[ad_1]
Introduction
Immediate engineering has grow to be pivotal in leveraging Giant Language fashions (LLMs) for numerous functions. As you all know, fundamental immediate engineering covers basic methods. Nonetheless, advancing to extra subtle strategies permits us to create extremely efficient, context-aware, and sturdy language fashions. This text will delve into a number of superior immediate engineering methods utilizing LangChain. I’ve added code examples and sensible insights for builders.
In superior immediate engineering, we craft complicated prompts and use LangChain’s capabilities to construct clever, context-aware functions. This consists of dynamic prompting, context-aware prompts, meta-prompting, and utilizing reminiscence to keep up state throughout interactions. These methods can considerably improve the efficiency and reliability of LLM-powered functions.
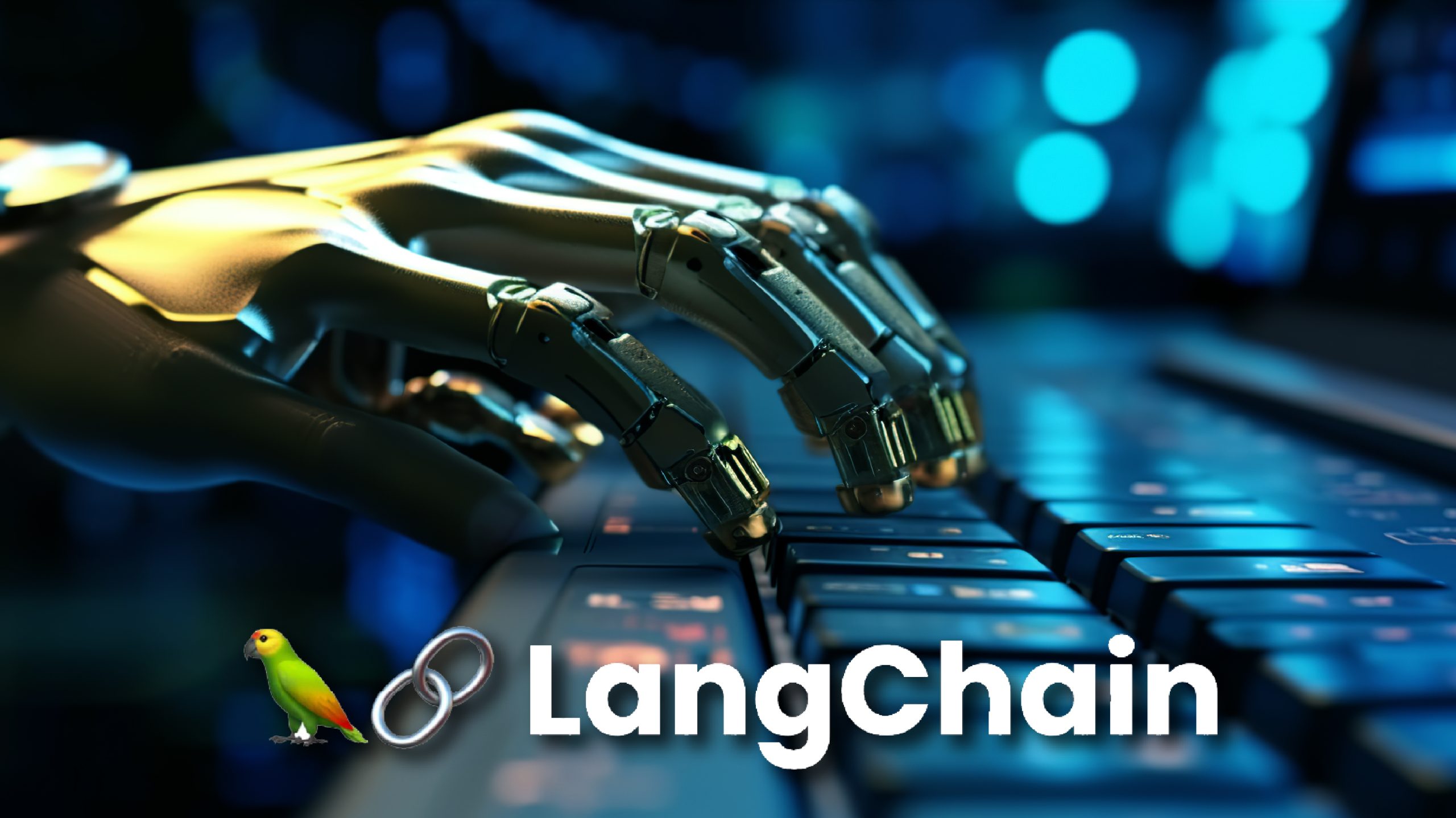
Studying Aims
- Study to create multi-step prompts that information the mannequin by way of complicated reasoning and workflows.
