[ad_1]
Right this moment, most purposes can ship a whole bunch of requests for a single web page.
For instance, my Twitter residence web page sends round 300 requests, and an Amazon
product particulars web page sends round 600 requests. A few of them are for static
belongings (JavaScript, CSS, font information, icons, and so on.), however there are nonetheless
round 100 requests for async information fetching – both for timelines, mates,
or product suggestions, in addition to analytics occasions. That’s fairly a
lot.
The principle cause a web page might comprise so many requests is to enhance
efficiency and consumer expertise, particularly to make the applying really feel
sooner to the tip customers. The period of clean pages taking 5 seconds to load is
lengthy gone. In fashionable internet purposes, customers sometimes see a fundamental web page with
type and different parts in lower than a second, with further items
loading progressively.
Take the Amazon product element web page for example. The navigation and prime
bar seem virtually instantly, adopted by the product pictures, temporary, and
descriptions. Then, as you scroll, “Sponsored” content material, rankings,
suggestions, view histories, and extra seem.Typically, a consumer solely needs a
fast look or to check merchandise (and examine availability), making
sections like “Prospects who purchased this merchandise additionally purchased” much less important and
appropriate for loading through separate requests.
Breaking down the content material into smaller items and loading them in
parallel is an efficient technique, but it surely’s removed from sufficient in massive
purposes. There are a lot of different features to think about in relation to
fetch information accurately and effectively. Knowledge fetching is a chellenging, not
solely as a result of the character of async programming does not match our linear mindset,
and there are such a lot of components may cause a community name to fail, but additionally
there are too many not-obvious instances to think about below the hood (information
format, safety, cache, token expiry, and so on.).
On this article, I want to talk about some widespread issues and
patterns it’s best to contemplate in relation to fetching information in your frontend
purposes.
We’ll start with the Asynchronous State Handler sample, which decouples
information fetching from the UI, streamlining your utility structure. Subsequent,
we’ll delve into Fallback Markup, enhancing the intuitiveness of your information
fetching logic. To speed up the preliminary information loading course of, we’ll
discover methods for avoiding Request
Waterfall and implementing Parallel Knowledge Fetching. Our dialogue will then cowl Code Splitting to defer
loading non-critical utility elements and Prefetching information primarily based on consumer
interactions to raise the consumer expertise.
I imagine discussing these ideas by a simple instance is
the perfect strategy. I purpose to begin merely after which introduce extra complexity
in a manageable approach. I additionally plan to maintain code snippets, significantly for
styling (I am using TailwindCSS for the UI, which may end up in prolonged
snippets in a React part), to a minimal. For these within the
full particulars, I’ve made them accessible on this
repository.
Developments are additionally occurring on the server aspect, with methods like
Streaming Server-Aspect Rendering and Server Elements gaining traction in
varied frameworks. Moreover, numerous experimental strategies are
rising. Nonetheless, these subjects, whereas probably simply as essential, is likely to be
explored in a future article. For now, this dialogue will focus
solely on front-end information fetching patterns.
It is necessary to notice that the methods we’re protecting are usually not
unique to React or any particular frontend framework or library. I’ve
chosen React for illustration functions as a consequence of my intensive expertise with
it in recent times. Nonetheless, rules like Code Splitting,
Prefetching are
relevant throughout frameworks like Angular or Vue.js. The examples I will share
are widespread eventualities you may encounter in frontend growth, regardless
of the framework you employ.
That stated, let’s dive into the instance we’re going to make use of all through the
article, a Profile
display screen of a Single-Web page Software. It is a typical
utility you might need used earlier than, or at the least the situation is typical.
We have to fetch information from server aspect after which at frontend to construct the UI
dynamically with JavaScript.
Introducing the applying
To start with, on Profile
we’ll present the consumer’s temporary (together with
identify, avatar, and a brief description), after which we additionally wish to present
their connections (much like followers on Twitter or LinkedIn
connections). We’ll have to fetch consumer and their connections information from
distant service, after which assembling these information with UI on the display screen.
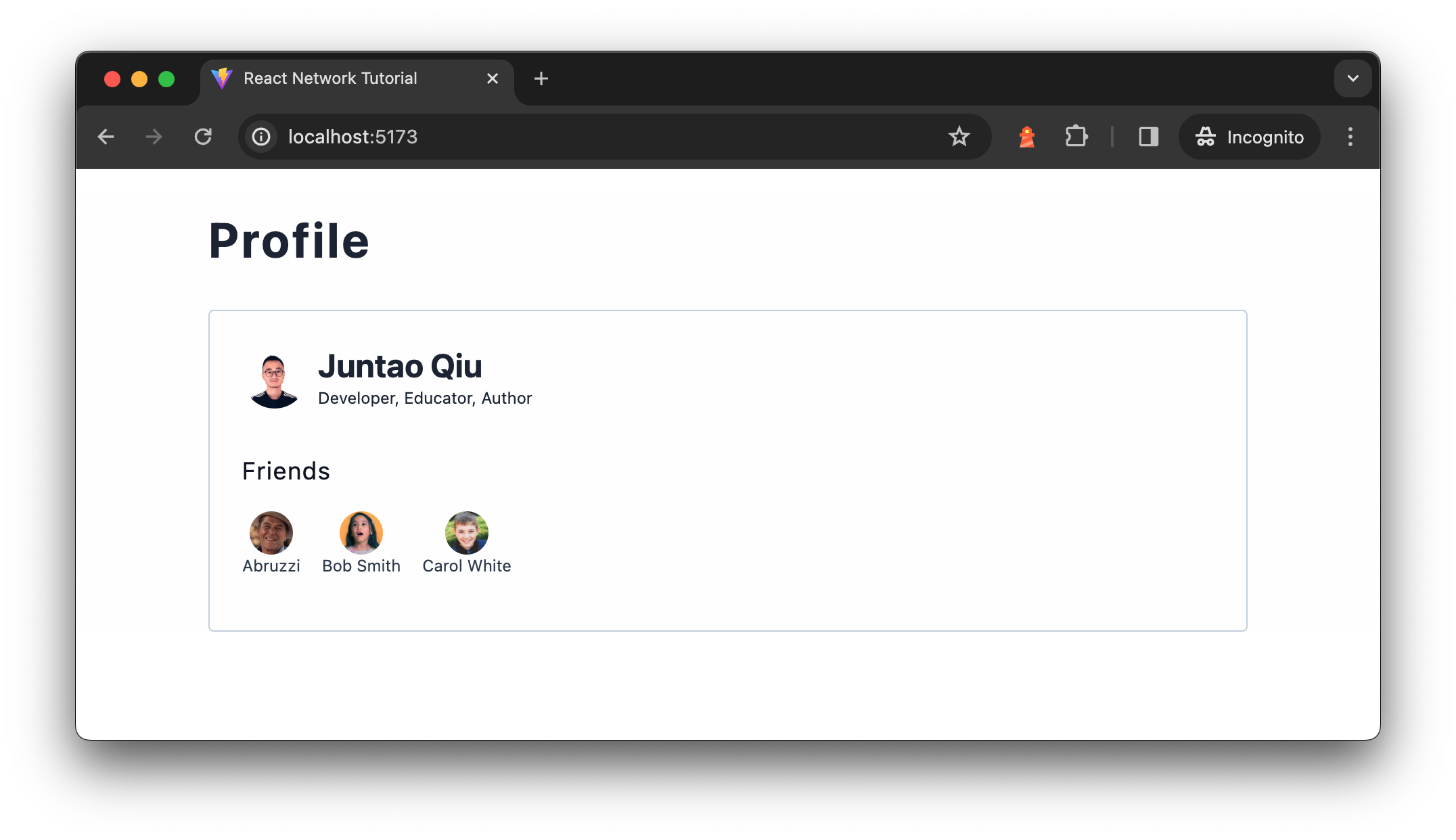
Determine 1: Profile display screen
The information are from two separate API calls, the consumer temporary API
/customers/<id>
returns consumer temporary for a given consumer id, which is an easy
object described as follows:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
And the buddy API /customers/<id>/mates
endpoint returns an inventory of
mates for a given consumer, every listing merchandise within the response is identical as
the above consumer information. The explanation we now have two endpoints as an alternative of returning
a mates
part of the consumer API is that there are instances the place one
might have too many mates (say 1,000), however most individuals do not have many.
This in-balance information construction will be fairly tough, particularly after we
have to paginate. The purpose right here is that there are instances we have to deal
with a number of community requests.
A quick introduction to related React ideas
As this text leverages React for instance varied patterns, I do
not assume you recognize a lot about React. Relatively than anticipating you to spend so much
of time looking for the correct elements within the React documentation, I’ll
briefly introduce these ideas we’ll make the most of all through this
article. If you happen to already perceive what React elements are, and the
use of the
useState
and useEffect
hooks, chances are you’ll
use this hyperlink to skip forward to the following
part.
For these in search of a extra thorough tutorial, the new React documentation is a superb
useful resource.
What’s a React Element?
In React, elements are the elemental constructing blocks. To place it
merely, a React part is a perform that returns a chunk of UI,
which will be as simple as a fraction of HTML. Contemplate the
creation of a part that renders a navigation bar:
import React from 'react'; perform Navigation() { return ( <nav> <ol> <li>House</li> <li>Blogs</li> <li>Books</li> </ol> </nav> ); }
At first look, the combination of JavaScript with HTML tags might sound
unusual (it is referred to as JSX, a syntax extension to JavaScript. For these
utilizing TypeScript, an analogous syntax referred to as TSX is used). To make this
code useful, a compiler is required to translate the JSX into legitimate
JavaScript code. After being compiled by Babel,
the code would roughly translate to the next:
perform Navigation() { return React.createElement( "nav", null, React.createElement( "ol", null, React.createElement("li", null, "House"), React.createElement("li", null, "Blogs"), React.createElement("li", null, "Books") ) ); }
Word right here the translated code has a perform referred to as
React.createElement
, which is a foundational perform in
React for creating parts. JSX written in React elements is compiled
right down to React.createElement
calls behind the scenes.
