[ad_1]
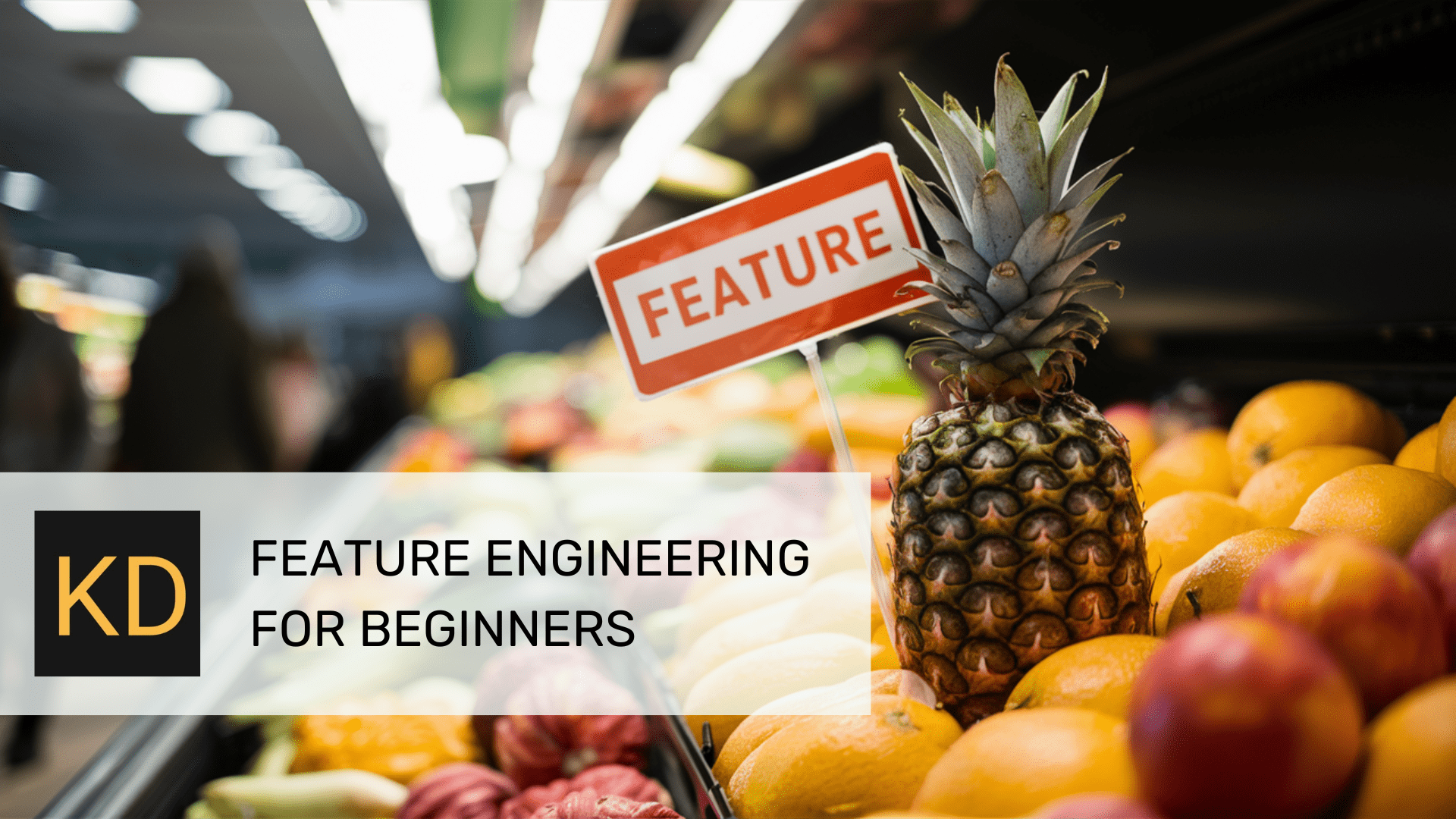
Picture created by Creator
Introduction
Function engineering is without doubt one of the most vital features of the machine studying pipeline. It’s the follow of making and modifying options, or variables, for the needs of enhancing mannequin efficiency. Nicely-designed options can rework weak fashions into robust ones, and it’s by way of function engineering that fashions can grow to be each extra sturdy and correct. Function engineering acts because the bridge between the dataset and the mannequin, giving the mannequin every little thing it must successfully clear up an issue.