- Discover superior immediate engineering methods to regulate prompts based mostly on real-time context and person interactions for adaptive functions.
- Develop prompts that evolve with the dialog or job to keep up relevance and coherence.
- Generate and refine prompts autonomously utilizing the mannequin’s inner state and suggestions mechanisms.
- Implement reminiscence mechanisms to keep up context and knowledge throughout interactions for coherent functions.
- Use superior immediate engineering in real-world functions like schooling, help, artistic writing, and analysis.
This text was printed as part of the Information Science Blogathon.
Setting Up LangChain
Ensure you arrange LangChain accurately. A strong setup and familiarity with the framework are essential for superior functions. I hope you all know the right way to arrange LangChain in Python.
Set up
First, set up LangChain utilizing pip:
pip set up langchain
Fundamental setup
from langchain import LangChain
from langchain.fashions import OpenAI
# Initialize the LangChain framework
lc = LangChain()
# Initialize the OpenAI mannequin
mannequin = OpenAI(api_key='your_openai_api_key')
Superior Immediate Structuring
Superior immediate structuring is a complicated model that goes past easy directions or contextual prompts. It includes creating multi-step prompts that information the mannequin by way of logical steps. This method is important for duties that require detailed explanations, step-by-step reasoning, or complicated workflows. By breaking the duty into smaller, manageable parts, superior immediate structuring can assist improve the mannequin’s potential to generate coherent, correct, and contextually related responses.
Purposes of Superior Immediate Structuring
- Academic Instruments: Superior immediate engineering instruments can create detailed academic content material, resembling step-by-step tutorials, complete explanations of complicated subjects, and interactive studying modules.
- Technical Help:It could assist present detailed technical help, troubleshooting steps, and diagnostic procedures for varied techniques and functions.
- Artistic Writing: In artistic domains, superior immediate engineering can assist generate intricate story plots, character developments, and thematic explorations by guiding the mannequin by way of a collection of narrative-building steps.
- Analysis Help: For analysis functions, structured prompts can help in literature critiques, knowledge evaluation, and the synthesis of knowledge from a number of sources, guaranteeing a radical and systematic means.
Key Parts of Superior Immediate Structuring
Listed below are superior immediate engineering structuring:
- Step-by-Step Directions: By offering the mannequin with a transparent sequence of steps to comply with, we are able to considerably enhance the standard of its output. That is notably helpful for problem-solving, procedural explanations, and detailed descriptions. Every step ought to construct logically on the earlier one, guiding the mannequin by way of a structured thought course of.
- Intermediate Targets: To assist make sure the mannequin stays on monitor, we are able to set intermediate objectives or checkpoints throughout the immediate. These objectives act as mini-prompts inside the principle immediate, permitting the mannequin to concentrate on one facet of the duty at a time. This method will be notably efficient in duties that contain a number of phases or require the mixing of assorted items of knowledge.
- Contextual Hints and Clues: Incorporating contextual hints and clues throughout the immediate can assist the mannequin perceive the broader context of the duty. Examples embody offering background info, defining key phrases, or outlining the anticipated format of the response. Contextual clues be certain that the mannequin’s output is aligned with the person’s expectations and the particular necessities of the duty.
- Position Specification: Defining a particular position for the mannequin can improve its efficiency. As an illustration, asking the mannequin to behave as an skilled in a specific area (e.g., a mathematician, a historian, a medical physician) can assist tailor its responses to the anticipated stage of experience and elegance. Position specification can enhance the mannequin’s potential to undertake totally different personas and adapt its language accordingly.
- Iterative Refinement: Superior immediate structuring typically includes an iterative course of the place the preliminary immediate is refined based mostly on the mannequin’s responses. This suggestions loop permits builders to fine-tune the immediate, making changes to enhance readability, coherence, and accuracy. Iterative refinement is essential for optimizing complicated prompts and attaining the specified output.
Instance: Multi-Step Reasoning
immediate = """
You might be an skilled mathematician. Remedy the next drawback step-by-step:
Downside: If a automobile travels at a velocity of 60 km/h for two hours, how far does it journey?
