[ad_1]
At present, most purposes can ship lots of of requests for a single web page.
For instance, my Twitter residence web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
belongings (JavaScript, CSS, font information, icons, and so on.), however there are nonetheless
round 100 requests for async knowledge fetching – both for timelines, associates,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The primary cause a web page might include so many requests is to enhance
efficiency and person expertise, particularly to make the appliance really feel
quicker to the tip customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In fashionable internet purposes, customers sometimes see a fundamental web page with
type and different parts in lower than a second, with further items
loading progressively.
Take the Amazon product element web page for example. The navigation and high
bar seem virtually instantly, adopted by the product pictures, temporary, and
descriptions. Then, as you scroll, “Sponsored” content material, scores,
suggestions, view histories, and extra seem.Typically, a person solely needs a
fast look or to match merchandise (and examine availability), making
sections like “Prospects who purchased this merchandise additionally purchased” much less important and
appropriate for loading by way of separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, but it surely’s removed from sufficient in giant
purposes. There are various different points to think about on the subject of
fetch knowledge accurately and effectively. Information fetching is a chellenging, not
solely as a result of the character of async programming does not match our linear mindset,
and there are such a lot of elements could cause a community name to fail, but additionally
there are too many not-obvious circumstances to think about underneath the hood (knowledge
format, safety, cache, token expiry, and so on.).
On this article, I want to focus on some frequent issues and
patterns you need to take into account on the subject of fetching knowledge in your frontend
purposes.
We’ll start with the Asynchronous State Handler sample, which decouples
knowledge fetching from the UI, streamlining your utility structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your knowledge
fetching logic. To speed up the preliminary knowledge loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Information Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical utility elements and Prefetching knowledge primarily based on person
interactions to raise the person expertise.
I consider discussing these ideas by a simple instance is
the very best method. I intention to begin merely after which introduce extra complexity
in a manageable means. I additionally plan to maintain code snippets, notably for
styling (I am using TailwindCSS for the UI, which can lead to prolonged
snippets in a React element), to a minimal. For these within the
full particulars, I’ve made them obtainable on this
repository.
Developments are additionally occurring on the server aspect, with strategies like
Streaming Server-Facet Rendering and Server Parts gaining traction in
varied frameworks. Moreover, a lot of experimental strategies are
rising. Nonetheless, these matters, whereas doubtlessly simply as essential, may be
explored in a future article. For now, this dialogue will focus
solely on front-end knowledge fetching patterns.
It is essential to notice that the strategies we’re protecting will not be
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions attributable to my in depth expertise with
it lately. Nonetheless, ideas like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I am going to share
are frequent situations you would possibly encounter in frontend improvement, regardless
of the framework you employ.
That stated, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display of a Single-Web page Utility. It is a typical
utility you might need used earlier than, or not less than the situation is typical.
We have to fetch knowledge from server aspect after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the appliance
To start with, on Profile
we’ll present the person’s temporary (together with
identify, avatar, and a brief description), after which we additionally need to present
their connections (just like followers on Twitter or LinkedIn
connections). We’ll have to fetch person and their connections knowledge from
distant service, after which assembling these knowledge with UI on the display.
