[ad_1]


Picture by Creator
SQLite is a light-weight, serverless relational database administration system (RDBMS) that’s extensively used as a result of its simplicity and ease of embedding inside functions.
So whether or not you are constructing a small software, managing information regionally, or prototyping a undertaking, SQLite offers a handy resolution for storing and querying structured information. On this tutorial, you’ll discover ways to work with SQLite databases from Python utilizing the built-in sqlite3 module.
Notably, you’ll discover ways to connect with an SQLite database from Python and carry out primary CRUD operations. Let’s get began.
Setting Up the Setting
As a primary step create a devoted digital atmosphere in your undertaking (within the undertaking listing) and activate it. You are able to do it utilizing the built-in venv module like so:
$ python3 -m venv v1
$ supply v1/bin/activate
On this tutorial, we’ll use Faker to generate artificial data. So set up it utilizing pip:
The sqlite3 module is constructed into the Python customary library, so you do not have to put in it. So in case you’ve put in Faker and are utilizing a latest model of Python, you’re good to go!
Connecting to an SQLite Database
Within the undertaking listing, create a Python script and get began. As a primary step to work together with the database, we should always set up a reference to the database.
To hook up with a pattern database instance.db, you need to use the join()
perform from the sqlite3 module like so:
conn = sqlite3.join(‘instance.db’)
If the database already exists, then it connects to it. Else it creates the database within the working listing.
After connecting to the database, we’ll create a database cursor that may assist us run queries. The cursor object has strategies to execute queries and fetch the outcomes of the question. It really works very equally to a file handler.


Database Cursor | Picture by Creator
It’s usually useful to make use of the connection as a context supervisor in a with assertion like so:
import sqlite3
# Hook up with the db
with sqlite3.join('instance.db') as conn:
# create db cursor
# run queries
# commit modifications
This fashion you don’t have to fret about closing the connection object. The connection is mechanically closed when the execution exits the with block. We’ll explicitly shut the cursor objects on this tutorial, although.
Creating Database Tables
Now let’s create a clients
desk with the required fields within the database. To take action, we first create a cursor object. We then run a CREATE TABLE assertion, and go within the question string to the execute()
methodology known as on the cursor object:
import sqlite3
# Hook up with the db
with sqlite3.join('instance.db') as conn:
cursor = conn.cursor()
# Create clients desk
cursor.execute('''
CREATE TABLE IF NOT EXISTS clients (
id INTEGER PRIMARY KEY,
first_name TEXT NOT NULL,
last_name TEXT NOT NULL,
e mail TEXT UNIQUE NOT NULL,
telephone TEXT,
num_orders INTEGER
);
''')
conn.commit()
print("Prospects desk created efficiently.")
cursor.shut()
If you run the script, you need to see the next output:
Output >>>
Prospects desk created efficiently.
Performing CRUD Operations
Let’s carry out some primary CRUD operations on the database desk. Should you’d like chances are you’ll create separate scripts for every operation.
Inserting Data
Now we’ll insert some data into the clients
desk. We’ll use Faker to generate artificial data. To maintain the outputs readable, I’ve inserted solely 10 data. However chances are you’ll insert as many data as you’d like.
import sqlite3
import random
from faker import Faker
# Initialize Faker object
faux = Faker()
Faker.seed(24)
# Hook up with the db
with sqlite3.join('instance.db') as conn:
cursor = conn.cursor()
# Insert buyer data
num_records = 10
for _ in vary(num_records):
first_name = faux.first_name()
last_name = faux.last_name()
e mail = faux.e mail()
telephone = faux.phone_number()
num_orders = random.randint(0,100)
cursor.execute('''
INSERT INTO clients (first_name, last_name, e mail, telephone, num_orders)
VALUES (?, ?, ?, ?, ?)
''', (first_name, last_name, e mail, telephone, num_orders))
print(f"{num_records} buyer data inserted efficiently.")
conn.commit()
cursor.shut()
Discover how we use parameterized queries: as an alternative of hardcoding the values into the INSERT assertion, we use ? placeholders and go in a tuple of values.
Operating the script ought to give:
Output >>>
10 buyer data inserted efficiently.
Studying and Updating Data
Now that we’ve inserted data into the desk, let’s run a question to learn in all of the data. Discover how we use the execute()
methodology to run queries and the fetchall()
methodology on the cursor to retrieve the outcomes of the question.
