How To Reuse React Elements | by Sabesan Sathananthan | Codezillas
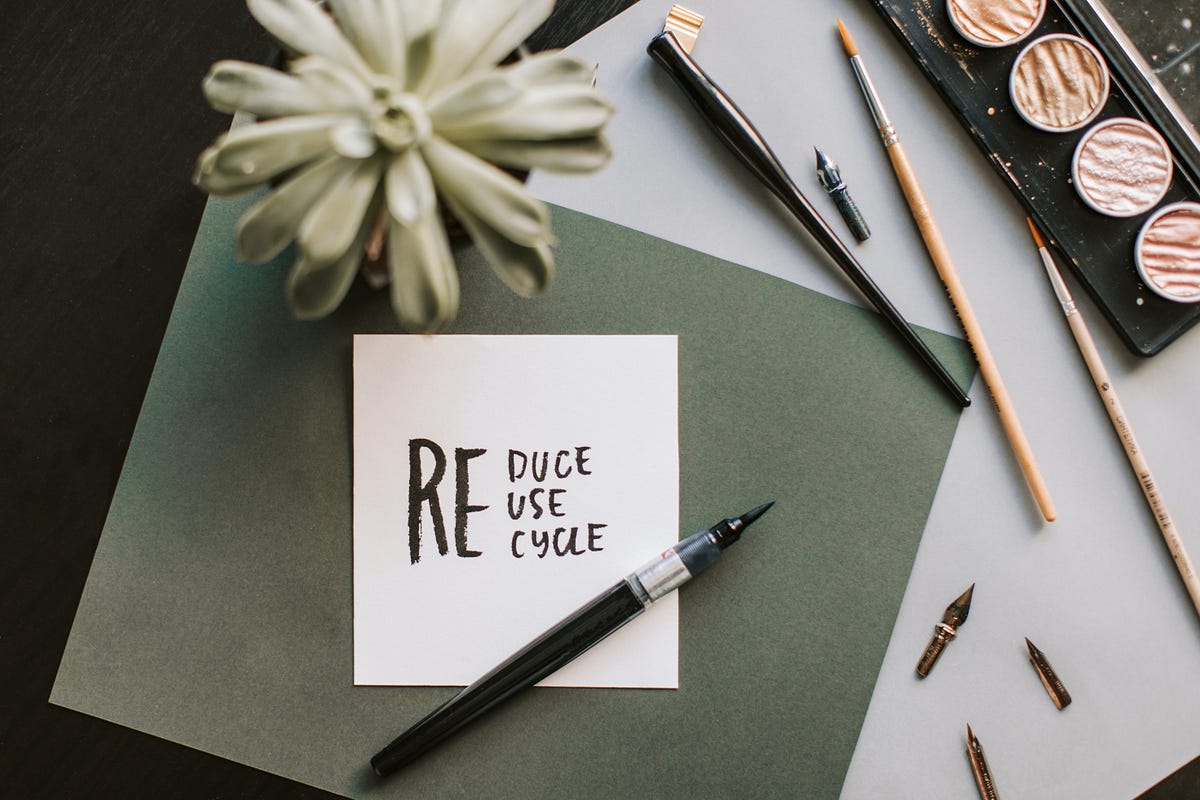
After Mixin
, HOC high-order parts tackle the heavy duty and turn into the really useful resolution for logical reuse between parts. Excessive-order parts reveal a high-order ambiance from their names. In actual fact, this idea must be derived from high-order features of JavaScript
. The high-order operate is a operate that accepts a operate as enter or output. It may be thought that currying is a higher-order operate. The definition of higher-order parts can also be given within the React
doc. Greater-order parts obtain parts and return new parts. operate. The particular that means is: Excessive-order parts could be seen as an implementation of React
ornament sample. Excessive-order parts are a operate, and the operate accepts a element as a parameter and returns a brand new element. It’ll return an enhanced React
parts. Excessive-order parts could make our code extra reusable, logical and summary, can hijack the render
methodology, and may management props
and state
.
