[ad_1]
Massive-scale knowledge evaluation has change into a transformative device for many industries, with functions that embrace fraud detection for the banking business, scientific analysis for healthcare, and predictive upkeep and high quality management for manufacturing. Nonetheless, processing such huge quantities of knowledge could be a problem, even with the ability of recent computing {hardware}. Many instruments are actually out there to handle the problem, with some of the common being Apache Spark, an open supply analytics engine designed to hurry up the processing of very giant knowledge units.
Spark gives a strong structure able to dealing with immense quantities of knowledge. There are a number of Spark optimization strategies that streamline processes and knowledge dealing with, together with performing duties in reminiscence and storing ceaselessly accessed knowledge in a cache, thus lowering latency throughout retrieval. Spark can also be designed for scalability; knowledge processing could be distributed throughout a number of computer systems, growing the out there computing energy. Spark is related to many tasks: It helps a wide range of programming languages (e.g., Java, Scala, R, and Python) and consists of varied libraries (e.g., MLlib for machine studying, GraphX for working with graphs, and Spark Streaming for processing streaming knowledge).
Whereas Spark’s default settings present start line, there are a number of changes that may improve its efficiency—thus permitting many companies to make use of it to its full potential. There are two areas to think about when excited about optimization strategies in Spark: computation effectivity and optimizing the communication between nodes.
How Does Spark Work?
Earlier than discussing optimization strategies intimately, it’s useful to have a look at how Spark handles knowledge. The basic knowledge construction in Spark is the resilient distributed knowledge set, or RDD. Understanding how RDDs work is vital when contemplating easy methods to use Apache Spark. An RDD represents a fault-tolerant, distributed assortment of knowledge able to being processed in parallel throughout a cluster of computer systems. RDDs are immutable; their contents can’t be modified as soon as they’re created.
Spark’s quick processing speeds are enabled by RDDs. Whereas many frameworks depend on exterior storage methods corresponding to a Hadoop Distributed File System (HDFS) for reusing and sharing knowledge between computations, RDDs assist in-memory computation. Performing processing and knowledge sharing in reminiscence avoids the substantial overhead brought on by replication, serialization, and disk learn/write operations, to not point out community latency, when utilizing an exterior storage system. Spark is usually seen as a successor to MapReduce, the information processing part of Hadoop, an earlier framework from Apache. Whereas the 2 methods share comparable performance, Spark’s in-memory processing permits it to run as much as 100 occasions quicker than MapReduce, which processes knowledge on disk.
To work with the information in an RDD, Spark gives a wealthy set of transformations and actions. Transformations produce new RDDs from the information in present ones utilizing operations corresponding to filter()
, be a part of()
, or map()
. The filter()
perform creates a brand new RDD with parts that fulfill a given situation, whereas be a part of()
creates a brand new RDD by combining two present RDDs based mostly on a standard key. map()
is used to use a change to every aspect in an information set, for instance, making use of a mathematical operation corresponding to calculating a proportion to each document in an RDD, outputting the leads to a brand new RDD. An motion, however, doesn’t create a brand new RDD, however returns the results of a computation on the information set. Actions embrace operations corresponding to depend()
, first()
, or accumulate()
. The depend()
motion returns the variety of parts in an RDD, whereas first()
returns simply the primary aspect. accumulate()
merely retrieves the entire parts in an RDD.
Transformations additional differ from actions in that they’re lazy. The execution of transformations shouldn’t be speedy. As a substitute, Spark retains observe of the transformations that should be utilized to the bottom RDD, and the precise computation is triggered solely when an motion is known as.
Understanding RDDs and the way they work can present precious perception into Spark tuning and optimization; nevertheless, though an RDD is the muse of Spark’s performance, it won’t be essentially the most environment friendly knowledge construction for a lot of functions.
