[ad_1]


Picture by Writer
Outliers are irregular observations that differ considerably from the remainder of your information. They might happen because of experimentation error, measurement error, or just that variability is current inside the information itself. These outliers can severely impression your mannequin’s efficiency, resulting in biased outcomes – very like how a high performer in relative grading at universities can elevate the typical and have an effect on the grading standards. Dealing with outliers is a vital a part of the information cleansing process.
On this article, I am going to share how one can spot outliers and other ways to cope with them in your dataset.
Detecting Outliers
There are a number of strategies used to detect outliers. If I had been to categorise them, right here is the way it seems to be:
- Visualization-Primarily based Strategies: Plotting scatter plots or field plots to see information distribution and examine it for irregular information factors.
- Statistics-Primarily based Strategies: These approaches contain z scores and IQR (Interquartile Vary) which provide reliability however could also be much less intuitive.
I will not cowl these strategies extensively to remain centered, on the subject. Nonetheless, I am going to embody some references on the finish, for exploration. We are going to use the IQR methodology in our instance. Right here is how this methodology works:
IQR (Interquartile Vary) = Q3 (seventy fifth percentile) – Q1 (twenty fifth percentile)
The IQR methodology states that any information factors under Q1 – 1.5 * IQR or above Q3 + 1.5 * IQR are marked as outliers. Let’s generate some random information factors and detect the outliers utilizing this methodology.
