Gantt Charts utilizing Python
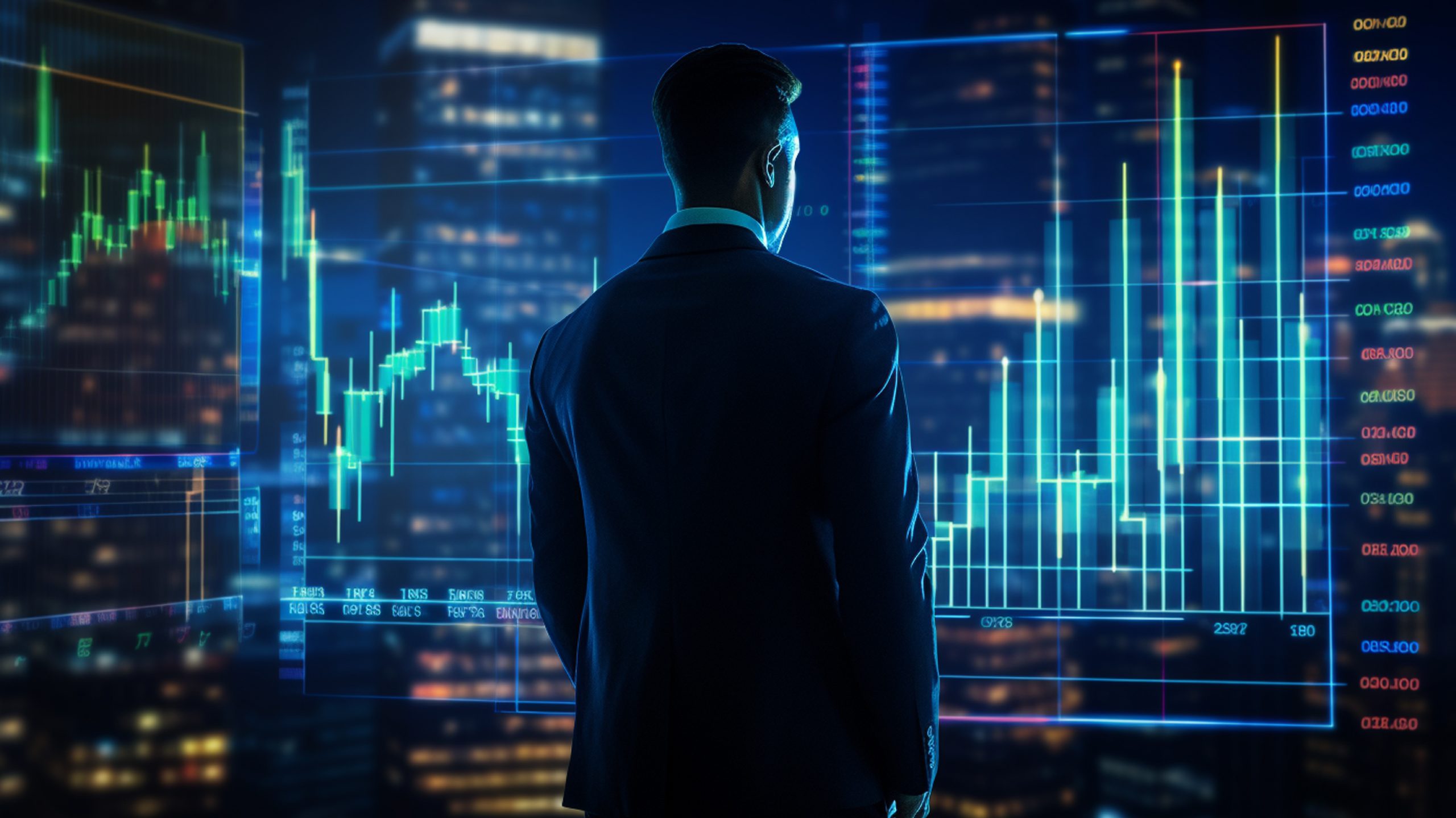
Introduction
This text describes Gantt charts and emphasizes how necessary they’re for creating mission schedules. It additionally reveals how they will present timeframes, dependencies, and duties. It gives detailed directions for making Gantt charts with Python’s matplotlib and plotly instruments. Additionally demonstrating the best way to use them to effectively and successfully show mission timelines.
Overview
- Find out about Gantt charts, their components, and the importance of mission administration with them.
- Be taught how to use the Python matplotlib library to construct and modify Gantt charts.
- Develop abilities to create interactive and visually interesting Gantt charts utilizing the plotly library.