The essential syntax of React.createElement
is:
React.createElement(sort, [props], [...children])
sort
: A string (e.g., ‘div’, ‘span’) indicating the kind of
DOM node to create, or a React part (class or useful) for
extra subtle constructions.props
: An object containing properties handed to the
ingredient or part, together with occasion handlers, kinds, and attributes
likeclassName
andid
.kids
: These optionally available arguments will be further
React.createElement
calls, strings, numbers, or any combine
thereof, representing the ingredient’s kids.
For example, a easy ingredient will be created with
React.createElement
as follows:
React.createElement('div', { className: 'greeting' }, 'Hey, world!');
That is analogous to the JSX model:
<div className="greeting">Hey, world!</div>
Beneath the floor, React invokes the native DOM API (e.g.,
doc.createElement("ol")
) to generate DOM parts as needed.
You possibly can then assemble your customized elements right into a tree, much like
HTML code:
import React from 'react'; import Navigation from './Navigation.tsx'; import Content material from './Content material.tsx'; import Sidebar from './Sidebar.tsx'; import ProductList from './ProductList.tsx'; perform App() { return <Web page />; } perform Web page() { return <Container> <Navigation /> <Content material> <Sidebar /> <ProductList /> </Content material> <Footer /> </Container>; }
In the end, your utility requires a root node to mount to, at
which level React assumes management and manages subsequent renders and
re-renders:
import ReactDOM from "react-dom/consumer"; import App from "./App.tsx"; const root = ReactDOM.createRoot(doc.getElementById('root')); root.render(<App />);
Producing Dynamic Content material with JSX
The preliminary instance demonstrates a simple use case, however
let’s discover how we are able to create content material dynamically. For example, how
can we generate an inventory of information dynamically? In React, as illustrated
earlier, a part is basically a perform, enabling us to move
parameters to it.
import React from 'react'; perform Navigation({ nav }) { return ( <nav> <ol> {nav.map(merchandise => <li key={merchandise}>{merchandise}</li>)} </ol> </nav> ); }
On this modified Navigation
part, we anticipate the
parameter to be an array of strings. We make the most of the map
perform to iterate over every merchandise, remodeling them into
<li>
parts. The curly braces {}
signify
that the enclosed JavaScript expression must be evaluated and
rendered. For these curious concerning the compiled model of this dynamic
content material dealing with:
perform Navigation(props) { var nav = props.nav; return React.createElement( "nav", null, React.createElement( "ol", null, nav.map(perform(merchandise) { return React.createElement("li", { key: merchandise }, merchandise); }) ) ); }
As a substitute of invoking Navigation
as an everyday perform,
using JSX syntax renders the part invocation extra akin to
writing markup, enhancing readability:
// As a substitute of this Navigation(["Home", "Blogs", "Books"]) // We do that <Navigation nav={["Home", "Blogs", "Books"]} />
Elements in React can obtain numerous information, often known as props, to
modify their conduct, very similar to passing arguments right into a perform (the
distinction lies in utilizing JSX syntax, making the code extra acquainted and
readable to these with HTML data, which aligns effectively with the ability
set of most frontend builders).
import React from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App() { let showNewOnly = false; // This flag's worth is often set primarily based on particular logic. const filteredBooks = showNewOnly ? booksData.filter(ebook => ebook.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks} /> </div> ); }
On this illustrative code snippet (non-functional however supposed to
display the idea), we manipulate the BookList
part’s displayed content material by passing it an array of books. Relying
on the showNewOnly
flag, this array is both all accessible
books or solely these which can be newly revealed, showcasing how props can
be used to dynamically alter part output.
Managing Inside State Between Renders: useState
Constructing consumer interfaces (UI) typically transcends the era of
static HTML. Elements often have to “bear in mind” sure states and
reply to consumer interactions dynamically. For example, when a consumer
clicks an “Add” button in a Product part, it’s a necessity to replace
the ShoppingCart part to replicate each the full worth and the
up to date merchandise listing.
Within the earlier code snippet, making an attempt to set the
showNewOnly
variable to true
inside an occasion
handler doesn’t obtain the specified impact:
perform App () { let showNewOnly = false; const handleCheckboxChange = () => { showNewOnly = true; // this does not work }; const filteredBooks = showNewOnly ? booksData.filter(ebook => ebook.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
This strategy falls brief as a result of native variables inside a perform
part don’t persist between renders. When React re-renders this
part, it does so from scratch, disregarding any adjustments made to
native variables since these don’t set off re-renders. React stays
unaware of the necessity to replace the part to replicate new information.
This limitation underscores the need for React’s
state
. Particularly, useful elements leverage the
useState
hook to recollect states throughout renders. Revisiting
the App
instance, we are able to successfully bear in mind the
showNewOnly
state as follows:
import React, { useState } from 'react'; import Checkbox from './Checkbox'; import BookList from './BookList'; perform App () { const [showNewOnly, setShowNewOnly] = useState(false); const handleCheckboxChange = () => { setShowNewOnly(!showNewOnly); }; const filteredBooks = showNewOnly ? booksData.filter(ebook => ebook.isNewPublished) : booksData; return ( <div> <Checkbox checked={showNewOnly} onChange={handleCheckboxChange}> Present New Revealed Books Solely </Checkbox> <BookList books={filteredBooks}/> </div> ); };
The useState
hook is a cornerstone of React’s Hooks system,
launched to allow useful elements to handle inner state. It
introduces state to useful elements, encapsulated by the next
syntax:
const [state, setState] = useState(initialState);
initialState
: This argument is the preliminary
worth of the state variable. It may be a easy worth like a quantity,
string, boolean, or a extra advanced object or array. The
initialState
is just used in the course of the first render to
initialize the state.- Return Worth:
useState
returns an array with
two parts. The primary ingredient is the present state worth, and the
second ingredient is a perform that enables updating this worth. Through the use of
array destructuring, we assign names to those returned objects,
sometimesstate
andsetState
, although you’ll be able to
select any legitimate variable names. state
: Represents the present worth of the
state. It is the worth that shall be used within the part’s UI and
logic.setState
: A perform to replace the state. This perform
accepts a brand new state worth or a perform that produces a brand new state primarily based
on the earlier state. When referred to as, it schedules an replace to the
part’s state and triggers a re-render to replicate the adjustments.
React treats state as a snapshot; updating it does not alter the
present state variable however as an alternative triggers a re-render. Throughout this
re-render, React acknowledges the up to date state, guaranteeing the
BookList
part receives the proper information, thereby
reflecting the up to date ebook listing to the consumer. This snapshot-like
conduct of state facilitates the dynamic and responsive nature of React
elements, enabling them to react intuitively to consumer interactions and
different adjustments.
Managing Aspect Results: useEffect
Earlier than diving deeper into our dialogue, it is essential to deal with the
idea of unwanted effects. Unwanted effects are operations that work together with
the surface world from the React ecosystem. Frequent examples embrace
fetching information from a distant server or dynamically manipulating the DOM,
similar to altering the web page title.
React is primarily involved with rendering information to the DOM and does
not inherently deal with information fetching or direct DOM manipulation. To
facilitate these unwanted effects, React supplies the useEffect
hook. This hook permits the execution of unwanted effects after React has
accomplished its rendering course of. If these unwanted effects end in information
adjustments, React schedules a re-render to replicate these updates.
The useEffect
Hook accepts two arguments:
- A perform containing the aspect impact logic.
- An optionally available dependency array specifying when the aspect impact must be
re-invoked.
Omitting the second argument causes the aspect impact to run after
each render. Offering an empty array []
signifies that your impact
doesn’t depend upon any values from props or state, thus not needing to
re-run. Together with particular values within the array means the aspect impact
solely re-executes if these values change.
When coping with asynchronous information fetching, the workflow inside
useEffect
entails initiating a community request. As soon as the information is
retrieved, it’s captured through the useState
hook, updating the
part’s inner state and preserving the fetched information throughout
renders. React, recognizing the state replace, undertakes one other render
cycle to include the brand new information.
Here is a sensible instance about information fetching and state
administration:
import { useEffect, useState } from "react"; sort Person = { id: string; identify: string; }; const UserSection = ({ id }) => { const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return <div> <h2>{consumer?.identify}</h2> </div>; };
Within the code snippet above, inside useEffect
, an
asynchronous perform fetchUser
is outlined after which
instantly invoked. This sample is critical as a result of
useEffect
doesn’t immediately assist async capabilities as its
callback. The async perform is outlined to make use of await
for
the fetch operation, guaranteeing that the code execution waits for the
response after which processes the JSON information. As soon as the information is on the market,
it updates the part’s state through setUser
.
The dependency array tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data
on the finish of the
useEffect
name ensures that the impact runs once more provided that
id
adjustments, which prevents pointless community requests on
each render and fetches new consumer information when the id
prop
updates.
This strategy to dealing with asynchronous information fetching inside
useEffect
is an ordinary follow in React growth, providing a
structured and environment friendly option to combine async operations into the
React part lifecycle.
As well as, in sensible purposes, managing completely different states
similar to loading, error, and information presentation is important too (we’ll
see it the way it works within the following part). For instance, contemplate
implementing standing indicators inside a Person part to replicate
loading, error, or information states, enhancing the consumer expertise by
offering suggestions throughout information fetching operations.