As a result of we’ve saved the outcomes of the earlier question in `all_customers`, let’s additionally run an UPDATE question to replace the num_orders
akin to the id 1. Right here’s the code snippet:
import sqlite3
# Hook up with the db
with sqlite3.join('instance.db') as conn:
cursor = conn.cursor()
# Fetch and show all clients
cursor.execute('SELECT id, first_name, last_name, e mail, num_orders FROM clients')
all_customers = cursor.fetchall()
print("All Prospects:")
for buyer in all_customers:
print(buyer)
# Replace num_orders for a selected buyer
if all_customers:
customer_id = all_customers[0][0] # Take the ID of the primary buyer
new_num_orders = all_customers[0][4] + 1 # Increment num_orders by 1
cursor.execute('''
UPDATE clients
SET num_orders = ?
WHERE id = ?
''', (new_num_orders, customer_id))
print(f"Orders up to date for buyer ID {customer_id}: now has {new_num_orders} orders.")
conn.commit()
cursor.shut()
This outputs each the data and the message after the replace question:
Output >>>
All Prospects:
(1, 'Jennifer', 'Franco', '[email protected]', 54)
(2, 'Grace', 'King', '[email protected]', 43)
(3, 'Lori', 'Braun', '[email protected]', 99)
(4, 'Wendy', 'Hubbard', '[email protected]', 11)
(5, 'Morgan', 'Wright', '[email protected]', 4)
(6, 'Juan', 'Watson', '[email protected]', 51)
(7, 'Randy', 'Smith', '[email protected]', 32)
(8, 'Jimmy', 'Johnson', '[email protected]', 64)
(9, 'Gina', 'Ellison', '[email protected]', 85)
(10, 'Cory', 'Joyce', '[email protected]', 41)
Orders up to date for buyer ID 1: now has 55 orders.
Deleting Data
To delete a buyer with a selected buyer ID, let’s run a DELETE assertion as proven:
import sqlite3
# Specify the client ID of the client to delete
cid_to_delete = 3
with sqlite3.join('instance.db') as conn:
cursor = conn.cursor()
# Execute DELETE assertion to take away the client with the desired ID
cursor.execute('''
DELETE FROM clients
WHERE id = ?
''', (cid_to_delete,))
conn.commit()
f"Buyer with ID {cid_to_delete} deleted efficiently.")
cursor.shut()
This outputs:
Buyer with ID 3 deleted efficiently.
