[ad_1]
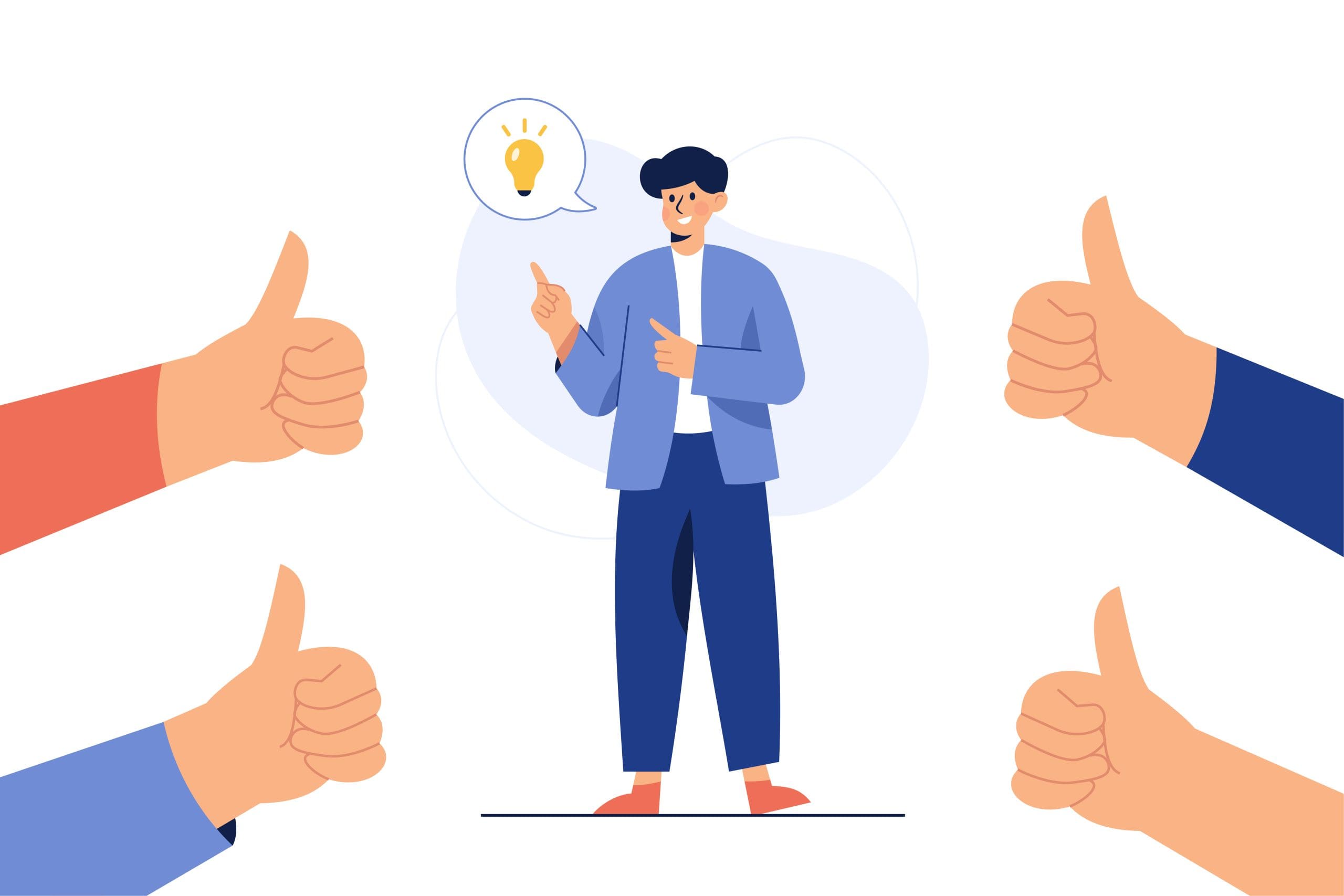
Picture by jcomp on Freepik
We’ve relied on software program in our telephones and computer systems within the trendy period. Many functions, reminiscent of e-commerce, film streaming, recreation platforms, and others, have modified how we dwell, as these functions make issues simpler. To make issues even higher, the enterprise typically gives options that permit suggestions from the information.
Our Prime 5 Free Course Suggestions
1. Google Cybersecurity Certificates – Get on the quick monitor to a profession in cybersecurity.
2. Pure Language Processing in TensorFlow – Construct NLP programs
3. Python for All people – Develop applications to assemble, clear, analyze, and visualize knowledge
4. Google IT Assist Skilled Certificates
5. AWS Cloud Options Architect – Skilled Certificates
The premise of advice programs is to foretell what the consumer may thinking about based mostly on the enter. The system would offer the closest gadgets based mostly on both the similarity between the gadgets (content-based filtering) or the habits (collaborative filtering).
With many approaches to the advice system structure, we will use the Hugging Face Transformers package deal. In the event you didn’t know, Hugging Face Transformers is an open-source Python package deal that enables APIs to simply entry all of the pre-trained NLP fashions that assist duties reminiscent of textual content processing, technology, and lots of others.
This text will use the Hugging Face Transformers package deal to develop a easy advice system based mostly on embedding similarity. Let’s get began.
Develop a Suggestion System with Hugging Face Transformers
Earlier than we begin the tutorial, we have to set up the required packages. To try this, you need to use the next code:
pip set up transformers torch pandas scikit-learn
You possibly can choose the acceptable model on your atmosphere by way of their web site for the Torch set up.
