[ad_1]
Immediately, most functions can ship tons of of requests for a single web page.
For instance, my Twitter house web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
belongings (JavaScript, CSS, font recordsdata, icons, and so on.), however there are nonetheless
round 100 requests for async information fetching – both for timelines, buddies,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The primary cause a web page could comprise so many requests is to enhance
efficiency and consumer expertise, particularly to make the appliance really feel
sooner to the top customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In trendy internet functions, customers usually see a primary web page with
model and different components in lower than a second, with further items
loading progressively.
Take the Amazon product element web page for example. The navigation and prime
bar seem nearly instantly, adopted by the product pictures, temporary, and
descriptions. Then, as you scroll, “Sponsored” content material, rankings,
suggestions, view histories, and extra seem.Usually, a consumer solely needs a
fast look or to check merchandise (and verify availability), making
sections like “Clients who purchased this merchandise additionally purchased” much less crucial and
appropriate for loading through separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, however it’s removed from sufficient in giant
functions. There are a lot of different facets to contemplate in the case of
fetch information accurately and effectively. Information fetching is a chellenging, not
solely as a result of the character of async programming would not match our linear mindset,
and there are such a lot of components could cause a community name to fail, but in addition
there are too many not-obvious circumstances to contemplate beneath the hood (information
format, safety, cache, token expiry, and so on.).
On this article, I wish to talk about some widespread issues and
patterns you must think about in the case of fetching information in your frontend
functions.
We’ll start with the Asynchronous State Handler sample, which decouples
information fetching from the UI, streamlining your software structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your information
fetching logic. To speed up the preliminary information loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Information Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical software components and Prefetching information based mostly on consumer
interactions to raise the consumer expertise.
I imagine discussing these ideas by way of a simple instance is
one of the best method. I purpose to start out merely after which introduce extra complexity
in a manageable manner. I additionally plan to maintain code snippets, significantly for
styling (I am using TailwindCSS for the UI, which can lead to prolonged
snippets in a React element), to a minimal. For these within the
full particulars, I’ve made them out there on this
repository.
Developments are additionally occurring on the server facet, with strategies like
Streaming Server-Aspect Rendering and Server Elements gaining traction in
numerous frameworks. Moreover, a variety of experimental strategies are
rising. Nevertheless, these matters, whereas probably simply as essential, may be
explored in a future article. For now, this dialogue will focus
solely on front-end information fetching patterns.
It is necessary to notice that the strategies we’re overlaying are usually not
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions attributable to my intensive expertise with
it lately. Nevertheless, ideas like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I will share
are widespread eventualities you would possibly encounter in frontend improvement, regardless
of the framework you utilize.
That stated, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display of a Single-Web page Utility. It is a typical
software you may need used earlier than, or no less than the state of affairs is typical.
We have to fetch information from server facet after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the appliance
To start with, on Profile
we’ll present the consumer’s temporary (together with
title, avatar, and a brief description), after which we additionally wish to present
their connections (much like followers on Twitter or LinkedIn
connections). We’ll have to fetch consumer and their connections information from
distant service, after which assembling these information with UI on the display.
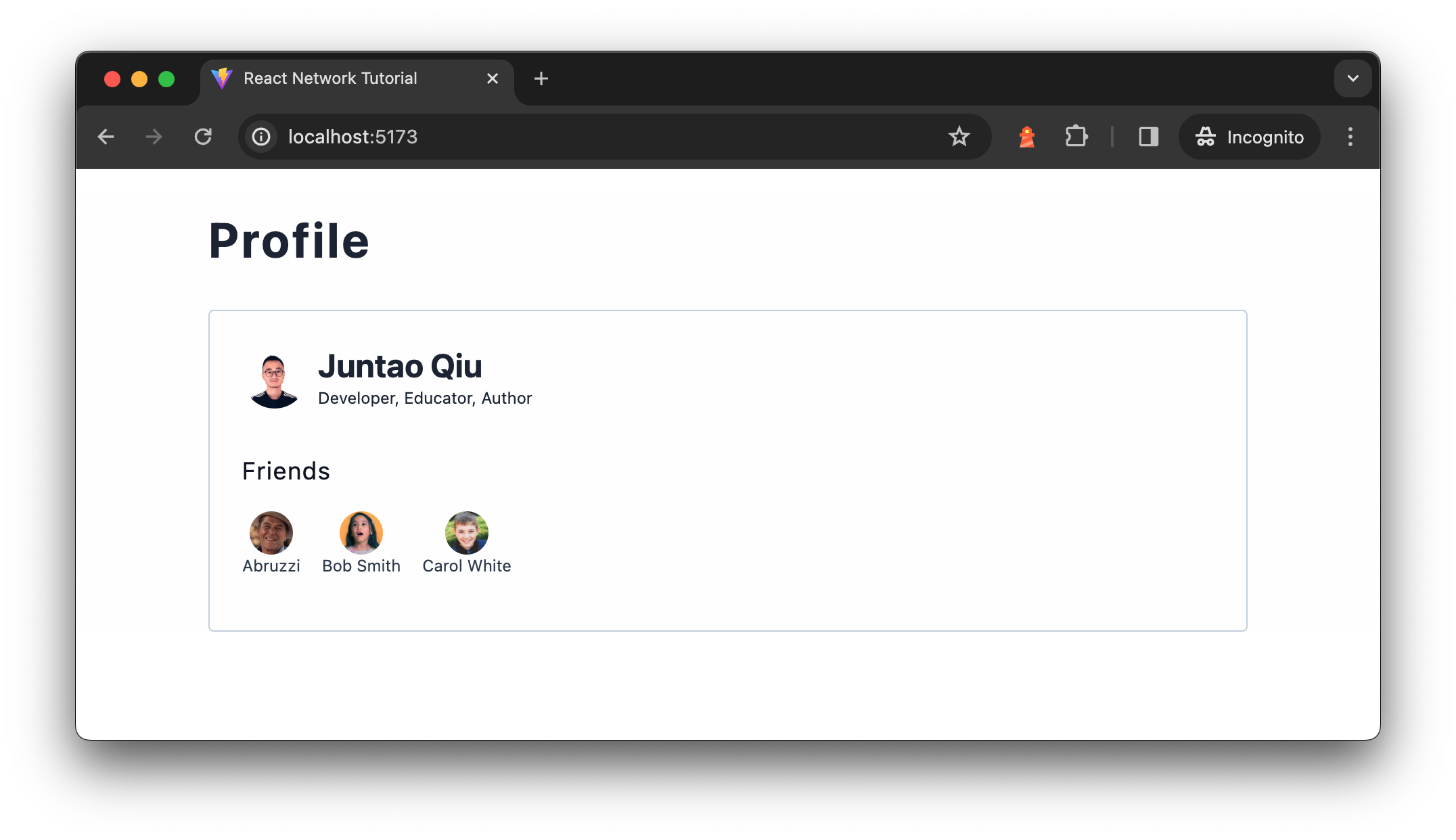
Determine 1: Profile display
The information are from two separate API calls, the consumer temporary API
/customers/<id>
returns consumer temporary for a given consumer id, which is a straightforward
object described as follows:
{ "id": "u1", "title": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the good friend API /customers/<id>/buddies
endpoint returns an inventory of
buddies for a given consumer, every record merchandise within the response is similar as
the above consumer information. The rationale we’ve got two endpoints as a substitute of returning
a buddies
part of the consumer API is that there are circumstances the place one
may have too many buddies (say 1,000), however most individuals do not have many.
This in-balance information construction might be fairly difficult, particularly after we
have to paginate. The purpose right here is that there are circumstances we have to deal
with a number of community requests.
A quick introduction to related React ideas
As this text leverages React for instance numerous patterns, I do
not assume a lot about React. Reasonably than anticipating you to spend so much
of time looking for the fitting components within the React documentation, I’ll
briefly introduce these ideas we will make the most of all through this
article. In case you already perceive what React parts are, and the
use of the
useState
and useEffect
hooks, you might
use this hyperlink to skip forward to the following
part.
For these searching for a extra thorough tutorial, the new React documentation is a wonderful
useful resource.
What’s a React Element?
