Construct RAG Utility with Cohere Command-R & Rerank – Half 2
Introduction
Within the earlier article, we experimented with Cohere’s Command-R mannequin and Rerank mannequin to generate responses and rerank doc sources. We have now carried out a easy RAG pipeline utilizing them to generate responses to person’s questions on ingested paperwork. Nonetheless, what now we have carried out may be very easy and unsuitable for the final person, because it has no person interface to work together with the chatbot immediately. On this article, we are going to modularize the codebase for straightforward interpretation and scaling and construct a Streamlit utility that may function an interface to work together with the RAG pipeline. The interface will probably be a chatbot interface that the person can use to work together with it. So, we are going to implement a further reminiscence part inside the utility, permitting customers to ask follow-up queries on earlier responses.
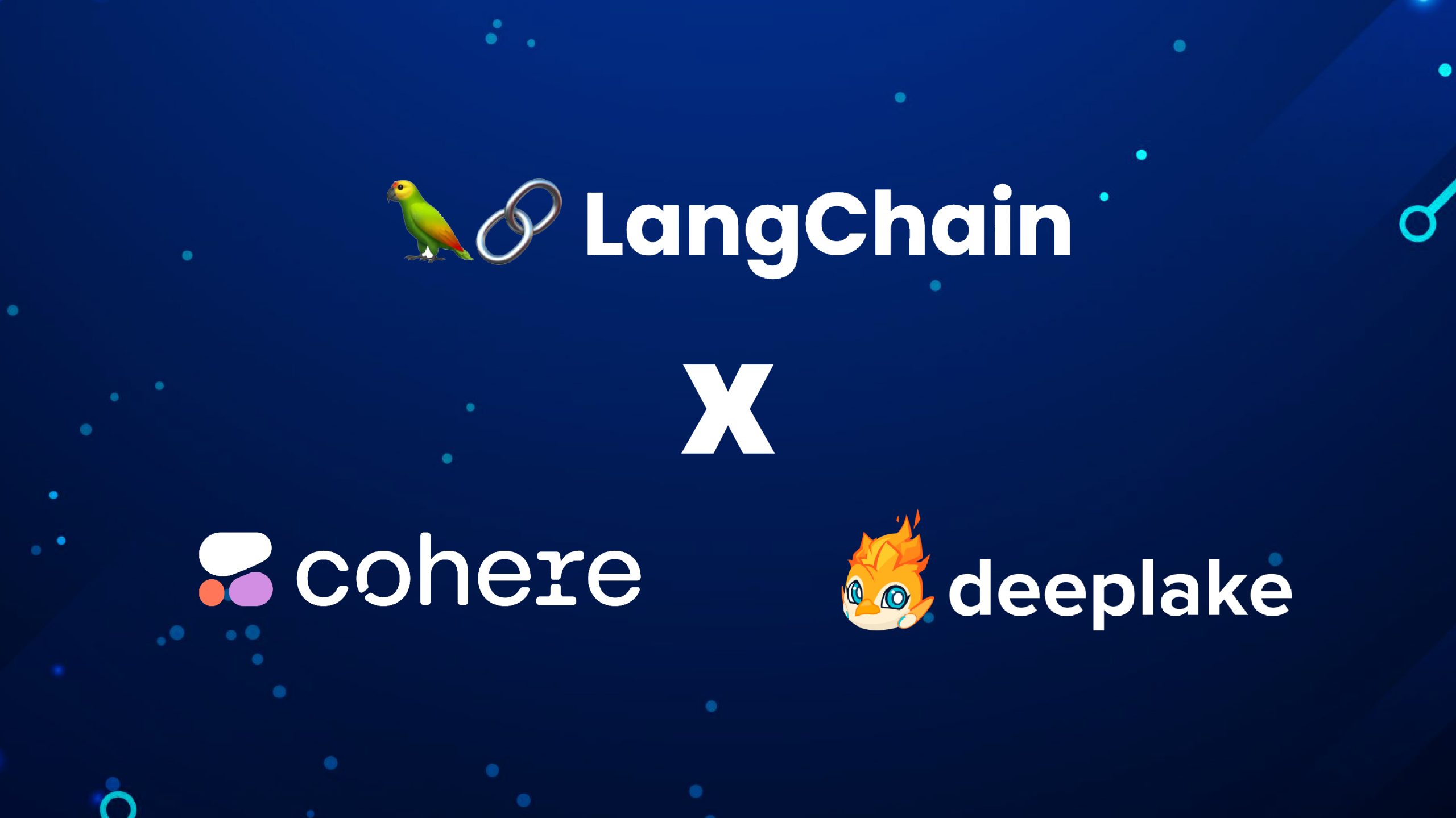
Studying Aims
- Utilizing object-oriented programming (OOP) rules, develop a reusable, modular codebase for numerous RAG pipelines.