- Be taught to determine and visualize job dependencies and mission timelines successfully utilizing Gantt charts.
Gantt Charts in Python
Gantt chart – your go-to device for mapping out mission schedules and protecting your workforce on monitor! This versatile visualizer, named after Henry L. Gantt is all about exhibiting duties and their dependencies in a transparent and concise means.
A typical Gantt chart options horizontal bars that characterize particular person duties or occasions, with begin and finish dates that offer you a birds-eye view of the mission timeline. It’s like having a roadmap to your mission’s success! With this chart, you possibly can:
- Spot relationships between duties.
- Establish dependencies.
- Create a practical schedule that retains your mission on tempo.
With assistance from these efficient instruments, you possibly can create charts which might be simple for everybody to understand and that look improbable.
Creating Gantt Charts utilizing Matplotlib
Let’s now take a look at the best way to use the matplotlib library in Python to provide Gantt charts.
import matplotlib.pyplot as plt
import pandas as pd
import matplotlib.dates as mdates
# Outline the info
df = pd.DataFrame([
dict(Task="Job A", Start="2009-01-01", Finish="2009-02-28"),
dict(Task="Job B", Start="2009-03-05", Finish="2009-04-15"),
dict(Task="Job C", Start="2009-02-20", Finish="2009-05-30")
])
# Convert Begin and End to datetime
df['Start'] = pd.to_datetime(df['Start'])
df['Finish'] = pd.to_datetime(df['Finish'])
# Calculate the length
df['Duration'] = df['Finish'] - df['Start']
# Create the determine and axis
fig, ax = plt.subplots()
# Create bars for every job
for i, row in df.iterrows():
ax.barh(row['Task'], row['Duration'].days, left=row['Start'])
# Format the x-axis as dates
ax.xaxis_date()
ax.xaxis.set_major_locator(mdates.MonthLocator())
ax.xaxis.set_major_formatter(mdates.DateFormatter('%Y-%m-%d'))
# Set labels
ax.set_xlabel('Date')
ax.set_ylabel('Activity')
ax.set_title('Gantt Chart')
# Invert the y-axis to have the duties listed from prime to backside
ax.invert_yaxis()
# Rotate date labels for higher readability
plt.xticks(rotation=45)
# Present the plot
plt.tight_layout()
plt.present()
Output:
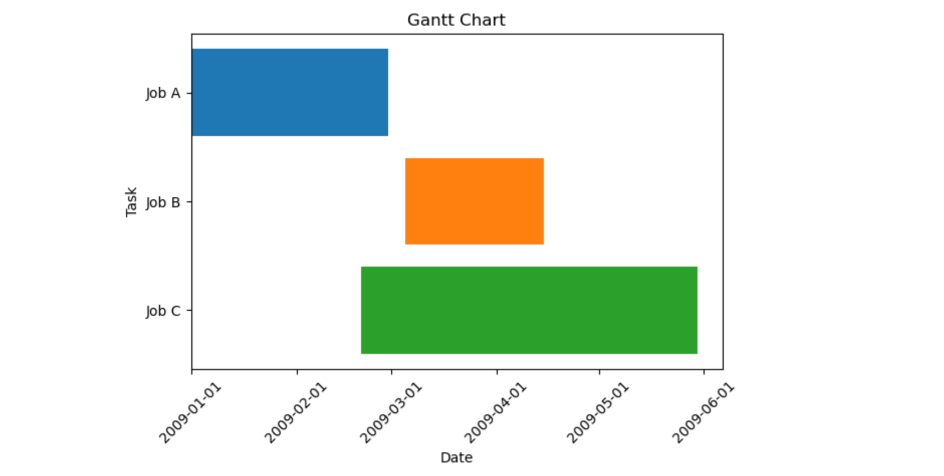
Right here, matplotlib’s `subplots` operate is used to create a determine and an axis.
The determine will comprise the Gantt chart. A loop iterates over every row within the DataFrame, making a horizontal bar (h-bar) for every job utilizing Matplotlib’s `barh` operate. The peak of every bar is about to the length of the duty, and the left edge of every bar is about to the beginning date of the duty.
Word: The time length of every job goes from begin to end.