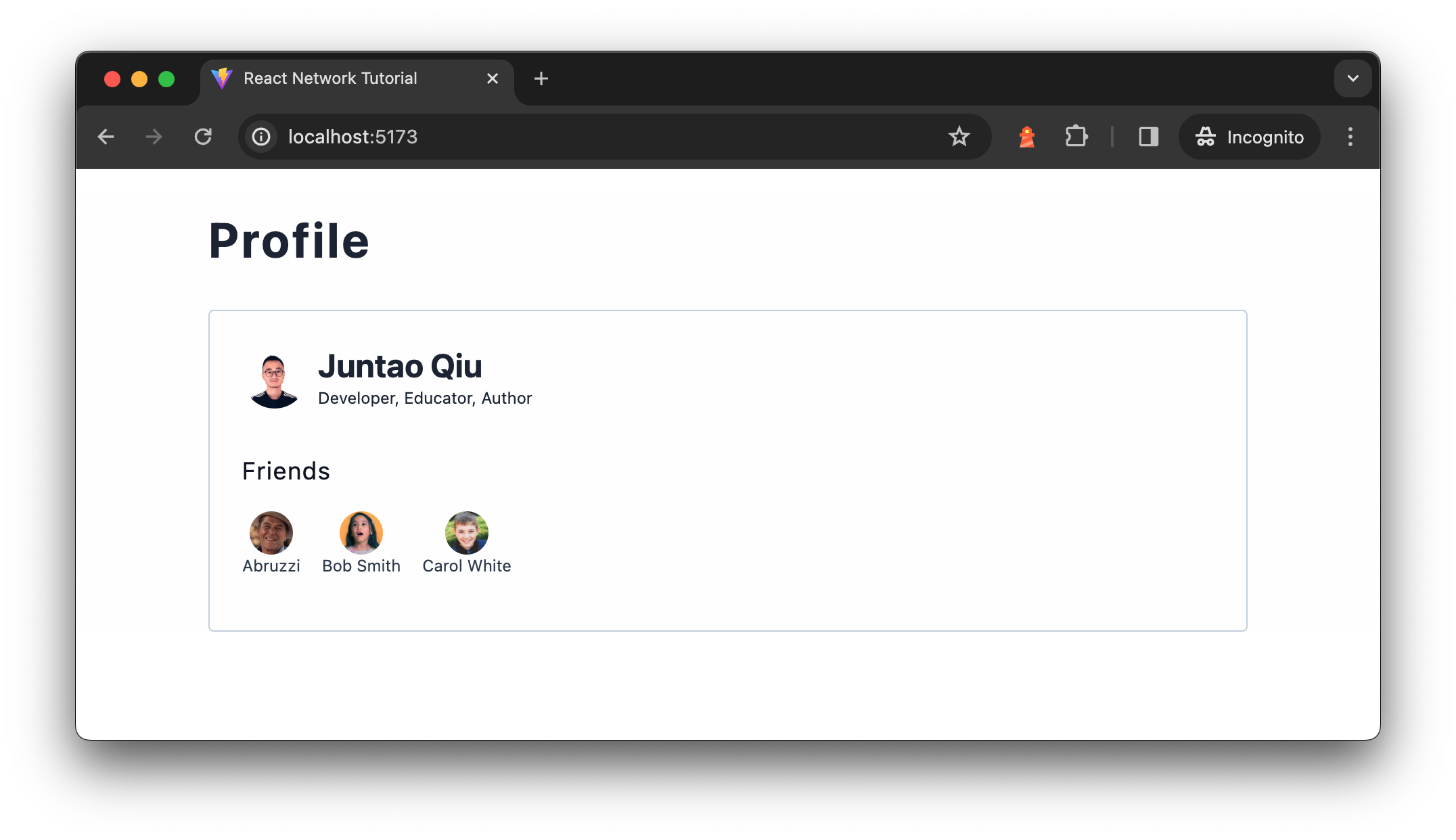
Determine 1: Profile display
The info are from two separate API calls, the person temporary API
/customers/<id>
returns person temporary for a given person id, which is a straightforward
object described as follows:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Writer", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the buddy API /customers/<id>/associates
endpoint returns a listing of
associates for a given person, every record merchandise within the response is identical as
the above person knowledge. The rationale we’ve got two endpoints as an alternative of returning
a associates
part of the person API is that there are circumstances the place one
may have too many associates (say 1,000), however most individuals do not have many.
This in-balance knowledge construction will be fairly tough, particularly once we
have to paginate. The purpose right here is that there are circumstances we have to deal
with a number of community requests.
A short introduction to related React ideas
As this text leverages React as an example varied patterns, I do
not assume you realize a lot about React. Quite than anticipating you to spend so much
of time looking for the proper elements within the React documentation, I’ll
briefly introduce these ideas we will make the most of all through this
article. In case you already perceive what React elements are, and the
use of the
useState
and useEffect
hooks, you might
use this hyperlink to skip forward to the subsequent
part.
For these looking for a extra thorough tutorial, the new React documentation is a wonderful
useful resource.
What’s a React Element?
In React, elements are the elemental constructing blocks. To place it
merely, a React element is a perform that returns a bit of UI,
which will be as simple as a fraction of HTML. Contemplate the
creation of a element that renders a navigation bar:
import React from 'react'; perform Navigation() { return ( <nav> <ol> <li>Residence</li> <li>Blogs</li> <li>Books</li> </ol> </nav> ); }
At first look, the combination of JavaScript with HTML tags might sound
unusual (it is known as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, an identical syntax known as TSX is used). To make this
code practical, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
perform Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "Residence"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Word right here the translated code has a perform known as
React.createElement
, which is a foundational perform in
React for creating parts. JSX written in React elements is compiled
right down to React.createElement
calls behind the scenes.
