[ad_1]
Introduction
For creating eye-catching and academic statistics visuals, Seaborn offers a high-level interface. Seaborn makes it easy to construct and modify line plots, that are useful for displaying information traits over time. This tutorial goes over arrange your surroundings, make each easy and customized line plots, and customise your plots with completely different results.
Overview
- Learn to arrange Seaborn and generate pattern information utilizing NumPy for creating line plots.
- Develop expertise to create primary line plots in Seaborn and customise them by altering line kinds, colours, and including markers.
- Perceive plot a number of traces on a single plot to check completely different datasets successfully.
- Grasp including annotations to spotlight key factors and saving plots as picture recordsdata for higher information communication.
Setting Up Your Atmosphere
Earlier than you start, guarantee you could have the mandatory libraries put in. You possibly can set up Seaborn and its dependencies utilizing pip:
pip set up seaborn matplotlib numpy
Importing Libraries
First, import the required libraries. Seaborn depends on Matplotlib for underlying plotting and NumPy for information manipulation.
import seaborn as sns
import numpy as np
import matplotlib.pyplot as plt
Producing Pattern Information
For demonstration functions, let’s generate some pattern information utilizing NumPy. We’ll create a easy dataset representing a sine wave and a cosine wave.
# Generate 1000 evenly spaced values from 0 to 10
x = np.linspace(0, 10, 1000)
# Generate corresponding sine and cosine values
y = np.sin(x)
y2 = np.cos(x)
Making a Primary Line Plot
This part covers use Seaborn to construct a easy line plot, with an emphasis on surroundings setup, pattern information era, and plot creation that’s instructive. It covers customizing Seaborn to enhance readability by using its user-friendly options.
plt.determine(figsize=(10, 6)) # Set the determine dimension
sns.lineplot(x=x, y=y, label="Sine Wave") # Create a line plot with a label
plt.title('Primary Line Plot with Seaborn') # Add a title
plt.xlabel('X-axis') # Add X-axis label
plt.ylabel('Y-axis') # Add Y-axis label
plt.legend() # Show the legend
plt.present() # Show the plot
Output:
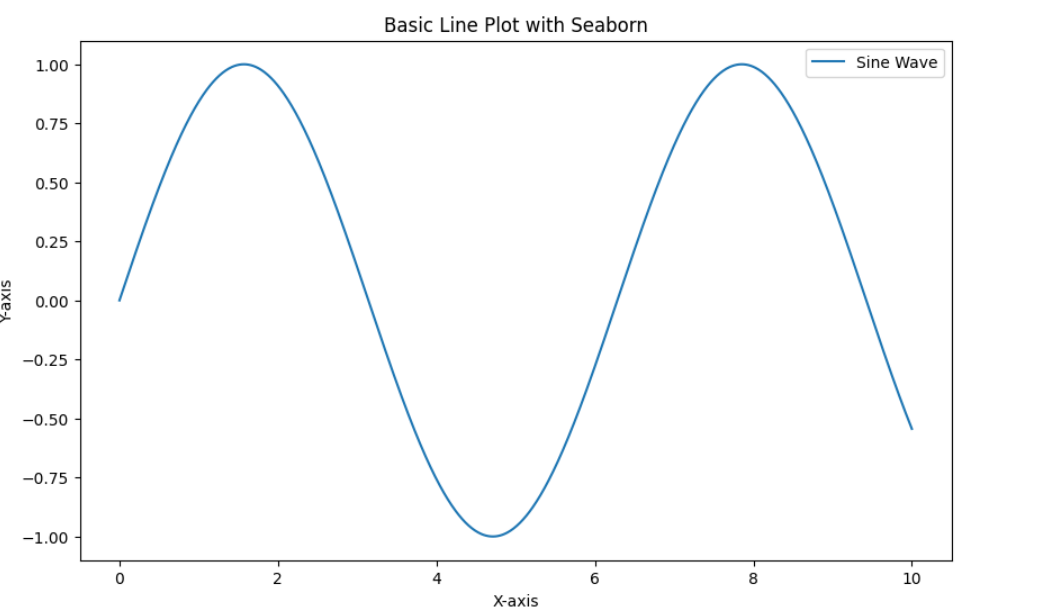
Customizing the Line Plot
You possibly can change the colours, kinds, and different components of your Seaborn line plots.
