[ad_1]
Right this moment, most purposes can ship a whole bunch of requests for a single web page.
For instance, my Twitter house web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
belongings (JavaScript, CSS, font recordsdata, icons, and so on.), however there are nonetheless
round 100 requests for async information fetching – both for timelines, pals,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The primary motive a web page might include so many requests is to enhance
efficiency and person expertise, particularly to make the applying really feel
quicker to the top customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In trendy internet purposes, customers sometimes see a primary web page with
type and different parts in lower than a second, with further items
loading progressively.
Take the Amazon product element web page for instance. The navigation and prime
bar seem nearly instantly, adopted by the product photos, temporary, and
descriptions. Then, as you scroll, “Sponsored” content material, scores,
suggestions, view histories, and extra seem.Usually, a person solely desires a
fast look or to match merchandise (and verify availability), making
sections like “Prospects who purchased this merchandise additionally purchased” much less crucial and
appropriate for loading through separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, however it’s removed from sufficient in giant
purposes. There are various different points to think about in terms of
fetch information appropriately and effectively. Information fetching is a chellenging, not
solely as a result of the character of async programming would not match our linear mindset,
and there are such a lot of components may cause a community name to fail, but in addition
there are too many not-obvious instances to think about below the hood (information
format, safety, cache, token expiry, and so on.).
On this article, I wish to talk about some frequent issues and
patterns you must take into account in terms of fetching information in your frontend
purposes.
We’ll start with the Asynchronous State Handler sample, which decouples
information fetching from the UI, streamlining your utility structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your information
fetching logic. To speed up the preliminary information loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Information Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical utility components and Prefetching information based mostly on person
interactions to raise the person expertise.
I imagine discussing these ideas by means of a simple instance is
the perfect method. I purpose to start out merely after which introduce extra complexity
in a manageable approach. I additionally plan to maintain code snippets, significantly for
styling (I am using TailwindCSS for the UI, which can lead to prolonged
snippets in a React part), to a minimal. For these within the
full particulars, I’ve made them obtainable on this
repository.
Developments are additionally taking place on the server aspect, with strategies like
Streaming Server-Aspect Rendering and Server Parts gaining traction in
varied frameworks. Moreover, a lot of experimental strategies are
rising. Nonetheless, these subjects, whereas probably simply as essential, may be
explored in a future article. For now, this dialogue will focus
solely on front-end information fetching patterns.
It is essential to notice that the strategies we’re masking are usually not
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions attributable to my intensive expertise with
it in recent times. Nonetheless, ideas like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I am going to share
are frequent eventualities you would possibly encounter in frontend growth, regardless
of the framework you employ.
That mentioned, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display screen of a Single-Web page Software. It is a typical
utility you may need used earlier than, or not less than the state of affairs is typical.
We have to fetch information from server aspect after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the applying
To start with, on Profile
we’ll present the person’s temporary (together with
identify, avatar, and a brief description), after which we additionally need to present
their connections (just like followers on Twitter or LinkedIn
connections). We’ll have to fetch person and their connections information from
distant service, after which assembling these information with UI on the display screen.
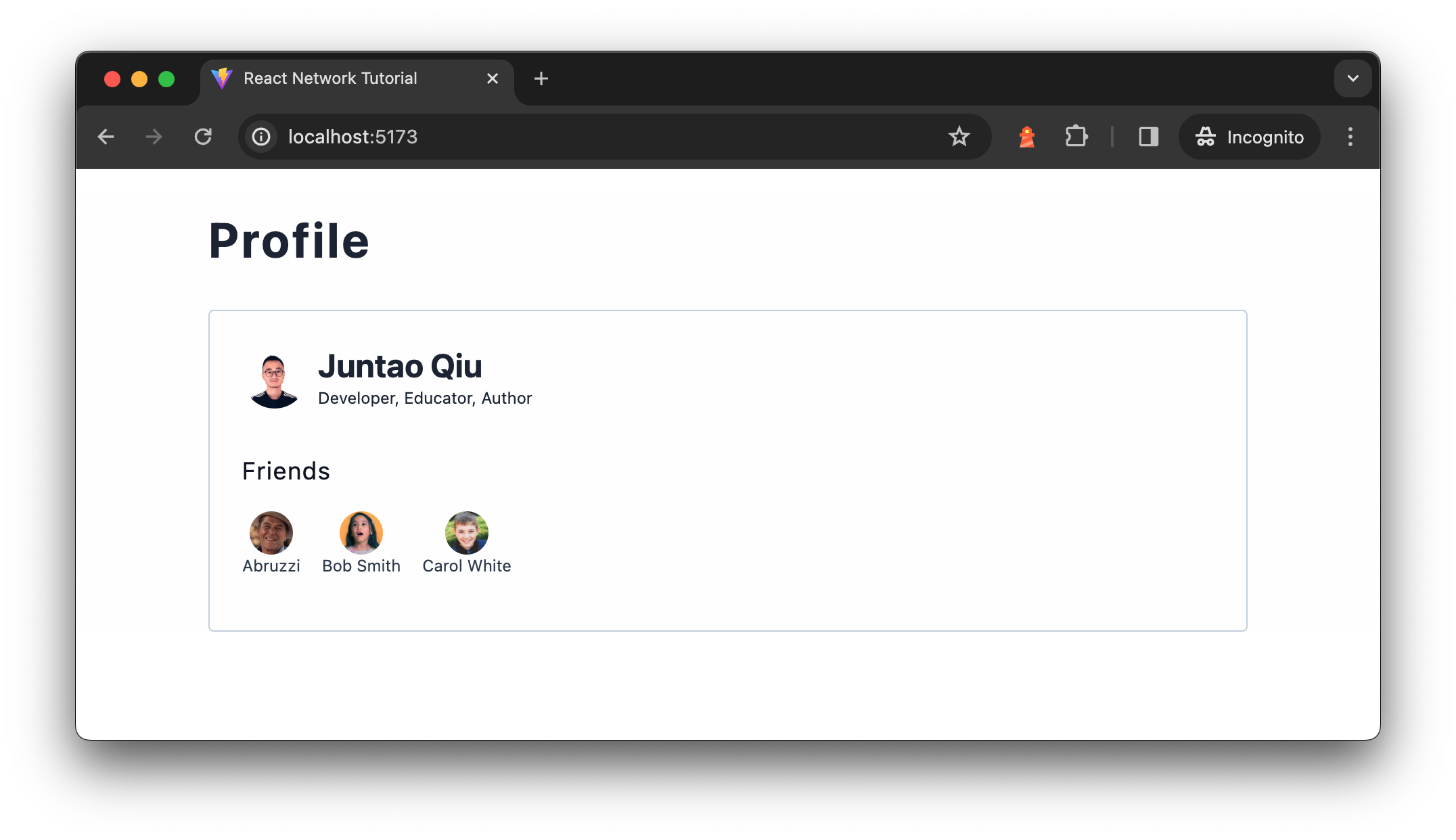
Determine 1: Profile display screen
The information are from two separate API calls, the person temporary API
/customers/<id>
returns person temporary for a given person id, which is a straightforward
object described as follows:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Writer", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the good friend API /customers/<id>/pals
endpoint returns an inventory of
pals for a given person, every checklist merchandise within the response is identical as
the above person information. The rationale we’ve got two endpoints as a substitute of returning
a pals
part of the person API is that there are instances the place one
might have too many pals (say 1,000), however most individuals do not have many.
This in-balance information construction might be fairly tough, particularly once we
have to paginate. The purpose right here is that there are instances we have to deal
with a number of community requests.
A quick introduction to related React ideas
As this text leverages React as an instance varied patterns, I do
not assume you already know a lot about React. Moderately than anticipating you to spend so much
of time looking for the suitable components within the React documentation, I’ll
briefly introduce these ideas we’ll make the most of all through this
article. If you happen to already perceive what React parts are, and the
use of the
useState
and useEffect
hooks, you might
use this hyperlink to skip forward to the following
part.
For these looking for a extra thorough tutorial, the new React documentation is a superb
useful resource.
What’s a React Part?
In React, parts are the basic constructing blocks. To place it
merely, a React part is a perform that returns a bit of UI,
which might be as simple as a fraction of HTML. Take into account the
creation of a part that renders a navigation bar:
import React from 'react'; perform Navigation() { return ( <nav> <ol> <li>House</li> <li>Blogs</li> <li>Books</li> </ol> </nav> ); }
At first look, the combination of JavaScript with HTML tags might sound
unusual (it is referred to as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, an analogous syntax referred to as TSX is used). To make this
code purposeful, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
perform Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "House"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Notice right here the translated code has a perform referred to as
React.createElement
, which is a foundational perform in
React for creating parts. JSX written in React parts is compiled
all the way down to React.createElement
calls behind the scenes.
The fundamental syntax of React.createElement
is:
React.createElement(sort, [props], [...children])
sort
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React part (class or purposeful) for
extra refined constructions.props
: An object containing properties handed to the
aspect or part, together with occasion handlers, kinds, and attributes
likeclassName
andid
.kids
: These non-obligatory arguments might be further
React.createElement
calls, strings, numbers, or any combine
thereof, representing the aspect’s kids.
For example, a easy aspect might be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Good day, world!');
That is analogous to the JSX model:
<div className="greeting">Good day, world!</div>
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM parts as vital.
You’ll be able to then assemble your customized parts right into a tree, just like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; perform App() { return <Web page />; } perform Web page() { return <Container> <Navigation /> <Content material> <Sidebar /> <ProductList /> </Content material> <Footer /> </Container>; }
In the end, your utility requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/shopper"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render(<App />);
Producing Dynamic Content material with JSX
The preliminary instance demonstrates a simple use case, however
let’s discover how we are able to create content material dynamically. For example, how
can we generate an inventory of knowledge dynamically? In React, as illustrated
earlier, a part is essentially a perform, enabling us to move
parameters to it.
import React from 'react'; perform Navigation({ nav }) { return ( <nav> <ol> {nav.map(merchandise => <li key={merchandise}>{merchandise}</li>)} </ol> </nav> ); }
On this modified Navigation
part, we anticipate the
parameter to be an array of strings. We make the most of the map
perform to iterate over every merchandise, reworking them into
<li>
parts. The curly braces {}
signify
that the enclosed JavaScript expression must be evaluated and
rendered. For these curious in regards to the compiled model of this dynamic
content material dealing with:
perform Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(perform(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As a substitute of invoking Navigation
as a daily perform,
using JSX syntax renders the part invocation extra akin to
writing markup, enhancing readability:
// As a substitute of this Navigation(["Home", "Blogs", "Books"]) // We do that <Navigation nav={["Home", "Blogs", "Books"]} />
Parts in React can obtain numerous information, referred to as props, to
modify their habits, very similar to passing arguments right into a perform (the
distinction lies in utilizing JSX syntax, making the code extra acquainted and
readable to these with HTML information, which aligns properly with the talent
set of most frontend builders).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App() { let showNewOnly = false; // This flag's worth is often set based mostly on particular logic. const filteredBooks = showNewOnly ? booksData.filter(e book => e book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks} /> </div> ); }
On this illustrative code snippet (non-functional however meant to
exhibit the idea), we manipulate the BookList
part’s displayed content material by passing it an array of books. Relying
on the showNewOnly
flag, this array is both all obtainable
books or solely these which are newly printed, showcasing how props can
be used to dynamically modify part output.