The fundamental syntax of React.createElement
is:
React.createElement(kind, [props], [...children])
kind
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React element (class or practical) for
extra refined buildings.props
: An object containing properties handed to the
aspect or element, together with occasion handlers, types, and attributes
likeclassName
andid
.kids
: These non-obligatory arguments will be further
React.createElement
calls, strings, numbers, or any combine
thereof, representing the aspect’s kids.
As an example, a easy aspect will be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Hiya, world!');
That is analogous to the JSX model:
<div className="greeting">Hiya, world!</div>
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM parts as crucial.
You may then assemble your customized elements right into a tree, just like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; perform App() { return <Web page />; } perform Web page() { return <Container> <Navigation /> <Content material> <Sidebar /> <ProductList /> </Content material> <Footer /> </Container>; }
Finally, your utility requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/shopper"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render(<App />);
Producing Dynamic Content material with JSX
The preliminary instance demonstrates a simple use case, however
let’s discover how we will create content material dynamically. As an example, how
can we generate a listing of information dynamically? In React, as illustrated
earlier, a element is basically a perform, enabling us to go
parameters to it.
import React from 'react'; perform Navigation({ nav }) { return ( <nav> <ol> {nav.map(merchandise => <li key={merchandise}>{merchandise}</li>)} </ol> </nav> ); }
On this modified Navigation
element, we anticipate the
parameter to be an array of strings. We make the most of the map
perform to iterate over every merchandise, reworking them into
<li>
parts. The curly braces {}
signify
that the enclosed JavaScript expression ought to be evaluated and
rendered. For these curious in regards to the compiled model of this dynamic
content material dealing with:
perform Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(perform(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As an alternative of invoking Navigation
as a daily perform,
using JSX syntax renders the element invocation extra akin to
writing markup, enhancing readability:
// As an alternative of this Navigation(["Home", "Blogs", "Books"]) // We do that <Navigation nav={["Home", "Blogs", "Books"]} />
Parts in React can obtain various knowledge, often known as props, to
modify their conduct, very similar to passing arguments right into a perform (the
distinction lies in utilizing JSX syntax, making the code extra acquainted and
readable to these with HTML information, which aligns effectively with the ability
set of most frontend builders).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App() { let showNewOnly = false; // This flag's worth is usually set primarily based on particular logic. const filteredBooks = showNewOnly ? booksData.filter(e book => e book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks} /> </div> ); }
On this illustrative code snippet (non-functional however supposed to
exhibit the idea), we manipulate the BookList
element’s displayed content material by passing it an array of books. Relying
on the showNewOnly
flag, this array is both all obtainable
books or solely these which are newly revealed, showcasing how props can
be used to dynamically modify element output.
Managing Inside State Between Renders: useState
Constructing person interfaces (UI) typically transcends the technology of
static HTML. Parts steadily have to “bear in mind” sure states and
reply to person interactions dynamically. As an example, when a person
clicks an “Add” button in a Product element, it’s a necessity to replace
the ShoppingCart element to mirror each the entire value and the
up to date merchandise record.
Within the earlier code snippet, making an attempt to set the
showNewOnly
variable to true
inside an occasion
handler doesn’t obtain the specified impact:
perform App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this does not work }; const filteredBooks = showNewOnly ? booksData.filter(e book => e book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
This method falls quick as a result of native variables inside a perform
element don’t persist between renders. When React re-renders this
element, it does so from scratch, disregarding any modifications made to
native variables since these don’t set off re-renders. React stays
unaware of the necessity to replace the element to mirror new knowledge.
This limitation underscores the need for React’s
state
. Particularly, practical elements leverage the
useState
hook to recollect states throughout renders. Revisiting
the App
instance, we will successfully bear in mind the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(e book => e book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow practical elements to handle inner state. It
introduces state to practical elements, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra advanced object or array. The
initialState
is just used throughout the first render to
initialize the state.- Return Worth:
useState
returns an array with
two parts. The primary aspect is the present state worth, and the
second aspect is a perform that enables updating this worth. By utilizing
array destructuring, we assign names to those returned gadgets,
sometimesstate
andsetState
, although you may
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that will likely be used within the element’s UI and
logic.setState
: A perform to replace the state. This perform
accepts a brand new state worth or a perform that produces a brand new state primarily based
on the earlier state. When known as, it schedules an replace to the
element’s state and triggers a re-render to mirror the modifications.