Altering Line Types and Colours
Making visible adjustments to your plot utilizing Seaborn is straightforward. It lets you merely regulate the road type, coloration, and width.
plt.determine(figsize=(10, 6))
sns.lineplot(x=x, y=y, coloration="blue", linestyle="--", linewidth=2, label="Sine Wave")
plt.title('Personalized Line Plot with Seaborn')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.present()
Output:
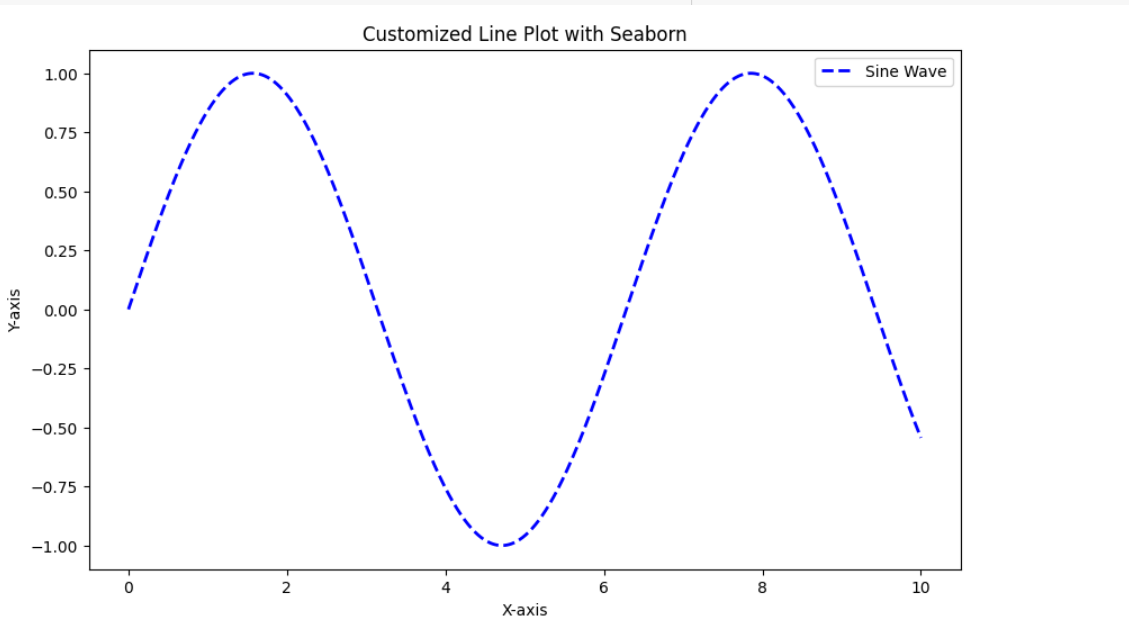
Including Markers
To attract consideration to sure information factors, you should use markers in your line plot.
plt.determine(figsize=(10, 6))
sns.lineplot(x=x, y=y, coloration="inexperienced", linestyle="-", linewidth=1, marker="o", markersize=4, label="Sine Wave with Markers")
plt.title('Line Plot with Markers in Seaborn')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.present()
Output:
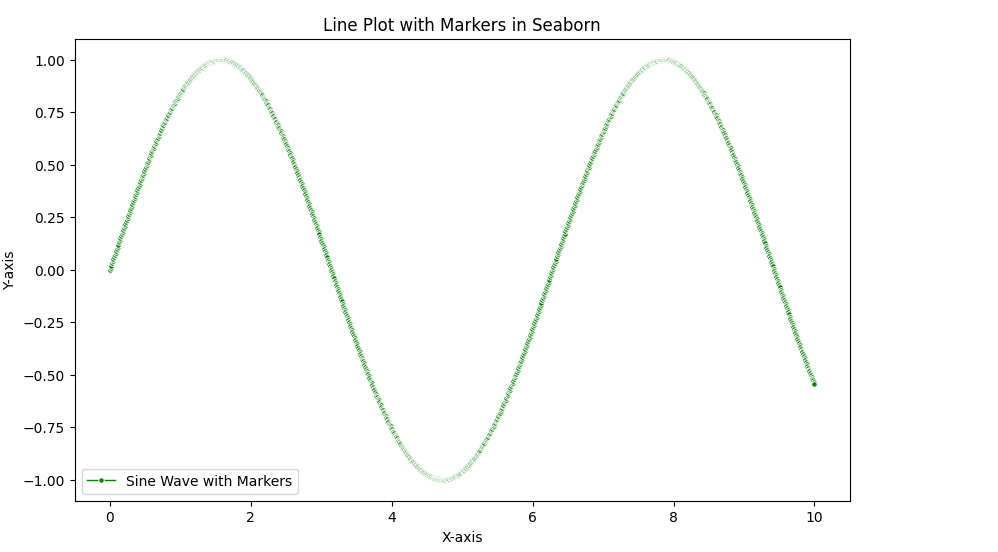
A number of Traces
You may as well plot a number of traces on the identical plot to check completely different datasets. That is extensively used options of line plot.
