Python Database Fundamentals | Developer.com
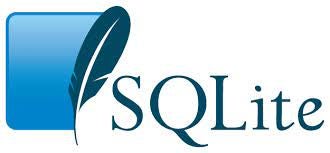
Databases are an vital a part of most fashionable software program improvement. They function a repository for storing, organizing, manipulating, and retrieving information and knowledge. Python, being a flexible programming language, provides a number of modules and libraries for working with databases. We are going to discover the basics of database programming in Python, with a concentrate on utilizing the SQLite database system, which is light-weight, straightforward to make use of, and a part of the Python commonplace library.
Soar to:
Introduction to SQLite
Databases could be regarded as a structured assortment of knowledge that’s organized in such a way that functions can shortly choose and retrieve particular items of knowledge which might be usually associated to at least one one other (however not all the time). Databases are obligatory for storing and managing information in functions, together with small scripts and even large-scale, data-driven internet functions.
SQLite is a C library that capabilities as a disk-based database. In contrast to most different database administration methods (DBMS), SQLite doesn’t require a separate server course of. As well as, SQLite supplies entry to the database utilizing a nonstandard variant of the structured question language (SQL). It’s a nice choice for embedded methods, testing, and small to medium-sized functions.
SQLite is an ideal database to start out with for freshmen because of its simplicity, straightforward configuration, and minimal setup necessities. It’s a Serverless database, which implies builders don’t must arrange a separate server to make use of it. As well as, SQLite databases are saved in a single file; this makes them straightforward to share and transfer between completely different methods. Beneath, we stroll via the fundamentals of working with SQLite utilizing Python, opening doorways for extra superior database ideas down the road.
Learn: 10 Finest Python Certifications
Methods to Set Up the Dev Setting
Earlier than we start, we now have to first make sure that Python is put in in your pc. To take action, open a terminal or command immediate and kind:
python --version
If Python isn’t put in, you have to to obtain and set up it from the official Python web site. You can even learn to set up Python in our tutorial: Methods to Set up Python.
Putting in SQLite
Python comes with the sqlite3 module, which supplies an interface to the SQLite database. Programmers don’t want to put in something additional to work with SQLite in Python.
Connecting to a Database
As said, the sqlite3 module is a part of the Python commonplace library and supplies a strong set of instruments for working with SQLite databases. Earlier than we are able to use it, we should import the module into our Python scripts. We are able to achieve this within the following method:
import sqlite3
Establishing a Database Connection in Python
With the intention to work together with an SQLite database, programmers must first set up a database connection. This may be achieved utilizing the join operate contained within the sqlite3 module. Word that if the famous database file doesn’t exist, SQLite will create it.
# Hook up with the named database (or, if it doesn't exist, create one) conn = sqlite3.join('pattern.db')
Making a Cursor in SQLite
With the intention to execute database queries and retrieve ends in an SQLite database, you have to first create a cursor object. This course of happens after you create your connection object.
# Methods to create a cursor object with a purpose to execute SQL queries cursor = conn.cursor()
Making a Desk
In relational database administration methods (RDBMS), information is organized into tables, every of which is made up of rows (horizontal) and columns (vertical). A desk represents a selected idea, and columns outline the attributes of that idea. As an example, a database would possibly maintain details about autos. The columns inside that desk is likely to be labeled make, sort, yr, and mannequin. The rows, in the meantime, would maintain information factors that aligned with every of these columns. As an example, Lincoln, automotive, 2023, Nautilus.
Learn: PyCharm IDE Evaluation
Methods to Construction Knowledge with SQL
SQL is the usual language for working inside relational databases. SQL supplies instructions for information and database manipulation that embrace creating, retrieving, updating, and deleting information. To create a desk, database builders use the CREATE TABLE assertion.
Beneath, we create a easy desk to retailer details about college students, together with their student_id, full_name, and age:
# Create a desk cursor.execute(''' CREATE TABLE IF NOT EXISTS college students ( student_id INTEGER PRIMARY KEY, full_name TEXT NOT NULL, age INTEGER NOT NULL ) ''') # Commit our adjustments conn.commit()
Within the above code snippet, CREATE TABLE defines the desk title, column names, and their respective information varieties. The PRIMARY KEY of the student_id column is used to make sure that every id worth is exclusive, as main values should all the time be distinctive.