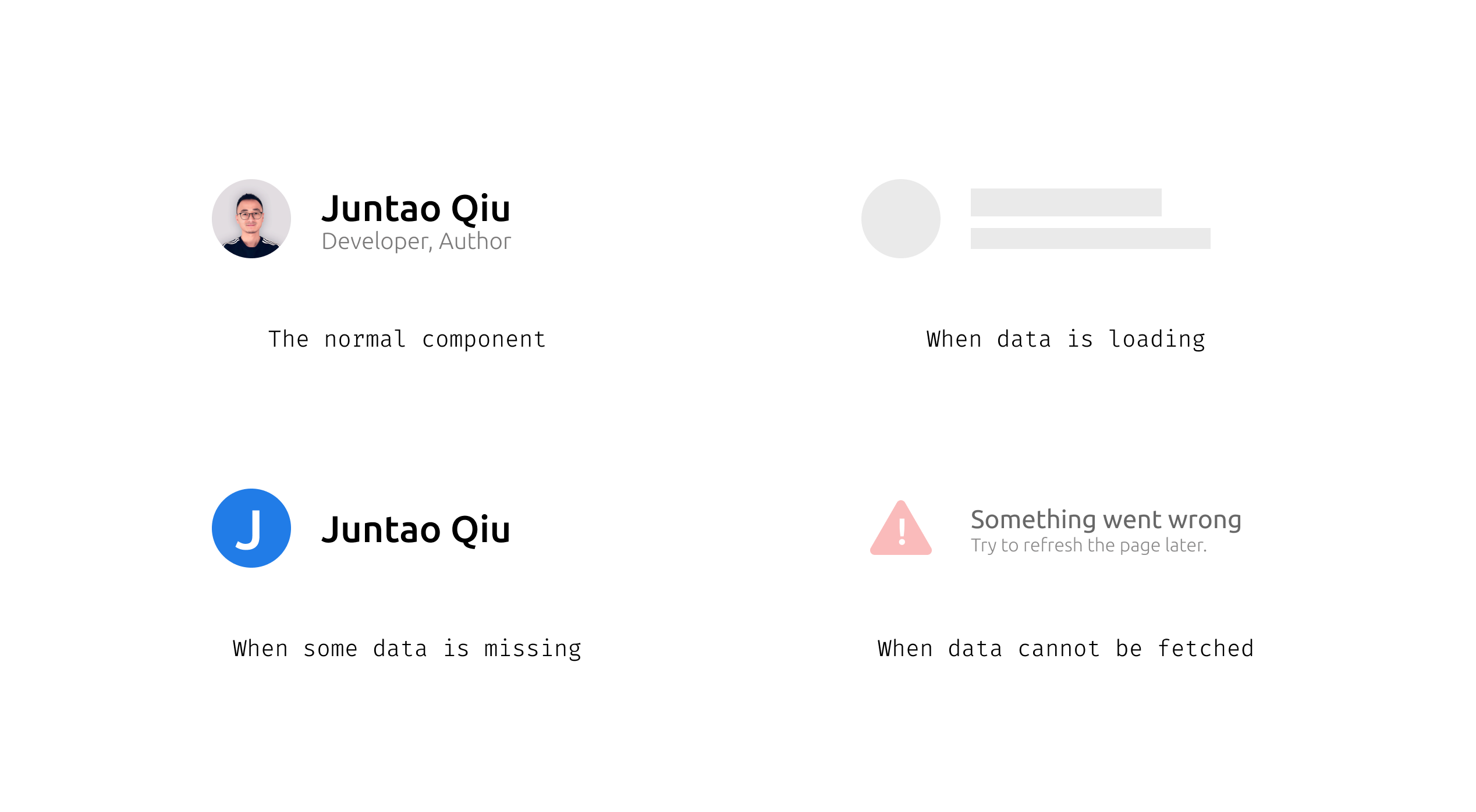
Determine 2: Completely different statuses of a
part
This overview presents only a fast glimpse into the ideas utilized
all through this text. For a deeper dive into further ideas and
patterns, I like to recommend exploring the new React
documentation or consulting different on-line sources.
With this basis, it’s best to now be geared up to hitch me as we delve
into the information fetching patterns mentioned herein.
Implement the Profile part
Let’s create the Profile
part to make a request and
render the consequence. In typical React purposes, this information fetching is
dealt with inside a useEffect
block. Here is an instance of how
this is likely to be carried out:
import { useEffect, useState } from "react"; const Profile = ({ id }: { id: string }) => { const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { const response = await fetch(`/api/customers/${id}`); const jsonData = await response.json(); setUser(jsonData); }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return ( <UserBrief consumer={consumer} /> ); };
This preliminary strategy assumes community requests full
instantaneously, which is commonly not the case. Actual-world eventualities require
dealing with various community situations, together with delays and failures. To
handle these successfully, we incorporate loading and error states into our
part. This addition permits us to offer suggestions to the consumer throughout
information fetching, similar to displaying a loading indicator or a skeleton display screen
if the information is delayed, and dealing with errors once they happen.
Right here’s how the improved part seems to be with added loading and error
administration:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; import sort { Person } from "../varieties.ts"; const Profile = ({ id }: { id: string }) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const information = await get<Person>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); if (loading || !consumer) { return <div>Loading...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Now in Profile
part, we provoke states for loading,
errors, and consumer information with useState
. Utilizing
useEffect
, we fetch consumer information primarily based on id
,
toggling loading standing and dealing with errors accordingly. Upon profitable
information retrieval, we replace the consumer state, else show a loading
indicator.
The get
perform, as demonstrated beneath, simplifies
fetching information from a selected endpoint by appending the endpoint to a
predefined base URL. It checks the response’s success standing and both
returns the parsed JSON information or throws an error for unsuccessful requests,
streamlining error dealing with and information retrieval in our utility. Word
it is pure TypeScript code and can be utilized in different non-React elements of the
utility.
const baseurl = "https://icodeit.com.au/api/v2"; async perform get<T>(url: string): Promise<T> { const response = await fetch(`${baseurl}${url}`); if (!response.okay) { throw new Error("Community response was not okay"); } return await response.json() as Promise<T>; }
React will attempt to render the part initially, however as the information
consumer
isn’t accessible, it returns “loading…” in a
div
. Then the useEffect
is invoked, and the
request is kicked off. As soon as sooner or later, the response returns, React
re-renders the Profile
part with consumer
fulfilled, so now you can see the consumer part with identify, avatar, and
title.
If we visualize the timeline of the above code, you will notice
the next sequence. The browser firstly downloads the HTML web page, and
then when it encounters script tags and magnificence tags, it would cease and
obtain these information, after which parse them to kind the ultimate web page. Word
that this can be a comparatively difficult course of, and I’m oversimplifying
right here, however the fundamental concept of the sequence is right.
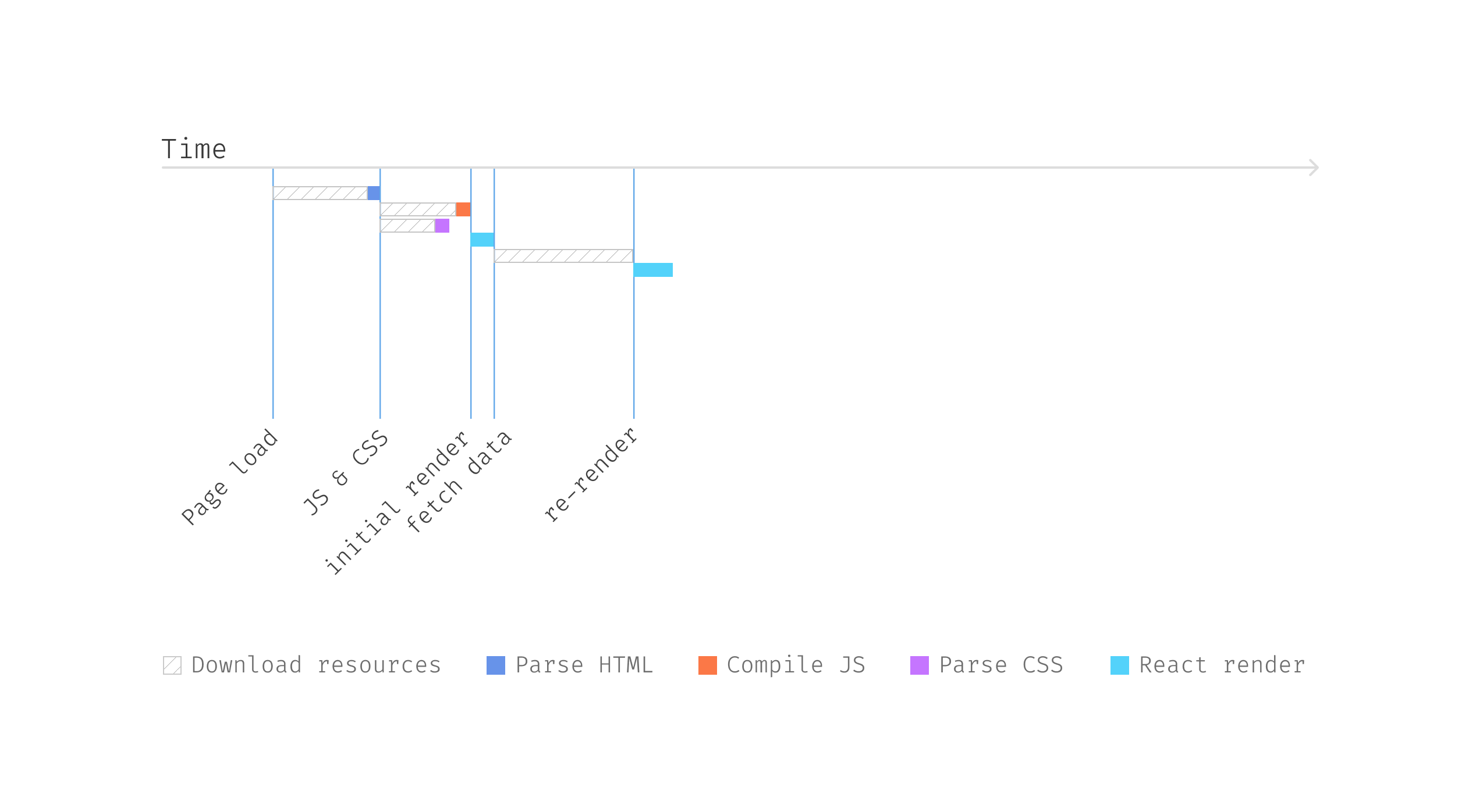
Determine 3: Fetching consumer
information
So React can begin to render solely when the JS are parsed and executed,
after which it finds the useEffect
for information fetching; it has to attend till
the information is on the market for a re-render.
