Information Fetching Patterns in Single-Web page Purposes
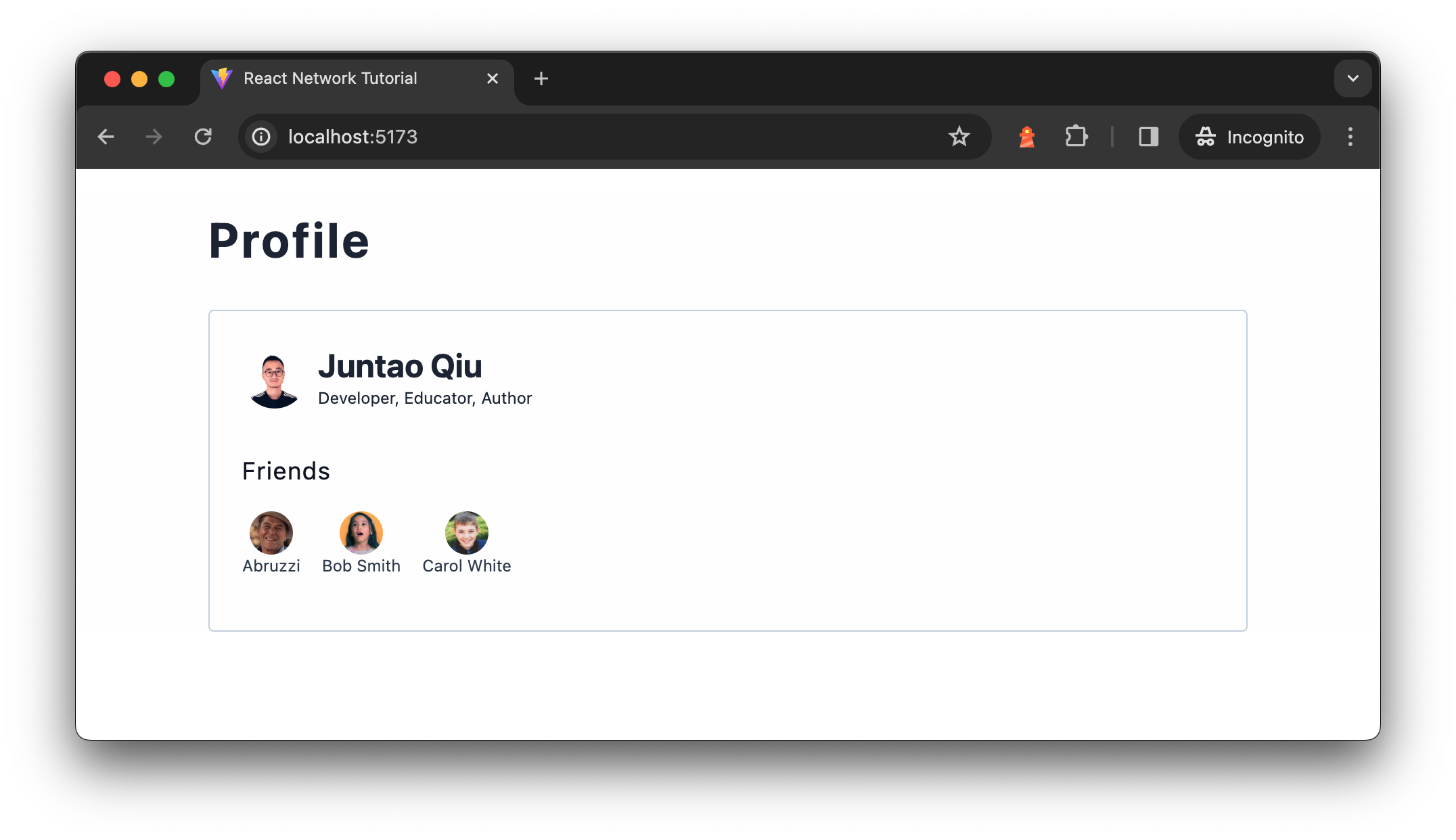
Right now, most functions can ship lots of of requests for a single web page.
For instance, my Twitter residence web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
property (JavaScript, CSS, font recordsdata, icons, and so on.), however there are nonetheless
round 100 requests for async knowledge fetching – both for timelines, mates,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The primary purpose a web page might include so many requests is to enhance
efficiency and consumer expertise, particularly to make the appliance really feel
quicker to the top customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In trendy net functions, customers sometimes see a primary web page with
model and different parts in lower than a second, with extra items
loading progressively.
Take the Amazon product element web page for instance. The navigation and high
bar seem nearly instantly, adopted by the product photographs, transient, and
descriptions. Then, as you scroll, “Sponsored” content material, scores,
suggestions, view histories, and extra seem.Typically, a consumer solely needs a
fast look or to match merchandise (and verify availability), making
sections like “Clients who purchased this merchandise additionally purchased” much less important and
appropriate for loading through separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, however it’s removed from sufficient in giant
functions. There are a lot of different facets to contemplate on the subject of
fetch knowledge appropriately and effectively. Information fetching is a chellenging, not
solely as a result of the character of async programming does not match our linear mindset,
and there are such a lot of elements may cause a community name to fail, but additionally
there are too many not-obvious instances to contemplate underneath the hood (knowledge
format, safety, cache, token expiry, and so on.).
On this article, I want to talk about some widespread issues and
patterns you need to think about on the subject of fetching knowledge in your frontend
functions.
We’ll start with the Asynchronous State Handler sample, which decouples
knowledge fetching from the UI, streamlining your software structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your knowledge
fetching logic. To speed up the preliminary knowledge loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Information Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical software elements and Prefetching knowledge primarily based on consumer
interactions to raise the consumer expertise.
I imagine discussing these ideas by means of a simple instance is
the perfect strategy. I goal to start out merely after which introduce extra complexity
in a manageable approach. I additionally plan to maintain code snippets, notably for
styling (I am using TailwindCSS for the UI, which may end up in prolonged
snippets in a React part), to a minimal. For these within the
full particulars, I’ve made them out there on this
repository.
Developments are additionally taking place on the server facet, with strategies like
Streaming Server-Aspect Rendering and Server Elements gaining traction in
numerous frameworks. Moreover, quite a lot of experimental strategies are
rising. Nevertheless, these matters, whereas doubtlessly simply as essential, is perhaps
explored in a future article. For now, this dialogue will focus
solely on front-end knowledge fetching patterns.
It is vital to notice that the strategies we’re overlaying are usually not
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions as a result of my in depth expertise with
it lately. Nevertheless, rules like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I am going to share
are widespread eventualities you would possibly encounter in frontend growth, regardless
of the framework you employ.
That mentioned, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display screen of a Single-Web page Software. It is a typical
software you may need used earlier than, or a minimum of the situation is typical.
We have to fetch knowledge from server facet after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the appliance
To start with, on Profile
we’ll present the consumer’s transient (together with
identify, avatar, and a brief description), after which we additionally need to present
their connections (much like followers on Twitter or LinkedIn
connections). We’ll must fetch consumer and their connections knowledge from
distant service, after which assembling these knowledge with UI on the display screen.
Determine 1: Profile display screen
The info are from two separate API calls, the consumer transient API
/customers/<id>
returns consumer transient for a given consumer id, which is a straightforward
object described as follows:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the buddy API /customers/<id>/mates
endpoint returns a listing of
mates for a given consumer, every listing merchandise within the response is similar as
the above consumer knowledge. The explanation we’ve two endpoints as an alternative of returning
a mates
part of the consumer API is that there are instances the place one
might have too many mates (say 1,000), however most individuals haven’t got many.
This in-balance knowledge construction might be fairly tough, particularly after we
must paginate. The purpose right here is that there are instances we have to deal
with a number of community requests.
A short introduction to related React ideas
As this text leverages React for instance numerous patterns, I do
not assume you already know a lot about React. Quite than anticipating you to spend so much
of time looking for the proper elements within the React documentation, I’ll
briefly introduce these ideas we will make the most of all through this
article. For those who already perceive what React parts are, and the
use of the
useState
and useEffect
hooks, it’s possible you’ll
use this hyperlink to skip forward to the following
part.
For these searching for a extra thorough tutorial, the new React documentation is a wonderful
useful resource.
What’s a React Part?
In React, parts are the elemental constructing blocks. To place it
merely, a React part is a perform that returns a bit of UI,
which might be as simple as a fraction of HTML. Take into account the
creation of a part that renders a navigation bar:
import React from 'react'; perform Navigation() { return ( <nav> <ol> <li>Dwelling</li> <li>Blogs</li> <li>Books</li> </ol> </nav> ); }
At first look, the combination of JavaScript with HTML tags might sound
unusual (it is known as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, the same syntax known as TSX is used). To make this
code useful, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
perform Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "Dwelling"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Word right here the translated code has a perform known as
React.createElement
, which is a foundational perform in
React for creating parts. JSX written in React parts is compiled
right down to React.createElement
calls behind the scenes.
The fundamental syntax of React.createElement
is:
React.createElement(kind, [props], [...children])
kind
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React part (class or useful) for
extra subtle buildings.props
: An object containing properties handed to the
ingredient or part, together with occasion handlers, types, and attributes
likeclassName
andid
.kids
: These elective arguments might be extra
React.createElement
calls, strings, numbers, or any combine
thereof, representing the ingredient’s kids.
As an example, a easy ingredient might be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Hiya, world!');
That is analogous to the JSX model:
<div className="greeting">Hiya, world!</div>
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM parts as essential.
You may then assemble your customized parts right into a tree, much like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; perform App() { return <Web page />; } perform Web page() { return <Container> <Navigation /> <Content material> <Sidebar /> <ProductList /> </Content material> <Footer /> </Container>; }
Finally, your software requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/shopper"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render(<App />);
Producing Dynamic Content material with JSX
The preliminary instance demonstrates a simple use case, however
let’s discover how we are able to create content material dynamically. As an example, how
can we generate a listing of information dynamically? In React, as illustrated
earlier, a part is basically a perform, enabling us to cross
parameters to it.
import React from 'react'; perform Navigation({ nav }) { return ( <nav> <ol> {nav.map(merchandise => <li key={merchandise}>{merchandise}</li>)} </ol> </nav> ); }
On this modified Navigation
part, we anticipate the
parameter to be an array of strings. We make the most of the map
perform to iterate over every merchandise, reworking them into
<li>
parts. The curly braces {}
signify
that the enclosed JavaScript expression ought to be evaluated and
rendered. For these curious in regards to the compiled model of this dynamic
content material dealing with:
perform Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(perform(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As an alternative of invoking Navigation
as a daily perform,
using JSX syntax renders the part invocation extra akin to
writing markup, enhancing readability:
// As an alternative of this Navigation(["Home", "Blogs", "Books"]) // We do that <Navigation nav={["Home", "Blogs", "Books"]} />
Elements in React can obtain numerous knowledge, often called props, to
modify their habits, very like passing arguments right into a perform (the
distinction lies in utilizing JSX syntax, making the code extra acquainted and
readable to these with HTML data, which aligns effectively with the ability
set of most frontend builders).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App() { let showNewOnly = false; // This flag's worth is often set primarily based on particular logic. const filteredBooks = showNewOnly ? booksData.filter(ebook => ebook.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks} /> </div> ); }
On this illustrative code snippet (non-functional however supposed to
show the idea), we manipulate the BookList
part’s displayed content material by passing it an array of books. Relying
on the showNewOnly
flag, this array is both all out there
books or solely these which are newly printed, showcasing how props can
be used to dynamically alter part output.
Managing Inside State Between Renders: useState
Constructing consumer interfaces (UI) typically transcends the technology of
static HTML. Elements often must “bear in mind” sure states and
reply to consumer interactions dynamically. As an example, when a consumer
clicks an “Add” button in a Product part, it’s a necessity to replace
the ShoppingCart part to replicate each the full value and the
up to date merchandise listing.
Within the earlier code snippet, making an attempt to set the
showNewOnly
variable to true
inside an occasion
handler doesn’t obtain the specified impact:
perform App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this does not work }; const filteredBooks = showNewOnly ? booksData.filter(ebook => ebook.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
This strategy falls quick as a result of native variables inside a perform
part don’t persist between renders. When React re-renders this
part, it does so from scratch, disregarding any modifications made to
native variables since these don’t set off re-renders. React stays
unaware of the necessity to replace the part to replicate new knowledge.
This limitation underscores the need for React’s
state
. Particularly, useful parts leverage the
useState
hook to recollect states throughout renders. Revisiting
the App
instance, we are able to successfully bear in mind the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(ebook => ebook.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Printed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow useful parts to handle inside state. It
introduces state to useful parts, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra advanced object or array. The
initialState
is simply used in the course of the first render to
initialize the state.- Return Worth:
useState
returns an array with
two parts. The primary ingredient is the present state worth, and the
second ingredient is a perform that permits updating this worth. Through the use of
array destructuring, we assign names to those returned gadgets,
sometimesstate
andsetState
, although you may
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that can be used within the part’s UI and
logic.setState
: A perform to replace the state. This perform
accepts a brand new state worth or a perform that produces a brand new state primarily based
on the earlier state. When known as, it schedules an replace to the
part’s state and triggers a re-render to replicate the modifications.
React treats state as a snapshot; updating it does not alter the
current state variable however as an alternative triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, making certain the
BookList
part receives the right knowledge, thereby
reflecting the up to date ebook listing to the consumer. This snapshot-like
habits of state facilitates the dynamic and responsive nature of React
parts, enabling them to react intuitively to consumer interactions and
different modifications.
Managing Aspect Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to handle the
idea of unintended effects. Uncomfortable side effects are operations that work together with
the skin world from the React ecosystem. Frequent examples embrace
fetching knowledge from a distant server or dynamically manipulating the DOM,
equivalent to altering the web page title.