React treats state as a snapshot; updating it does not alter the
current state variable however as an alternative triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, guaranteeing the
BookList
element receives the right knowledge, thereby
reflecting the up to date e book record to the person. This snapshot-like
conduct of state facilitates the dynamic and responsive nature of React
elements, enabling them to react intuitively to person interactions and
different modifications.
Managing Facet Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to deal with the
idea of unintended effects. Uncomfortable side effects are operations that work together with
the surface world from the React ecosystem. Widespread examples embrace
fetching knowledge from a distant server or dynamically manipulating the DOM,
equivalent to altering the web page title.
React is primarily involved with rendering knowledge to the DOM and does
not inherently deal with knowledge fetching or direct DOM manipulation. To
facilitate these unintended effects, React supplies the useEffect
hook. This hook permits the execution of unintended effects after React has
accomplished its rendering course of. If these unintended effects lead to knowledge
modifications, React schedules a re-render to mirror these updates.
The useEffect
Hook accepts two arguments:
- A perform containing the aspect impact logic.
- An non-obligatory dependency array specifying when the aspect impact ought to be
re-invoked.
Omitting the second argument causes the aspect impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t depend upon any values from props or state, thus not needing to
re-run. Together with particular values within the array means the aspect impact
solely re-executes if these values change.
When coping with asynchronous knowledge fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the info is
retrieved, it’s captured by way of the useState
hook, updating the
element’s inner state and preserving the fetched knowledge throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new knowledge.
This is a sensible instance about knowledge fetching and state
administration:
import { useEffect, useState } from "react"; kind Person = { id: string; identify: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-14:Information-Fetching-Patterns-in-Single-Web page-Functions); return <div> <h2>{person?.identify}</h2> </div>; };
Within the code snippet above, inside useEffect
, an
asynchronous perform fetchUser
is outlined after which
instantly invoked. This sample is critical as a result of
useEffect
doesn’t instantly assist async features as its
callback. The async perform is outlined to make use of await
for
the fetch operation, guaranteeing that the code execution waits for the
response after which processes the JSON knowledge. As soon as the info is offered,
it updates the element’s state by way of setUser
.
The dependency array tag:martinfowler.com,2024-05-14:Information-Fetching-Patterns-in-Single-Web page-Functions
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
modifications, which prevents pointless community requests on
each render and fetches new person knowledge when the id
prop
updates.
This method to dealing with asynchronous knowledge fetching inside
useEffect
is an ordinary apply in React improvement, providing a
structured and environment friendly technique to combine async operations into the
React element lifecycle.
As well as, in sensible purposes, managing totally different states
equivalent to loading, error, and knowledge presentation is important too (we’ll
see it the way it works within the following part). For instance, take into account
implementing standing indicators inside a Person element to mirror
loading, error, or knowledge states, enhancing the person expertise by
offering suggestions throughout knowledge fetching operations.