Now within the browser, we are able to see a “loading…” when the applying
begins, after which after a number of seconds (we are able to simulate such case by add
some delay within the API endpoints) the consumer temporary part reveals up when information
is loaded.
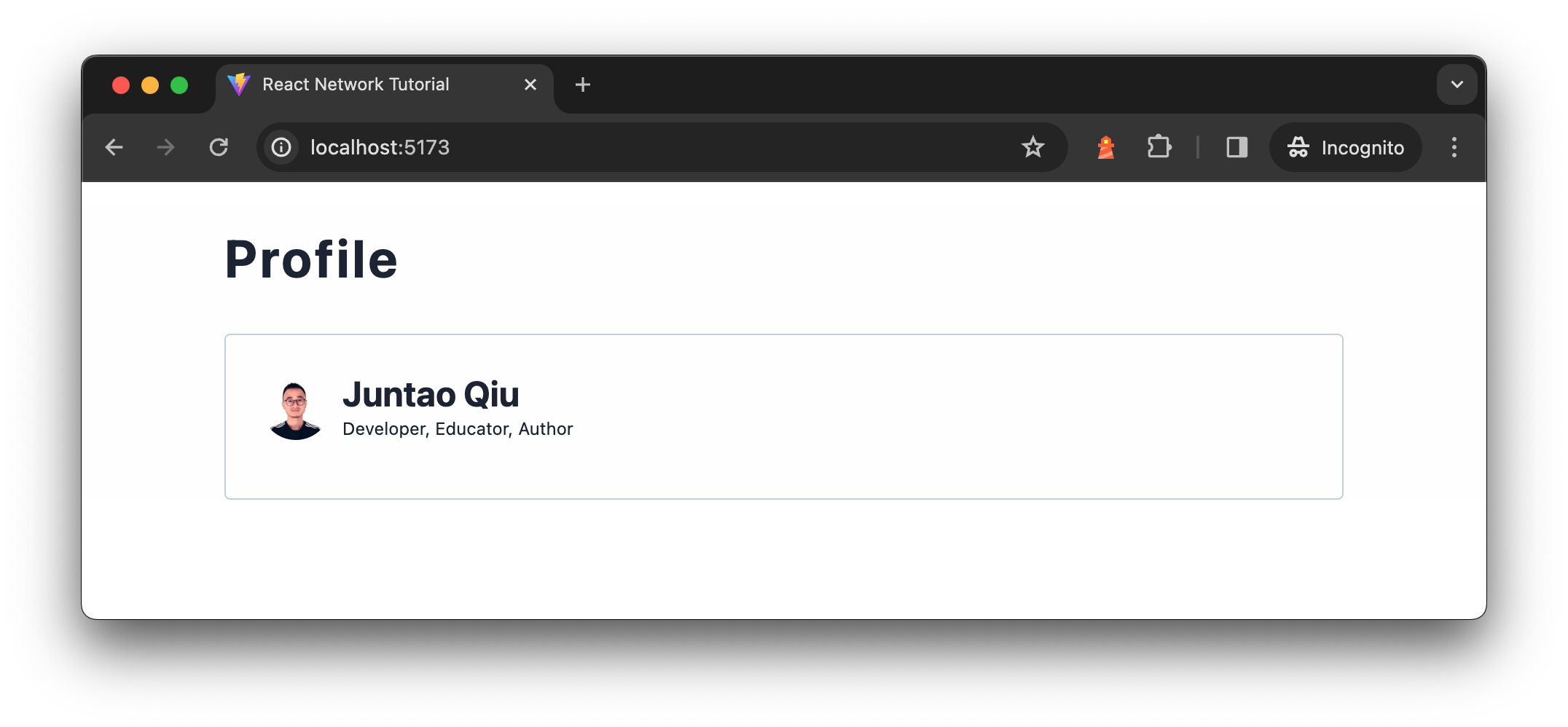
Determine 4: Person temporary part
This code construction (in useEffect to set off request, and replace states
like loading
and error
correspondingly) is
extensively used throughout React codebases. In purposes of normal dimension, it is
widespread to seek out quite a few cases of such identical data-fetching logic
dispersed all through varied elements.
Asynchronous State Handler
Wrap asynchronous queries with meta-queries for the state of the
question.
Distant calls will be sluggish, and it is important to not let the UI freeze
whereas these calls are being made. Due to this fact, we deal with them asynchronously
and use indicators to point out {that a} course of is underway, which makes the
consumer expertise higher – figuring out that one thing is occurring.
Moreover, distant calls may fail as a consequence of connection points,
requiring clear communication of those failures to the consumer. Due to this fact,
it is best to encapsulate every distant name inside a handler module that
manages outcomes, progress updates, and errors. This module permits the UI
to entry metadata concerning the standing of the decision, enabling it to show
different data or choices if the anticipated outcomes fail to
materialize.
A easy implementation could possibly be a perform getAsyncStates
that
returns these metadata, it takes a URL as its parameter and returns an
object containing data important for managing asynchronous
operations. This setup permits us to appropriately reply to completely different
states of a community request, whether or not it is in progress, efficiently
resolved, or has encountered an error.
const { loading, error, information } = getAsyncStates(url); if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
The idea right here is that getAsyncStates
initiates the
community request routinely upon being referred to as. Nonetheless, this may not
all the time align with the caller’s wants. To supply extra management, we are able to additionally
expose a fetch
perform throughout the returned object, permitting
the initiation of the request at a extra applicable time, in accordance with the
caller’s discretion. Moreover, a refetch
perform might
be supplied to allow the caller to re-initiate the request as wanted,
similar to after an error or when up to date information is required. The
fetch
and refetch
capabilities will be an identical in
implementation, or refetch
may embrace logic to examine for
cached outcomes and solely re-fetch information if needed.
const { loading, error, information, fetch, refetch } = getAsyncStates(url); const onInit = () => { fetch(); }; const onRefreshClicked = () => { refetch(); }; if (loading) { // Show a loading spinner } if (error) { // Show an error message } // Proceed to render utilizing the information
This sample supplies a flexible strategy to dealing with asynchronous
requests, giving builders the pliability to set off information fetching
explicitly and handle the UI’s response to loading, error, and success
states successfully. By decoupling the fetching logic from its initiation,
purposes can adapt extra dynamically to consumer interactions and different
runtime situations, enhancing the consumer expertise and utility
reliability.
Implementing Asynchronous State Handler in React with hooks
The sample will be carried out in several frontend libraries. For
occasion, we might distill this strategy right into a customized Hook in a React
utility for the Profile part:
import { useEffect, useState } from "react"; import { get } from "../utils.ts"; const useUser = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [user, setUser] = useState<Person | undefined>(); useEffect(() => { const fetchUser = async () => { attempt { setLoading(true); const information = await get<Person>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; fetchUser(); }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return { loading, error, consumer, }; };
Please be aware that within the customized Hook, we haven’t any JSX code –
that means it’s very UI free however sharable stateful logic. And the
useUser
launch information routinely when referred to as. Inside the Profile
part, leveraging the useUser
Hook simplifies its logic:
import { useUser } from './useUser.ts'; import UserBrief from './UserBrief.tsx'; const Profile = ({ id }: { id: string }) => { const { loading, error, consumer } = useUser(id); if (loading || !consumer) { return <div>Loading...</div>; } if (error) { return <div>One thing went fallacious...</div>; } return ( <> {consumer && <UserBrief consumer={consumer} />} </> ); };
Generalizing Parameter Utilization
In most purposes, fetching several types of information—from consumer
particulars on a homepage to product lists in search outcomes and
suggestions beneath them—is a standard requirement. Writing separate
fetch capabilities for every sort of information will be tedious and troublesome to
keep. A greater strategy is to summary this performance right into a
generic, reusable hook that may deal with varied information varieties
effectively.
Contemplate treating distant API endpoints as providers, and use a generic
useService
hook that accepts a URL as a parameter whereas managing all
the metadata related to an asynchronous request:
import { get } from "../utils.ts"; perform useService<T>(url: string) { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(); const [data, setData] = useState<T | undefined>(); const fetch = async () => { attempt { setLoading(true); const information = await get<T>(url); setData(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, information, fetch, }; }
This hook abstracts the information fetching course of, making it simpler to
combine into any part that should retrieve information from a distant
supply. It additionally centralizes widespread error dealing with eventualities, similar to
treating particular errors in a different way:
import { useService } from './useService.ts'; const { loading, error, information: consumer, fetch: fetchUser, } = useService(`/customers/${id}`);
Through the use of useService, we are able to simplify how elements fetch and deal with
information, making the codebase cleaner and extra maintainable.
Variation of the sample
A variation of the useUser
could be expose the
fetchUsers
perform, and it doesn’t set off the information
fetching itself:
import { useState } from "react"; const useUser = (id: string) => { // outline the states const fetchUser = async () => { attempt { setLoading(true); const information = await get<Person>(`/customers/${id}`); setUser(information); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }; return { loading, error, consumer, fetchUser, }; };
After which on the calling website, Profile
part use
useEffect
to fetch the information and render completely different
states.
const Profile = ({ id }: { id: string }) => { const { loading, error, consumer, fetchUser } = useUser(id); useEffect(() => { fetchUser(); }, []); // render correspondingly };
The benefit of this division is the flexibility to reuse these stateful
logics throughout completely different elements. For example, one other part
needing the identical information (a consumer API name with a consumer ID) can merely import
the useUser
Hook and make the most of its states. Completely different UI
elements may select to work together with these states in varied methods,
maybe utilizing different loading indicators (a smaller spinner that
suits to the calling part) or error messages, but the elemental
logic of fetching information stays constant and shared.
When to make use of it
Separating information fetching logic from UI elements can generally
introduce pointless complexity, significantly in smaller purposes.