Managing Inside State Between Renders: useState
Constructing person interfaces (UI) usually transcends the technology of
static HTML. Parts often have to “bear in mind” sure states and
reply to person interactions dynamically. For example, when a person
clicks an “Add” button in a Product part, it’s a necessity to replace
the ShoppingCart part to mirror each the full worth and the
up to date merchandise checklist.
Within the earlier code snippet, trying to set the
showNewOnly
variable to true
inside an occasion
handler doesn’t obtain the specified impact:
perform App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this does not work }; const filteredBooks = showNewOnly ? booksData.filter(e book => e book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
This method falls quick as a result of native variables inside a perform
part don’t persist between renders. When React re-renders this
part, it does so from scratch, disregarding any adjustments made to
native variables since these don’t set off re-renders. React stays
unaware of the necessity to replace the part to mirror new information.
This limitation underscores the need for React’s
state
. Particularly, purposeful parts leverage the
useState
hook to recollect states throughout renders. Revisiting
the App
instance, we are able to successfully bear in mind the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(e book => e book.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow purposeful parts to handle inner state. It
introduces state to purposeful parts, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra advanced object or array. The
initialState
is just used through the first render to
initialize the state.- Return Worth:
useState
returns an array with
two parts. The primary aspect is the present state worth, and the
second aspect is a perform that enables updating this worth. Through the use of
array destructuring, we assign names to those returned gadgets,
sometimesstate
andsetState
, although you’ll be able to
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that will probably be used within the part’s UI and
logic.setState
: A perform to replace the state. This perform
accepts a brand new state worth or a perform that produces a brand new state based mostly
on the earlier state. When referred to as, it schedules an replace to the
part’s state and triggers a re-render to mirror the adjustments.
React treats state as a snapshot; updating it would not alter the
present state variable however as a substitute triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, making certain the
BookList
part receives the right information, thereby
reflecting the up to date e book checklist to the person. This snapshot-like
habits of state facilitates the dynamic and responsive nature of React
parts, enabling them to react intuitively to person interactions and
different adjustments.
Managing Aspect Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to deal with the
idea of negative effects. Unwanted side effects are operations that work together with
the surface world from the React ecosystem. Frequent examples embrace
fetching information from a distant server or dynamically manipulating the DOM,
reminiscent of altering the web page title.
React is primarily involved with rendering information to the DOM and does
not inherently deal with information fetching or direct DOM manipulation. To
facilitate these negative effects, React supplies the useEffect
hook. This hook permits the execution of negative effects after React has
accomplished its rendering course of. If these negative effects lead to information
adjustments, React schedules a re-render to mirror these updates.
The useEffect
Hook accepts two arguments:
- A perform containing the aspect impact logic.
- An non-obligatory dependency array specifying when the aspect impact must be
re-invoked.
Omitting the second argument causes the aspect impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t rely upon any values from props or state, thus not needing to
re-run. Together with particular values within the array means the aspect impact
solely re-executes if these values change.
When coping with asynchronous information fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the information is
retrieved, it’s captured through the useState
hook, updating the
part’s inner state and preserving the fetched information throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new information.
This is a sensible instance about information fetching and state
administration:
import { useEffect, useState } from "react"; sort Consumer = { id: string; identify: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-23:Code-Splitting-in-Single-Web page-Purposes); return <div> <h2>{person?.identify}</h2> </div>; };
Within the code snippet above, inside useEffect
, an
asynchronous perform fetchUser
is outlined after which
instantly invoked. This sample is important as a result of
useEffect
doesn’t immediately assist async features as its
callback. The async perform is outlined to make use of await
for
the fetch operation, making certain that the code execution waits for the
response after which processes the JSON information. As soon as the information is obtainable,
it updates the part’s state through setUser
.
The dependency array tag:martinfowler.com,2024-05-23:Code-Splitting-in-Single-Web page-Purposes
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
adjustments, which prevents pointless community requests on
each render and fetches new person information when the id
prop
updates.
This method to dealing with asynchronous information fetching inside
useEffect
is a typical apply in React growth, providing a
structured and environment friendly strategy to combine async operations into the
React part lifecycle.
As well as, in sensible purposes, managing completely different states
reminiscent of loading, error, and information presentation is crucial too (we’ll
see it the way it works within the following part). For instance, take into account
implementing standing indicators inside a Consumer part to mirror
loading, error, or information states, enhancing the person expertise by
offering suggestions throughout information fetching operations.
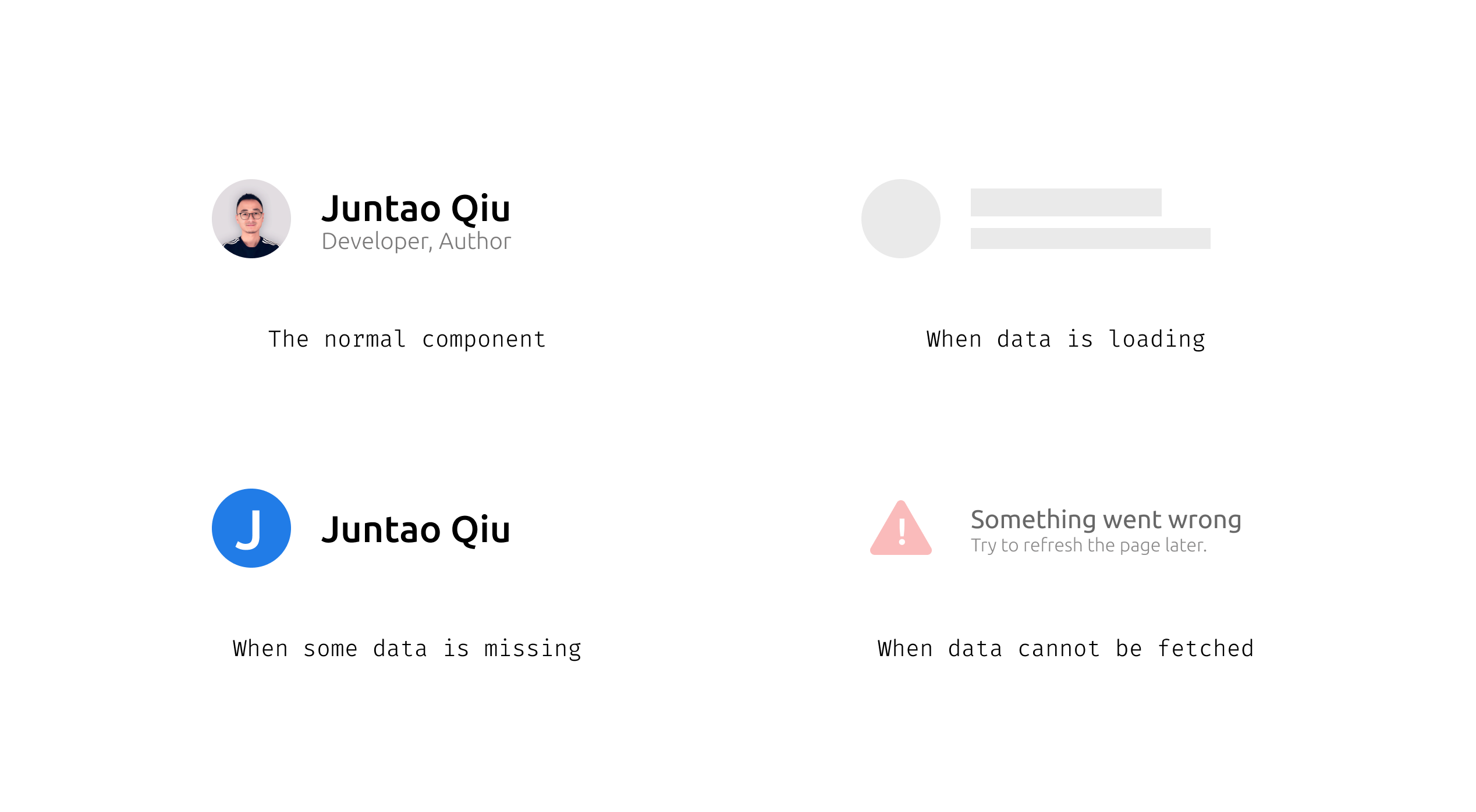
Determine 2: Completely different statuses of a
part
This overview affords only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into further ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line sources.
With this basis, you must now be outfitted to hitch me as we delve
into the information fetching patterns mentioned herein.
Implement the Profile part
Let’s create the Profile
part to make a request and
render the outcome. In typical React purposes, this information fetching is
dealt with inside a useEffect
block. This is an instance of how
this may be applied:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-23:Code-Splitting-in-Single-Web page-Purposes); return ( <UserBrief person={person} /> ); };
This preliminary method assumes community requests full
instantaneously, which is usually not the case. Actual-world eventualities require
dealing with various community circumstances, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
part. This addition permits us to supply suggestions to the person throughout
information fetching, reminiscent of displaying a loading indicator or a skeleton display screen
if the information is delayed, and dealing with errors once they happen.
Right here’s how the improved part seems with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import sort { Consumer } from "../varieties.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { strive { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-23:Code-Splitting-in-Single-Web page-Purposes); if (loading || !person) { return <div>Loading...</div>; } return ( <> {person && <UserBrief person={person} />} </> ); };
Now in Profile
part, we provoke states for loading,
errors, and person information with useState
. Utilizing
useEffect
, we fetch person information based mostly on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
information retrieval, we replace the person state, else show a loading
indicator.
The get
perform, as demonstrated under, simplifies
fetching information from a selected endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON information or throws an error for unsuccessful requests,
streamlining error dealing with and information retrieval in our utility. Notice
it is pure TypeScript code and can be utilized in different non-React components of the
utility.
const baseurl = "https://icodeit.com.au/api/v2"; async perform get<T>(url: string): Promise<T> { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise<T>; }
React will attempt to render the part initially, however as the information
person
isn’t obtainable, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as in some unspecified time in the future, the response returns, React
re-renders the Profile
part with person
fulfilled, so now you can see the person part with identify, avatar, and
title.
If we visualize the timeline of the above code, you will notice
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and elegance tags, it’d cease and
obtain these recordsdata, after which parse them to kind the ultimate web page. Notice
that it is a comparatively sophisticated course of, and I’m oversimplifying
right here, however the primary concept of the sequence is appropriate.
