[ad_1]
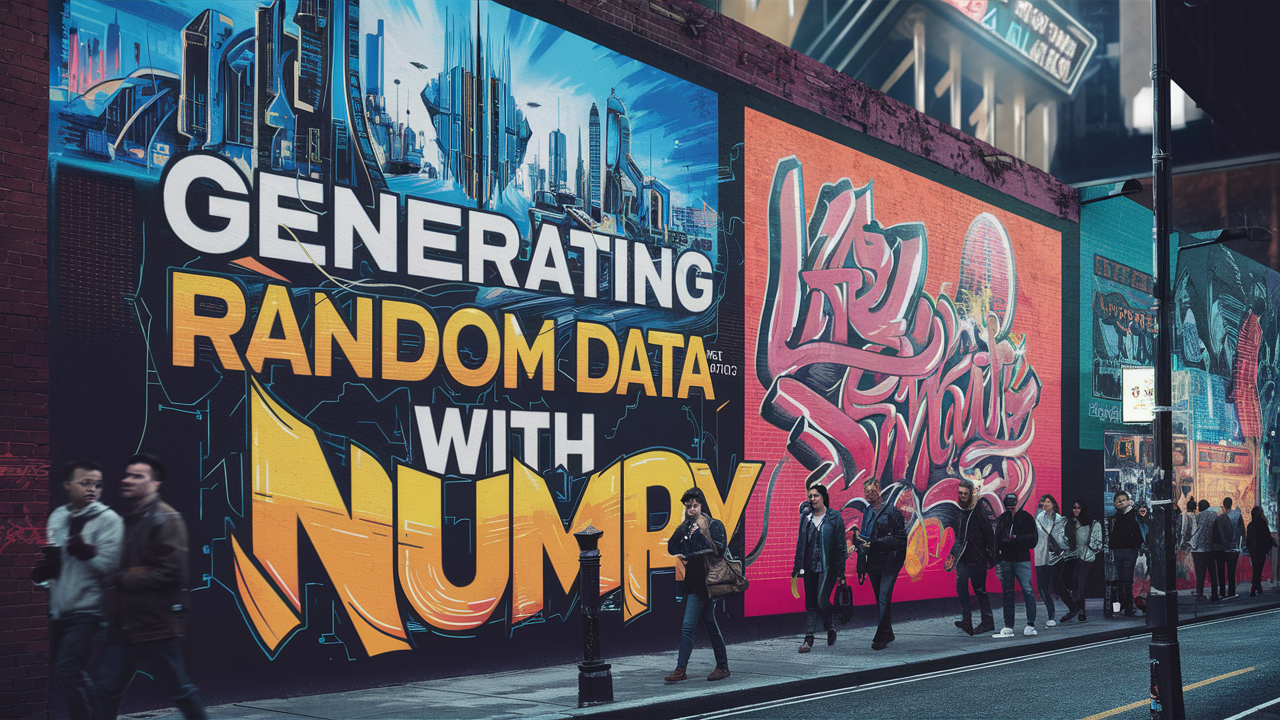
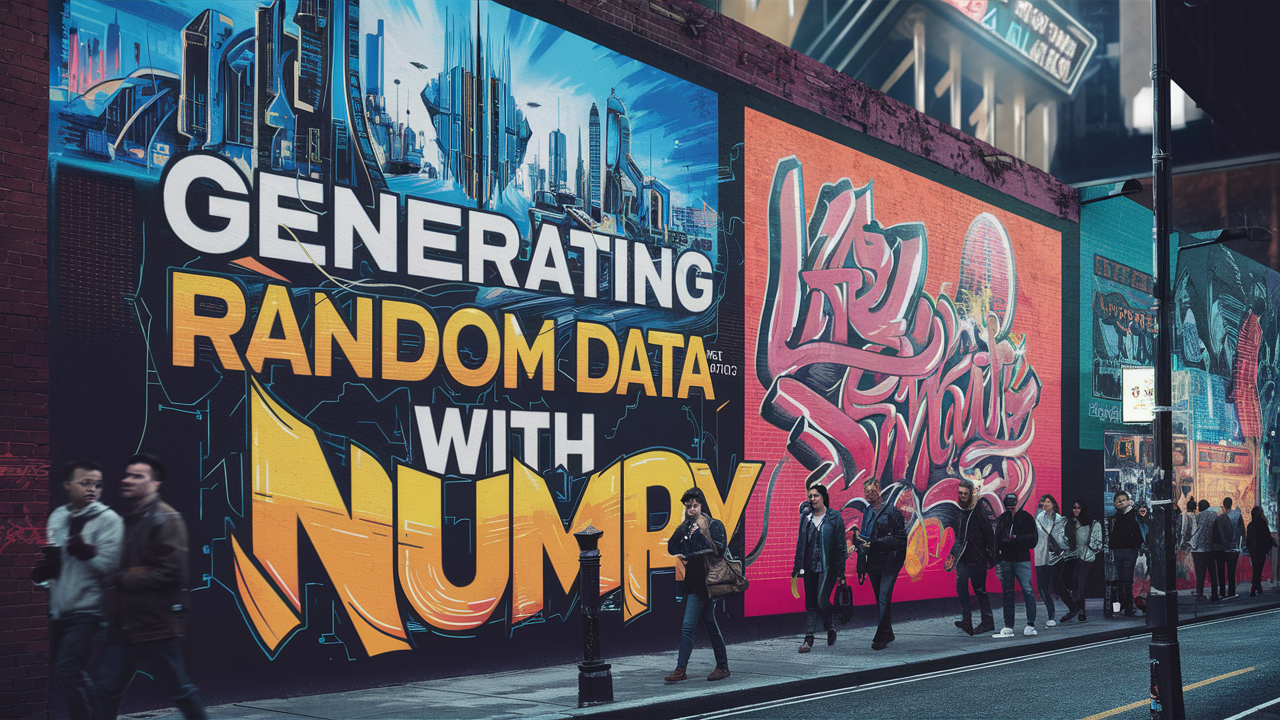
Picture by Editor | Ideogram
Random information consists of values generated by way of varied instruments with out predictable patterns. The prevalence of values depends upon the chance distribution from which they’re drawn as a result of they’re unpredictable.
