[ad_1]
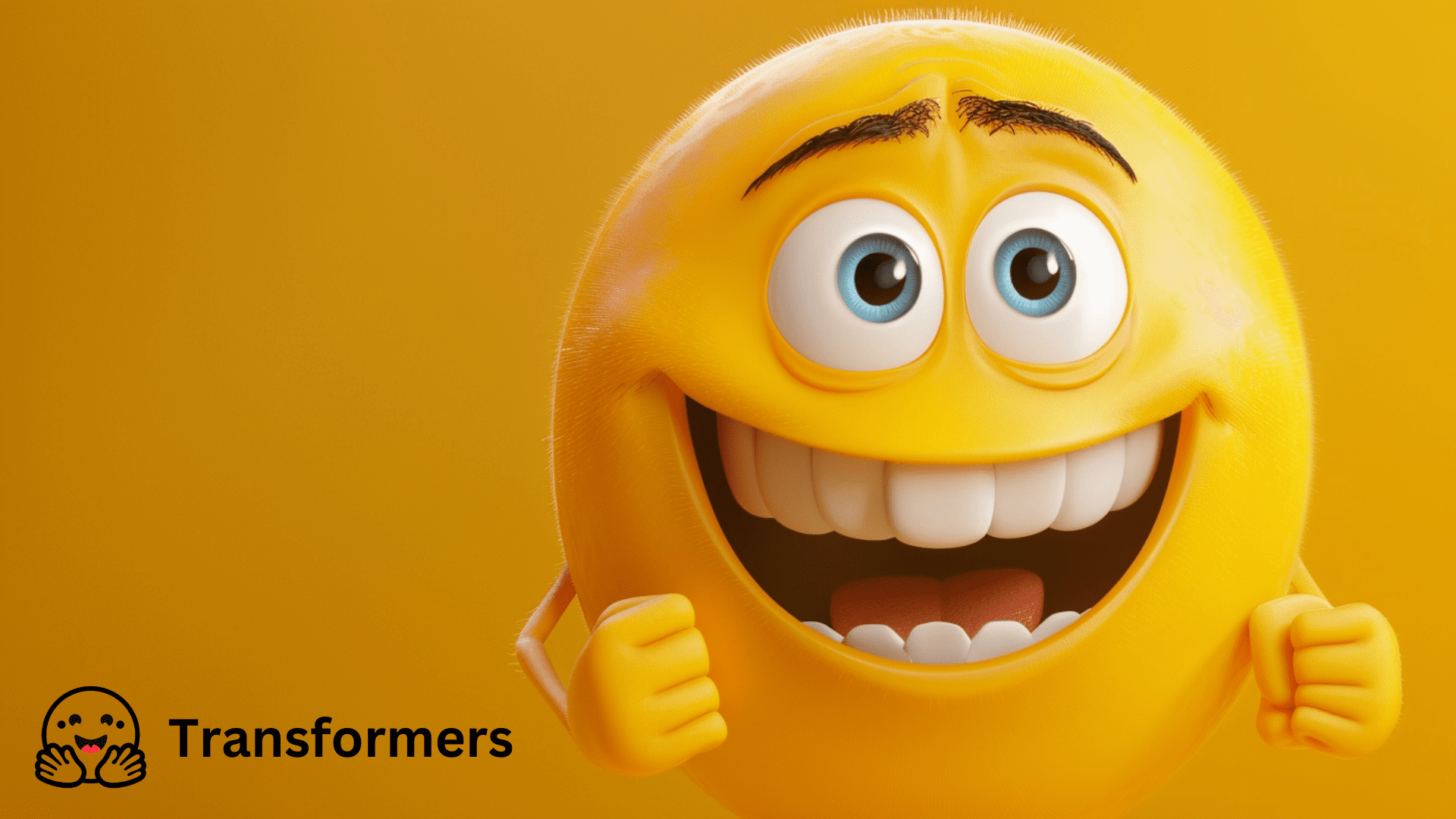
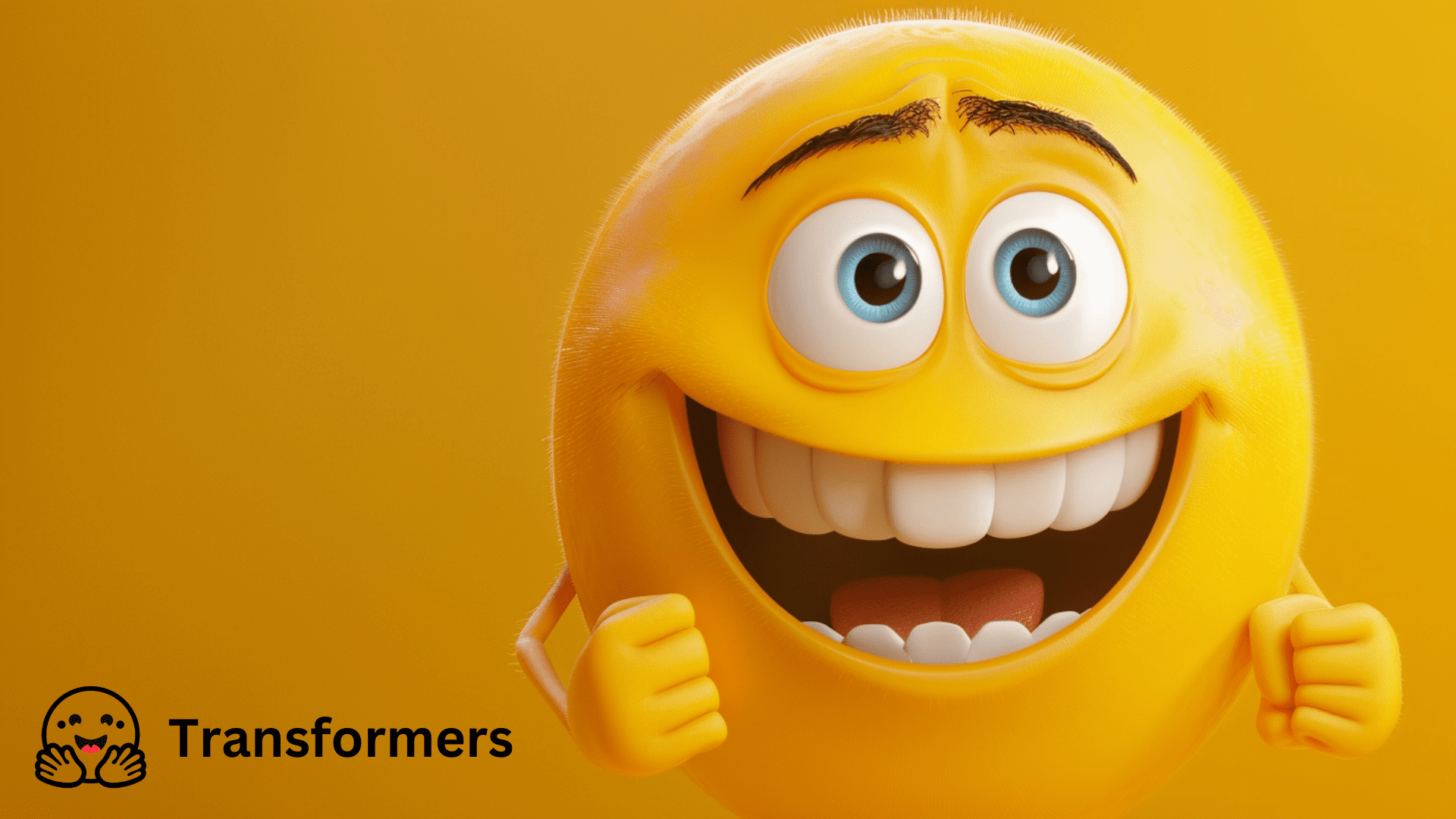
Picture created by Writer utilizing Midjourney
Introduction
Sentiment evaluation refers to pure language processing (NLP) methods which can be used to guage the sentiment expressed inside a physique of textual content and is an important expertise behind trendy functions of buyer suggestions evaluation, social media sentiment monitoring, and market analysis. Sentiment helps companies and different organizations assess public opinion, provide improved customer support, and increase their services or products.
BERT, which is brief for Bidirectional Encoder Representations from Transformers, is a language processing mannequin that, when initially launched, improved the cutting-edge of NLP by having an necessary understanding of phrases in context, surpassing prior fashions by a substantial margin. BERT’s bidirectionality — studying each the left and proper context of a given phrase — proved particularly priceless in use circumstances corresponding to sentiment evaluation.
All through this complete walk-through, you’ll discover ways to fine-tune BERT on your personal sentiment evaluation tasks, utilizing the Hugging Face Transformers library. Whether or not you’re a newcomer or an present NLP practitioner, we’re going to cowl quite a lot of sensible methods and issues in the middle of this step-by-step tutorial to make sure that you’re nicely outfitted to fine-tune BERT correctly on your personal functions.
