How To Create Minimal Docker Photographs for Python Functions
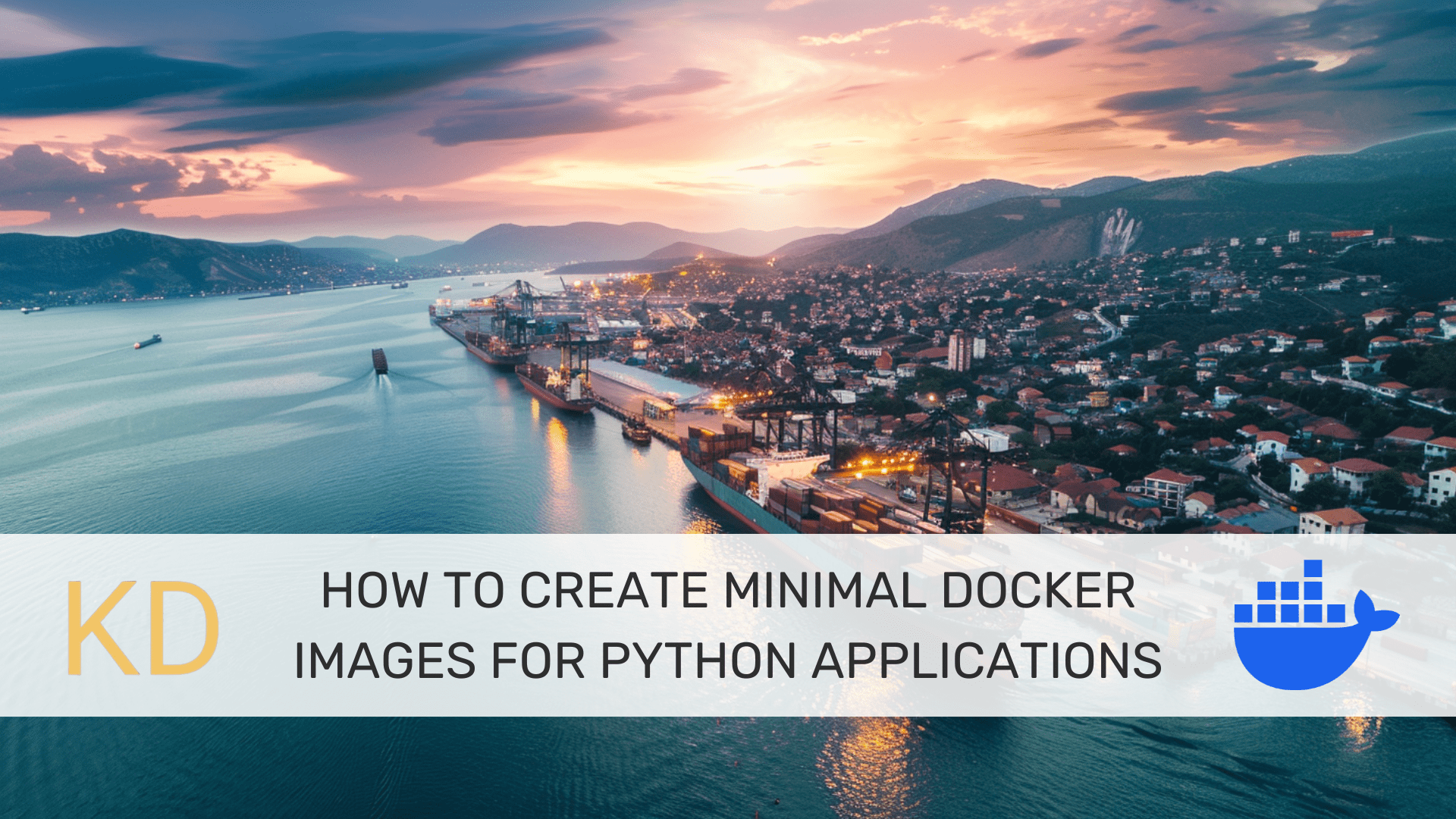
Picture by Editor | Midjourney & Canva
Creating minimal Docker pictures for Python apps enhances safety by decreasing the assault floor, facilitates sooner picture builds, and improves general utility maintainability. Let’s discover ways to create minimal Docker pictures for Python functions.
Conditions
Earlier than you get began:
- You must have Docker put in. Get Docker in your working system in case you haven’t already.
- A pattern Python utility you could construct the minimal picture for. You may as well observe together with the instance app we create.
Create a Pattern Python Software
Let’s create a easy Flask utility for stock administration. This utility will help you add, view, replace, and delete stock gadgets. We’ll then dockerize the appliance utilizing the usual Python 3.11 picture.
