Posit AI Weblog: torch 0.2.0
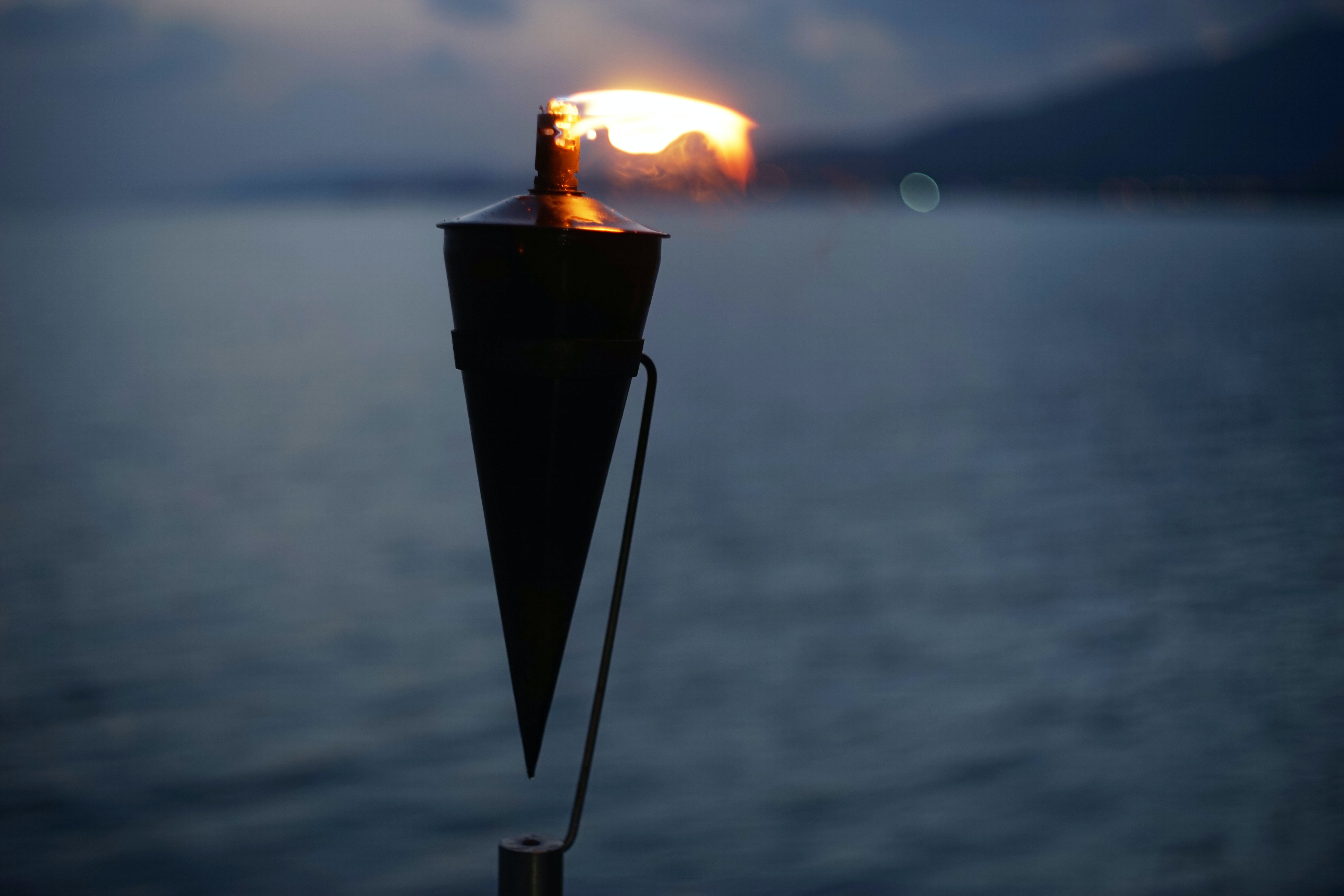
We’re blissful to announce that the model 0.2.0 of torch
simply landed on CRAN.
This launch consists of many bug fixes and a few good new options
that we are going to current on this weblog submit. You may see the total changelog
within the NEWS.md file.
The options that we are going to focus on intimately are:
- Preliminary assist for JIT tracing
- Multi-worker dataloaders
- Print strategies for
nn_modules
Multi-worker dataloaders
dataloaders
now reply to the num_workers
argument and
will run the pre-processing in parallel staff.
For instance, say we’ve the next dummy dataset that does
an extended computation:
library(torch)
dat <- dataset(
"mydataset",
initialize = perform(time, len = 10) {
self$time <- time
self$len <- len
},
.getitem = perform(i) {
Sys.sleep(self$time)
torch_randn(1)
},
.size = perform() {
self$len
}
)
ds <- dat(1)
system.time(ds[1])
consumer system elapsed
0.029 0.005 1.027
We are going to now create two dataloaders, one which executes
sequentially and one other executing in parallel.
seq_dl <- dataloader(ds, batch_size = 5)
par_dl <- dataloader(ds, batch_size = 5, num_workers = 2)
We are able to now examine the time it takes to course of two batches sequentially to
the time it takes in parallel:
seq_it <- dataloader_make_iter(seq_dl)
par_it <- dataloader_make_iter(par_dl)
two_batches <- perform(it) {
dataloader_next(it)
dataloader_next(it)
"okay"
}
system.time(two_batches(seq_it))
system.time(two_batches(par_it))
consumer system elapsed
0.098 0.032 10.086
consumer system elapsed
0.065 0.008 5.134
Observe that it’s batches which are obtained in parallel, not particular person observations. Like that, we can assist
datasets with variable batch sizes sooner or later.