Make the mandatory imports and generate the random information utilizing np.random
:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
# Generate random information
np.random.seed(42)
information = pd.DataFrame({
'worth': np.random.regular(0, 1, 1000)
})
Detect the outliers from the dataset utilizing the IQR Technique:
# Perform to detect outliers utilizing IQR
def detect_outliers_iqr(information):
Q1 = information.quantile(0.25)
Q3 = information.quantile(0.75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
return (information < lower_bound) | (information > upper_bound)
# Detect outliers
outliers = detect_outliers_iqr(information['value'])
print(f"Variety of outliers detected: {sum(outliers)}")
Output ⇒ Variety of outliers detected: 8
Visualize the dataset utilizing scatter and field plots to see the way it seems to be
# Visualize the information with outliers utilizing scatter plot and field plot
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(15, 6))
# Scatter plot
ax1.scatter(vary(len(information)), information['value'], c=['blue' if not x else 'red' for x in outliers])
ax1.set_title('Dataset with Outliers Highlighted (Scatter Plot)')
ax1.set_xlabel('Index')
ax1.set_ylabel('Worth')
# Field plot
sns.boxplot(x=information['value'], ax=ax2)
ax2.set_title('Dataset with Outliers (Field Plot)')
ax2.set_xlabel('Worth')
plt.tight_layout()
plt.present()
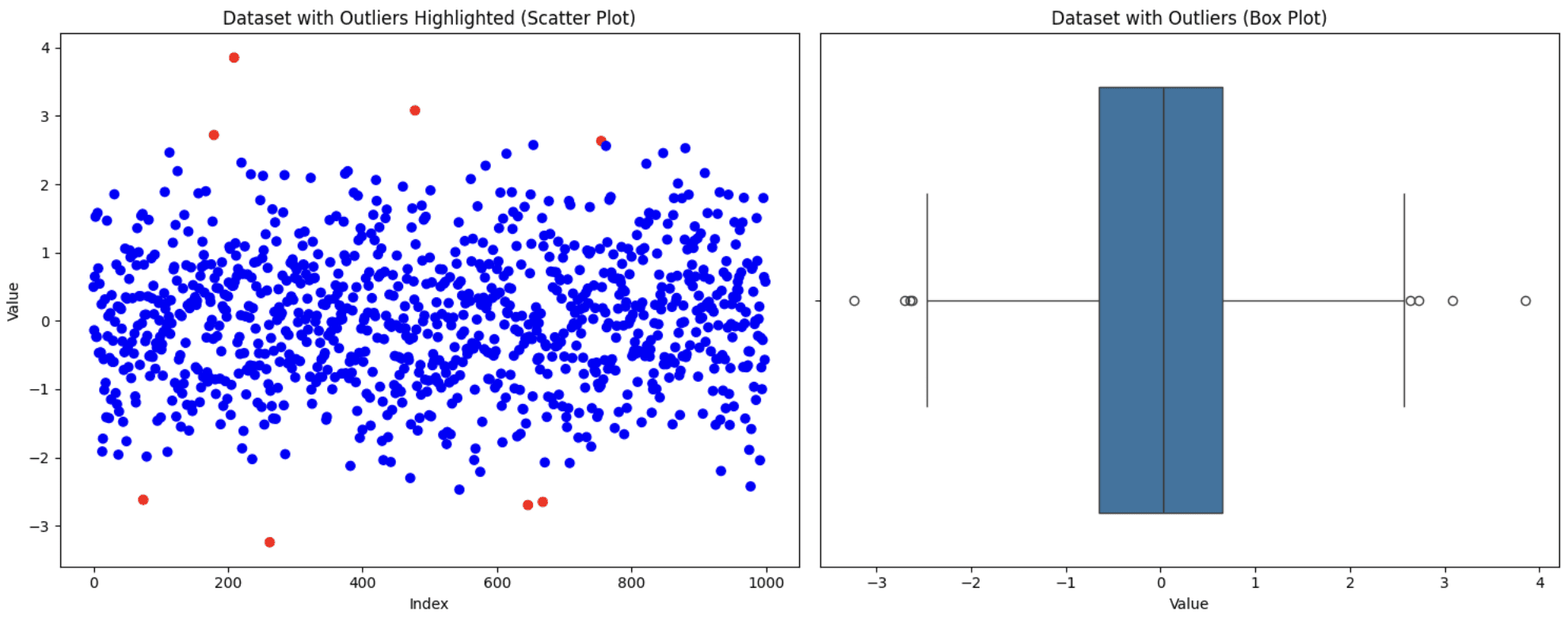
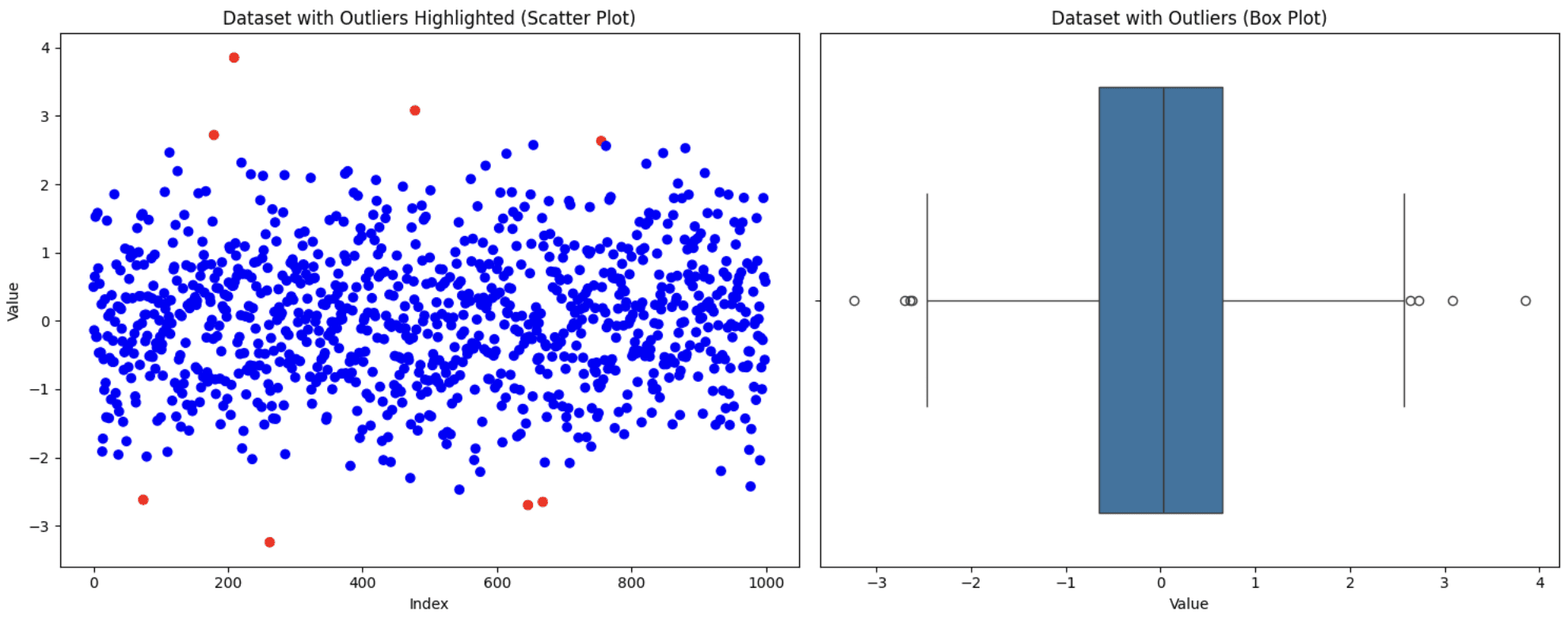
Authentic Dataset
Now that we’ve detected the outliers, let’s focus on a few of the other ways to deal with the outliers.