React is primarily involved with rendering knowledge to the DOM and does
not inherently deal with knowledge fetching or direct DOM manipulation. To
facilitate these unintended effects, React offers the useEffect
hook. This hook permits the execution of unintended effects after React has
accomplished its rendering course of. If these unintended effects end in knowledge
modifications, React schedules a re-render to replicate these updates.
The useEffect
Hook accepts two arguments:
- A perform containing the facet impact logic.
- An elective dependency array specifying when the facet impact ought to be
re-invoked.
Omitting the second argument causes the facet impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t rely on any values from props or state, thus not needing to
re-run. Together with particular values within the array means the facet impact
solely re-executes if these values change.
When coping with asynchronous knowledge fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the info is
retrieved, it’s captured through the useState
hook, updating the
part’s inside state and preserving the fetched knowledge throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new knowledge.
This is a sensible instance about knowledge fetching and state
administration:
import { useEffect, useState } from "react"; kind Consumer = { id: string; identify: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return <div> <h2>{consumer?.identify}</h2> </div>; };
Within the code snippet above, inside useEffect
, an
asynchronous perform fetchUser
is outlined after which
instantly invoked. This sample is critical as a result of
useEffect
doesn’t instantly help async capabilities as its
callback. The async perform is outlined to make use of await
for
the fetch operation, making certain that the code execution waits for the
response after which processes the JSON knowledge. As soon as the info is offered,
it updates the part’s state through setUser
.
The dependency array tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
modifications, which prevents pointless community requests on
each render and fetches new consumer knowledge when the id
prop
updates.
This strategy to dealing with asynchronous knowledge fetching inside
useEffect
is a normal follow in React growth, providing a
structured and environment friendly solution to combine async operations into the
React part lifecycle.
As well as, in sensible functions, managing totally different states
equivalent to loading, error, and knowledge presentation is important too (we’ll
see it the way it works within the following part). For instance, think about
implementing standing indicators inside a Consumer part to replicate
loading, error, or knowledge states, enhancing the consumer expertise by
offering suggestions throughout knowledge fetching operations.
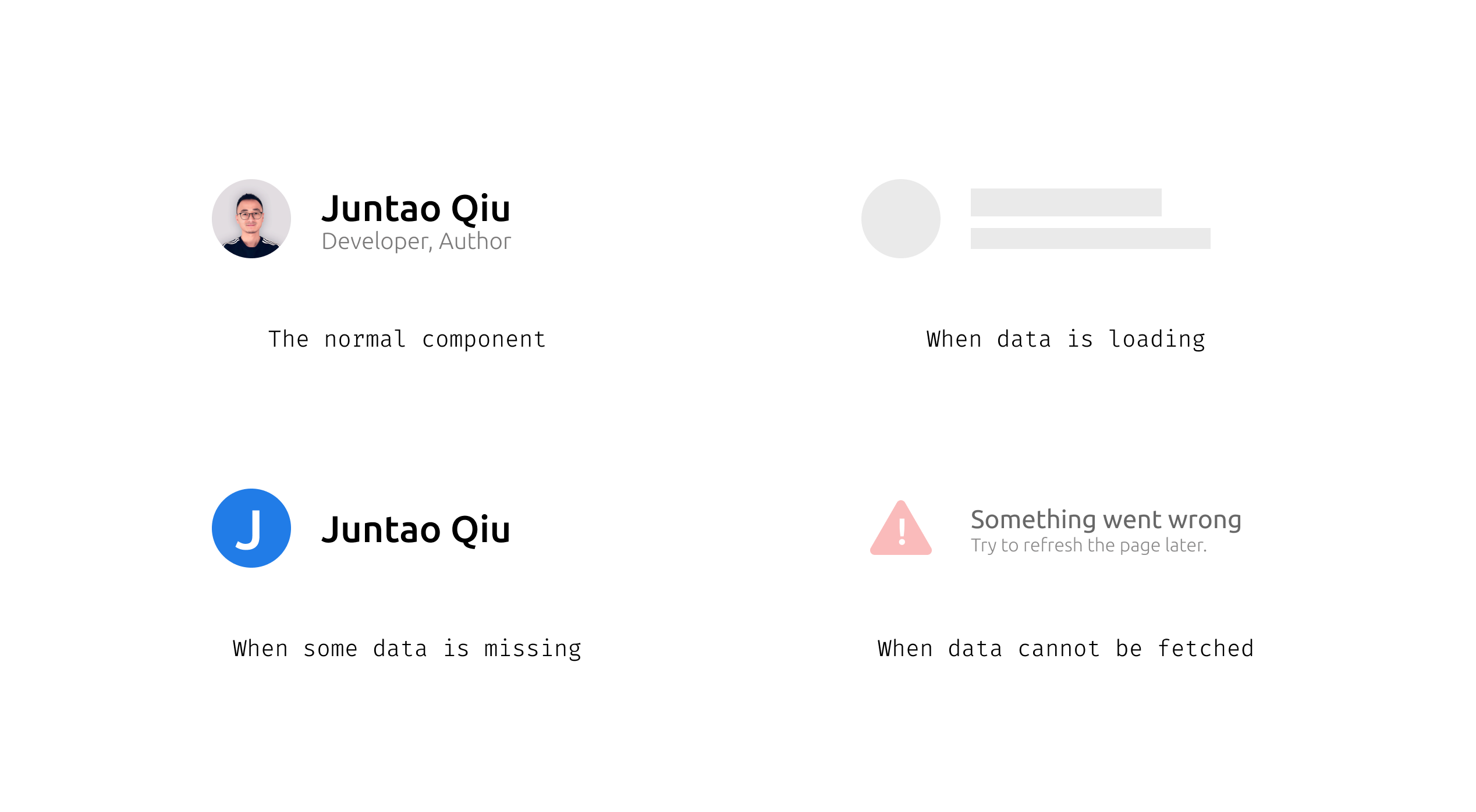
Determine 2: Completely different statuses of a
part
This overview presents only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into extra ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line assets.
With this basis, you need to now be outfitted to hitch me as we delve
into the info fetching patterns mentioned herein.
Implement the Profile part
Let’s create the Profile
part to make a request and
render the consequence. In typical React functions, this knowledge fetching is
dealt with inside a useEffect
block. This is an instance of how
this is perhaps applied:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return ( <UserBrief consumer={consumer} /> ); };
This preliminary strategy assumes community requests full
instantaneously, which is usually not the case. Actual-world eventualities require
dealing with various community circumstances, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
part. This addition permits us to supply suggestions to the consumer throughout
knowledge fetching, equivalent to displaying a loading indicator or a skeleton display screen
if the info is delayed, and dealing with errors once they happen.
Right here’s how the improved part appears with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import kind { Consumer } from "../varieties.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Consumer>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); if (loading || !consumer) { return <div>Loading...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Now in Profile
part, we provoke states for loading,
errors, and consumer knowledge with useState
. Utilizing
useEffect
, we fetch consumer knowledge primarily based on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
knowledge retrieval, we replace the consumer state, else show a loading
indicator.
The get
perform, as demonstrated beneath, simplifies
fetching knowledge from a selected endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON knowledge or throws an error for unsuccessful requests,
streamlining error dealing with and knowledge retrieval in our software. Word
it is pure TypeScript code and can be utilized in different non-React elements of the
software.
const baseurl = "https://icodeit.com.au/api/v2"; async perform get<T>(url: string): Promise<T> { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise<T>; }
React will attempt to render the part initially, however as the info
consumer
isn’t out there, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as sooner or later, the response returns, React
re-renders the Profile
part with consumer
fulfilled, so now you can see the consumer part with identify, avatar, and
title.
If we visualize the timeline of the above code, you will note
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and elegance tags, it would cease and
obtain these recordsdata, after which parse them to kind the ultimate web page. Word
that it is a comparatively sophisticated course of, and I’m oversimplifying
right here, however the primary concept of the sequence is appropriate.
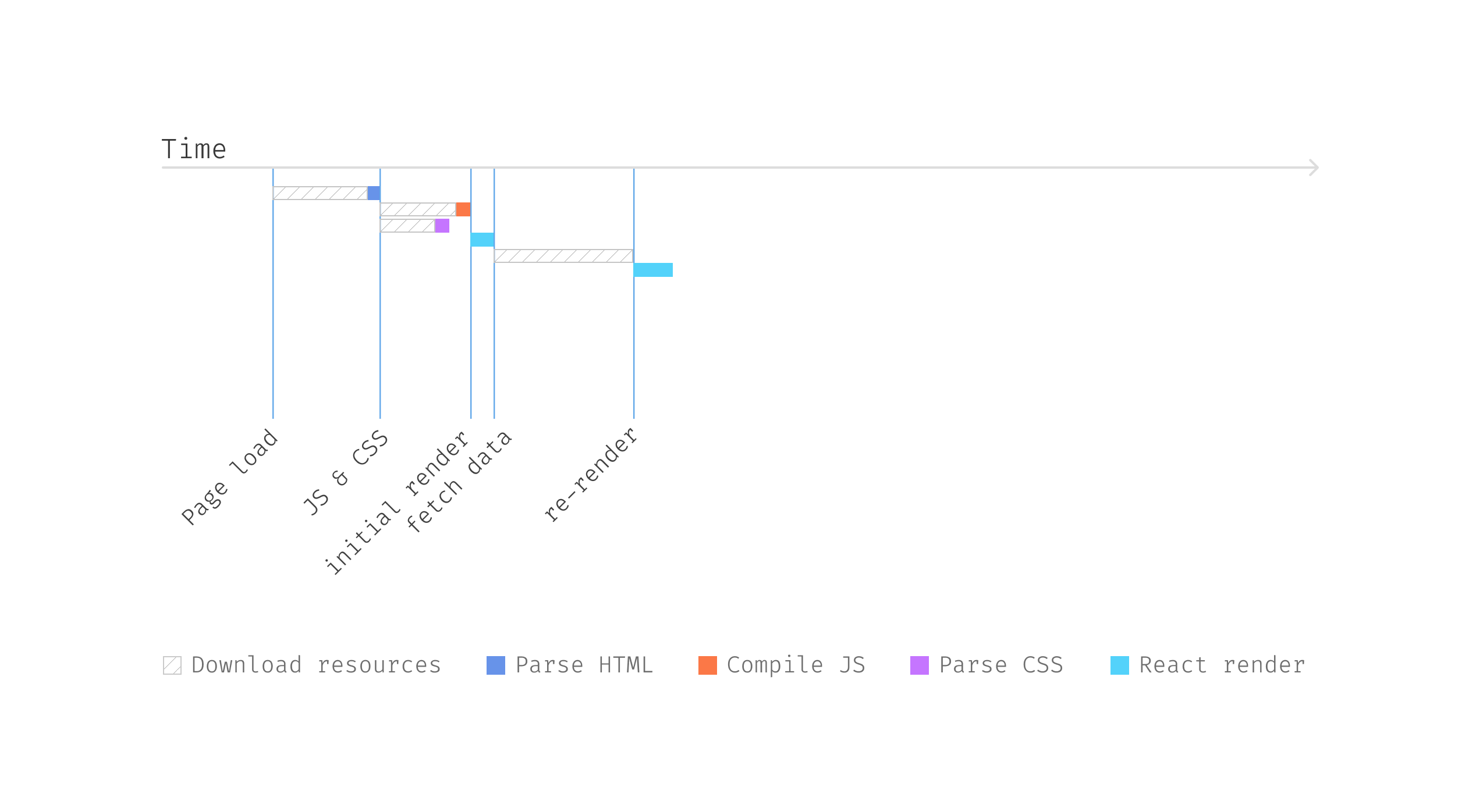
Determine 3: Fetching consumer
knowledge
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for knowledge fetching; it has to attend till
the info is offered for a re-render.
Now within the browser, we are able to see a “loading…” when the appliance
begins, after which after a number of seconds (we are able to simulate such case by add
some delay within the API endpoints) the consumer transient part exhibits up when knowledge
is loaded.
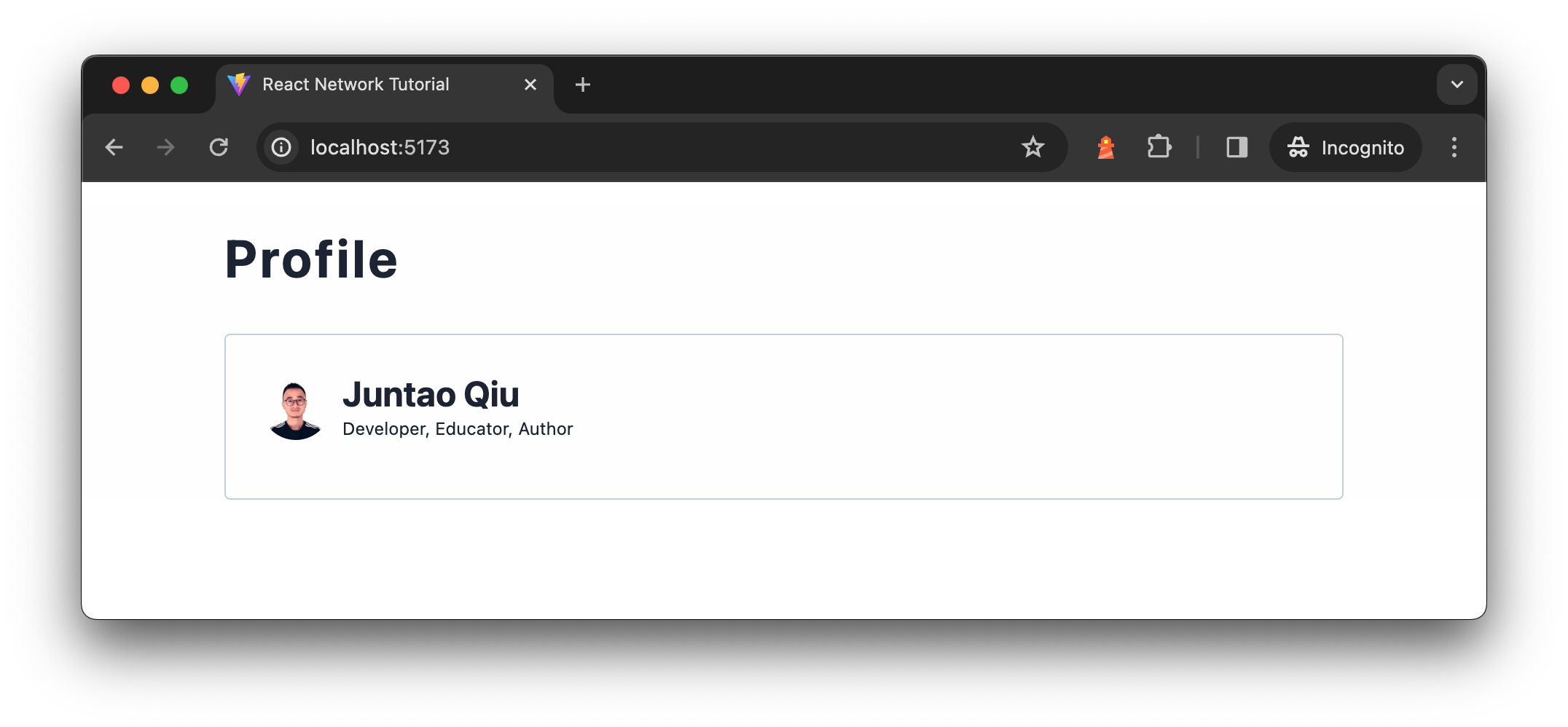
Determine 4: Consumer transient part
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
extensively used throughout React codebases. In functions of normal measurement, it is
widespread to search out quite a few cases of such similar data-fetching logic
dispersed all through numerous parts.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls might be sluggish, and it is important to not let the UI freeze
whereas these calls are being made. Subsequently, we deal with them asynchronously
and use indicators to indicate {that a} course of is underway, which makes the
consumer expertise higher – understanding that one thing is occurring.