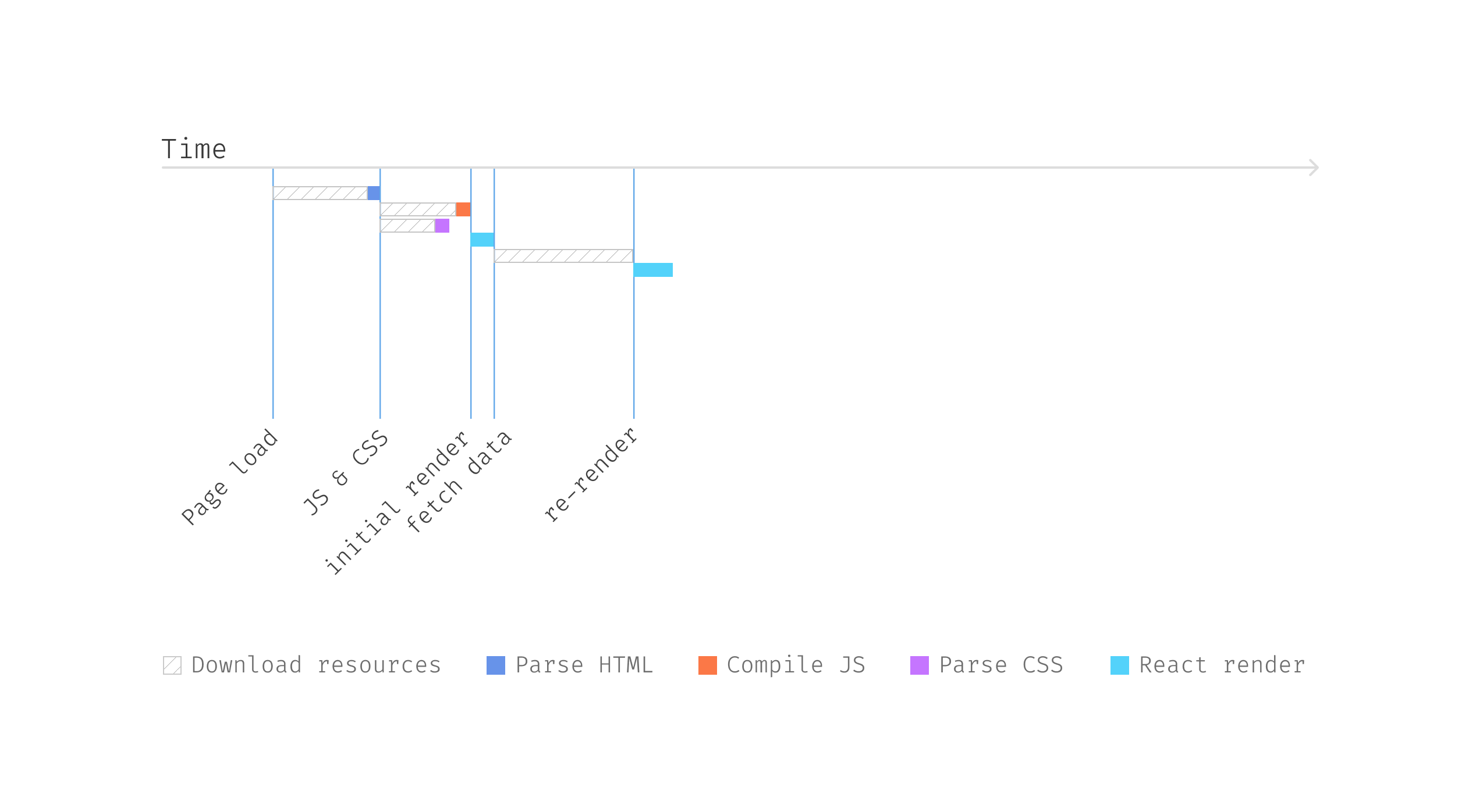
Determine 3: Fetching person
information
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for information fetching; it has to attend till
the information is obtainable for a re-render.
Now within the browser, we are able to see a “loading…” when the applying
begins, after which after a couple of seconds (we are able to simulate such case by add
some delay within the API endpoints) the person temporary part reveals up when information
is loaded.
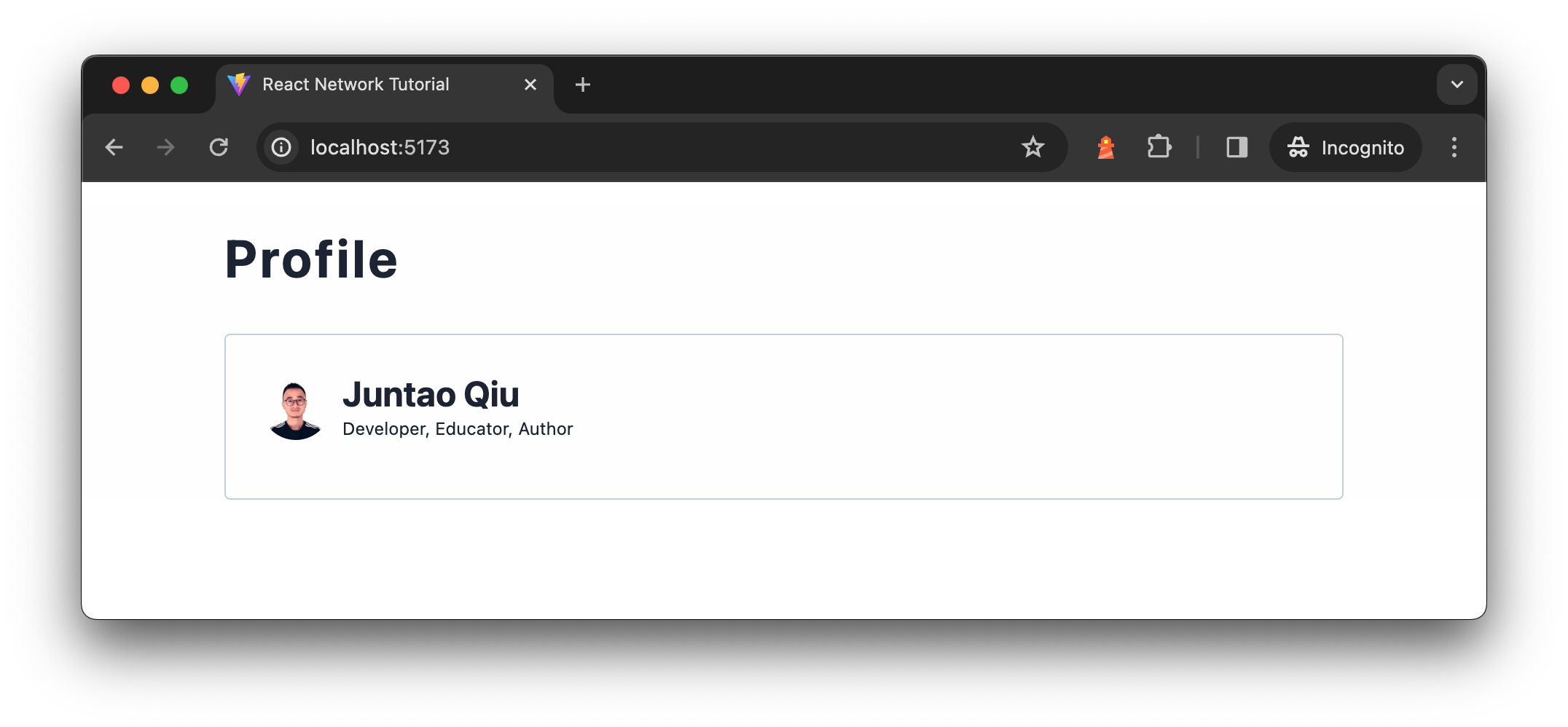
Determine 4: Consumer temporary part
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
broadly used throughout React codebases. In purposes of normal measurement, it is
frequent to seek out quite a few cases of such identical data-fetching logic
dispersed all through varied parts.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls might be gradual, and it is important to not let the UI freeze
whereas these calls are being made. Due to this fact, we deal with them asynchronously
and use indicators to point out {that a} course of is underway, which makes the
person expertise higher – figuring out that one thing is occurring.
Moreover, distant calls would possibly fail attributable to connection points,
requiring clear communication of those failures to the person. Due to this fact,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata in regards to the standing of the decision, enabling it to show
various data or choices if the anticipated outcomes fail to
materialize.
A easy implementation could possibly be a perform getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing data important for managing asynchronous
operations. This setup permits us to appropriately reply to completely different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, information } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
The idea right here is that getAsyncStates
initiates the
community request mechanically upon being referred to as. Nonetheless, this won’t
all the time align with the caller’s wants. To supply extra management, we are able to additionally
expose a fetch
perform throughout the returned object, permitting
the initiation of the request at a extra applicable time, based on the
caller’s discretion. Moreover, a refetch
perform might
be offered to allow the caller to re-initiate the request as wanted,
reminiscent of after an error or when up to date information is required. The
fetch
and refetch
features might be an identical in
implementation, or refetch
would possibly embrace logic to verify for
cached outcomes and solely re-fetch information if vital.
const { loading, error, information, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
This sample supplies a flexible method to dealing with asynchronous
requests, giving builders the flexibleness to set off information fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
purposes can adapt extra dynamically to person interactions and different
runtime circumstances, enhancing the person expertise and utility
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample might be applied in several frontend libraries. For
occasion, we might distill this method right into a customized Hook in a React
utility for the Profile part:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { strive { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-23:Code-Splitting-in-Single-Web page-Purposes); return { loading, error, person, }; };
Please word that within the customized Hook, we have no JSX code –
that means it’s very UI free however sharable stateful logic. And the
useUser
launch information mechanically when referred to as. Inside the Profile
part, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, person } = useUser(id); if (loading || !person) { return <div>Loading...</div>; } if (error) { return <div>One thing went mistaken...</div>; } return ( <> {person && <UserBrief person={person} />} </> ); };
Generalizing Parameter Utilization
In most purposes, fetching various kinds of information—from person
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a typical requirement. Writing separate
fetch features for every sort of knowledge might be tedious and tough to
preserve. A greater method is to summary this performance right into a
generic, reusable hook that may deal with varied information varieties
effectively.
Take into account treating distant API endpoints as providers, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; perform useService<T>(url: string) { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [data, setData] = useState<T | undefined>(); const fetch = async () => { strive { setLoading(true); const information = await get<T>(url); setData(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, information, fetch, }; }
This hook abstracts the information fetching course of, making it simpler to
combine into any part that should retrieve information from a distant
supply. It additionally centralizes frequent error dealing with eventualities, reminiscent of
treating particular errors in a different way:
import { useService } from './useService.ts'; const { loading, error, information: person, fetch: fetchUser, } = useService(`/customers/${id}`);
Through the use of useService, we are able to simplify how parts fetch and deal with
information, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
could be expose the
fetchUsers
perform, and it doesn’t set off the information
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { strive { setLoading(true); const information = await get<Consumer>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, person, fetchUser, }; };
After which on the calling web site, Profile
part use
useEffect
to fetch the information and render completely different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, person, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the flexibility to reuse these stateful
logics throughout completely different parts. For example, one other part
needing the identical information (a person API name with a person ID) can merely import
the useUser
Hook and make the most of its states. Completely different UI
parts would possibly select to work together with these states in varied methods,
maybe utilizing various loading indicators (a smaller spinner that
suits to the calling part) or error messages, but the basic
logic of fetching information stays constant and shared.
When to make use of it
Separating information fetching logic from UI parts can typically
introduce pointless complexity, significantly in smaller purposes.
Conserving this logic built-in throughout the part, just like the
css-in-js method, simplifies navigation and is simpler for some
builders to handle. In my article, Modularizing
React Purposes with Established UI Patterns, I explored
varied ranges of complexity in utility constructions. For purposes
which are restricted in scope — with just some pages and a number of other information
fetching operations — it is usually sensible and in addition really helpful to
preserve information fetching inside the UI parts.
