[ad_1]
Introduction
OpenCV’s contour operate is a basic characteristic in laptop imaginative and prescient that enables for the detection and evaluation of the shapes and limits of objects inside a picture. By defining contours as curves connecting steady factors alongside a boundary with the identical shade or depth, this operate allows numerous functions, from object detection to form evaluation and picture segmentation.
Picture processing has revolutionized the best way visible information is analyzed and understood. The OpenCV library is likely one of the strongest instruments in real-time laptop vision-based functions. This library accommodates a operate referred to as `discover Contours` used for picture segmentation, form evaluation, and object detection. Let’s dive deep into an in depth clarification of the discover Contours operate in OpenCV: what’s it used for, how does it work, and numerous means you could possibly apply the above to your initiatives.
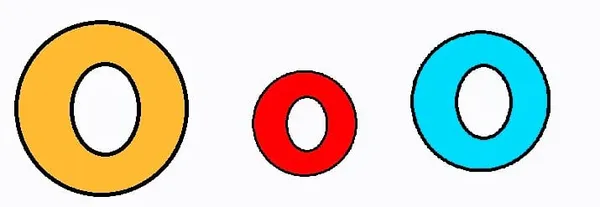
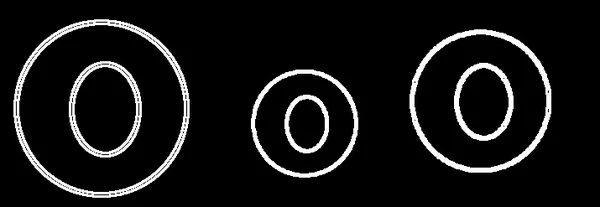
Studying Outcomes
- Perceive the idea of contours in picture processing and their significance in laptop imaginative and prescient.
- Implement the `discover Contours` operate in OpenCV to detect and analyze object boundaries in pictures.
- Achieve in-depth data of the parameters used within the `discover Contours` operate and the way they have an effect on the contour detection course of.
- Discover numerous sensible functions of contours, together with object detection, form evaluation, and have extraction.
This text was printed as part of the Information Science Blogathon.
What’s OpenCV?
OpenCV, or Open Supply Laptop Imaginative and prescient Library, is a crucial toolkit that gives expansive instruments and functionalities for picture and videotape processing. It helps a variety of operations, related as picture recognition, stir shadowing, and level discovery. Amongst these, determine discovery is a vital operation that enables us to establish and dissect the shapes of objects inside a picture.
Contours are curves that be part of all the continual factors alongside a boundary having the identical shade or depth. In easier phrases, contours could be seen as the perimeters or outlines of objects in a picture. They’re important for figuring out and dealing with particular shapes and objects in laptop imaginative and prescient duties.
What are Contours?
Contours are helpful in lots of functions reminiscent of object detection, form evaluation, and picture segmentation. By detecting contours, you may:
- Determine the boundaries of objects inside a picture.
- Analyze shapes to find out their properties (e.g., space, perimeter).
- Section pictures by separating objects from the background.
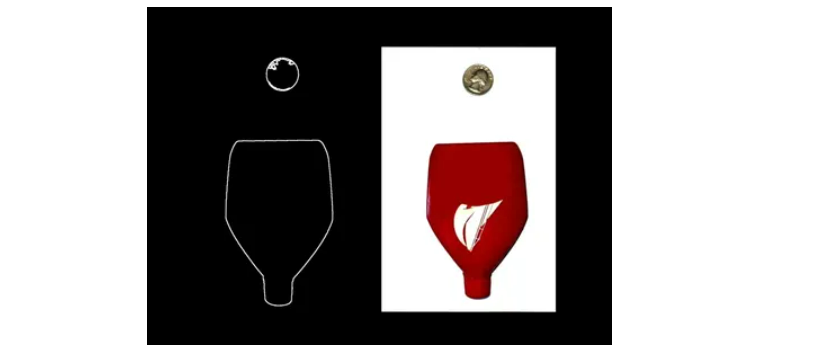
As within the above picture, the boundary of the item and the form could be derived by segmenting the objects (bottle and the coin) from the background utilizing the OpenCV contour operate.
Why do Contours Matter?
By processing much less information, contours make a picture easier whereas sustaining all the necessary particulars concerning the form and construction of the item. They’re important for jobs requiring the localization and identification of objects.
How discover Contours Works?
The `discover Contours` operate in OpenCV is designed to retrieve contours from a binary picture. A binary picture is a grayscale picture the place the pixels are both black or white, which makes it simpler to tell apart the perimeters of objects.
Steps to seek out Contour
- Convert to Grayscale: Convert the picture to a grayscale picture, which simplifies the processing.
- Apply Thresholding: Convert the grayscale picture to a binary picture by making use of a threshold.