Moreover, distant calls would possibly fail as a result of connection points,
requiring clear communication of those failures to the consumer. Subsequently,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata in regards to the standing of the decision, enabling it to show
different info or choices if the anticipated outcomes fail to
materialize.
A easy implementation may very well be a perform getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing info important for managing asynchronous
operations. This setup permits us to appropriately reply to totally different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, knowledge } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the info
The idea right here is that getAsyncStates
initiates the
community request mechanically upon being known as. Nevertheless, this may not
all the time align with the caller’s wants. To supply extra management, we are able to additionally
expose a fetch
perform inside the returned object, permitting
the initiation of the request at a extra acceptable time, in line with the
caller’s discretion. Moreover, a refetch
perform might
be supplied to allow the caller to re-initiate the request as wanted,
equivalent to after an error or when up to date knowledge is required. The
fetch
and refetch
capabilities might be similar in
implementation, or refetch
would possibly embrace logic to verify for
cached outcomes and solely re-fetch knowledge if essential.
const { loading, error, knowledge, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the info
This sample offers a flexible strategy to dealing with asynchronous
requests, giving builders the flexibleness to set off knowledge fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
functions can adapt extra dynamically to consumer interactions and different
runtime circumstances, enhancing the consumer expertise and software
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample might be applied in numerous frontend libraries. For
occasion, we might distill this strategy right into a customized Hook in a React
software for the Profile part:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Consumer | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Consumer>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return { loading, error, consumer, }; };
Please word that within the customized Hook, we have no JSX code –
which means it’s very UI free however sharable stateful logic. And the
useUser
launch knowledge mechanically when known as. Inside the Profile
part, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, consumer } = useUser(id); if (loading || !consumer) { return <div>Loading...</div>; } if (error) { return <div>One thing went mistaken...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Generalizing Parameter Utilization
In most functions, fetching various kinds of knowledge—from consumer
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a typical requirement. Writing separate
fetch capabilities for every kind of information might be tedious and troublesome to
keep. A greater strategy is to summary this performance right into a
generic, reusable hook that may deal with numerous knowledge varieties
effectively.
Take into account treating distant API endpoints as providers, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; perform useService<T>(url: string) { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [data, setData] = useState<T | undefined>(); const fetch = async () => { attempt { setLoading(true); const knowledge = await get<T>(url); setData(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, knowledge, fetch, }; }
This hook abstracts the info fetching course of, making it simpler to
combine into any part that should retrieve knowledge from a distant
supply. It additionally centralizes widespread error dealing with eventualities, equivalent to
treating particular errors in another way:
import { useService } from './useService.ts'; const { loading, error, knowledge: consumer, fetch: fetchUser, } = useService(`/customers/${id}`);
Through the use of useService, we are able to simplify how parts fetch and deal with
knowledge, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
could be expose the
fetchUsers
perform, and it doesn’t set off the info
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { attempt { setLoading(true); const knowledge = await get<Consumer>(`/customers/${id}`); setUser(knowledge); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, consumer, fetchUser, }; };
After which on the calling website, Profile
part use
useEffect
to fetch the info and render totally different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, consumer, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the power to reuse these stateful
logics throughout totally different parts. As an example, one other part
needing the identical knowledge (a consumer API name with a consumer ID) can merely import
the useUser
Hook and make the most of its states. Completely different UI
parts would possibly select to work together with these states in numerous methods,
maybe utilizing different loading indicators (a smaller spinner that
matches to the calling part) or error messages, but the elemental
logic of fetching knowledge stays constant and shared.
When to make use of it
Separating knowledge fetching logic from UI parts can typically
introduce pointless complexity, notably in smaller functions.
Conserving this logic built-in inside the part, much like the
css-in-js strategy, simplifies navigation and is simpler for some
builders to handle. In my article, Modularizing
React Purposes with Established UI Patterns, I explored
numerous ranges of complexity in software buildings. For functions
which are restricted in scope — with just some pages and a number of other knowledge
fetching operations — it is typically sensible and in addition really useful to
keep knowledge fetching inside the UI parts.
Nevertheless, as your software scales and the event group grows,
this technique might result in inefficiencies. Deep part timber can sluggish
down your software (we are going to see examples in addition to the right way to tackle
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling knowledge fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to stability simplicity with structured approaches as your
venture evolves. This ensures your growth practices stay
efficient and attentive to the appliance’s wants, sustaining optimum
efficiency and developer effectivity whatever the venture
scale.
Implement the Pals listing
Now let’s take a look on the second part of the Profile – the buddy
listing. We are able to create a separate part Pals
and fetch knowledge in it
(through the use of a useService customized hook we outlined above), and the logic is
fairly much like what we see above within the Profile
part.
const Pals = ({ id }: { id: string }) => { const { loading, error, knowledge: mates } = useService(`/customers/${id}/mates`); // loading & error dealing with... return ( <div> <h2>Pals</h2> <div> {mates.map((consumer) => ( // render consumer listing ))} </div> </div> ); };
After which within the Profile part, we are able to use Pals as a daily
part, and cross in id
as a prop:
const Profile = ({ id }: { id: string }) => { //... return ( <> {consumer && <UserBrief consumer={consumer} />} <Pals id={id} /> </> ); };
The code works nice, and it appears fairly clear and readable,
UserBrief
renders a consumer
object handed in, whereas
Pals
handle its personal knowledge fetching and rendering logic
altogether. If we visualize the part tree, it will be one thing like
this:
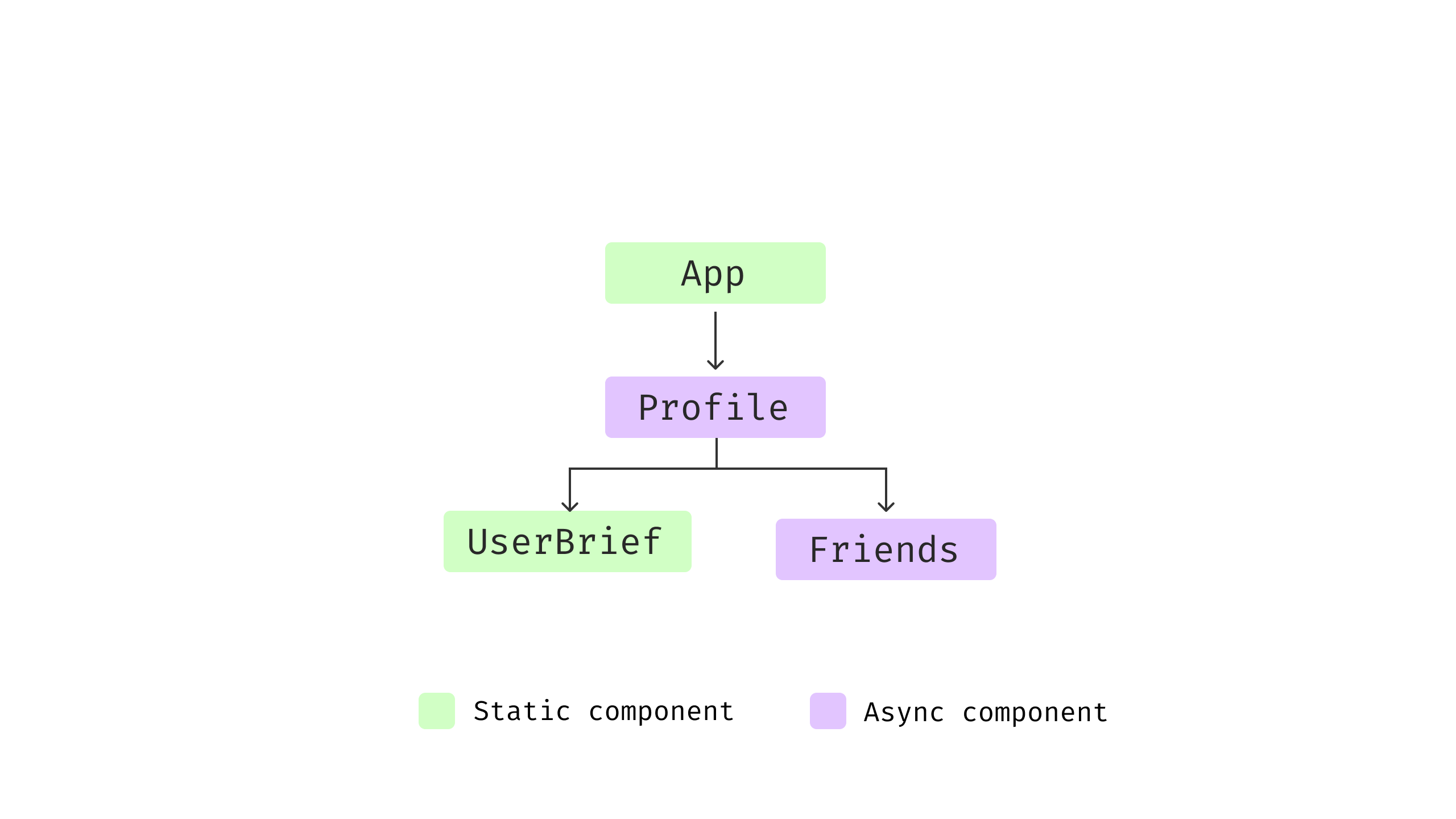
Determine 5: Part construction
Each the Profile
and Pals
have logic for
knowledge fetching, loading checks, and error dealing with. Since there are two
separate knowledge fetching calls, and if we have a look at the request timeline, we
will discover one thing attention-grabbing.
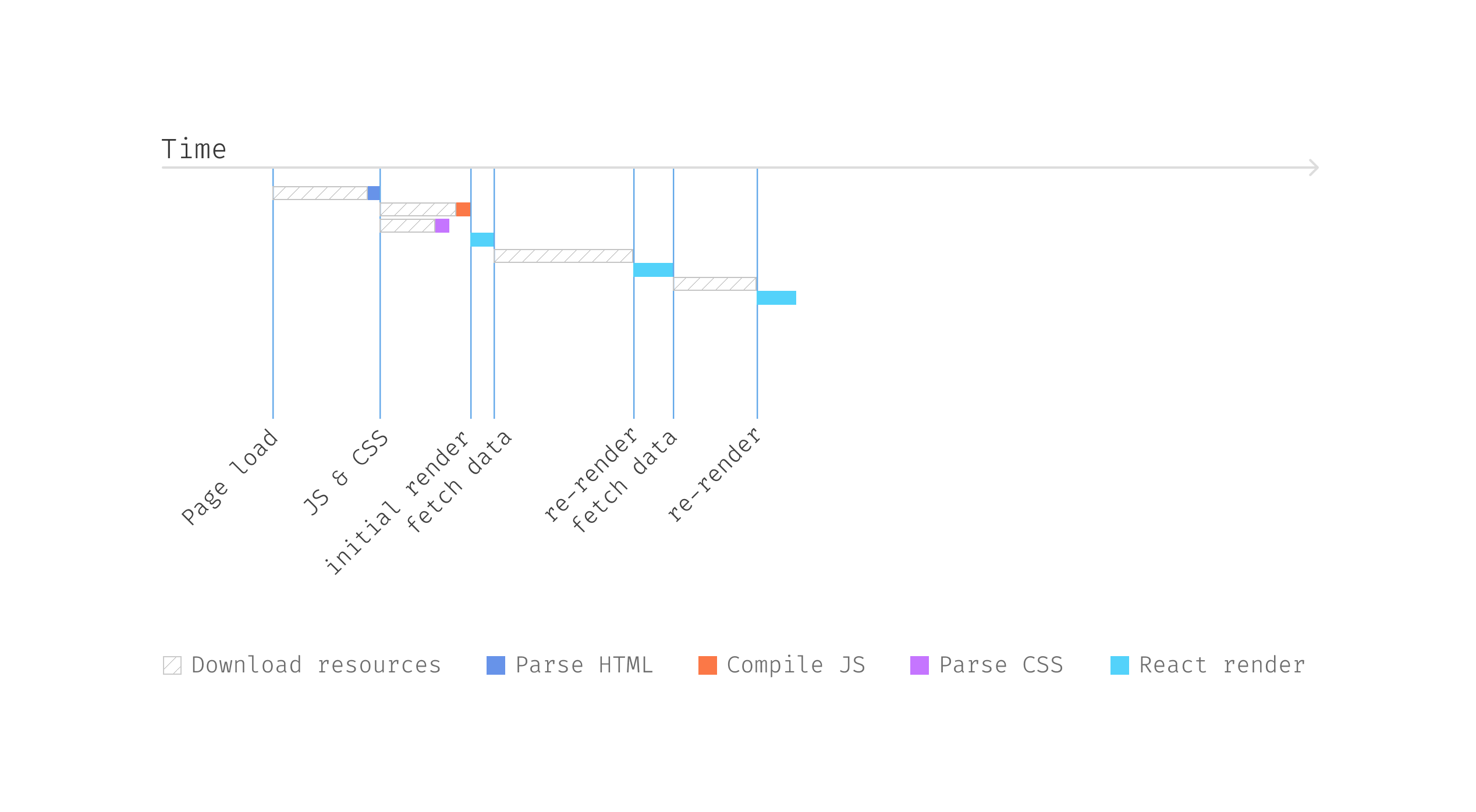
Determine 6: Request waterfall
The Pals
part will not provoke knowledge fetching till the consumer
state is ready. That is known as the Fetch-On-Render strategy,
the place the preliminary rendering is paused as a result of the info is not out there,
requiring React to attend for the info to be retrieved from the server
facet.