- Create an ingestion pipeline for doc ingestion elements and a question pipeline for query-related elements. Each are unbiased and might run individually.
- Join solely the question pipeline to the Streamlit app for person queries, with an choice so as to add doc ingestion by modifying the code.
- Implement a reminiscence part to allow follow-up queries based mostly on earlier responses.
- Flip pocket book experiments into demo-able purposes inside the Python ecosystem.
- Facilitate sooner prototype growth with minimal code adjustments by creating reusable code for future RAG pipelines.
This text was revealed as part of the Information Science Blogathon.
Doc QnA Pipeline Growth
Step one in constructing a prototype or deployable utility is defining the configurations and constants used inside numerous utility sections. The appliance has a number of configurable choices, resembling chunk measurement and overlap within the Ingestion pipeline, the API key for Cohere endpoints, and the temperature for LLM technology. These configurations will probably be in a central config file, accessible from wherever inside the utility.
We might want to comply with a folder construction for this venture. We may have a ‘src’ listing the place all the mandatory information will probably be saved, and the app.py file will probably be within the root listing. Under is the construction that we are going to comply with:
.
├── .venv
├── src
│ ├── config.py
│ ├── constants.py
│ ├── ingestion.py
│ └── qna.py
├── app.py
└── necessities.txt
We are going to create two information for 2 functions: A config.py file to carry the key keys, a vector retailer path, and some different configurations and a constants.py file to carry all of the constants used within the utility just like the chunk measurement, chunk overlap, and immediate template. Under are the contents for the config.py file:
COHERE_EMBEDDING_MODEL_NAME = "embed-english-v3.0"
COHERE_MODEL_NAME = "command-r"
COHERE_RERANK_MODEL_NAME = "rerank-english-v3.0"
DEEPLAKE_VECTORSTORE = "/path/to/doc/vectorstore"
API_KEY = “”
Under are the contents for constants.py file:
PDF_CHARSPLITTER_CHUNKSIZE = 1000
PDF_CHARSPLITTER_CHUNK_OVERLAP = 100
TEMPERATURE = 0.3
TOP_K = 25
CONTEXT_THRESHOLD = 0.8
PROMPT_TEMPLATE = """
<YOUR PROMPT HERE>
Chat Historical past: {chat_history} Context: {context} Query: {query} Reply:
"""
Within the config.py file, I’ve put the Cohere API key, names of all of the fashions used, and path to the doc vector retailer. Within the constants.py file, I’ve put immediate template and different ingestion and technology configurations like chunk measurement and chunk overlap values, temperature for LLM technology, top_k for the topmost related chunks, and the context threshold to filter out chunks which have relevancy
rating under 0.8. The contents of the config.py and constants.py information may be modified based mostly on use circumstances.
Half 1 – Ingestion
Subsequent, we are going to have a look at how we are able to modularize the Ingestion pipeline. We are going to create a single class named Ingestion and add a way to generate embeddings and retailer them within the vector retailer. Word
that we are going to have single information for our use case for every pipeline. Because the complexity of the use case will increase, a number of information may be created to deal with every pipeline part. This can guarantee
code readability and ease in additional adjustments and updates.