In your challenge listing, it is best to have app.py, necessities.txt, and Dockerfile:
inventory_app/
├── app.py
├── Dockerfile
├── necessities.txt
Right here’s the code for the Flask app for stock administration:
# app.py
from flask import Flask, request, jsonify
app = Flask(__name__)
# In-memory database for simplicity
stock = {}
@app.route('/stock', strategies=['POST'])
def add_item():
merchandise = request.get_json()
item_id = merchandise.get('id')
if not item_id:
return jsonify({"error": "Merchandise ID is required"}), 400
if item_id in stock:
return jsonify({"error": "Merchandise already exists"}), 400
stock[item_id] = merchandise
return jsonify(merchandise), 201
@app.route('/stock/', strategies=['GET'])
def get_item(item_id):
merchandise = stock.get(item_id)
if not merchandise:
return jsonify({"error": "Merchandise not discovered"}), 404
return jsonify(merchandise)
@app.route('/stock/', strategies=['PUT'])
def update_item(item_id):
if item_id not in stock:
return jsonify({"error": "Merchandise not discovered"}), 404
updated_item = request.get_json()
stock[item_id] = updated_item
return jsonify(updated_item)
@app.route('/stock/', strategies=['DELETE'])
def delete_item(item_id):
if item_id not in stock:
return jsonify({"error": "Merchandise not discovered"}), 404
del stock[item_id]
return '', 204
if __name__ == '__main__':
app.run(host="0.0.0.0", port=5000)
It is a minimal Flask utility that implements fundamental CRUD (Create, Learn, Replace, Delete) operations for an in-memory stock database. It makes use of Flask to create an internet server that listens for HTTP requests on port 5000. When a request is acquired:
- For a POST request to
/stock
, it provides a brand new merchandise to the stock. - For a GET request to
/stock/<item_id>
, it retrieves the merchandise with the desired ID from the stock. - For a PUT request to
/stock/<item_id>
, it updates the merchandise with the desired ID within the stock. - For a DELETE request to
/stock/<item_id>
, it deletes the merchandise with the desired ID from the stock.
Now create the necessities.txt file:
Subsequent create the Dockerfile:
# Use the official Python 3.11 picture
FROM python:3.11
# Set the working listing
WORKDIR /app
# Set up dependencies
COPY necessities.txt necessities.txt
RUN pip set up --no-cache-dir -r necessities.txt
# Copy the present listing contents into the container at /app
COPY . .
# Expose the port the app runs on
EXPOSE 5000
# Run the appliance
CMD ["python3", "app.py"]
Lastly construct the picture (we use the tag full
to establish that this makes use of the default Python picture):
$ docker construct -t inventory-app:full .
As soon as the construct is full you may run the docker pictures
command:
$ docker pictures
REPOSITORY TAG IMAGE ID CREATED SIZE
inventory-app full 4e623743f556 2 hours in the past 1.02GB
You’ll see that this tremendous easy app is about 1.02 GB in measurement. Properly, it’s because the bottom picture we used the default Python 3.11 picture has a lot of Debian packages and is about 1.01 GB in measurement. So we have to discover a smaller base picture.
Properly, listed here are the choices:
python:version-alpine
pictures are primarily based on Alpine Linux and offers you the smallest ultimate picture. However you want to have the ability to set up packages as nicely, sure? However that’s a problem with alpine pictures.python:version-slim
comes with the minimal variety of Debian packages wanted to run Python. And also you’ll (nearly at all times) have the ability to set up most required Python packages withpip
.
So your base picture must be small. However not too small that you just face compatibility points and wrap your head round putting in dependencies (fairly frequent for Python functions). That’s why we’ll use the python:3.11-slim
base picture within the subsequent step and construct our picture.


Selecting the Optimum Base Picture | Picture by Creator
Use the Slim Python Base Picture
Now rewrite the Dockerfile to make use of the python:3.11-slim
base picture like so:
# Use the official light-weight Python 3.11-slim picture
FROM python:3.11-slim
# Set the working listing
WORKDIR /app
# Set up dependencies
COPY necessities.txt necessities.txt
RUN pip set up --no-cache-dir -r necessities.txt
# Copy the present listing contents into the container at /app
COPY . .
# Expose the port the app runs on
EXPOSE 5000
# Run the appliance
CMD ["python3", "app.py"]
Let’s construct the picture (tagged slim
):
$ docker construct -t inventory-app:slim .
The python:3.11-slim
base picture is of measurement 131 MB. And the inventory-app:slim
picture is round 146 MB which is way smaller than the 1.02GB picture we had earlier:
$ docker pictures
REPOSITORY TAG IMAGE ID CREATED SIZE
inventory-app slim 32784c60a992 About an hour in the past 146MB
inventory-app full 4e623743f556 2 hours in the past 1.02GB
You may as well use multi-stage builds to make the ultimate picture smaller. However that is for an additional tutorial!
Extra Sources
Listed here are just a few helpful assets:
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, knowledge science, and content material creation. Her areas of curiosity and experience embody DevOps, knowledge science, and pure language processing. She enjoys studying, writing, coding, and occasional! At present, she’s engaged on studying and sharing her information with the developer neighborhood by authoring tutorials, how-to guides, opinion items, and extra. Bala additionally creates partaking useful resource overviews and coding tutorials.