This ready interval is considerably inefficient, contemplating that whereas
React’s rendering course of solely takes a number of milliseconds, knowledge fetching can
take considerably longer, typically seconds. In consequence, the Pals
part spends most of its time idle, ready for knowledge. This situation
results in a typical problem often called the Request Waterfall, a frequent
incidence in frontend functions that contain a number of knowledge fetching
operations.
Parallel Information Fetching
Run distant knowledge fetches in parallel to attenuate wait time
Think about after we construct a bigger software {that a} part that
requires knowledge might be deeply nested within the part tree, to make the
matter worse these parts are developed by totally different groups, it’s laborious
to see whom we’re blocking.
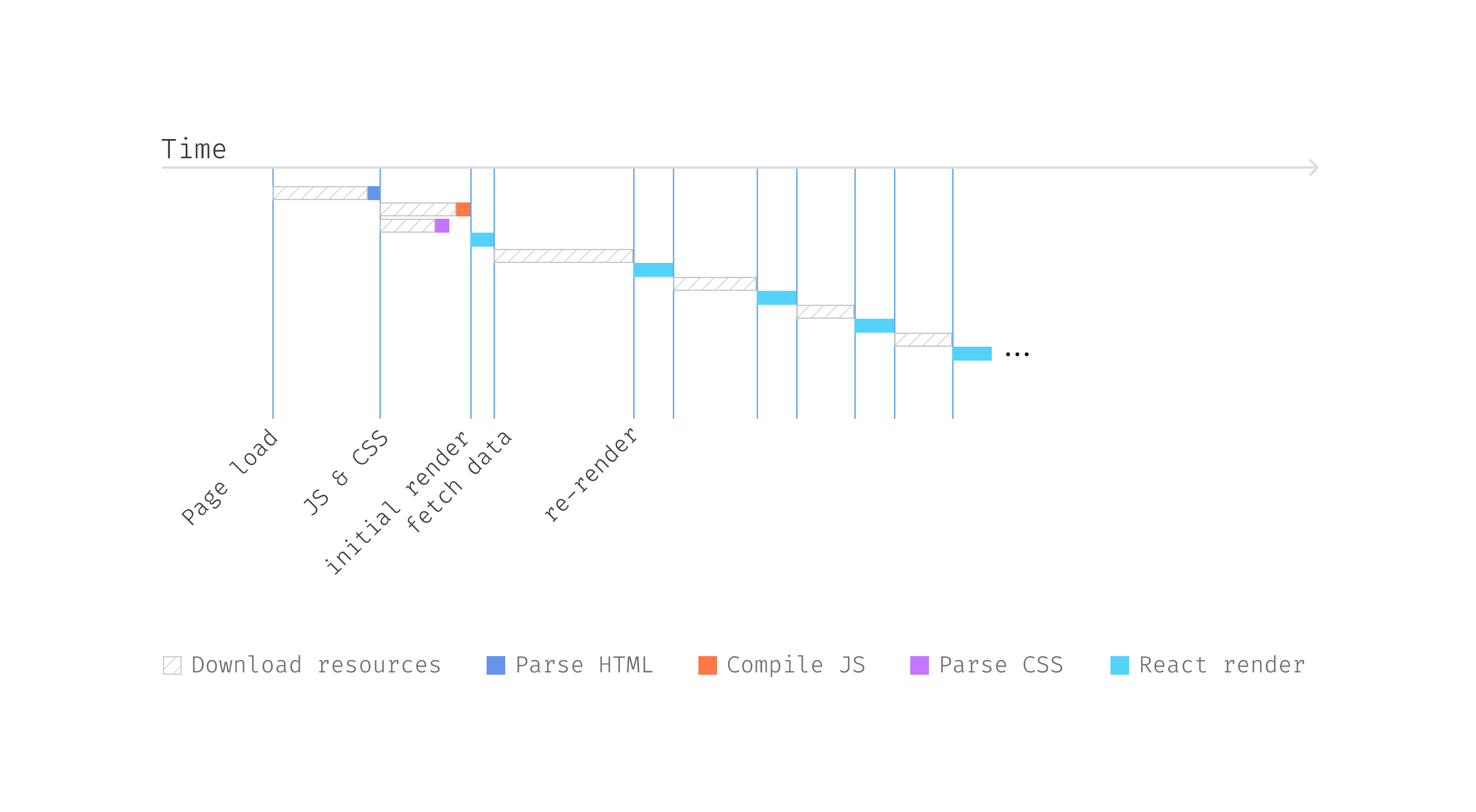
Determine 7: Request waterfall
Request Waterfalls can degrade consumer
expertise, one thing we goal to keep away from. Analyzing the info, we see that the
consumer API and mates API are unbiased and might be fetched in parallel.
Initiating these parallel requests turns into important for software
efficiency.
One strategy is to centralize knowledge fetching at the next stage, close to the
root. Early within the software’s lifecycle, we begin all knowledge fetches
concurrently. Elements depending on this knowledge wait just for the
slowest request, sometimes leading to quicker general load occasions.
We might use the Promise API Promise.all
to ship
each requests for the consumer’s primary info and their mates listing.
Promise.all
is a JavaScript technique that permits for the
concurrent execution of a number of guarantees. It takes an array of guarantees
as enter and returns a single Promise that resolves when all the enter
guarantees have resolved, offering their outcomes as an array. If any of the
guarantees fail, Promise.all
instantly rejects with the
purpose of the primary promise that rejects.
As an example, on the software’s root, we are able to outline a complete
knowledge mannequin:
kind ProfileState = { consumer: Consumer; mates: Consumer[]; }; const getProfileData = async (id: string) => Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/mates`), ]); const App = () => { // fetch knowledge on the very begining of the appliance launch const onInit = () => { const [user, friends] = await getProfileData(id); } // render the sub tree correspondingly }
Implementing Parallel Information Fetching in React
Upon software launch, knowledge fetching begins, abstracting the
fetching course of from subcomponents. For instance, in Profile part,
each UserBrief and Pals are presentational parts that react to
the handed knowledge. This manner we might develop these part individually
(including types for various states, for instance). These presentational
parts usually are straightforward to check and modify as we’ve separate the
knowledge fetching and rendering.
We are able to outline a customized hook useProfileData
that facilitates
parallel fetching of information associated to a consumer and their mates through the use of
Promise.all
. This technique permits simultaneous requests, optimizing the
loading course of and structuring the info right into a predefined format identified
as ProfileData
.
Right here’s a breakdown of the hook implementation:
import { useCallback, useEffect, useState } from "react"; kind ProfileData = { consumer: Consumer; mates: Consumer[]; }; const useProfileData = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(undefined); const [profileState, setProfileState] = useState<ProfileData>(); const fetchProfileState = useCallback(async () => { attempt { setLoading(true); const [user, friends] = await Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/mates`), ]); setProfileState({ consumer, mates }); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }, tag:martinfowler.com,2024-05-29:Prefetching-in-Single-Web page-Purposes); return { loading, error, profileState, fetchProfileState, }; };
This hook offers the Profile
part with the
essential knowledge states (loading
, error
,
profileState
) together with a fetchProfileState
perform, enabling the part to provoke the fetch operation as
wanted. Word right here we use useCallback
hook to wrap the async
perform for knowledge fetching. The useCallback hook in React is used to
memoize capabilities, making certain that the identical perform occasion is
maintained throughout part re-renders except its dependencies change.
Just like the useEffect, it accepts the perform and a dependency
array, the perform will solely be recreated if any of those dependencies
change, thereby avoiding unintended habits in React’s rendering
cycle.
The Profile
part makes use of this hook and controls the info fetching
timing through useEffect
:
const Profile = ({ id }: { id: string }) => { const { loading, error, profileState, fetchProfileState } = useProfileData(id); useEffect(() => { fetchProfileState(); }, [fetchProfileState]); if (loading) { return <div>Loading...</div>; } if (error) { return <div>One thing went mistaken...</div>; } return ( <> {profileState && ( <> <UserBrief consumer={profileState.consumer} /> <Pals customers={profileState.mates} /> </> )} </> ); };
This strategy is also referred to as Fetch-Then-Render, suggesting that the goal
is to provoke requests as early as potential throughout web page load.
Subsequently, the fetched knowledge is utilized to drive React’s rendering of
the appliance, bypassing the necessity to handle knowledge fetching amidst the
rendering course of. This technique simplifies the rendering course of,
making the code simpler to check and modify.
And the part construction, if visualized, could be just like the
following illustration
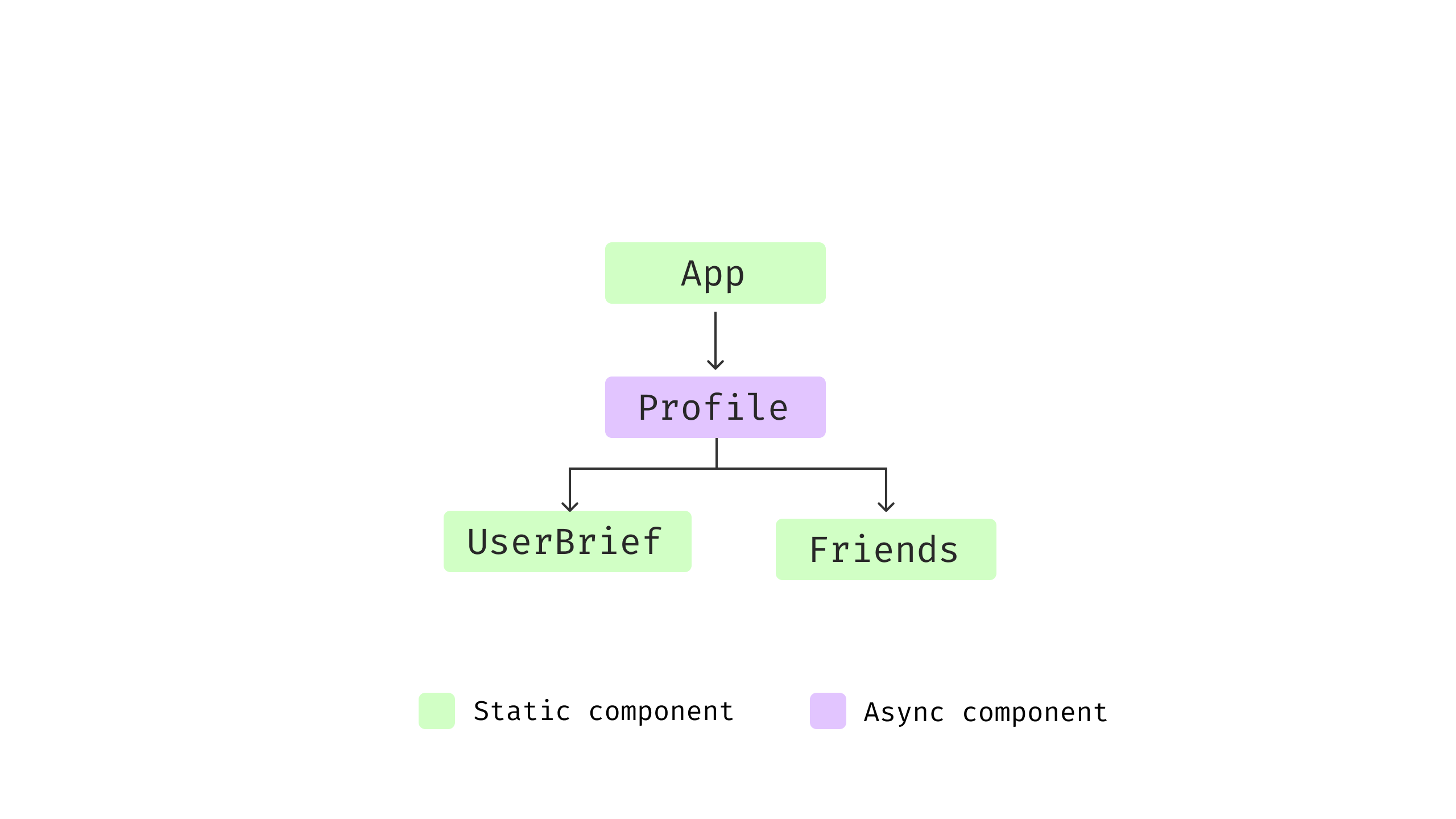
Determine 8: Part construction after refactoring
And the timeline is far shorter than the earlier one as we ship two
requests in parallel. The Pals
part can render in a number of
milliseconds as when it begins to render, the info is already prepared and
handed in.