This can be a information supposed for brand spanking new information scientists, information engineers, and machine studying practitioners. The target of this text is to speak basic function engineering ideas and supply a toolbox of methods that may be utilized to real-world eventualities. My goal is that, by the tip of this text, you can be armed with sufficient working data about function engineering to use it to your personal datasets to be fully-equipped to start creating highly effective machine studying fashions.
Understanding Options
Options are measurable traits of any phenomenon that we’re observing. They’re the granular parts that make up the information with which fashions function upon to make predictions. Examples of options can embody issues like age, revenue, a timestamp, longitude, worth, and virtually anything one can consider that may be measured or represented in some kind.
There are totally different function varieties, the primary ones being:
- Numerical Options: Steady or discrete numeric varieties (e.g. age, wage)
- Categorical Options: Qualitative values representing classes (e.g. gender, shoe dimension kind)
- Textual content Options: Phrases or strings of phrases (e.g. “this” or “that” or “even this”)
- Time Collection Options: Knowledge that’s ordered by time (e.g. inventory costs)
Options are essential in machine studying as a result of they instantly affect a mannequin’s skill to make predictions. Nicely-constructed options enhance mannequin efficiency, whereas unhealthy options make it tougher for a mannequin to supply robust predictions. Function choice and have engineering are preprocessing steps within the machine studying course of which might be used to organize the information to be used by studying algorithms.
A distinction is made between function choice and have engineering, although each are essential in their very own proper:
- Function Choice: The culling of vital options from all the set of all out there options, thus decreasing dimensionality and selling mannequin efficiency
- Function Engineering: The creation of recent options and subsequent altering of present ones, all in assistance from making a mannequin carry out higher
By choosing solely an important options, function choice helps to solely depart behind the sign within the information, whereas function engineering creates new options that assist to mannequin the result higher.
Primary Methods in Function Engineering
Whereas there are a variety of primary function engineering methods at our disposal, we are going to stroll by way of among the extra vital and well-used of those.
Dealing with Lacking Values
It is not uncommon for datasets to comprise lacking data. This may be detrimental to a mannequin’s efficiency, which is why it is very important implement methods for coping with lacking information. There are a handful of frequent strategies for rectifying this concern:
- Imply/Median Imputation: Filling lacking areas in a dataset with the imply or median of the column
- Mode Imputation: Filling lacking spots in a dataset with the most typical entry in the identical column
- Interpolation: Filling in lacking information with values of knowledge factors round it
These fill-in strategies must be utilized primarily based on the character of the information and the potential impact that the tactic might need on the tip mannequin.
Coping with lacking data is essential in holding the integrity of the dataset in tact. Right here is an instance Python code snippet that demonstrates numerous information filling strategies utilizing the pandas
library.
import pandas as pd
from sklearn.impute import SimpleImputer
# Pattern DataFrame
information = {'age': [25, 30, np.nan, 35, 40], 'wage': [50000, 60000, 55000, np.nan, 65000]}
df = pd.DataFrame(information)
# Fill in lacking ages utilizing the imply
mean_imputer = SimpleImputer(technique='imply')
df['age'] = mean_imputer.fit_transform(df[['age']])
# Fill within the lacking salaries utilizing the median
median_imputer = SimpleImputer(technique='median')
df['salary'] = median_imputer.fit_transform(df[['salary']])
print(df)
Encoding of Categorical Variables
Recalling that almost all machine studying algorithms are finest (or solely) outfitted to take care of numeric information, categorical variables should usually be mapped to numerical values to ensure that mentioned algorithms to higher interpret them. The commonest encoding schemes are the next:
- One-Sizzling Encoding: Producing separate columns for every class
- Label Encoding: Assigning an integer to every class
- Goal Encoding: Encoding classes by their particular person final result variable averages
The encoding of categorical information is important for planting the seeds of understanding in lots of machine studying fashions. The precise encoding methodology is one thing you’ll choose primarily based on the precise state of affairs, together with each the algorithm at use and the dataset.