In React, parts are the elemental constructing blocks. To place it
merely, a React element is a operate that returns a bit of UI,
which might be as easy as a fraction of HTML. Think about the
creation of a element that renders a navigation bar:
import React from 'react'; operate Navigation() { return ( <nav> <ol> <li>House</li> <li>Blogs</li> <li>Books</li> </ol> </nav> ); }
At first look, the combination of JavaScript with HTML tags might sound
unusual (it is known as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, the same syntax known as TSX is used). To make this
code purposeful, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
operate Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "House"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Notice right here the translated code has a operate known as
React.createElement
, which is a foundational operate in
React for creating components. JSX written in React parts is compiled
all the way down to React.createElement
calls behind the scenes.
The fundamental syntax of React.createElement
is:
React.createElement(sort, [props], [...children])
sort
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React element (class or purposeful) for
extra subtle constructions.props
: An object containing properties handed to the
factor or element, together with occasion handlers, types, and attributes
likeclassName
andid
.youngsters
: These non-compulsory arguments might be further
React.createElement
calls, strings, numbers, or any combine
thereof, representing the factor’s youngsters.
As an illustration, a easy factor might be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Whats up, world!');
That is analogous to the JSX model:
<div className="greeting">Whats up, world!</div>
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM components as vital.
You possibly can then assemble your customized parts right into a tree, much like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; operate App() { return <Web page />; } operate Web page() { return <Container> <Navigation /> <Content material> <Sidebar /> <ProductList /> </Content material> <Footer /> </Container>; }
Finally, your software requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/consumer"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render(<App />);
Producing Dynamic Content material with JSX
The preliminary instance demonstrates a simple use case, however
let’s discover how we will create content material dynamically. As an illustration, how
can we generate an inventory of knowledge dynamically? In React, as illustrated
earlier, a element is basically a operate, enabling us to go
parameters to it.
import React from 'react'; operate Navigation({ nav }) { return ( <nav> <ol> {nav.map(merchandise => <li key={merchandise}>{merchandise}</li>)} </ol> </nav> ); }
On this modified Navigation
element, we anticipate the
parameter to be an array of strings. We make the most of the map
operate to iterate over every merchandise, remodeling them into
<li>
components. The curly braces {}
signify
that the enclosed JavaScript expression must be evaluated and
rendered. For these curious in regards to the compiled model of this dynamic
content material dealing with:
operate Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(operate(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As an alternative of invoking Navigation
as a daily operate,
using JSX syntax renders the element invocation extra akin to
writing markup, enhancing readability:
// As an alternative of this Navigation(["Home", "Blogs", "Books"]) // We do that <Navigation nav={["Home", "Blogs", "Books"]} />
Elements in React can obtain various information, referred to as props, to
modify their conduct, very like passing arguments right into a operate (the
distinction lies in utilizing JSX syntax, making the code extra acquainted and
readable to these with HTML data, which aligns properly with the talent
set of most frontend builders).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; operate App() { let showNewOnly = false; // This flag's worth is often set based mostly on particular logic. const filteredBooks = showNewOnly ? booksData.filter(e-book => e-book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks} /> </div> ); }
On this illustrative code snippet (non-functional however meant to
show the idea), we manipulate the BookList
element’s displayed content material by passing it an array of books. Relying
on the showNewOnly
flag, this array is both all out there
books or solely these which might be newly printed, showcasing how props can
be used to dynamically regulate element output.
Managing Inside State Between Renders: useState
Constructing consumer interfaces (UI) typically transcends the technology of
static HTML. Elements often have to “keep in mind” sure states and
reply to consumer interactions dynamically. As an illustration, when a consumer
clicks an “Add” button in a Product element, it is necessary to replace
the ShoppingCart element to mirror each the overall worth and the
up to date merchandise record.
Within the earlier code snippet, making an attempt to set the
showNewOnly
variable to true
inside an occasion
handler doesn’t obtain the specified impact:
operate App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this does not work }; const filteredBooks = showNewOnly ? booksData.filter(e-book => e-book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
This method falls quick as a result of native variables inside a operate
element don’t persist between renders. When React re-renders this
element, it does so from scratch, disregarding any modifications made to
native variables since these don’t set off re-renders. React stays
unaware of the necessity to replace the element to mirror new information.
This limitation underscores the need for React’s
state
. Particularly, purposeful parts leverage the
useState
hook to recollect states throughout renders. Revisiting
the App
instance, we will successfully keep in mind the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; operate App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(e-book => e-book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow purposeful parts to handle inside state. It
introduces state to purposeful parts, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra complicated object or array. The
initialState
is simply used through the first render to
initialize the state.- Return Worth:
useState
returns an array with
two components. The primary factor is the present state worth, and the
second factor is a operate that permits updating this worth. Through the use of
array destructuring, we assign names to those returned gadgets,
usuallystate
andsetState
, although you’ll be able to
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that might be used within the element’s UI and
logic.setState
: A operate to replace the state. This operate
accepts a brand new state worth or a operate that produces a brand new state based mostly
on the earlier state. When known as, it schedules an replace to the
element’s state and triggers a re-render to mirror the modifications.
React treats state as a snapshot; updating it would not alter the
present state variable however as a substitute triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, making certain the
BookList
element receives the proper information, thereby
reflecting the up to date e-book record to the consumer. This snapshot-like
conduct of state facilitates the dynamic and responsive nature of React
parts, enabling them to react intuitively to consumer interactions and
different modifications.
Managing Aspect Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to handle the
idea of unintended effects. Uncomfortable side effects are operations that work together with
the surface world from the React ecosystem. Frequent examples embrace
fetching information from a distant server or dynamically manipulating the DOM,
corresponding to altering the web page title.
React is primarily involved with rendering information to the DOM and does
not inherently deal with information fetching or direct DOM manipulation. To
facilitate these unintended effects, React offers the useEffect
hook. This hook permits the execution of unintended effects after React has
accomplished its rendering course of. If these unintended effects end in information
modifications, React schedules a re-render to mirror these updates.
The useEffect
Hook accepts two arguments:
- A operate containing the facet impact logic.
- An non-compulsory dependency array specifying when the facet impact must be
re-invoked.
Omitting the second argument causes the facet impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t rely upon any values from props or state, thus not needing to
re-run. Together with particular values within the array means the facet impact
solely re-executes if these values change.
When coping with asynchronous information fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the information is
retrieved, it’s captured through the useState
hook, updating the
element’s inside state and preserving the fetched information throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new information.
Here is a sensible instance about information fetching and state
administration:
import { useEffect, useState } from "react"; sort Consumer = { id: string; title: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Information-Fetching); return <div> <h2>{consumer?.title}</h2> </div>; };
Within the code snippet above, inside useEffect
, an
asynchronous operate fetchUser
is outlined after which
instantly invoked. This sample is important as a result of
useEffect
doesn’t straight assist async capabilities as its
callback. The async operate is outlined to make use of await
for
the fetch operation, making certain that the code execution waits for the
response after which processes the JSON information. As soon as the information is on the market,
it updates the element’s state through setUser
.
The dependency array tag:martinfowler.com,2024-05-15:Parallel-Information-Fetching
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
modifications, which prevents pointless community requests on
each render and fetches new consumer information when the id
prop
updates.
This method to dealing with asynchronous information fetching inside
useEffect
is an ordinary follow in React improvement, providing a
structured and environment friendly solution to combine async operations into the
React element lifecycle.
As well as, in sensible functions, managing completely different states
corresponding to loading, error, and information presentation is important too (we’ll
see it the way it works within the following part). For instance, think about
implementing standing indicators inside a Consumer element to mirror
loading, error, or information states, enhancing the consumer expertise by
offering suggestions throughout information fetching operations.