Filtering Data Utilizing the WHERE Clause
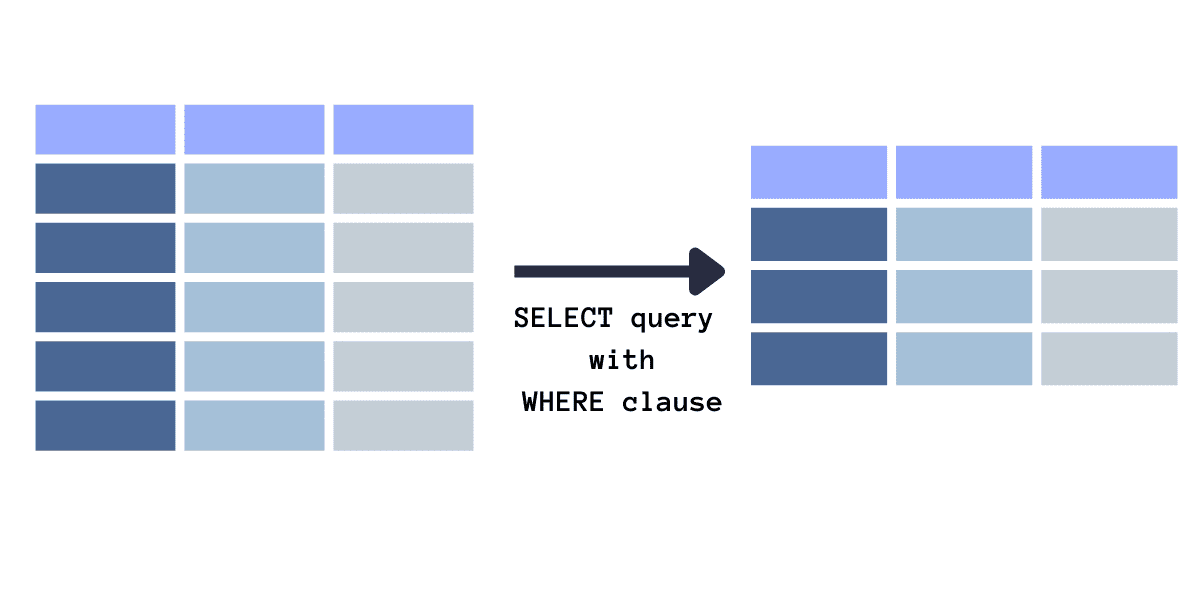
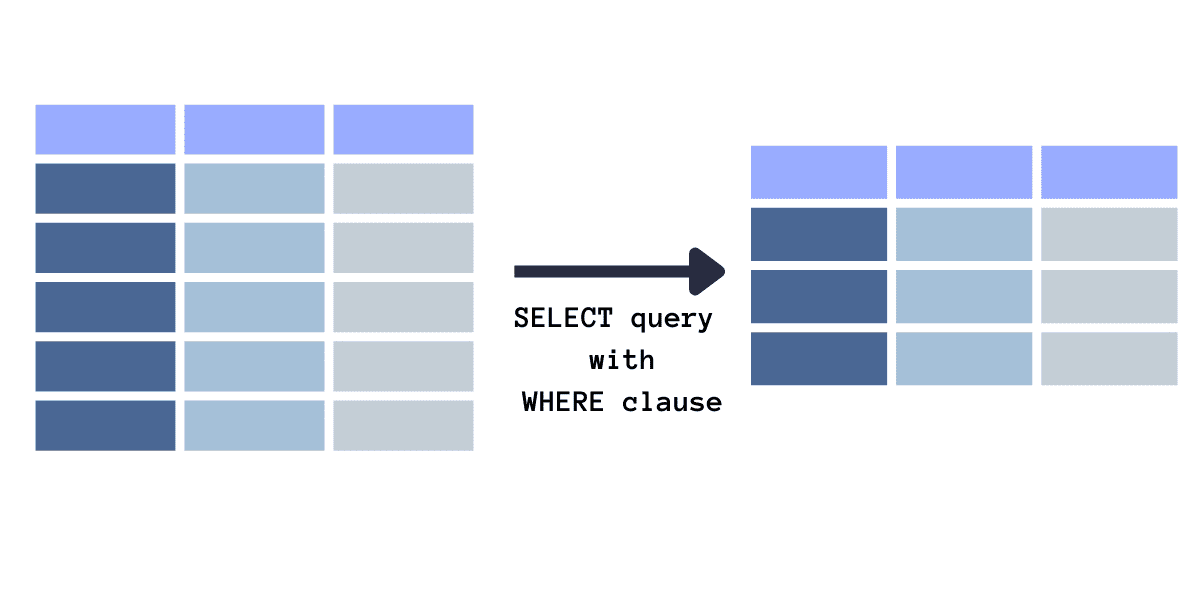
Picture by Creator
Let’s say we wish to fetch data of consumers who’ve made fewer than 10 orders, say to run focused campaigns and the like. For this, we run a SELECT question with the WHERE clause specifying the situation for filtering (on this case the variety of orders). Here is how one can obtain this:
import sqlite3
# Outline the edge for the variety of orders
order_threshold = 10
with sqlite3.join('instance.db') as conn:
cursor = conn.cursor()
# Fetch clients with lower than 10 orders
cursor.execute('''
SELECT id, first_name, last_name, e mail, num_orders
FROM clients
WHERE num_orders < ?
''', (order_threshold,))
# Fetch all matching clients
filtered_customers = cursor.fetchall()
# Show filtered clients
if filtered_customers:
print("Prospects with lower than 10 orders:")
for buyer in filtered_customers:
print(buyer)
else:
print("No clients discovered with lower than 10 orders.")
And right here’s the output:
Output >>>
Prospects with lower than 10 orders:
(5, 'Morgan', 'Wright', '[email protected]', 4)
Wrapping Up
And that’s a wrap! This was a information to getting began with SQLite with Python. I hope you discovered it useful. You’ll find all of the code on GitHub. Within the subsequent half, we’ll have a look at working joins and subqueries, managing transactions in SQLite, and extra. Till then, glad coding!
Should you’re keen on studying how database indexes work, learn How To Velocity Up SQL Queries Utilizing Indexes [Python Edition].
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, information science, and content material creation. Her areas of curiosity and experience embody DevOps, information science, and pure language processing. She enjoys studying, writing, coding, and low! At present, she’s engaged on studying and sharing her information with the developer neighborhood by authoring tutorials, how-to guides, opinion items, and extra. Bala additionally creates partaking useful resource overviews and coding tutorials.
[ad_2]