Selecting the Proper Information Buildings
Whereas an RDD is the fundamental knowledge construction of Spark, it’s a lower-level API that requires a extra verbose syntax and lacks the optimizations offered by higher-level knowledge constructions. Spark shifted towards a extra user-friendly and optimized API with the introduction of DataFrames—higher-level abstractions constructed on prime of RDDs. The information in a DataFrame is organized into named columns, structuring it extra like the information in a relational database. DataFrame operations additionally profit from Catalyst, Spark SQL’s optimized execution engine, which may enhance computational effectivity, doubtlessly bettering efficiency. Transformations and actions could be run on DataFrames the best way they’re in RDDs.
Due to their higher-level API and optimizations, DataFrames are usually simpler to make use of and provide higher efficiency; nevertheless, as a consequence of their lower-level nature, RDDs can nonetheless be helpful for outlining customized operations, in addition to debugging advanced knowledge processing duties. RDDs provide extra granular management over partitioning and reminiscence utilization. When coping with uncooked, unstructured knowledge, corresponding to textual content streams, binary recordsdata, or customized codecs, RDDs could be extra versatile, permitting for customized parsing and manipulation within the absence of a predefined construction.
Following Caching Finest Practices
Caching is a vital approach that may result in vital enhancements in computational effectivity. Ceaselessly accessed knowledge and intermediate computations could be cached, or persevered, in a reminiscence location that enables for quicker retrieval. Spark gives built-in caching performance, which could be significantly useful for machine studying algorithms, graph processing, and every other software during which the identical knowledge have to be accessed repeatedly. With out caching, Spark would recompute an RDD or DataFrame and all of its dependencies each time an motion was referred to as.
The next Python code block makes use of PySpark, Spark’s Python API, to cache a DataFrame named df
:
df.cache()
You will need to remember that caching requires cautious planning, as a result of it makes use of the reminiscence assets of Spark’s employee nodes, which carry out such duties as executing computations and storing knowledge. If the information set is considerably bigger than the out there reminiscence, otherwise you’re caching RDDs or DataFrames with out reusing them in subsequent steps, the potential overflow and different reminiscence administration points might introduce bottlenecks in efficiency.
Optimizing Spark’s Information Partitioning
Spark’s structure is constructed round partitioning, the division of huge quantities of knowledge into smaller, extra manageable items referred to as partitions. Partitioning permits Spark to course of giant quantities of knowledge in parallel by distributing computation throughout a number of nodes, every dealing with a subset of the entire knowledge.
Whereas Spark gives a default partitioning technique usually based mostly on the variety of out there CPU cores, it additionally gives choices for customized partitioning. Customers may as an alternative specify a customized partitioning perform, corresponding to dividing knowledge on a sure key.
Variety of Partitions
Some of the essential components affecting the effectivity of parallel processing is the variety of partitions. If there aren’t sufficient partitions, the out there reminiscence and assets could also be underutilized. However, too many partitions can result in elevated efficiency overhead as a consequence of activity scheduling and coordination. The optimum variety of partitions is normally set as an element of the entire variety of cores out there within the cluster.
Partitions could be set utilizing repartition()
and coalesce()
. On this instance, the DataFrame is repartitioned into 200 partitions:
df = df.repartition(200) # repartition methodology
df = df.coalesce(200) # coalesce methodology
The repartition()
methodology will increase or decreases the variety of partitions in an RDD or DataFrame and performs a full shuffle of the information throughout the cluster, which could be pricey when it comes to processing and community latency. The coalesce()
methodology decreases the variety of partitions in an RDD or DataFrame and, not like repartition()
, doesn’t carry out a full shuffle, as an alternative combining adjoining partitions to cut back the general quantity.
Dealing With Skewed Information
In some conditions, sure partitions could comprise considerably extra knowledge than others, resulting in a situation generally known as skewed knowledge. Skewed knowledge may cause inefficiencies in parallel processing as a consequence of an uneven workload distribution among the many employee nodes. To handle skewed knowledge in Spark, intelligent strategies corresponding to splitting or salting can be utilized.