Nonetheless, as your utility scales and the event crew grows,
this technique might result in inefficiencies. Deep part bushes can gradual
down your utility (we’ll see examples in addition to tips on how to deal with
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling information fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to stability simplicity with structured approaches as your
mission evolves. This ensures your growth practices stay
efficient and conscious of the applying’s wants, sustaining optimum
efficiency and developer effectivity whatever the mission
scale.
Implement the Pals checklist
Now let’s take a look on the second part of the Profile – the good friend
checklist. We are able to create a separate part Pals
and fetch information in it
(by utilizing a useService customized hook we outlined above), and the logic is
fairly just like what we see above within the Profile
part.
const Pals = ({ id }: { id: string }) => { const { loading, error, information: pals } = useService(`/customers/${id}/pals`); // loading & error dealing with... return ( <div> <h2>Pals</h2> <div> {pals.map((person) => ( // render person checklist ))} </div> </div> ); };
After which within the Profile part, we are able to use Pals as a daily
part, and move in id
as a prop:
const Profile = ({ id }: { id: string }) => { //... return ( <> {person && <UserBrief person={person} />} <Pals id={id} /> </> ); };
The code works high-quality, and it seems fairly clear and readable,
UserBrief
renders a person
object handed in, whereas
Pals
handle its personal information fetching and rendering logic
altogether. If we visualize the part tree, it could be one thing like
this:
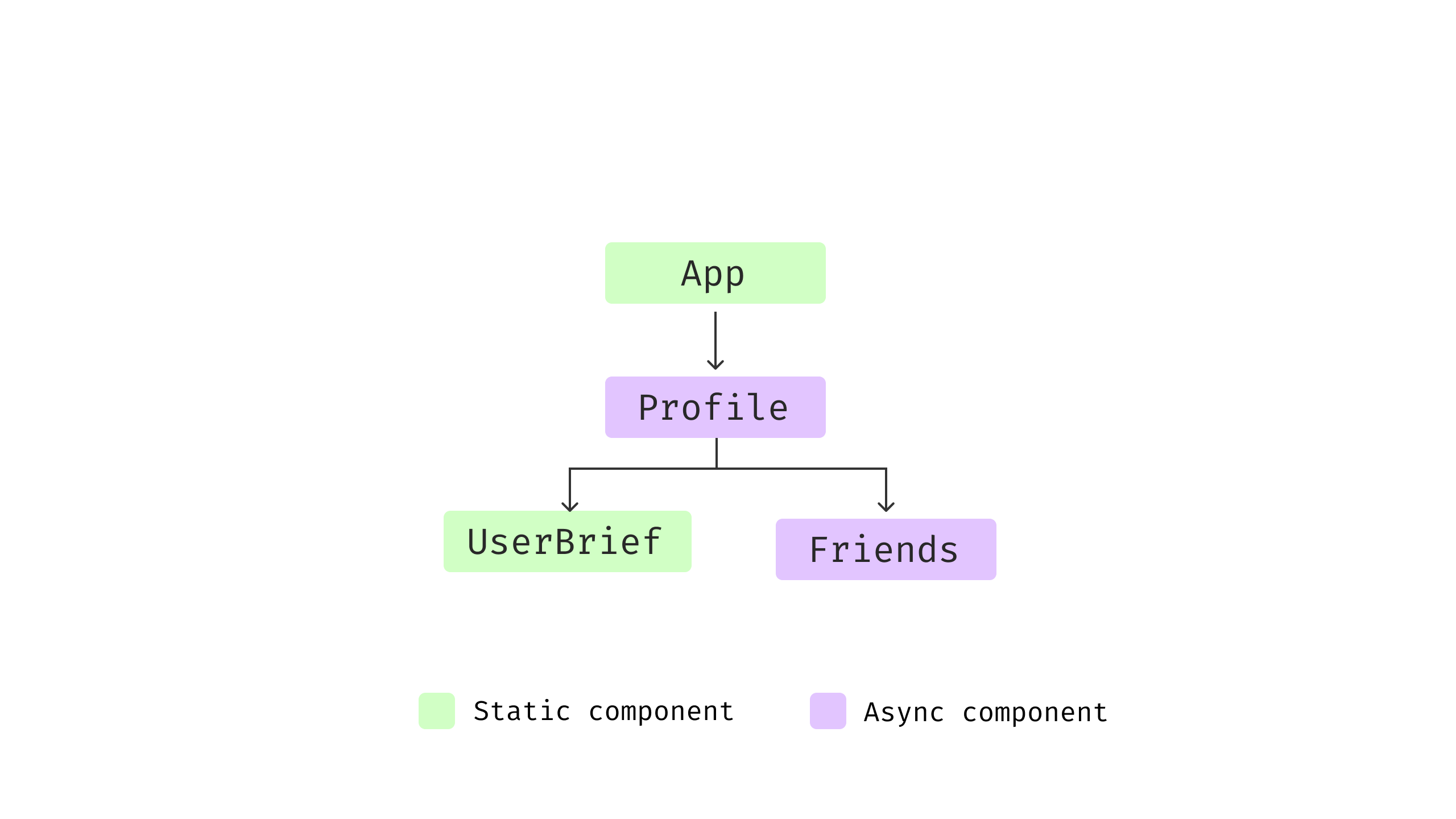
Determine 5: Part construction
Each the Profile
and Pals
have logic for
information fetching, loading checks, and error dealing with. Since there are two
separate information fetching calls, and if we have a look at the request timeline, we
will discover one thing attention-grabbing.
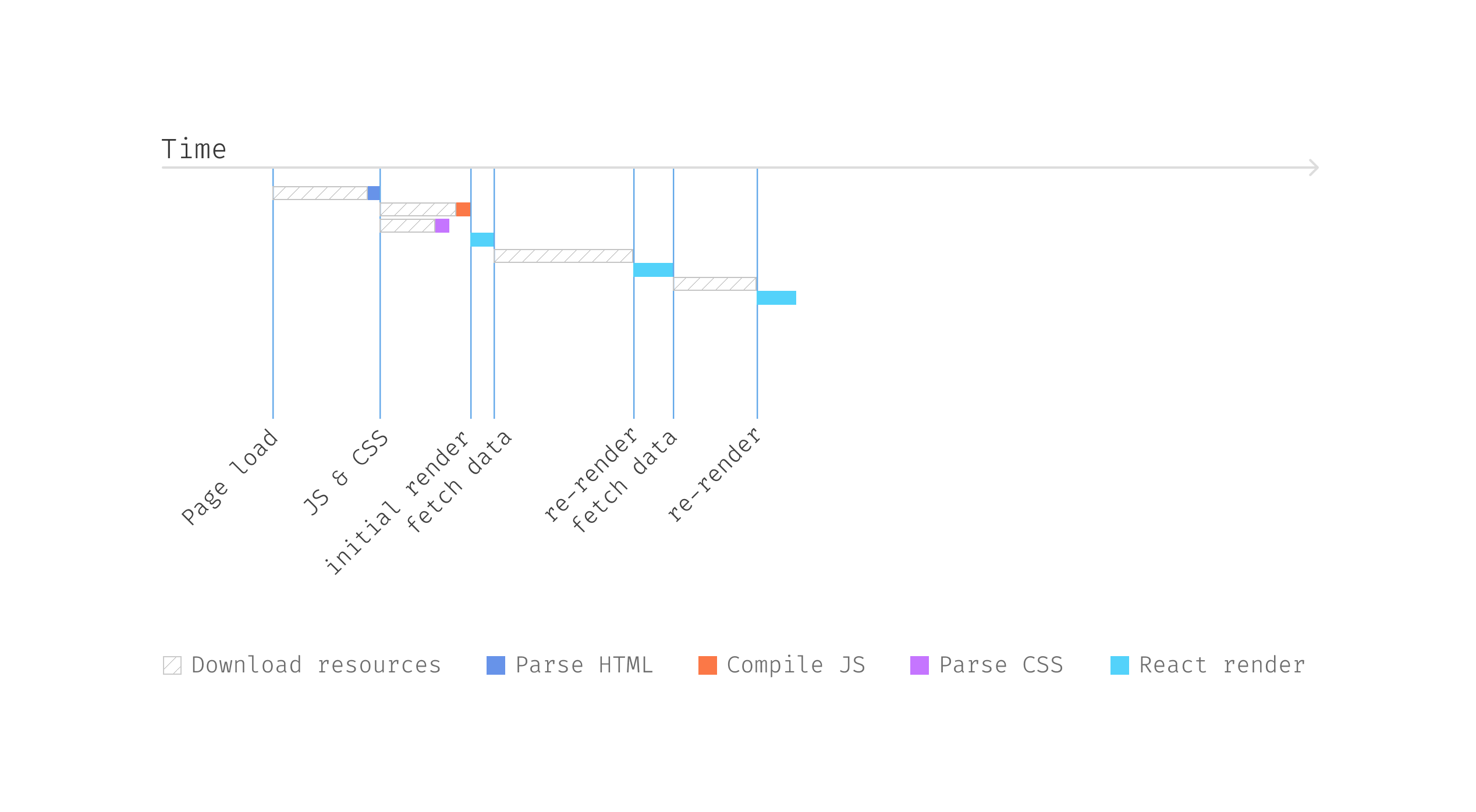
Determine 6: Request waterfall
The Pals
part will not provoke information fetching till the person
state is about. That is known as the Fetch-On-Render method,
the place the preliminary rendering is paused as a result of the information is not obtainable,
requiring React to attend for the information to be retrieved from the server
aspect.
This ready interval is considerably inefficient, contemplating that whereas
React’s rendering course of solely takes a couple of milliseconds, information fetching can
take considerably longer, usually seconds. Consequently, the Pals
part spends most of its time idle, ready for information. This state of affairs
results in a typical problem referred to as the Request Waterfall, a frequent
incidence in frontend purposes that contain a number of information fetching
operations.
Parallel Information Fetching
Run distant information fetches in parallel to attenuate wait time
Think about once we construct a bigger utility {that a} part that
requires information might be deeply nested within the part tree, to make the
matter worse these parts are developed by completely different groups, it’s onerous
to see whom we’re blocking.