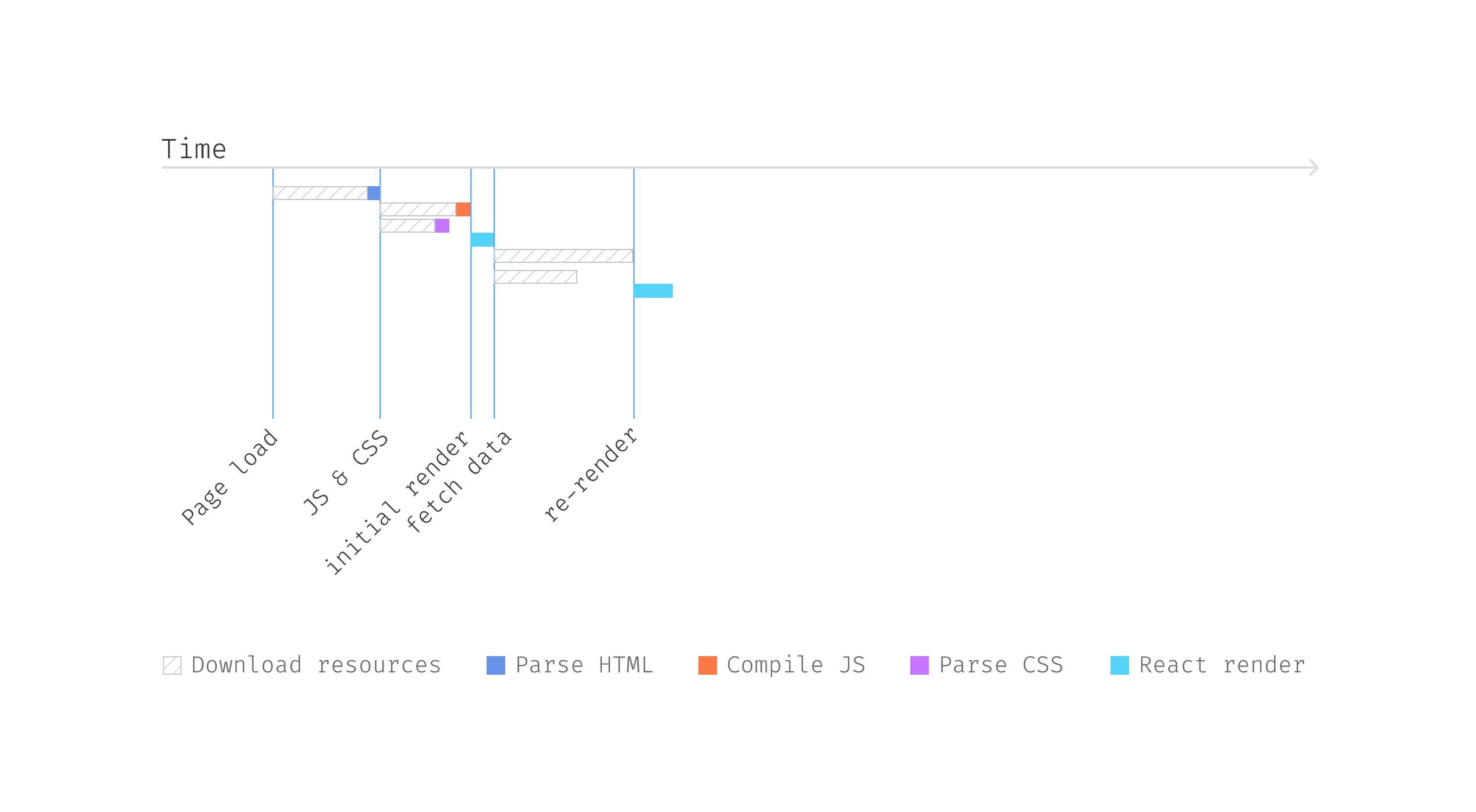
Determine 9: Parallel requests
Word that the longest wait time depends upon the slowest community
request, which is far quicker than the sequential ones. And if we might
ship as many of those unbiased requests on the similar time at an higher
stage of the part tree, a greater consumer expertise might be
anticipated.
As functions develop, managing an rising variety of requests at
root stage turns into difficult. That is notably true for parts
distant from the basis, the place passing down knowledge turns into cumbersome. One
strategy is to retailer all knowledge globally, accessible through capabilities (like
Redux or the React Context API), avoiding deep prop drilling.
When to make use of it
Working queries in parallel is beneficial at any time when such queries could also be
sluggish and do not considerably intervene with every others’ efficiency.
That is normally the case with distant queries. Even when the distant
machine’s I/O and computation is quick, there’s all the time potential latency
points within the distant calls. The primary drawback for parallel queries
is setting them up with some type of asynchronous mechanism, which can be
troublesome in some language environments.
The primary purpose to not use parallel knowledge fetching is after we do not
know what knowledge must be fetched till we have already fetched some
knowledge. Sure eventualities require sequential knowledge fetching as a result of
dependencies between requests. As an example, think about a situation on a
Profile
web page the place producing a customized advice feed
depends upon first buying the consumer’s pursuits from a consumer API.
This is an instance response from the consumer API that features
pursuits:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
In such instances, the advice feed can solely be fetched after
receiving the consumer’s pursuits from the preliminary API name. This
sequential dependency prevents us from using parallel fetching, as
the second request depends on knowledge obtained from the primary.
Given these constraints, it turns into vital to debate different
methods in asynchronous knowledge administration. One such technique is
Fallback Markup. This strategy permits builders to specify what
knowledge is required and the way it ought to be fetched in a approach that clearly
defines dependencies, making it simpler to handle advanced knowledge
relationships in an software.
One other instance of when arallel Information Fetching just isn’t relevant is
that in eventualities involving consumer interactions that require real-time
knowledge validation.
Take into account the case of a listing the place every merchandise has an “Approve” context
menu. When a consumer clicks on the “Approve” possibility for an merchandise, a dropdown
menu seems providing selections to both “Approve” or “Reject.” If this
merchandise’s approval standing may very well be modified by one other admin concurrently,
then the menu choices should replicate probably the most present state to keep away from
conflicting actions.
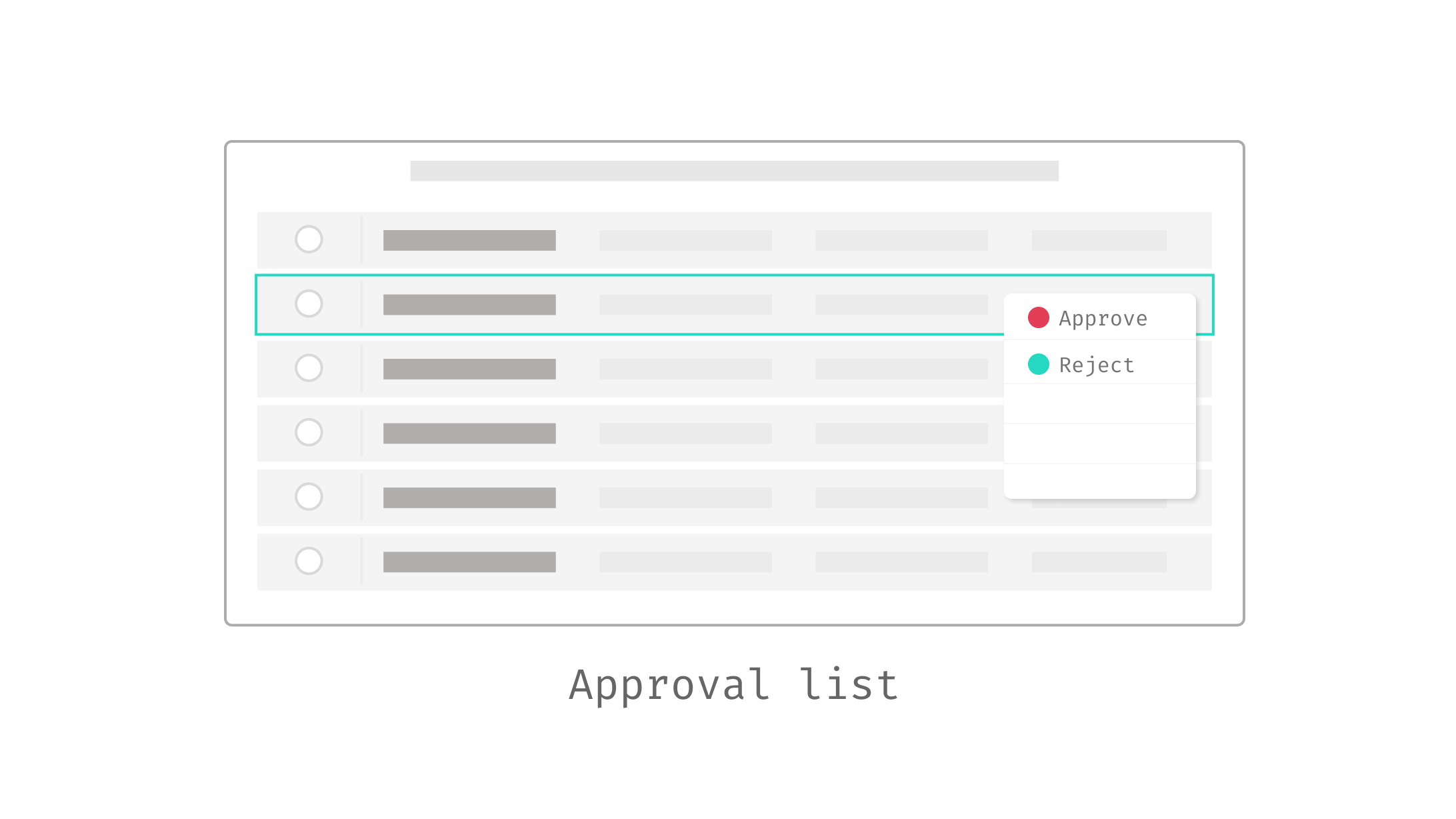
Determine 10: The approval listing that require in-time
states
To deal with this, a service name is initiated every time the context
menu is activated. This service fetches the newest standing of the merchandise,
making certain that the dropdown is constructed with probably the most correct and
present choices out there at that second. In consequence, these requests
can’t be made in parallel with different data-fetching actions for the reason that
dropdown’s contents rely totally on the real-time standing fetched from
the server.
Fallback Markup
Specify fallback shows within the web page markup
This sample leverages abstractions supplied by frameworks or libraries
to deal with the info retrieval course of, together with managing states like
loading, success, and error, behind the scenes. It permits builders to
concentrate on the construction and presentation of information of their functions,
selling cleaner and extra maintainable code.
Let’s take one other have a look at the Pals
part within the above
part. It has to take care of three totally different states and register the
callback in useEffect
, setting the flag appropriately on the proper time,
organize the totally different UI for various states:
const Pals = ({ id }: { id: string }) => { //... const { loading, error, knowledge: mates, fetch: fetchFriends, } = useService(`/customers/${id}/mates`); useEffect(() => { fetchFriends(); }, []); if (loading) { // present loading indicator } if (error) { // present error message part } // present the acutal buddy listing };
You’ll discover that inside a part we’ve to take care of
totally different states, even we extract customized Hook to cut back the noise in a
part, we nonetheless must pay good consideration to dealing with
loading
and error
inside a part. These
boilerplate code might be cumbersome and distracting, typically cluttering the
readability of our codebase.
If we consider declarative API, like how we construct our UI with JSX, the
code might be written within the following method that lets you concentrate on
what the part is doing – not the right way to do it:
<WhenError fallback={<ErrorMessage />}> <WhenInProgress fallback={<Loading />}> <Pals /> </WhenInProgress> </WhenError>
Within the above code snippet, the intention is straightforward and clear: when an
error happens, ErrorMessage
is displayed. Whereas the operation is in
progress, Loading is proven. As soon as the operation completes with out errors,
the Pals part is rendered.
And the code snippet above is fairly similiar to what already be
applied in a number of libraries (together with React and Vue.js). For instance,
the brand new Suspense
in React permits builders to extra successfully handle
asynchronous operations inside their parts, enhancing the dealing with of
loading states, error states, and the orchestration of concurrent
duties.
Implementing Fallback Markup in React with Suspense
Suspense
in React is a mechanism for effectively dealing with
asynchronous operations, equivalent to knowledge fetching or useful resource loading, in a
declarative method. By wrapping parts in a Suspense
boundary,
builders can specify fallback content material to show whereas ready for the
part’s knowledge dependencies to be fulfilled, streamlining the consumer
expertise throughout loading states.
Whereas with the Suspense API, within the Pals
you describe what you
need to get after which render:
import useSWR from "swr"; import { get } from "../utils.ts"; perform Pals({ id }: { id: string }) { const { knowledge: customers } = useSWR("/api/profile", () => get<Consumer[]>(`/customers/${id}/mates`), { suspense: true, }); return ( <div> <h2>Pals</h2> <div> {mates.map((consumer) => ( <Buddy consumer={consumer} key={consumer.id} /> ))} </div> </div> ); }
And declaratively once you use the Pals
, you employ
Suspense
boundary to wrap across the Pals
part:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
Suspense
manages the asynchronous loading of the
Pals
part, exhibiting a FriendsSkeleton
placeholder till the part’s knowledge dependencies are
resolved. This setup ensures that the consumer interface stays responsive
and informative throughout knowledge fetching, enhancing the general consumer
expertise.
Use the sample in Vue.js
It is value noting that Vue.js can also be exploring the same
experimental sample, the place you may make use of Fallback Markup utilizing:
<Suspense> <template #default> <AsyncComponent /> </template> <template #fallback> Loading... </template> </Suspense>
Upon the primary render, <Suspense>
makes an attempt to render
its default content material behind the scenes. Ought to it encounter any
asynchronous dependencies throughout this part, it transitions right into a
pending state, the place the fallback content material is displayed as an alternative. As soon as all
the asynchronous dependencies are efficiently loaded,
<Suspense>
strikes to a resolved state, and the content material
initially supposed for show (the default slot content material) is
rendered.
