[ad_1]
Introduction
Python code profiling is important to comprehending efficiency. It facilitates useful resource optimization and bottleneck identification. This text examines the worth of profiling, its parts, and the explanations efficiency optimization wants it. By studying and using profiling methods, you possibly can optimize your code and guarantee improved efficiency and useful resource utilization for more practical and environment friendly purposes. On this article, we’ll have a look at Python’s two most distinguished profiling instruments: timeit and cProfile.
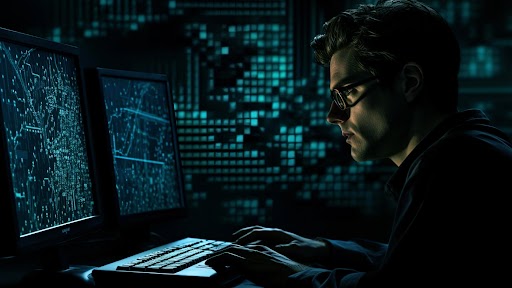
Understanding the Significance of Profiling
What’s Code Profiling?
Code profiling is the method of measuring a program’s efficiency. It tracks the time and reminiscence a program consumes. Profiling instruments gather knowledge about perform calls and their execution time. This knowledge helps builders perceive which elements of the code are sluggish or resource-heavy. By analyzing this data, they will goal particular areas for optimization.
Why Profiling is Important for Efficiency Optimization
Profiling is important for a number of causes. First, it helps determine efficiency bottlenecks. Realizing the place your code is sluggish allows you to focus your optimization efforts successfully. Second, profiling can reveal scalability points. As your codebase grows, it might not carry out properly with elevated load or knowledge quantity. Early identification of those points helps make your code extra strong and scalable. Third, profiling can enhance the consumer expertise. Optimized code runs sooner, offering a smoother expertise for customers. Lastly, environment friendly code reduces computational prices. This could result in important financial savings, particularly in large-scale purposes.
Overview of timeit and cProfile
Timeit and cProfile are two of Python’s most generally used profiling instruments. Timeit is a wonderful device for measuring and analyzing the execution time of temporary code segments. It’s straightforward to make use of and a normal library merchandise. However, cProfile is extra complete. It offers detailed data on how lengthy every perform in your code takes to execute. This makes it best for profiling total scripts and figuring out bottlenecks.
Getting Began with timeit
Fundamentals of the timeit Module
The timeit module is constructed into Python and measures the execution time of small code snippets. It’s easy and environment friendly for evaluating totally different strategies of performing the identical process. Through the use of timeit, you possibly can perceive which method is quicker and by how a lot.
Utilizing timeit on the Command Line
You’ll be able to run timeit from the command line to rapidly measure execution occasions. Right here’s a fundamental instance:
python -m timeit -s 'nums = [6, 9, 2, 3, 7]' 'checklist(reversed(nums))'python -m ti
On this command, -s specifies the setup code, and the next argument is the code to be timed. This measures the time taken to reverse an inventory.
Integrating timeit in Python Scripts
Utilizing timeit inside Python scripts can also be straightforward. You’ll be able to import the module and use its capabilities instantly. Right here’s an instance:
mport timeit
setup_code = "nums = [6, 9, 2, 3, 7]"
stmt = "checklist(reversed(nums))"
# Time the execution of the assertion
execution_time = timeit.timeit(stmt, setup=setup_code, quantity=100000)
print(f"Execution time: {execution_time} seconds")
This script occasions the checklist reversal operation 100,000 occasions and prints the overall execution time.
Sensible Examples with timeit
Timing Listing Reversal
Let’s examine two strategies of reversing an inventory: utilizing reversed() and checklist slicing. We’ll use timeit to measure the efficiency of every technique.
import timeit
setup_code = "nums = [6, 9, 2, 3, 7]"
stmt1 = "checklist(reversed(nums))"
stmt2 = "nums[::-1]"
# Timing reversed() technique
time_reversed = timeit.timeit(stmt1, setup=setup_code, quantity=100000)
print(f"Utilizing reversed(): {time_reversed} seconds")
# Timing checklist slicing technique
time_slicing = timeit.timeit(stmt2, setup=setup_code, quantity=100000)
print(f"Utilizing checklist slicing: {time_slicing} seconds")
Working this script will present which technique is quicker. Sometimes, checklist slicing is faster attributable to its simplicity and direct entry in reminiscence.
