[ad_1]
Introduction
Methodology overloading and methodology overriding are two elementary ideas in object-oriented programming (OOP) you should know. They’ll vastly improve the flexibleness and performance of your code, particularly in fields like information science and synthetic intelligence, which require environment friendly and maintainable code. Though these two phrases would possibly sound comparable, their underlying mechanisms are considerably completely different. They even have very completely different use circumstances. On this article, we are going to be taught what methodology overloading and overriding are, whereas understanding their variations and exploring their purposes.
New to OOP? Right here’s a beginner-level article to get you began with the fundamentals: Object-Oriented Programming in Python For Absolute Rookies
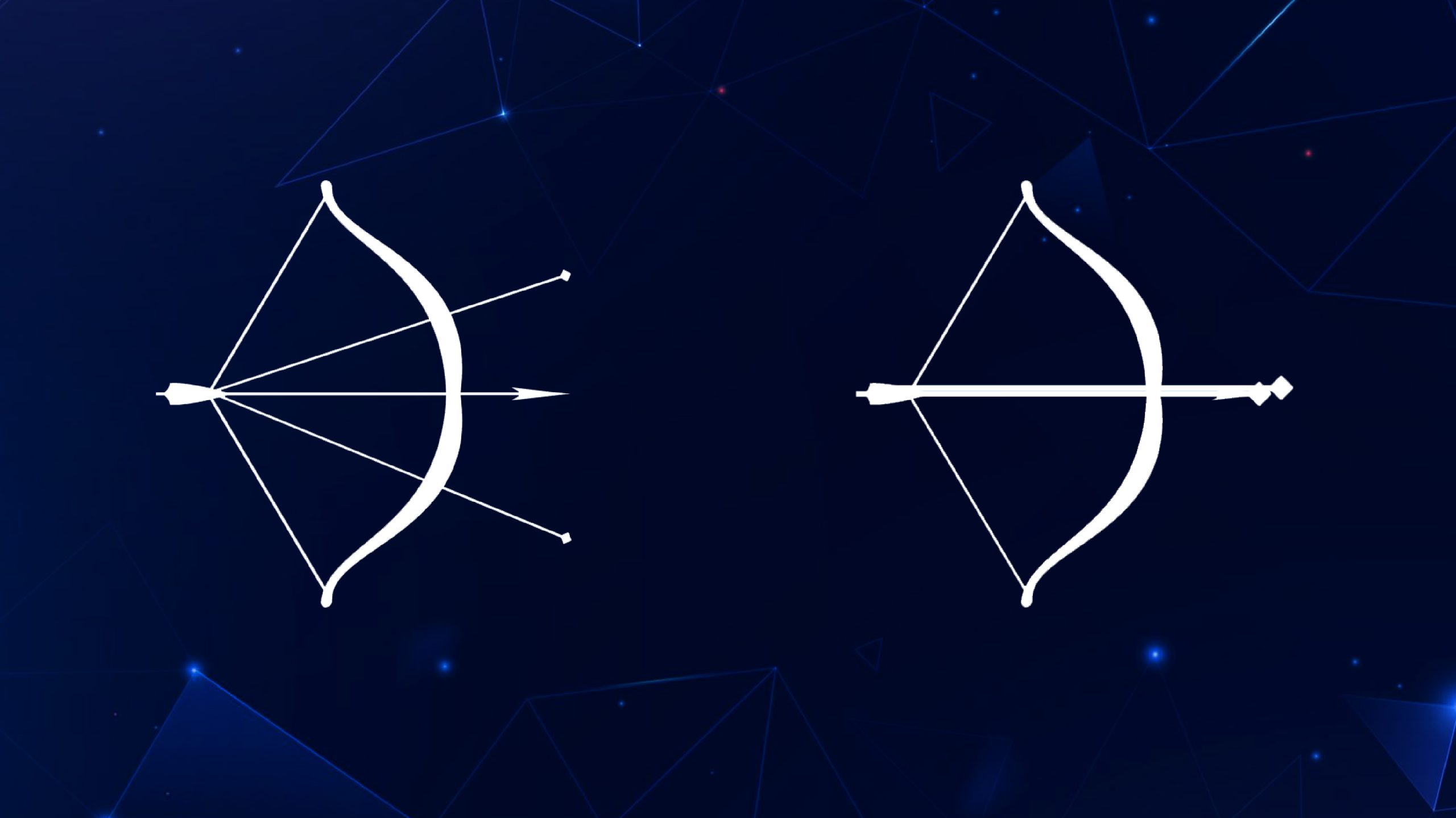
Overview
- Perceive what methodology overloading and methodology overriding are.