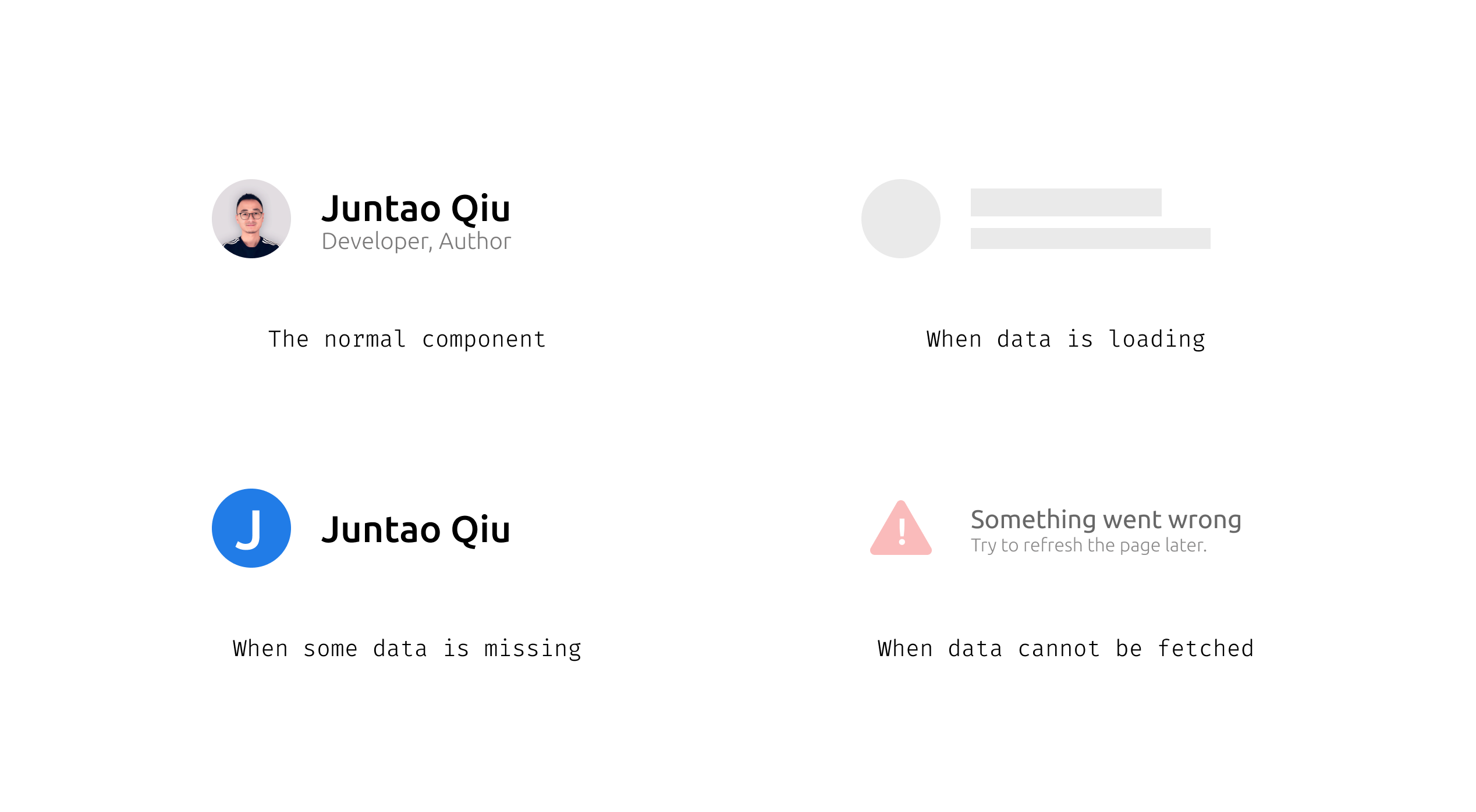
Determine 2: Totally different statuses of a
element
This overview affords only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into further ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line assets.
With this basis, you need to now be geared up to affix me as we delve
into the info fetching patterns mentioned herein.
Implement the Profile element
Let’s create the Profile
element to make a request and
render the end result. In typical React purposes, this knowledge fetching is
dealt with inside a useEffect
block. This is an instance of how
this may be applied:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-14:Information-Fetching-Patterns-in-Single-Web page-Functions); return ( <UserBrief person={person} /> ); };
This preliminary method assumes community requests full
instantaneously, which is commonly not the case. Actual-world situations require
dealing with various community situations, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
element. This addition permits us to offer suggestions to the person throughout
knowledge fetching, equivalent to displaying a loading indicator or a skeleton display
if the info is delayed, and dealing with errors once they happen.
Right here’s how the improved element seems with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import kind { Person } from "../varieties.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Person>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-14:Information-Fetching-Patterns-in-Single-Web page-Functions); if (loading || !person) { return <div>Loading...</div>; } return ( <> {person && <UserBrief person={person} />} </> ); };
Now in Profile
element, we provoke states for loading,
errors, and person knowledge with useState
. Utilizing
useEffect
, we fetch person knowledge primarily based on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
knowledge retrieval, we replace the person state, else show a loading
indicator.
The get
perform, as demonstrated under, simplifies
fetching knowledge from a selected endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON knowledge or throws an error for unsuccessful requests,
streamlining error dealing with and knowledge retrieval in our utility. Word
it is pure TypeScript code and can be utilized in different non-React elements of the
utility.
const baseurl = "https://icodeit.com.au/api/v2"; async perform get<T>(url: string): Promise<T> { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise<T>; }
React will attempt to render the element initially, however as the info
person
isn’t obtainable, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as in some unspecified time in the future, the response returns, React
re-renders the Profile
element with person
fulfilled, so now you can see the person part with identify, avatar, and
title.