Step 1: Establish the system to make use of.
Components: Distance = Pace * Time
Step 2: Substitute the values into the system.
Calculation: Distance = 60 km/h * 2 hours
Step 3: Carry out the multiplication.
Outcome: Distance = 120 km
Reply: The automobile travels 120 km.
"""
response = mannequin.generate(immediate)
print(response)
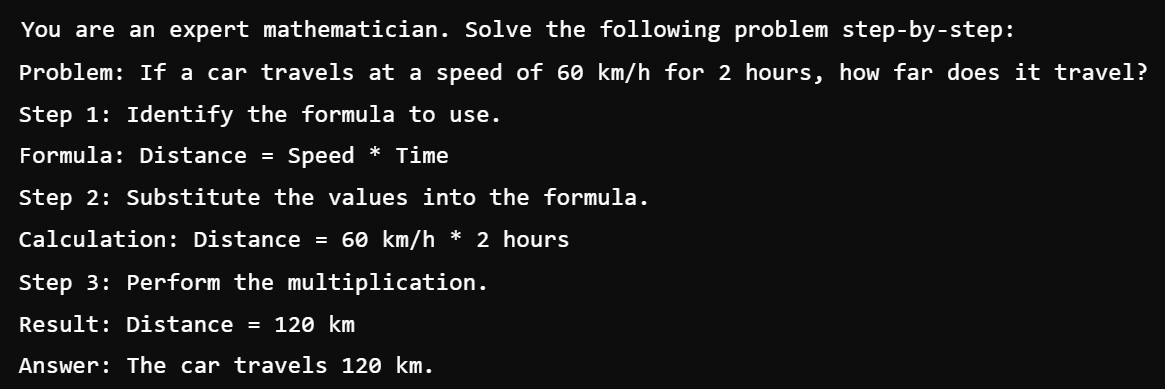
Dynamic Prompting
In Dynamic prompting, we modify the immediate based mostly on the context or earlier interactions, enabling extra adaptive and responsive interactions with the language mannequin. In contrast to static prompts, which stay mounted all through the interplay, dynamic prompts can evolve based mostly on the evolving dialog or the particular necessities of the duty at hand. This flexibility in Dynamic prompting permits builders to create extra participating, contextually related, and customized experiences for customers interacting with language fashions.
Purposes of Dynamic Prompting
- Conversational Brokers: Dynamic prompting is important for constructing conversational brokers that may interact in pure, contextually related dialogues with customers, offering customized help and knowledge retrieval.
- Interactive Studying Environments: In academic sectors, dynamic prompting can improve interactive studying environments by adapting the educational content material to the learner’s progress and preferences and may present tailor-made suggestions and help.
- Data Retrieval Programs: Dynamic prompting can enhance the effectiveness of knowledge retrieval techniques by dynamically adjusting and updating the search queries based mostly on the person’s context and preferences, resulting in extra correct and related search outcomes.
- Customized Suggestions: Dynamic prompting can energy customized suggestion techniques by dynamically producing prompts based mostly on person preferences and searching historical past. This technique suggests related content material and merchandise to customers based mostly on their pursuits and previous interactions.
Strategies for Dynamic Prompting
- Contextual Question Growth: This includes increasing the preliminary immediate with extra context gathered from the continuing dialog or the person’s enter. This expanded immediate provides the mannequin a richer understanding of the present context, enabling extra knowledgeable and related responses.
- Consumer Intent Recognition: By analyzing the person’s intent and extracting the important thing info from their queries, builders can dynamically generate prompts that tackle the particular wants and necessities expressed by the person. This can make sure the mannequin’s responses are tailor-made to the person’s intentions, resulting in extra satisfying interactions.
- Adaptive Immediate Technology: Dynamic prompting can even generate prompts on the fly based mostly on the mannequin’s inner state and the present dialog historical past. These dynamically generated prompts can information the mannequin in the direction of producing coherent responses that align with the continuing dialogue and the person’s expectations.
- Immediate Refinement by way of Suggestions: By including suggestions mechanisms into the prompting course of, builders can refine the immediate based mostly on the mannequin’s responses and the person’s suggestions. This iterative suggestions loop allows steady enchancment and adaptation, resulting in extra correct and efficient interactions over time.
Instance: Dynamic FAQ Generator
faqs = {
"What's LangChain?": "LangChain is a framework for constructing functions powered by giant language fashions.",
"How do I set up LangChain?": "You may set up LangChain utilizing pip: `pip set up langchain`."