Evaluating Mixin
and HOC
, Mixin
is a mixed-in mode. In precise use, Mixin
continues to be very highly effective, permitting us to share the identical methodology in a number of parts, however it would additionally proceed so as to add new strategies and attributes to the parts. The element itself cannot solely understand but additionally have to do associated processing (resembling naming conflicts, state upkeep, and so forth.). As soon as the combined modules improve, the complete element turns into troublesome to take care of. Mixin
might introduce invisible attributes, resembling within the Mixin
methodology used within the rendering element brings invisible property props
and states
to the element. Mixin
might depend upon one another and is coupled with one another, which isn’t conducive to code upkeep. As well as, the strategies in numerous Mixin
might battle with one another. Beforehand React
formally really useful utilizing Mixin
to unravel issues associated to cross-cutting issues, however as a result of utilizing Mixin
might trigger extra hassle, the official advice is now to make use of HOC
. Excessive-order element HOC
belong to the concept of purposeful programming
. The wrapped parts is not going to pay attention to the existence of high-order parts, and the parts returned by high-order parts may have a purposeful enhancement impact on the unique parts. Primarily based on this, React
formally recommends the usage of high-order parts.
Though HOC
doesn’t have so many deadly issues, it additionally has some minor flaws:
- Scalability restriction:
HOC
can’t fully substituteMixin
. In some eventualities,Mixin
can howeverHOC
can’t. For instance,PureRenderMixin
, as a result ofHOC
can’t entry theState
of subcomponents from the skin, and on the identical time filter out pointless updates by means ofshouldComponentUpdate
. Subsequently,React
After supportingES6Class
,React.PureComponent
is offered to unravel this downside. Ref
switch downside:Ref
is lower off. The switch downside ofRef
is kind of annoying beneath the layers of packaging. The operateRef
can alleviate a part of it (permittingHOC
to study node creation and destruction), so theReact.forwardRef API
API was launched later.WrapperHell
:HOC
is flooded, andWrapperHell
seems (there is no such thing as a downside that can not be solved by one layer, if there may be, then two layers). Multi-layer abstraction additionally will increase complexity and value of understanding. That is probably the most important defect. InHOC
mode There isn’t any good resolution.
Instance
Particularly, a high-order element is a operate whose parameter is a element and the return worth is a brand new element. A element converts props
right into a UI
however a high-order element converts a element into one other element. HOC
is quite common in React
third-party libraries, resembling Redux
’s join
and Relay
’s createFragmentContainer
.
Consideration must be paid right here, don’t attempt to modify the element prototype within the HOC
in any manner, however ought to use the mix methodology to understand the operate by packaging the element within the container element. Beneath regular circumstances, there are two methods to implement high-order parts:
- Property agent
Props Proxy
. - Reverse inheritance
Inheritance Inversion
.
Property Agent
For instance, we are able to add a saved id
attribute worth to the incoming element. We will add a props
to this element by means of high-order parts. After all, we are able to additionally function on the props
within the WrappedComponent
element in JSX
. Word that it’s not to govern the incoming WrappedComponent
class, we should always in a roundabout way modify the incoming element, however can function on it within the means of mixture.
We will additionally use high-order parts to load the state of latest parts into the packaged parts. For instance, we are able to use high-order parts to transform uncontrolled parts into managed parts.
Or our goal is to wrap it with different parts to attain the aim of structure or fashion.
Reverse inheritance
Reverse inheritance implies that the returned element inherits the earlier element. In reverse inheritance, we are able to do a whole lot of operations, modify state
, props
and even flip the Ingredient Tree
. There is a crucial level within the reverse inheritance that reverse inheritance can’t be sure that the whole sub-component tree is parsed. Which means if the parsed factor tree accommodates parts (operate
sort or Class
sort), the sub-components of the element can not be manipulated.
Once we use reverse inheritance to implement high-order parts, we are able to management rendering by means of rendering hijacking. Particularly, we are able to consciously management the rendering means of WrappedComponent
to manage the outcomes of rendering management. For instance, we are able to determine whether or not to render parts in line with some parameters.
We will even hijack the life cycle of the unique element by rewriting.