There are numerous advantages to utilizing Random Information in our experiments, together with real-world information simulation, artificial information for machine studying coaching, or statistical sampling functions.
NumPy is a robust bundle that helps many mathematical and statistical computations, together with random information technology. From easy information to advanced multi-dimensional arrays and matrices, NumPy might assist us facilitate the necessity for random information technology.
This text will focus on additional how we might generate Random information with Numpy. So, let’s get into it.
Random Information Era with NumPy
That you must have the NumPy bundle put in in your setting. For those who haven’t carried out that, you should use pip to put in them.
When the bundle has been efficiently put in, we’ll transfer on to the principle a part of the article.
First, we’d set the seed quantity for reproducibility functions. After we carry out random occurrences with the pc, we should do not forget that what we do is pseudo-random. The pseudo-random idea is when information appears random however is deterministic if we all know the place the beginning factors which we name seed.
To set the seed in NumPy, we’ll use the next code:
import numpy as np
np.random.seed(101)
You can provide any optimistic integer numbers because the seed quantity, which might develop into our start line. Additionally, the .random
methodology from the NumPy would develop into our most important operate for this text.
As soon as we’ve got set the seed, we’ll attempt to generate random quantity information with NumPy. Let’s attempt to generate 5 completely different float numbers randomly.
Output>>
array([0.51639863, 0.57066759, 0.02847423, 0.17152166, 0.68527698])
It is attainable to get the multi-dimensional array utilizing NumPy. For instance, the next code would lead to 3×3 array crammed with random float numbers.
Output>>
array([[0.26618856, 0.77888791, 0.89206388],
[0.0756819 , 0.82565261, 0.02549692],
[0.5902313 , 0.5342532 , 0.58125755]])
Subsequent, we might generate an integer random quantity from sure vary. We are able to try this with this code:
np.random.randint(1, 1000, dimension=5)
Output>>
array([974, 553, 645, 576, 937])
All the information generated by random sampling beforehand adopted the uniform distribution. It implies that all the information have the same probability to happen. If we iterate the information technology course of to infinity occasions, all of the quantity taken frequency can be near equal.
We are able to generate random information from varied distributions. Right here, we attempt to generate ten random information from the usual regular distribution.
np.random.regular(0, 1, 10)
Output>>
array([-1.31984116, 1.73778011, 0.25983863, -0.317497 , 0.0185246 ,
-0.42062671, 1.02851771, -0.7226102 , -1.17349046, 1.05557983])
The code above takes the Z-score worth from the traditional distribution with imply zero and STD one.
We are able to generate random information following different distributions. Right here is how we use the Poisson distribution to generate random information.
Output>>
array([10, 6, 3, 3, 8, 3, 6, 8, 3, 3])
The random pattern information from Poisson Distribution within the code above would simulate random occasions at a selected common price (5), however the quantity generated might differ.
We might generate random information following the binomial distribution.
np.random.binomial(10, 0.5, 10)
Output>>
array([5, 7, 5, 4, 5, 6, 5, 7, 4, 7])
The code above simulates the experiments we carry out following the Binomial distribution. Simply think about that we carry out coin flips ten occasions (first parameter ten and second parameter chance 0.5); what number of occasions does it present heads? As proven within the output above, we did the experiment ten occasions (the third parameter).
Let’s strive the exponential distribution. With this code, we are able to generate information following the exponential distribution.
np.random.exponential(1, 10)
Output>>
array([0.7916478 , 0.59574388, 0.1622387 , 0.99915554, 0.10660882,
0.3713874 , 0.3766358 , 1.53743068, 1.82033544, 1.20722031])
Exponential distribution explains the time between occasions. For instance, the code above will be mentioned to be ready for the bus to enter the station, which takes a random period of time however, on common, takes 1 minute.
For a complicated technology, you may all the time mix the distribution outcomes to create pattern information following a customized distribution. For instance, 70% of the generated random information under follows a standard distribution, whereas the remaining follows an exponential distribution.
def combined_distribution(dimension=10):
# regular distribution
normal_samples = np.random.regular(loc=0, scale=1, dimension=int(0.7 * dimension))
#exponential distribution
exponential_samples = np.random.exponential(scale=1, dimension=int(0.3 * dimension))
# Mix the samples
combined_samples = np.concatenate([normal_samples, exponential_samples])
# Shuffle thes samples
np.random.shuffle(combined_samples)
return combined_samples
samples = combined_distribution()
samples
Output>>
array([-1.42085224, -0.04597935, -1.22524869, 0.22023681, 1.13025524,
0.74561453, 1.35293768, 1.20491792, -0.7179921 , -0.16645063])
These customized distributions are far more highly effective, particularly if we wish to simulate our information to observe precise case information (which is often extra messy).
Conclusion
NumPy is a robust Python bundle for mathematical and statistical computation. It generates random information that can be utilized for a lot of occasions, reminiscent of information simulations, artificial information for machine studying, and plenty of others.
On this article, we’ve got mentioned how we are able to generate random information with NumPy, together with strategies that would enhance our information technology expertise.
Cornellius Yudha Wijaya is a knowledge science assistant supervisor and information author. Whereas working full-time at Allianz Indonesia, he likes to share Python and information suggestions by way of social media and writing media. Cornellius writes on a wide range of AI and machine studying subjects.
[ad_2]