[ad_1]
After a decade or extra the place Single-Web page-Functions generated by
JavaScript frameworks have
change into the norm, we see that server-side rendered HTML is changing into
fashionable once more, additionally due to libraries comparable to HTMX or Turbo. Writing a wealthy net UI in a
historically server-side language like Go or Java is not simply doable,
however a really engaging proposition.
We then face the issue of the best way to write automated exams for the HTML
elements of our net functions. Whereas the JavaScript world has advanced highly effective and refined methods to check the UI,
ranging in dimension from unit-level to integration to end-to-end, in different
languages we would not have such a richness of instruments accessible.
When writing an online software in Go or Java, HTML is often generated
by means of templates, which include small fragments of logic. It’s definitely
doable to check them not directly by means of end-to-end exams, however these exams
are sluggish and costly.
We will as an alternative write unit exams that use CSS selectors to probe the
presence and proper content material of particular HTML parts inside a doc.
Parameterizing these exams makes it straightforward so as to add new exams and to obviously
point out what particulars every take a look at is verifying. This method works with any
language that has entry to an HTML parsing library that helps CSS
selectors; examples are supplied in Go and Java.
Motivation
Why test-drive HTML templates? In any case, probably the most dependable strategy to verify
{that a} template works is to render it to HTML and open it in a browser,
proper?
There’s some fact on this; unit exams can’t show {that a} template
works as anticipated when rendered in a browser, so checking them manually
is important. And if we make a
mistake within the logic of a template, often the template breaks
in an apparent manner, so the error is shortly noticed.
However:
- Counting on guide exams solely is dangerous; what if we make a change that breaks
a template, and we do not take a look at it as a result of we didn’t suppose it might impression the
template? We might get an error at runtime! - Templates typically include logic, comparable to if-then-else’s or iterations over arrays of things,
and when the array is empty, we regularly want to indicate one thing completely different.
Handbook checking all circumstances, for all of those bits of logic, turns into unsustainable in a short time - There are errors that aren’t seen within the browser. Browsers are extraordinarily
tolerant of inconsistencies in HTML, counting on heuristics to repair our damaged
HTML, however then we’d get completely different ends in completely different browsers, on completely different units. It is good
to verify that the HTML buildings we’re constructing in our templates correspond to
what we expect.
It seems that test-driving HTML templates is straightforward; let’s examine the best way to
do it in Go and Java. I shall be utilizing as a place to begin the TodoMVC
template, which is a pattern software used to showcase JavaScript
frameworks.
We are going to see strategies that may be utilized to any programming language and templating expertise, so long as we’ve
entry to an appropriate HTML parser.
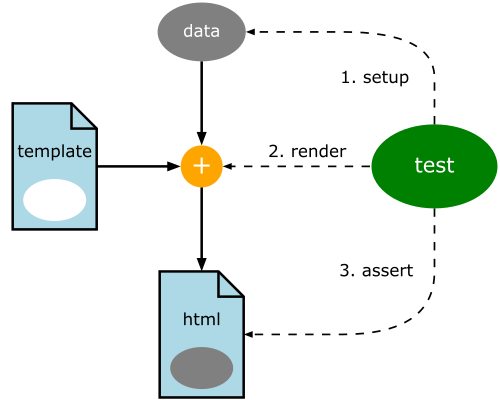
This text is a bit lengthy; it’s your decision to try the
last resolution in Go or
in Java,
or leap to the conclusions.
Degree 1: checking for sound HTML
The primary factor we wish to verify is that the HTML we produce is
mainly sound. I do not imply to verify that HTML is legitimate in keeping with the
W3C; it might be cool to do it, but it surely’s higher to start out with a lot less complicated and quicker checks.
As an illustration, we would like our exams to
break if the template generates one thing like
<div>foo</p>
Let’s have a look at the best way to do it in levels: we begin with the next take a look at that
tries to compile the template. In Go we use the usual html/template
bundle.
Go
func Test_wellFormedHtml(t *testing.T) { templ := template.Should(template.ParseFiles("index.tmpl")) _ = templ }
In Java, we use jmustache
as a result of it is quite simple to make use of; Freemarker or
Velocity are different widespread selections.
Java
@Check void indexIsSoundHtml() { var template = Mustache.compiler().compile( new InputStreamReader( getClass().getResourceAsStream("/index.tmpl"))); }
If we run this take a look at, it should fail, as a result of the index.tmpl
file does
not exist. So we create it, with the above damaged HTML. Now the take a look at ought to go.
Then we create a mannequin for the template to make use of. The appliance manages a todo-list, and
we are able to create a minimal mannequin for demonstration functions.
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Should(template.ParseFiles("index.tmpl"))
mannequin := todo.NewList()
_ = templ
_ = mannequin
}
Java
@Check
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var mannequin = new TodoList();
}
Now we render the template, saving the ends in a bytes buffer (Go) or as a String
(Java).
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Should(template.ParseFiles("index.tmpl"))
mannequin := todo.NewList()
var buf bytes.Buffer
err := templ.Execute(&buf, mannequin)
if err != nil {
panic(err)
}
}
Java
@Check
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var mannequin = new TodoList();
var html = template.execute(mannequin);
}
At this level, we wish to parse the HTML and we anticipate to see an
error, as a result of in our damaged HTML there’s a div
component that
is closed by a p
component. There may be an HTML parser within the Go
normal library, however it’s too lenient: if we run it on our damaged HTML, we do not get an
error. Fortunately, the Go normal library additionally has an XML parser that may be
configured to parse HTML (due to this Stack Overflow reply)
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Should(template.ParseFiles("index.tmpl"))
mannequin := todo.NewList()
// render the template right into a buffer
var buf bytes.Buffer
err := templ.Execute(&buf, mannequin)
if err != nil {
panic(err)
}
// verify that the template will be parsed as (lenient) XML
decoder := xml.NewDecoder(bytes.NewReader(buf.Bytes()))
decoder.Strict = false
decoder.AutoClose = xml.HTMLAutoClose
decoder.Entity = xml.HTMLEntity
for {
_, err := decoder.Token()
change err {
case io.EOF:
return // We're carried out, it is legitimate!
case nil:
// do nothing
default:
t.Fatalf("Error parsing html: %s", err)
}
}
}
This code configures the HTML parser to have the best degree of leniency
for HTML, after which parses the HTML token by token. Certainly, we see the error
message we wished:
--- FAIL: Test_wellFormedHtml (0.00s) index_template_test.go:61: Error parsing html: XML syntax error on line 4: surprising finish component </p>
In Java, a flexible library to make use of is jsoup:
Java
@Check
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var mannequin = new TodoList();
var html = template.execute(mannequin);
var parser = Parser.htmlParser().setTrackErrors(10);
Jsoup.parse(html, "", parser);
assertThat(parser.getErrors()).isEmpty();
}
And we see it fail:
java.lang.AssertionError: Anticipating empty however was:<[<1:13>: Unexpected EndTag token [</p>] when in state [InBody],
Success! Now if we copy over the contents of the TodoMVC
template to our index.tmpl
file, the take a look at passes.
The take a look at, nonetheless, is just too verbose: we extract two helper capabilities, in
order to make the intention of the take a look at clearer, and we get
Go
func Test_wellFormedHtml(t *testing.T) { mannequin := todo.NewList() buf := renderTemplate("index.tmpl", mannequin) assertWellFormedHtml(t, buf) }
Java
@Check void indexIsSoundHtml() { var mannequin = new TodoList(); var html = renderTemplate("/index.tmpl", mannequin); assertSoundHtml(html); }
[ad_2]