Creating Gantt Chart utilizing Plotly
Let’s now take a look at the best way to use Plotly library in Python to provide Gantt charts.
import plotly.figure_factory as ff
df = [dict(Task="Job A", Start="2009-01-01", Finish="2009-02-28"),
dict(Task="Job B", Start="2009-03-05", Finish="2009-04-15"),
dict(Task="Job C", Start="2009-02-20", Finish="2009-05-30")]
fig = ff.create_gantt(df)
fig.present()
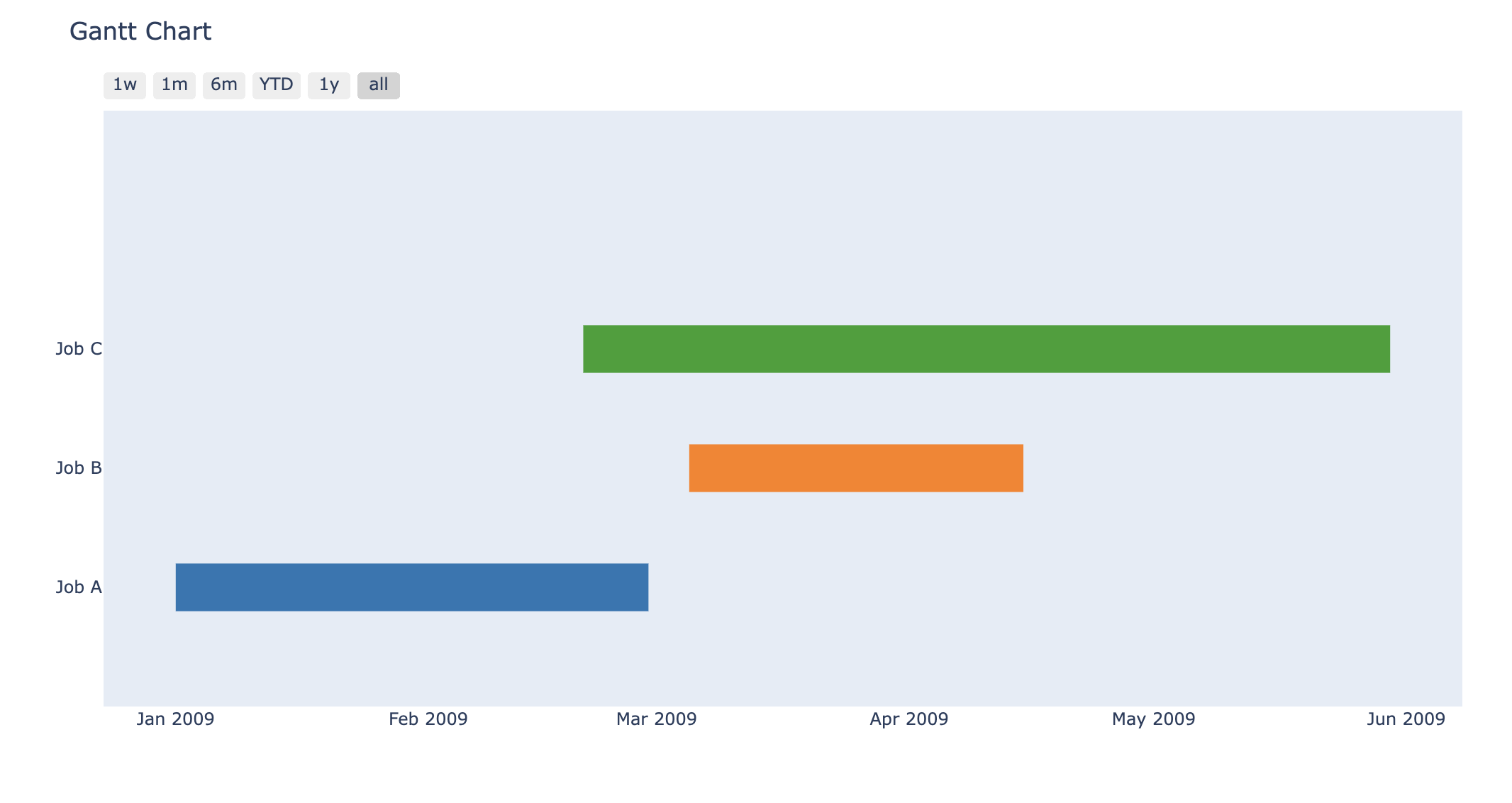
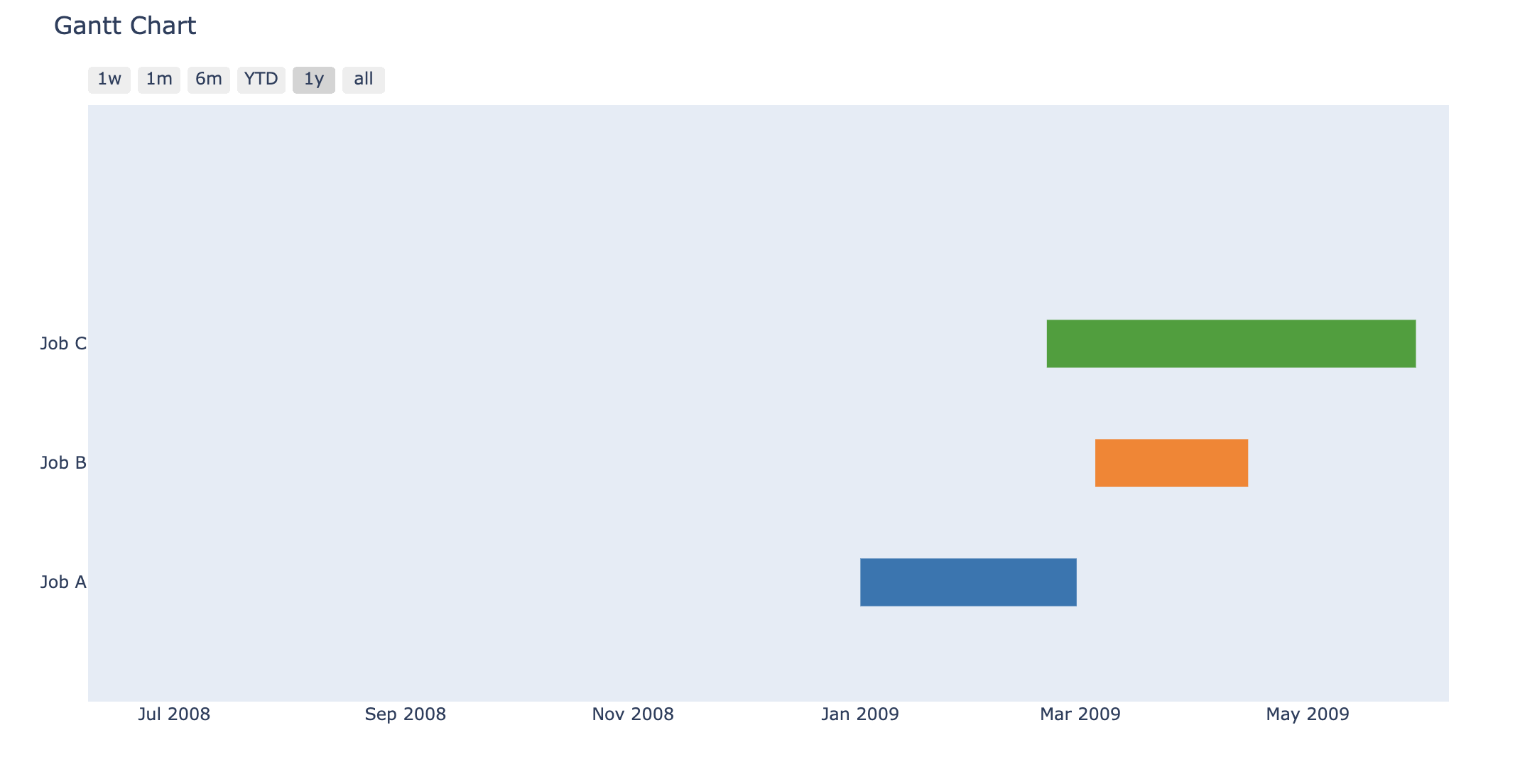
As you possibly can see ‘plotly’ gives a pleasant implementation of Gantt charts with a lot easier syntax and customization of the timeframe. The charts generated by plotly are additionally downloadable.
Conclusion
Gantt charts are extremely helpful instruments for displaying job progress, dependencies, and mission schedules. It’s possible you’ll make extremely configurable and interactive Gantt charts that enhance mission planning and monitoring by using Python packages equivalent to matplotlib and plotly. On this article we explored creating Gantt charts in Python.
Ceaselessly Requested Questions
A. Gantt charts present the sequence, length, and dependencies of actions with every work represented by a horizontal bar and displayed on a timeline. They’re visible mission administration instruments that facilitate understanding.
A. You possibly can create Gantt charts in Python utilizing libraries like matplotlib and plotly. Matplotlib gives in depth customization choices and is nice for static charts, whereas plotly gives interactive charts with an easier syntax, permitting for simple updates and information visualization.
A. Plotly has a number of advantages, equivalent to simply customizable and updatable charts, simple use, and interactive and aesthetically pleasing charts. Plotly charts are versatile for a variety of use instances since they are often downloaded and included into on-line apps.