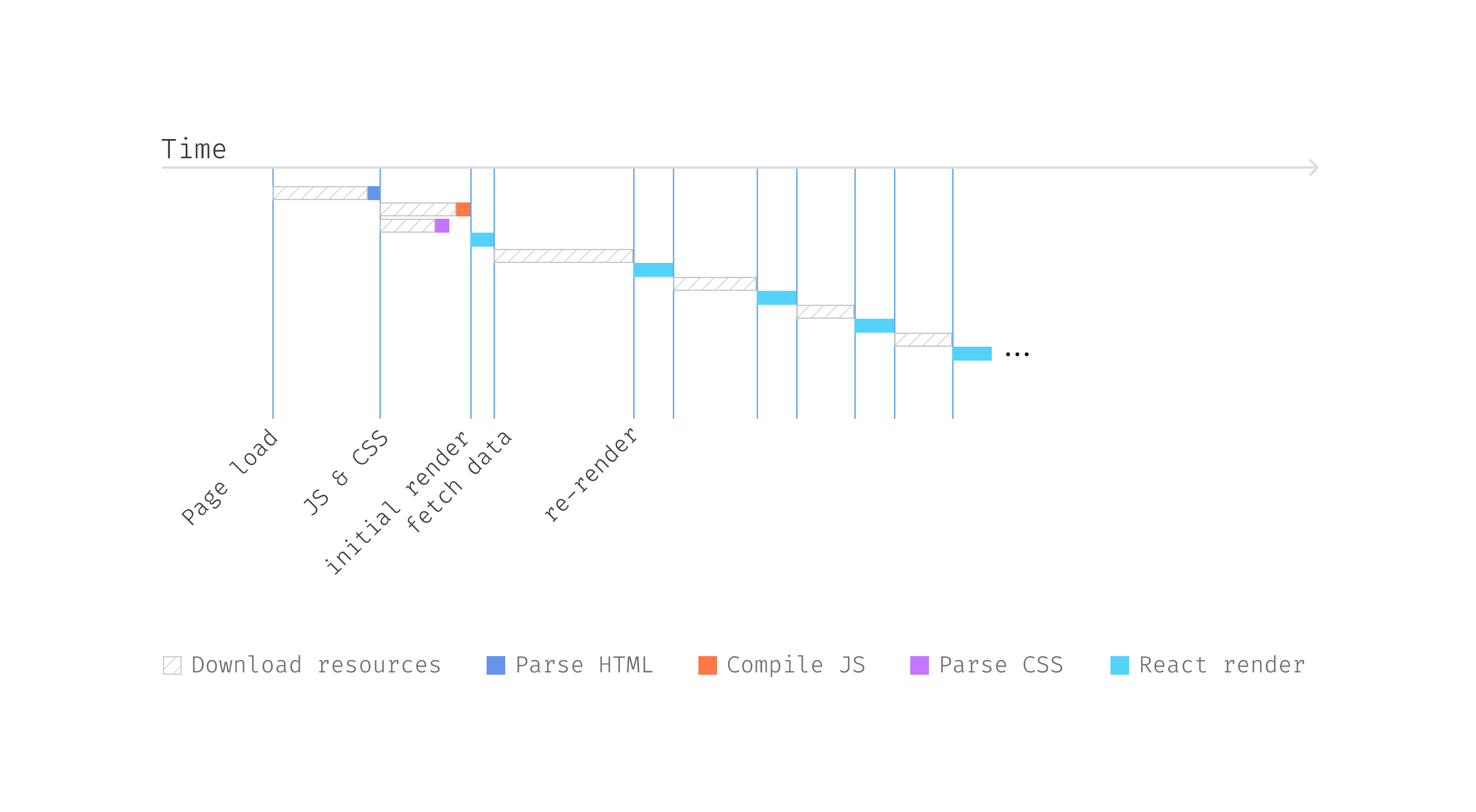
Determine 7: Request waterfall
Request Waterfalls can degrade person
expertise, one thing we purpose to keep away from. Analyzing the information, we see that the
person API and pals API are unbiased and might be fetched in parallel.
Initiating these parallel requests turns into crucial for utility
efficiency.
One method is to centralize information fetching at the next degree, close to the
root. Early within the utility’s lifecycle, we begin all information fetches
concurrently. Parts depending on this information wait just for the
slowest request, sometimes leading to quicker total load instances.
We might use the Promise API Promise.all
to ship
each requests for the person’s primary data and their pals checklist.
Promise.all
is a JavaScript technique that enables for the
concurrent execution of a number of guarantees. It takes an array of guarantees
as enter and returns a single Promise that resolves when the entire enter
guarantees have resolved, offering their outcomes as an array. If any of the
guarantees fail, Promise.all
instantly rejects with the
motive of the primary promise that rejects.
For example, on the utility’s root, we are able to outline a complete
information mannequin:
sort ProfileState = { person: Consumer; pals: Consumer[]; }; const getProfileData = async (id: string) => Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/pals`), ]); const App = () => { // fetch information on the very begining of the applying launch const onInit = () => { const [user, friends] = await getProfileData(id); } // render the sub tree correspondingly }
Implementing Parallel Information Fetching in React
Upon utility launch, information fetching begins, abstracting the
fetching course of from subcomponents. For instance, in Profile part,
each UserBrief and Pals are presentational parts that react to
the handed information. This fashion we might develop these part individually
(including kinds for various states, for instance). These presentational
parts usually are simple to check and modify as we’ve got separate the
information fetching and rendering.
We are able to outline a customized hook useProfileData
that facilitates
parallel fetching of knowledge associated to a person and their pals by utilizing
Promise.all
. This technique permits simultaneous requests, optimizing the
loading course of and structuring the information right into a predefined format recognized
as ProfileData
.
Right here’s a breakdown of the hook implementation:
import { useCallback, useEffect, useState } from "react"; sort ProfileData = { person: Consumer; pals: Consumer[]; }; const useProfileData = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(undefined); const [profileState, setProfileState] = useState<ProfileData>(); const fetchProfileState = useCallback(async () => { strive { setLoading(true); const [user, friends] = await Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/pals`), ]); setProfileState({ person, pals }); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }, tag:martinfowler.com,2024-05-23:Code-Splitting-in-Single-Web page-Purposes); return { loading, error, profileState, fetchProfileState, }; };
This hook supplies the Profile
part with the
vital information states (loading
, error
,
profileState
) together with a fetchProfileState
perform, enabling the part to provoke the fetch operation as
wanted. Notice right here we use useCallback
hook to wrap the async
perform for information fetching. The useCallback hook in React is used to
memoize features, making certain that the identical perform occasion is
maintained throughout part re-renders until its dependencies change.
Just like the useEffect, it accepts the perform and a dependency
array, the perform will solely be recreated if any of those dependencies
change, thereby avoiding unintended habits in React’s rendering
cycle.
The Profile
part makes use of this hook and controls the information fetching
timing through useEffect
:
const Profile = ({ id }: { id: string }) => { const { loading, error, profileState, fetchProfileState } = useProfileData(id); useEffect(() => { fetchProfileState(); }, [fetchProfileState]); if (loading) { return <div>Loading...</div>; } if (error) { return <div>One thing went mistaken...</div>; } return ( <> {profileState && ( <> <UserBrief person={profileState.person} /> <Pals customers={profileState.pals} /> </> )} </> ); };
This method is also referred to as Fetch-Then-Render, suggesting that the purpose
is to provoke requests as early as attainable throughout web page load.
Subsequently, the fetched information is utilized to drive React’s rendering of
the applying, bypassing the necessity to handle information fetching amidst the
rendering course of. This technique simplifies the rendering course of,
making the code simpler to check and modify.
And the part construction, if visualized, could be just like the
following illustration
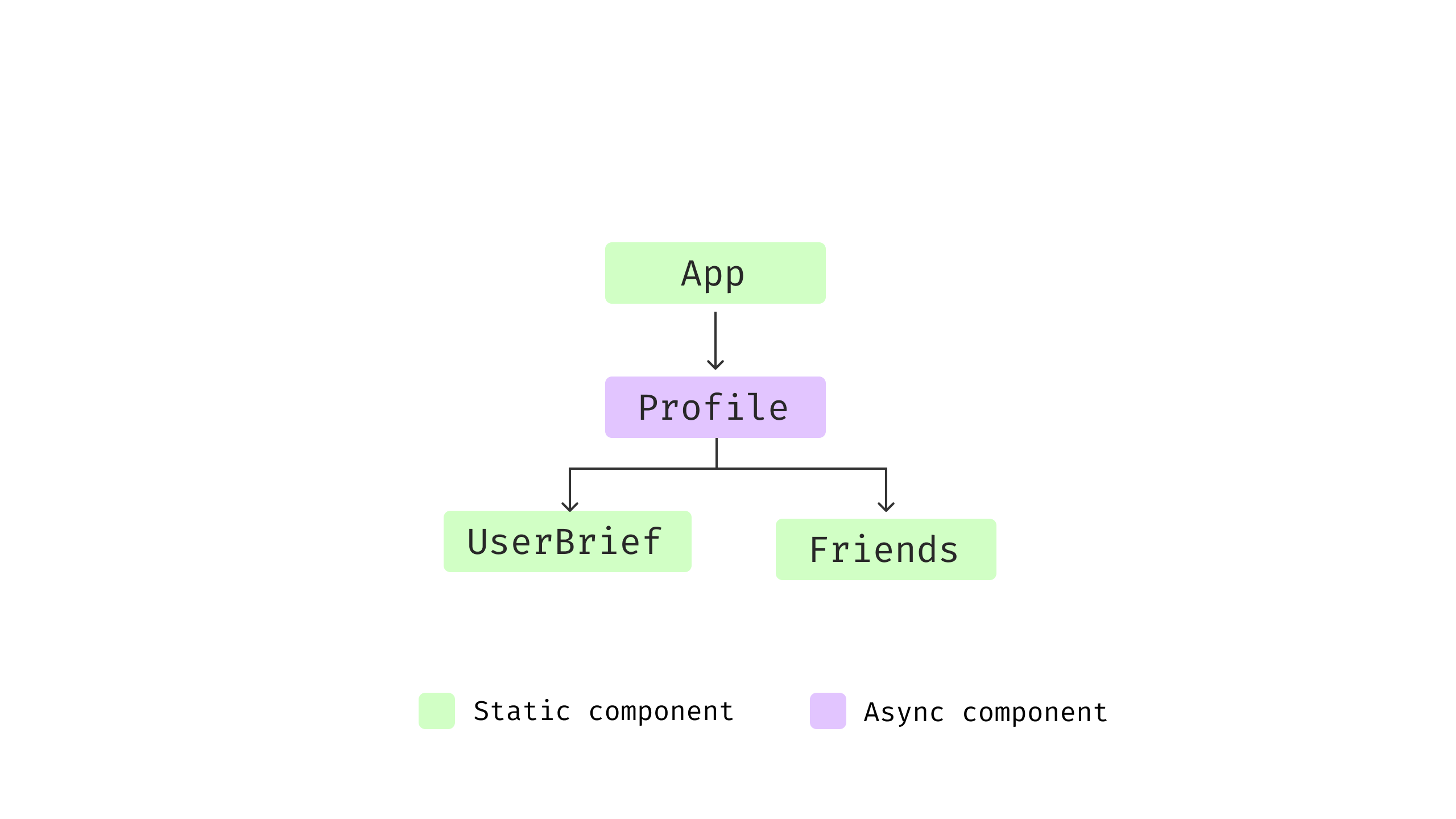
Determine 8: Part construction after refactoring
And the timeline is far shorter than the earlier one as we ship two
requests in parallel. The Pals
part can render in a couple of
milliseconds as when it begins to render, the information is already prepared and
handed in.