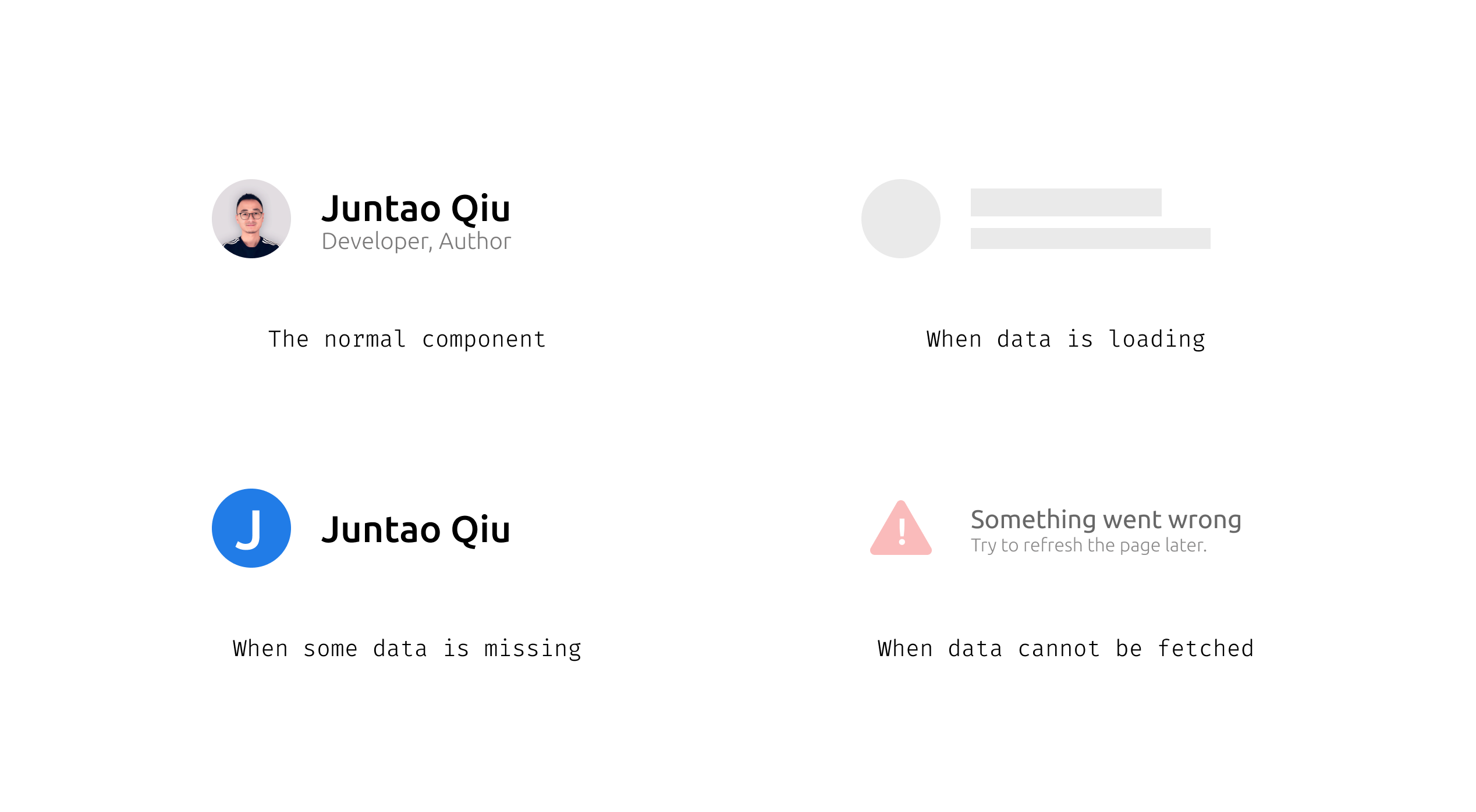
Determine 2: Totally different statuses of a
element
This overview affords only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into further ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line assets.
With this basis, you must now be geared up to hitch me as we delve
into the information fetching patterns mentioned herein.
Implement the Profile element
Let’s create the Profile
element to make a request and
render the outcome. In typical React functions, this information fetching is
dealt with inside a useEffect
block. Here is an instance of how
this may be applied:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Information-Fetching); return ( <UserBrief consumer={consumer} /> ); };
This preliminary method assumes community requests full
instantaneously, which is usually not the case. Actual-world eventualities require
dealing with various community circumstances, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
element. This addition permits us to offer suggestions to the consumer throughout
information fetching, corresponding to displaying a loading indicator or a skeleton display
if the information is delayed, and dealing with errors once they happen.
Right here’s how the improved element appears with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import sort { Consumer } from "../sorts.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Information-Fetching); if (loading || !consumer) { return <div>Loading...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Now in Profile
element, we provoke states for loading,
errors, and consumer information with useState
. Utilizing
useEffect
, we fetch consumer information based mostly on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
information retrieval, we replace the consumer state, else show a loading
indicator.
The get
operate, as demonstrated under, simplifies
fetching information from a selected endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON information or throws an error for unsuccessful requests,
streamlining error dealing with and information retrieval in our software. Notice
it is pure TypeScript code and can be utilized in different non-React components of the
software.
const baseurl = "https://icodeit.com.au/api/v2"; async operate get<T>(url: string): Promise<T> { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise<T>; }
React will attempt to render the element initially, however as the information
consumer
isn’t out there, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as in some unspecified time in the future, the response returns, React
re-renders the Profile
element with consumer
fulfilled, so now you can see the consumer part with title, avatar, and
title.
If we visualize the timeline of the above code, you will notice
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and elegance tags, it would cease and
obtain these recordsdata, after which parse them to kind the ultimate web page. Notice
that this can be a comparatively difficult course of, and I’m oversimplifying
right here, however the primary concept of the sequence is right.
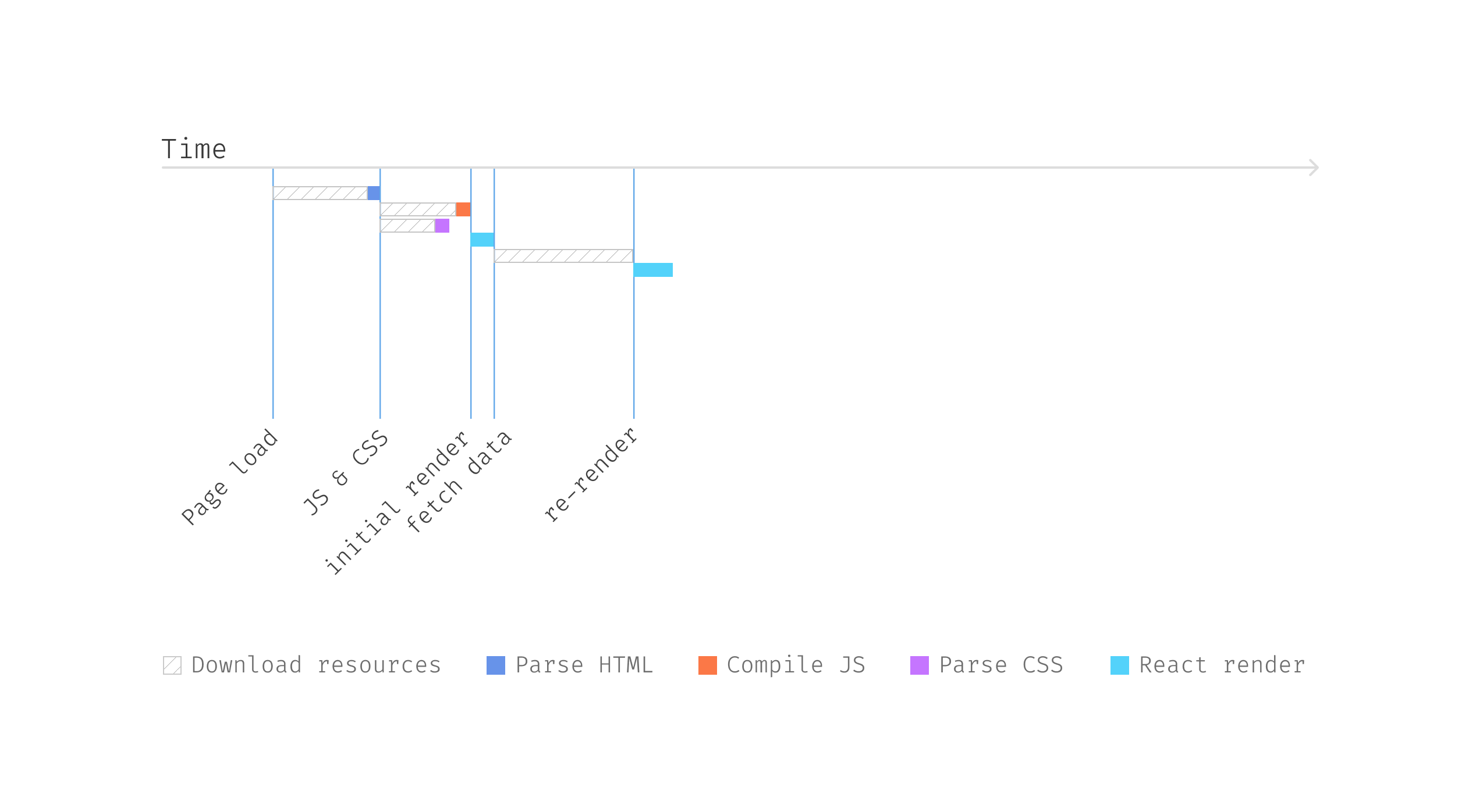
Determine 3: Fetching consumer
information
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for information fetching; it has to attend till
the information is on the market for a re-render.