Deciding Placement for the Loading Part
You could surprise the place to position the FriendsSkeleton
part and who ought to handle it. Sometimes, with out utilizing Fallback
Markup, this determination is easy and dealt with instantly inside the
part that manages the info fetching:
const Pals = ({ id }: { id: string }) => { // Information fetching logic right here... if (loading) { // Show loading indicator } if (error) { // Show error message part } // Render the precise buddy listing };
On this setup, the logic for displaying loading indicators or error
messages is of course located inside the Pals
part. Nevertheless,
adopting Fallback Markup shifts this duty to the
part’s client:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
In real-world functions, the optimum strategy to dealing with loading
experiences relies upon considerably on the specified consumer interplay and
the construction of the appliance. As an example, a hierarchical loading
strategy the place a dad or mum part ceases to indicate a loading indicator
whereas its kids parts proceed can disrupt the consumer expertise.
Thus, it is essential to fastidiously think about at what stage inside the
part hierarchy the loading indicators or skeleton placeholders
ought to be displayed.
Consider Pals
and FriendsSkeleton
as two
distinct part states—one representing the presence of information, and the
different, the absence. This idea is considerably analogous to utilizing a Speical Case sample in object-oriented
programming, the place FriendsSkeleton
serves because the ‘null’
state dealing with for the Pals
part.
The hot button is to find out the granularity with which you need to
show loading indicators and to take care of consistency in these
selections throughout your software. Doing so helps obtain a smoother and
extra predictable consumer expertise.
When to make use of it
Utilizing Fallback Markup in your UI simplifies code by enhancing its readability
and maintainability. This sample is especially efficient when using
normal parts for numerous states equivalent to loading, errors, skeletons, and
empty views throughout your software. It reduces redundancy and cleans up
boilerplate code, permitting parts to focus solely on rendering and
performance.
Fallback Markup, equivalent to React’s Suspense, standardizes the dealing with of
asynchronous loading, making certain a constant consumer expertise. It additionally improves
software efficiency by optimizing useful resource loading and rendering, which is
particularly useful in advanced functions with deep part timber.
Nevertheless, the effectiveness of Fallback Markup depends upon the capabilities of
the framework you might be utilizing. For instance, React’s implementation of Suspense for
knowledge fetching nonetheless requires third-party libraries, and Vue’s help for
related options is experimental. Furthermore, whereas Fallback Markup can scale back
complexity in managing state throughout parts, it might introduce overhead in
less complicated functions the place managing state instantly inside parts might
suffice. Moreover, this sample might restrict detailed management over loading and
error states—conditions the place totally different error varieties want distinct dealing with would possibly
not be as simply managed with a generic fallback strategy.
Introducing UserDetailCard part
Let’s say we’d like a characteristic that when customers hover on high of a Buddy
,
we present a popup to allow them to see extra particulars about that consumer.
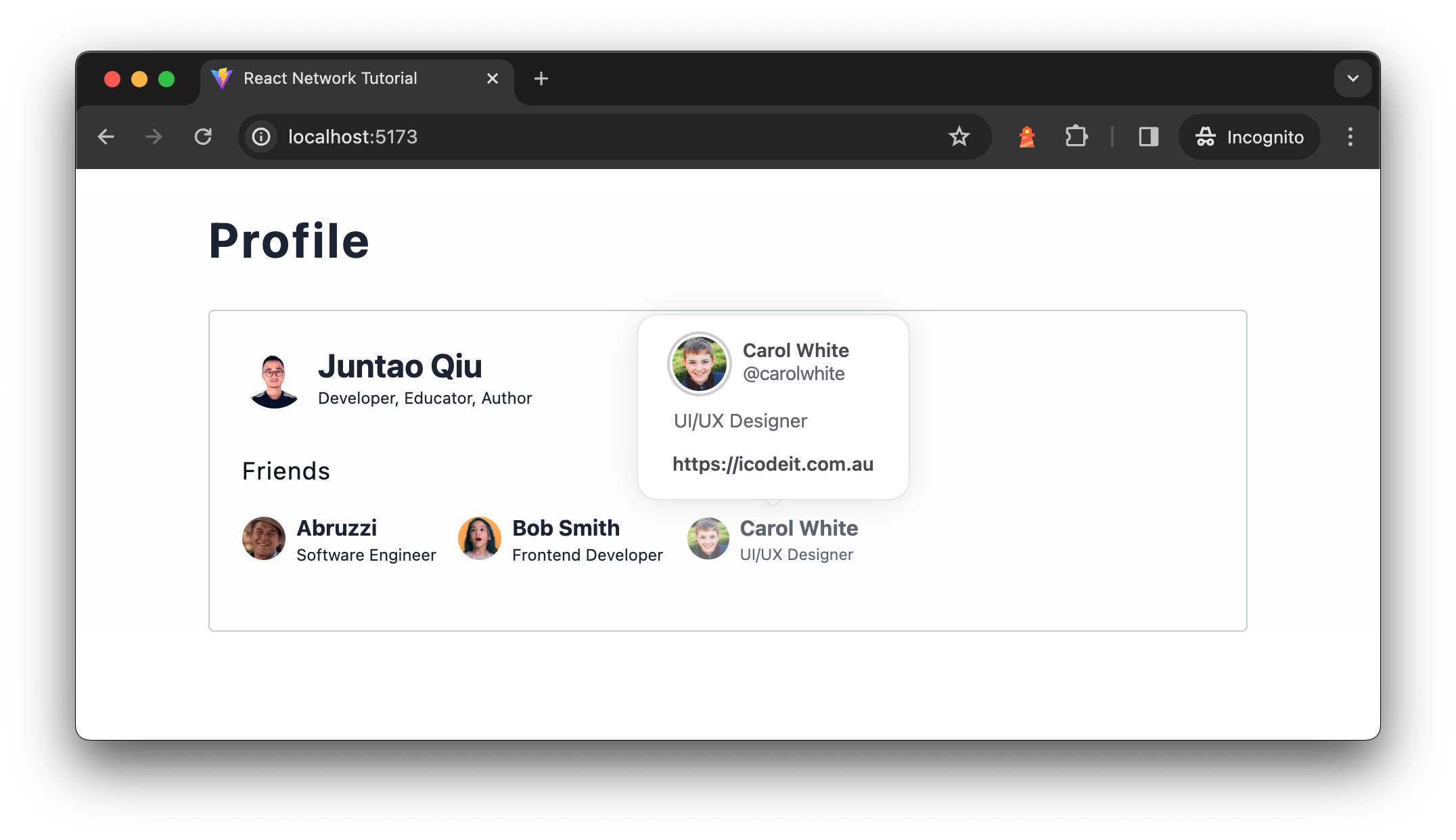
Determine 11: Exhibiting consumer element
card part when hover
When the popup exhibits up, we have to ship one other service name to get
the consumer particulars (like their homepage and variety of connections, and so on.). We
might want to replace the Buddy
part ((the one we use to
render every merchandise within the Pals listing) ) to one thing just like the
following.
import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./consumer.tsx"; import UserDetailCard from "./user-detail-card.tsx"; export const Buddy = ({ consumer }: { consumer: Consumer }) => { return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button> <UserBrief consumer={consumer} /> </button> </PopoverTrigger> <PopoverContent> <UserDetailCard id={consumer.id} /> </PopoverContent> </Popover> ); };
The UserDetailCard
, is fairly much like the
Profile
part, it sends a request to load knowledge after which
renders the consequence as soon as it will get the response.
export perform UserDetailCard({ id }: { id: string }) { const { loading, error, element } = useUserDetail(id); if (loading || !element) { return <div>Loading...</div>; } return ( <div> {/* render the consumer element*/} </div> ); }
We’re utilizing Popover
and the supporting parts from
nextui
, which offers loads of stunning and out-of-box
parts for constructing trendy UI. The one drawback right here, nonetheless, is that
the package deal itself is comparatively massive, additionally not everybody makes use of the characteristic
(hover and present particulars), so loading that further giant package deal for everybody
isn’t very best – it will be higher to load the UserDetailCard
on demand – at any time when it’s required.
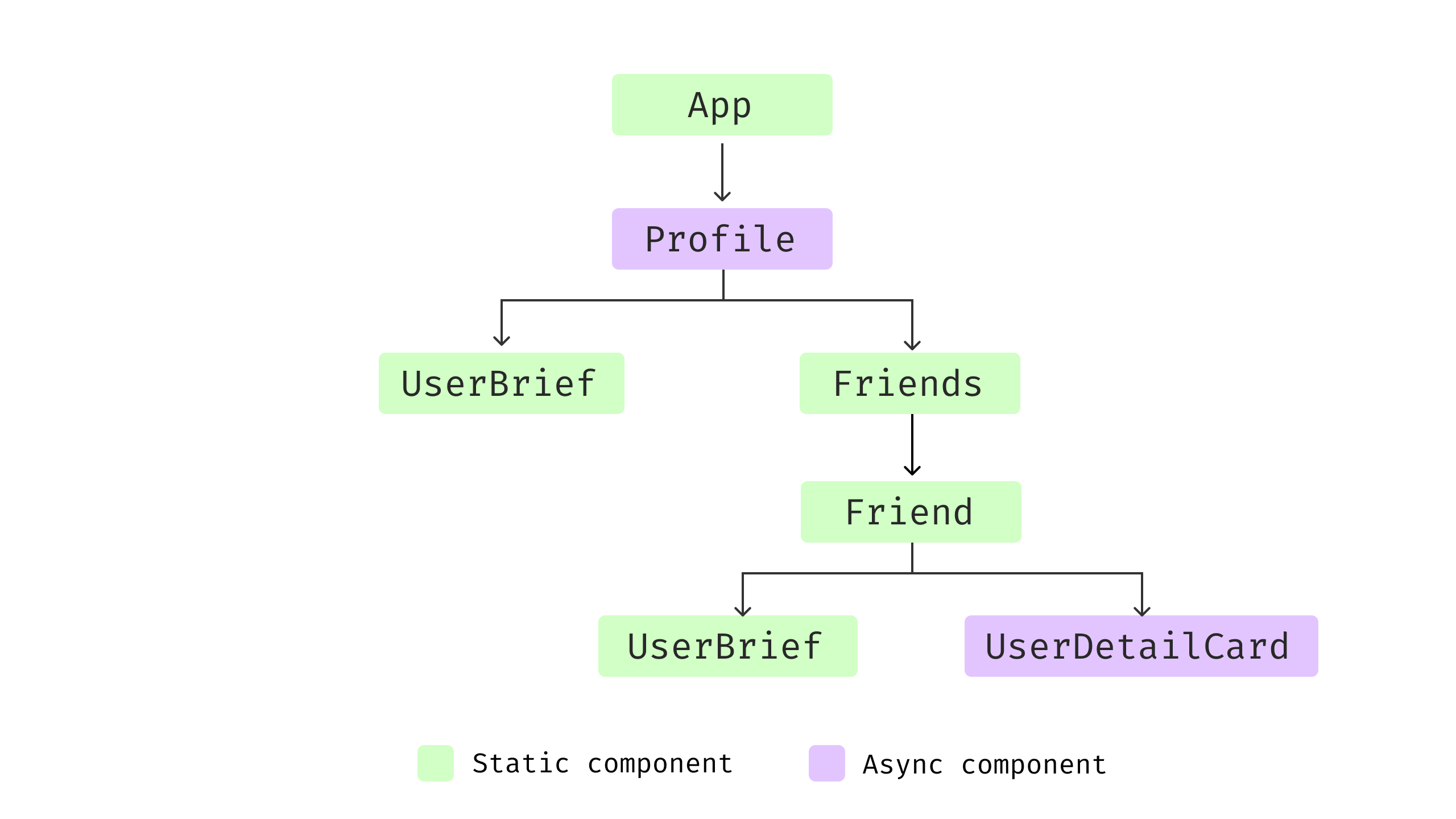
Determine 12: Part construction with
UserDetailCard
Code Splitting
Divide code into separate modules and dynamically load them as
wanted.
Code Splitting addresses the difficulty of enormous bundle sizes in net
functions by dividing the bundle into smaller chunks which are loaded as
wanted, somewhat than suddenly. This improves preliminary load time and
efficiency, particularly vital for big functions or these with
many routes.
This optimization is often carried out at construct time, the place advanced
or sizable modules are segregated into distinct bundles. These are then
dynamically loaded, both in response to consumer interactions or
preemptively, in a fashion that doesn’t hinder the important rendering path
of the appliance.
Leveraging the Dynamic Import Operator
The dynamic import operator in JavaScript streamlines the method of
loading modules. Although it might resemble a perform name in your code,
equivalent to import("./user-detail-card.tsx")
, it is vital to
acknowledge that import
is definitely a key phrase, not a
perform. This operator allows the asynchronous and dynamic loading of
JavaScript modules.
With dynamic import, you may load a module on demand. For instance, we
solely load a module when a button is clicked:
button.addEventListener("click on", (e) => { import("/modules/some-useful-module.js") .then((module) => { module.doSomethingInteresting(); }) .catch(error => { console.error("Didn't load the module:", error); }); });
The module just isn’t loaded in the course of the preliminary web page load. As an alternative, the
import()
name is positioned inside an occasion listener so it solely
be loaded when, and if, the consumer interacts with that button.