Utilizing timeit, you may make knowledgeable choices about optimizing small however crucial elements of your code, guaranteeing higher efficiency and effectivity.
Benchmarking Completely different Algorithms
Benchmarking helps examine the efficiency of various algorithms. Utilizing timeit, you possibly can determine essentially the most environment friendly one. Right here’s how one can benchmark sorting algorithms:
import timeit
setup_code = "import random; nums = [random.randint(0, 1000) for _ in range(1000)]"
stmt1 = "sorted(nums)"
stmt2 = "nums.type()"
# Timing sorted() perform
time_sorted = timeit.timeit(stmt1, setup=setup_code, quantity=1000)
print(f"Utilizing sorted(): {time_sorted} seconds")
# Timing type() technique
time_sort = timeit.timeit(stmt2, setup=setup_code, quantity=1000)
print(f"Utilizing type(): {time_sort} seconds")
This script compares the efficiency of Python’s sorted() perform and the checklist’s type() technique on an inventory of 1000 random integers.
Deep Dive into cProfile
Fundamentals of the cProfile Module
cProfile is a built-in Python module that gives detailed statistics about program execution. It measures the time spent in every perform and counts how usually it’s referred to as. This makes it best for profiling total scripts.
Working cProfile from the Command Line
To profile a Python script, you possibly can run cProfile instantly from the command line. Right here’s an instance:
python -m cProfile my_script.py
This command profiles my_script.py and prints an in depth report of perform calls and execution occasions.
Embedding cProfile in Python Scripts
You can even embed cProfile inside your Python scripts. This lets you profile particular sections of your code. Right here’s how:
import cProfile
def my_function():
# Your code right here
go
if __name__ == "__main__":
profiler = cProfile.Profile()
profiler.allow()
my_function()
profiler.disable()
profiler.print_stats(type="time")
Analyzing cProfile Output
cProfile generates detailed output, which could be overwhelming. Understanding the right way to analyze this output is essential for efficient profiling.
Decoding Perform Name Statistics
The cProfile output consists of a number of columns, similar to:
- ncalls: Variety of calls to the perform
- tottime: Complete time spent within the perform
- percall: Time per name
- cumtime: Cumulative time spent within the perform, together with subcalls
- filename:lineno(perform): Location and identify of the perform
Right here’s an instance of the right way to interpret this output:
1000 0.020 0.000 0.040 0.000 {built-in technique builtins.sorted}
1000 0.020 0.000 0.040 0.000 {built-in technique builtins.sorted}
This line signifies that the sorted perform was referred to as 1000 occasions, taking a complete of 0.020 seconds, with a mean of 0.00002 seconds per name.
Utilizing pstats for Detailed Evaluation
The pstats module lets you analyze cProfile output extra successfully. You’ll be able to type and filter profiling statistics to deal with particular areas of your code.
import cProfile
import pstats
def my_function():
# Your code right here
go
if __name__ == "__main__":
profiler = cProfile.Profile()
profiler.allow()
my_function()
profiler.disable()
stats = pstats.Stats(profiler)
stats.sort_stats(pstats.SortKey.TIME)
stats.print_stats()
This script makes use of pstats to type the profiling output by time, it makes it simpler to determine the capabilities that eat essentially the most time.
Through the use of timeit and cProfile, you possibly can achieve priceless insights into your code’s efficiency. These instruments will assist you determine bottlenecks and optimize your code for higher effectivity.
Evaluating timeit and cProfile
When to Use timeit
Use Timeit to measure the execution time of small code snippets or particular person capabilities. It’s best for benchmarking particular elements of your code to check totally different approaches. As an illustration, use timeit to check the efficiency of two totally different sorting algorithms.
Instance:
import timeit
setup_code = "import random; nums = [random.randint(0, 1000) for _ in range(1000)]"
stmt1 = "sorted(nums)"
stmt2 = "nums.type()"
# Timing sorted() perform
time_sorted = timeit.timeit(stmt1, setup=setup_code, quantity=1000)
print(f"Utilizing sorted(): {time_sorted} seconds")
# Timing type() technique
time_sort = timeit.timeit(stmt2, setup=setup_code, quantity=1000)
print(f"Utilizing type(): {time_sort} seconds")
When to Use cProfile
Use cProfile if you want detailed details about the efficiency of your total script. It’s wonderful for figuring out which capabilities eat essentially the most time. That is significantly helpful for bigger initiatives the place you want a complete view of efficiency bottlenecks.
