[ad_1]
Introduction
In depth-first search (DFS), all nodes are explored alongside some department and backtracked. Consider it as being in a maze: DFS goes down one path till it reaches a dead-end earlier than retracing its steps to take one other, proper? It’s the similar as happening, validating the tunnel, and so forth for all tunnels.
DFS is helpful for:
- Visiting each node in a graph.
- Checking how totally different nodes are linked.
Whereas DFS dives deep, one other methodology known as Breadth-First Search (BFS) checks all neighbors on the present stage earlier than transferring deeper. Each strategies are essential, however Depth First Search (DFS) helps you go so far as potential down one path earlier than attempting one other.
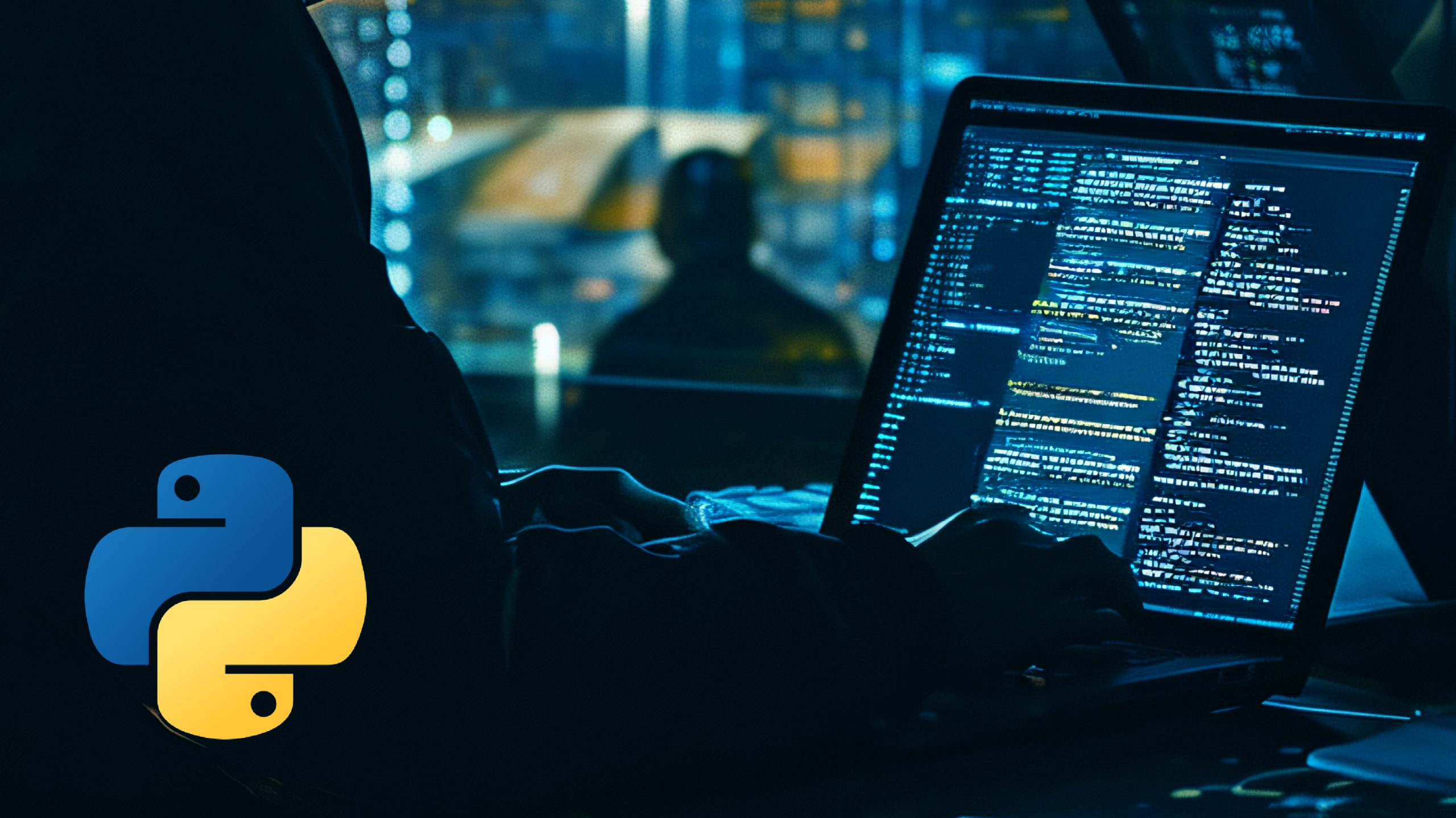
The Overview
- DFS will exhaustively go to a single path earlier than backtracking to a node with an unvisited path.