Protecting this logic built-in throughout the part, much like the
css-in-js strategy, simplifies navigation and is less complicated for some
builders to handle. In my article, Modularizing
React Functions with Established UI Patterns, I explored
varied ranges of complexity in utility constructions. For purposes
which can be restricted in scope — with just some pages and a number of other information
fetching operations — it is typically sensible and likewise beneficial to
keep information fetching inside the UI elements.
Nonetheless, as your utility scales and the event staff grows,
this technique might result in inefficiencies. Deep part bushes can sluggish
down your utility (we’ll see examples in addition to easy methods to handle
them within the following sections) and generate redundant boilerplate code.
Introducing an Asynchronous State Handler can mitigate these points by
decoupling information fetching from UI rendering, enhancing each efficiency
and maintainability.
It’s essential to steadiness simplicity with structured approaches as your
mission evolves. This ensures your growth practices stay
efficient and conscious of the applying’s wants, sustaining optimum
efficiency and developer effectivity whatever the mission
scale.
Implement the Pals listing
Now let’s take a look on the second part of the Profile – the buddy
listing. We are able to create a separate part Pals
and fetch information in it
(through the use of a useService customized hook we outlined above), and the logic is
fairly much like what we see above within the Profile
part.
const Pals = ({ id }: { id: string }) => { const { loading, error, information: mates } = useService(`/customers/${id}/mates`); // loading & error dealing with... return ( <div> <h2>Pals</h2> <div> {mates.map((consumer) => ( // render consumer listing ))} </div> </div> ); };
After which within the Profile part, we are able to use Pals as an everyday
part, and move in id
as a prop:
const Profile = ({ id }: { id: string }) => { //... return ( <> {consumer && <UserBrief consumer={consumer} />} <Pals id={id} /> </> ); };
The code works superb, and it seems to be fairly clear and readable,
UserBrief
renders a consumer
object handed in, whereas
Pals
handle its personal information fetching and rendering logic
altogether. If we visualize the part tree, it might be one thing like
this:
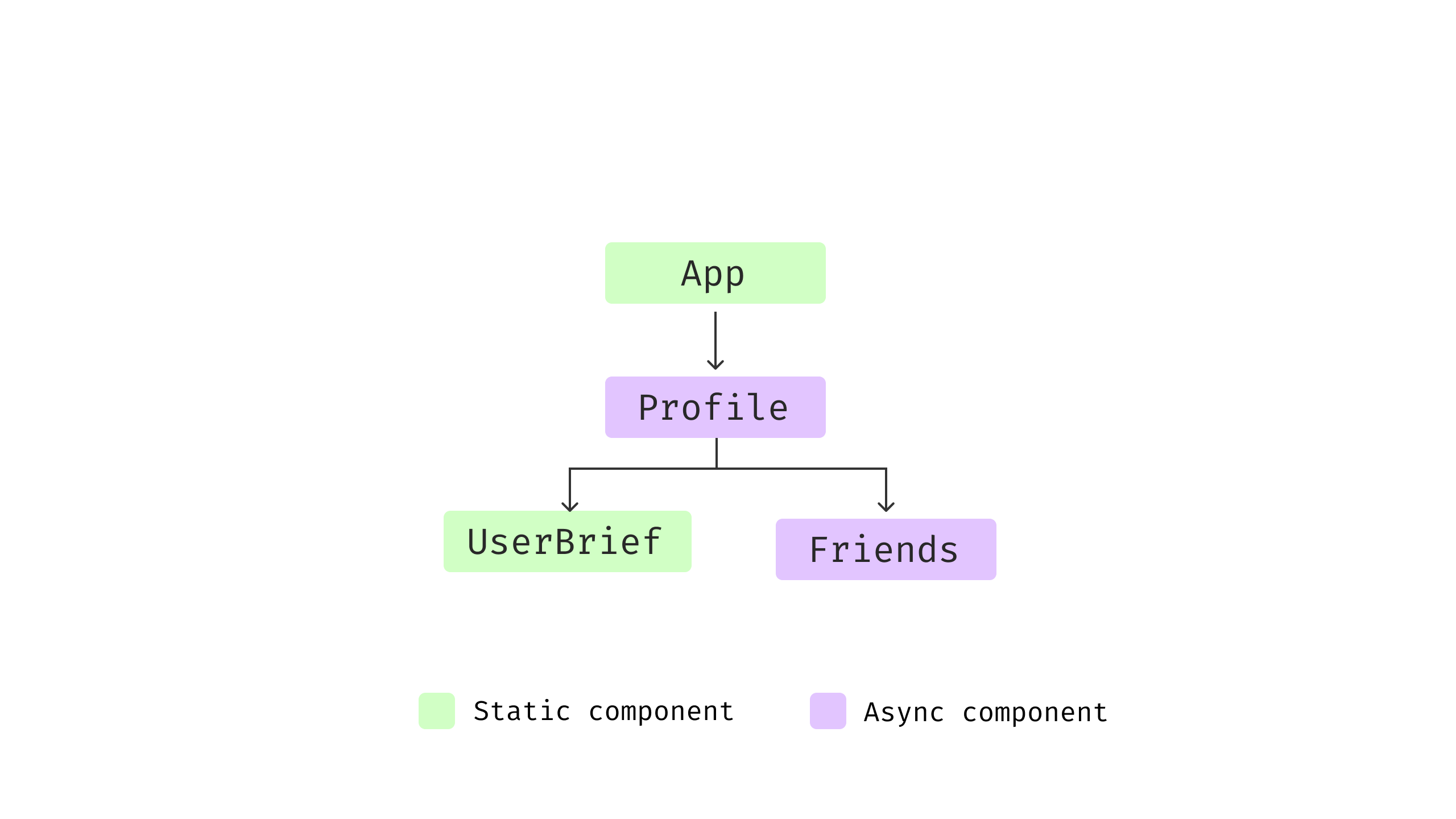
Determine 5: Element construction
Each the Profile
and Pals
have logic for
information fetching, loading checks, and error dealing with. Since there are two
separate information fetching calls, and if we have a look at the request timeline, we
will discover one thing attention-grabbing.
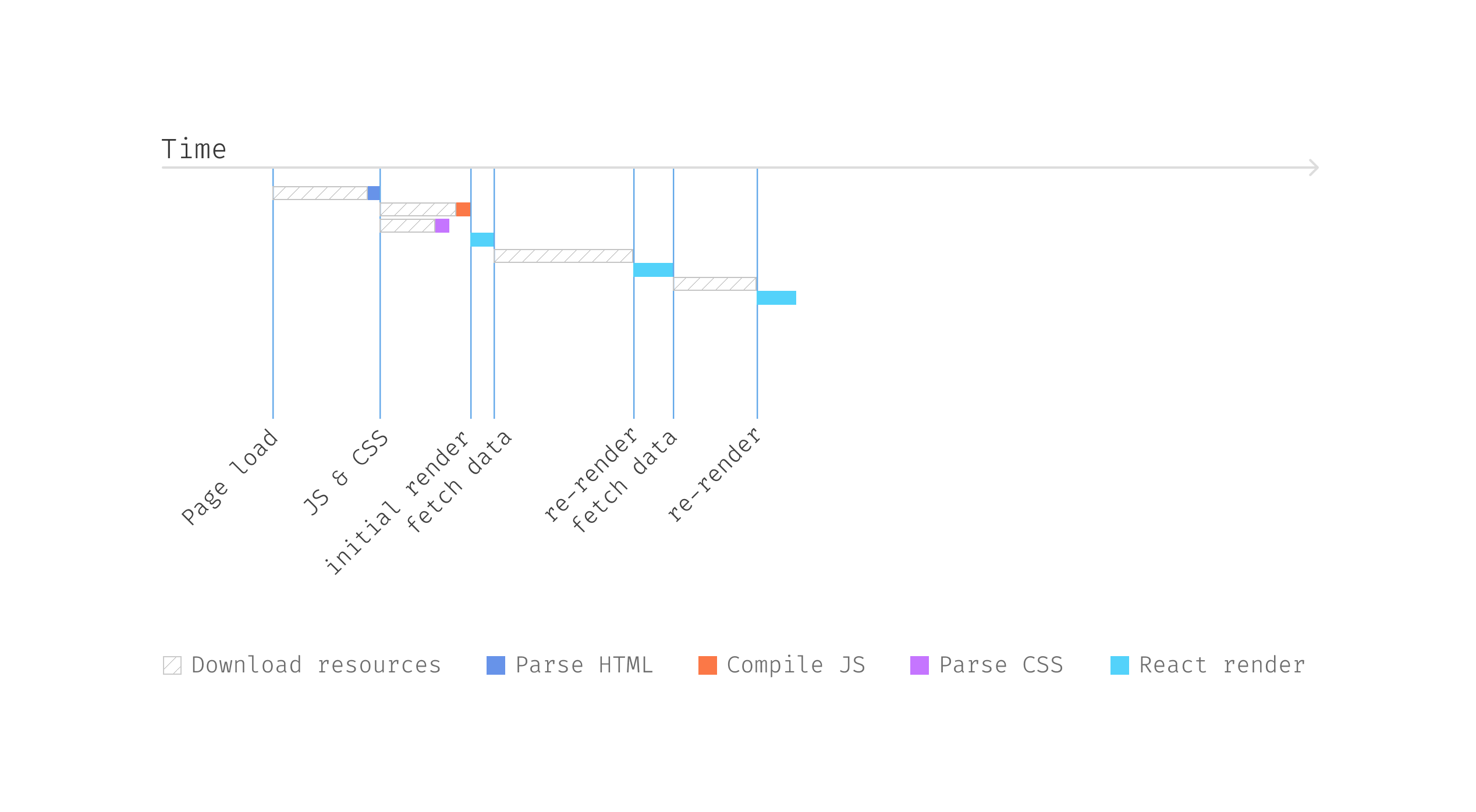
Determine 6: Request waterfall
The Pals
part will not provoke information fetching till the consumer
state is ready. That is known as the Fetch-On-Render strategy,
the place the preliminary rendering is paused as a result of the information is not accessible,
requiring React to attend for the information to be retrieved from the server
aspect.