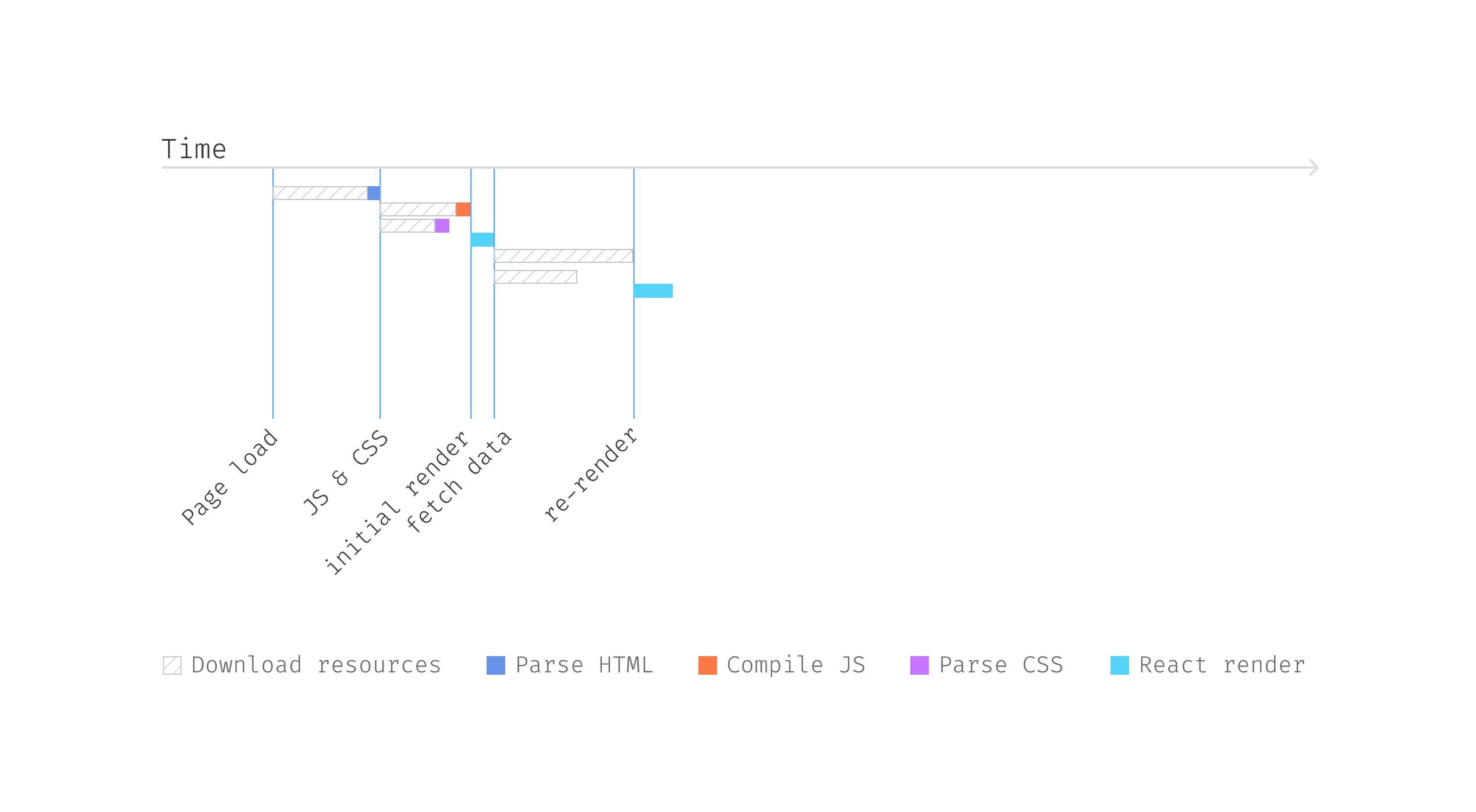
Determine 9: Parallel requests
Notice that the longest wait time is dependent upon the slowest community
request, which is far quicker than the sequential ones. And if we might
ship as many of those unbiased requests on the identical time at an higher
degree of the part tree, a greater person expertise might be
anticipated.
As purposes develop, managing an growing variety of requests at
root degree turns into difficult. That is significantly true for parts
distant from the foundation, the place passing down information turns into cumbersome. One
method is to retailer all information globally, accessible through features (like
Redux or the React Context API), avoiding deep prop drilling.
When to make use of it
Working queries in parallel is helpful every time such queries could also be
gradual and do not considerably intervene with every others’ efficiency.
That is normally the case with distant queries. Even when the distant
machine’s I/O and computation is quick, there’s all the time potential latency
points within the distant calls. The primary drawback for parallel queries
is setting them up with some form of asynchronous mechanism, which can be
tough in some language environments.
The primary motive to not use parallel information fetching is once we do not
know what information must be fetched till we have already fetched some
information. Sure eventualities require sequential information fetching attributable to
dependencies between requests. For example, take into account a state of affairs on a
Profile
web page the place producing a customized suggestion feed
is dependent upon first buying the person’s pursuits from a person API.
This is an instance response from the person API that features
pursuits:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Writer", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
In such instances, the advice feed can solely be fetched after
receiving the person’s pursuits from the preliminary API name. This
sequential dependency prevents us from using parallel fetching, as
the second request depends on information obtained from the primary.
Given these constraints, it turns into essential to debate various
methods in asynchronous information administration. One such technique is
Fallback Markup. This method permits builders to specify what
information is required and the way it must be fetched in a approach that clearly
defines dependencies, making it simpler to handle advanced information
relationships in an utility.
One other instance of when arallel Information Fetching just isn’t relevant is
that in eventualities involving person interactions that require real-time
information validation.
Take into account the case of an inventory the place every merchandise has an “Approve” context
menu. When a person clicks on the “Approve” possibility for an merchandise, a dropdown
menu seems providing decisions to both “Approve” or “Reject.” If this
merchandise’s approval standing could possibly be modified by one other admin concurrently,
then the menu choices should mirror probably the most present state to keep away from
conflicting actions.
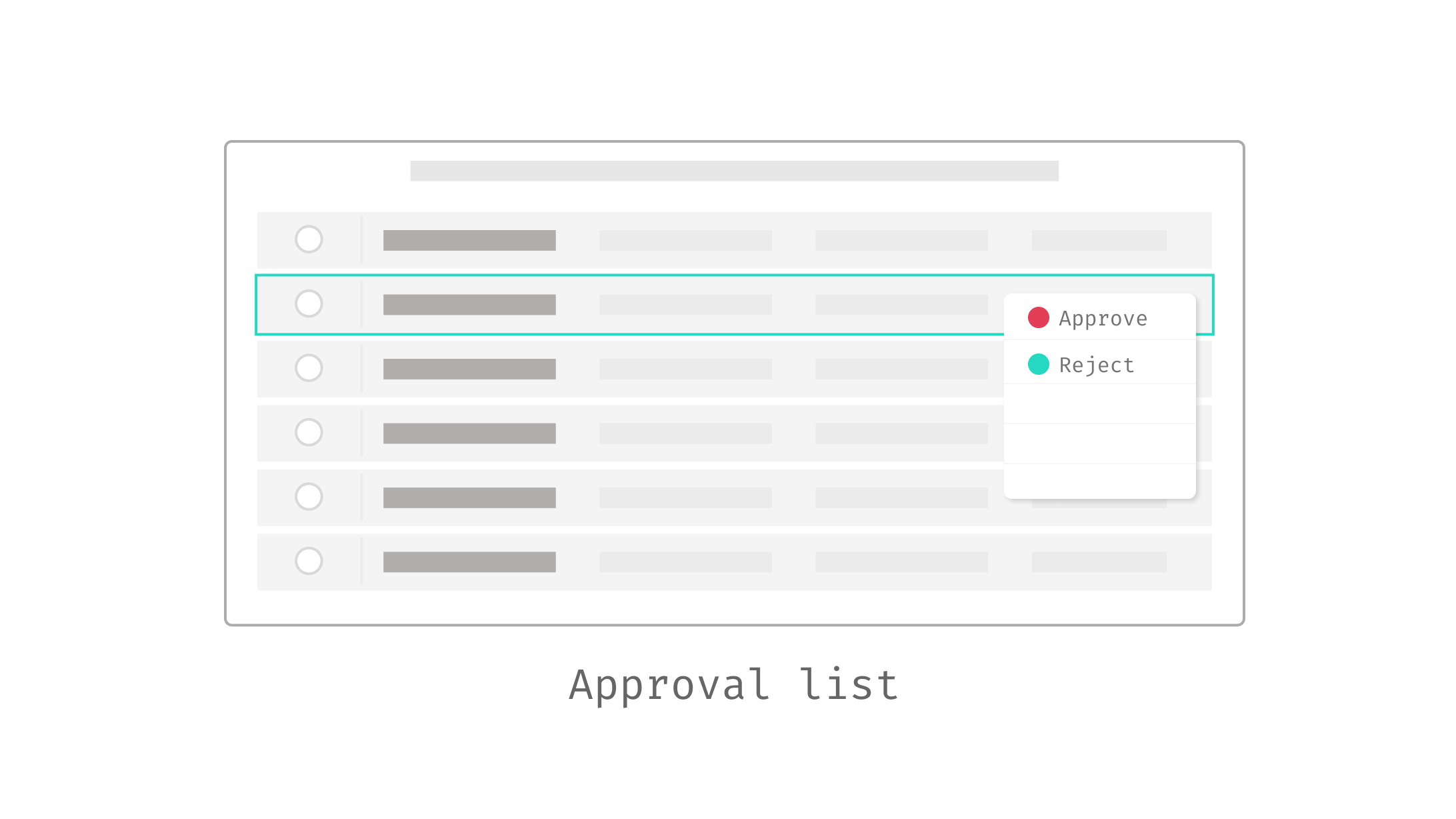
Determine 10: The approval checklist that require in-time
states
To deal with this, a service name is initiated every time the context
menu is activated. This service fetches the newest standing of the merchandise,
making certain that the dropdown is constructed with probably the most correct and
present choices obtainable at that second. Consequently, these requests
can’t be made in parallel with different data-fetching actions because the
dropdown’s contents rely fully on the real-time standing fetched from
the server.
Fallback Markup
Specify fallback shows within the web page markup
This sample leverages abstractions offered by frameworks or libraries
to deal with the information retrieval course of, together with managing states like
loading, success, and error, behind the scenes. It permits builders to
deal with the construction and presentation of knowledge of their purposes,
selling cleaner and extra maintainable code.
Let’s take one other have a look at the Pals
part within the above
part. It has to take care of three completely different states and register the
callback in useEffect
, setting the flag appropriately on the proper time,
prepare the completely different UI for various states:
const Pals = ({ id }: { id: string }) => { //... const { loading, error, information: pals, fetch: fetchFriends, } = useService(`/customers/${id}/pals`); useEffect(() => { fetchFriends(); }, []); if (loading) { // present loading indicator } if (error) { // present error message part } // present the acutal good friend checklist };
You’ll discover that inside a part we’ve got to cope with
completely different states, even we extract customized Hook to cut back the noise in a
part, we nonetheless have to pay good consideration to dealing with
loading
and error
inside a part. These
boilerplate code might be cumbersome and distracting, usually cluttering the
readability of our codebase.
If we consider declarative API, like how we construct our UI with JSX, the
code might be written within the following method that permits you to deal with
what the part is doing – not tips on how to do it:
<WhenError fallback={<ErrorMessage />}> <WhenInProgress fallback={<Loading />}> <Pals /> </WhenInProgress> </WhenError>
Within the above code snippet, the intention is easy and clear: when an
error happens, ErrorMessage
is displayed. Whereas the operation is in
progress, Loading is proven. As soon as the operation completes with out errors,
the Pals part is rendered.
And the code snippet above is fairly similiar to what already be
applied in a couple of libraries (together with React and Vue.js). For instance,
the brand new Suspense
in React permits builders to extra successfully handle
asynchronous operations inside their parts, bettering the dealing with of
loading states, error states, and the orchestration of concurrent
duties.
