[ad_1]
Introduction
LLMs (giant language fashions) have gotten more and more related in numerous companies and organizations. Their capability to grasp and analyze information and make sense of advanced info can drive innovation, enhance operational effectivity, and ship customized experiences throughout numerous industries. Integrating with numerous instruments permits us to construct LLM purposes that may automate duties, present insights, and assist decision-making processes.
Nevertheless, constructing these purposes may be advanced and time-consuming, requiring a framework to streamline growth and guarantee scalability. A framework supplies standardized instruments and processes, making growing, deploying, and sustaining efficient LLM purposes simpler. So, let’s find out about LangChain, the most well-liked framework for growing LLM purposes.
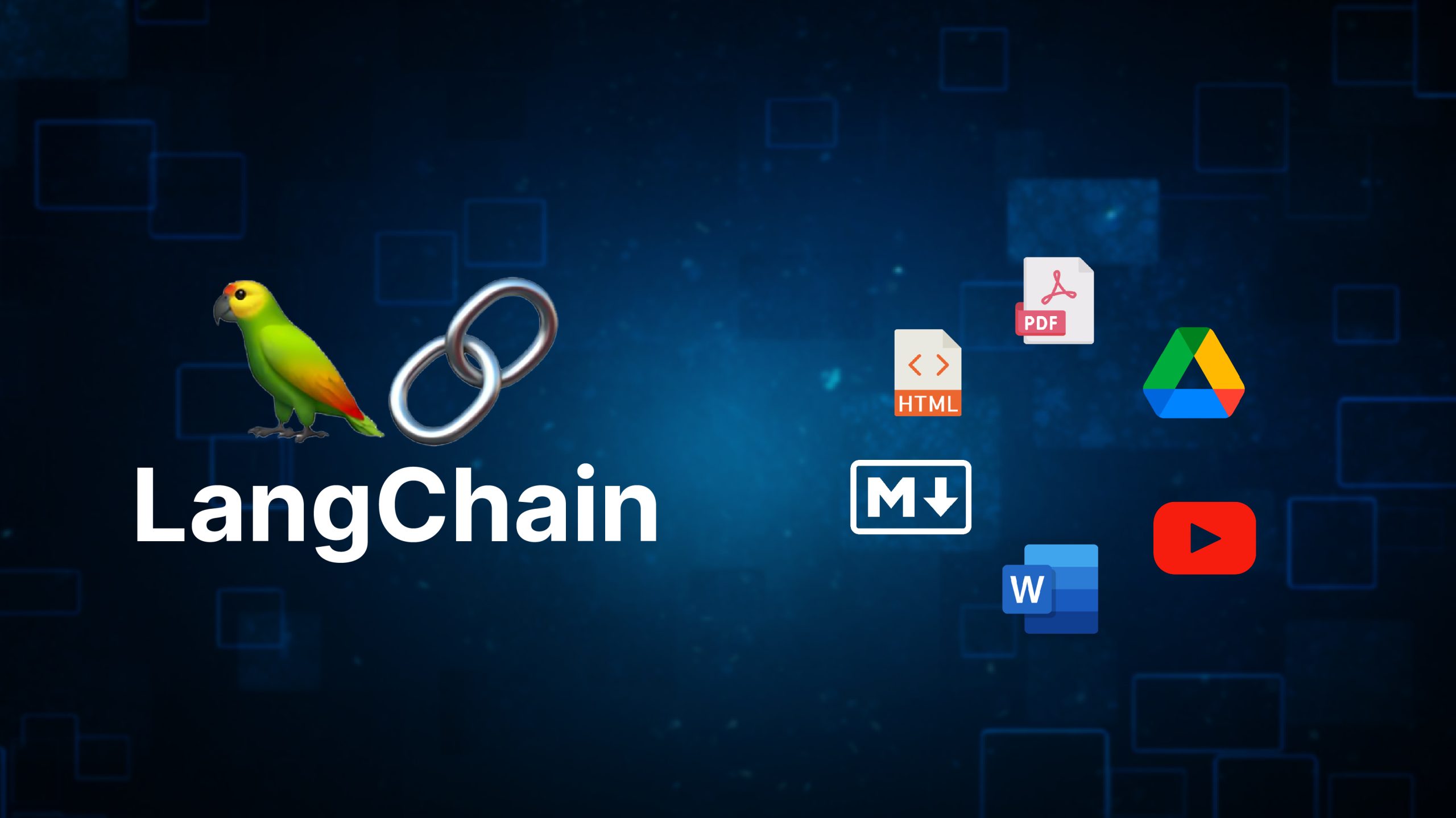
Overview
- LangChain Doc Loaders convert information from numerous codecs (e.g., CSV, PDF, HTML) into standardized Doc objects for LLM purposes.
