[ad_1]
Introduction
String matching in Python may be difficult, however Pregex makes it simple with its easy and environment friendly pattern-matching capabilities. On this article, we’ll discover how Pregex may also help you discover patterns in textual content effortlessly. We’ll cowl the advantages of utilizing Pregex, a step-by-step information to getting began, sensible examples, suggestions for environment friendly string matching, integration with different Python libraries, and finest practices to comply with. Whether or not you’re a newbie or an skilled programmer, Pregex can simplify your string-matching duties and improve your Python tasks.
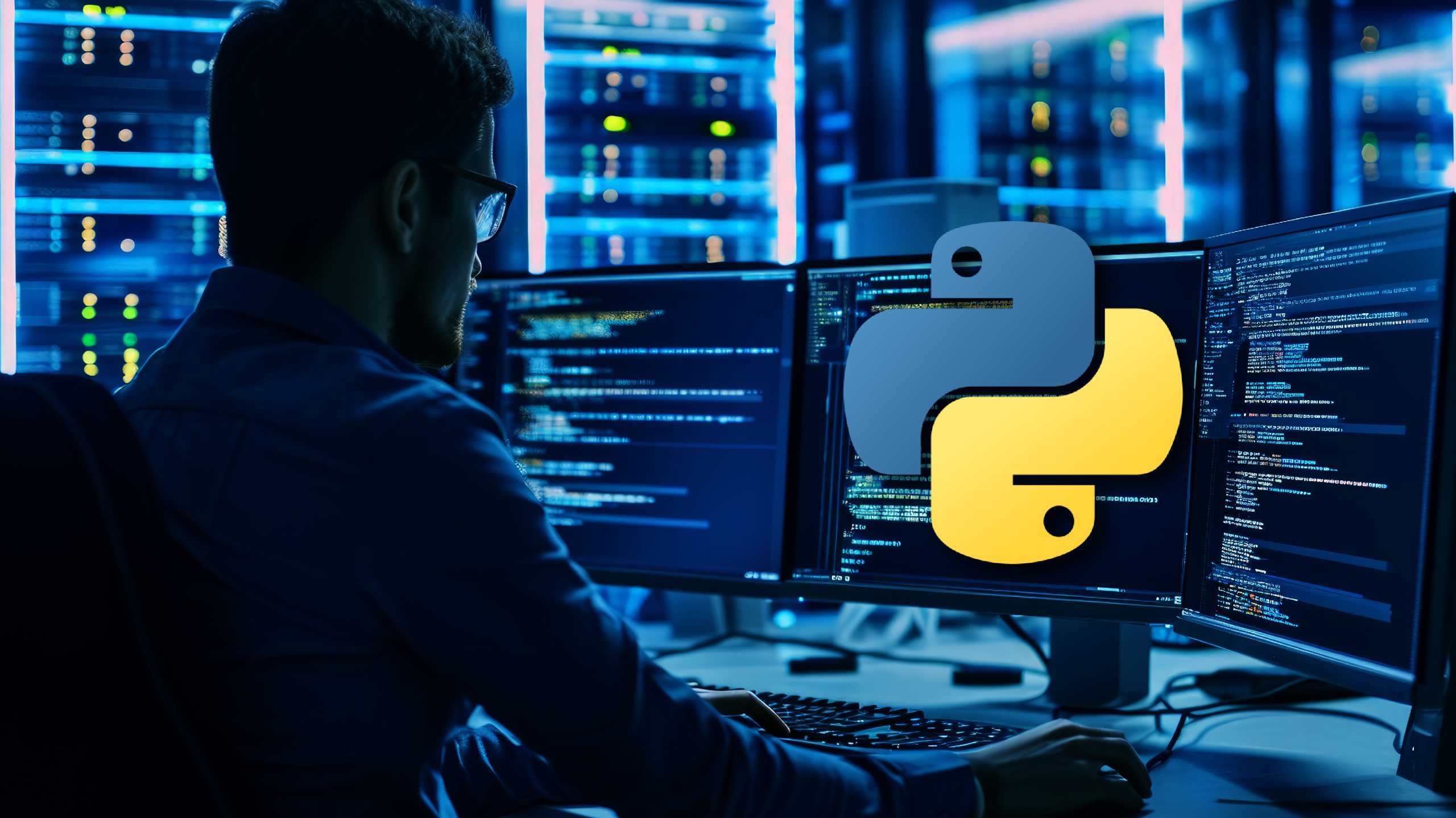
Advantages of Utilizing Pregex for String Matching
Pregex is a Python utility that simplifies the method of figuring out patterns in textual content with out requiring data of advanced programming. As a result of it simplifies and manages the code, Pregex advantages novice and seasoned programmers. Pregex makes organising and making use of patterns simple, accelerating improvement and reducing error charges. Moreover, this accessibility facilitates faster code updates and debugging, sustaining tasks’ flexibility and effectivity.
