[ad_1]
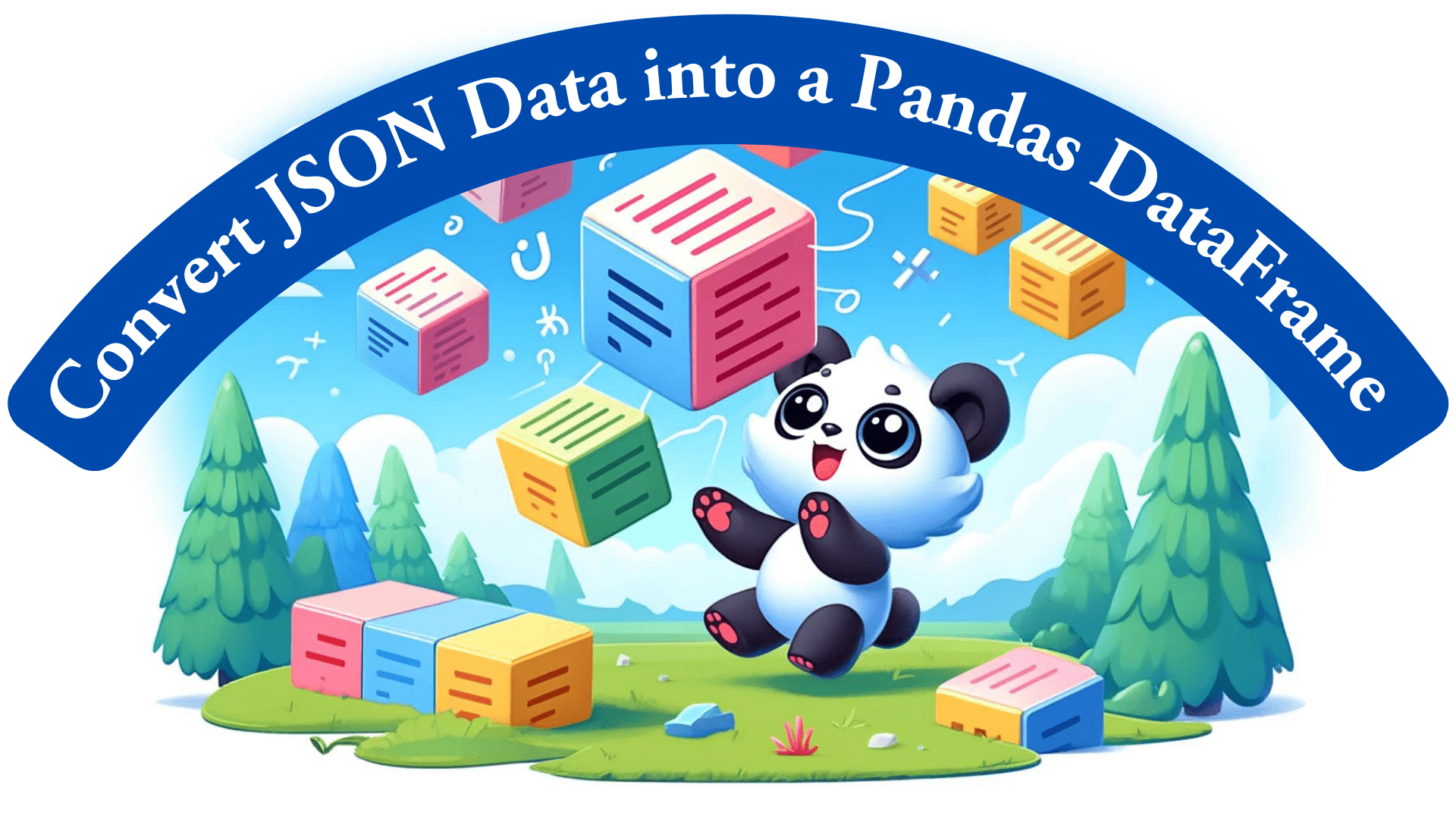
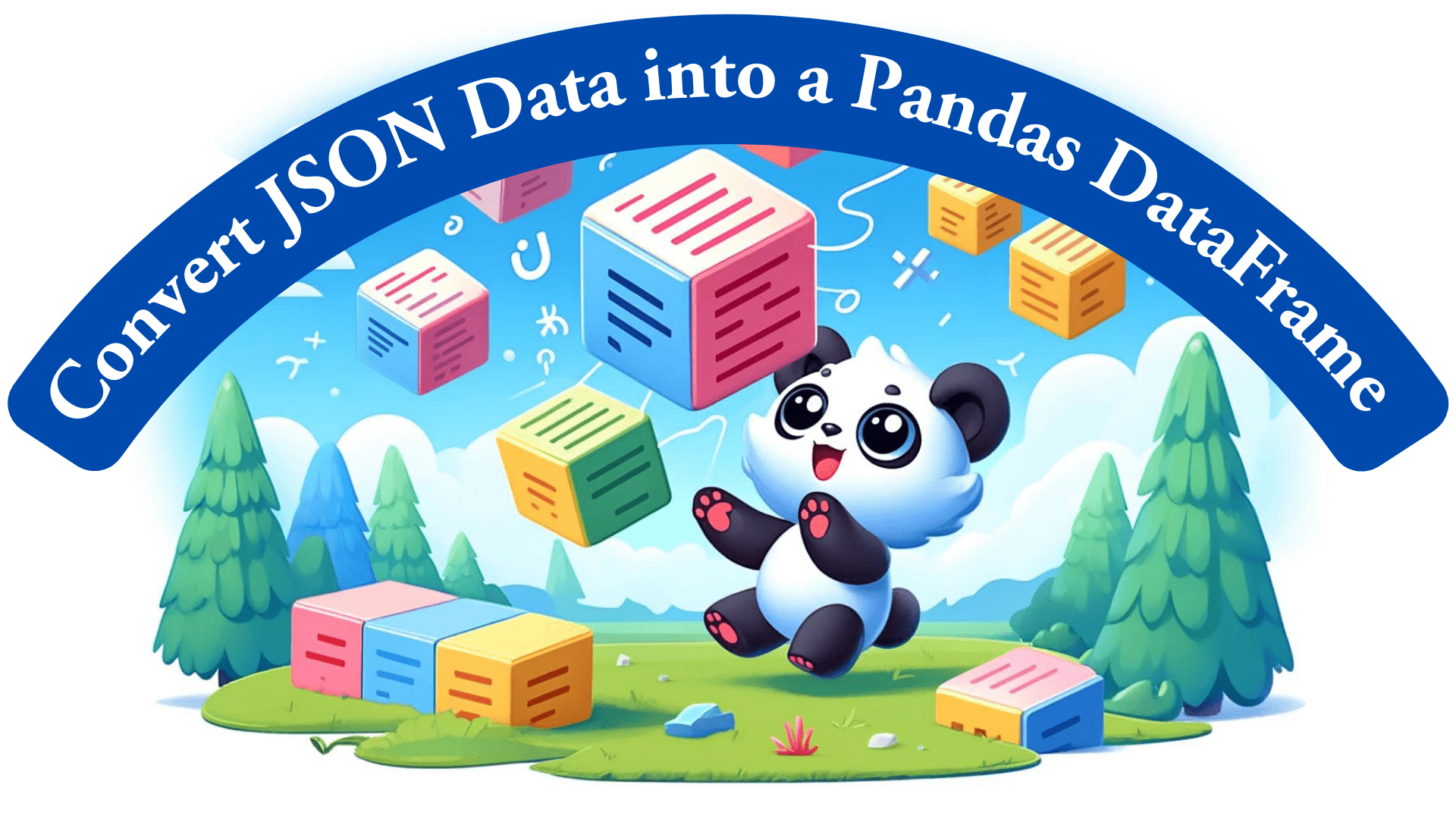
Picture by Creator | DALLE-3 & Canva
If you happen to’ve ever had the possibility to work with knowledge, you’ve got most likely come throughout the necessity to load JSON recordsdata (quick for JavaScript Object Notation) right into a Pandas DataFrame for additional evaluation. JSON recordsdata retailer knowledge in a format that’s clear for individuals to learn and likewise easy for computer systems to know. Nevertheless, JSON recordsdata can typically be difficult to navigate by way of. Subsequently, we load them right into a extra structured format like DataFrames – that’s arrange like a spreadsheet with rows and columns.
I’ll present you two other ways to transform JSON knowledge right into a Pandas DataFrame. Earlier than we talk about these strategies, let’s suppose this dummy nested JSON file that I will use for instance all through this text.
{
"books": [
{
"title": "One Hundred Years of Solitude",
"author": "Gabriel Garcia Marquez",
"reviews": [
{
"reviewer": {
"name": "Kanwal Mehreen",
"location": "Islamabad, Pakistan"
},
"rating": 4.5,
"comments": "Magical and completely breathtaking!"
},
{
"reviewer": {
"name": "Isabella Martinez",
"location": "Bogotá, Colombia"
},
"rating": 4.7,
"comments": "A marvelous journey through a world of magic."
}
]
},
{
"title": "Issues Fall Aside",
"writer": "Chinua Achebe",
"critiques": [
{
"reviewer": {
"name": "Zara Khan",
"location": "Lagos, Nigeria"
},
"rating": 4.9,
"comments": "Things Fall Apart is the best of contemporary African literature."