- DFS-Recursive makes use of a name stack to handle traversal and goes deeper into every path.
- Makes use of a separate stack to keep up the exploration; subsequently, no recursion depth downside.
- DFS’s time complexity is O(V+E)O(V + E)O(V+E), and its area complexity is O(V)O(V)O(V).
- DFS is cool for a lot of issues. A number of the most typical are pathfinding, cycle detection, topological sorting, and puzzle fixing.
- Distinction between DFS and BFS BFS explores every stage first after which goes to the following stage, whereas DFS goes by one department after which strikes to the present.
How Does Depth First Search (DFS) Work?
The DFS algorithm entails visiting nodes as deeply as potential earlier than backtracking. Right here’s a step-by-step rationalization:
- Beginning node: The search will begin on the root node, within the case of a tree, or from an arbitrary node, within the case of the graph.
- Go to: Mark node as visited.
- Discover: For every adjoining node, recursively go to the node if it has not been visited but.
- Backtrack: When a node with no unvisited adjoining nodes is reached, backtrack to the earlier node and proceed the method.
Additionally learn: A Full Python Tutorial to Study Information Science from Scratch
Depth First Search (DFS) Algorithm
DFS—Depth First Search is a recursive algorithm. To implement it for a graph, we are able to both use recursion or implicit recursion utilizing Stack.
Recursive Implementation
The recursive implementation of DFS leverages the decision stack to handle the traversal state. Here’s a Python implementation:
Code:
def dfs_recursive(graph, node, visited):
if node not in visited:
print(node, finish=' ')
visited.add(node)
for neighbour in graph[node]:
dfs_recursive(graph, neighbour, visited)
# Instance utilization:
graph = {
'A': ['B', 'C', 'D'],
'B': ['E'],
'C': ['G', 'F'],
'D': ['H'],
'E': ['I'],
'F': ['J'],
'G': ['K']
}
visited = set()
print("DFS traversal utilizing recursive strategy:")
dfs_recursive(graph, 'A', visited)
Iterative Implementation
The iterative implementation makes use of an express stack to handle the traversal. This may be helpful to keep away from potential points with recursion depth in languages that restrict the decision stack measurement.
Code:
def dfs_iterative(graph, begin):
visited = set()
stack = [start]
whereas stack:
node = stack.pop()
if node not in visited:
print(node, finish=' ')
visited.add(node)
stack.prolong(reversed(graph[node])) # Add in reverse order to keep up the order of traversal
# Instance utilization:
graph = {
'A': ['B', 'C', 'D'],
'B': ['E'],
'C': ['G', 'F'],
'D': ['H'],
'E': ['I'],
'F': ['J'],
'G': ['K']
}
print("nDFS traversal utilizing iterative strategy:")
dfs_iterative(graph, 'A')
Rationalization
The code examples confer with the graph as an adjacency listing. Every node is sort of a key, itemizing all of the nodes straight linked to it. To keep away from revisiting the identical node, now we have a set named visited, which shops the earlier node.
- Recursive DFS: The dfs_recursive operate calls itself for every unvisited neighbor of the present node, diving deep into every path.
- Iterative DFS: The dfs_iterative operate makes use of a stack (a listing the place you add and take away gadgets) to handle which nodes to go to subsequent. This stack works like the decision stack within the recursive methodology, serving to observe the order of visits.