Implementing Fallback Markup in React with Suspense
Suspense
in React is a mechanism for effectively dealing with
asynchronous operations, reminiscent of information fetching or useful resource loading, in a
declarative method. By wrapping parts in a Suspense
boundary,
builders can specify fallback content material to show whereas ready for the
part’s information dependencies to be fulfilled, streamlining the person
expertise throughout loading states.
Whereas with the Suspense API, within the Pals
you describe what you
need to get after which render:
import useSWR from "swr"; import { get } from "../utils.ts"; perform Pals({ id }: { id: string }) { const { information: customers } = useSWR("/api/profile", () => get<Consumer[]>(`/customers/${id}/pals`), { suspense: true, }); return ( <div> <h2>Pals</h2> <div> {pals.map((person) => ( <Pal person={person} key={person.id} /> ))} </div> </div> ); }
And declaratively whenever you use the Pals
, you employ
Suspense
boundary to wrap across the Pals
part:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
Suspense
manages the asynchronous loading of the
Pals
part, displaying a FriendsSkeleton
placeholder till the part’s information dependencies are
resolved. This setup ensures that the person interface stays responsive
and informative throughout information fetching, bettering the general person
expertise.
Use the sample in Vue.js
It is price noting that Vue.js can also be exploring an analogous
experimental sample, the place you’ll be able to make use of Fallback Markup utilizing:
<Suspense> <template #default> <AsyncComponent /> </template> <template #fallback> Loading... </template> </Suspense>
Upon the primary render, <Suspense>
makes an attempt to render
its default content material behind the scenes. Ought to it encounter any
asynchronous dependencies throughout this section, it transitions right into a
pending state, the place the fallback content material is displayed as a substitute. As soon as all
the asynchronous dependencies are efficiently loaded,
<Suspense>
strikes to a resolved state, and the content material
initially meant for show (the default slot content material) is
rendered.
Deciding Placement for the Loading Part
You might marvel the place to position the FriendsSkeleton
part and who ought to handle it. Usually, with out utilizing Fallback
Markup, this choice is simple and dealt with immediately throughout the
part that manages the information fetching:
const Pals = ({ id }: { id: string }) => { // Information fetching logic right here... if (loading) { // Show loading indicator } if (error) { // Show error message part } // Render the precise good friend checklist };
On this setup, the logic for displaying loading indicators or error
messages is of course located throughout the Pals
part. Nonetheless,
adopting Fallback Markup shifts this accountability to the
part’s shopper:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
In real-world purposes, the optimum method to dealing with loading
experiences relies upon considerably on the specified person interplay and
the construction of the applying. For example, a hierarchical loading
method the place a dad or mum part ceases to point out a loading indicator
whereas its kids parts proceed can disrupt the person expertise.
Thus, it is essential to fastidiously take into account at what degree throughout the
part hierarchy the loading indicators or skeleton placeholders
must be displayed.
Consider Pals
and FriendsSkeleton
as two
distinct part states—one representing the presence of knowledge, and the
different, the absence. This idea is considerably analogous to utilizing a Speical Case sample in object-oriented
programming, the place FriendsSkeleton
serves because the ‘null’
state dealing with for the Pals
part.
The secret is to find out the granularity with which you need to
show loading indicators and to take care of consistency in these
selections throughout your utility. Doing so helps obtain a smoother and
extra predictable person expertise.
When to make use of it
Utilizing Fallback Markup in your UI simplifies code by enhancing its readability
and maintainability. This sample is especially efficient when using
customary parts for varied states reminiscent of loading, errors, skeletons, and
empty views throughout your utility. It reduces redundancy and cleans up
boilerplate code, permitting parts to focus solely on rendering and
performance.
Fallback Markup, reminiscent of React’s Suspense, standardizes the dealing with of
asynchronous loading, making certain a constant person expertise. It additionally improves
utility efficiency by optimizing useful resource loading and rendering, which is
particularly useful in advanced purposes with deep part bushes.
Nonetheless, the effectiveness of Fallback Markup is dependent upon the capabilities of
the framework you might be utilizing. For instance, React’s implementation of Suspense for
information fetching nonetheless requires third-party libraries, and Vue’s assist for
comparable options is experimental. Furthermore, whereas Fallback Markup can cut back
complexity in managing state throughout parts, it could introduce overhead in
less complicated purposes the place managing state immediately inside parts might
suffice. Moreover, this sample might restrict detailed management over loading and
error states—conditions the place completely different error varieties want distinct dealing with would possibly
not be as simply managed with a generic fallback method.
Introducing UserDetailCard part
Let’s say we want a function that when customers hover on prime of a Pal
,
we present a popup to allow them to see extra particulars about that person.
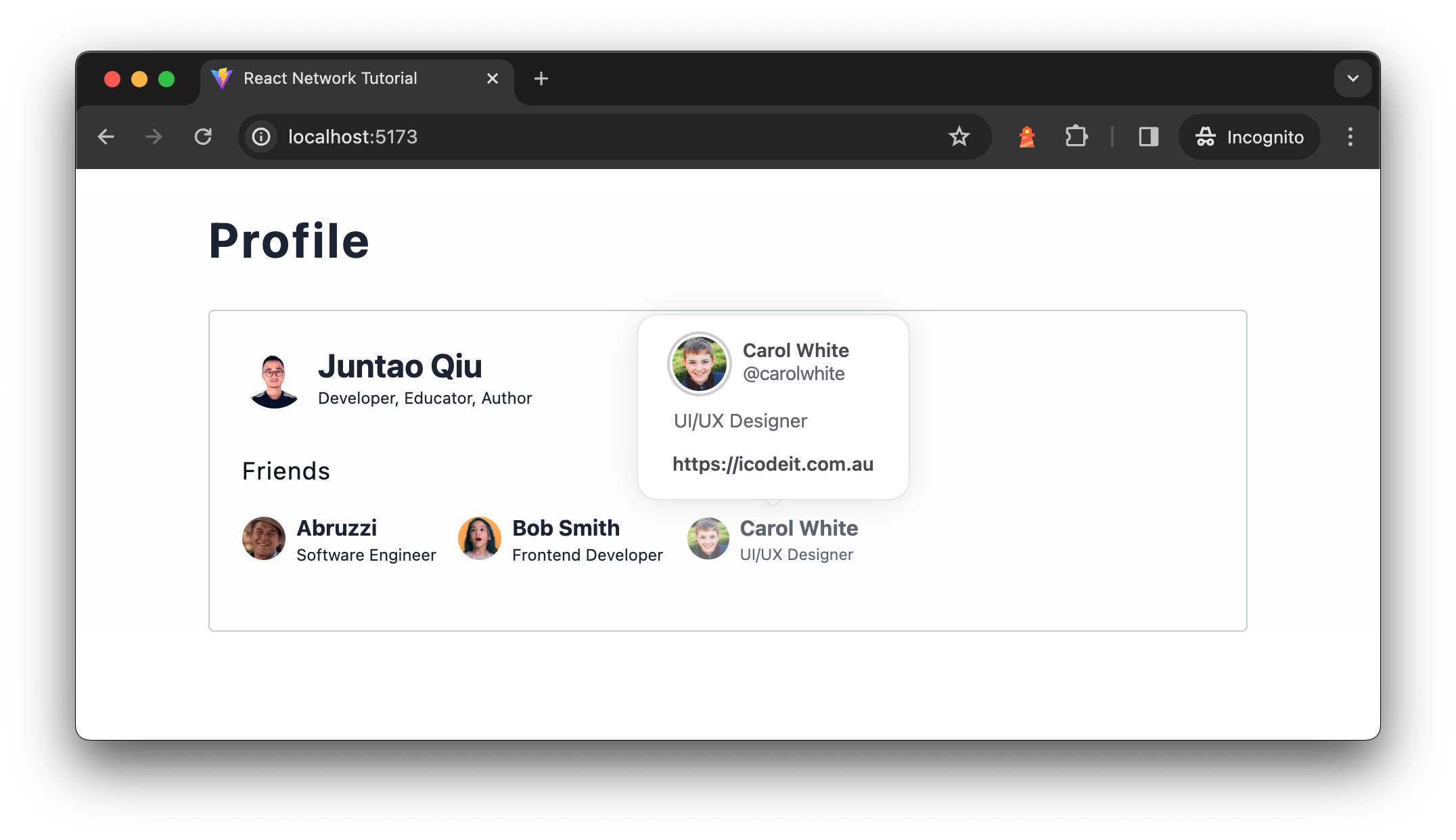
Determine 11: Displaying person element
card part when hover
When the popup reveals up, we have to ship one other service name to get
the person particulars (like their homepage and variety of connections, and so on.). We
might want to replace the Pal
part ((the one we use to
render every merchandise within the Pals checklist) ) to one thing just like the
following.
import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./person.tsx"; import UserDetailCard from "./user-detail-card.tsx"; export const Pal = ({ person }: { person: Consumer }) => { return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button> <UserBrief person={person} /> </button> </PopoverTrigger> <PopoverContent> <UserDetailCard id={person.id} /> </PopoverContent> </Popover> ); };
The UserDetailCard
, is fairly just like the
Profile
part, it sends a request to load information after which
renders the outcome as soon as it will get the response.
export perform UserDetailCard({ id }: { id: string }) { const { loading, error, element } = useUserDetail(id); if (loading || !element) { return <div>Loading...</div>; } return ( <div> {/* render the person element*/} </div> ); }
We’re utilizing Popover
and the supporting parts from
nextui
, which supplies a variety of stunning and out-of-box
parts for constructing trendy UI. The one drawback right here, nonetheless, is that
the bundle itself is comparatively massive, additionally not everybody makes use of the function
(hover and present particulars), so loading that further giant bundle for everybody
isn’t perfect – it could be higher to load the UserDetailCard
on demand – every time it’s required.
