Extracting Embedded Objects with LlamaParse
Introduction
LlamaParse is a doc parsing library developed by Llama Index to effectively and successfully parse paperwork equivalent to PDFs, PPTs, and so on.
Creating RAG functions on high of PDF paperwork presents a major problem many people face, particularly with the complicated process of parsing embedded objects equivalent to tables, figures, and so on. The character of those objects usually signifies that typical parsing methods battle to interpret and extract the knowledge encoded inside them precisely.
The software program growth neighborhood has launched numerous libraries and frameworks in response to this widespread subject. Examples of those options embody LLMSherpa and unstructured.io. These instruments present sturdy and versatile options to among the most persistent points when parsing complicated PDFs.
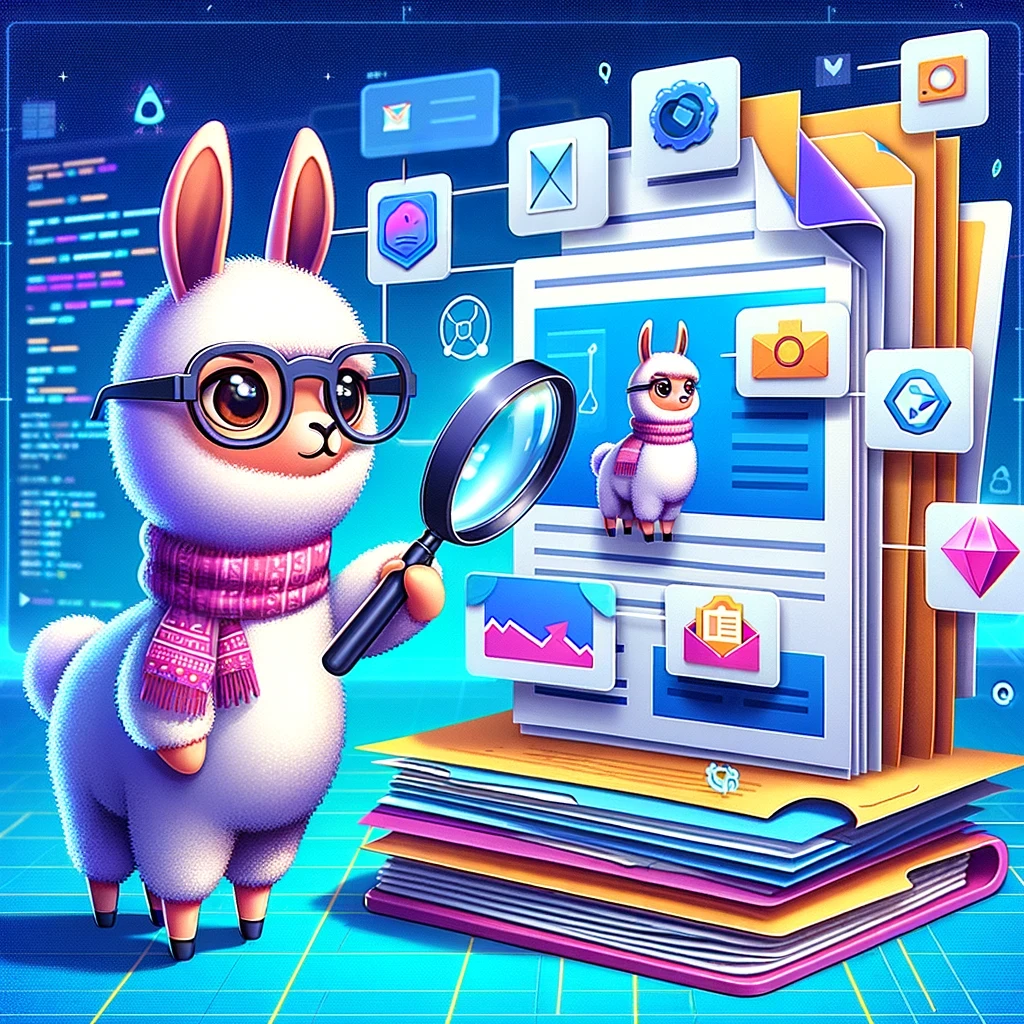
The newest addition to this listing of invaluable instruments is LlamaParse. LlamaParse was developed by Llama Index, one of the vital well-regarded LLM frameworks at present obtainable. Due to this, LlamaParse might be instantly built-in with the Llama Index. This seamless integration represents a major benefit, because it simplifies the implementation course of and ensures a better degree of compatibility between the 2 instruments. In conclusion, LlamaParse is a promising new device that makes parsing complicated PDFs much less daunting and extra environment friendly.