plt.determine(figsize=(10, 6))
sns.lineplot(x=x, y=y, label="Sine Wave")
sns.lineplot(x=x, y=y2, label="Cosine Wave", linestyle="--")
plt.title('A number of Traces Plot with Seaborn')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.present()
Output:
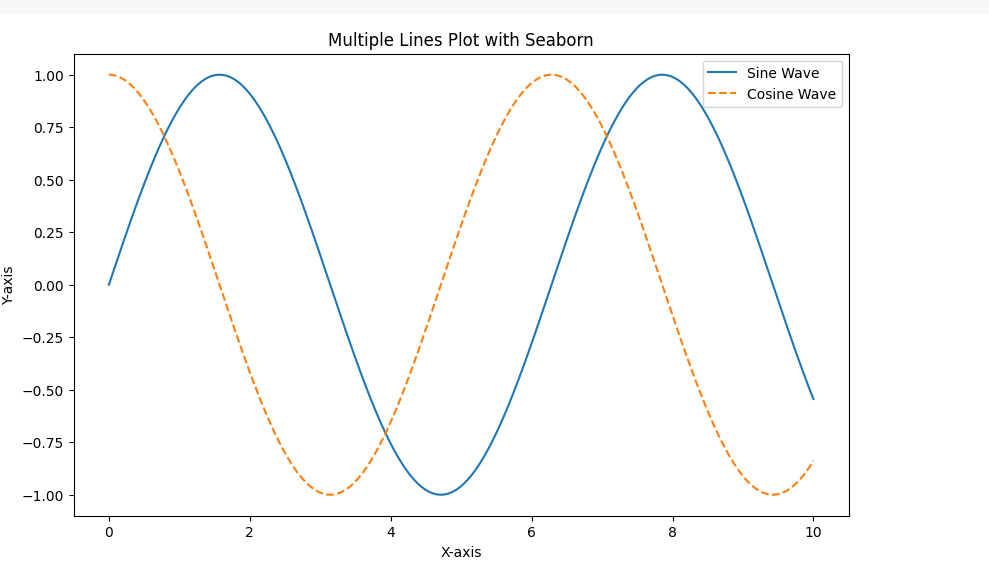
Including Annotations
Annotations can spotlight particular factors on the road plot. In addition they present extra info in your plot as proven within the code beneath:
plt.determine(figsize=(10, 6))
sns.lineplot(x=x, y=y, label="Sine Wave")
sns.lineplot(x=x, y=y2, label="Cosine Wave", linestyle="--")
plt.title('Line Plot with Annotations in Seaborn')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# Annotate the purpose the place sine and cosine intersect
plt.annotate('Intersection', xy=(np.pi/4, np.sin(np.pi/4)), xytext=(3, 0.5),
arrowprops=dict(facecolor="black", shrink=0.05))
plt.legend()
plt.present()
Output:
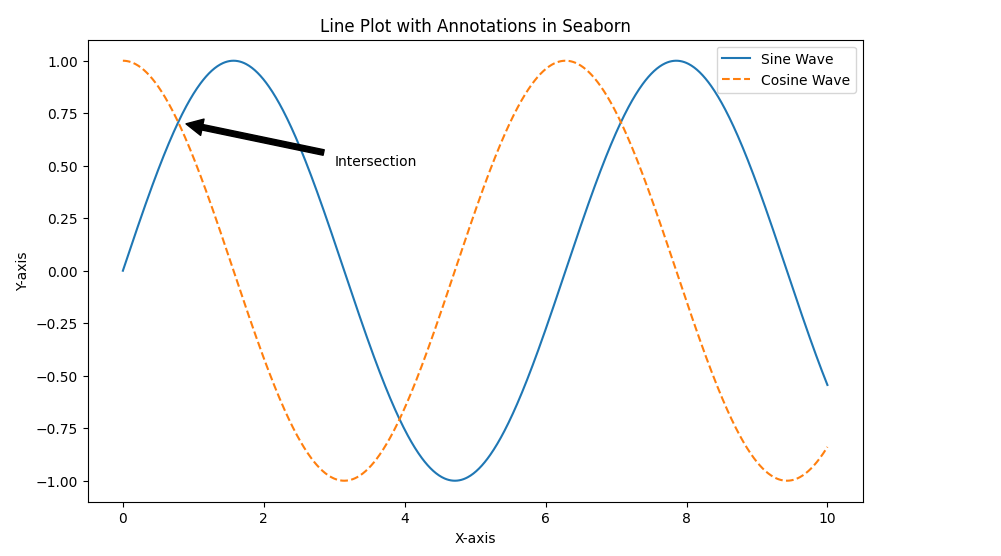
Saving the Plot
It can save you the plot to a file utilizing savefig.
