How you can use sum() Perform in Python?
Introduction
On this article, you’ll uncover an in-depth exploration of the sum()
perform in Python, an important built-in utility for aggregating numbers throughout numerous iterable varieties. We start by explaining the basic syntax and parameters of the sum() perform, adopted by detailed examples showcasing its software to lists, dictionaries, units, and tuples. Moreover, you’ll study error dealing with to stop widespread pitfalls and see sensible eventualities, equivalent to calculating averages, the place sum()
proves invaluable. This information goals to boost your understanding and utilization of sum()
in on a regular basis programming duties, making your code extra environment friendly and readable.
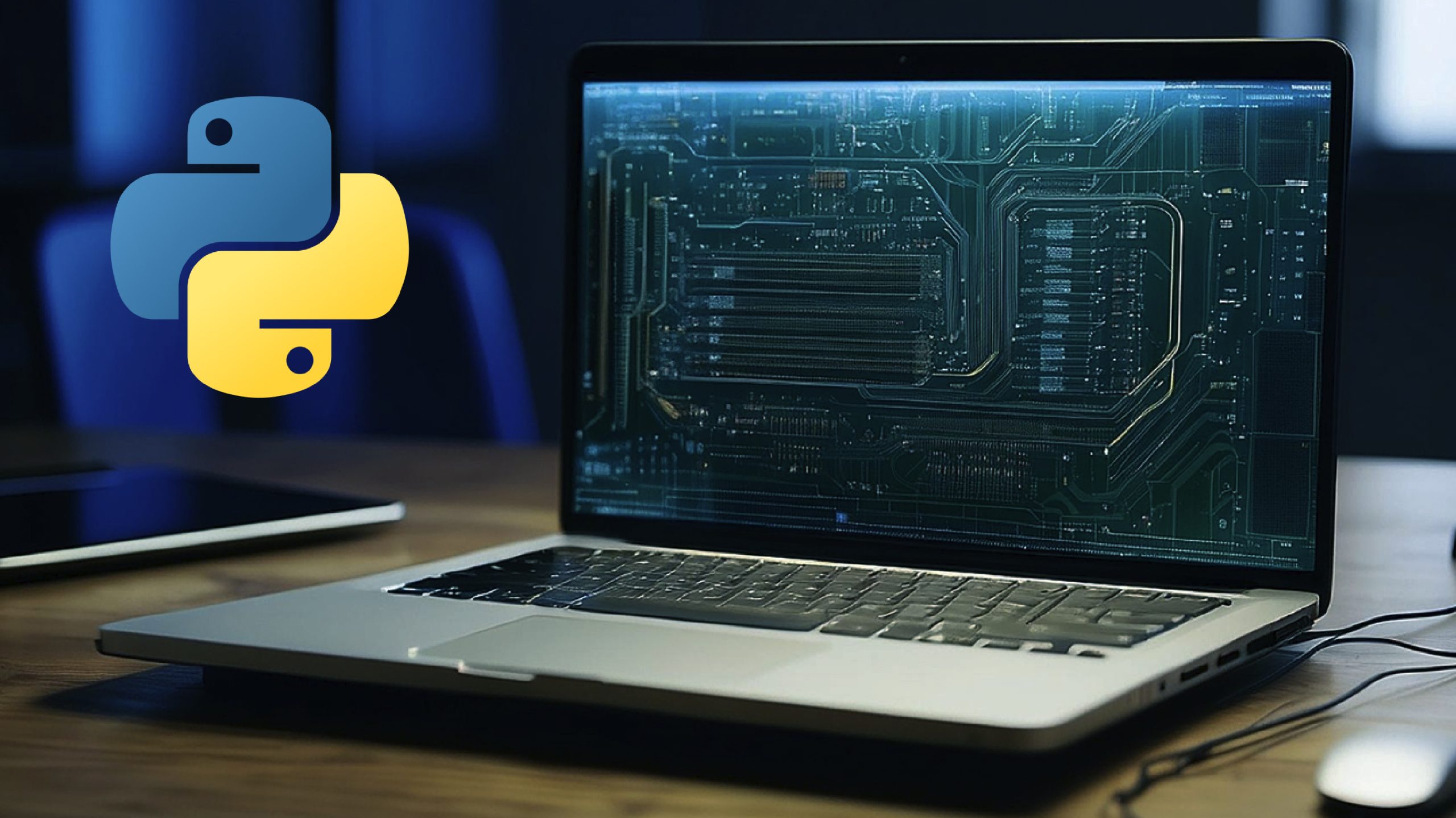
What’s sum() Perform?
It’s possible you’ll compute the sum of the numbers in an iterable utilizing Python’s strong built-in sum() perform. In jobs involving information evaluation and processing, this perform is often utilized. Allow us to perceive the syntax of sum() perform.
Syntax of sum()
Perform
The sum() perform syntax is simple:
sum(iterable, begin)
- iterable: Any iterable holding numerical values, equivalent to a listing, tuple, set, dictionary, and so on., might be this.