Under is the code for the Ingestion class:
import timeimport time
import src.constants as fixed
import src.config as cfg
from langchain_cohere import CohereEmbeddings
from langchain.document_loaders.pdf import PyPDFLoader
from langchain.text_splitter import CharacterTextSplitter
class Ingestion:
def __init__(self):
self.text_vectorstore = None
self.embeddings = CohereEmbeddings(
mannequin=cfg.COHERE_EMBEDDING_MODEL_NAME,
cohere_api_key=cfg.API_KEY,
)
def create_and_add_embeddings(
self,
file_path: str,
):
self.text_vectorstore = DeepLake(
dataset_path=cfg.DEEPLAKE_VECTORSTORE,
embedding=self.embeddings,
verbose=False,
num_workers=4,
)
loader = PyPDFLoader(file_path=file_path)
text_splitter = CharacterTextSplitter(
separator="n",
chunk_size=fixed.PDF_CHARSPLITTER_CHUNKSIZE,
chunk_overlap=fixed.PDF_CHARSPLITTER_CHUNK_OVERLAP,
)
pages = loader.load()
chunks = text_splitter.split_documents(pages)
_ = self.text_vectorstore.add_documents(paperwork=chunks)
import src.constants as fixed
import src.config as cfg
from langchain_cohere import CohereEmbeddings
from langchain.document_loaders.pdf import PyPDFLoader
from langchain.text_splitter import CharacterTextSplitter
class Ingestion:
def __init__(self):
self.text_vectorstore = None
self.embeddings = CohereEmbeddings(
mannequin=cfg.COHERE_EMBEDDING_MODEL_NAME,
cohere_api_key=cfg.API_KEY,
)
def create_and_add_embeddings(
self,
file_path: str,
):
self.text_vectorstore = DeepLake(
dataset_path=cfg.DEEPLAKE_VECTORSTORE,
embedding=self.embeddings,
verbose=False,
num_workers=4,
)
loader = PyPDFLoader(file_path=file_path)
text_splitter = CharacterTextSplitter(
separator="n",
chunk_size=fixed.PDF_CHARSPLITTER_CHUNKSIZE,
chunk_overlap=fixed.PDF_CHARSPLITTER_CHUNK_OVERLAP,
)
pages = loader.load()
chunks = text_splitter.split_documents(pages)
_ = self.text_vectorstore.add_documents(paperwork=chunks)
Let’s perceive every a part of the above code. First, we import all needed packages, together with the constants and config information. Then, we outline the category Ingestion and its class constructor utilizing the __init__ technique. We set the text_vectorstore variable to None, which will probably be initialized with the vector retailer occasion later. Then, we initialize the Embeddings mannequin occasion utilizing the mannequin identify and the API key from the config.
Subsequent, we create the create_and_add_embeddings technique, which takes the file_path to which the doc is ingested. Inside this technique, we first initialize the vector retailer utilizing the vector retailer path and embeddings. We have now additionally set the num_workers to 4 in order that 4 CPU cores are utilized for sooner processing. Then, we initialize the PDF Loader object utilizing the file_path, after which we use the Character Splitter to separate the chunks. We then load the PDF file and break up the pages into additional chunks. The ultimate chunks are then added to the vector retailer.