Getting Began with Pregex in Python
You should first set up the library to begin utilizing Pregex in your Python undertaking. You possibly can simply set up Pregex utilizing pip:
pip set up pregex
Primary Sample Matching
After you have put in Pregex, you need to use it to do primary sample matching. For instance, to test if a string incorporates a selected phrase, you need to use the next code:
from pregex.core.pre import Pregex
textual content = "Hey, World!"
sample = Pregex("Hey")
consequence = sample.get_matches(textual content)
if consequence:
print("Sample discovered!")
else:
print("Sample not discovered.")
Output: Sample discovered!
Rationalization
- Import the Pregex class from the pregex.core.pre module.
- Outline the textual content to look:
- textual content = “Hey, World!”: That is the textual content during which we need to discover the sample.
- Create a sample:
- sample = Pregex(“Hey”): This creates a Pregex object with the sample “Hey”.
- Discover matches:
- consequence = sample.get_matches(textual content): This makes use of the get_matches methodology to seek out occurrences of the sample “Hey” within the textual content.
- Test and print outcomes:
- The if assertion checks if any matches have been discovered.
- If matches are discovered, it prints “Sample discovered!”.
- If no matches are discovered, it prints “Sample not discovered.”
Superior Sample Matching Methods
Pregex additionally helps superior pattern-matching strategies equivalent to utilizing anchors, quantifiers, grouping, and capturing matches. These strategies let you create extra advanced patterns for matching strings.
Examples of String Matching with Pregex
Matching E mail Addresses
textual content="Hey there, [email protected]"
from pregex.core.courses import AnyButFrom
from pregex.core.quantifiers import OneOrMore, AtLeast
from pregex.core.assertions import MatchAtLineEnd
consumer = OneOrMore(AnyButFrom("@", ' '))
firm = OneOrMore(AnyButFrom("@", ' ', '.'))
area = MatchAtLineEnd(AtLeast(AnyButFrom("@", ' ', '.'), 3))
pre = (
consumer +
"@" +
firm +
'.' +
area
)
outcomes = pre.get_matches(textual content)
print(outcomes)
Output: [‘[email protected]’]
Rationalization
- Import obligatory Pregex courses:
- AnyButFrom: Matches any character besides these specified.
- OneOrMore: Matches a number of occurrences of the previous factor.
- AtLeast: Matches at the very least a specified variety of occurrences of the previous factor.
- MatchAtLineEnd: Asserts that the next sample have to be on the finish of the road.
- Outline patterns for e mail components:
- consumer: Matches the half earlier than the “@” image (OneOrMore(AnyButFrom(“@”, ‘ ‘))).
- firm: Matches the half between the “@” image and the final dot (OneOrMore(AnyButFrom(“@”, ‘ ‘, ‘.’))).
- area: Matches the half after the final dot (MatchAtLineEnd(AtLeast(AnyButFrom(“@”, ‘ ‘, ‘.’), 3))).
- Mix the patterns:
- Concatenate consumer, “@”, firm, and area to kind the entire e mail sample.
- Discover matches within the textual content:
- Use the get_matches methodology to seek out and print any e mail addresses within the textual content.
Extracting URLs, Figuring out Cellphone Numbers, and Parsing Knowledge from Textual content may be completed equally utilizing Pregex.
Additionally Learn: Introduction to Strings in Python For Newbies
Ideas for Environment friendly String Matching with Pregex
Utilizing Anchors and Quantifiers, Grouping and Capturing Matches, Dealing with Particular Characters, and Efficiency Optimization are important for environment friendly string matching with Pregex.
Integrating Pregex with Different Python Libraries
Pregex may be seamlessly built-in with different Python libraries, equivalent to Pandas, Common Expressions, and NLP libraries, to reinforce its performance and utility in numerous functions.
Greatest Practices for String Matching with Pregex
Writing clear and concise patterns, testing and validating patterns, and error dealing with and exception administration are a number of the finest practices to comply with when working with Pregex for string matching.
Additionally Learn: String Knowledge Construction in Python | Full Case research
Conclusion
In conclusion, Pregex is a priceless instrument for string matching in Python, providing an easier and extra intuitive method than conventional common expressions. By following the information and finest practices outlined on this article, you’ll be able to leverage Pregex’s energy to match strings in your Python tasks effectively. So, give Pregex a attempt to streamline your string-matching duties immediately!
For extra articles on Python, discover our article part immediately.
[ad_2]