Since it’s truly an inheritance relationship, we are able to learn the props
and state
of the element. If essential, we are able to even add, modify, and delete the props
and state
. After all, the premise is that the dangers brought on by the modification have to be managed by your self. In some instances, we might have to cross in some parameters for the high-order attributes, then we are able to cross within the parameters within the type of currying, and cooperate with the high-order parts to finish the operation much like the closure of the element.
word
Don’t change the unique parts
Don’t attempt to modify the element prototype in HOC
, or change it in different methods.
Doing so may have some undesirable penalties. One is that the enter element can not be used as earlier than the HOC
enhancement. What’s extra critical is that when you use one other HOC
that additionally modifies componentDidUpdate
to boost it, the earlier HOC
will likely be invalid, and this HOC
can’t be utilized to purposeful parts that haven’t any life cycle.
Modifying the HOC
of the incoming element is a nasty abstraction, and the caller should understand how they’re carried out to keep away from conflicts with different HOC
. HOC
shouldn’t modify the incoming parts, however ought to use a mixture of parts to attain features by packaging the parts in container parts.
Filter props
HOC
provides options to parts and shouldn’t considerably change the conference itself. The parts returned by HOC
ought to keep comparable interfaces with the unique parts. HOC
ought to transparently transmit props
that don’t have anything to do with itself, and most HOC
ought to embrace a render
methodology much like the next.
Most composability
Not all HOCs
are the identical. Generally it solely accepts one parameter, which is the packaged element.
const NavbarWithRouter = withRouter(Navbar);
HOC
can normally obtain a number of parameters. For instance, in Relay
, HOC moreover receives a configuration object to specify the information dependency of the element.
const CommentWithRelay = Relay.createContainer(Remark, config);
The most typical HOC signatures are as follows, join is a higher-order operate that returns higher-order parts.
This manner could appear complicated or pointless, but it surely has a helpful property, just like the single-parameter HOC
returned by the join
operate has the signature Part => Part
, and features with the identical output sort and enter sort could be simply mixed. The identical attributes additionally permit join
and different HOCs
to imagine the position of decorator. As well as, many third-party libraries present compose device features, together with lodash
, Redux
, and Ramda
.
Don’t use HOC within the render methodology
React
’s diff
algorithm makes use of the element identifier to find out whether or not it ought to replace the prevailing subtree or discard it and mount the brand new subtree. If the element returned from the render
is similar because the element within the earlier render ===
, React
passes The subtree is distinguished from the brand new subtree to recursively replace the subtree, and if they don’t seem to be equal, the earlier subtree is totally unloaded.
Often, you don’t want to think about this when utilizing it, however it is vitally necessary for HOC
, as a result of it implies that you shouldn’t apply HOC
to a element within the render
methodology of the element.
This isn’t only a efficiency problem. Re-mounting the element will trigger the state of the element and all its subcomponents to be misplaced. If the HOC
is created outdoors the element, the element will solely be created as soon as. So each time you render
will probably be the identical element. Usually talking, that is constant together with your anticipated efficiency. In uncommon instances, it is advisable to name HOC
dynamically, you’ll be able to name it within the element’s lifecycle methodology or its constructor.
Remember to copy static strategies
Generally it’s helpful to outline static strategies on React
parts. For instance, the Relay
container exposes a static methodology getFragment
to facilitate the composition of GraphQL
fragments. However if you apply HOC
to a element, the unique element will likely be packaged with a container element, which implies that the brand new element doesn’t have any static strategies of the unique element.
To resolve this downside, you’ll be able to copy these strategies to the container element earlier than returning.
However to do that, it is advisable to know which strategies must be copied. You should use hoist-non-react-statics
to robotically copy all non-React
static strategies.
Along with exporting parts, one other possible resolution is to moreover export this static methodology.
Refs is not going to be handed
Though the conference of high-level parts is to cross all props
to the packaged element, this doesn’t apply to refs
, as a result of ref
is just not truly a prop
, identical to a key
, it’s particularly dealt with by React
. If the ref
is added to the return element of the HOC
, the ref
reference factors to the container element, not the packaged element. This downside could be explicitly forwarded to the interior element by means of the React.forwardRefAPI
refs
.