Half 2 – QnA
Now that now we have the ingestion pipeline setup, we are going to create the QnA pipeline. Under is the code for the QnA class:
import time
import src.constants as fixed
import src.config as cfg
from pymongo import MongoClient
from langchain_cohere import CohereEmbeddings
from langchain_cohere import ChatCohere
from langchain.reminiscence.chat_message_histories.sql import SQLChatMessageHistory
from langchain.reminiscence import ConversationBufferWindowMemory
from langchain.chains.conversational_retrieval.base import ConversationalRetrievalChain
from langchain.prompts import PromptTemplate
from langchain.retrievers import ContextualCompressionRetriever
from langchain_cohere import CohereRerank
class QnA:
def __init__(self):
self.embeddings = CohereEmbeddings(
mannequin=cfg.COHERE_EMBEDDING_MODEL_NAME,
cohere_api_key=cfg.API_KEY,
)
self.mannequin = ChatCohere(
mannequin=cfg.COHERE_MODEL_NAME,
cohere_api_key=cfg.API_KEY,
temperature=fixed.TEMPERATURE,
)
self.cohere_rerank = CohereRerank(
cohere_api_key=cfg.API_KEY,
mannequin=cfg.COHERE_RERANK_MODEL_NAME,
)
self.text_vectorstore = None
self.text_retriever = None
def ask_question(
self,
question,
session_id,
verbose: bool = False,
):
start_time = time.time()
self.init_vectorstore()
memory_key = "chat_history"
historical past = SQLChatMessageHistory(
session_id=session_id,
connection_string="sqlite:///reminiscence.db",
)
PROMPT = PromptTemplate(
template=fixed.PROMPT_TEMPLATE,
input_variables=["chat_history", "context", "question"],
)
reminiscence = ConversationBufferWindowMemory(
memory_key=memory_key,
output_key="reply",
input_key="query",
chat_memory=historical past,
ok=2,
return_messages=True,
)
chain_type_kwargs = {"immediate": PROMPT}
qa = ConversationalRetrievalChain.from_llm(
llm=self.mannequin,
combine_docs_chain_kwargs=chain_type_kwargs,
retriever=self.text_retriever,
verbose=verbose,
reminiscence=reminiscence,
return_source_documents=True,
chain_type="stuff",
)
response = qa.invoke({"query": question})
exec_time = time.time() - start_time
return response
def init_vectorstore(self):
self.text_vectorstore = DeepLake(
dataset_path=cfg.DEEPLAKE_VECTORSTORE,
embedding=self.embeddings,
verbose=False,
read_only=True,
num_workers=4,
)
self.text_retriever = ContextualCompressionRetriever(
base_compressor=self.cohere_rerank,
base_retriever=self.text_vectorstore.as_retriever(
search_type="similarity",
search_kwargs={
"fetch_k": 20,
"ok": fixed.TOP_K,
},
),
)
We created a QnA class with an initializer that units up the question-answering system. It creates an occasion of the CohereEmbeddings class for producing textual content embeddings utilizing the mannequin’s identify and API key. It additionally initializes the ChatCohere class for conversational duties with a temperature worth for textual content randomness and the CohereRerank class for reranking responses based mostly on relevance.
The ask_question technique takes a question, session ID, and non-obligatory verbose flag. The init_vectorstore technique initializes the vector database and retriever elements. A reminiscence key and an occasion of SQLChatMessageHistory manages dialog historical past. The PromptTemplate codecs the question and historical past, and the ConversationBufferWindowMemory manages the dialog buffer reminiscence.
The ConversationalRetrievalChain class combines the retriever and language mannequin for question-answering. It’s initialized with the language mannequin, immediate template, retriever, and different settings. The invoke technique generates a response based mostly on the question and historical past and calculates the execution time of ask_question.
The init_vectorstore technique units up the vector database and retriever. The DeepLake occasion initializes the vector database with the trail, embedding mannequin, and different parameters. The ContextualCompressionRetriever manages the retriever part with the reranking mannequin and vector database, specifying the search sort and parameters.