}
def generate_prompt(query):
return f"""
You're a educated assistant. Reply the next query:
Query: {query}
"""
for query in faqs:
immediate = generate_prompt(query)
response = mannequin.generate(immediate)
print(f"Query: {query}nAnswer: {response}n")
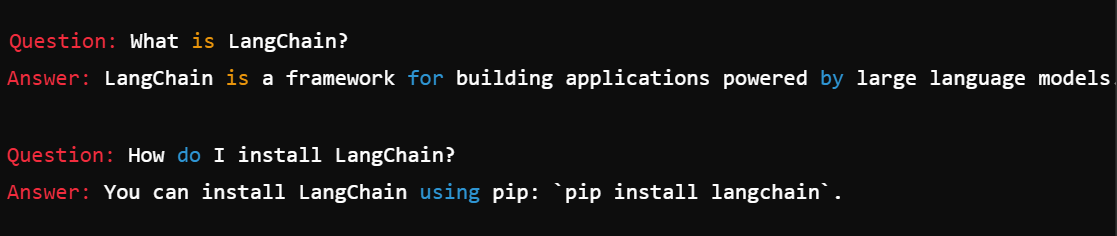
Context-Conscious Prompts
Context-aware prompts signify a classy method to participating with language fashions. It includes the immediate to dynamically modify based mostly on the context of the dialog or the duty at hand. In contrast to static prompts, which stay mounted all through the interplay, context-aware prompts evolve and adapt in actual time, enabling extra nuanced and related interactions with the mannequin. This method leverages the contextual info throughout the interplay to information the mannequin’s responses. It helps in producing output that’s coherent, correct, and aligned with the person’s expectations.
Purposes of Context-Conscious Prompts
- Conversational Assistants: Context-aware prompts are important for constructing conversational assistants to have interaction in pure, contextually related dialogues with customers, offering customized help and knowledge retrieval.
- Activity-Oriented Dialog Programs: In task-oriented dialog techniques, context-aware prompts allow the mannequin to know and reply to person queries within the context of the particular job or area and information the dialog towards attaining the specified purpose.
- Interactive Storytelling: Context-aware prompts can improve interactive storytelling experiences by adapting the narrative based mostly on the person’s selections and actions, guaranteeing a customized and immersive storytelling expertise.
- Buyer Help Programs: Context-aware prompts can enhance the effectiveness of buyer help techniques by tailoring the responses to the person’s question and historic interactions, offering related and useful help.
Strategies for Context-Conscious Prompts
- Contextual Data Integration: Context-aware prompts take contextual info from the continuing dialog, together with earlier messages, person intent, and related exterior knowledge sources. This contextual info enriches the immediate, giving the mannequin a deeper understanding of the dialog’s context and enabling extra knowledgeable responses.
- Contextual Immediate Growth: Context-aware prompts dynamically increase and adapt based mostly on the evolving dialog, including new info and adjusting the immediate’s construction as wanted. This flexibility permits the immediate to stay related and responsive all through the interplay and guides the mannequin towards producing coherent and contextually applicable responses.
- Contextual Immediate Refinement: Because the dialog progresses, context-aware prompts might bear iterative refinement based mostly on suggestions from the mannequin’s responses and the person’s enter. This iterative course of permits builders to constantly modify and optimize the immediate to make sure that it precisely captures the evolving context of the dialog.
- Multi-Flip Context Retention: Context-aware prompts preserve a reminiscence of earlier interactions after which add this historic context to the immediate. This permits the mannequin to generate coherent responses with the continuing dialogue and supply a dialog that’s extra up to date and coherent than a message.
Instance: Contextual Dialog
dialog = [
"User: Hi, who won the 2020 US presidential election?",
"AI: Joe Biden won the 2020 US presidential election.",
"User: What were his major campaign promises?"