Splitting
In some circumstances, skewed partitions could be separated into a number of partitions. If a numerical vary causes the information to be skewed, the vary can typically be cut up up into smaller sub-ranges. For instance, if numerous college students scored between 65% to 75% on an examination, the check scores could be divided into a number of sub-ranges, corresponding to 65% to 68%, 69% to 71%, and 72% to 75%.
If a selected key worth is inflicting the skew, the DataFrame could be divided based mostly on that key. Within the instance code under, a skew within the knowledge is brought on by numerous data which have an id
worth of “12345.” The filter()
transformation is used twice: as soon as to pick out all data with an id
worth of “12345,” and as soon as to pick out all data the place the id
worth shouldn’t be “12345.” The data are positioned into two new DataFrames: df_skew
, which incorporates solely the rows which have an id
worth of “12345,” and df_non_skew
, which incorporates the entire different rows. Information processing could be carried out on df_skew
and df_non_skew
individually, after which the ensuing knowledge could be mixed:
from pyspark.sql.features import rand
# Cut up the DataFrame into two DataFrames based mostly on the skewed key.
df_skew = df.filter(df['id'] == 12345) # incorporates all rows the place id = 12345
df_non_skew = df.filter(df['id'] != 12345) # incorporates all different rows
# Repartition the skewed DataFrame into extra partitions.
df_skew = df_skew.repartition(10)
# Now operations could be carried out on each DataFrames individually.
df_result_skew = df_skew.groupBy('id').depend() # simply an instance operation
df_result_non_skew = df_non_skew.groupBy('id').depend()
# Mix the outcomes of the operations collectively utilizing union().
df_result = df_result_skew.union(df_result_non_skew)
Salting
One other methodology of distributing knowledge extra evenly throughout partitions is so as to add a “salt” to the important thing or keys which can be inflicting the skew. The salt worth, usually a random quantity, is appended to the unique key, and the salted secret’s used for partitioning. This forces a extra even distribution of knowledge.
For example this idea, let’s think about our knowledge is cut up into partitions for 3 cities within the US state of Illinois: Chicago has many extra residents than the close by cities of Oak Park or Lengthy Grove, inflicting the information to be skewed.