If we visualize the timeline of the above code, you will notice
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and elegance tags, it’d cease and
obtain these information, after which parse them to type the ultimate web page. Word
that this can be a comparatively sophisticated course of, and I’m oversimplifying
right here, however the fundamental thought of the sequence is right.
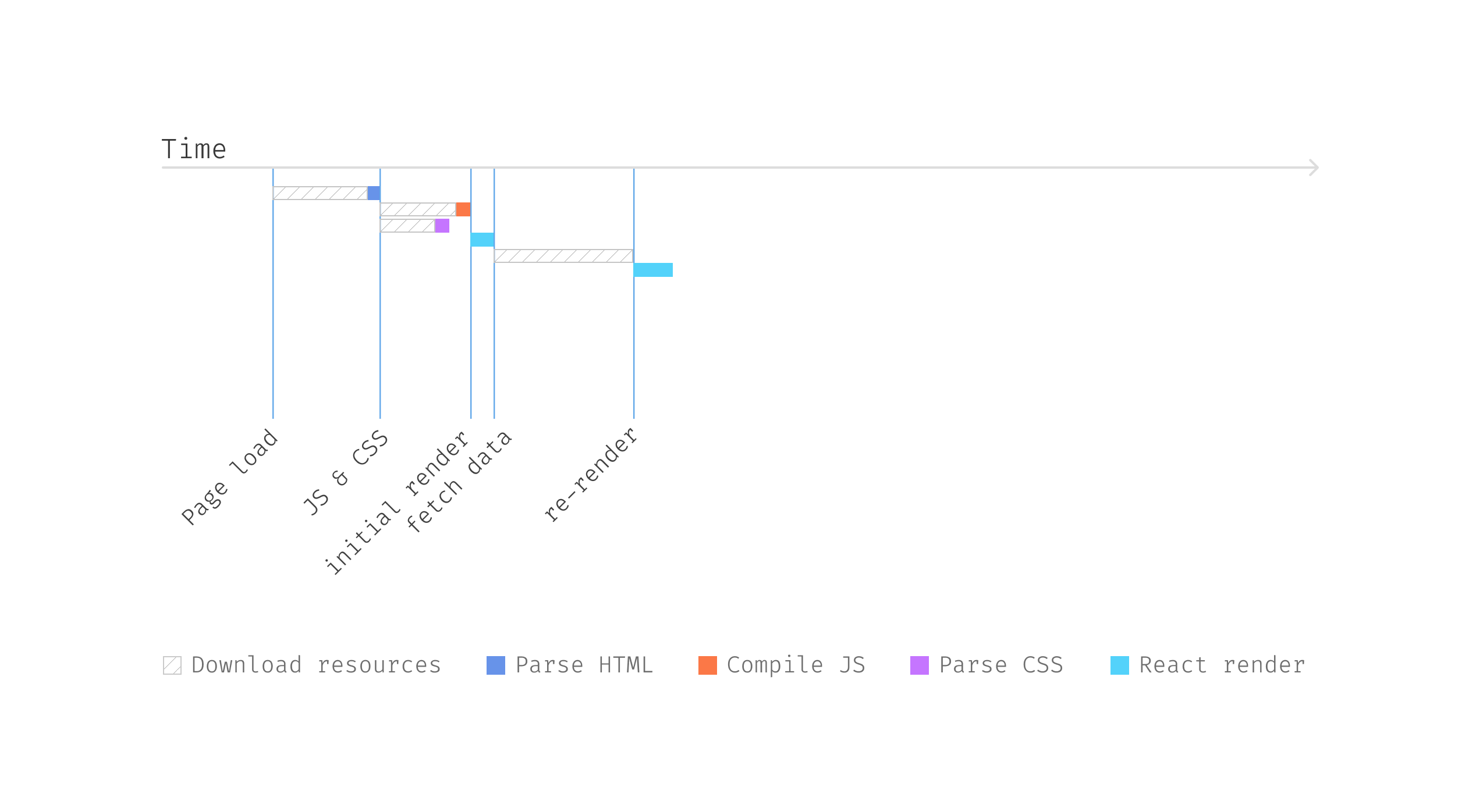
Determine 3: Fetching person
knowledge
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for knowledge fetching; it has to attend till
the info is offered for a re-render.