]
context = "n".be part of(dialog)
immediate = f"""
Proceed the dialog based mostly on the next context:
{context}
AI:
"""
response = mannequin.generate(immediate)
print(response)
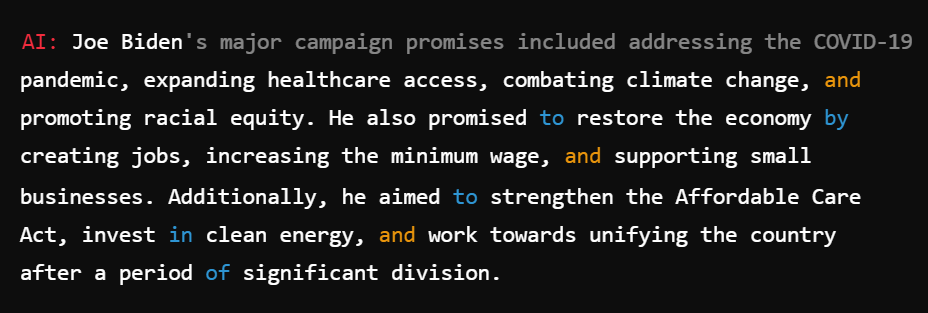
Meta-prompting is used to boost the sophistication and flexibility of language fashions. In contrast to typical prompts, which offer express directions or queries to the mannequin, meta-prompts function at the next stage of abstraction, which guides the mannequin in producing or refining prompts autonomously. This meta-level steerage empowers the mannequin to regulate its prompting technique dynamically based mostly on the duty necessities, person interactions, and inner state. It ends in fostering a extra agile and responsive dialog.
Purposes of Meta-Prompting
- Adaptive Immediate Engineering: Meta-prompting allows the mannequin to regulate its prompting technique dynamically based mostly on the duty necessities and the person’s enter, resulting in extra adaptive and contextually related interactions.
- Artistic Immediate Technology: Meta-prompting explores immediate areas, enabling the mannequin to generate numerous and revolutionary prompts. It evokes new heights of thought and expression.
- Activity-Particular Immediate Technology: Meta-prompting allows the technology of prompts tailor-made to particular duties or domains, guaranteeing that the mannequin’s responses align with the person’s intentions and the duty’s necessities.
- Autonomous Immediate Refinement: Meta-prompting permits the mannequin to refine prompts autonomously based mostly on suggestions and expertise. This helps the mannequin constantly enhance and refine its prompting technique.
Additionally learn: Immediate Engineering: Definition, Examples, Suggestions & Extra
Strategies for Meta-Prompting
- Immediate Technology by Instance: Meta-prompting can contain producing prompts based mostly on examples supplied by the person from the duty context. By analyzing these examples, the mannequin identifies comparable patterns and buildings that inform the technology of latest prompts tailor-made to the duty’s particular necessities.
- Immediate Refinement by way of Suggestions: Meta-prompting permits the mannequin to refine prompts iteratively based mostly on suggestions from its personal responses and the person’s enter. This suggestions loop permits the mannequin to study from its errors and modify its prompting technique to enhance the standard of its output over time.
- Immediate Technology from Activity Descriptions: Meta-prompting can present pure language understanding methods to extract key info from job descriptions or person queries and use this info to generate prompts tailor-made to the duty at hand. This ensures that the generated prompts are aligned with the person’s intentions and the particular necessities of the duty.
- Immediate Technology based mostly on Mannequin State: Meta-prompting generates prompts by taking account of the interior state of the mannequin, together with its data base, reminiscence, and inference capabilities. This occurs by leveraging the mannequin’s present data and reasoning skills. This enables the mannequin to generate contextually related prompts and align with its present state of understanding.
Instance: Producing Prompts for a Activity
task_description = "Summarize the important thing factors of a information article."
meta_prompt = f"""
You might be an skilled in immediate engineering. Create a immediate for the next job:
Activity: {task_description}
Immediate:
"""
response = mannequin.generate(meta_prompt)
print(response)
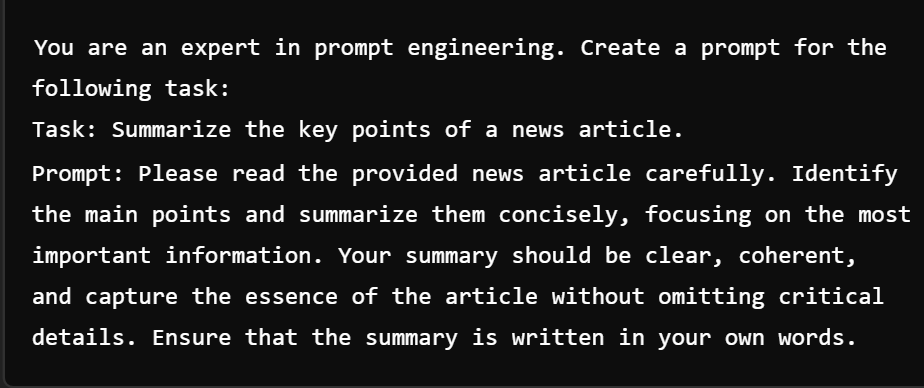
Leveraging Reminiscence and State
Leveraging reminiscence and state inside language fashions allows the mannequin to retain context and knowledge throughout interactions, which helps empower language fashions to exhibit extra human-like behaviors, resembling sustaining conversational context, monitoring dialogue historical past, and adapting responses based mostly on earlier interactions. By including reminiscence and state mechanisms into the prompting course of, builders can create extra coherent, context-aware, and responsive interactions with language fashions.