plt.determine(figsize=(10, 6))
sns.lineplot(x=x, y=y, label="Sine Wave")
sns.lineplot(x=x, y=y2, label="Cosine Wave", linestyle="--")
plt.title('Line Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.savefig('line_plot_seaborn.png') # Save the plot as a PNG file
plt.present()
Full Code Instance
Beneath is the whole code instance that features all of the customizations that we have now mentioned above.
import seaborn as sns
import matplotlib.pyplot as plt
import numpy as np
# Generate pattern information
x = np.linspace(0, 10, 1000)
y = np.sin(x)
y2 = np.cos(x)
# Create and customise the plot
plt.determine(figsize=(10, 6))
sns.lineplot(x=x, y=y, coloration="blue", linestyle="-", linewidth=2, marker="o", markersize=4, label="Sine Wave")
sns.lineplot(x=x, y=y2, coloration="purple", linestyle="--", linewidth=2, label="Cosine Wave")
plt.title('Full Line Plot Instance with Seaborn')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# Annotate the purpose the place sine and cosine intersect
plt.annotate('Intersection', xy=(np.pi/4, np.sin(np.pi/4)), xytext=(3, 0.5),
arrowprops=dict(facecolor="black", shrink=0.05))
plt.legend()
plt.grid(True)
plt.savefig('complete_line_plot_seaborn.png')
plt.present()
Output:
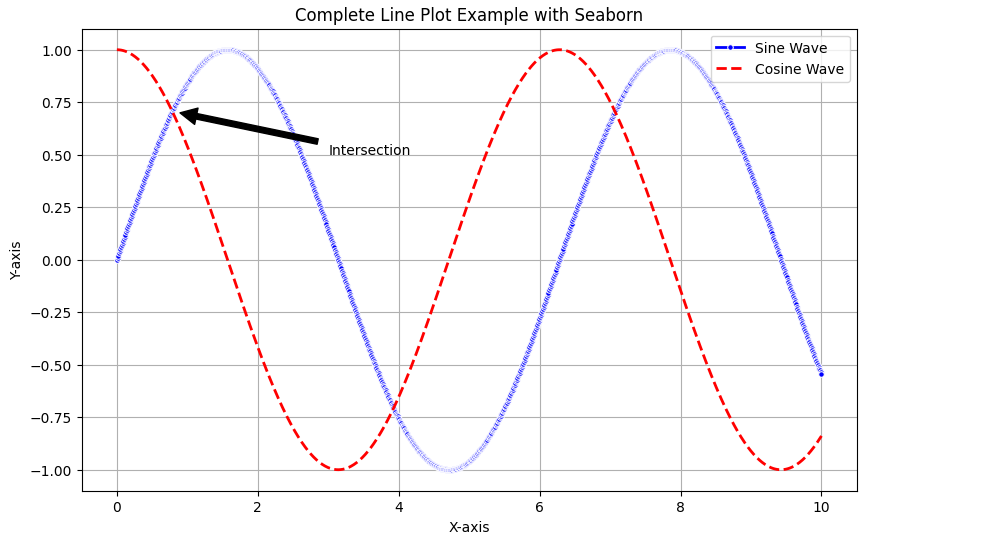
Conclusion
Your skill to create and modify line plots in Seaborn will considerably improve your information visualization talents. Chances are you’ll now effectively annotate info, produce and show information, modify charts, evaluate varied datasets, and handle your system. These expertise will allow you to create visually interesting line plots. It additionally assist in creating instructive line graphs that successfully talk your information findings. On this article we explored creating line plot with seaborn.
Don’t miss this opportunity to enhance your expertise and advance your profession. Be taught Python with us! This course is appropriate for all ranges.
Incessantly Requested Questions
A. Seaborn is a Python information visualization library based mostly on Matplotlib. It offers a high-level interface for drawing enticing and informative statistical graphics.
A. Sure, you could alter the markers, colours, and line kinds of line plots in Seaborn to enhance their visible enchantment and successfully talk information insights.
A. Sure, Seaborn is beginner-friendly and presents intuitive methods to create visually interesting statistical plots with minimal code.
[ad_2]