Half 3 – Streamlit UI
Now that each the Ingestion and QnA pipelines are prepared, we are going to construct the Streamlit interface that may make the most of the pipelines. Under is your complete code for the Streamlit interface:
import streamlit as st
from src.qna import QnA
from dataclasses import dataclass
@dataclass
class Message:
actor: str
payload: str
def predominant():
st.set_page_config(
page_title="KnowledgeGPT",
page_icon="????",
structure="centered",
initial_sidebar_state="collapsed",
)
st.header("????KnowledgeGPT")
USER = "person"
ASSISTANT = "ai"
MESSAGES = "messages"
with st.spinner(textual content="Initializing..."):
st.session_state["qna"] = QnA()
qna = st.session_state["qna"]
if MESSAGES not in st.session_state:
st.session_state[MESSAGES] = [
Message(
actor=ASSISTANT,
payload="Hi! How can I help you?",
)
]
msg: Message
for msg in st.session_state[MESSAGES]:
st.chat_message(msg.actor).write(msg.payload)
immediate: str = st.chat_input("Enter a immediate right here")
if immediate:
st.session_state[MESSAGES].append(Message(actor=USER, payload=immediate))
st.chat_message(USER).write(immediate)
with st.spinner(textual content="Considering..."):
response = qna.ask_question(
question=immediate, session_id="AWDAA-adawd-ADAFAEF"
)
st.session_state[MESSAGES].append(Message(actor=ASSISTANT, payload=response))
st.chat_message(ASSISTANT).write(response)
if __name__ == "__main__":
predominant()
Streamlit UI Performance
The Streamlit UI serves because the user-facing part of our utility. Right here’s a breakdown of its performance:
- Web page Configuration: The st.set_page_config operate units the web page title, icon, structure, and preliminary state of the sidebar.
- Constants: We outline constants for the person (USER), assistant (ASSISTANT), and messages (MESSAGES) to enhance code readability.
