[ad_1]
Introduction
Welcome to our complete information on Armstrong numbers! Right here, we discover the intriguing idea of Armstrong numbers. We start by defining Armstrong numbers and their distinctive properties by examples. You’ll discover ways to examine if a quantity is an Armstrong quantity utilizing Python. We may also uncover numerous strategies like iterative, recursive, and mathematical approaches. Moreover, we delve into superior methods equivalent to utilizing listing comprehension, purposeful programming, and extra. This text serves as a useful useful resource for understanding Armstrong numbers and their functions in programming and past.
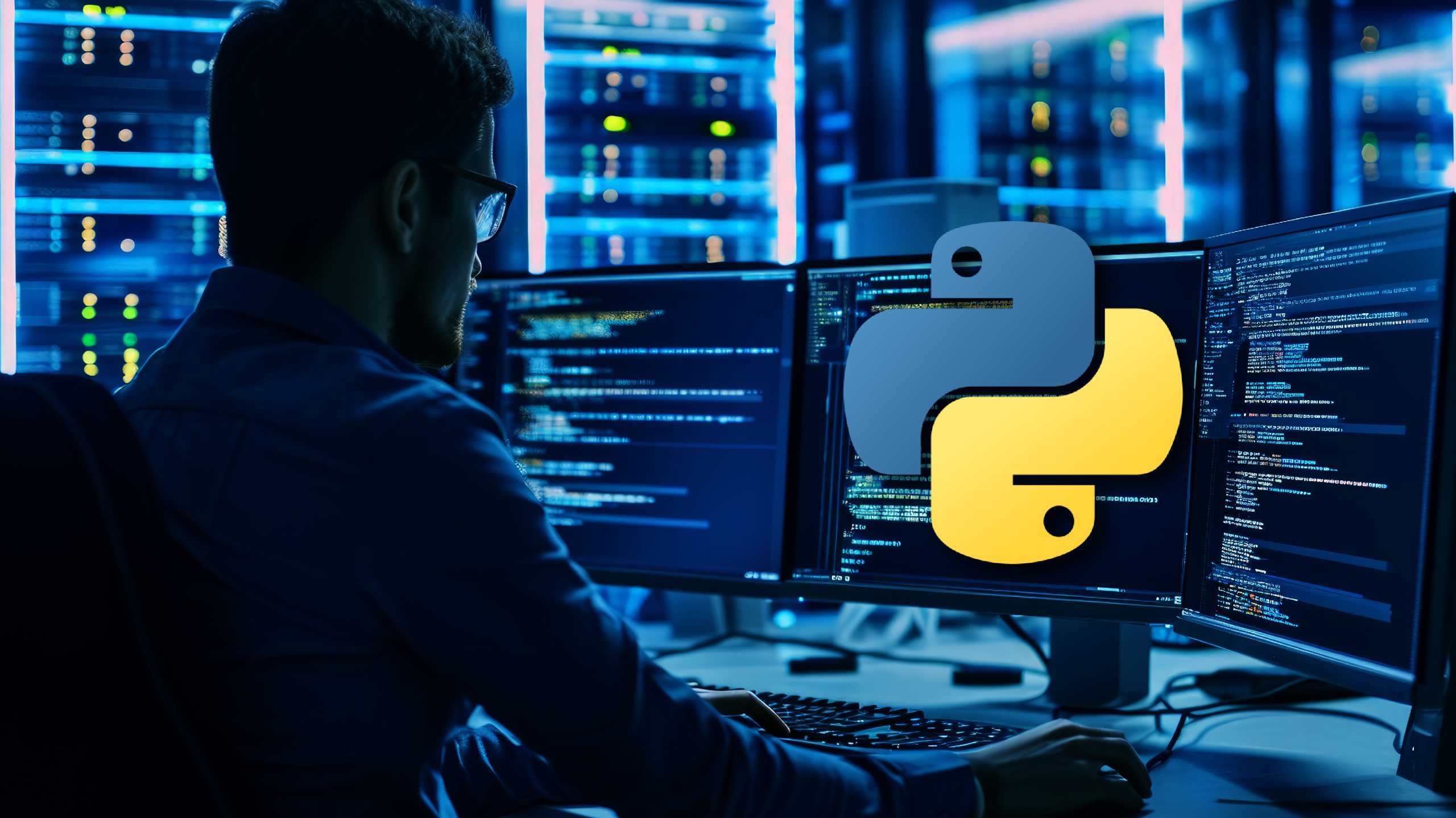
Studying Outcomes
- Perceive the definition and traits of Armstrong numbers.