Now within the browser, we will see a “loading…” when the appliance
begins, after which after just a few seconds (we will simulate such case by add
some delay within the API endpoints) the consumer temporary part reveals up when information
is loaded.
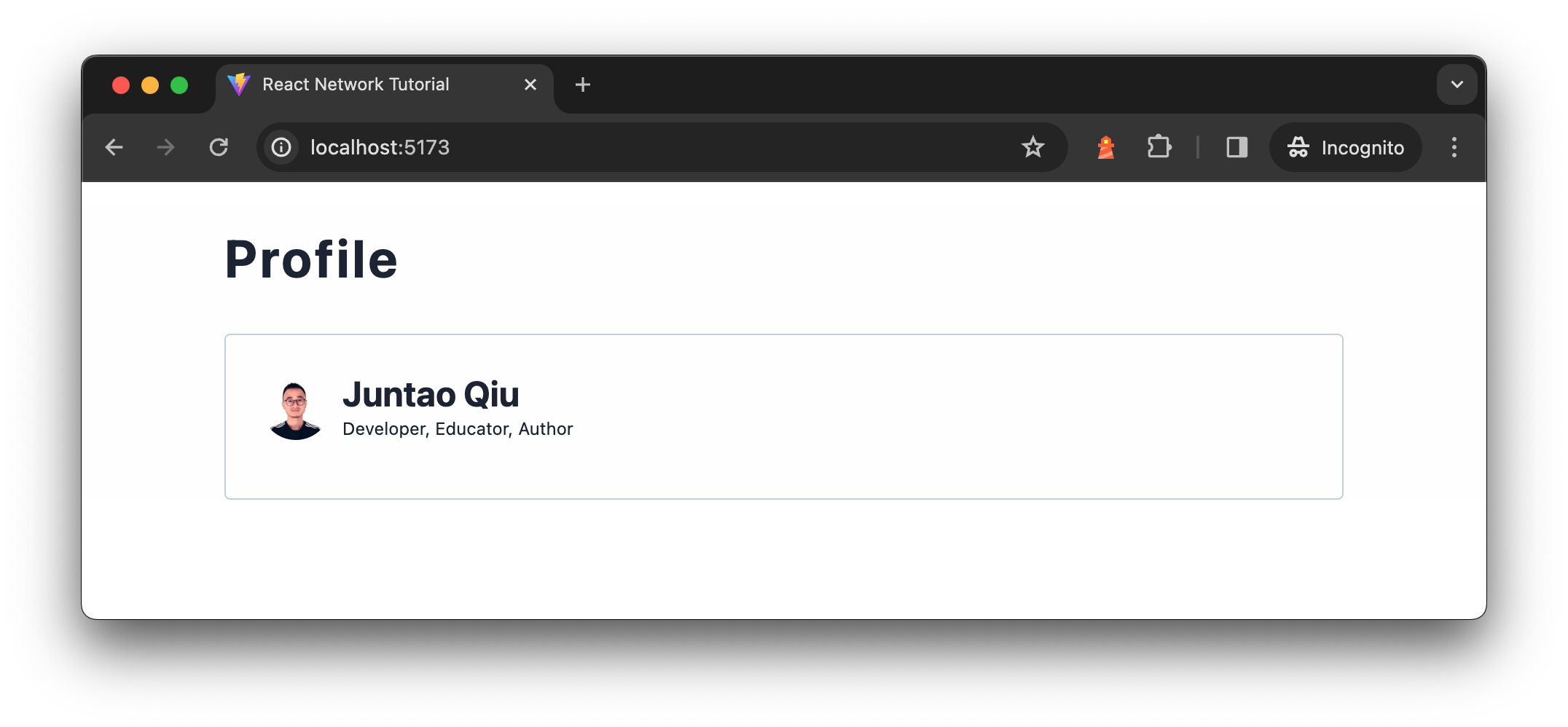
Determine 4: Consumer temporary element
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
broadly used throughout React codebases. In functions of standard dimension, it is
widespread to search out quite a few situations of such similar data-fetching logic
dispersed all through numerous parts.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls might be gradual, and it is important to not let the UI freeze
whereas these calls are being made. Due to this fact, we deal with them asynchronously
and use indicators to indicate {that a} course of is underway, which makes the
consumer expertise higher – realizing that one thing is occurring.
Moreover, distant calls would possibly fail attributable to connection points,
requiring clear communication of those failures to the consumer. Due to this fact,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata in regards to the standing of the decision, enabling it to show
different data or choices if the anticipated outcomes fail to
materialize.
A easy implementation could possibly be a operate getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing data important for managing asynchronous
operations. This setup permits us to appropriately reply to completely different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, information } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
The idea right here is that getAsyncStates
initiates the
community request robotically upon being known as. Nevertheless, this won’t
at all times align with the caller’s wants. To supply extra management, we will additionally
expose a fetch
operate throughout the returned object, permitting
the initiation of the request at a extra applicable time, in response to the
caller’s discretion. Moreover, a refetch
operate may
be offered to allow the caller to re-initiate the request as wanted,
corresponding to after an error or when up to date information is required. The
fetch
and refetch
capabilities might be similar in
implementation, or refetch
would possibly embrace logic to verify for
cached outcomes and solely re-fetch information if vital.
const { loading, error, information, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
This sample offers a flexible method to dealing with asynchronous
requests, giving builders the pliability to set off information fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
functions can adapt extra dynamically to consumer interactions and different
runtime circumstances, enhancing the consumer expertise and software
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample might be applied in several frontend libraries. For
occasion, we may distill this method right into a customized Hook in a React
software for the Profile element:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-15:Parallel-Information-Fetching); return { loading, error, consumer, }; };
Please be aware that within the customized Hook, we have no JSX code –
that means it’s very UI free however sharable stateful logic. And the
useUser
launch information robotically when known as. Throughout the Profile
element, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, consumer } = useUser(id); if (loading || !consumer) { return <div>Loading...</div>; } if (error) { return <div>One thing went flawed...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Generalizing Parameter Utilization
In most functions, fetching various kinds of information—from consumer
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a standard requirement. Writing separate
fetch capabilities for every sort of knowledge might be tedious and tough to
preserve. A greater method is to summary this performance right into a
generic, reusable hook that may deal with numerous information sorts
effectively.
Think about treating distant API endpoints as providers, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; operate useService<T>(url: string) { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [data, setData] = useState<T | undefined>(); const fetch = async () => { attempt { setLoading(true); const information = await get<T>(url); setData(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, information, fetch, }; }
This hook abstracts the information fetching course of, making it simpler to
combine into any element that should retrieve information from a distant
supply. It additionally centralizes widespread error dealing with eventualities, corresponding to
treating particular errors in a different way:
import { useService } from './useService.ts'; const { loading, error, information: consumer, fetch: fetchUser, } = useService(`/customers/${id}`);
Through the use of useService, we will simplify how parts fetch and deal with
information, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
could be expose the
fetchUsers
operate, and it doesn’t set off the information
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { attempt { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, consumer, fetchUser, }; };
After which on the calling website, Profile
element use
useEffect
to fetch the information and render completely different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, consumer, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the power to reuse these stateful
logics throughout completely different parts. As an illustration, one other element
needing the identical information (a consumer API name with a consumer ID) can merely import
the useUser
Hook and make the most of its states. Totally different UI
parts would possibly select to work together with these states in numerous methods,
maybe utilizing different loading indicators (a smaller spinner that
matches to the calling element) or error messages, but the elemental
logic of fetching information stays constant and shared.