- begin: This elective argument provides a specified worth to the sum of the iterable’s values. Within the absence of 1, it defaults to 0.
Simplified Syntax Variations
sum(a)
: Calculates the entire quantity within the checklist a, with 0 because the preliminary worth by default.sum(a, begin)
: Computes the sum of the numbers within the checklista
and provides thebegin
worth to the consequence.
Primary Utilization
Let’s begin with a fundamental instance the place we sum a listing of numbers to raised perceive how the sum() perform capabilities. This fundamental instance will illustrate the core performance of sum() in a simple method.
numbers = [1, 2, 3, 4, 5]
whole = sum(numbers)
print(whole) # Output: 15
Utilizing the beginning Parameter
The begin
parameter means that you can add a price to the sum of the iterable. Right here’s how you should use it:
numbers = [1, 2, 3, 4, 5]
whole = sum(numbers, 10)
print(whole) # Output: 25
Examples of Utilizing sum()
Allow us to now discover some examples of utilizing sum().
Summing Numbers in a Record
Let’s begin with a fundamental instance of summing numbers in a listing.
bills = [200, 150, 50, 300]
total_expenses = sum(bills)
print(total_expenses) # Output: 700
Summing Values in a Dictionary
You’ll be able to sum the values of a dictionary utilizing the values()
methodology.
my_dict = {'x': 21, 'y': 22, 'z': 23}
whole = sum(my_dict.values())
print(whole) # Output: 66
Summing Parts in a Set
Units, like lists, might be summed instantly.
unique_numbers = {12, 14, 15}
total_unique = sum(unique_numbers)
print(total_unique) # Output: 39
Summing Parts in a Tuple
Tuples, just like lists, will also be summed.
scores = (90, 85, 88, 92)
total_scores = sum(scores)
print(total_scores) # Output: 355
Summing Numbers Utilizing a For Loop
Though the sum()
perform is handy, you may as well sum numbers manually utilizing a for loop.
numbers = [100, 200, 300, 400, 500]
# Initialize the sum to 0
whole = 0
# Iterate by means of the checklist and add every quantity to the entire
for num in numbers:
whole += num
print("The sum of the numbers is:", whole) # Output: 1500
Summing with a Generator Expression
Generator expressions can be utilized to sum a collection of numbers generated on the fly:
whole = sum(i for i in vary(1, 6))
print(whole) # Output: 15
Summing the Parts of a Record of Lists
Generally, you might have a listing of lists and need to sum the weather inside every checklist. Right here’s how you are able to do it:
list_of_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
whole = sum(sum(inner_list) for inner_list in list_of_lists)
print(whole) # Output: 45
Superior Purposes
Allow us to discover some superior functions of sum() perform.
Summing Non-Numeric Values
Whereas sum() is primarily used for numeric values, you should use it together with different capabilities to sum non-numeric values. For instance, summing the lengths of strings in a listing:
phrases = ["apple", "banana", "cherry"]
total_length = sum(len(phrase) for phrase in phrases)
print(total_length) # Output: 16
Summing with Conditional Logic
You’ll be able to incorporate conditional logic inside a generator expression to sum values that meet sure standards:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
total_even = sum(n for n in numbers if n % 2 == 0)
print(total_even) # Output: 30
Error Dealing with
The sum() perform raises a TypeError if the iterable accommodates non-numeric values.
array1 = ["apple"]
strive:
# Try to sum the checklist of strings
whole = sum(array1)
besides TypeError as e:
print(e) # Output: unsupported operand sort(s) for +: 'int' and 'str'
Sensible Software
Allow us to now look into the sensible functions of sum() perform.
Summing the Ages of a Record of Individuals
class Individual:
def __init__(self, identify, age):
self.identify = identify
self.age = age
folks = [Person("Alice", 30), Person("Bob", 25), Person("Charlie", 35)]
# Calculate the entire sum of ages
total_age = sum(individual.age for individual in folks)
print(total_age) # Output: 90
Monetary Calculations
Sum() can be utilized for monetary calculations, equivalent to computing the entire bills or earnings over a interval.
Calculating Complete Bills:
bills = {
'hire': 1200,
'groceries': 300,
'utilities': 150,
'leisure': 100
}
# Calculate the entire sum of bills
total_expenses = sum(bills.values())
print(total_expenses) # Output: 1750
Calculating Yearly Earnings:
monthly_income = [2500, 2700, 3000, 3200, 2900, 3100, 2800, 2600, 3300, 3500, 3400, 3600]
# Calculate the entire sum of yearly earnings
yearly_income = sum(monthly_income)
print(yearly_income) # Output: 37600
Conclusion
For including up the numbers in several iterables, Python’s sum() methodology offers a versatile and efficient instrument. It’s a important instrument for any programmer’s toolset due to its simplicity of utilization and Python’s sturdy error dealing with. The sum() perform makes aggregation less complicated and allows easy, quick code, no matter whether or not one is working with lists, tuples, units, or dictionaries.