}]}]}
The above-mentioned JSON knowledge represents an inventory of books, the place every e-book has a title, writer, and an inventory of critiques. Every evaluation, in flip, has a reviewer (with a reputation and site) and a ranking and feedback.
Methodology 1: Utilizing the json.load()
and pd.DataFrame()
features
The best and most simple method is to make use of the built-in json.load()
perform to parse our JSON knowledge. It will convert it right into a Python dictionary, and we will then create the DataFrame straight from the ensuing Python knowledge construction. Nevertheless, it has an issue – it will possibly solely deal with single nested knowledge. So, for the above case, in case you solely use these steps with this code:
import json
import pandas as pd
#Load the JSON knowledge
with open('books.json','r') as f:
knowledge = json.load(f)
#Create a DataFrame from the JSON knowledge
df = pd.DataFrame(knowledge['books'])
df
Your output may seem like this:
Output:
Within the critiques column, you may see your complete dictionary. Subsequently, if you need the output to seem appropriately, you need to manually deal with the nested construction. This may be performed as follows:
#Create a DataFrame from the nested JSON knowledge
df = pd.DataFrame([
{
'title': book['title'],
'writer': e-book['author'],
'reviewer_name': evaluation['reviewer']['name'],
'reviewer_location': evaluation['reviewer']['location'],
'ranking': evaluation['rating'],
'feedback': evaluation['comments']
}
for e-book in knowledge['books']
for evaluation in e-book['reviews']
])
Up to date Output:
Right here, we’re utilizing checklist comprehension to create a flat checklist of dictionaries, the place every dictionary incorporates the e-book data and the corresponding evaluation. We then create the Pandas DataFrae utilizing this.
Nevertheless the difficulty with this method is that it calls for extra handbook effort to handle the nested construction of the JSON knowledge. So, what now? Do we’ve got another choice?
Completely! I imply, come on. Provided that we’re within the twenty first century, dealing with such an issue and not using a answer appears unrealistic. Let’s examine the opposite method.
Methodology 2 (Really helpful): Utilizing the json_normalize()
perform
The json_normalize()
perform from the Pandas library is a greater option to handle nested JSON knowledge. It robotically flattens the nested construction of the JSON knowledge, making a DataFrame from the ensuing knowledge. Let’s check out the code:
import pandas as pd
import json
#Load the JSON knowledge
with open('books.json', 'r') as f:
knowledge = json.load(f)
#Create the DataFrame utilizing json_normalize()
df = pd.json_normalize(
knowledge=knowledge['books'],
meta=['title', 'author'],
record_path="critiques",
errors="increase"
)
df
Output:
The json_normalize()
perform takes the next parameters:
- knowledge: The enter knowledge, which is usually a checklist of dictionaries or a single dictionary. On this case, it is the info dictionary loaded from the JSON file.
- record_path: The trail within the JSON knowledge to the data you wish to normalize. On this case, it is the ‘critiques’ key.
- meta: Extra fields to incorporate within the normalized output from the JSON doc. On this case, we’re utilizing the ‘title’ and ‘writer’ fields. Notice that columns in metadata normally seem on the finish. That is how this perform works. So far as the evaluation is anxious, it would not matter, however for some magical cause, you need these columns to seem earlier than. Sorry, however you need to do them manually.
- errors: The error dealing with technique, which will be ‘ignore’, ‘increase’, or ‘warn’. We have now set it to ‘increase’, so if there are any errors in the course of the normalization course of, it can increase an exception.
Wrapping Up
Each of those strategies have their very own benefits and use instances, and the selection of methodology is determined by the construction and complexity of the JSON knowledge. If the JSON knowledge has a really nested construction, the json_normalize()
perform is likely to be the best option, as it will possibly deal with the nested knowledge robotically. If the JSON knowledge is comparatively easy and flat, the pd.read_json()
perform is likely to be the simplest and most simple method.
When coping with massive JSON recordsdata, it is essential to consider reminiscence utilization and efficiency since loading the entire file into reminiscence won’t work. So, you might need to look into different choices like streaming the info, lazy loading, or utilizing a extra memory-efficient format like Parquet.
Kanwal Mehreen Kanwal is a machine studying engineer and a technical author with a profound ardour for knowledge science and the intersection of AI with medication. She co-authored the e-book “Maximizing Productiveness with ChatGPT”. As a Google Era Scholar 2022 for APAC, she champions variety and educational excellence. She’s additionally acknowledged as a Teradata Variety in Tech Scholar, Mitacs Globalink Analysis Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having based FEMCodes to empower girls in STEM fields.
[ad_2]