Now within the browser, we will see a “loading…” when the appliance
begins, after which after a couple of seconds (we will simulate such case by add
some delay within the API endpoints) the person temporary part exhibits up when knowledge
is loaded.
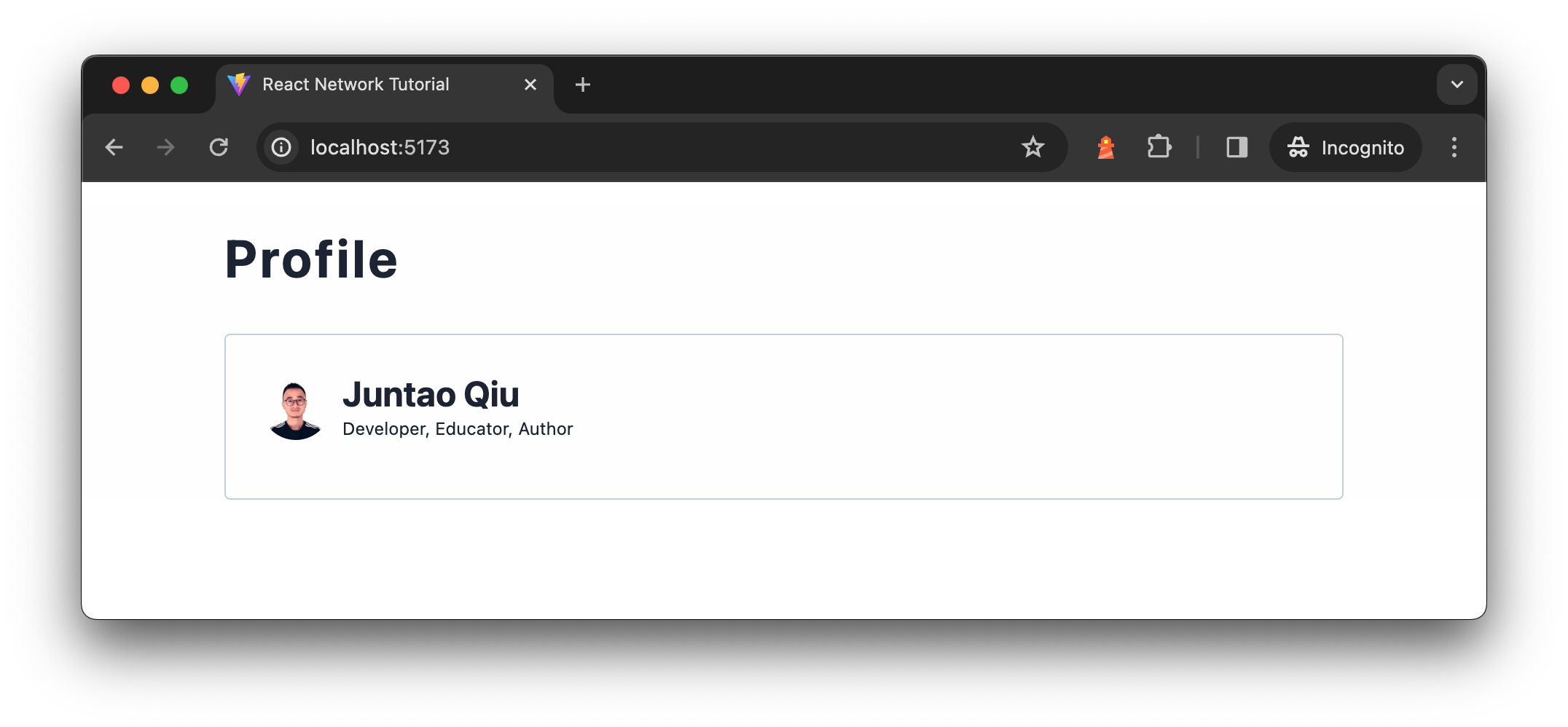
Determine 4: Person temporary element
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
extensively used throughout React codebases. In purposes of normal dimension, it is
frequent to search out quite a few cases of such identical data-fetching logic
dispersed all through varied elements.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls will be gradual, and it is important to not let the UI freeze
whereas these calls are being made. Subsequently, we deal with them asynchronously
and use indicators to point out {that a} course of is underway, which makes the
person expertise higher – understanding that one thing is occurring.