- Know the syntax and implementation of every of them.
- Know the advantages and sensible purposes of each.
- Study the important thing variations between methodology overloading and overriding.
What’s Methodology Overloading?
Methodology overloading, often known as compile-time polymorphism, is a characteristic in OOP that enables a category to have a number of strategies with the identical title however completely different parameters. The compiler differentiates these strategies primarily based on the quantity, kind, and order of parameters. This lets you carry out comparable actions via a single methodology title, which improves the readability and reusability of your code.
Methodology overloading is fairly simple in most programming languages like Java and C++. Python, nevertheless, doesn’t help it in the identical means. In Python, you possibly can outline strategies with the identical title, however the final methodology outlined will overwrite the earlier ones. As a substitute, Python makes use of default arguments, variable-length arguments, and key phrase arguments to attain comparable performance.
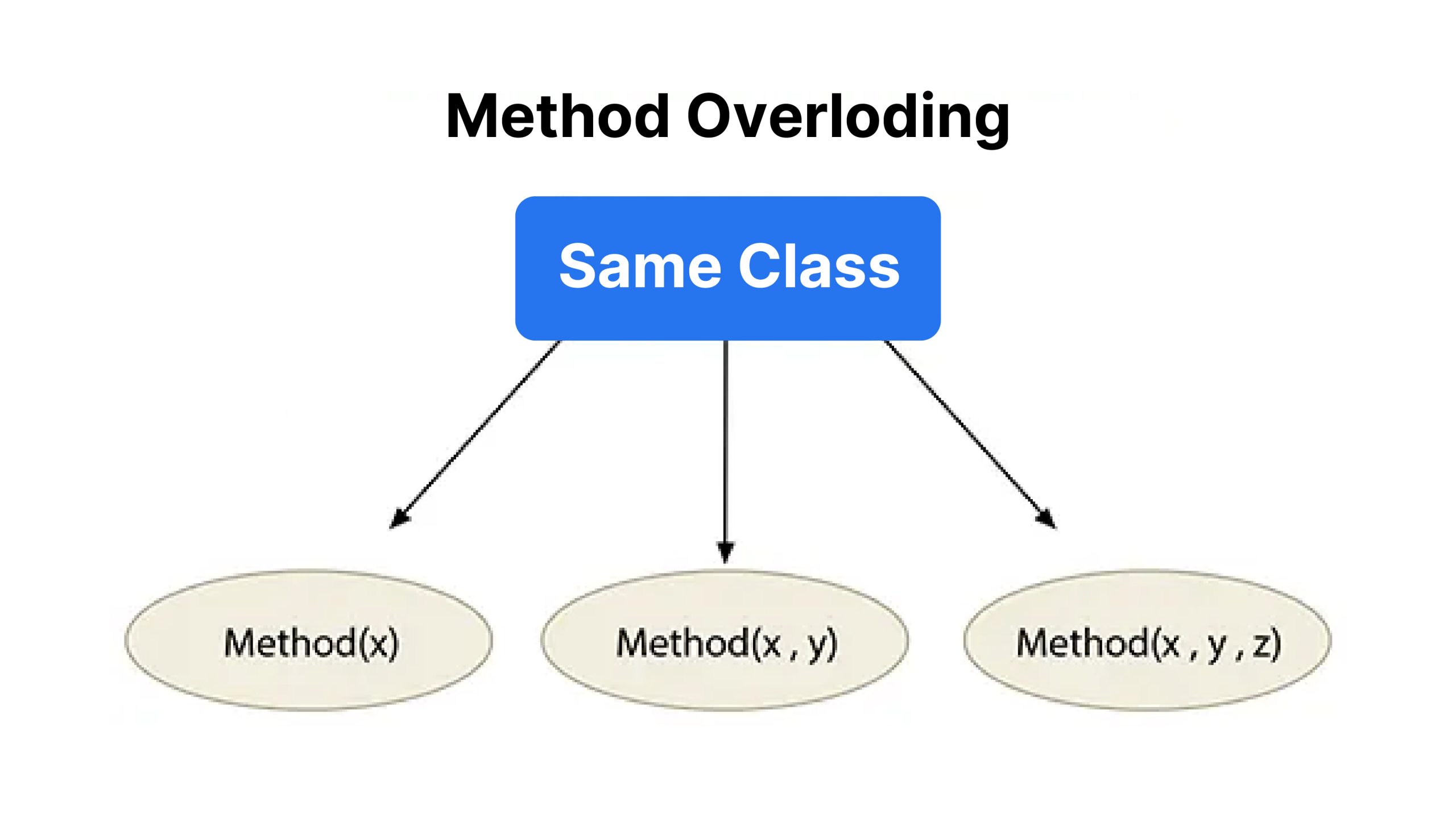
Syntax
Right here’s an instance of methodology overloading in Java:
class MathOperations {
// Methodology so as to add two integers
int add(int a, int b) {
return a + b;
}
// Overloaded methodology so as to add three integers
int add(int a, int b, int c) {
return a + b + c;
}
// Overloaded methodology so as to add two doubles
double add(double a, double b) {
return a + b;
}
}
On this instance, the add
methodology is overloaded with completely different parameter lists. The suitable methodology is invoked primarily based on the arguments handed throughout the methodology name.