- Learn how to make use of Python to find out if a given quantity is an Armstrong quantity.
- Discover numerous strategies and approaches to calculate Armstrong numbers.
- Achieve familiarity with programming ideas equivalent to loops, conditionals, capabilities and recursion by Armstrong quantity workouts.
- Acknowledge how Armstrong numbers can be utilized to enhance problem-solving and programming abilities.
What’s an Armstrong Quantity?
Armstrong quantity is a quantity that is the same as the sum of its personal digits every raised to the facility of the variety of digits. It’s also often called a narcissistic quantity or pluperfect quantity.
For instance:
- 153 is an Armstrong quantity as a result of 13+53+33=1531^3 + 5^3 + 3^3 = 15313+53+33=153
- 9474 is an Armstrong quantity as a result of 94+44+74+44=94749^4 + 4^4 + 7^4 + 4^4 = 947494+44+74+44=9474
Common Formulation:
abcd… = pow(a,n) + pow(b,n) + pow(c,n) + pow(d,n) + ….
Steps to Test Armstrong Quantity in Python
Allow us to now look into the fundamental steps on how we are able to examine whether or not a quantity is Armstrong or not.
- Step1: Enter the quantity.
- Step2: Calculate the variety of digits.
- Step3: Compute the sum of powers.
- Step4: Test if the sum equals the unique quantity.
Python Program to Test Armstrong Quantity
Let’s create a program that checks if a given quantity is an Armstrong quantity utilizing Python.
# Python program to find out whether or not
# a quantity is an Armstrong quantity or not
# Perform to compute the facility of a digit
def compute_power(base, exponent):
if exponent == 0:
return 1
half_power = compute_power(base, exponent // 2)
if exponent % 2 == 0:
return half_power * half_power
else:
return base * half_power * half_power
# Perform to find out the variety of digits within the quantity
def digit_count(quantity):
depend = 0
whereas quantity > 0:
depend += 1
quantity //= 10
return depend
# Perform to examine if the quantity is an Armstrong quantity
def is_armstrong_number(quantity):
num_digits = digit_count(quantity)
temp = quantity
armstrong_sum = 0
whereas temp > 0:
digit = temp % 10
armstrong_sum += compute_power(digit, num_digits)
temp //= 10
return armstrong_sum == quantity
# Driver code to check the operate
test_numbers = [153, 1253]
for num in test_numbers:
print(f"{num} is an Armstrong quantity: {is_armstrong_number(num)}")
Output:
153 is an Armstrong quantity: True
1253 is an Armstrong quantity: False
Time Complexity: O(n)
- Right here, n is the variety of digits within the quantity.
- The time complexity is O(n) as a result of we iterate by every digit of the quantity.
Auxiliary House: O(1)
- The house complexity is O(1) as we use a continuing quantity of house.
Strategies to Calculate Armstrong Quantity
We have now a number of strategies utilizing which we are able to discover Armstrong quantity. Allow us to look into these strategies one after the other intimately with code and their output.
Utilizing Iterative Technique
By iteratively growing every digit of the quantity to the facility of all of the digits and including the outcomes, the strategy works by all the variety of digits. Starting customers can profit from this technique as a result of it’s easy to make use of and comprehend. It employs elementary management constructions like as conditionals and loops. This technique is totally different from others as a result of its simplicity and direct strategy with out requiring further capabilities or superior ideas.
def is_armstrong_number(num):
digits = [int(d) for d in str(num)]
num_digits = len(digits)
sum_of_powers = sum(d ** num_digits for d in digits)
return num == sum_of_powers
# Test a quantity
print(is_armstrong_number(153)) # Output: True
print(is_armstrong_number(123)) # Output: False
Utilizing Recursive Technique
The recursive technique makes use of a recursive operate to calculate the sum of the digits raised to the facility of the variety of digits. It breaks down the issue into smaller cases, calling itself with a lowered quantity till a base case is met. This technique is elegant and helpful for studying recursion, which is a elementary idea in laptop science. It differs from the iterative technique by avoiding express loops and as a substitute counting on operate calls.