- QnA Occasion Initialization: We initialize the QnA occasion and retailer it within the st.session_state dictionary. This ensures that the occasion persists throughout totally different app periods.
- Chat Messages Initialization: If MESSAGES isn’t current in st.session_state, we initialize it with a welcome message from the assistant.
- Show Chat Messages: The code iterates by way of the MESSAGES record and shows every message together with the sender (person or assistant).
- Consumer Enter: Immediate the person to enter a immediate utilizing st.chat_input.
- Processing Consumer Enter: If the person gives a immediate, code appends it to the MESSAGES record and generates the assistant’s response utilizing the ask_question technique of the QnA occasion.
- Show Assistant Response: Append the assistant’s response to the MESSAGES record and show it to the person.
Lastly, we run the primary technique to launch the app. We will begin the app utilizing the next command:
streamlit run app.py
Working of the App
Under is a brief demo of how the app works:
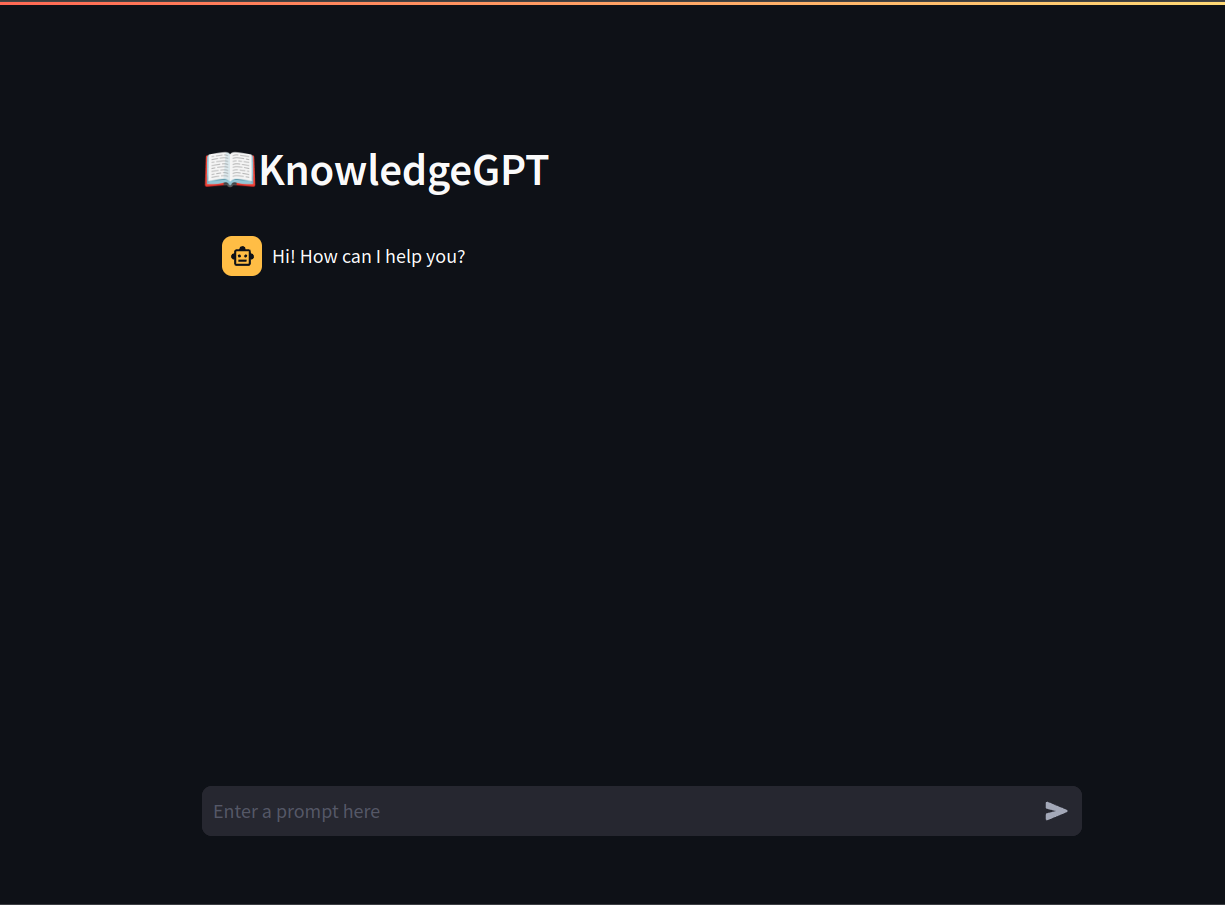
Right here’s how KnowledgeGPT will work:
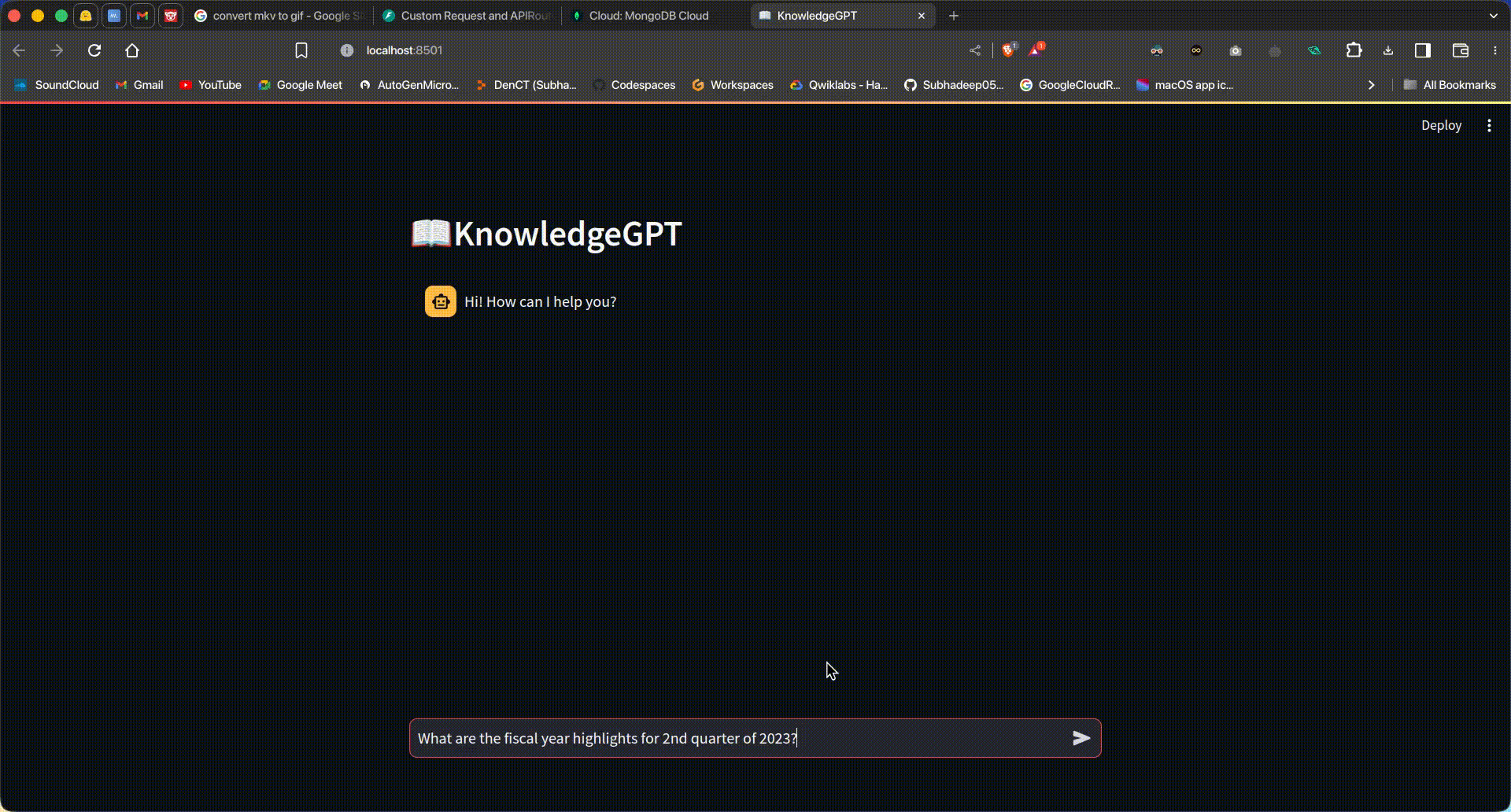
Conclusion
On this article, we’ve remodeled our preliminary RAG pipeline experiment right into a extra strong and user-friendly utility. Modifying the codebase has improved readability, maintainability, and scalability. Separate ingestion and question pipelines permit unbiased growth and upkeep, enhancing the appliance’s total scalability.
Integrating a modular backend with a Streamlit interface creates a seamless person expertise by way of a chatbot interface that helps follow-up queries, making interactions dynamic and conversational. Utilizing object-oriented programming rules, we’ve structured our code for readability and reusability, which is important for scaling and adapting to new necessities.