Advantages of Methodology Overloading
- Code Readability: Utilizing the identical methodology title for comparable operations makes the code extra intuitive and simpler to know.
- Reusability: Overloaded strategies can carry out completely different duties with out the necessity for distinctive methodology names. This reduces code redundancy.
- Flexibility: Builders can add new performance by introducing new overloaded strategies with out affecting the present code.
Sensible Functions of Methodology Overloading
- Mathematical Libraries: To carry out operations on completely different information varieties equivalent to integers, floats, and so forth.
- String Manipulation Libraries: To deal with numerous types of enter information equivalent to substrings, character arrays, and so forth.
- Utility Capabilities: For common utility strategies that have to deal with numerous information varieties.
- Constructor Overloading: To instantiate objects in numerous methods primarily based on the offered parameters.
What’s Methodology Overriding?
Methodology overriding, often known as runtime polymorphism, is a characteristic in OOP that enables a subclass to offer a selected implementation of a technique already outlined in its superclass. That is required for polymorphism, or dynamic methodology dispatch, the place the tactic to be executed is set at runtime primarily based on the article’s kind. On this case, the overriding methodology within the subclass ought to have the identical title, return kind, and parameter checklist as the tactic within the superclass.
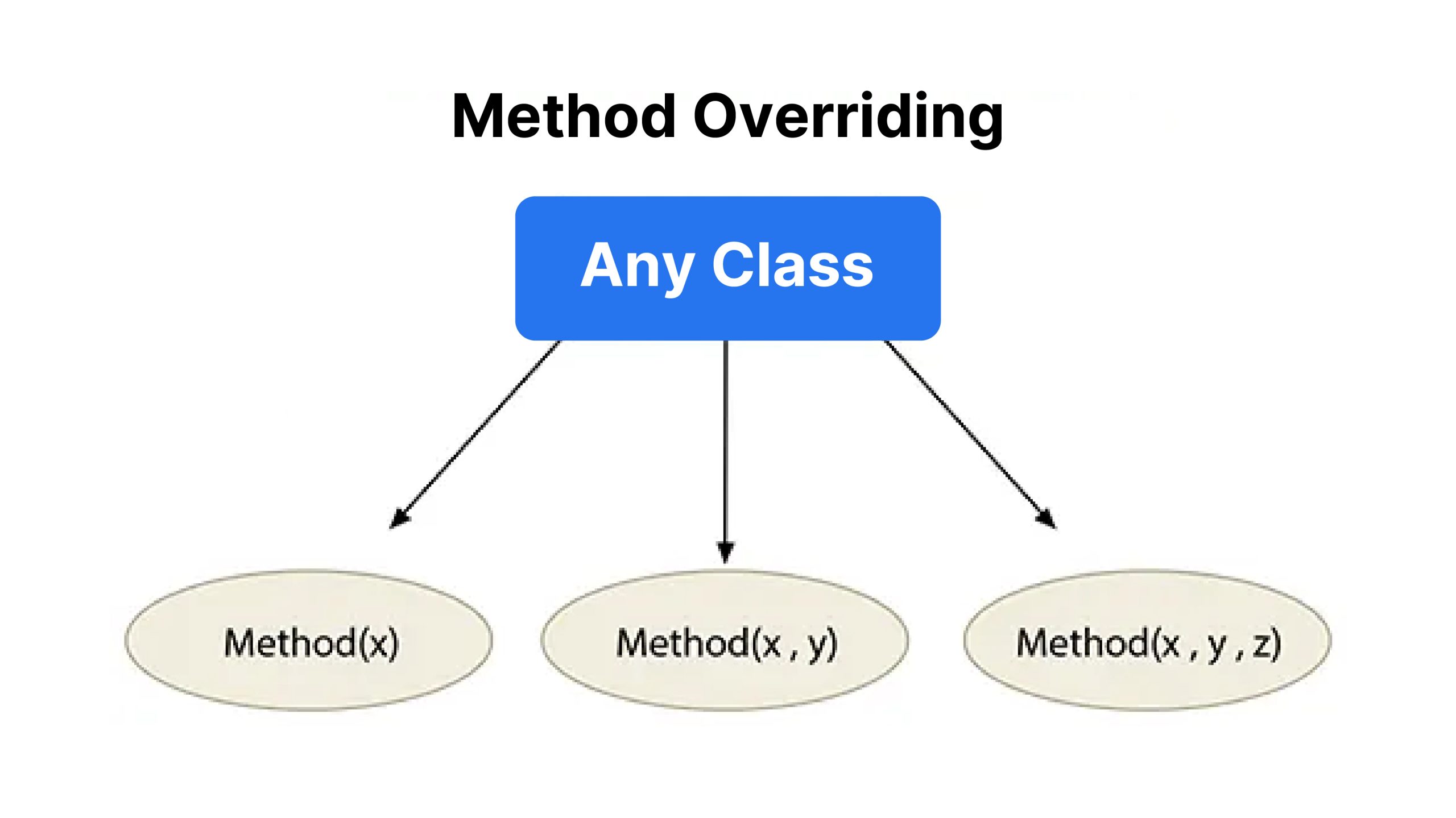
Syntax
Right here’s an instance of overriding in Python:
class Mother or father:
def present(self):
print("That is the dad or mum class")
class Youngster(Mother or father):
def present(self):
print("That is the kid class")
On this case, the present
methodology within the Youngster
class overrides the present
methodology within the Mother or father
class.
Advantages of Methodology Overriding
- Dynamic Polymorphism: Supplies flexibility by enabling the choice of the suitable methodology at runtime.
- Extensibility: Permits subclasses to increase or modify the performance of a superclass methodology.
- Code Maintainability: Permits subclasses to offer particular implementations whereas reusing the interface outlined by the superclass.
- Inheritance Utilization: Promotes the usage of inheritance by permitting subclasses to refine or substitute the conduct of superclass strategies.
- Reusability: Inherits strategies from the superclass and modifies them solely when crucial.
Sensible Functions of Methodology Overriding
- Customized Conduct: To tailor a superclass methodology to fulfill the particular wants of a subclass.
- Framework Growth: To increase or modify base framework courses and customise the conduct of pre-defined strategies.