Studying Targets
- Acknowledge Doc Parsing Challenges: Perceive the difficulties in parsing complicated PDFs with embedded objects.
- Introduction to LlamaParse: Study what LlamaParse is and its seamless integration with Llama Index.
- Setup and Initialization: Create a LlamaCloud account, get hold of an API key, and set up the required libraries.
- Implementing LlamaParse: Comply with the steps to initialize the LLM, load, and parse paperwork.
- Making a Vector Index and Querying Knowledge: Study to create a vector retailer index, arrange a question engine, and extract particular data from parsed paperwork.
This text was revealed as part of the Knowledge Science Blogathon.
Steps to create a RAG utility on high of PDF utilizing LlamaParse
Step 1: Get the API key
LlamaParse is part of LlamaCloud platform, therefore it’s good to have a LlamaCloud account to get an api key.
First, you have to create an account on LlamaCloud and log in to create an API key.
Step 2: Set up the required libraries
Now open your Jupyter Pocket book/Colab and set up the required libraries. Right here, we solely want to put in two libraries: llama-index and llama-parse. We might be utilizing OpenAI’s mannequin for querying and embedding.
!pip set up llama-index
!pip set up llama-parse
Step 3: Set the surroundings variables
import os
os.environ['OPENAI_API_KEY'] = 'sk-proj-****'
os.environ["LLAMA_CLOUD_API_KEY"] = 'llx-****'
Step 4: Initialize the LLM and embedding mannequin
Right here, I’m utilizing gpt-3.5-turbo-0125 because the LLM and OpenAI’s text-embedding-3-small because the embedding mannequin. We’ll use the Settings module to switch the default LLM and the embedding mannequin.
from llama_index.llms.openai import OpenAI
from llama_index.embeddings.openai import OpenAIEmbedding
from llama_index.core import Settings
embed_model = OpenAIEmbedding(mannequin="text-embedding-3-small")
llm = OpenAI(mannequin="gpt-3.5-turbo-0125")
Settings.llm = llm
Settings.embed_model = embed_model
Step 5: Parse the Doc
Now, we are going to load our doc and convert it to the markdown sort. It’s then parsed utilizing MarkdownElementNodeParser.
The desk I used is taken from ncrb.gov.in and might be discovered right here: https://ncrb.gov.in/accidental-deaths-suicides-in-india-adsi. It has knowledge embedded at completely different ranges.
Beneath is the snapshot of the desk that i’m making an attempt to parse.
from llama_parse import LlamaParse
from llama_index.core.node_parser import MarkdownElementNodeParser
paperwork = LlamaParse(result_type="markdown").load_data("./Table_2021.pdf")
node_parser = MarkdownElementNodeParser(
llm=llm, num_workers=8
)
nodes = node_parser.get_nodes_from_documents(paperwork)
base_nodes, objects = node_parser.get_nodes_and_objects(nodes)
Step 6: Create the vector index and question engine
Now, we are going to create a vector retailer index utilizing the llama index’s built-in implementation to create a question engine on high of it. We are able to additionally use vector shops equivalent to chromadb, pinecone for this.
from llama_index.core import VectorStoreIndex
recursive_index = VectorStoreIndex(nodes=base_nodes + objects)
recursive_query_engine = recursive_index.as_query_engine(
similarity_top_k=5
)
Step 7: Querying the Index
question = 'Extract the desk as a dict and exclude any details about 2020. Additionally embody % var'
response = recursive_query_engine.question(question)
print(response)
The above consumer question will question the underlying vector index and return the embedded contents within the PDF doc in JSON format, as proven within the picture beneath.