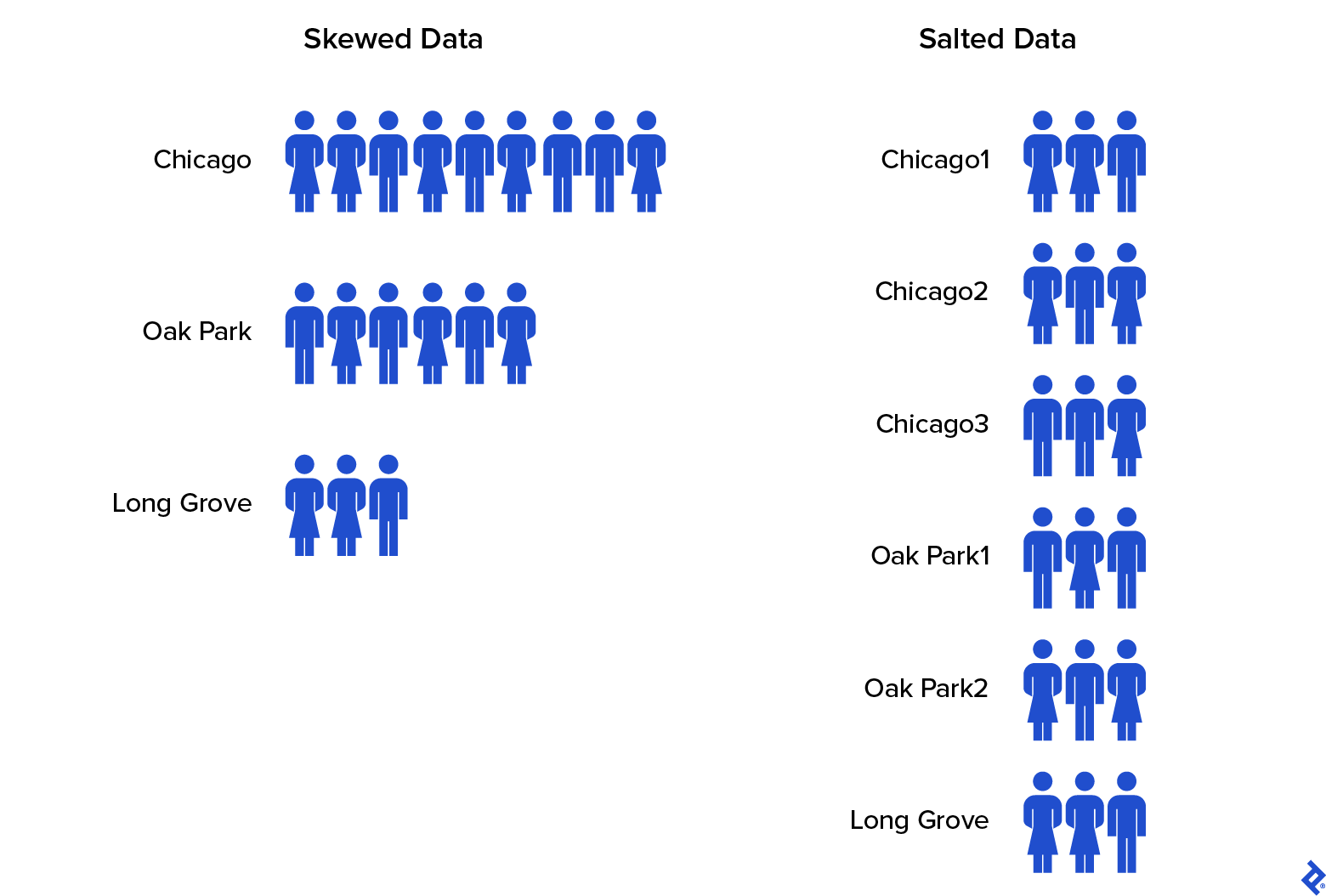
To distribute the information extra evenly, utilizing PySpark, we mix the column metropolis
with a randomly generated integer to create a brand new key, referred to as salted_city
. “Chicago” turns into “Chicago1,” “Chicago2,” and “Chicago3,” with the brand new keys every representing a smaller variety of data. The brand new keys can be utilized with actions or transformations corresponding to groupby()
or depend()
:
# On this instance, the DataFrame 'df' has a skewed column 'metropolis'.
skewed_column = 'metropolis'
# Create a brand new column 'salted_city'.
# 'salted_id' consists of the unique 'id' with a random integer between 0-10 added behind it
df = df.withColumn('salted_city', (df[skewed_column].solid("string") + (rand()*10).solid("int").solid("string")))
# Now operations could be carried out on 'salted_city' as an alternative of 'metropolis'.
# Let’s say we're doing a groupBy operation.
df_grouped = df.groupby('salted_city').depend()
# After the transformation, the salt could be eliminated.
df_grouped = df_grouped.withColumn('original_city', df_grouped['salted_city'].substr(0, len(df_grouped['salted_city'])-1))
Broadcasting
A be a part of()
is a standard operation during which two knowledge units are mixed based mostly on a number of frequent keys. Rows from two totally different knowledge units could be merged right into a single knowledge set by matching values within the specified columns. As a result of knowledge shuffling throughout a number of nodes is required, a be a part of()
could be a pricey operation when it comes to community latency.
In situations during which a small knowledge set is being joined with a bigger knowledge set, Spark gives an optimization approach referred to as broadcasting. If one of many knowledge units is sufficiently small to suit into the reminiscence of every employee node, it may be despatched to all nodes, lowering the necessity for pricey shuffle operations. The be a part of()
operation merely occurs regionally on every node.
Within the following instance, the small DataFrame df2
is broadcast throughout the entire employee nodes, and the be a part of()
operation with the massive DataFrame df1
is carried out regionally on every node:
from pyspark.sql.features import broadcast
df1.be a part of(broadcast(df2), 'id')
df2
have to be sufficiently small to suit into the reminiscence of every employee node; a DataFrame that’s too giant will trigger out-of-memory errors.