You need to use dynamic import operator in React and libraries like
Vue.js. React simplifies the code splitting and lazy load by means of the
React.lazy
and Suspense
APIs. By wrapping the
import assertion with React.lazy
, and subsequently wrapping
the part, as an example, UserDetailCard
, with
Suspense
, React defers the part rendering till the
required module is loaded. Throughout this loading part, a fallback UI is
offered, seamlessly transitioning to the precise part upon load
completion.
import React, { Suspense } from "react"; import { Popover, PopoverContent, PopoverTrigger } from "@nextui-org/react"; import { UserBrief } from "./consumer.tsx"; const UserDetailCard = React.lazy(() => import("./user-detail-card.tsx")); export const Buddy = ({ consumer }: { consumer: Consumer }) => { return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button> <UserBrief consumer={consumer} /> </button> </PopoverTrigger> <PopoverContent> <Suspense fallback={<div>Loading...</div>}> <UserDetailCard id={consumer.id} /> </Suspense> </PopoverContent> </Popover> ); };
This snippet defines a Buddy
part displaying consumer
particulars inside a popover from Subsequent UI, which seems upon interplay.
It leverages React.lazy
for code splitting, loading the
UserDetailCard
part solely when wanted. This
lazy-loading, mixed with Suspense
, enhances efficiency
by splitting the bundle and exhibiting a fallback in the course of the load.
If we visualize the above code, it renders within the following
sequence.
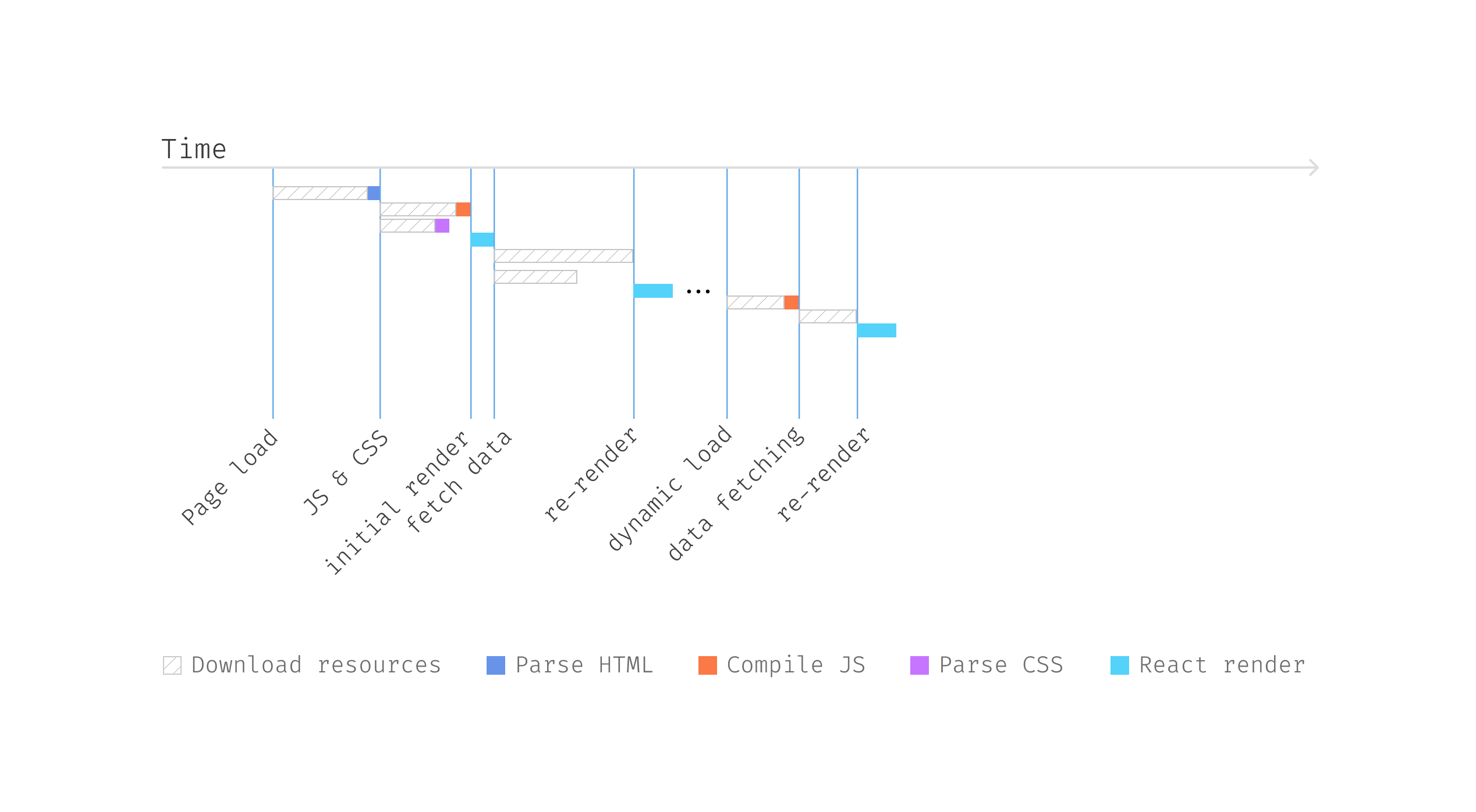
Determine 13: Dynamic load part
when wanted
Word that when the consumer hovers and we obtain
the JavaScript bundle, there can be some further time for the browser to
parse the JavaScript. As soon as that a part of the work is completed, we are able to get the
consumer particulars by calling /customers/<id>/particulars
API.
Ultimately, we are able to use that knowledge to render the content material of the popup
UserDetailCard
.
When to make use of it
Splitting out further bundles and loading them on demand is a viable
technique, however it’s essential to contemplate the way you implement it. Requesting
and processing a further bundle can certainly save bandwidth and lets
customers solely load what they want. Nevertheless, this strategy may additionally sluggish
down the consumer expertise in sure eventualities. For instance, if a consumer
hovers over a button that triggers a bundle load, it might take a number of
seconds to load, parse, and execute the JavaScript essential for
rendering. Despite the fact that this delay happens solely in the course of the first
interplay, it may not present the perfect expertise.
To enhance perceived efficiency, successfully utilizing React Suspense to
show a skeleton or one other loading indicator might help make the
loading course of appear faster. Moreover, if the separate bundle is
not considerably giant, integrating it into the primary bundle may very well be a
extra simple and cost-effective strategy. This manner, when a consumer
hovers over parts like UserBrief
, the response might be
speedy, enhancing the consumer interplay with out the necessity for separate
loading steps.
Lazy load in different frontend libraries
Once more, this sample is extensively adopted in different frontend libraries as
effectively. For instance, you should utilize defineAsyncComponent
in Vue.js to
obtain the samiliar consequence – solely load a part once you want it to
render:
<template> <Popover placement="backside" show-arrow offset="10"> <!-- the remainder of the template --> </Popover> </template> <script> import { defineAsyncComponent } from 'vue'; import Popover from 'path-to-popover-component'; import UserBrief from './UserBrief.vue'; const UserDetailCard = defineAsyncComponent(() => import('./UserDetailCard.vue')); // rendering logic </script>
The perform defineAsyncComponent
defines an async
part which is lazy loaded solely when it’s rendered identical to the
React.lazy
.
As you may need already seen the seen, we’re operating right into a Request Waterfall right here once more: we load the
JavaScript bundle first, after which when it execute it sequentially name
consumer particulars API, which makes some further ready time. We might request
the JavaScript bundle and the community request parallely. That means,
at any time when a Buddy
part is hovered, we are able to set off a
community request (for the info to render the consumer particulars) and cache the
consequence, in order that by the point when the bundle is downloaded, we are able to use
the info to render the part instantly.
Prefetching
Prefetch knowledge earlier than it might be wanted to cut back latency whether it is.
Prefetching entails loading assets or knowledge forward of their precise
want, aiming to lower wait occasions throughout subsequent operations. This
approach is especially useful in eventualities the place consumer actions can
be predicted, equivalent to navigating to a unique web page or displaying a modal
dialog that requires distant knowledge.
In follow, prefetching might be
applied utilizing the native HTML <hyperlink>
tag with a
rel="preload"
attribute, or programmatically through the
fetch
API to load knowledge or assets upfront. For knowledge that
is predetermined, the only strategy is to make use of the
<hyperlink>
tag inside the HTML <head>
:
<!doctype html> <html lang="en"> <head> <hyperlink rel="preload" href="https://martinfowler.com/bootstrap.js" as="script"> <hyperlink rel="preload" href="https://martinfowler.com/customers/u1" as="fetch" crossorigin="nameless"> <hyperlink rel="preload" href="https://martinfowler.com/customers/u1/mates" as="fetch" crossorigin="nameless"> <script kind="module" src="https://martinfowler.com/app.js"></script> </head> <physique> <div id="root"></div> </physique> </html>
With this setup, the requests for bootstrap.js
and consumer API are despatched
as quickly because the HTML is parsed, considerably sooner than when different
scripts are processed. The browser will then cache the info, making certain it
is prepared when your software initializes.
Nevertheless, it is typically not potential to know the exact URLs forward of
time, requiring a extra dynamic strategy to prefetching. That is sometimes
managed programmatically, typically by means of occasion handlers that set off
prefetching primarily based on consumer interactions or different circumstances.
For instance, attaching a mouseover
occasion listener to a button can
set off the prefetching of information. This technique permits the info to be fetched
and saved, maybe in a neighborhood state or cache, prepared for speedy use
when the precise part or content material requiring the info is interacted with
or rendered. This proactive loading minimizes latency and enhances the
consumer expertise by having knowledge prepared forward of time.
doc.getElementById('button').addEventListener('mouseover', () => { fetch(`/consumer/${consumer.id}/particulars`) .then(response => response.json()) .then(knowledge => { sessionStorage.setItem('userDetails', JSON.stringify(knowledge)); }) .catch(error => console.error(error)); });
And within the place that wants the info to render, it reads from
sessionStorage
when out there, in any other case exhibiting a loading indicator.
Usually the consumer experiense could be a lot quicker.
Implementing Prefetching in React
For instance, we are able to use preload
from the
swr
package deal (the perform identify is a bit deceptive, however it
is performing a prefetch right here), after which register an
onMouseEnter
occasion to the set off part of
Popover
,
import { preload } from "swr"; import { getUserDetail } from "../api.ts"; const UserDetailCard = React.lazy(() => import("./user-detail-card.tsx")); export const Buddy = ({ consumer }: { consumer: Consumer }) => { const handleMouseEnter = () => { preload(`/consumer/${consumer.id}/particulars`, () => getUserDetail(consumer.id)); }; return ( <Popover placement="backside" showArrow offset={10}> <PopoverTrigger> <button onMouseEnter={handleMouseEnter}> <UserBrief consumer={consumer} /> </button> </PopoverTrigger> <PopoverContent> <Suspense fallback={<div>Loading...</div>}> <UserDetailCard id={consumer.id} /> </Suspense> </PopoverContent> </Popover> ); };
That approach, the popup itself can have a lot much less time to render, which
brings a greater consumer expertise.
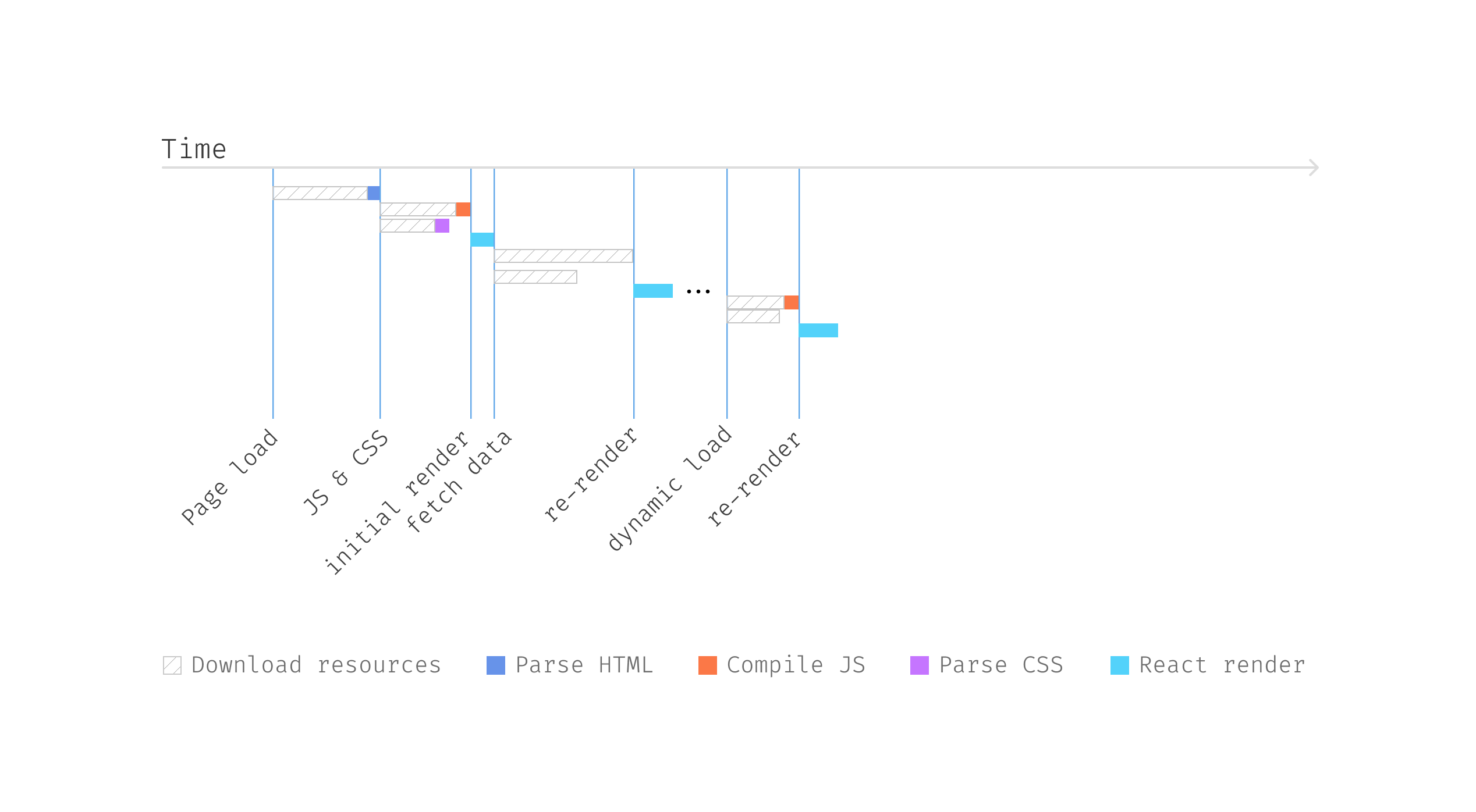
Determine 14: Dynamic load with prefetch
in parallel
So when a consumer hovers on a Buddy
, we obtain the
corresponding JavaScript bundle in addition to obtain the info wanted to
render the UserDetailCard, and by the point UserDetailCard
renders, it sees the present knowledge and renders instantly.