When to make use of it
Separating information fetching logic from UI parts can typically
introduce pointless complexity, significantly in smaller functions.
Holding this logic built-in throughout the element, much like the
css-in-js method, simplifies navigation and is simpler for some
builders to handle. In my article, Modularizing
React Purposes with Established UI Patterns, I explored
numerous ranges of complexity in software constructions. For functions
which might be restricted in scope — with just some pages and several other information
fetching operations — it is typically sensible and in addition really helpful to
preserve information fetching inside the UI parts.
Nevertheless, as your software scales and the event crew grows,
this technique could result in inefficiencies. Deep element bushes can gradual
down your software (we are going to see examples in addition to how you can handle
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling information fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to stability simplicity with structured approaches as your
undertaking evolves. This ensures your improvement practices stay
efficient and aware of the appliance’s wants, sustaining optimum
efficiency and developer effectivity whatever the undertaking
scale.
Implement the Pals record
Now let’s take a look on the second part of the Profile – the good friend
record. We are able to create a separate element Pals
and fetch information in it
(through the use of a useService customized hook we outlined above), and the logic is
fairly much like what we see above within the Profile
element.
const Pals = ({ id }: { id: string }) => { const { loading, error, information: buddies } = useService(`/customers/${id}/buddies`); // loading & error dealing with... return ( <div> <h2>Pals</h2> <div> {buddies.map((consumer) => ( // render consumer record ))} </div> </div> ); };
After which within the Profile element, we will use Pals as a daily
element, and go in id
as a prop:
const Profile = ({ id }: { id: string }) => { //... return ( <> {consumer && <UserBrief consumer={consumer} />} <Pals id={id} /> </> ); };
The code works high-quality, and it appears fairly clear and readable,
UserBrief
renders a consumer
object handed in, whereas
Pals
handle its personal information fetching and rendering logic
altogether. If we visualize the element tree, it might be one thing like
this:
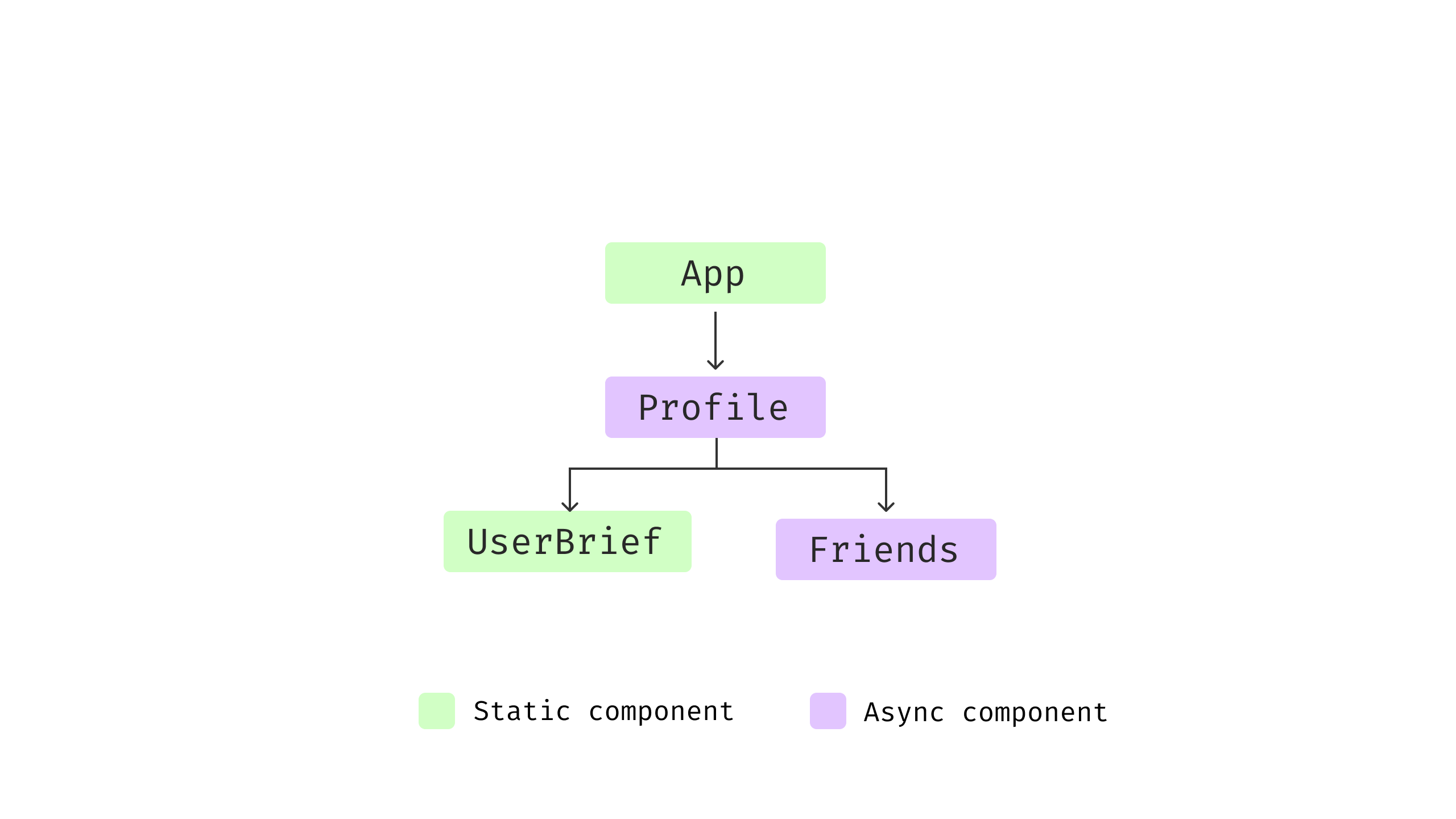
Determine 5: Element construction
Each the Profile
and Pals
have logic for
information fetching, loading checks, and error dealing with. Since there are two
separate information fetching calls, and if we take a look at the request timeline, we
will discover one thing fascinating.
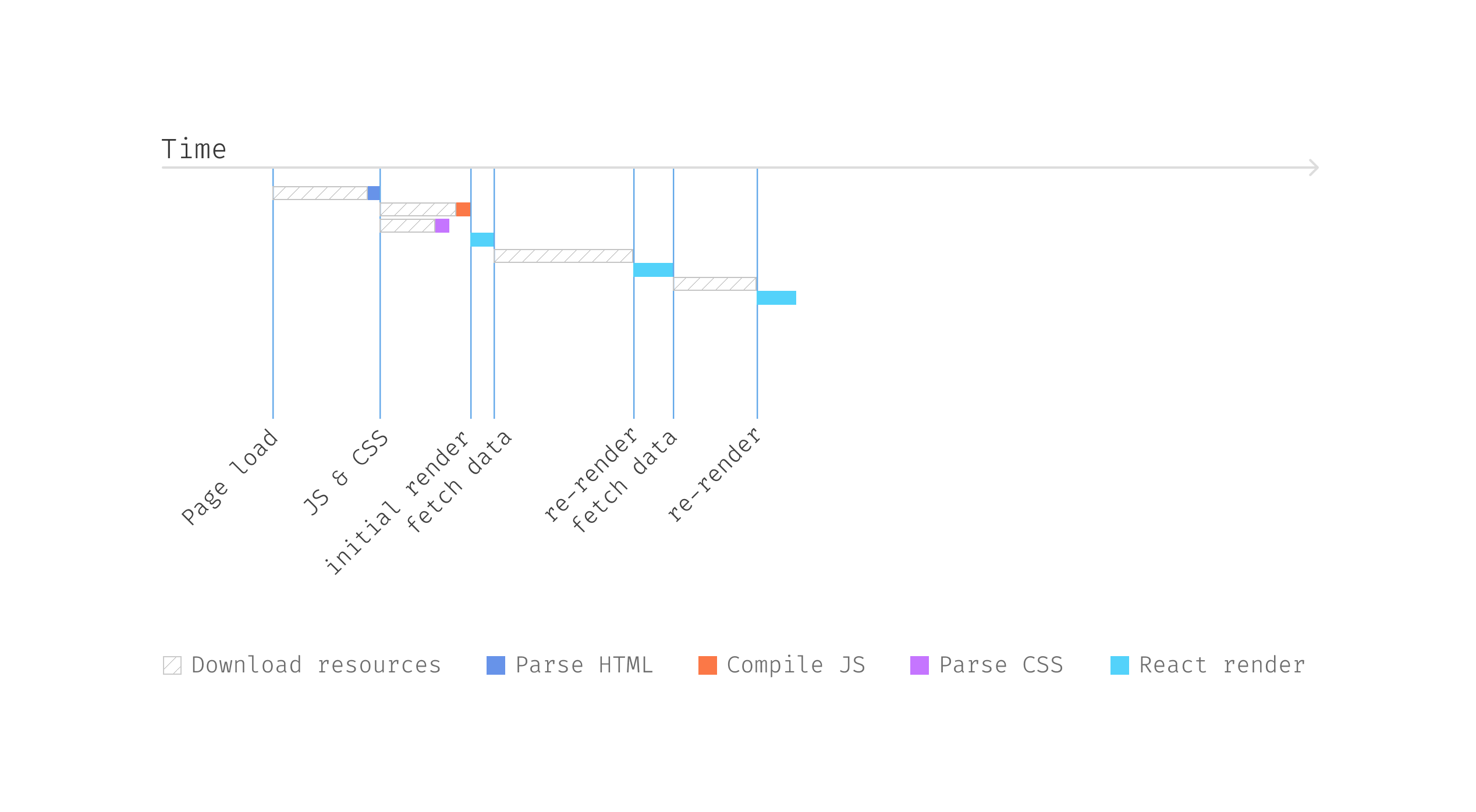
Determine 6: Request waterfall
The Pals
element will not provoke information fetching till the consumer
state is about. That is known as the Fetch-On-Render method,
the place the preliminary rendering is paused as a result of the information is not out there,
requiring React to attend for the information to be retrieved from the server
facet.