Dealing with Outliers
1. Eradicating Outliers
This is likely one of the easiest approaches however not all the time the suitable one. It’s worthwhile to take into account sure elements. If eradicating these outliers considerably reduces your dataset dimension or in the event that they maintain beneficial insights, then excluding them out of your evaluation not be essentially the most favorable choice. Nonetheless, in the event that they’re because of measurement errors and few in quantity, then this method is appropriate. Let’s apply this method to the dataset generated above:
# Take away outliers
data_cleaned = information[~outliers]
print(f"Authentic dataset dimension: {len(information)}")
print(f"Cleaned dataset dimension: {len(data_cleaned)}")
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(15, 6))
# Scatter plot
ax1.scatter(vary(len(data_cleaned)), data_cleaned['value'])
ax1.set_title('Dataset After Eradicating Outliers (Scatter Plot)')
ax1.set_xlabel('Index')
ax1.set_ylabel('Worth')
# Field plot
sns.boxplot(x=data_cleaned['value'], ax=ax2)
ax2.set_title('Dataset After Eradicating Outliers (Field Plot)')
ax2.set_xlabel('Worth')
plt.tight_layout()
plt.present()


Eradicating Outliers
Discover that the distribution of the information can truly be modified by eradicating outliers. Should you take away some preliminary outliers, the definition of what’s an outlier could very properly change. Due to this fact, information that will have been within the regular vary earlier than, could also be thought-about outliers underneath a brand new distribution. You’ll be able to see a brand new outlier with the brand new field plot.
2. Capping Outliers
This method is used when you do not need to discard your information factors however preserving these excessive values also can impression your evaluation. So, you set a threshold for the utmost and the minimal values after which carry the outliers inside this vary. You’ll be able to apply this capping to outliers or to your dataset as a complete too. Let’s apply the capping technique to our full dataset to carry it inside the vary of the Fifth-Ninety fifth percentile. Right here is how one can execute this:
def cap_outliers(information, lower_percentile=5, upper_percentile=95):
lower_limit = np.percentile(information, lower_percentile)
upper_limit = np.percentile(information, upper_percentile)
return np.clip(information, lower_limit, upper_limit)
information['value_capped'] = cap_outliers(information['value'])
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(15, 6))
# Scatter plot
ax1.scatter(vary(len(information)), information['value_capped'])
ax1.set_title('Dataset After Capping Outliers (Scatter Plot)')
ax1.set_xlabel('Index')
ax1.set_ylabel('Worth')
# Field plot
sns.boxplot(x=information['value_capped'], ax=ax2)
ax2.set_title('Dataset After Capping Outliers (Field Plot)')
ax2.set_xlabel('Worth')
plt.tight_layout()
plt.present()


Capping Outliers
You’ll be able to see from the graph that the higher and decrease factors within the scatter plot seem like in a line because of capping.
3. Imputing Outliers
Typically eradicating values from the evaluation is not an possibility as it could result in info loss, and also you additionally don’t need these values to be set to max or min like in capping. On this state of affairs, one other method is to substitute these values with extra significant choices like imply, median, or mode. The selection varies relying on the area of information underneath commentary, however be aware of not introducing biases whereas utilizing this method. Let’s substitute our outliers with the mode (essentially the most incessantly occurring worth) worth and see how the graph seems:
information['value_imputed'] = information['value'].copy()
median_value = information['value'].median()
information.loc[outliers, 'value_imputed'] = median_value
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(15, 6))
# Scatter plot
ax1.scatter(vary(len(information)), information['value_imputed'])
ax1.set_title('Dataset After Imputing Outliers (Scatter Plot)')
ax1.set_xlabel('Index')
ax1.set_ylabel('Worth')
# Field plot
sns.boxplot(x=information['value_imputed'], ax=ax2)
ax2.set_title('Dataset After Imputing Outliers (Field Plot)')
ax2.set_xlabel('Worth')
plt.tight_layout()
plt.present()


Imputing Outliers
Discover that now we have no outliers, however this does not assure that outliers will probably be eliminated since after the imputation, the IQR additionally modifications. It’s worthwhile to experiment to see what matches greatest in your case.