Utilizing a number of staff is not essentially sooner than serial execution as a result of there’s a substantial overhead
when passing tensors from a employee to the principle session as
effectively as when initializing the employees.
This characteristic is enabled by the highly effective callr
package deal
and works in all working methods supported by torch
. callr
let’s
us create persistent R classes, and thus, we solely pay as soon as the overhead of transferring probably massive dataset
objects to staff.
Within the strategy of implementing this characteristic we’ve made
dataloaders behave like coro
iterators.
This implies which you can now use coro
’s syntax
for looping by means of the dataloaders:
coro::loop(for(batch in par_dl) {
print(batch$form)
})
[1] 5 1
[1] 5 1
That is the primary torch
launch together with the multi-worker
dataloaders characteristic, and also you may run into edge circumstances when
utilizing it. Do tell us for those who discover any issues.
Preliminary JIT assist
Packages that make use of the torch
package deal are inevitably
R packages and thus, they at all times want an R set up so as
to execute.
As of model 0.2.0, torch
permits customers to JIT hint
torch
R features into TorchScript. JIT (Simply in time) tracing will invoke
an R perform with instance inputs, report all operations that
occured when the perform was run and return a script_function
object
containing the TorchScript illustration.
The good factor about that is that TorchScript packages are simply
serializable, optimizable, and they are often loaded by one other
program written in PyTorch or LibTorch with out requiring any R
dependency.
Suppose you’ve the next R perform that takes a tensor,
and does a matrix multiplication with a set weight matrix and
then provides a bias time period:
w <- torch_randn(10, 1)
b <- torch_randn(1)
fn <- perform(x) {
a <- torch_mm(x, w)
a + b
}
This perform might be JIT-traced into TorchScript with jit_trace
by passing the perform and instance inputs:
x <- torch_ones(2, 10)
tr_fn <- jit_trace(fn, x)
tr_fn(x)
torch_tensor
-0.6880
-0.6880
[ CPUFloatType{2,1} ]
Now all torch
operations that occurred when computing the results of
this perform have been traced and reworked right into a graph:
graph(%0 : Float(2:10, 10:1, requires_grad=0, system=cpu)):
%1 : Float(10:1, 1:1, requires_grad=0, system=cpu) = prim::Fixed[value=-0.3532 0.6490 -0.9255 0.9452 -1.2844 0.3011 0.4590 -0.2026 -1.2983 1.5800 [ CPUFloatType{10,1} ]]()
%2 : Float(2:1, 1:1, requires_grad=0, system=cpu) = aten::mm(%0, %1)
%3 : Float(1:1, requires_grad=0, system=cpu) = prim::Fixed[value={-0.558343}]()
%4 : int = prim::Fixed[value=1]()
%5 : Float(2:1, 1:1, requires_grad=0, system=cpu) = aten::add(%2, %3, %4)
return (%5)
The traced perform might be serialized with jit_save
:
jit_save(tr_fn, "linear.pt")
It may be reloaded in R with jit_load
, nevertheless it will also be reloaded in Python
with torch.jit.load
:
right here. It will permit you additionally to take good thing about TorchScript to make your fashions
run sooner!
Additionally word that tracing has some limitations, particularly when your code has loops
or management stream statements that rely on tensor information. See ?jit_trace
to
be taught extra.
New print methodology for nn_modules
On this launch we’ve additionally improved the nn_module
printing strategies so as
to make it simpler to know what’s inside.
For instance, for those who create an occasion of an nn_linear
module you’ll
see:
An `nn_module` containing 11 parameters.
── Parameters ──────────────────────────────────────────────────────────────────
● weight: Float [1:1, 1:10]
● bias: Float [1:1]
You instantly see the entire variety of parameters within the module in addition to
their names and shapes.
This additionally works for customized modules (probably together with sub-modules). For instance:
my_module <- nn_module(
initialize = perform() {
self$linear <- nn_linear(10, 1)
self$param <- nn_parameter(torch_randn(5,1))
self$buff <- nn_buffer(torch_randn(5))
}
)
my_module()
An `nn_module` containing 16 parameters.
── Modules ─────────────────────────────────────────────────────────────────────
● linear: <nn_linear> #11 parameters
── Parameters ──────────────────────────────────────────────────────────────────
● param: Float [1:5, 1:1]
── Buffers ─────────────────────────────────────────────────────────────────────
● buff: Float [1:5]
We hope this makes it simpler to know nn_module
objects.
We have now additionally improved autocomplete assist for nn_modules
and we’ll now
present all sub-modules, parameters and buffers when you kind.
torchaudio
torchaudio
is an extension for torch
developed by Athos Damiani (@athospd
), offering audio loading, transformations, frequent architectures for sign processing, pre-trained weights and entry to generally used datasets. An virtually literal translation from PyTorch’s Torchaudio library to R.
torchaudio
is just not but on CRAN, however you may already strive the event model
obtainable right here.
You may as well go to the pkgdown
web site for examples and reference documentation.
Different options and bug fixes
Because of group contributions we’ve discovered and stuck many bugs in torch
.
We have now additionally added new options together with:
You may see the total listing of adjustments within the NEWS.md file.
Thanks very a lot for studying this weblog submit, and be at liberty to succeed in out on GitHub for assist or discussions!
The picture used on this submit preview is by Oleg Illarionov on Unsplash