This ready interval is considerably inefficient, contemplating that whereas
React’s rendering course of solely takes a number of milliseconds, information fetching can
take considerably longer, typically seconds. Because of this, the Pals
part spends most of its time idle, ready for information. This situation
results in a standard problem often known as the Request Waterfall, a frequent
prevalence in frontend purposes that contain a number of information fetching
operations.
Parallel Knowledge Fetching
Run distant information fetches in parallel to attenuate wait time
Think about after we construct a bigger utility {that a} part that
requires information will be deeply nested within the part tree, to make the
matter worse these elements are developed by completely different groups, it’s onerous
to see whom we’re blocking.
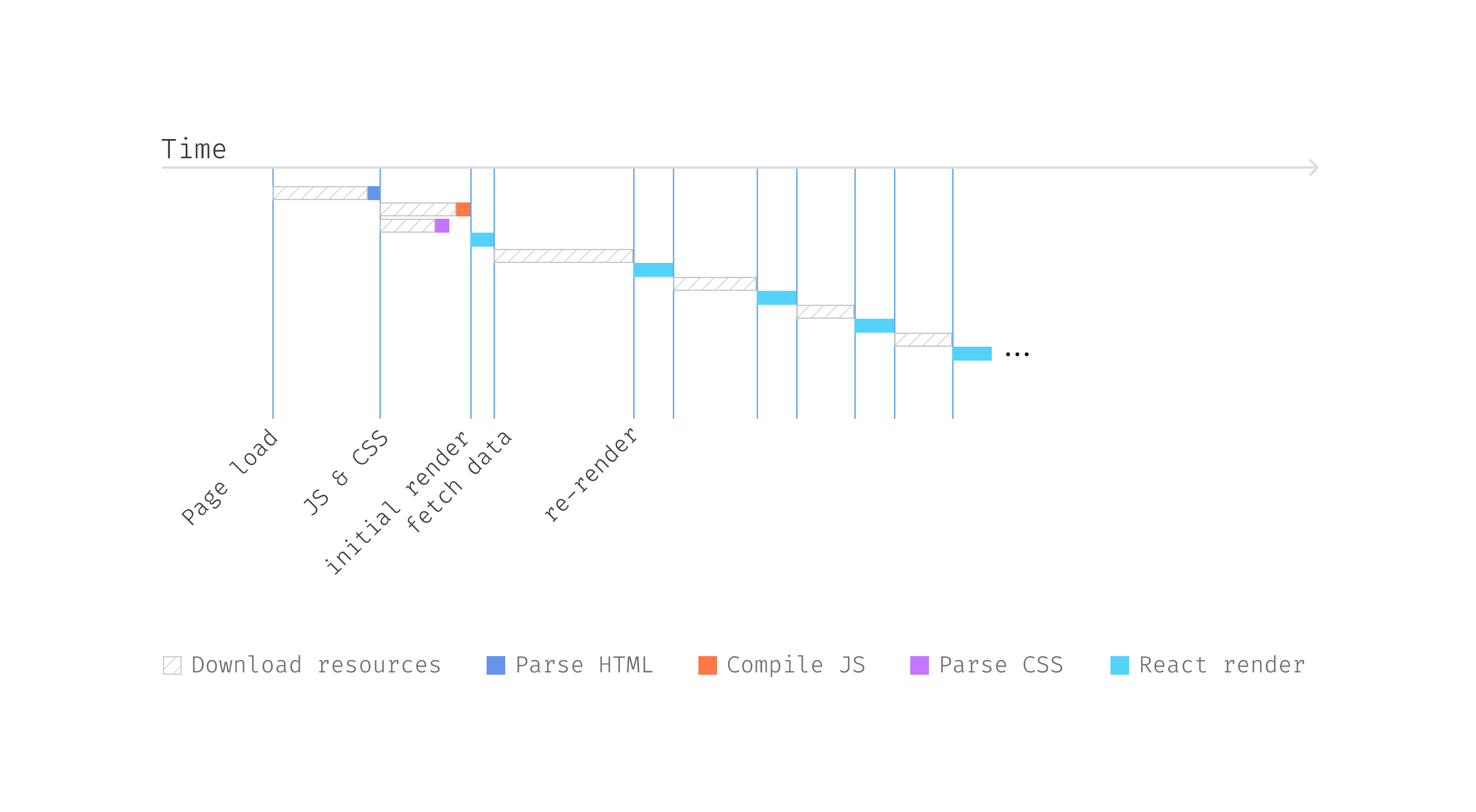
Determine 7: Request waterfall
Request Waterfalls can degrade consumer
expertise, one thing we purpose to keep away from. Analyzing the information, we see that the
consumer API and mates API are impartial and will be fetched in parallel.
Initiating these parallel requests turns into important for utility
efficiency.
One strategy is to centralize information fetching at the next stage, close to the
root. Early within the utility’s lifecycle, we begin all information fetches
concurrently. Elements depending on this information wait just for the
slowest request, sometimes leading to sooner general load instances.
We might use the Promise API Promise.all
to ship
each requests for the consumer’s fundamental data and their mates listing.
Promise.all
is a JavaScript technique that enables for the
concurrent execution of a number of guarantees. It takes an array of guarantees
as enter and returns a single Promise that resolves when the entire enter
guarantees have resolved, offering their outcomes as an array. If any of the
guarantees fail, Promise.all
instantly rejects with the
cause of the primary promise that rejects.
For example, on the utility’s root, we are able to outline a complete
information mannequin:
sort ProfileState = { consumer: Person; mates: Person[]; }; const getProfileData = async (id: string) => Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/mates`), ]); const App = () => { // fetch information on the very begining of the applying launch const onInit = () => { const [user, friends] = await getProfileData(id); } // render the sub tree correspondingly }
Implementing Parallel Knowledge Fetching in React
Upon utility launch, information fetching begins, abstracting the
fetching course of from subcomponents. For instance, in Profile part,
each UserBrief and Pals are presentational elements that react to
the handed information. This fashion we might develop these part individually
(including kinds for various states, for instance). These presentational
elements usually are straightforward to check and modify as we now have separate the
information fetching and rendering.
We are able to outline a customized hook useProfileData
that facilitates
parallel fetching of information associated to a consumer and their mates through the use of
Promise.all
. This technique permits simultaneous requests, optimizing the
loading course of and structuring the information right into a predefined format identified
as ProfileData
.
Right here’s a breakdown of the hook implementation:
import { useCallback, useEffect, useState } from "react"; sort ProfileData = { consumer: Person; mates: Person[]; }; const useProfileData = (id: string) => { const [loading, setLoading] = useState<boolean>(false); const [error, setError] = useState<Error | undefined>(undefined); const [profileState, setProfileState] = useState<ProfileData>(); const fetchProfileState = useCallback(async () => { attempt { setLoading(true); const [user, friends] = await Promise.all([ get<User>(`/users/${id}`), get<User[]>(`/customers/${id}/mates`), ]); setProfileState({ consumer, mates }); } catch (e) { setError(e as Error); } lastly { setLoading(false); } }, tag:martinfowler.com,2024-05-21:Utilizing-markup-for-fallbacks-when-fetching-data); return { loading, error, profileState, fetchProfileState, }; };
This hook supplies the Profile
part with the
needed information states (loading
, error
,
profileState
) together with a fetchProfileState
perform, enabling the part to provoke the fetch operation as
wanted. Word right here we use useCallback
hook to wrap the async
perform for information fetching. The useCallback hook in React is used to
memoize capabilities, guaranteeing that the identical perform occasion is
maintained throughout part re-renders except its dependencies change.
Much like the useEffect, it accepts the perform and a dependency
array, the perform will solely be recreated if any of those dependencies
change, thereby avoiding unintended conduct in React’s rendering
cycle.
The Profile
part makes use of this hook and controls the information fetching
timing through useEffect
:
const Profile = ({ id }: { id: string }) => { const { loading, error, profileState, fetchProfileState } = useProfileData(id); useEffect(() => { fetchProfileState(); }, [fetchProfileState]); if (loading) { return <div>Loading...</div>; } if (error) { return <div>One thing went fallacious...</div>; } return ( <> {profileState && ( <> <UserBrief consumer={profileState.consumer} /> <Pals customers={profileState.mates} /> </> )} </> ); };
This strategy is often known as Fetch-Then-Render, suggesting that the purpose
is to provoke requests as early as doable throughout web page load.
Subsequently, the fetched information is utilized to drive React’s rendering of
the applying, bypassing the necessity to handle information fetching amidst the
rendering course of. This technique simplifies the rendering course of,
making the code simpler to check and modify.