- GUI Libraries: To deal with consumer interactions otherwise in numerous elements.
- API Design: To supply default implementations in base courses and permit overrides in subclasses for particular behaviors.
- Design Patterns: To override the steps of an algorithm by subclasses in patterns just like the Template Methodology sample.
Key Variations Between Methodology Overloading and Overriding
Characteristic | Methodology Overloading | Methodology Overriding |
Definition | A number of strategies with the identical title however completely different parameter lists. | A subclass supplies a selected implementation of a technique outlined within the superclass. |
Binding Time | Compile-time | Runtime |
Parameters | Should differ in quantity, kind, or order | Have to be the identical |
Return Sort | Might be completely different | Have to be the identical |
Inheritance | Not required | Required |
Objective | To carry out comparable duties with completely different inputs | To supply a selected implementation for a superclass methodology |
Conclusion
You will need to know the right way to use methodology overloading and overriding for writing environment friendly and maintainable object-oriented code. Methodology overloading enhances code readability and reusability by permitting comparable operations via a single methodology title. In the meantime, methodology overriding permits dynamic polymorphism, permitting subclasses to customise or substitute the conduct of superclass strategies.
These ideas will aid you broaden functionalities and create versatile, reusable code buildings. They’re actually helpful in complicated fields like information science and synthetic intelligence. By studying these methods, you possibly can improve your programming expertise and produce extra maintainable, scalable, and adaptable software program options.
Ceaselessly Requested Questions
A. Whereas Python doesn’t help conventional methodology overloading, you possibly can obtain comparable conduct utilizing default arguments or variable-length argument lists.
A. Methodology overriding permits a subclass to offer a selected implementation for a technique already outlined in its superclass.
A. No, methodology overloading is just not thought of polymorphism. Methodology overriding, nevertheless, is.
A. Though Python doesn’t help methodology overloading within the conventional sense, you possibly can simulate it utilizing default arguments or by dealing with completely different numbers of arguments manually.
A. A standard instance of methodology overriding is a graphical consumer interface framework the place a base class has a draw
methodology, and completely different subclasses like Circle
, Rectangle
, and Triangle
override this methodology to attract particular shapes.
[ad_2]