Setting Up the Atmosphere
There are some vital stipulations that must be completed previous to fine-tuning our mannequin. Particularly, this may require Hugging Face Transformers, along with each PyTorch and Hugging Face’s datasets library at a minimal. You would possibly accomplish that as follows.
pip set up transformers torch datasets
And that is it.
Preprocessing the Knowledge
You will want to decide on some knowledge to be utilizing to coach up the textual content classifier. Right here, we’ll be working with the IMDb film assessment dataset, this being one of many locations used to show sentiment evaluation. Let’s go forward and cargo the dataset utilizing the datasets
library.
from datasets import load_dataset
dataset = load_dataset("imdb")
print(dataset)
We might want to tokenize our knowledge to organize it for pure language processing algorithms. BERT has a particular tokenization step which ensures that when a sentence fragment is reworked, it can keep as coherent for people as it could possibly. Let’s see how we are able to tokenize our knowledge through the use of BertTokenizer
from Transformers.
from transformers import BertTokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
def tokenize_function(examples):
return tokenizer(examples['text'], padding="max_length", truncation=True)
tokenized_datasets = dataset.map(tokenize_function, batched=True)
Making ready the Dataset
Let’s break up the dataset into coaching and validation units to judge the mannequin’s efficiency. Right here’s how we’ll accomplish that.
from datasets import train_test_split
train_testvalid = tokenized_datasets['train'].train_test_split(test_size=0.2)
train_dataset = train_testvalid['train']
valid_dataset = train_testvalid['test']
DataLoaders assist handle batches of information effectively throughout the coaching course of. Right here is how we’ll create DataLoaders for our coaching and validation datasets.
from torch.utils.knowledge import DataLoader
train_dataloader = DataLoader(train_dataset, shuffle=True, batch_size=8)
valid_dataloader = DataLoader(valid_dataset, batch_size=8)
Setting Up the BERT Mannequin for Nice-Tuning
We are going to use the BertForSequenceClassification
class for loading our mannequin, which has been pre-trained for sequence classification duties. That is how we’ll accomplish that.
from transformers import BertForSequenceClassification, AdamW
mannequin = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
Coaching the Mannequin
Coaching our mannequin entails defining the coaching loop, specifying a loss operate, an optimizer, and extra coaching arguments. Right here is how we are able to arrange and run the coaching loop.
from transformers import Coach, TrainingArguments
training_args = TrainingArguments(
output_dir="./outcomes",
evaluation_strategy="epoch",
learning_rate=2e-5,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
num_train_epochs=3,
weight_decay=0.01,
)
coach = Coach(
mannequin=mannequin,
args=training_args,
train_dataset=train_dataset,
eval_dataset=valid_dataset,
)
coach.practice()
Evaluating the Mannequin
Evaluating the mannequin entails checking its efficiency utilizing metrics corresponding to accuracy, precision, recall, and F1-score. Right here is how we are able to consider our mannequin.
metrics = coach.consider()
print(metrics)
Making Predictions
After fine-tuning, we at the moment are ready to make use of the mannequin for making predictions on new knowledge. That is how we are able to carry out inference with our mannequin on our validation set.
predictions = coach.predict(valid_dataset)
print(predictions)
Abstract
This tutorial has lined fine-tuning BERT for sentiment evaluation with Hugging Face Transformers, and included establishing the surroundings, dataset preparation and tokenization, DataLoader creation, mannequin loading, and coaching, in addition to mannequin analysis and real-time mannequin prediction.
Nice-tuning BERT for sentiment evaluation may be priceless in lots of real-world conditions, corresponding to analyzing buyer suggestions, monitoring social media tone, and rather more. Through the use of completely different datasets and fashions, you possibly can increase upon this on your personal pure language processing tasks.
For added info on these matters, try the next sources:
These sources are price investigating in an effort to dive extra deeply into these points and advance your pure language processing and sentiment evaluation skills.
Matthew Mayo (@mattmayo13) holds a Grasp’s diploma in pc science and a graduate diploma in knowledge mining. As Managing Editor, Matthew goals to make advanced knowledge science ideas accessible. His skilled pursuits embrace pure language processing, machine studying algorithms, and exploring rising AI. He’s pushed by a mission to democratize information within the knowledge science group. Matthew has been coding since he was 6 years outdated.
[ad_2]