And the part construction, if visualized, could be just like the
following illustration
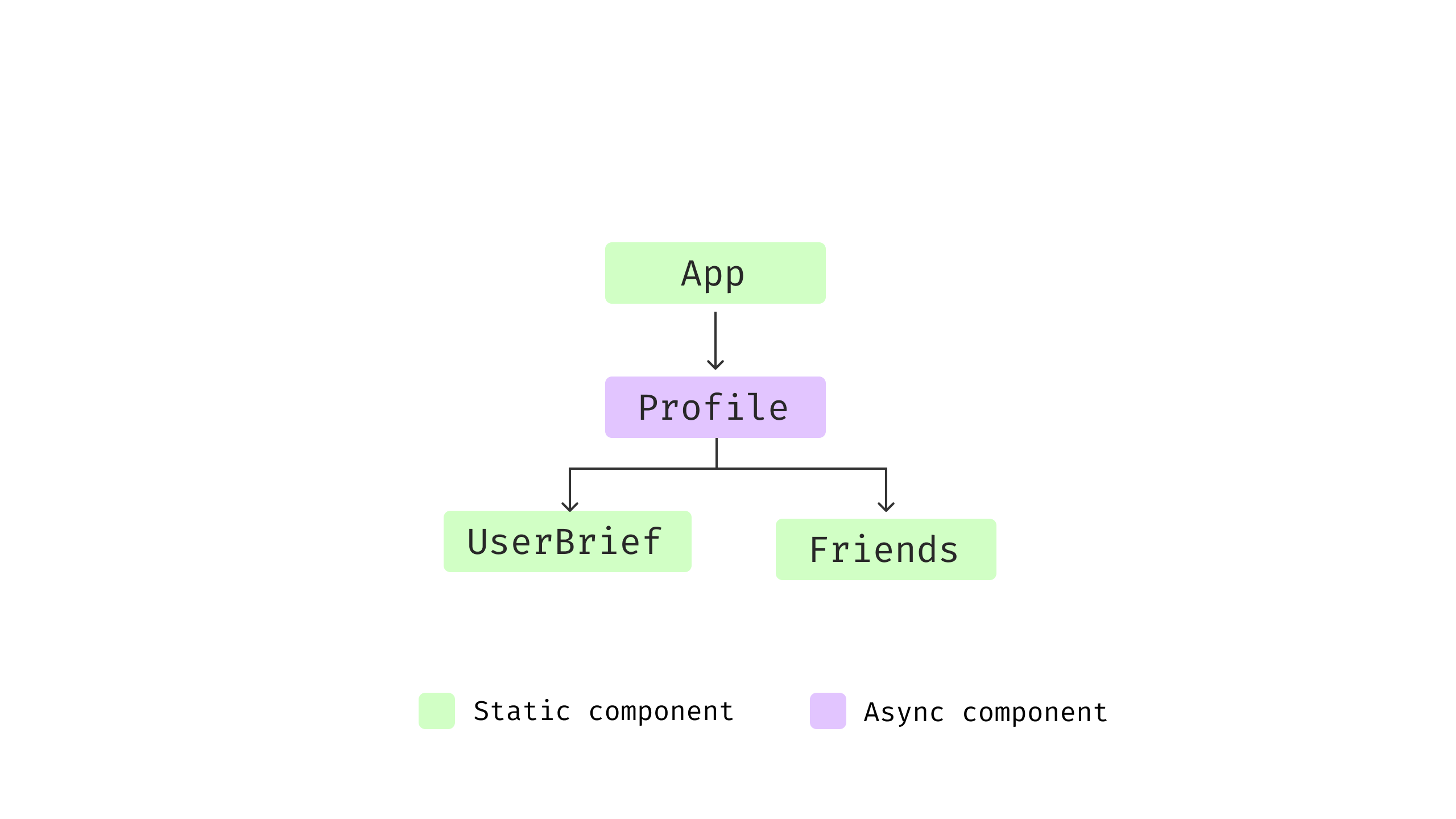
Determine 8: Element construction after refactoring
And the timeline is far shorter than the earlier one as we ship two
requests in parallel. The Pals
part can render in a number of
milliseconds as when it begins to render, the information is already prepared and
handed in.
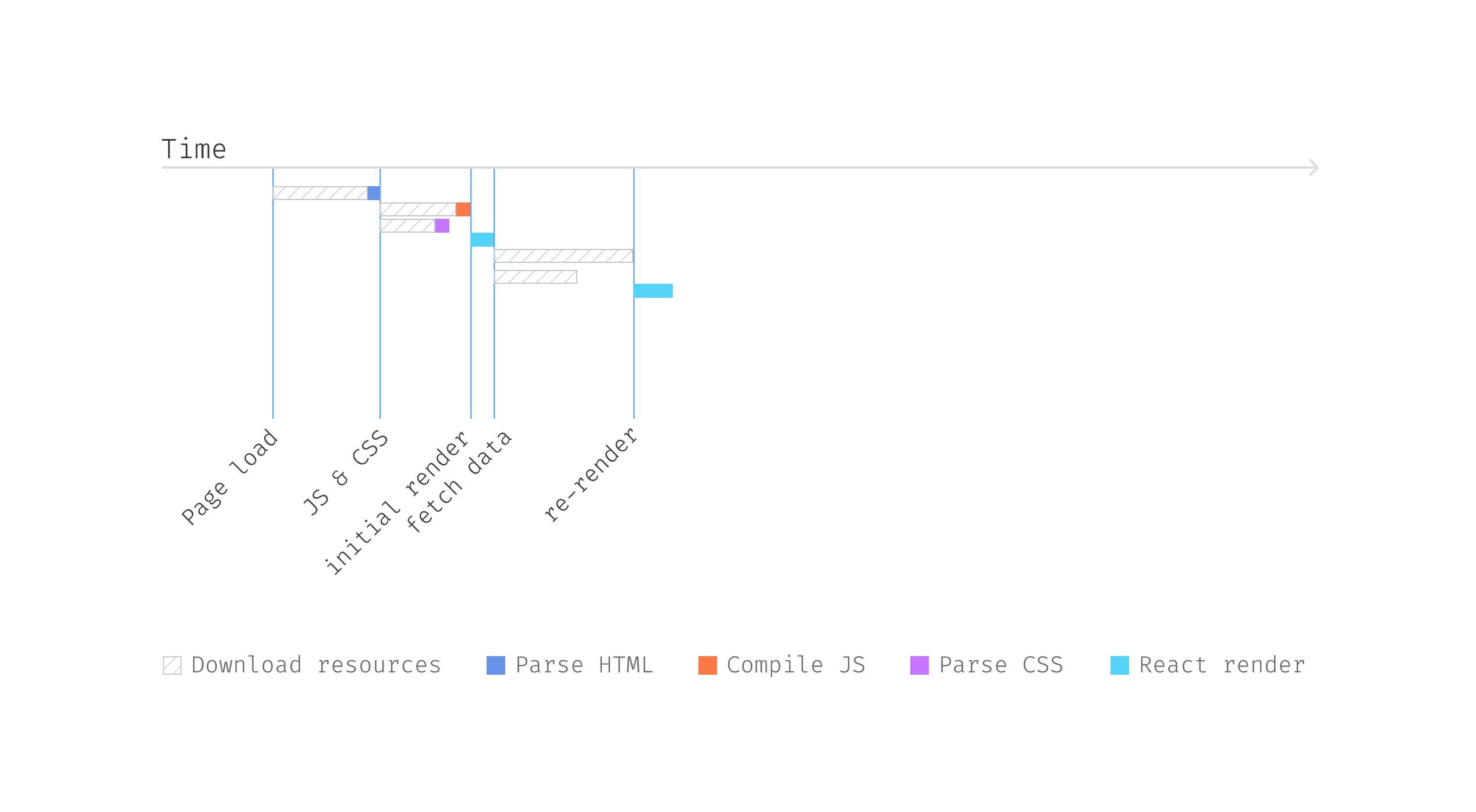
Determine 9: Parallel requests
Word that the longest wait time depends upon the slowest community
request, which is far sooner than the sequential ones. And if we might
ship as many of those impartial requests on the identical time at an higher
stage of the part tree, a greater consumer expertise will be
anticipated.
As purposes increase, managing an rising variety of requests at
root stage turns into difficult. That is significantly true for elements
distant from the foundation, the place passing down information turns into cumbersome. One
strategy is to retailer all information globally, accessible through capabilities (like
Redux or the React Context API), avoiding deep prop drilling.
When to make use of it
Operating queries in parallel is helpful every time such queries could also be
sluggish and do not considerably intrude with every others’ efficiency.
That is often the case with distant queries. Even when the distant
machine’s I/O and computation is quick, there’s all the time potential latency
points within the distant calls. The principle drawback for parallel queries
is setting them up with some form of asynchronous mechanism, which can be
troublesome in some language environments.
The principle cause to not use parallel information fetching is after we do not
know what information must be fetched till we have already fetched some
information. Sure eventualities require sequential information fetching as a consequence of
dependencies between requests. For example, contemplate a situation on a
Profile
web page the place producing a personalised suggestion feed
depends upon first buying the consumer’s pursuits from a consumer API.
Here is an instance response from the consumer API that features
pursuits:
{ "id": "u1", "identify": "Juntao Qiu", "bio": "Developer, Educator, Creator", "pursuits": [ "Technology", "Outdoors", "Travel" ] }
In such instances, the advice feed can solely be fetched after
receiving the consumer’s pursuits from the preliminary API name. This
sequential dependency prevents us from using parallel fetching, as
the second request depends on information obtained from the primary.
Given these constraints, it turns into necessary to debate different
methods in asynchronous information administration. One such technique is
Fallback Markup. This strategy permits builders to specify what
information is required and the way it must be fetched in a approach that clearly
defines dependencies, making it simpler to handle advanced information
relationships in an utility.
One other instance of when arallel Knowledge Fetching will not be relevant is
that in eventualities involving consumer interactions that require real-time
information validation.
Contemplate the case of an inventory the place every merchandise has an “Approve” context
menu. When a consumer clicks on the “Approve” choice for an merchandise, a dropdown
menu seems providing decisions to both “Approve” or “Reject.” If this
merchandise’s approval standing could possibly be modified by one other admin concurrently,
then the menu choices should replicate probably the most present state to keep away from
conflicting actions.