If we want to add information to a desk, we are able to use the INSERT INTO assertion. This assertion lets builders specify which desk and column(s) to insert information into.
Inserting Knowledge right into a Desk
Beneath is an instance of the right way to insert information into an SQLite database with the SQL command INSERT INTO:
# Insert information into our desk cursor.execute("INSERT INTO college students (full_name, age) VALUES (?, ?)", ('Ron Doe', 49)) cursor.execute("INSERT INTO college students (full_name, age) VALUES (?, ?)", ('Dana Doe', 49)) # Commit adjustments conn.commit()
On this code instance, we used parameterized queries to insert information into our college students desk. The values are tuples, which helps forestall SQL injection assaults, improves code readability, and is taken into account a greatest apply.
Methods to Question Knowledge in SQLite
The SQL SELECT assertion is used after we need to question information from a given desk. It permits programmers to specify which columns they need to retrieve, filter rows (primarily based on standards), and type any outcomes.
Methods to Execute Database Queries in Python
To execute a question in Python, you should utilize the execute technique on a cursor object, as proven within the instance SQL assertion:
# Methods to question information cursor.execute("SELECT * FROM college students") rows = cursor.fetchall()
The fetchall technique within the code above retrieves each row from the final question that was executed. As soon as retrieved — or fetched — we are able to then iterate over our question outcomes and show the information:
# Show the outcomes of our question for row in rows: print(row)
Right here, we print the information saved within the college students desk. We are able to customise the SELECT assertion to retrieve particular columns if we would like, or filter outcomes primarily based on situations and standards as nicely.
Updating and Deleting Knowledge in SQLite
There are occasions after we will need to replace current information. On these events, we’ll use the UPDATE assertion. If we need to delete information, we’d use the DELETE FROM assertion as an alternative. To start, we’ll replace the age of our pupil with the title ‘Ron Doe’:
# Updating our information cursor.execute("UPDATE college students SET age=? WHERE title=?", (50, 'Ron Doe')) # Commit our adjustments conn.commit()
On this code, we up to date Ron Doe’s age from 49 to 50.
However what if we needed to delete a report? Within the beneath instance, we’ll delete the report for the coed named Dana Doe:
# Deleting a report cursor.execute("DELETE FROM college students WHERE title=?", ('Dana Doe',)) # Commit our adjustments conn.commit()
Finest Practices for Working With Databases in Python
Beneath we spotlight some greatest practices and ideas for working with databases in Python, together with:
- Use parameterized queries
- Use exception dealing with
- Shut database connections
Use Parameterized Queries
Builders and database directors ought to all the time use parameterized queries with a purpose to forestall SQL injection assaults. Parameterized queries are safer as a result of they separate SQL code from information, decreasing the danger of malicious actors. Right here is an instance of the right way to use parameterized queries:
# Methods to use parameterized queries cursor.execute("INSERT INTO college students (full_name, age) VALUES (?, ?)", ('Ron Die', 49))
Use Exception Dealing with
Programmers ought to all the time encase database operations in try-except blocks to deal with potential errors gracefully. Some widespread exceptions embrace sqlite3.OperationalError and sqlite3.IntegrityError.
strive: # Database operation instance besides sqlite3.Error as e: print(f" The SQLite error reads: {e}")
Shut Database Connections
Finest database practices name for builders to all the time shut database connections and cursors if you end up completed working with databases. This makes positive that sources are launched and pending adjustments are dedicated.
# Methods to shut the cursor and database connection cursor.shut() conn.shut()
Ultimate Ideas on Python Database Fundamentals
On this database programming and Python tutorial, we coated the fundamentals of working with databases in Python utilizing SQLite. We discovered how to connect with a database, create tables, and insert, question, replace, and delete information. We additionally mentioned greatest practices for working with databases, which included utilizing parameterized queries, dealing with exceptions, and shutting database connections.
Wish to learn to work with Python and different database methods? Try our tutorial on Python Database Programming with MongoDB.