This ready interval is considerably inefficient, contemplating that whereas
React’s rendering course of solely takes just a few milliseconds, information fetching can
take considerably longer, typically seconds. Consequently, the Pals
element spends most of its time idle, ready for information. This state of affairs
results in a standard problem referred to as the Request Waterfall, a frequent
prevalence in frontend functions that contain a number of information fetching
operations.
Parallel Information Fetching
Run distant information fetches in parallel to reduce wait time
Think about after we construct a bigger software {that a} element that
requires information might be deeply nested within the element tree, to make the
matter worse these parts are developed by completely different groups, it’s exhausting
to see whom we’re blocking.
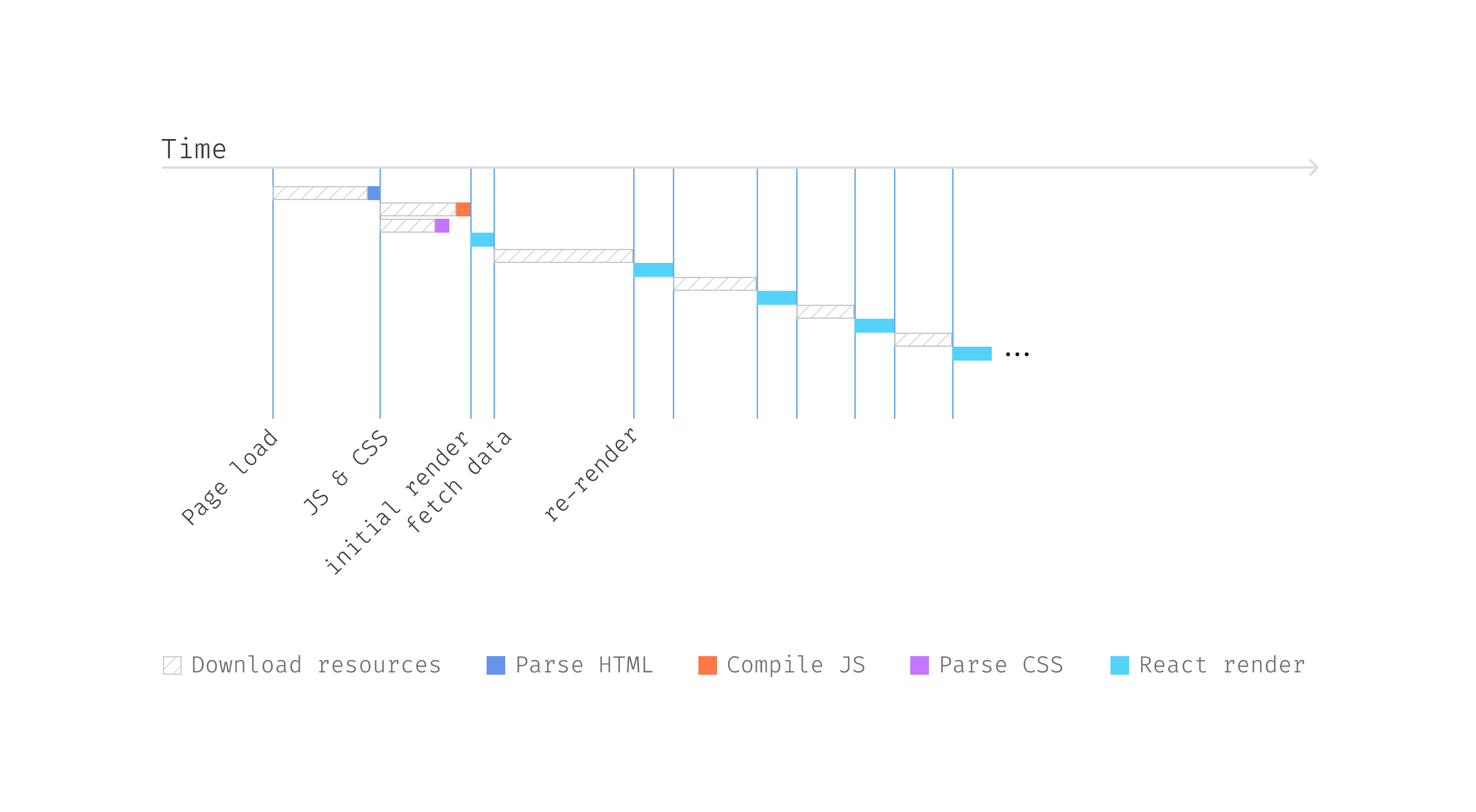
Determine 7: Request waterfall
Request Waterfalls can degrade consumer
expertise, one thing we purpose to keep away from. Analyzing the information, we see that the
consumer API and buddies API are unbiased and might be fetched in parallel.
Initiating these parallel requests turns into crucial for software
efficiency.
One method is to centralize information fetching at the next stage, close to the
root. Early within the software’s lifecycle, we begin all information fetches
concurrently. Elements depending on this information wait just for the
slowest request, usually leading to sooner general load instances.
We may use the Promise API Promise.all
to ship
each requests for the consumer’s primary data and their buddies record.
Promise.all
is a JavaScript technique that permits for the
concurrent execution of a number of guarantees. It takes an array of guarantees
as enter and returns a single Promise that resolves when the entire enter
guarantees have resolved, offering their outcomes as an array. If any of the
guarantees fail, Promise.all
instantly rejects with the
cause of the primary promise that rejects.
As an illustration, on the software’s root, we will outline a complete
information mannequin:
sort ProfileState = { consumer: Consumer; buddies: Consumer[]; }; const getProfileData = async (id: string) => Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/buddies`), ]); const App = () => { // fetch information on the very begining of the appliance launch const onInit = () => { const [user, friends] = await getProfileData(id); } // render the sub tree correspondingly }
Implementing Parallel Information Fetching in React
Upon software launch, information fetching begins, abstracting the
fetching course of from subcomponents. For instance, in Profile element,
each UserBrief and Pals are presentational parts that react to
the handed information. This manner we may develop these element individually
(including types for various states, for instance). These presentational
parts usually are simple to check and modify as we’ve got separate the
information fetching and rendering.
We are able to outline a customized hook useProfileData
that facilitates
parallel fetching of knowledge associated to a consumer and their buddies through the use of
Promise.all
. This technique permits simultaneous requests, optimizing the
loading course of and structuring the information right into a predefined format recognized
as ProfileData
.