Our implementation of configurations and constants administration, together with the setup of ingestion and QnA pipelines, gives a transparent path for builders. This setup simplifies the transition from a Jupyter Pocket book experiment to a deployable utility, preserving the venture inside the Python ecosystem.
This text affords a complete information to creating an interactive doc QnA utility with Cohere’s fashions. By uniting theoretical experimentation and sensible implementation, it allows builders to construct environment friendly and scalable options. With the given code and clear directions, you are actually able to develop, customise, and launch your personal RAG-based purposes, expediting the creation of clever doc question programs.
Key Takeaways
- Enhances maintainability and scalability by separating ingestion and question pipelines.
- Offers a user-friendly chatbot interface for dynamic interactions.
- Ensures a structured, reusable, and scalable codebase.
- Centralized configurations in devoted information for flexibility and ease of administration.
- Effectively handles doc ingestion and person queries utilizing Cohere’s fashions.
- Permits dealing with of follow-up queries for coherent, context-aware interactions.
- Facilitates fast prototyping and growth of different RAG pipelines.
The media proven on this article should not owned by Analytics Vidhya and is used on the Writer’s discretion.
Often Requested Questions
A. Completely! In actual fact, that’s the ultimate method of making gen AI pipelines. As soon as the pipelines are prepared, they need to be wrapped with a RESTful API for use from the frontend.
A. The Streamlit interface gives a user-friendly chatbot interface for interacting with the RAG pipeline, making it simple for customers to ask questions and obtain responses.
Ans. Sure. The aim of constructing a modularized pipeline is to have the ability to sew it to any frontend UI, be it Streamlit, Gradio, or JavaScript-based UI frameworks.