- They facilitate the seamless integration and processing of various information sources, comparable to YouTube, Wikipedia, and GitHub, into Doc objects.
- Doc loaders in LangChain allow builders to handle and standardize content material for big language mannequin workflows effectively.
- They assist a variety of knowledge codecs and sources, enhancing the flexibility and scalability of LLM-powered purposes.
- LangChain’s doc loaders streamline the conversion of uncooked information into structured codecs, which is important for constructing and sustaining efficient LLM purposes.
LangChain Overview
LangChain has functionalities starting from loading, splitting, embedding, and retrieving the information for the LLM to parsing the output of the LLM. It contains including instruments and agentic capabilities to the LLM and lots of of third-party integrations. LangChain ecosystem additionally contains LangGraph to construct stateful brokers and LangSmith to productionize LLM purposes. We will be taught extra about LangChain right here at Constructing LLM-Powered Purposes with LangChain.
In a collection of articles, we are going to find out about totally different elements of the Langchain. Because it all begins with information, we are going to begin by loading information from numerous file sorts and information sources with doc loaders from Langchain.
What are Doc Loaders?
Doc loaders convert information from various information codecs to standardized Doc objects. The Doc object consists of page_content, which has the information as a string, optionally an ID for the Doc, and metadata that gives info on the information.
Let’s create a doc object to be taught the way it works:
To get began, set up the LangChain framework utilizing ‘pip set up langchain’
from langchain_core.paperwork import Doc
information = Doc(page_content="That is the article about doc loaders of Langchain", id=1, metadata={'supply':'AV'})
information
>>> Doc(id='1', metadata={'supply': 'AV'}, page_content="That is the article about doc loaders of Langchain")
information.page_content
>>> That is the article about doc loaders of Langchain'
information.id = 2 # this adjustments the id of the Doc object
As we are able to see, we are able to create a Doc object with page_content, id, and metadata and entry and modify its contents.
Kinds of Doc Loaders
There are greater than 200 doc loaders in LangChain. They are often categorized as follows
- Primarily based on file sort: These doc loaders parse and cargo the paperwork based mostly on the file sort. Instance file sorts embrace CSV, PDF, HTML, Markdown, and so on.
- Primarily based on information supply: They get the information from totally different information sources and cargo it into Doc objects. Examples of knowledge sources embrace YouTube, Wikipedia, and GitHub.
Information sources may be additional labeled as private and non-private. Public information sources like YouTube or Wikipedia don’t want entry tokens, whereas non-public information sources like AWS or Azure do. Let’s use just a few doc loaders to grasp how they work. Additional we are going to speak concerning the – LangChain Doc Loaders convert information from numerous codecs (e.g., CSV, PDF, HTML) into standardized Doc objects for LLM purposes.
CSV(Comma-separated Values)
CSV information may be loaded with CSVLoader
. It hundreds every row as a Doc.
from langchain_community.document_loaders.csv_loader import CSVLoader
loader = CSVLoader(file_path= "./iris.csv", metadata_columns=['species'], csv_args={"delimiter": ","})
information = loader.load()
len(information)
>>> 150 # for 150 rows
we are able to add any columns to the metadata utilizing metadata_columns. We will additionally add the column to the supply as an alternative of the file identify.
information[0].metadata
>>> {'supply': './iris.csv', 'row': 0, 'species': 'setosa'}
# we are able to change the supply as 'setosa' with the parameter source_column='species'
for file in information[:1]:
print(file)
>>> page_content="sepal_length: 5.1
sepal_width: 3.5
petal_length: 1.4
petal_width: 0.2" metadata={'supply': './iris.csv', 'row': 0, 'species': 'setosa'}
Langchain dataloaders load the doc into Doc objects.