Right here’s a breakdown of the hook implementation:
import { useCallback, useEffect, useState } from "react"; sort ProfileData = { consumer: Consumer; buddies: Consumer[]; }; const useProfileData = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(undefined); const [profileState, setProfileState] = useState<ProfileData>(); const fetchProfileState = useCallback(async () => { attempt { setLoading(true); const [user, friends] = await Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/buddies`), ]); setProfileState({ consumer, buddies }); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }, tag:martinfowler.com,2024-05-15:Parallel-Information-Fetching); return { loading, error, profileState, fetchProfileState, }; };
This hook offers the Profile
element with the
vital information states (loading
, error
,
profileState
) together with a fetchProfileState
operate, enabling the element to provoke the fetch operation as
wanted. Notice right here we use useCallback
hook to wrap the async
operate for information fetching. The useCallback hook in React is used to
memoize capabilities, making certain that the identical operate occasion is
maintained throughout element re-renders except its dependencies change.
Much like the useEffect, it accepts the operate and a dependency
array, the operate will solely be recreated if any of those dependencies
change, thereby avoiding unintended conduct in React’s rendering
cycle.
The Profile
element makes use of this hook and controls the information fetching
timing through useEffect
:
const Profile = ({ id }: { id: string }) => { const { loading, error, profileState, fetchProfileState } = useProfileData(id); useEffect(() => { fetchProfileState(); }, [fetchProfileState]); if (loading) { return <div>Loading...</div>; } if (error) { return <div>One thing went flawed...</div>; } return ( <> {profileState && ( <> <UserBrief consumer={profileState.consumer} /> <Pals customers={profileState.buddies} /> </> )} </> ); };
This method is often known as Fetch-Then-Render, suggesting that the purpose
is to provoke requests as early as potential throughout web page load.
Subsequently, the fetched information is utilized to drive React’s rendering of
the appliance, bypassing the necessity to handle information fetching amidst the
rendering course of. This technique simplifies the rendering course of,
making the code simpler to check and modify.
And the element construction, if visualized, could be just like the
following illustration
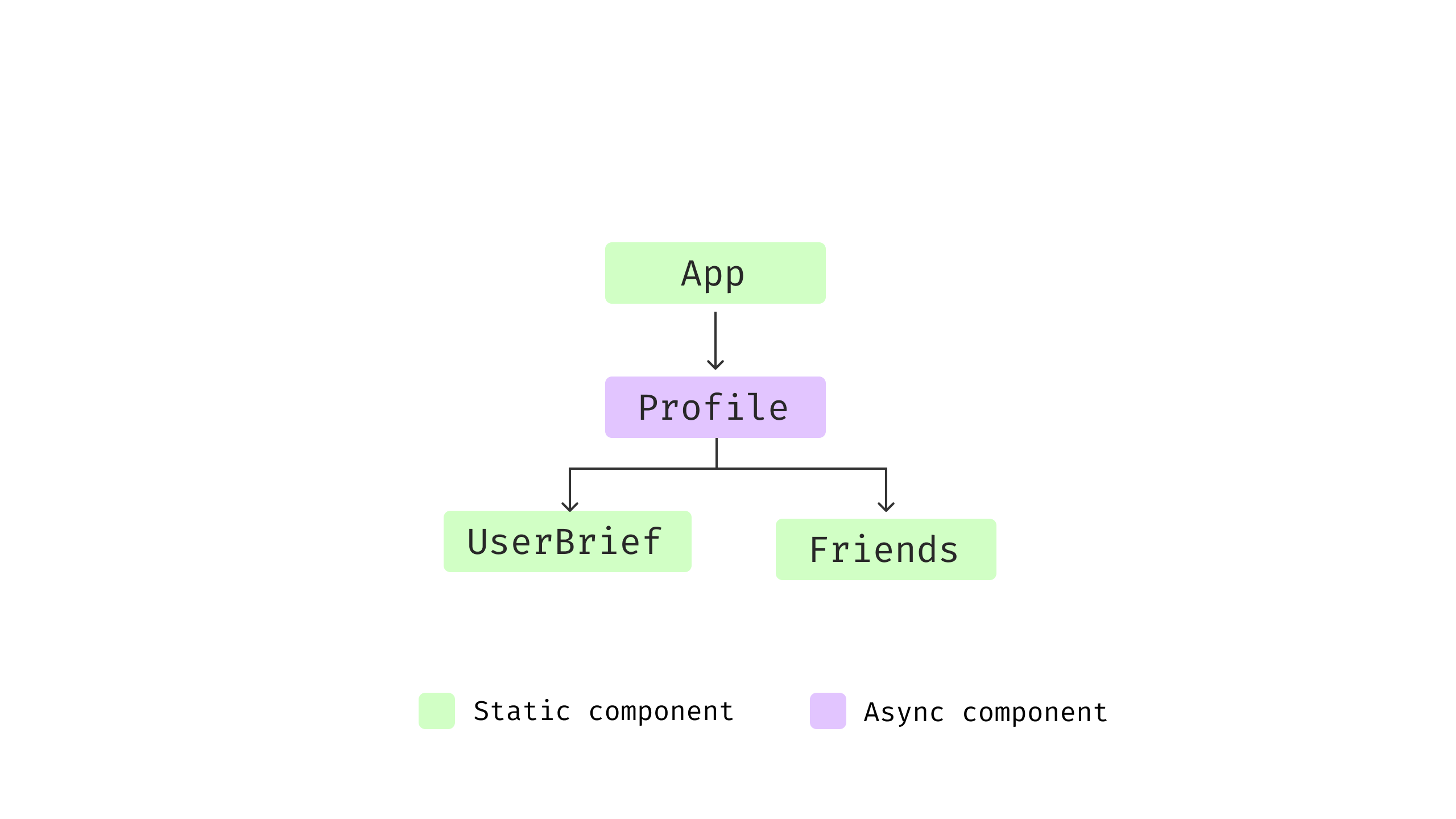
Determine 8: Element construction after refactoring
And the timeline is way shorter than the earlier one as we ship two
requests in parallel. The Pals
element can render in just a few
milliseconds as when it begins to render, the information is already prepared and
handed in.
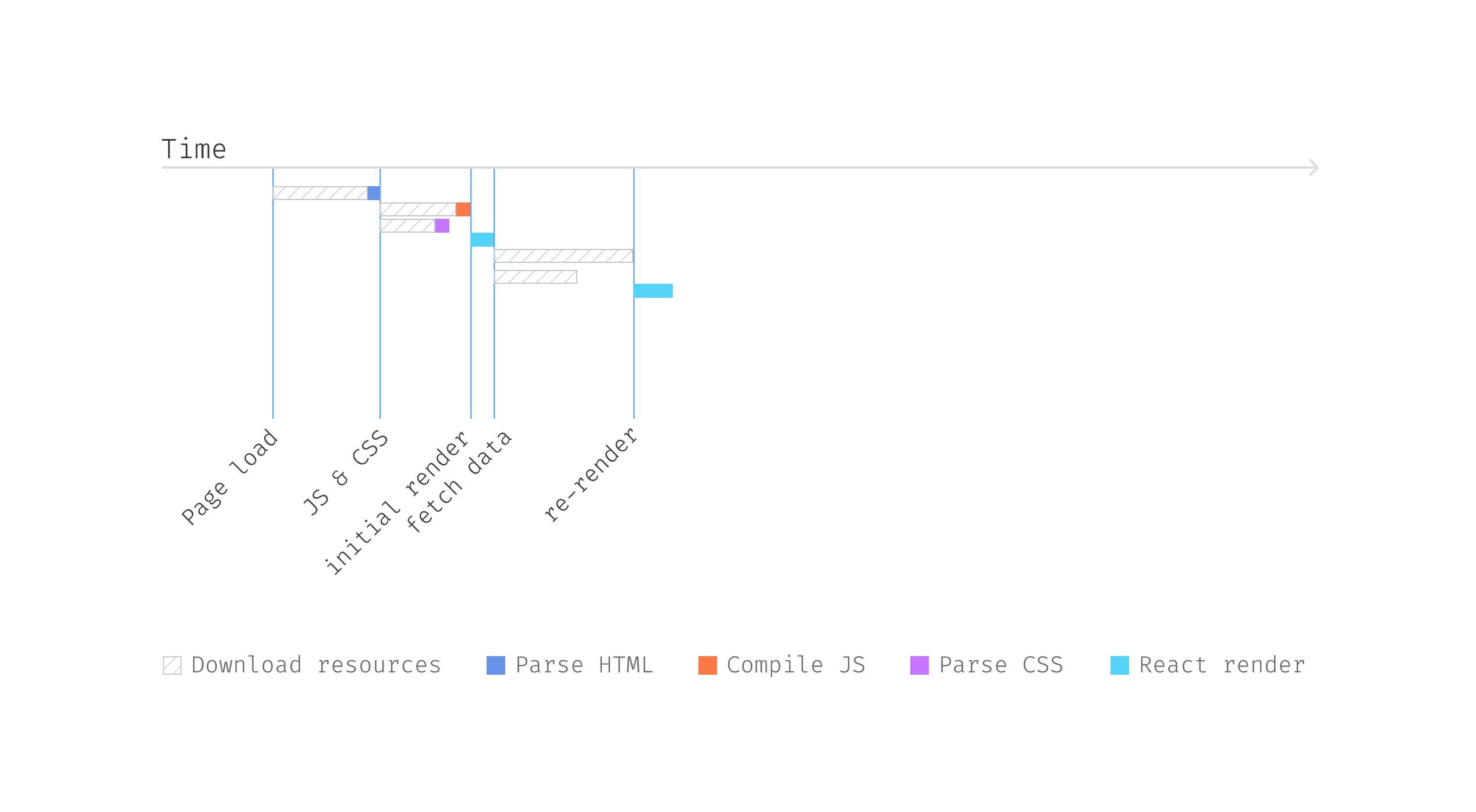
Determine 9: Parallel requests
Notice that the longest wait time will depend on the slowest community
request, which is way sooner than the sequential ones. And if we may
ship as many of those unbiased requests on the similar time at an higher
stage of the element tree, a greater consumer expertise might be
anticipated.