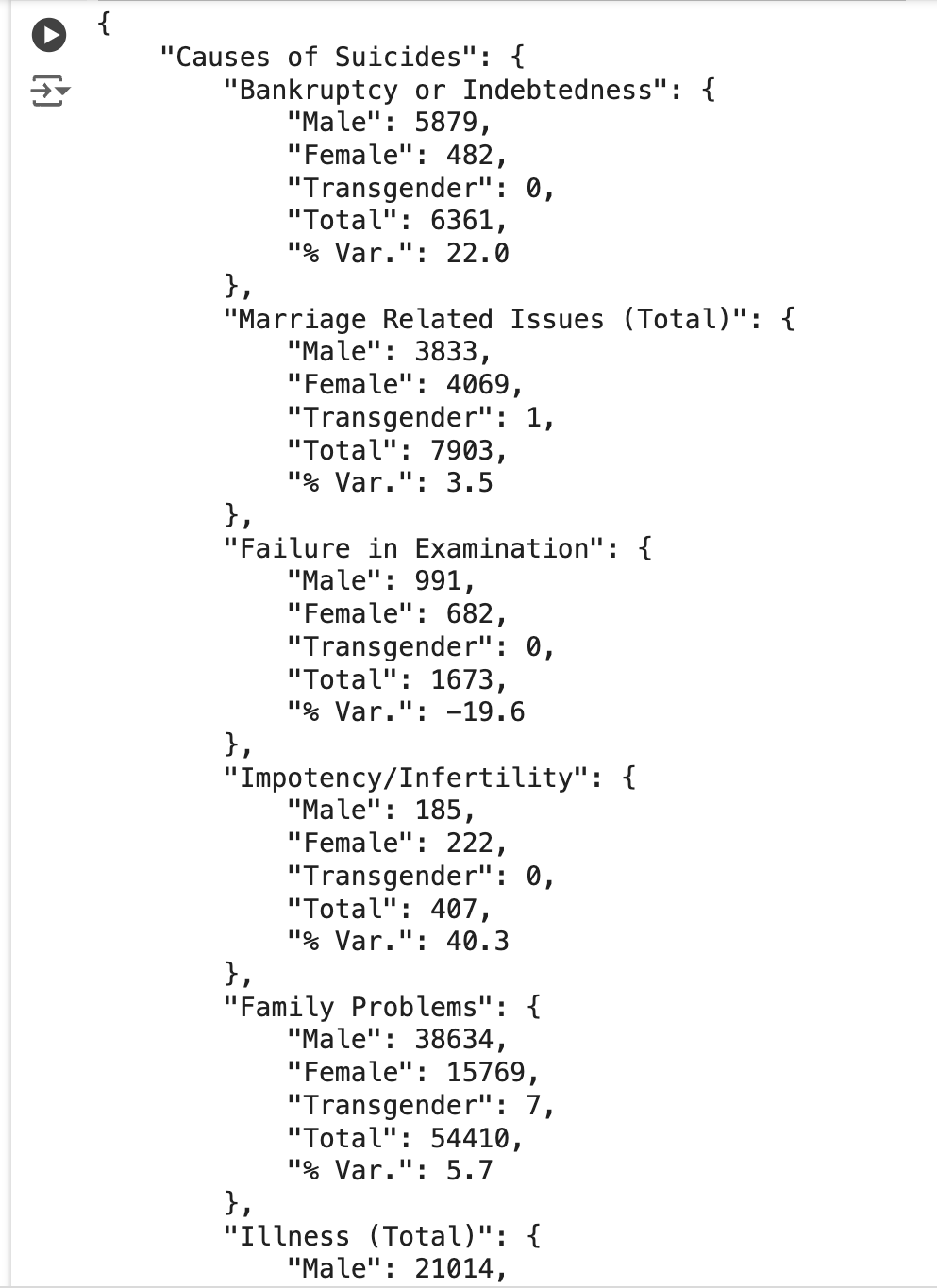
As you possibly can see within the screenshot, the desk was extracted in a clear JSON format.