Each strategies guarantee all elements of the graph are explored, however they do it otherwise.
Time and Area Complexity
Right here is the time and area complexity of DFS:
- Time complexity: The time complexity of DFS is O(V + E), the place V and E are the variety of vertices and edges, respectively. Within the worst case, every vertex and edge might be searched as soon as.
- Area Complexity: Area complexity can be O(V) since we have to hold observe of visited nodes. In recursion, we’d run a recursion stack, or we could push nodes into the stack in iterative.
Functions of Depth First Search (DFS)
Depth First Search (DFS) has quite a few purposes in laptop science and real-world issues:
- Pathfinding: DFS can be helpful for locating a path between two nodes in a graph.
- Cycle Detection: It helps detect cycles in a graph and is helpful in numerous domains, like dependency decision.
- Use circumstances for topological sorting: Scheduling the duties so that every process relies on the others and have to be carried out in linear order.
- Graph Traversal & Related Elements: DFS in an undirected graph to establish all linked elements.
- Maze and Puzzle Fixing: Clear up advanced maze and puzzles by traversing each potential path.
Actual-World Instance
Suppose you need to discover all of the paths in a community of computer systems in order that the info might be transmitted appropriately. DFS is an algorithm that performs a depth-first search in a graph. It may be used to begin from a selected laptop and observe connections so far as they go, backtracking when no extra connections could be adopted.
Code:
def find_paths(graph, begin, finish, path=[]):
path = path + [start]
if begin == finish:
return [path]
if begin not in graph:
return []
paths = []
for node in graph[start]:
if node not in path:
new_paths = find_paths(graph, node, finish, path)
for p in new_paths:
paths.append(p)
return paths
# Instance utilization:
community = {
'Computer1': ['Computer2', 'Computer3'],
'Computer2': ['Computer4'],
'Computer3': ['Computer4', 'Computer5'],
'Computer4': ['Computer6'],
'Computer5': ['Computer6'],
'Computer6': []
}
begin="Computer1"
finish = 'Computer6'
print(f"All paths from {begin} to {finish}:")
paths = find_paths(community, begin, finish)
for path in paths:
print(" -> ".be part of(path))
DFS vs BFS
Whereas DFS dives deep right into a graph, BFS explores all neighbors of a node earlier than transferring to the following stage. Every has its benefits:
- DFS: Makes use of much less reminiscence and might discover a path with out exploring all nodes.
- BFS: Ensures discovering the shortest path in an unweighted graph.
Conclusion
DFS, or Depth-First Search, is a strong utility for traversing graphs and utilizing them in tree issues. DFS is helpful when fixing puzzles, discovering your method in a maze, or organizing duties. The 2 methods to make use of DFS are:
- Recursive DFS: this makes use of operate calls to trace the place you’re coming from within the graph.
- Iterative DFS: Utilizing a stack to deal with the steps.
The two strategies assured full protection of the graph; each node was explored. Here’s a listing of how DFS can discover paths, detect cycles, kind duties, and join elements in a graph. Gaining information about DFS will enable you to clear up powerful issues. After seeing the examples, you possibly can discover DFS in your code.
So, are you in search of Python programs on-line? If sure, discover – Introduction to Python at present!
Regularly Requested Questions
A. The first aim of DFS is to traverse all of the nodes in a graph, visiting every node precisely as soon as (which suggests no node is visited twice or extra), whereas recursively visiting as deep as potential alongside a department earlier than backtracking.
A. DFS could be applied utilizing recursion, however iterative implementation is desired, particularly the place the stack is proscribed, or the recursion depth restrict just isn’t excessive, to stop stack overflow.
A. DFS handles cycles by retaining observe of visited nodes, guaranteeing every node is visited solely as soon as to stop infinite loops.
A. DFS doesn’t assure the shortest path in an unweighted graph; Breadth-First Search (BFS) is healthier suited to this function.
[ad_2]