As functions broaden, managing an growing variety of requests at
root stage turns into difficult. That is significantly true for parts
distant from the basis, the place passing down information turns into cumbersome. One
method is to retailer all information globally, accessible through capabilities (like
Redux or the React Context API), avoiding deep prop drilling.
When to make use of it
Working queries in parallel is beneficial at any time when such queries could also be
gradual and do not considerably intrude with every others’ efficiency.
That is normally the case with distant queries. Even when the distant
machine’s I/O and computation is quick, there’s at all times potential latency
points within the distant calls. The primary drawback for parallel queries
is setting them up with some type of asynchronous mechanism, which can be
tough in some language environments.
The primary cause to not use parallel information fetching is after we do not
know what information must be fetched till we have already fetched some
information. Sure eventualities require sequential information fetching attributable to
dependencies between requests. As an illustration, think about a state of affairs on a
Profile
web page the place producing a personalised advice feed
will depend on first buying the consumer’s pursuits from a consumer API.
Here is an instance response from the consumer API that features
pursuits:
{ "id": "u1", "title": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
In such circumstances, the advice feed can solely be fetched after
receiving the consumer’s pursuits from the preliminary API name. This
sequential dependency prevents us from using parallel fetching, as
the second request depends on information obtained from the primary.
Given these constraints, it turns into necessary to debate different
methods in asynchronous information administration. One such technique is
Fallback Markup. This method permits builders to specify what
information is required and the way it must be fetched in a manner that clearly
defines dependencies, making it simpler to handle complicated information
relationships in an software.
One other instance of when arallel Information Fetching isn’t relevant is
that in eventualities involving consumer interactions that require real-time
information validation.
Think about the case of an inventory the place every merchandise has an “Approve” context
menu. When a consumer clicks on the “Approve” choice for an merchandise, a dropdown
menu seems providing decisions to both “Approve” or “Reject.” If this
merchandise’s approval standing could possibly be modified by one other admin concurrently,
then the menu choices should mirror essentially the most present state to keep away from
conflicting actions.
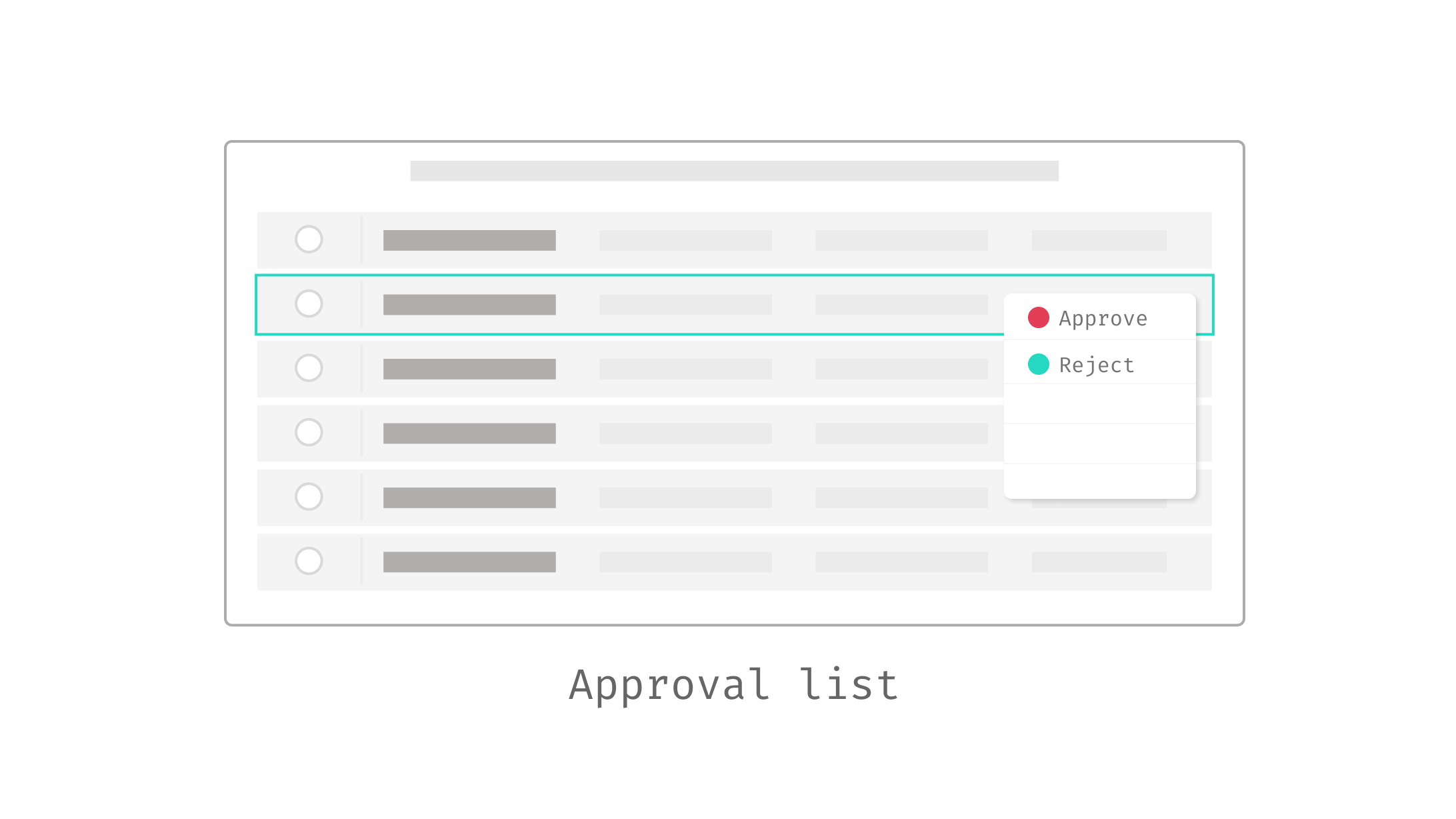
Determine 10: The approval record that require in-time
states
To deal with this, a service name is initiated every time the context
menu is activated. This service fetches the newest standing of the merchandise,
making certain that the dropdown is constructed with essentially the most correct and
present choices out there at that second. Consequently, these requests
can’t be made in parallel with different data-fetching actions for the reason that
dropdown’s contents rely fully on the real-time standing fetched from
the server.
[ad_2]