[ad_1]
Introduction
When you’ve been studying Python, I’m certain you may have come throughout the time period ‘self.’ This small phrase performs an enormous position in how Python courses work. Everytime you create a technique inside a category, you employ ‘self’ to confer with the occasion that calls the tactic. This helps you entry and modify the occasion variables and strategies inside the class. On this article, we’ll dive deep into what self means, why it’s necessary, and discover the way it’s utilized in Python programming.
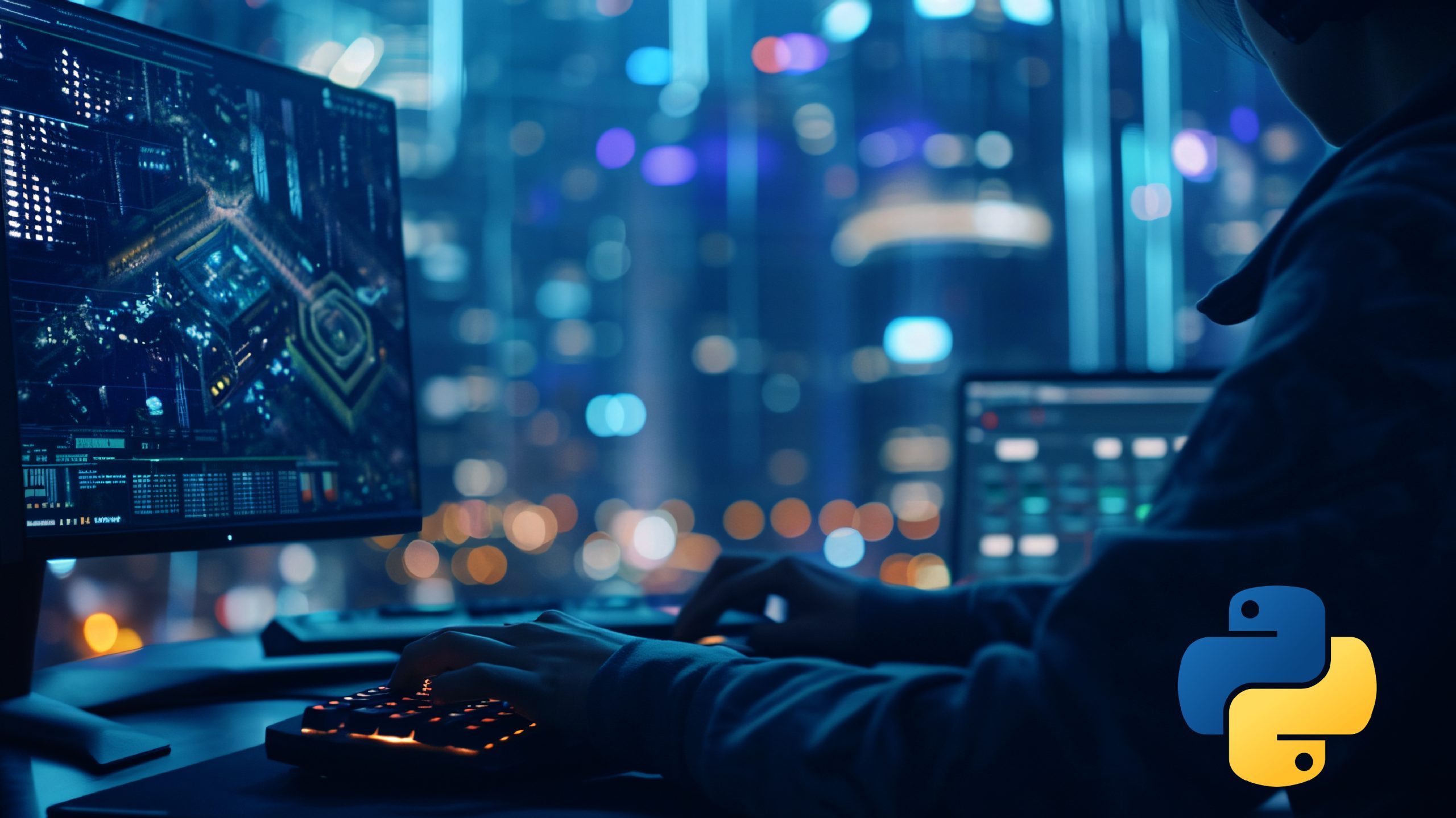
Overview
- Perceive the which means and significance of ‘self’ in Python class.