4. Making use of a Transformation
Transformation is utilized to your full dataset as an alternative of particular outliers. You principally change the best way your information is represented to cut back the impression of the outliers. There are a number of transformation methods like log transformation, sq. root transformation, box-cox transformation, Z-scaling, Yeo-Johnson transformation, min-max scaling, and so forth. Selecting the best transformation in your case depends upon the character of the information and your finish aim of the evaluation. Listed here are a couple of suggestions that will help you choose the suitable transformation method:
- For right-skewed information: Use log, sq. root, or Field-Cox transformation. Log is even higher whenever you wish to compress small quantity values which might be unfold over a big scale. Sq. root is healthier when, aside from proper skew, you need a much less excessive transformation and likewise wish to deal with zero values, whereas Field-Cox additionally normalizes your information, which the opposite two do not.
- For left-skewed information: Replicate the information first after which apply the methods talked about for right-skewed information.
- To stabilize variance: Use Field-Cox or Yeo-Johnson (much like Field-Cox however handles zero and destructive values as properly).
- For mean-centering and scaling: Use z-score standardization (commonplace deviation = 1).
- For range-bound scaling (mounted vary i.e., [2,5]): Use min-max scaling.
Let’s generate a right-skewed dataset and apply the log transformation to the entire information to see how this works:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import seaborn as sns
# Generate right-skewed information
np.random.seed(42)
information = np.random.exponential(scale=2, dimension=1000)
df = pd.DataFrame(information, columns=['value'])
# Apply Log Transformation (shifted to keep away from log(0))
df['log_value'] = np.log1p(df['value'])
fig, axes = plt.subplots(2, 2, figsize=(15, 10))
# Authentic Information - Scatter Plot
axes[0, 0].scatter(vary(len(df)), df['value'], alpha=0.5)
axes[0, 0].set_title('Authentic Information (Scatter Plot)')
axes[0, 0].set_xlabel('Index')
axes[0, 0].set_ylabel('Worth')
# Authentic Information - Field Plot
sns.boxplot(x=df['value'], ax=axes[0, 1])
axes[0, 1].set_title('Authentic Information (Field Plot)')
axes[0, 1].set_xlabel('Worth')
# Log Reworked Information - Scatter Plot
axes[1, 0].scatter(vary(len(df)), df['log_value'], alpha=0.5)
axes[1, 0].set_title('Log Reworked Information (Scatter Plot)')
axes[1, 0].set_xlabel('Index')
axes[1, 0].set_ylabel('Log(Worth)')
# Log Reworked Information - Field Plot
sns.boxplot(x=df['log_value'], ax=axes[1, 1])
axes[1, 1].set_title('Log Reworked Information (Field Plot)')
axes[1, 1].set_xlabel('Log(Worth)')
plt.tight_layout()
plt.present()


Making use of Log Transformation
You’ll be able to see {that a} easy transformation has dealt with a lot of the outliers itself and lowered them to only one. This reveals the facility of transformation in dealing with outliers. On this case, it’s essential to be cautious and know your information properly sufficient to decide on acceptable transformation as a result of failing to take action could trigger issues for you.
Wrapping Up
This brings us to the top of our dialogue about outliers, other ways to detect them, and tips on how to deal with them. This text is a part of the pandas collection, and you may verify different articles on my creator web page. As talked about above, listed here are some extra assets so that you can examine extra about outliers:
- Outlier detection strategies in Machine Studying
- Totally different transformations in Machine Studying
- Varieties Of Transformations For Higher Regular Distribution
Kanwal Mehreen Kanwal is a machine studying engineer and a technical author with a profound ardour for information science and the intersection of AI with medication. She co-authored the book “Maximizing Productiveness with ChatGPT”. As a Google Technology Scholar 2022 for APAC, she champions range and tutorial excellence. She’s additionally acknowledged as a Teradata Variety in Tech Scholar, Mitacs Globalink Analysis Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having based FEMCodes to empower girls in STEM fields.
[ad_2]