As for the dataset instance, we might use the Anime advice dataset instance from Kaggle.
As soon as the atmosphere and the dataset are prepared, we’ll begin the tutorial. First, we have to learn the dataset and put together them.
import pandas as pd
df = pd.read_csv('anime.csv')
df = df.dropna()
df['description'] = df['name'] +' '+ df['genre'] + ' ' +df['type']+' episodes: '+ df['episodes']
Within the code above, we learn the dataset with Pandas and dropped all of the lacking knowledge. Then, we create a function known as “description” that comprises all the knowledge from the accessible knowledge, reminiscent of title, style, kind, and episode quantity. The brand new column would turn into our foundation for the advice system. It could be higher to have extra full info, such because the anime plot and abstract, however let’s be content material with this one for now.
Subsequent, we might use Hugging Face Transformers to load an embedding mannequin and remodel the textual content right into a numerical vector. Particularly, we might use sentence embedding to remodel the entire sentence.
The advice system can be based mostly on the embedding from all of the anime “description” we’ll carry out quickly. We’d use the cosine similarity methodology, which measures the similarity of two vectors. By measuring the similarity between the anime “description” embedding and the consumer’s question enter embedding, we will get exact gadgets to suggest.
The embedding similarity strategy sounds easy, however it may be highly effective in comparison with the basic advice system mannequin, as it may well seize the semantic relationship between phrases and supply contextual that means for the advice course of.
We’d use the embedding mannequin sentence transformers from the Hugging Face for this tutorial. To remodel the sentence into embedding, we might use the next code.
from transformers import AutoTokenizer, AutoModel
import torch
import torch.nn.practical as F
def mean_pooling(model_output, attention_mask):
token_embeddings = model_output[0] #First factor of model_output comprises all token embeddings
input_mask_expanded = attention_mask.unsqueeze(-1).broaden(token_embeddings.dimension()).float()
return torch.sum(token_embeddings * input_mask_expanded, 1) / torch.clamp(input_mask_expanded.sum(1), min=1e-9)
tokenizer = AutoTokenizer.from_pretrained('sentence-transformers/all-MiniLM-L6-v2')
mannequin = AutoModel.from_pretrained('sentence-transformers/all-MiniLM-L6-v2')
def get_embeddings(sentences):
encoded_input = tokenizer(sentences, padding=True, truncation=True, return_tensors="pt")
with torch.no_grad():
model_output = mannequin(**encoded_input)
sentence_embeddings = mean_pooling(model_output, encoded_input['attention_mask'])
sentence_embeddings = F.normalize(sentence_embeddings, p=2, dim=1)
return sentence_embeddings
Strive the embedding course of and see the vector end result with the next code. Nonetheless, I’d not present the output because it’s fairly lengthy.
sentences = ['Some great movie', 'Another funny movie']
end result = get_embeddings(sentences)
print("Sentence embeddings:")
print(end result)
To make issues simpler, Hugging Face maintains a Python package deal for embedding sentence transformers, which might decrease the entire transformation course of in 3 traces of code. Set up the mandatory package deal utilizing the code under.
pip set up -U sentence-transformers
Then, we will remodel the entire anime “description” with the next code.
from sentence_transformers import SentenceTransformer
mannequin = SentenceTransformer('sentence-transformers/all-MiniLM-L6-v2')
anime_embeddings = mannequin.encode(df['description'].tolist())
With the embedding database is prepared, we might create a perform to take consumer enter and carry out cosine similarity as a advice system.
from sklearn.metrics.pairwise import cosine_similarity
def get_recommendations(question, embeddings, df, top_n=5):
query_embedding = mannequin.encode([query])
similarities = cosine_similarity(query_embedding, embeddings)
top_indices = similarities[0].argsort()[-top_n:][::-1]
return df.iloc[top_indices]
Now that every part is prepared, we will strive the advice system. Right here is an instance of buying the highest 5 anime suggestions from the consumer enter question.
question = "Humorous anime I can watch with mates"
suggestions = get_recommendations(question, anime_embeddings, df)
print(suggestions[['name', 'genre']])
Output>>
title
7363 Sentou Yousei Shoujo Tasukete! Mave-chan
8140 Anime TV de Hakken! Tamagotchi
4294 SKET Dance: SD Character Flash Anime
1061 Isshuukan Associates.
2850 Oshiete! Galko-chan
style
7363 Comedy, Parody, Sci-Fi, Shounen, Tremendous Energy
8140 Comedy, Fantasy, Youngsters, Slice of Life
4294 Comedy, Faculty, Shounen
1061 Comedy, Faculty, Shounen, Slice of Life
2850 Comedy, Faculty, Slice of Life
The result’s all the comedy anime, as we wish the humorous anime. Most of them additionally embody anime, which is appropriate to observe with mates from the style. After all, the advice can be even higher if we had extra detailed info.
Conclusion
A Suggestion System is a device for predicting what customers is perhaps thinking about based mostly on the enter. Utilizing Hugging Face Transformers, we will construct a advice system that makes use of the embedding and cosine similarity strategy. The embedding strategy is highly effective as it may well account for the textual content’s semantic relationship and contextual that means.
Cornellius Yudha Wijaya is a knowledge science assistant supervisor and knowledge author. Whereas working full-time at Allianz Indonesia, he likes to share Python and knowledge ideas by way of social media and writing media. Cornellius writes on quite a lot of AI and machine studying subjects.
[ad_2]