def sum_of_powers(num, num_digits):
if num == 0:
return 0
else:
return (num % 10) ** num_digits + sum_of_powers(num // 10, num_digits)
def is_armstrong_number(num):
num_digits = len(str(num))
return num == sum_of_powers(num, num_digits)
# Test a quantity
print(is_armstrong_number(153)) # Output: True
print(is_armstrong_number(123)) # Output: False
Utilizing Mathematical Strategy
This technique makes use of mathematical operations to isolate every digit, compute the required energy, and sum the outcomes. By using arithmetic operations like division and modulus, it processes every digit in an easy method. This strategy emphasizes the mathematical basis of the issue, differing from others by specializing in the quantity’s arithmetic properties slightly than looping or recursion.
def is_armstrong_number(num):
num_digits = len(str(num))
temp = num
sum_of_powers = 0
whereas temp > 0:
digit = temp % 10
sum_of_powers += digit ** num_digits
temp //= 10
return num == sum_of_powers
# Test a quantity
print(is_armstrong_number(153)) # Output: True
print(is_armstrong_number(123)) # Output: False
Utilizing Record Comprehension
This system creates a listing of numerals raised to the required energy utilizing Python’s listing comprehension, then sums the listing. This technique is succinct and leverages the expressive syntax of Python to create lists. It’s totally different from conventional loop-based strategies as a result of it compresses the logic right into a single, readable line of code, making it extra Pythonic and sometimes extra readable.
def is_armstrong_number(num):
num_digits = len(str(num))
return num == sum([int(digit) ** num_digits for digit in str(num)])
# Test a quantity
print(is_armstrong_number(153)) # Output: True
print(is_armstrong_number(123)) # Output: False
Utilizing Purposeful Programming
To deal with the numbers, this strategy makes use of higher-order capabilities like map and scale back. Each digit within the listing is given a operate by map, which then aggregates the outcomes right into a single worth. It shows paradigms for purposeful programming, that are distinct from crucial programming types present in different methods. This technique could end in code that’s extra condensed and presumably more practical.
from functools import scale back
def is_armstrong_number(num):
num_digits = len(str(num))
return num == scale back(lambda acc, digit: acc + int(digit) ** num_digits, str(num), 0)
# Test a quantity
print(is_armstrong_number(153)) # Output: True
print(is_armstrong_number(123)) # Output: False
Utilizing a Generator Perform
A generator operate is used to yield Armstrong numbers as much as a specified restrict, producing numbers on-the-fly with out storing them in reminiscence. This technique is reminiscence environment friendly and appropriate for producing massive sequences of numbers. It differs from others by leveraging Python’s generator capabilities, which may deal with massive knowledge units extra effectively than storing them in lists.
def armstrong_numbers(restrict):
for num in vary(restrict + 1):
num_digits = len(str(num))
if num == sum(int(digit) ** num_digits for digit in str(num)):
yield num
# Generate Armstrong numbers as much as 10000
for quantity in armstrong_numbers(10000):
print(quantity)
Utilizing String Manipulation
The quantity is handled as a string on this method, and the outcomes are summed after every character is iterated over to transform it again to an integer and lift it to the facility of the variety of digits. Not like arithmetic-based approaches, it’s simple and makes use of the string illustration of numbers utilizing string operations.
def is_armstrong_number(num):
num_str = str(num)
num_digits = len(num_str)
sum_of_powers = sum(int(digit) ** num_digits for digit in num_str)
return num == sum_of_powers
# Test a quantity
print(is_armstrong_number(153)) # Output: True
print(is_armstrong_number(123)) # Output: False
Utilizing a Lookup Desk for Powers
Precomputing the powers of digits and storing them in a lookup desk accelerates the calculation course of. This technique is environment friendly for big ranges of numbers, because it avoids redundant energy calculations. It differs from others by introducing a preprocessing step to optimize the primary computation.