Moreover, distant calls would possibly fail attributable to connection points,
requiring clear communication of those failures to the person. Subsequently,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata in regards to the standing of the decision, enabling it to show
various info or choices if the anticipated outcomes fail to
materialize.
A easy implementation could possibly be a perform getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing info important for managing asynchronous
operations. This setup permits us to appropriately reply to totally different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, knowledge } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the info
The belief right here is that getAsyncStates
initiates the
community request routinely upon being known as. Nonetheless, this won’t
all the time align with the caller’s wants. To supply extra management, we will additionally
expose a fetch
perform inside the returned object, permitting
the initiation of the request at a extra applicable time, in keeping with the
caller’s discretion. Moreover, a refetch
perform may
be supplied to allow the caller to re-initiate the request as wanted,
equivalent to after an error or when up to date knowledge is required. The
fetch
and refetch
features will be an identical in
implementation, or refetch
would possibly embrace logic to examine for
cached outcomes and solely re-fetch knowledge if crucial.
const { loading, error, knowledge, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the info
This sample supplies a flexible method to dealing with asynchronous
requests, giving builders the pliability to set off knowledge fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
purposes can adapt extra dynamically to person interactions and different
runtime situations, enhancing the person expertise and utility
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample will be applied in several frontend libraries. For
occasion, we may distill this method right into a customized Hook in a React
utility for the Profile element:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Person>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-14:Information-Fetching-Patterns-in-Single-Web page-Functions); return { loading, error, person, }; };
Please notice that within the customized Hook, we have no JSX code –
that means it’s very UI free however sharable stateful logic. And the
useUser
launch knowledge routinely when known as. Inside the Profile
element, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, person } = useUser(id); if (loading || !person) { return <div>Loading...</div>; } if (error) { return <div>One thing went improper...</div>; } return ( <> {person && <UserBrief person={person} />} </> ); };
Generalizing Parameter Utilization
In most purposes, fetching various kinds of knowledge—from person
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a typical requirement. Writing separate
fetch features for every kind of information will be tedious and troublesome to
preserve. A greater method is to summary this performance right into a
generic, reusable hook that may deal with varied knowledge varieties
effectively.
Contemplate treating distant API endpoints as providers, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; perform useService<T>(url: string) { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [data, setData] = useState<T | undefined>(); const fetch = async () => { attempt { setLoading(true); const knowledge = await get<T>(url); setData(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, knowledge, fetch, }; }
This hook abstracts the info fetching course of, making it simpler to
combine into any element that should retrieve knowledge from a distant
supply. It additionally centralizes frequent error dealing with situations, equivalent to
treating particular errors in another way:
import { useService } from './useService.ts'; const { loading, error, knowledge: person, fetch: fetchUser, } = useService(`/customers/${id}`);
By utilizing useService, we will simplify how elements fetch and deal with
knowledge, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
could be expose the
fetchUsers
perform, and it doesn’t set off the info
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Person>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, person, fetchUser, }; };
After which on the calling website, Profile
element use
useEffect
to fetch the info and render totally different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, person, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the power to reuse these stateful
logics throughout totally different elements. As an example, one other element
needing the identical knowledge (a person API name with a person ID) can merely import
the useUser
Hook and make the most of its states. Totally different UI
elements would possibly select to work together with these states in varied methods,
maybe utilizing various loading indicators (a smaller spinner that
suits to the calling element) or error messages, but the elemental
logic of fetching knowledge stays constant and shared.
When to make use of it
Separating knowledge fetching logic from UI elements can generally
introduce pointless complexity, notably in smaller purposes.
Preserving this logic built-in inside the element, just like the
css-in-js method, simplifies navigation and is less complicated for some
builders to handle. In my article, Modularizing
React Functions with Established UI Patterns, I explored
varied ranges of complexity in utility buildings. For purposes
which are restricted in scope — with just some pages and a number of other knowledge
fetching operations — it is typically sensible and likewise advisable to
preserve knowledge fetching inside the UI elements.
Nonetheless, as your utility scales and the event group grows,
this technique might result in inefficiencies. Deep element bushes can gradual
down your utility (we are going to see examples in addition to how one can handle
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling knowledge fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to stability simplicity with structured approaches as your
venture evolves. This ensures your improvement practices stay
efficient and attentive to the appliance’s wants, sustaining optimum
efficiency and developer effectivity whatever the venture
scale.
[ad_2]