HTML(Hyper Textual content Markup Language)
we are able to load an HTML web page both immediately from a saved HTML web page or a URL
from langchain_community.document_loaders import UnstructuredHTMLLoader
from langchain_community.document_loaders import UnstructuredURLLoader
loader = UnstructuredURLLoader(urls=['https://diataxis.fr'], mode="parts")
information = loader.load()
len(information)
>>> 61
All the HTML web page is loaded as one doc if the mode is single. if the mode is ‘parts, ‘ paperwork are made utilizing the HTML tags.
# accessing metadata and content material in a documnent
information[28].metadata
>>> {'languages': ['eng'], 'parent_id': '312017038db4f2ad1e9332fc5a40bb9d',
'filetype': 'textual content/html', 'url': 'https://diataxis.fr', 'class': 'NarrativeText'}
information[28].page_content
>>> "Diátaxis is a mind-set about and doing documentation"
Markdown
Markdown is a markup language for creating formatted textual content utilizing a easy textual content editor.
from langchain_community.document_loaders import UnstructuredMarkdownLoader
# can obtain from right here https://github.com/dsanr/best-of-ML/blob/important/README.md
loader = UnstructuredMarkdownLoader('README.md', mode="parts")
information = loader.load()
len(information)
>>> 1458
Along with single and parts, this additionally has a ‘paged’ mode, which partitions the file based mostly on the web page numbers.
information[700].metadata
>>> {'supply': 'README.md', 'last_modified': '2024-07-09T12:52:53', 'languages': ['eng'], 'filetype': 'textual content/markdown', 'filename': 'README.md', 'class': 'Title'}
information[700].page_content
>>> 'NeuralProphet (🥈28 · ⭐ 3.7K) - NeuralProphet: A easy forecasting package deal.'
JSON
We will copy the JSON content material from right here – The best way to load JSON?
from langchain_community.document_loaders import JSONLoader
loader = JSONLoader(file_path="chat.json", jq_schema=".", text_content=False)
information = loader.load()
len(information)
>>> 1
In JSONLoader, we have to point out the schema. If jq_schema = ‘.’ all of the content material is loaded. Relying on the content material we want from the json, we are able to change the schema. For instance, jq_schema=’.title’ for title, jq_schema=’.messages[].content material’ to get solely the content material of the messages.
MS Workplace docs
Let’s load an MS Phrase file for instance.
from langchain_community.document_loaders import UnstructuredWordDocumentLoader
loader = UnstructuredWordDocumentLoader(file_path="Polars.docx", mode="parts", chunking_strategy='by_title',
max_characters=200, new_after_n_chars=20)
information = loader.load()
len(information)
>>> 67
As we’ve got seen, Langchain makes use of the Unstructured library to load information in numerous codecs. Because the libraries are steadily up to date, discovering documentation for all of the parameters requires looking by means of the entire supply code. we are able to discover the parameters of this loader below the ‘add_chunking_strategy’ operate in Github.
PDF(Transportable Doc Format)
A number of PDF parser integrations can be found in Langchain. We will examine numerous parsers and select an appropriate one. Right here is the Benchmark.
A few of the out there parsers are PyMuPDF, PyPDF, PDFPlumber, and so on.
Let’s attempt with UnstructuredPDFLoader
from langchain_community.document_loaders import UnstructuredPDFLoader
loader = UnstructuredPDFLoader('how-to-formulate-successful-business-strategy.pdf', mode="parts", technique="auto")
information = loader.load()
len(information)
>>> 177
Right here is the code rationalization:
- The ‘technique’ parameter defines the way to course of the pdf.
- The ‘hi_res’ technique makes use of the Detectron2 mannequin to determine the doc’s structure.
- The ‘ocr_only’ technique makes use of Tesseract to extract the textual content even from the photographs.
- The ‘quick’ technique makes use of pdfminer to extract the textual content.