- Detect Contours: Use the `discover Contours` operate to detect contours within the binary picture.
import cv2
import numpy as np
#STEP1
#Changing to Grayscale
picture = cv2.imread("Picture.jpg", cv2.IMREAD_GRAYSCALE)
#STEP2
# Apply threshold to get a binary picture
_, thresh = cv2.threshold(picture, 127, 255, cv2.THRESH_BINARY)
# Invert the binary picture (black background, white objects)
thresh = cv2.bitwise_not(thresh)
#STEP3
# Detect contours
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# Create an empty black picture to attract contours on
contour_image = np.zeros_like(picture, dtype=np.uint8)
# Draw contours in white shade with thickness 2
cv2.drawContours(contour_image, contours, -1, (255, 255, 255), 2)
# Save the contour picture
cv2.imwrite('contour.jpg', contour_image)
# Show the contour picture (optionally available)
cv2.imshow('Contours', contour_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Pattern Picture of Enter and Output of Code
Beneath is the instance picture enter and output of code:
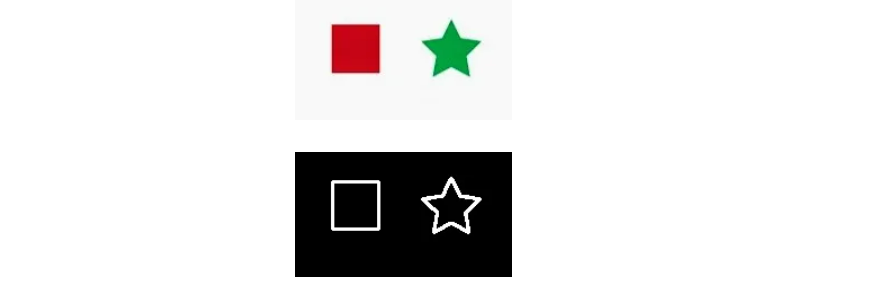
Parameters of discover Contours
The discover Contours operate has a number of parameters that affect its conduct and the outcomes it produces. Understanding these parameters is essential to successfully utilizing the operate.
Key Parameters
- Picture: The binary picture from which contours are to be discovered.
- Mode: The contour retrieval mode (e.g., cv2.RETR_EXTERNAL for retrieving solely the outer contours).
- Methodology: The contour approximation technique (e.g., cv2.CHAIN_APPROX_SIMPLE for a easy approximation).
Rationalization of Modes
- cv2.RETR_EXTERNAL: Retrieves solely the exterior contours.
- cv2.RETR_LIST: Retrieves all of the contours with none hierarchical relationships.
- cv2.RETR_CCOMP: Retrieves all of the contours and organizes them into two-level hierarchies.
- cv2.RETR_TREE: Retrieves all of the contours and reconstructs a full hierarchy of nested contours.
Rationalization of Strategies
- cv2.CHAIN_APPROX_NONE: Shops all of the factors of the contour.
- cv2.CHAIN_APPROX_SIMPLE: Removes all redundant factors and compresses the contour.
Sensible Purposes of Contours
Contours play an important function within the subject of laptop imaginative and prescient and picture processing, offering the foundational components for quite a few superior picture evaluation methods. They assist extract significant data from pictures and have a broad vary of functions, together with object detection, recognition, and form evaluation. On this part, we’ll discover sensible functions of contours, specializing in their significance in numerous fields and illustrating their makes use of by related examples.
Object Detection and Recognition
Contours play a pivotal function in object detection and recognition by figuring out the perimeters of objects inside a picture. That is important for applied sciences reminiscent of:
- Face Detection: Utilized in safety techniques and smartphones to establish and acknowledge faces.
- Handwritten Character Recognition: Converts handwritten textual content into digital format for functions like postal providers and digital note-taking.
- Object Identification in Complicated Scenes: Very important for autonomous automobiles and robotics to acknowledge and navigate by totally different objects.
Form Evaluation
Contours facilitate the evaluation and measurement of shapes, which is vital in numerous domains:
- Organic Analysis: Analyzes the shapes of organisms, reminiscent of plant leaves and animal cells, to categorise and examine them.
- Medical Imaging: Helps in delineating anatomical buildings in medical scans to diagnose and plan therapies.
- High quality Management in Manufacturing: Ensures that merchandise meet specified form and measurement standards, figuring out any defects or deviations.
Function Extraction and Object Classification
Use contours to extract options and classify objects based mostly on their form:
- Function Extraction: Identifies key factors and segments alongside an object’s contour to explain its traits.
- Form Descriptors: Supplies quantitative measures reminiscent of space and perimeter to check and classify totally different shapes and objects.
Sample Recognition and Matching
Contours help in recognizing and matching patterns inside or throughout pictures:
- Template Matching: Compares shapes in pictures to a template, utilized in industrial automation for sorting and figuring out objects.
- Gesture Recognition: Detects hand shapes and actions, enabling interplay with gadgets by gesture-based controls.
Conclusion
The Contours in OpenCV is an important device for anybody working in picture processing. It supplies a easy but highly effective solution to detect and analyze the shapes and limits of objects inside a picture. Whether or not you’re engaged on object detection, form evaluation, or picture segmentation, understanding how you can use contours successfully will enormously improve your capabilities in these areas.
By mastering the usage of contours and exploring superior methods, one can deal with a variety of advanced picture processing duties, making your functions extra strong and efficient. As we proceed to experiment and discover, we’ll discover much more artistic methods to leverage contours in initiatives.
Key Takeaways
- Understanding how Contours establish object shapes and limits in pictures for evaluation
- Implementing `discover Contours` to simplify picture information by detecting contours in pictures.
- Studying in-depth about parameters of `discover Contours` .
- Exploring Sensible Purposes of Contours and its use in the true world.
Continuously Requested Questions
findContours
operate in OpenCV?
A. The findContours operate detects and retrieves contours from a binary picture, serving to establish object boundaries.
A. Contours are curves that join steady factors alongside the boundary of an object with the identical shade or depth, representing object edges.
findContours
?
A. Key parameters embrace the picture (binary), mode (contour retrieval mode), and technique (contour approximation technique).
The media proven on this article isn’t owned by Analytics Vidhya and is used on the Writer’s discretion.
[ad_2]