Filtering Unused Information
When working with high-dimensional knowledge, minimizing computational overhead is crucial. Any rows or columns that aren’t completely required needs to be eliminated. Two key strategies that scale back computational complexity and reminiscence utilization are early filtering and column pruning:
Early filtering: Filtering operations needs to be utilized as early as attainable within the knowledge processing pipeline. This cuts down on the variety of rows that should be processed in subsequent transformations, lowering the general computational load and reminiscence assets.
Column pruning: Many computations contain solely a subset of columns in an information set. Columns that aren’t needed for knowledge processing needs to be eliminated. Column pruning can considerably lower the quantity of knowledge that must be processed and saved.
The next code reveals an instance of the choose()
operation used to prune columns. Solely the columns identify
and age
are loaded into reminiscence. The code additionally demonstrates easy methods to use the filter()
operation to solely embrace rows during which the worth of age
is larger than 21:
df = df.choose('identify', 'age').filter(df['age'] > 21)
Minimizing Utilization of Python Person-defined Capabilities
Python user-defined features (UDFs) are customized features written in Python that may be utilized to RDDs or DataFrames. With UDFs, customers can outline their very own customized logic or computations; nevertheless, there are efficiency concerns. Every time a Python UDF is invoked, knowledge must be serialized after which deserialized between the Spark JVM and the Python interpreter, which results in further overhead as a consequence of knowledge serialization, course of switching, and knowledge copying. This may considerably impression the pace of your knowledge processing pipeline.
Some of the efficient PySpark optimization strategies is to make use of PySpark’s built-in features at any time when attainable. PySpark comes with a wealthy library of features, all of that are optimized.
In circumstances during which advanced logic can’t be applied with the built-in features, utilizing vectorized UDFs, often known as Pandas UDFs, may also help to attain higher efficiency. Vectorized UDFs function on whole columns or arrays of knowledge, slightly than on particular person rows. This batch processing typically results in improved efficiency over row-wise UDFs.
Contemplate a activity during which the entire parts in a column have to be multiplied by two. Within the following instance, this operation is carried out utilizing a Python UDF:
from pyspark.sql.features import udf
from pyspark.sql.varieties import IntegerType
def multiply_by_two(n):
return n * 2
multiply_by_two_udf = udf(multiply_by_two, IntegerType())
df = df.withColumn("col1_doubled", multiply_by_two_udf(df["col1"]))
The multiply_by_two()
perform is a Python UDF which takes an integer n
and multiplies it by two. This perform is registered as a UDF utilizing udf()
and utilized to the column col1
inside the DataFrame df
.
The identical multiplication operation could be applied in a extra environment friendly method utilizing PySpark’s built-in features:
from pyspark.sql.features import col
df = df.withColumn("col1_doubled", col("col1") * 2)
In circumstances during which the operation can’t be carried out utilizing built-in features and a Python UDF is critical, a vectorized UDF can provide a extra environment friendly various:
from pyspark.sql.features import pandas_udf
from pyspark.sql.varieties import IntegerType
@pandas_udf(IntegerType())
def multiply_by_two_pd(s: pd.Sequence) -> pd.Sequence:
return s * 2
df = df.withColumn("col1_doubled", multiply_by_two_pd(df["col1"]))
This methodology applies the perform multiply_by_two_pd
to a complete collection of knowledge without delay, lowering the serialization overhead. Word that the enter and return of the multiply_by_two_pd
perform are each Pandas Sequence. A Pandas Sequence is a one-dimensional labeled array that can be utilized to characterize the information in a single column in a DataFrame.
Optimizing Efficiency in Information Processing
As machine studying and massive knowledge change into extra commonplace, engineers are adopting Apache Spark to deal with the huge quantities of knowledge that these applied sciences have to course of. Boosting the efficiency of Spark entails a spread of methods, all designed to optimize the utilization of accessible assets. Implementing the strategies mentioned right here will assist Spark course of giant volumes of knowledge way more effectively.
[ad_2]