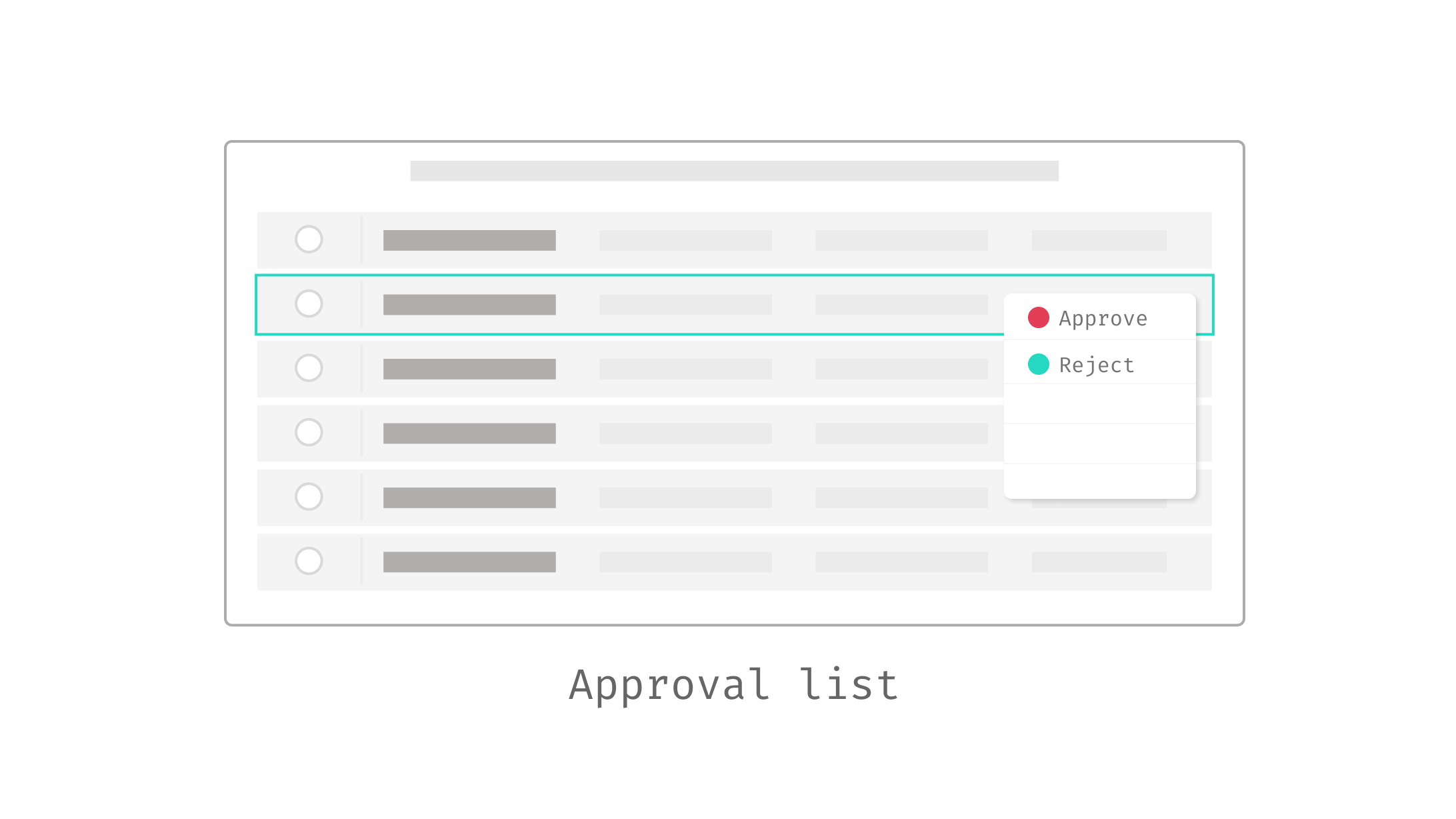
Determine 10: The approval listing that require in-time
states
To deal with this, a service name is initiated every time the context
menu is activated. This service fetches the newest standing of the merchandise,
guaranteeing that the dropdown is constructed with probably the most correct and
present choices accessible at that second. Because of this, these requests
can’t be made in parallel with different data-fetching actions because the
dropdown’s contents rely fully on the real-time standing fetched from
the server.
Fallback Markup
Specify fallback shows within the web page markup
This sample leverages abstractions supplied by frameworks or libraries
to deal with the information retrieval course of, together with managing states like
loading, success, and error, behind the scenes. It permits builders to
concentrate on the construction and presentation of information of their purposes,
selling cleaner and extra maintainable code.
Let’s take one other have a look at the Pals
part within the above
part. It has to take care of three completely different states and register the
callback in useEffect
, setting the flag accurately on the proper time,
organize the completely different UI for various states:
const Pals = ({ id }: { id: string }) => { //... const { loading, error, information: mates, fetch: fetchFriends, } = useService(`/customers/${id}/mates`); useEffect(() => { fetchFriends(); }, []); if (loading) { // present loading indicator } if (error) { // present error message part } // present the acutal buddy listing };
You’ll discover that inside a part we now have to take care of
completely different states, even we extract customized Hook to cut back the noise in a
part, we nonetheless have to pay good consideration to dealing with
loading
and error
inside a part. These
boilerplate code will be cumbersome and distracting, typically cluttering the
readability of our codebase.
If we consider declarative API, like how we construct our UI with JSX, the
code will be written within the following method that lets you concentrate on
what the part is doing – not easy methods to do it:
<WhenError fallback={<ErrorMessage />}> <WhenInProgress fallback={<Loading />}> <Pals /> </WhenInProgress> </WhenError>
Within the above code snippet, the intention is straightforward and clear: when an
error happens, ErrorMessage
is displayed. Whereas the operation is in
progress, Loading is proven. As soon as the operation completes with out errors,
the Pals part is rendered.
And the code snippet above is fairly similiar to what already be
carried out in a number of libraries (together with React and Vue.js). For instance,
the brand new Suspense
in React permits builders to extra successfully handle
asynchronous operations inside their elements, enhancing the dealing with of
loading states, error states, and the orchestration of concurrent
duties.
Implementing Fallback Markup in React with Suspense
Suspense
in React is a mechanism for effectively dealing with
asynchronous operations, similar to information fetching or useful resource loading, in a
declarative method. By wrapping elements in a Suspense
boundary,
builders can specify fallback content material to show whereas ready for the
part’s information dependencies to be fulfilled, streamlining the consumer
expertise throughout loading states.
Whereas with the Suspense API, within the Pals
you describe what you
wish to get after which render:
import useSWR from "swr"; import { get } from "../utils.ts"; perform Pals({ id }: { id: string }) { const { information: customers } = useSWR("/api/profile", () => get<Person[]>(`/customers/${id}/mates`), { suspense: true, }); return ( <div> <h2>Pals</h2> <div> {mates.map((consumer) => ( <Good friend consumer={consumer} key={consumer.id} /> ))} </div> </div> ); }
And declaratively whenever you use the Pals
, you employ
Suspense
boundary to wrap across the Pals
part:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
Suspense
manages the asynchronous loading of the
Pals
part, displaying a FriendsSkeleton
placeholder till the part’s information dependencies are
resolved. This setup ensures that the consumer interface stays responsive
and informative throughout information fetching, enhancing the general consumer
expertise.
Use the sample in Vue.js
It is value noting that Vue.js can be exploring an analogous
experimental sample, the place you’ll be able to make use of Fallback Markup utilizing:
<Suspense> <template #default> <AsyncComponent /> </template> <template #fallback> Loading... </template> </Suspense>
Upon the primary render, <Suspense>
makes an attempt to render
its default content material behind the scenes. Ought to it encounter any
asynchronous dependencies throughout this section, it transitions right into a
pending state, the place the fallback content material is displayed as an alternative. As soon as all
the asynchronous dependencies are efficiently loaded,
<Suspense>
strikes to a resolved state, and the content material
initially supposed for show (the default slot content material) is
rendered.
Deciding Placement for the Loading Element
It’s possible you’ll marvel the place to position the FriendsSkeleton
part and who ought to handle it. Sometimes, with out utilizing Fallback
Markup, this determination is simple and dealt with immediately throughout the
part that manages the information fetching:
const Pals = ({ id }: { id: string }) => { // Knowledge fetching logic right here... if (loading) { // Show loading indicator } if (error) { // Show error message part } // Render the precise buddy listing };
On this setup, the logic for displaying loading indicators or error
messages is of course located throughout the Pals
part. Nonetheless,
adopting Fallback Markup shifts this duty to the
part’s client:
<Suspense fallback={<FriendsSkeleton />}> <Pals id={id} /> </Suspense>
In real-world purposes, the optimum strategy to dealing with loading
experiences relies upon considerably on the specified consumer interplay and
the construction of the applying. For example, a hierarchical loading
strategy the place a father or mother part ceases to point out a loading indicator
whereas its kids elements proceed can disrupt the consumer expertise.
Thus, it is essential to rigorously contemplate at what stage throughout the
part hierarchy the loading indicators or skeleton placeholders
must be displayed.
Consider Pals
and FriendsSkeleton
as two
distinct part states—one representing the presence of information, and the
different, the absence. This idea is considerably analogous to utilizing a Speical Case sample in object-oriented
programming, the place FriendsSkeleton
serves because the ‘null’
state dealing with for the Pals
part.
The bottom line is to find out the granularity with which you wish to
show loading indicators and to take care of consistency in these
selections throughout your utility. Doing so helps obtain a smoother and
extra predictable consumer expertise.
When to make use of it
Utilizing Fallback Markup in your UI simplifies code by enhancing its readability
and maintainability. This sample is especially efficient when using
normal elements for varied states similar to loading, errors, skeletons, and
empty views throughout your utility. It reduces redundancy and cleans up
boilerplate code, permitting elements to focus solely on rendering and
performance.
Fallback Markup, similar to React’s Suspense, standardizes the dealing with of
asynchronous loading, guaranteeing a constant consumer expertise. It additionally improves
utility efficiency by optimizing useful resource loading and rendering, which is
particularly useful in advanced purposes with deep part bushes.
Nonetheless, the effectiveness of Fallback Markup depends upon the capabilities of
the framework you’re utilizing. For instance, React’s implementation of Suspense for
information fetching nonetheless requires third-party libraries, and Vue’s assist for
related options is experimental. Furthermore, whereas Fallback Markup can scale back
complexity in managing state throughout elements, it could introduce overhead in
easier purposes the place managing state immediately inside elements might
suffice. Moreover, this sample might restrict detailed management over loading and
error states—conditions the place completely different error varieties want distinct dealing with may
not be as simply managed with a generic fallback strategy.
[ad_2]