Purposes of Leveraging Reminiscence and State
- Contextual Conversational Brokers: Reminiscence and state mechanisms allow language fashions to behave as context-aware conversational brokers, sustaining context throughout interactions and producing responses which might be coherent with the continuing dialogue.
- Customized Suggestions: On this, language fashions can present customized suggestions tailor-made to the person’s preferences and previous interactions, bettering the relevance and effectiveness of advice techniques.
- Adaptive Studying Environments: It could improve interactive studying environments by monitoring learners’ progress and adapting the educational content material based mostly on their wants and studying trajectory.
- Dynamic Activity Execution: Language fashions can execute complicated duties over a number of interactions whereas coordinating their actions and responses based mostly on the duty’s evolving context.
Strategies for Leveraging Reminiscence and State
- Dialog Historical past Monitoring: Language fashions can preserve a reminiscence of earlier messages exchanged throughout a dialog, which permits them to retain context and monitor the dialogue historical past. By referencing this dialog historical past, fashions can generate extra coherent and contextually related responses that construct upon earlier interactions.
- Contextual Reminiscence Integration: Reminiscence mechanisms will be built-in into the prompting course of to offer the mannequin with entry to related contextual info. This helps builders in guiding the mannequin’s responses based mostly on its previous experiences and interactions.
- Stateful Immediate Technology: State administration methods permit language fashions to keep up an inner state that evolves all through the interplay. Builders can tailor the prompting technique to the mannequin’s inner context to make sure the generated prompts align with its present data and understanding.
- Dynamic State Replace: Language fashions can replace their inner state dynamically based mostly on new info obtained throughout the interplay. Right here, the mannequin constantly updates its state in response to person inputs and mannequin outputs, adapting its conduct in real-time and bettering its potential to generate contextually related responses.
Instance: Sustaining State in Conversations
from langchain.reminiscence import ConversationBufferMemory
reminiscence = ConversationBufferMemory()
dialog = [
"User: What's the weather like today?",
"AI: The weather is sunny with a high of 25°C.",
"User: Should I take an umbrella?"
]
for message in dialog:
reminiscence.add_message(message)
immediate = f"""
Proceed the dialog based mostly on the next context:
{reminiscence.get_memory()}
AI:
"""
response = mannequin.generate(immediate)
print(response)
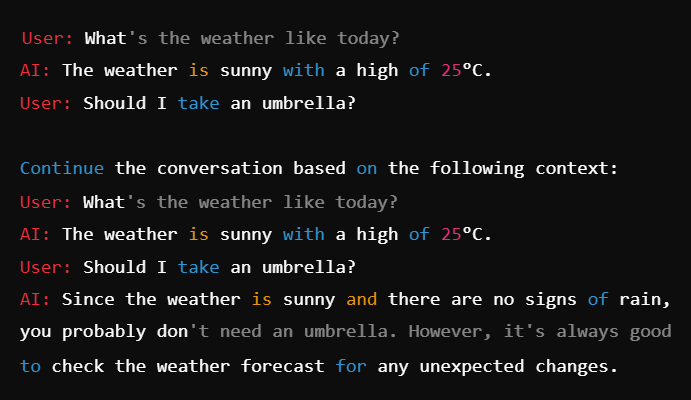
Sensible Examples
Instance 1: Superior Textual content Summarization
Utilizing dynamic and context-aware prompting to summarize complicated paperwork.
#importdocument = """
LangChain is a framework that simplifies the method of constructing functions utilizing giant language fashions. It offers instruments to create efficient prompts and combine with varied APIs and knowledge sources. LangChain permits builders to construct functions which might be extra environment friendly and scalable.