Step 8: Placing all of it collectively
from llama_index.llms.openai import OpenAI
from llama_index.embeddings.openai import OpenAIEmbedding
from llama_index.core import Settings
from llama_parse import LlamaParse
from llama_index.core.node_parser import MarkdownElementNodeParser
from llama_index.core import VectorStoreIndex
embed_model = OpenAIEmbedding(mannequin="text-embedding-3-small")
llm = OpenAI(mannequin="gpt-3.5-turbo-0125")
Settings.llm = llm
Settings.embed_model = embed_model
paperwork = LlamaParse(result_type="markdown").load_data("./Table_2021.pdf")
node_parser = MarkdownElementNodeParser(
llm=llm, num_workers=8
)
nodes = node_parser.get_nodes_from_documents(paperwork)
base_nodes, objects = node_parser.get_nodes_and_objects(nodes)
recursive_index = VectorStoreIndex(nodes=base_nodes + objects)
recursive_query_engine = recursive_index.as_query_engine(
similarity_top_k=5
)
question = 'Extract the desk as a dict and exclude any details about 2020. Additionally embody % var'
response = recursive_query_engine.question(question)
print(response)
Conclusion
LlamaParse is an environment friendly device for extracting complicated objects from numerous doc sorts, equivalent to PDF recordsdata with few strains of code. Nonetheless, it is very important be aware {that a} sure degree of experience in working with LLM frameworks, such because the llama index, is required to make the most of this device absolutely.
LlamaParse proves invaluable in dealing with duties of various complexity. Nonetheless, like every other device within the tech discipline, it isn’t solely proof against errors. Due to this fact, performing a radical utility analysis is very really useful independently or leveraging obtainable analysis instruments. Analysis libraries, equivalent to Ragas, Truera, and so on., present metrics to evaluate the accuracy and reliability of your outcomes. This step ensures potential points are recognized and resolved earlier than the applying is pushed to a manufacturing surroundings.
Key Takeaways
- LlamaParse is a device created by the Llama Index workforce. It extracts complicated embedded objects from paperwork like PDFs with just some strains of code.
- LlamaParse affords each free and paid plans. The free plan means that you can parse as much as 1000 pages per day.
- LlamaParse at present helps 10+ file sorts (.pdf, .pptx, .docx, .html, .xml, and extra).
- LlamaParse is a part of the LlamaCloud platform, so that you want a LlamaCloud account to get an API key.
- With LlamaParse, you possibly can present directions in pure language to format the output. It even helps picture extraction.
The media proven on this article usually are not owned by Analytics Vidhya and is used on the Writer’s discretion.
Often requested questions(FAQ)
A. LlamaIndex is the main LLM framework, together with LangChain, for constructing LLM functions. It helps join customized knowledge sources to massive language fashions (LLMs) and is a extensively used device for constructing RAG functions.
A. LlamaParse is an providing from Llama Index that may extract complicated tables and figures from paperwork like PDF, PPT, and so on. Due to this, LlamaParse might be instantly built-in with the Llama Index, permitting us to make use of it together with the wide range of brokers and instruments that the Llama Index affords.
A. Llama Index is an LLM framework for constructing customized LLM functions and supplies numerous instruments and brokers. LlamaParse is specifically targeted on extracting complicated embedded objects from paperwork like PDF, PPT, and so on.
A. The significance of LlamaParse lies in its capacity to transform complicated unstructured knowledge into tables, pictures, and so on., right into a structured format, which is essential within the trendy world the place most precious data is obtainable in unstructured type. This transformation is important for analytics functions. As an illustration, finding out an organization’s financials from its SEC filings, which might span round 100-200 pages, could be difficult with out such a device. LlamaParse supplies an environment friendly strategy to deal with and construction this huge quantity of unstructured knowledge, making it extra accessible and helpful for evaluation.
A. Sure, LLMSherpa and unstructured.io are the alternate options obtainable to LlamaParse.