Larger-order Features, Avro and Customized Serializers
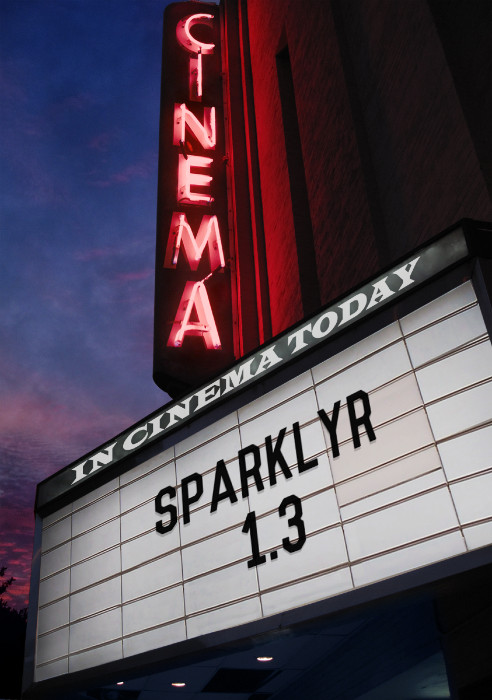
sparklyr
1.3 is now obtainable on CRAN, with the next main new options:
- Larger-order Features to simply manipulate arrays and structs
- Assist for Apache Avro, a row-oriented information serialization framework
- Customized Serialization utilizing R features to learn and write any information format
- Different Enhancements similar to compatibility with EMR 6.0 & Spark 3.0, and preliminary help for Flint time sequence library
To put in sparklyr
1.3 from CRAN, run
On this submit, we will spotlight some main new options launched in sparklyr 1.3, and showcase situations the place such options come in useful. Whereas numerous enhancements and bug fixes (particularly these associated to spark_apply()
, Apache Arrow, and secondary Spark connections) had been additionally an essential a part of this launch, they won’t be the subject of this submit, and will probably be a straightforward train for the reader to search out out extra about them from the sparklyr NEWS file.
Larger-order Features
Larger-order features are built-in Spark SQL constructs that enable user-defined lambda expressions to be utilized effectively to advanced information varieties similar to arrays and structs. As a fast demo to see why higher-order features are helpful, let’s say at some point Scrooge McDuck dove into his big vault of cash and located massive portions of pennies, nickels, dimes, and quarters. Having an impeccable style in information buildings, he determined to retailer the portions and face values of every thing into two Spark SQL array columns:
Thus declaring his web price of 4k pennies, 3k nickels, 2k dimes, and 1k quarters. To assist Scrooge McDuck calculate the whole worth of every sort of coin in sparklyr 1.3 or above, we are able to apply hof_zip_with()
, the sparklyr equal of ZIP_WITH, to portions
column and values
column, combining pairs of parts from arrays in each columns. As you might need guessed, we additionally have to specify methods to mix these parts, and what higher technique to accomplish that than a concise one-sided method ~ .x * .y
in R, which says we wish (amount * worth) for every sort of coin? So, we’ve got the next:
[1] 4000 15000 20000 25000
With the consequence 4000 15000 20000 25000
telling us there are in whole $40 {dollars} price of pennies, $150 {dollars} price of nickels, $200 {dollars} price of dimes, and $250 {dollars} price of quarters, as anticipated.
Utilizing one other sparklyr perform named hof_aggregate()
, which performs an AGGREGATE operation in Spark, we are able to then compute the online price of Scrooge McDuck based mostly on result_tbl
, storing the lead to a brand new column named whole
. Discover for this combination operation to work, we have to make sure the beginning worth of aggregation has information sort (particularly, BIGINT
) that’s per the info sort of total_values
(which is ARRAY<BIGINT>
), as proven beneath:
[1] 64000
So Scrooge McDuck’s web price is $640 {dollars}.
Different higher-order features supported by Spark SQL thus far embrace rework
, filter
, and exists
, as documented in right here, and much like the instance above, their counterparts (particularly, hof_transform()
, hof_filter()
, and hof_exists()
) all exist in sparklyr 1.3, in order that they are often built-in with different dplyr
verbs in an idiomatic method in R.