- ‘The default ‘auto’ technique makes use of any of the above methods based mostly on the paperwork and parameter arguments.
A number of Information
If we wish to load a number of information from a listing, we are able to use the next
from langchain_community.document_loaders import DirectoryLoader
loader = DirectoryLoader(".", glob="**/*.json", loader_cls=JSONLoader, loader_kwargs={'jq_schema': '.', 'text_content':False},
show_progress=True, use_multithreading=True)
docs = loader.load()
len(docs)
>>> 1
As we are able to see, we are able to point out which loader to make use of utilizing the loader_cls parameter and the loader’s arguments utilizing the loader_kwargs parameter.
YouTube
In order for you the abstract of a YouTube video or wish to search by means of its transcript, that is the loader you want. Be sure to use the video_id not your entire URL, as proven beneath
from langchain_community.document_loaders import YoutubeLoader
video_url="https://www.youtube.com/watch?v=LKCVKw9CzFo"
loader = YoutubeLoader(video_id='LKCVKw9CzFo', add_video_info=True)
information = loader.load()
len(information)
>>> 1
We will get the transcript utilizing information[0].page_content and video info utilizing information[0].metadata
Wikipedia
We get the Wikipedia article content material based mostly on a search question. The code beneath extracts the highest 5 articles based mostly on Wikipedia search outcomes. Be sure to set up the Wikipedia package deal with ‘pip set up Wikipedia’
from langchain_community.document_loaders import WikipediaLoader
loader = WikipediaLoader(question='Generative AI', load_max_docs=5, doc_content_chars_max=5000, load_all_available_meta=True)
information = loader.load()
len(information)
>>> 5
We will management article content material size with doc_content_chars_max. We will additionally get all of the details about the article.
information[0].metadata.keys()
>>> dict_keys(['title', 'summary', 'source', 'categories', 'page_url', 'image_urls', 'related_titles', 'parent_id', 'references', 'revision_id', 'sections'])
for i in information:
print(i.metadata['title'])
>>>Generative synthetic intelligence
AI increase
Generative pre-trained transformer
ChatGPT
Synthetic intelligence
Conclusion
LangChain provides a complete and versatile framework for loading information from numerous sources, making it a useful device for growing purposes powered by Massive Language Fashions (LLMs). By integrating a number of file sorts and information sources, comparable to CSV information, MS Workplace paperwork, PDF information, YouTube movies, and Wikipedia articles, LangChain permits builders to assemble and standardize various information into Doc objects, facilitating seamless information processing and evaluation.
Within the subsequent article, we are going to be taught why we have to break up the paperwork and the way to do it. Keep tuned to Analytics Vidhya Blogs for the following replace!
Continuously Requested Questions
Ans. LangChain provides a variety of functionalities, together with loading, splitting, embedding, and retrieving information. It additionally helps parsing LLM outputs, including instruments and agentic capabilities to LLMs, and integrating with lots of of third-party companies. Moreover, it contains elements like LangGraph for constructing stateful brokers and LangSmith for productionizing LLM purposes.
Ans. LangChain provides a variety of functionalities, together with loading, splitting, embedding, and retrieving information. It additionally helps parsing LLM outputs, including instruments and agentic capabilities to LLMs, and integrating with lots of of third-party companies. Moreover, it contains elements like LangGraph for constructing stateful brokers and LangSmith for productionizing LLM purposes.
Ans. Doc loaders in LangChain are instruments that convert information from numerous codecs (e.g., CSV, PDF, HTML) into standardized Doc objects. These objects embrace the information’s content material, an optionally available ID, and metadata. Doc loaders facilitate the seamless integration and processing of knowledge from various sources into LLM purposes.
Ans. LangChain helps over 200 doc loaders categorized by file sort (e.g., CSV, PDF, HTML) and information supply (e.g., YouTube, Wikipedia, GitHub). Public information sources like YouTube and Wikipedia may be accessed with out tokens, whereas non-public information sources like AWS or Azure require entry tokens. Every loader is designed to parse and cargo information appropriately based mostly on the particular format or supply.
[ad_2]