"""
immediate = f"""
Summarize the next doc:
{doc}
Abstract:
"""
response = mannequin.generate(immediate)
print(response)
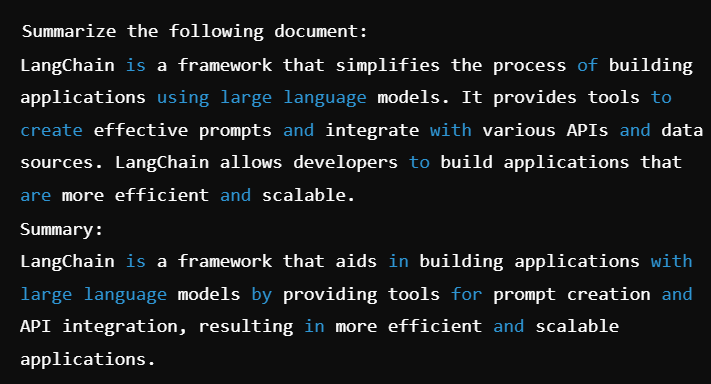
Instance 2: Complicated Query Answering
Combining multi-step reasoning and context-aware prompts for detailed Q&A.
query = "Clarify the idea of relativity."
immediate = f"""
You're a physicist. Clarify the idea of relativity in easy phrases.
Query: {query}
Reply:
"""
response = mannequin.generate(immediate)
print(response)
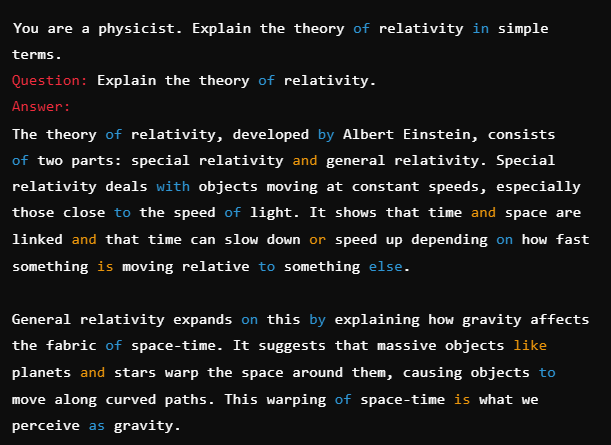
Conclusion
Superior immediate engineering with LangChain helps builders to construct sturdy, context-aware functions that leverage the complete potential of enormous language fashions. Steady experimentation and refinement of prompts are important for attaining optimum outcomes.
For complete knowledge administration options, discover YData Material. For instruments to profile datasets, think about using ydata-profiling. To generate artificial knowledge with preserved statistical properties, take a look at ydata-synthetic.
Key Takeaways
- Superior Immediate Engineering Structuring: Guides mannequin by way of multi-step reasoning with contextual cues.
- Dynamic Prompting: Adjusts prompts based mostly on real-time context and person interactions.
- Context-Conscious Prompts: Evolves prompts to keep up relevance and coherence with dialog context.
- Meta-Prompting: Generates and refines prompts autonomously, leveraging the mannequin’s capabilities.
- Leveraging Reminiscence and State: Maintains context and knowledge throughout interactions for coherent responses.
The media proven on this article aren’t owned by Analytics Vidhya and is used on the Writer’s discretion.
Continuously Requested Questions
A. LangChain can combine with APIs and knowledge sources to dynamically modify prompts based mostly on real-time person enter or exterior knowledge. You may create extremely adaptive and context-aware interactions by programmatically setting up prompts incorporating this info.
A. LangChain offers reminiscence administration capabilities that let you retailer and retrieve context throughout a number of interactions, important for creating conversational brokers that bear in mind person preferences and previous interactions.
A. Dealing with ambiguous or unclear queries requires designing prompts that information the mannequin in searching for clarification or offering context-aware responses. Greatest practices embody:
a. Explicitly Asking for Clarification: Immediate the mannequin to ask follow-up questions.
b. Offering A number of Interpretations: Design prompts permit the mannequin to current totally different interpretations.
A. Meta-prompting leverages the mannequin’s personal capabilities to generate or refine prompts, enhancing the general utility efficiency. This may be notably helpful for creating adaptive techniques that optimize conduct based mostly on suggestions and efficiency metrics.
A. Integrating LangChain with present machine studying fashions and workflows includes utilizing its versatile API to mix outputs from varied fashions and knowledge sources, making a cohesive system that leverages the strengths of a number of parts.
[ad_2]