- Discover ways to use ‘self’ in Python.
- Discover out what occurs while you don’t use ‘self’ in Python.
What Does `Self` Imply in Python?
In Python, ‘self` is a reference to the occasion of the category. Python makes use of the time period `’self’` to confer with the occasion that’s calling the category. In a category, it’s used to name up the occasion variables and strategies. The next is an evidence of the time period ‘self’`:
- Occasion Reference: `self` is a traditional identify for the primary parameter of strategies in a category. This time period additionally stands for the category object that calls that technique.
- Occasion Variables: It’s used to entry occasion variables and strategies from inside class strategies.
- Methodology Definition: `self` is the primary parameter of an occasion technique in Python. Thus any new user-defined technique in a category could have `self` argument to indicate the occasion it refers to.
Why is `Self` Vital?
Let’s now attempt to perceive the significance of ‘self’ in Python.
1. Entry to Occasion Variables
`Self` permits you to entry and modify occasion variables inside class strategies.
Instance :
class Particular person:
def __init__(self, identify, age):
self.identify = identify # self.identify is an occasion variable
self.age = age # self.age is an occasion variable
def greet(self):
print(f"Hey, my identify is {self.identify} and I'm {self.age} years outdated.")
2. Entry to Different Strategies
`Self` permits strategies inside the identical class to name different strategies.
Instance :
class Circle:
def __init__(self, radius):
self.radius = radius
def space(self):
return 3.14 * self.radius * self.radius
def perimeter(self):
return 2 * 3.14 * self.radius
def describe(self):
print(f"Circle with radius {self.radius}, space: {self.space()}, perimeter: {self.perimeter()}")
3. Distinguishing Between Class and Occasion Variables
Utilizing `self` helps distinguish between occasion variables and native variables inside strategies.
class Instance:
def __init__(self, worth):
self.worth = worth # occasion variable
def set_value(self, worth):
self.worth = worth # right here self.worth is the occasion variable, and worth is the native variable
Can One other Phrase Be Used As a substitute of `Self`?
Certainly, `self` may be changed with any legitimate variable identify. Nonetheless, that is dangerous since `self` is a widely known conference. It’s extremely discouraged when people come throughout a brand new identify, they could develop into puzzled and disoriented, inflicting a possible downside. Additionally, utilizing a unique identify may confuse different programmers who learn your code.
class Particular person:
def __init__(myself, identify, age):
myself.identify = identify
myself.age = age
def greet(myself):
print(f"Hey, my identify is {myself.identify} and I'm {myself.age} years outdated.")
On this instance, `myself` is used as an alternative of `self`, and it really works completely tremendous, however it isn’t really useful.