Instance:
import cProfile
def example_function():
# Your code right here
go
if __name__ == "__main__":
profiler = cProfile.Profile()
profiler.allow()
example_function()
profiler.disable()
profiler.print_stats(type="time")
Benefits and Limitations of Every Software
timeit:
- Benefits: Easy to make use of, a part of the usual library, nice for small code snippets.
- Limitations: Not appropriate for profiling total scripts, restricted to timing small sections of code.
cProfile:
- Benefits: Offers detailed perform name statistics, nice for profiling total scripts, helps determine bottlenecks.
- Limitations: Extra complicated to make use of, generates massive output, may add overhead.
Superior Profiling Python Methods
Combining timeit and cProfile
You’ll be able to mix timeit and cProfile to get detailed insights. Use timeit for exact timing and cProfile for complete profiling.
Instance:
import cProfile
import timeit
def example_function():
# Your code right here
go
if __name__ == "__main__":
# Utilizing timeit
setup_code = "from __main__ import example_function"
stmt = "example_function()"
print(timeit.timeit(stmt, setup=setup_code, quantity=1000))
# Utilizing cProfile
profiler = cProfile.Profile()
profiler.allow()
example_function()
profiler.disable()
profiler.print_stats(type="time")
Utilizing Third-Social gathering Profilers
Third-party profilers present further insights and are helpful for particular profiling wants.
line_profiler
line_profiler measures the execution time of particular person traces of code. This helps determine which traces are essentially the most time-consuming.
Instance:
pip set up line_profiler
from line_profiler import LineProfiler
def example_function():
# Your code right here
go
profiler = LineProfiler()
profiler.add_function(example_function)
profiler.enable_by_count()
example_function()
profiler.print_stats()
memory_profiler
memory_profiler tracks reminiscence utilization over time, serving to determine reminiscence leaks and optimize reminiscence utilization.
Instance:
pip set up memory_profiler
from memory_profiler import profile
@profile
def example_function():
# Your code right here
go
if __name__ == "__main__":
example_function()
Save the Script to a File:
Save the next script as memory_profile_example.py:
Run the Script with Reminiscence Profiling. Open your command line or terminal, navigate to the listing the place your script is saved, and run:
python -m memory_profiler memory_profile_example.py
Pyinstrument
Pyinstrument is a statistical profiler that gives a high-level overview of your program’s efficiency.
Instance:
from pyinstrument import Profiler
profiler = Profiler()
profiler.begin()
# Your code right here
example_function()
profiler.cease()
print(profiler.output_text(unicode=True, shade=True))
Suggestions and Greatest Practices for Efficient Profiling Python
Efficient profiling is essential for optimizing your code. Listed below are some ideas and greatest practices that will help you get essentially the most out of profiling.
- Figuring out Efficiency Bottlenecks: To determine efficiency bottlenecks, deal with the elements of your code that eat essentially the most time or assets. Use cProfile to get an in depth breakdown of perform calls and their execution occasions.
- Optimizing Code Primarily based on Profiling Outcomes: When you’ve recognized bottlenecks, optimize these areas. Search for inefficient algorithms, pointless computations, or redundant code.
Avoiding Widespread Pitfalls in Profiling Python
Keep away from these widespread pitfalls to make sure correct profiling outcomes:
- Profiling in Improvement Mode: Be certain that your surroundings displays the manufacturing setup.
- Small Enter Sizes: Use real looking knowledge sizes to get significant profiling outcomes.
- Ignoring Overheads: Remember that profiling provides overhead. Use instruments like pstats to attenuate this impact.
Conclusion
Profiling is a vital method for making your Python code extra environment friendly. Realizing the worth of profiling, using timeit and cProfile, and adhering to really helpful practices can drastically enhance your code’s efficiency. Common profiling assists in finding and resolving bottlenecks to make sure your purposes function successfully and effectively. As your codebase expands and adjustments, embrace profiling Python into your improvement course of to make sure peak efficiency.
Checkout our Introduction to Python Program to grasp Python!
[ad_2]