve: For binary classification, the ROC-AUC curve helps consider the mannequin’s means to differentiate between lessons.
from sklearn.metrics import roc_auc_score
auc = roc_auc_score(y_test, y_pred)
Imply Absolute Error (MAE) and Root Imply Squared Error (RMSE): For regression issues, these metrics assist quantify the prediction errors.
from sklearn.metrics import mean_absolute_error, mean_squared_error
mae = mean_absolute_error(y_test, y_pred)
rmse = mean_squared_error(y_test, y_pred, squared=False)
Evaluating a mannequin comprehensively ensures that it performs effectively not simply on coaching knowledge but in addition on new, unseen knowledge, making it strong and dependable.
Try extra machine studying interview questions.
Conclusion
Mastering coding questions in knowledge science is crucial to get the job you need on this ever-changing business. These questions measure not solely your technical abilities but in addition your important pondering and drawback fixing abilities. By means of constant observe and understanding of key ideas, you’ll be able to set up a strong basis that may enable you to in interviews and in your profession journey.
The sphere of knowledge science is aggressive, however with correct preparation, you’ll be able to emerge as a candidate able to deal with real-world points. Improve your abilities, keep abreast of the newest strategies and applied sciences, and consistently increase your information base. Fixing each coding drawback will get you nearer to changing into a reliable and efficient knowledge scientist.
We imagine this assortment of prime knowledge science coding questions and solutions has given you invaluable insights and a structured strategy to getting ready your self. Good luck along with your interview and will you obtain all of your profession aspirations within the thrilling world of knowledge science!
Steadily Requested Questions
A. Key abilities embody proficiency in Python or R, a robust understanding of statistics and chance, expertise with knowledge manipulation utilizing Pandas and NumPy, information of machine studying algorithms, and problem-solving skills. Gentle abilities like communication and teamwork are additionally essential.
A. Apply on coding platforms like LeetCode and HackerRank, deal with knowledge buildings and algorithms, work on real-world initiatives, evaluation others’ code, take part in coding competitions, and take on-line programs.
A. Mix technical and non-technical preparation: research widespread questions, do mock interviews, perceive the corporate, brush up on algorithms and machine studying, and observe explaining your options clearly.
A. Initiatives and portfolios are essential as they display your sensible abilities, creativity, and expertise. A well-documented portfolio with various initiatives can considerably increase your possibilities and function dialogue factors in interviews.
A. Evaluate core ideas and customary questions, observe coding and mock interviews, revisit your initiatives, analysis the corporate, put together questions for the interviewers, make sure you get sufficient relaxation, and handle stress successfully.
[ad_2]