Avro
One other spotlight of the sparklyr 1.3 launch is its built-in help for Avro information sources. Apache Avro is a extensively used information serialization protocol that mixes the effectivity of a binary information format with the flexibleness of JSON schema definitions. To make working with Avro information sources easier, in sparklyr 1.3, as quickly as a Spark connection is instantiated with spark_connect(..., bundle = "avro")
, sparklyr will robotically determine which model of spark-avro
bundle to make use of with that connection, saving lots of potential complications for sparklyr customers making an attempt to find out the proper model of spark-avro
by themselves. Much like how spark_read_csv()
and spark_write_csv()
are in place to work with CSV information, spark_read_avro()
and spark_write_avro()
strategies had been carried out in sparklyr 1.3 to facilitate studying and writing Avro information via an Avro-capable Spark connection, as illustrated within the instance beneath:
library(sparklyr)
# The `bundle = "avro"` possibility is just supported in Spark 2.4 or larger
sc <- spark_connect(grasp = "native", model = "2.4.5", bundle = "avro")
sdf <- sdf_copy_to(
sc,
tibble::tibble(
a = c(1, NaN, 3, 4, NaN),
b = c(-2L, 0L, 1L, 3L, 2L),
c = c("a", "b", "c", "", "d")
)
)
# This instance Avro schema is a JSON string that primarily says all columns
# ("a", "b", "c") of `sdf` are nullable.
avro_schema <- jsonlite::toJSON(listing(
sort = "report",
identify = "topLevelRecord",
fields = listing(
listing(identify = "a", sort = listing("double", "null")),
listing(identify = "b", sort = listing("int", "null")),
listing(identify = "c", sort = listing("string", "null"))
)
), auto_unbox = TRUE)
# persist the Spark information body from above in Avro format
spark_write_avro(sdf, "/tmp/information.avro", as.character(avro_schema))
# after which learn the identical information body again
spark_read_avro(sc, "/tmp/information.avro")
# Supply: spark<information> [?? x 3]
a b c
<dbl> <int> <chr>
1 1 -2 "a"
2 NaN 0 "b"
3 3 1 "c"
4 4 3 ""
5 NaN 2 "d"
Customized Serialization
Along with generally used information serialization codecs similar to CSV, JSON, Parquet, and Avro, ranging from sparklyr 1.3, custom-made information body serialization and deserialization procedures carried out in R will also be run on Spark employees through the newly carried out spark_read()
and spark_write()
strategies. We are able to see each of them in motion via a fast instance beneath, the place saveRDS()
known as from a user-defined author perform to avoid wasting all rows inside a Spark information body into 2 RDS information on disk, and readRDS()
known as from a user-defined reader perform to learn the info from the RDS information again to Spark:
# Supply: spark<?> [?? x 1]
id
<int>
1 1
2 2
3 3
4 4
5 5
6 6
7 7
Different Enhancements
Sparklyr.flint
Sparklyr.flint is a sparklyr extension that goals to make functionalities from the Flint time-series library simply accessible from R. It’s at present beneath lively growth. One piece of excellent information is that, whereas the unique Flint library was designed to work with Spark 2.x, a barely modified fork of it is going to work effectively with Spark 3.0, and inside the current sparklyr extension framework. sparklyr.flint
can robotically decide which model of the Flint library to load based mostly on the model of Spark it’s related to. One other bit of excellent information is, as beforehand talked about, sparklyr.flint
doesn’t know an excessive amount of about its personal future but. Perhaps you’ll be able to play an lively half in shaping its future!
EMR 6.0
This launch additionally contains a small however essential change that enables sparklyr to accurately hook up with the model of Spark 2.4 that’s included in Amazon EMR 6.0.
Beforehand, sparklyr robotically assumed any Spark 2.x it was connecting to was constructed with Scala 2.11 and tried to load any required Scala artifacts constructed with Scala 2.11 as effectively. This grew to become problematic when connecting to Spark 2.4 from Amazon EMR 6.0, which is constructed with Scala 2.12. Ranging from sparklyr 1.3, such drawback may be fastened by merely specifying scala_version = "2.12"
when calling spark_connect()
(e.g., spark_connect(grasp = "yarn-client", scala_version = "2.12")
).
Spark 3.0
Final however not least, it’s worthwhile to say sparklyr 1.3.0 is thought to be absolutely appropriate with the lately launched Spark 3.0. We extremely advocate upgrading your copy of sparklyr to 1.3.0 when you plan to have Spark 3.0 as a part of your information workflow in future.
Acknowledgement
In chronological order, we need to thank the next people for submitting pull requests in direction of sparklyr 1.3:
We’re additionally grateful for useful enter on the sparklyr 1.3 roadmap, #2434, and #2551 from [@javierluraschi](https://github.com/javierluraschi), and nice non secular recommendation on #1773 and #2514 from @mattpollock and @benmwhite.
Please be aware when you imagine you’re lacking from the acknowledgement above, it might be as a result of your contribution has been thought of a part of the following sparklyr launch quite than half of the present launch. We do make each effort to make sure all contributors are talked about on this part. In case you imagine there’s a mistake, please be happy to contact the writer of this weblog submit through e-mail (yitao at rstudio dot com) and request a correction.
If you happen to want to study extra about sparklyr
, we advocate visiting sparklyr.ai, spark.rstudio.com, and among the earlier launch posts similar to sparklyr 1.2 and sparklyr 1.1.
Thanks for studying!