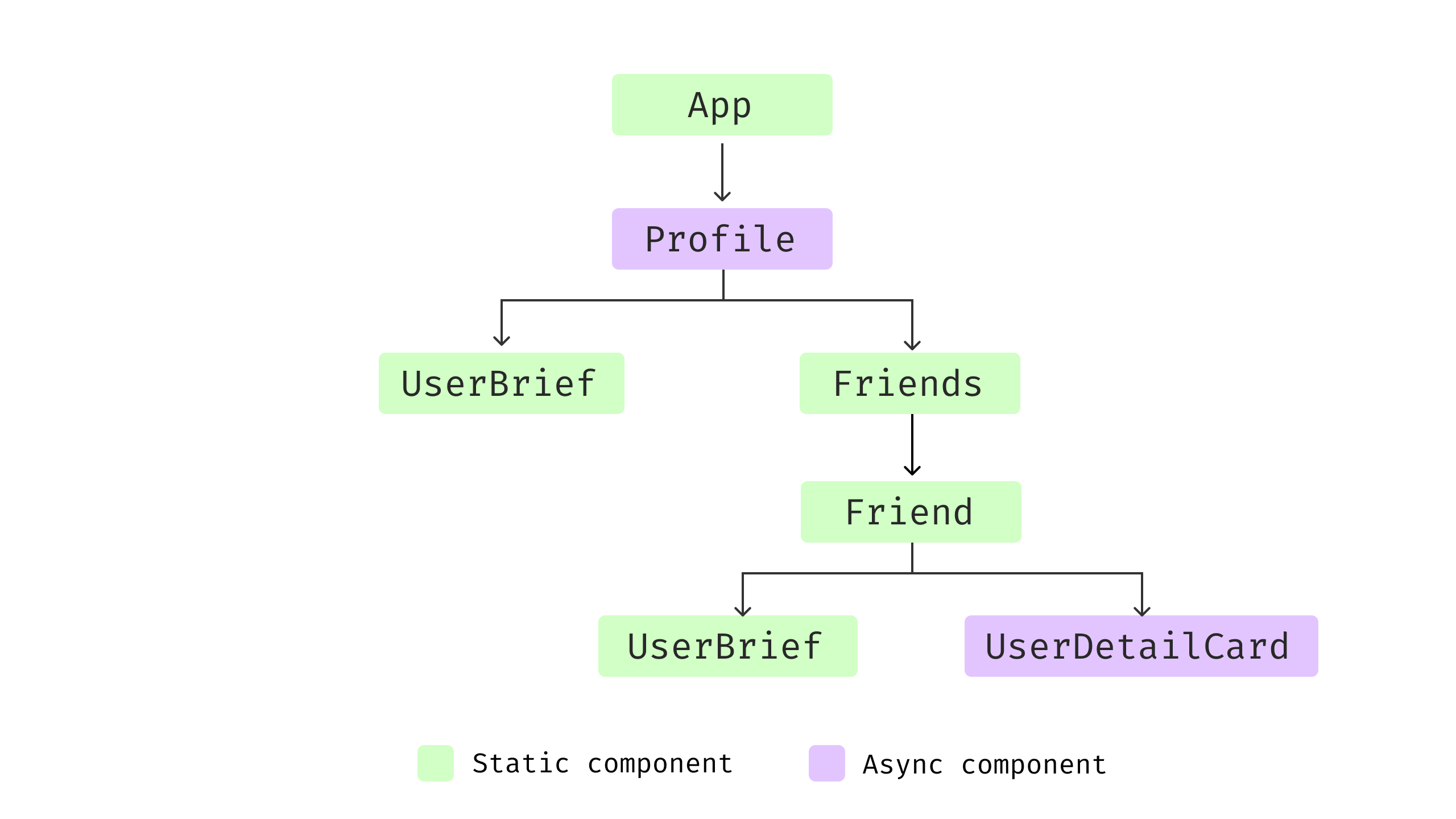
Determine 12: Part construction with
UserDetailCard
Code Splitting
Divide code into separate modules and dynamically load them as
wanted.
Code Splitting addresses the problem of enormous bundle sizes in internet
purposes by dividing the bundle into smaller chunks which are loaded as
wanted, moderately than suddenly. This improves preliminary load time and
efficiency, particularly essential for giant purposes or these with
many routes.
This optimization is often carried out at construct time, the place advanced
or sizable modules are segregated into distinct bundles. These are then
dynamically loaded, both in response to person interactions or
preemptively, in a way that doesn’t hinder the crucial rendering path
of the applying.
Leveraging the Dynamic Import Operator
The dynamic import operator in JavaScript streamlines the method of
loading modules. Although it could resemble a perform name in your code,
reminiscent of import("./user-detail-card.tsx")
, it is essential to
acknowledge that import
is definitely a key phrase, not a
perform. This operator allows the asynchronous and dynamic loading of
JavaScript modules.
With dynamic import, you’ll be able to load a module on demand. For instance, we
solely load a module when a button is clicked:
button.addEventListener("click on", (e) => { import("/modules/some-useful-module.js") .then((module) => { module.doSomethingInteresting(); }) .catch(error => { console.error("Did not load the module:", error); }); });
The module just isn’t loaded through the preliminary web page load. As a substitute, the
import()
name is positioned inside an occasion listener so it solely
be loaded when, and if, the person interacts with that button.
You need to use dynamic import operator in React and libraries like
Vue.js. React simplifies the code splitting and lazy load by means of the
React.lazy
and Suspense
APIs. By wrapping the
import assertion with React.lazy
, and subsequently wrapping
the part, as an example, UserDetailCard
, with
Suspense
, React defers the part rendering till the
required module is loaded. Throughout this loading section, a fallback UI is
offered, seamlessly transitioning to the precise part upon load
completion.
import React, { Suspense } from "react"; import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./person.tsx"; const UserDetailCard = React.lazy(() => import("./user-detail-card.tsx")); export const Pal = ({ person }: { person: Consumer }) => { return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button> <UserBrief person={person} /> </button> </PopoverTrigger> <PopoverContent> <Suspense fallback={<div>Loading...</div>}> <UserDetailCard id={person.id} /> </Suspense> </PopoverContent> </Popover> ); };
This snippet defines a Pal
part displaying person
particulars inside a popover from Subsequent UI, which seems upon interplay.
It leverages React.lazy
for code splitting, loading the
UserDetailCard
part solely when wanted. This
lazy-loading, mixed with Suspense
, enhances efficiency
by splitting the bundle and displaying a fallback through the load.
If we visualize the above code, it renders within the following
sequence.
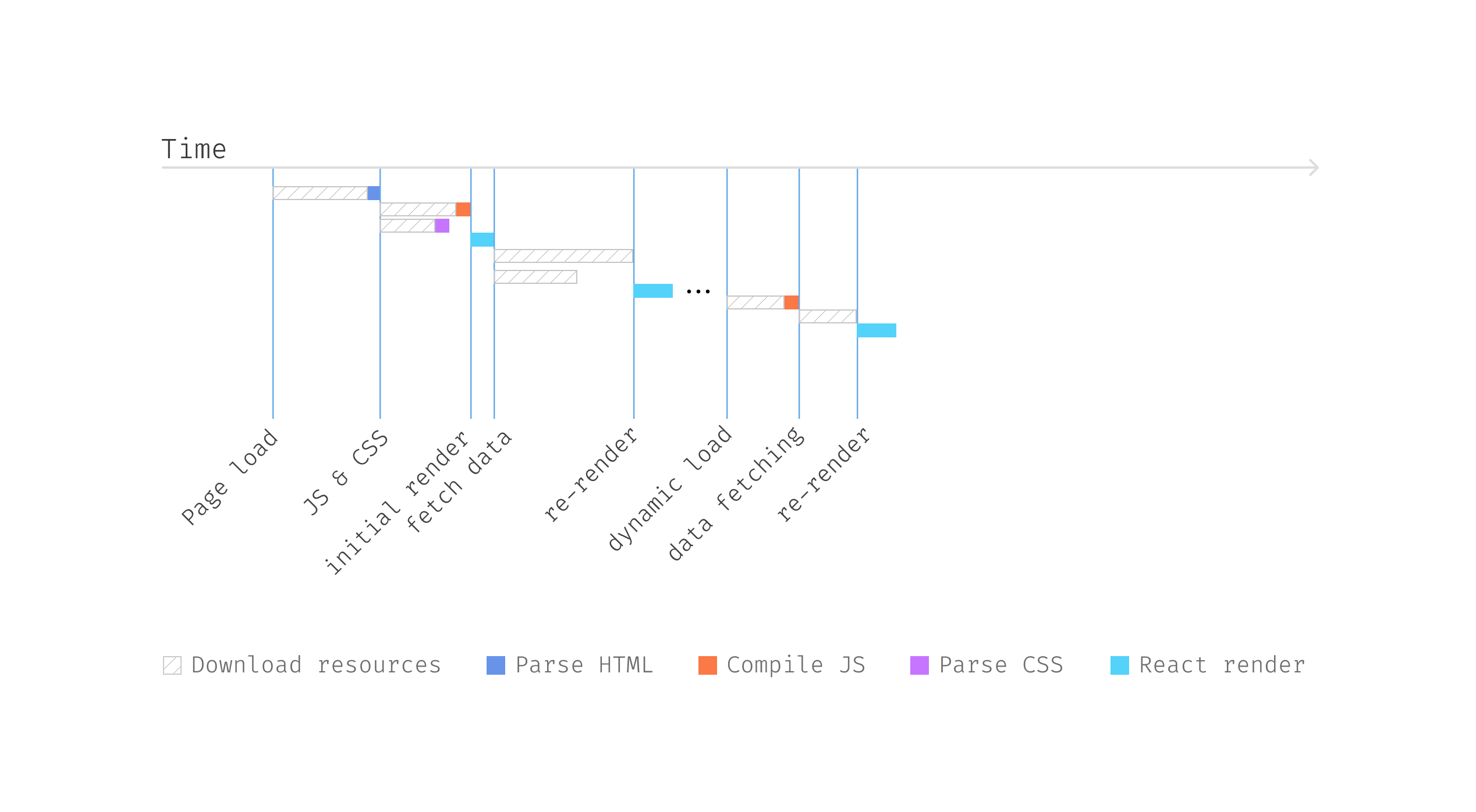
Determine 13: Dynamic load part
when wanted
Notice that when the person hovers and we obtain
the JavaScript bundle, there will probably be some further time for the browser to
parse the JavaScript. As soon as that a part of the work is completed, we are able to get the
person particulars by calling /customers/<id>/particulars
API.
Ultimately, we are able to use that information to render the content material of the popup
UserDetailCard
.
When to make use of it
Splitting out further bundles and loading them on demand is a viable
technique, however it’s essential to think about the way you implement it. Requesting
and processing a further bundle can certainly save bandwidth and lets
customers solely load what they want. Nonetheless, this method may additionally gradual
down the person expertise in sure eventualities. For instance, if a person
hovers over a button that triggers a bundle load, it might take a couple of
seconds to load, parse, and execute the JavaScript vital for
rendering. Although this delay happens solely through the first
interplay, it won’t present the perfect expertise.
To enhance perceived efficiency, successfully utilizing React Suspense to
show a skeleton or one other loading indicator will help make the
loading course of appear faster. Moreover, if the separate bundle is
not considerably giant, integrating it into the primary bundle could possibly be a
extra simple and cost-effective method. This fashion, when a person
hovers over parts like UserBrief
, the response might be
quick, enhancing the person interplay with out the necessity for separate
loading steps.
Lazy load in different frontend libraries
Once more, this sample is broadly adopted in different frontend libraries as
properly. For instance, you should use defineAsyncComponent
in Vue.js to
obtain the samiliar outcome – solely load a part whenever you want it to
render:
<template> <Popover placement="backside" show-arrow offset="10"> <!-- the remainder of the template --> </Popover> </template> <script> import { defineAsyncComponent } from 'vue'; import Popover from 'path-to-popover-component'; import UserBrief from './UserBrief.vue'; const UserDetailCard = defineAsyncComponent(() => import('./UserDetailCard.vue')); // rendering logic </script>
The perform defineAsyncComponent
defines an async
part which is lazy loaded solely when it’s rendered identical to the
React.lazy
.
As you may need already seen the seen, we’re operating right into a Request Waterfall right here once more: we load the
JavaScript bundle first, after which when it execute it sequentially name
person particulars API, which makes some further ready time. We might request
the JavaScript bundle and the community request parallely. That means,
every time a Pal
part is hovered, we are able to set off a
community request (for the information to render the person particulars) and cache the
outcome, in order that by the point when the bundle is downloaded, we are able to use
the information to render the part instantly.
[ad_2]