def precompute_powers(max_digits):
powers = {}
for digit in vary(10):
powers[digit] = [digit ** i for i in range(max_digits + 1)]
return powers
def is_armstrong_number(num, powers):
num_str = str(num)
num_digits = len(num_str)
sum_of_powers = sum(powers[int(digit)][num_digits] for digit in num_str)
return num == sum_of_powers
# Precompute powers as much as an inexpensive restrict (e.g., 10 digits)
powers = precompute_powers(10)
# Test a quantity
print(is_armstrong_number(153, powers)) # Output: True
print(is_armstrong_number(123, powers)) # Output: False
Utilizing NumPy for Vectorized Computation
NumPy, a robust numerical library, is used for vectorized operations on arrays of digits. This technique is extremely environment friendly for dealing with massive datasets as a result of NumPy’s optimized efficiency. It stands out by leveraging the facility of NumPy to carry out operations in a vectorized method, decreasing the necessity for express loops.
import numpy as np
def find_armstrong_numbers(restrict):
armstrong_numbers = []
for num in vary(restrict + 1):
digits = np.array([int(digit) for digit in str(num)])
num_digits = digits.measurement
if num == np.sum(digits ** num_digits):
armstrong_numbers.append(num)
return armstrong_numbers
# Discover Armstrong numbers as much as 10000
print(find_armstrong_numbers(10000))
Utilizing Memoization
Memoization shops beforehand computed sums of powers to keep away from redundant calculations, rushing up the checking course of. This technique is useful for repeated checks of comparable numbers. It’s totally different from others by incorporating a caching mechanism to optimize efficiency, particularly helpful in eventualities with many repeated calculations.
def is_armstrong_number(num, memo):
if num in memo:
return memo[num]
num_str = str(num)
num_digits = len(num_str)
sum_of_powers = sum(int(digit) ** num_digits for digit in num_str)
memo[num] = (num == sum_of_powers)
return memo[num]
# Initialize memoization dictionary
memo = {}
# Test a quantity
print(is_armstrong_number(153, memo)) # Output: True
print(is_armstrong_number(123, memo)) # Output: False
Purposes of Armstrong Numbers
Armstrong numbers have a number of functions in numerous fields. Listed below are a few of the notable functions:
- Quantity Principle and Arithmetic: Armstrong numbers are utilized in quantity principle to check properties of numbers. They function an fascinating instance of self-referential numbers in mathematical discussions.
- Programming and Algorithm Design: Armstrong numbers are generally used as workouts in programming programs to apply loops, conditionals, capabilities, and mathematical operations. They supply a sensible strategy to exhibit and perceive recursion and iterative approaches in programming.
- Error Detection in Telecommunications: In telecommunication methods, Armstrong numbers can be utilized as a easy error-checking mechanism. By verifying whether or not a quantity despatched is the same as the sum of its digits raised to an influence, errors in transmission might be detected.
- Cryptographic Purposes: In some cryptographic algorithms, Armstrong numbers can be utilized as a part of the validation course of. They could be used to generate keys or carry out checks to make sure knowledge integrity.
- Academic Functions: Armstrong numbers are incessantly employed in classroom instruction to coach pupils to arithmetic and programming ideas. They will function examples of the importance of efficient algorithms and the results of varied programming strategies.
Conclusion
This text explores Armstrong numbers, their definitions, properties, and Python strategies for figuring out them. It covers iterative, recursive, mathematical, and computational approaches. Armstrong numbers are helpful for studying mathematical ideas and enhancing programming skills. They’ve functions in error detection, cryptography, and schooling. This data equips people to deal with challenges and discover the world of numbers in programming and arithmetic.
Don’t miss this opportunity to enhance your abilities and advance your profession. Study Python with us! This course is appropriate for all ranges.
Regularly Requested Questions
A. An Armstrong quantity, also referred to as a narcissistic or pluperfect quantity, is a quantity that is the same as the sum of its personal digits every raised to the facility of the variety of digits. For instance, 153 is an Armstrong quantity.
A. Armstrong numbers are generally known as narcissistic numbers as a result of they preserve a property of self-reference or self-regard. It’s because the quantity itself is the same as the sum of its personal digits raised to the facility of the variety of digits.
A. Armstrong numbers are generally used as examples in programming workouts to exhibit numerous programming methods equivalent to loops, conditionals, capabilities, recursion, and mathematical computations. They supply a sensible strategy to take a look at and enhance programming abilities.
A. No, by definition, Armstrong numbers are optimistic integers. Detrimental numbers and 0 can’t be Armstrong numbers as a result of the sum of the digits raised to the facility of the variety of digits is not going to equal a adverse quantity or zero.
[ad_2]