Under is an instance Python script for the encoding of categorical options utilizing pandas
and parts of scikit-learn
.
import pandas as pd
from sklearn.preprocessing import OneHotEncoder, LabelEncoder
# Pattern DataFrame
information = {'colour': ['red', 'blue', 'green', 'blue', 'red']}
df = pd.DataFrame(information)
# Implementing one-hot encoding
one_hot_encoder = OneHotEncoder()
one_hot_encoding = one_hot_encoder.fit_transform(df[['color']]).toarray()
df_one_hot = pd.DataFrame(one_hot_encoding, columns=one_hot_encoder.get_feature_names_out(['color']))
# Implementing label encoding
label_encoder = LabelEncoder()
df['color_label'] = label_encoder.fit_transform(df['color'])
print(df)
print(df_one_hot)
Scaling and Normalizing Knowledge
For good efficiency of many machine studying strategies, scaling and normalization must be carried out in your information. There are a number of strategies for scaling and normalizing information, similar to:
- Standardization: Remodeling information in order that it has a imply of 0 and an ordinary deviation of 1
- Min-Max Scaling: Scaling information to a set vary, similar to [0, 1]
- Strong Scaling: Scaling excessive and low values iteratively by the median and interquartile vary, respectively
The scaling and normalization of knowledge is essential for making certain that function contributions are equitable. These strategies enable the various function values to contribute to a mannequin commensurately.
Under is an implementation, utilizing scikit-learn
, that exhibits the way to full information that has been scaled and normalized.
import pandas as pd
from sklearn.preprocessing import StandardScaler, MinMaxScaler, RobustScaler
# Pattern DataFrame
information = {'age': [25, 30, 35, 40, 45], 'wage': [50000, 60000, 55000, 65000, 70000]}
df = pd.DataFrame(information)
# Standardize information
scaler_standard = StandardScaler()
df['age_standard'] = scaler_standard.fit_transform(df[['age']])
# Min-Max Scaling
scaler_minmax = MinMaxScaler()
df['salary_minmax'] = scaler_minmax.fit_transform(df[['salary']])
# Strong Scaling
scaler_robust = RobustScaler()
df['salary_robust'] = scaler_robust.fit_transform(df[['salary']])
print(df)
The essential methods above together with the corresponding instance code present pragmatic options for lacking information, encoding categorical variables, and scaling and normalizing information utilizing powerhouse Python instruments pandas
and scikit-learn
. These methods could be built-in into your personal function engineering course of to enhance your machine studying fashions.
Superior Methods in Function Engineering
We now flip our consideration to to extra superior featured engineering methods, and embody some pattern Python code for implementing these ideas.
Function Creation
With function creation, new options are generated or modified to trend a mannequin with higher efficiency. Some methods for creating new options embody:
- Polynomial Options: Creation of higher-order options with present options to seize extra advanced relationships
- Interplay Phrases: Options generated by combining a number of options to derive interactions between them
- Area-Particular Function Era: Options designed primarily based on the intricacies of topics throughout the given drawback realm
Creating new options with tailored which means can enormously assist to spice up mannequin efficiency. The subsequent script showcases how function engineering can be utilized to carry latent relationships in information to gentle.
import pandas as pd
import numpy as np
# Pattern DataFrame
information = {'x1': [1, 2, 3, 4, 5], 'x2': [10, 20, 30, 40, 50]}
df = pd.DataFrame(information)
# Polynomial Options
df['x1_squared'] = df['x1'] ** 2
df['x1_x2_interaction'] = df['x1'] * df['x2']
print(df)
Dimensionality Discount
With the intention to simplify fashions and improve their efficiency, it may be helpful to downsize the variety of mannequin options. Dimensionality discount methods that may assist obtain this purpose embody:
- PCA (Principal Element Evaluation): Transformation of predictors into a brand new function set comprised of linearly impartial mannequin options
- t-SNE (t-Distributed Stochastic Neighbor Embedding): Dimension discount that’s used for visualization functions
- LDA (Linear Discriminant Evaluation): Discovering new mixtures of mannequin options which might be efficient for deconstructing totally different lessons
With the intention to shrink the scale of your dataset and preserve its relevancy, dimensional discount methods will assist. These methods had been devised to sort out the high-dimensional points associated to information, similar to overfitting and computational demand.