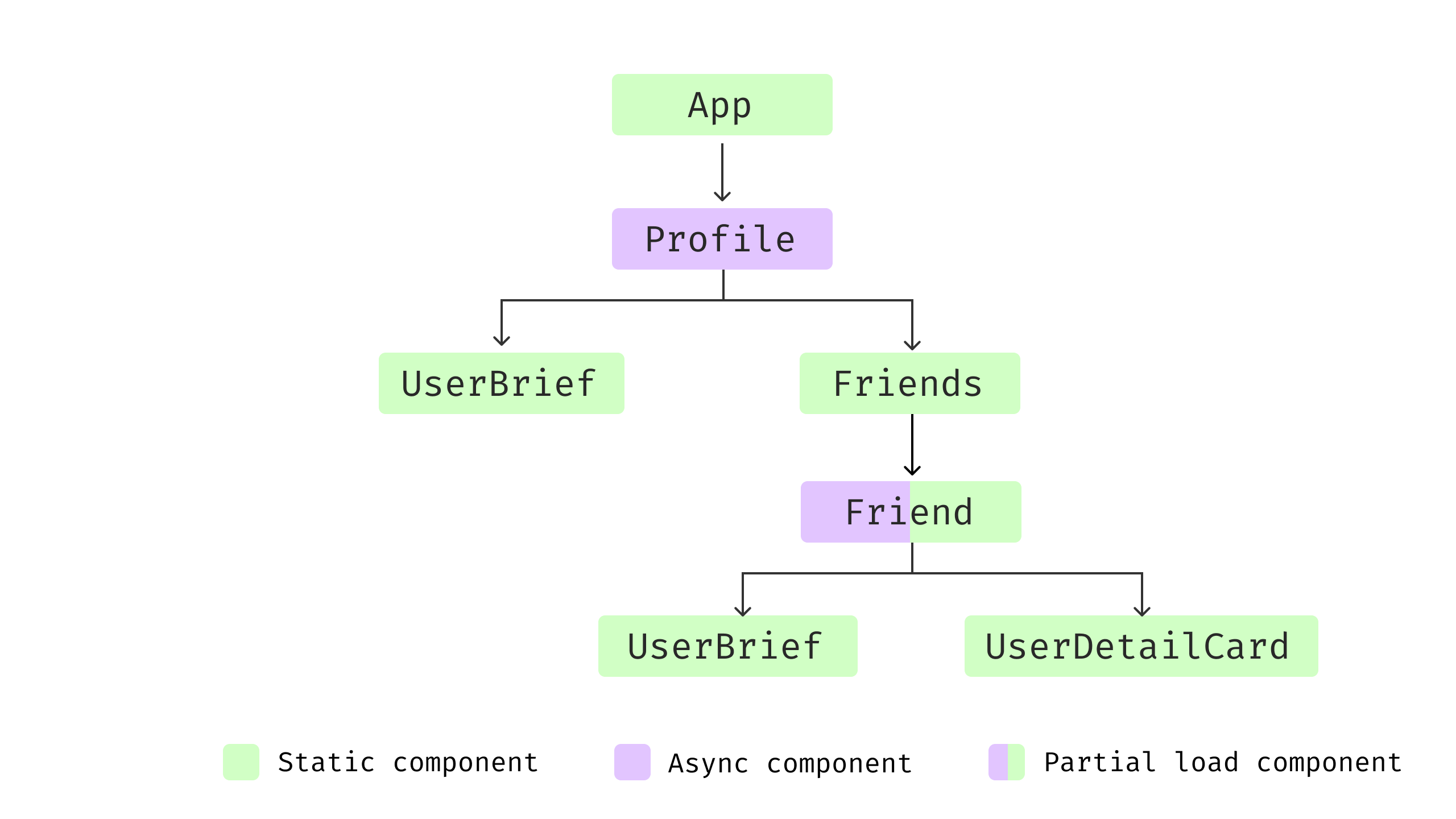
Determine 15: Part construction with
dynamic load
As the info fetching and loading is shifted to Buddy
part, and for UserDetailCard
, it reads from the native
cache maintained by swr
.
import useSWR from "swr"; export perform UserDetailCard({ id }: { id: string }) { const { knowledge: element, isLoading: loading } = useSWR( `/consumer/${id}/particulars`, () => getUserDetail(id) ); if (loading || !element) { return <div>Loading...</div>; } return ( <div> {/* render the consumer element*/} </div> ); }
This part makes use of the useSWR
hook for knowledge fetching,
making the UserDetailCard
dynamically load consumer particulars
primarily based on the given id
. useSWR
presents environment friendly
knowledge fetching with caching, revalidation, and automated error dealing with.
The part shows a loading state till the info is fetched. As soon as
the info is offered, it proceeds to render the consumer particulars.
In abstract, we have already explored important knowledge fetching methods:
Asynchronous State Handler , Parallel Information Fetching ,
Fallback Markup , Code Splitting and Prefetching . Elevating requests for parallel execution
enhances effectivity, although it isn’t all the time simple, particularly
when coping with parts developed by totally different groups with out full
visibility. Code splitting permits for the dynamic loading of
non-critical assets primarily based on consumer interplay, like clicks or hovers,
using prefetching to parallelize useful resource loading.
When to make use of it
Take into account making use of prefetching once you discover that the preliminary load time of
your software is turning into sluggish, or there are lots of options that are not
instantly essential on the preliminary display screen however may very well be wanted shortly after.
Prefetching is especially helpful for assets which are triggered by consumer
interactions, equivalent to mouse-overs or clicks. Whereas the browser is busy fetching
different assets, equivalent to JavaScript bundles or property, prefetching can load
extra knowledge upfront, thus getting ready for when the consumer truly must
see the content material. By loading assets throughout idle occasions, prefetching makes use of the
community extra effectively, spreading the load over time somewhat than inflicting spikes
in demand.
It’s clever to observe a basic guideline: do not implement advanced patterns like
prefetching till they’re clearly wanted. This is perhaps the case if efficiency
points change into obvious, particularly throughout preliminary masses, or if a major
portion of your customers entry the app from cellular units, which generally have
much less bandwidth and slower JavaScript engines. Additionally, think about that there are different
efficiency optimization ways equivalent to caching at numerous ranges, utilizing CDNs
for static property, and making certain property are compressed. These strategies can improve
efficiency with less complicated configurations and with out extra coding. The
effectiveness of prefetching depends on precisely predicting consumer actions.
Incorrect assumptions can result in ineffective prefetching and even degrade the
consumer expertise by delaying the loading of truly wanted assets.
Selecting the best sample
Choosing the suitable sample for knowledge fetching and rendering in
net growth just isn’t one-size-fits-all. Typically, a number of methods are
mixed to fulfill particular necessities. For instance, you would possibly must
generate some content material on the server facet – utilizing Server-Aspect Rendering
strategies – supplemented by client-side
Fetch-Then-Render for dynamic
content material. Moreover, non-essential sections might be break up into separate
bundles for lazy loading, presumably with Prefetching triggered by consumer
actions, equivalent to hover or click on.
Take into account the Jira difficulty web page for instance. The highest navigation and
sidebar are static, loading first to offer customers speedy context. Early
on, you are offered with the difficulty’s title, description, and key particulars
just like the Reporter and Assignee. For much less speedy info, equivalent to
the Historical past part at a problem’s backside, it masses solely upon consumer
interplay, like clicking a tab. This makes use of lazy loading and knowledge
fetching to effectively handle assets and improve consumer expertise.
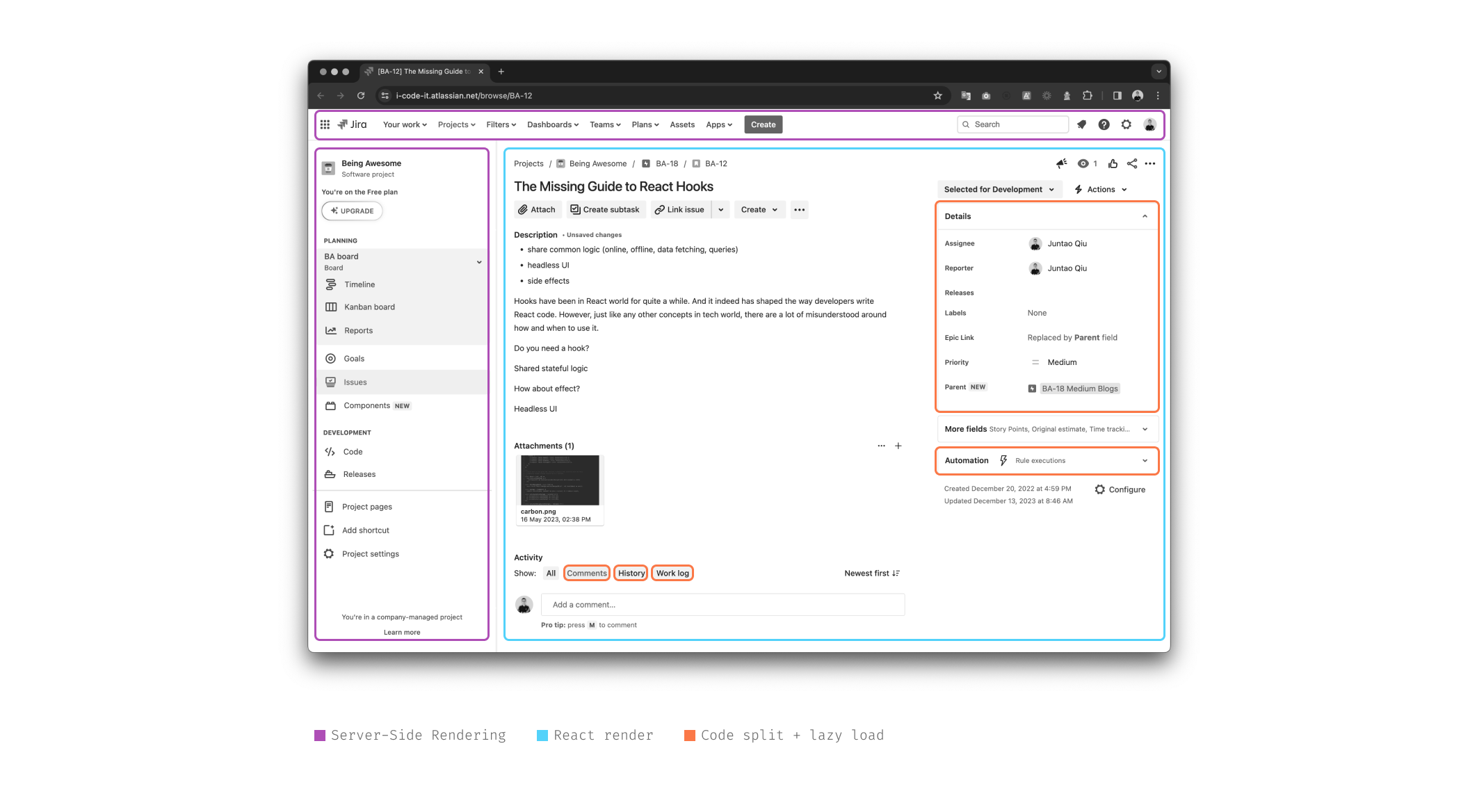
Determine 16: Utilizing patterns collectively
Furthermore, sure methods require extra setup in comparison with
default, much less optimized options. As an example, implementing Code Splitting requires bundler help. In case your present bundler lacks this
functionality, an improve could also be required, which may very well be impractical for
older, much less secure programs.
We have lined a variety of patterns and the way they apply to numerous
challenges. I notice there’s fairly a bit to absorb, from code examples
to diagrams. For those who’re in search of a extra guided strategy, I’ve put
collectively a complete tutorial on my
web site, or for those who solely need to take a look on the working code, they’re
all hosted on this github repo.
Conclusion
Information fetching is a nuanced side of growth, but mastering the
acceptable strategies can vastly improve our functions. As we conclude
our journey by means of knowledge fetching and content material rendering methods inside
the context of React, it is essential to focus on our primary insights:
- Asynchronous State Handler: Make the most of customized hooks or composable APIs to
summary knowledge fetching and state administration away out of your parts. This
sample centralizes asynchronous logic, simplifying part design and
enhancing reusability throughout your software. - Fallback Markup: React’s enhanced Suspense mannequin helps a extra
declarative strategy to fetching knowledge asynchronously, streamlining your
codebase. - Parallel Information Fetching: Maximize effectivity by fetching knowledge in
parallel, decreasing wait occasions and boosting the responsiveness of your
software. - Code Splitting: Make use of lazy loading for non-essential
parts in the course of the preliminary load, leveraging Suspense for sleek
dealing with of loading states and code splitting, thereby making certain your
software stays performant. - Prefetching: By preemptively loading knowledge primarily based on predicted consumer
actions, you may obtain a easy and quick consumer expertise.
Whereas these insights had been framed inside the React ecosystem, it is
important to acknowledge that these patterns are usually not confined to React
alone. They’re broadly relevant and useful methods that may—and
ought to—be tailored to be used with different libraries and frameworks. By
thoughtfully implementing these approaches, builders can create
functions that aren’t simply environment friendly and scalable, but additionally supply a
superior consumer expertise by means of efficient knowledge fetching and content material
rendering practices.