An indication of knowledge shrinking applied with scikit-learn
is proven subsequent.
import pandas as pd
from sklearn.decomposition import PCA
# Pattern DataFrame
information = {'feature1': [2.5, 0.5, 2.2, 1.9, 3.1], 'feature2': [2.4, 0.7, 2.9, 2.2, 3.0]}
df = pd.DataFrame(information)
# Use PCA for Dimensionality Discount
pca = PCA(n_components=1)
df_pca = pca.fit_transform(df)
df_pca = pd.DataFrame(df_pca, columns=['principal_component'])
print(df_pca)
Time Collection Function Engineering
With time-based datasets, particular function engineering methods should be used, similar to:
- Lag Options: Former information factors are used to derive mannequin predictive options
- Rolling Statistics: Knowledge statistics are calculated throughout information home windows, similar to rolling means
- Seasonal Decomposition: Knowledge is partitioned into sign, pattern, and random noise classes
Temporal fashions want various augmentation in comparison with direct mannequin becoming. These strategies observe temporal dependence and patterns to make the predictive mannequin sharper.
An indication of time sequence options augmenting utilized utilizing pandas
is proven subsequent as nicely.
import pandas as pd
import numpy as np
# Pattern DataFrame
date_rng = pd.date_range(begin="1/1/2022", finish='1/10/2022', freq='D')
information = {'date': date_rng, 'worth': [100, 110, 105, 115, 120, 125, 130, 135, 140, 145]}
df = pd.DataFrame(information)
df.set_index('date', inplace=True)
# Lag Options
df['value_lag1'] = df['value'].shift(1)
# Rolling Statistics
df['value_rolling_mean'] = df['value'].rolling(window=3).imply()
print(df)
The above examples show sensible purposes of superior function engineering methods, by way of utilization of pandas
and scikit-learn
. By using these strategies you possibly can improve the predictive energy of your mannequin.
Sensible Suggestions and Greatest Practices
Listed below are a couple of easy however vital suggestions to bear in mind whereas working by way of your function engineering course of.
- Iteration: Function engineering is a trial-and-error course of, and you’re going to get higher with it every time you iterate. Take a look at totally different function engineering concepts to search out the very best set of options.
- Area Data: Make the most of experience from those that know the subject material nicely when creating options. Generally refined relationships could be captured with realm-specific data.
- Validation and Understanding of Options: By understanding which options are most vital to your mode, you might be outfitted to make vital choices. Instruments for figuring out function significance embody:
- SHAP (SHapley Additive exPlanations): Serving to to quantify the contribution of every function in predictions
- LIME (Native Interpretable Mannequin-agnostic Explanations): Showcasing the which means of mannequin predictions in plain language
An optimum mixture of complexity and interpretability is important for having each good and easy to digest outcomes.
Conclusion
This brief information has addressed basic function engineering ideas, in addition to primary and superior methods, and sensible suggestions and finest practices. What many would take into account among the most vital function engineering practices — coping with lacking data, encoding of categorical information, scaling information, and creation of recent options — had been lined.
Function engineering is a follow that turns into higher with execution, and I hope you could have been capable of take one thing away with you which will enhance your information science abilities. I encourage you to use these methods to your personal work and to be taught out of your experiences.
Do not forget that, whereas the precise proportion varies relying on who tells it, a majority of any machine studying undertaking is spent within the information preparation and preprocessing section. Function engineering is part of this prolonged section, and as such must be considered with the import that it calls for. Studying to see function engineering what it’s — a serving to hand within the modeling course of — ought to make it extra digestible to newcomers.
Completely satisfied engineering!
Matthew Mayo (@mattmayo13) holds a Grasp’s diploma in laptop science and a graduate diploma in information mining. As Managing Editor, Matthew goals to make advanced information science ideas accessible. His skilled pursuits embody pure language processing, machine studying algorithms, and exploring rising AI. He’s pushed by a mission to democratize data within the information science group. Matthew has been coding since he was 6 years outdated.
[ad_2]