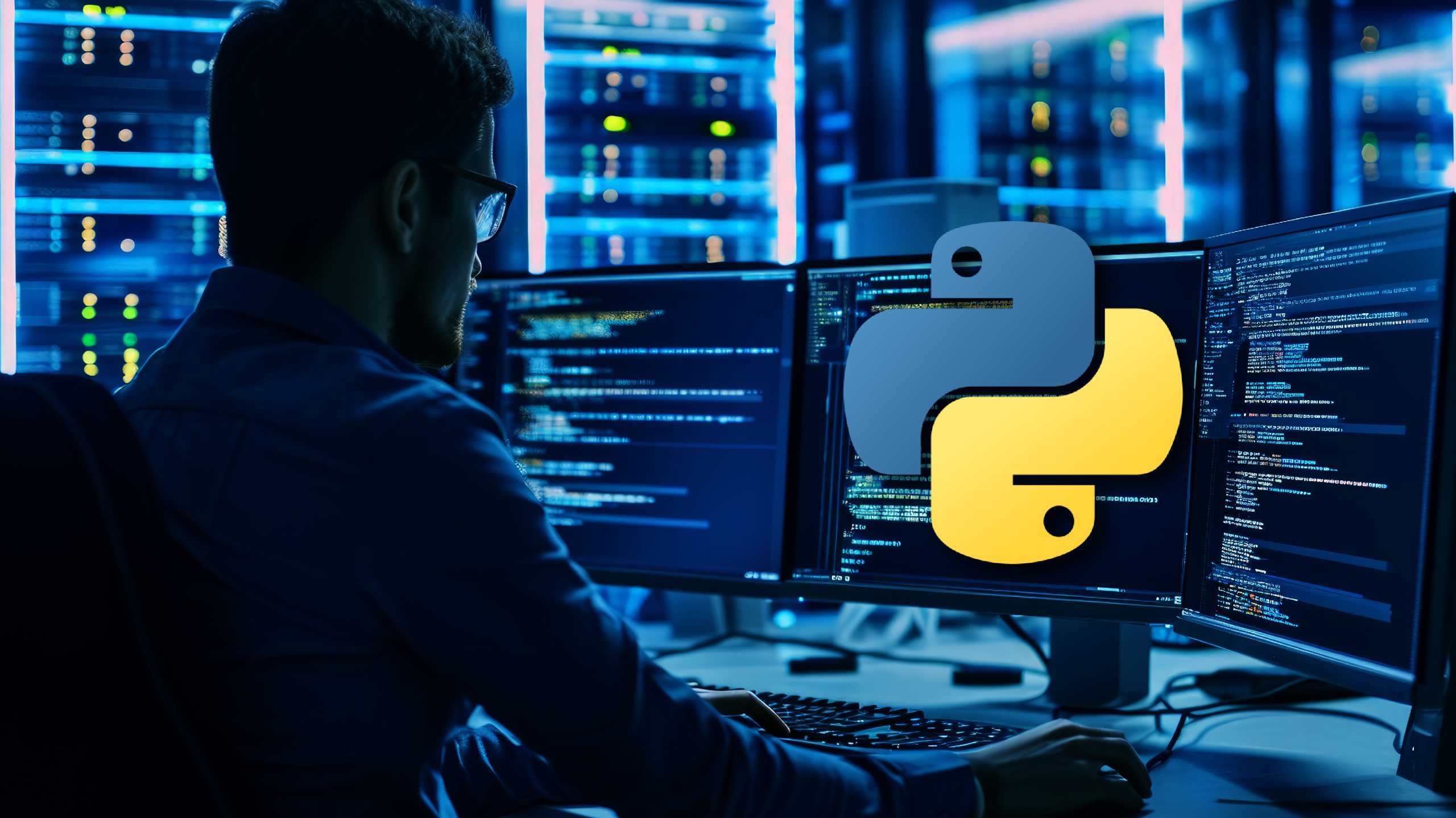
What Occurs If ‘Self` is Not Used?
If you don’t embrace `self` (or an equal first parameter) in your technique definitions, you’ll encounter errors while you attempt to name these strategies. It is because Python robotically passes the occasion as the primary argument to occasion strategies, and in case your technique signature doesn’t count on it, it should end in a `TypeError`.
class Particular person:
def __init__(identify, age): # Incorrect: lacking 'self'
identify = identify
age = age
def greet(): # Incorrect: lacking 'self'
print(f"Hey, my identify is {identify} and I'm {age} years outdated.")
# This may increase an error while you attempt to create an occasion
p = Particular person("Alice", 30)
Error:
TypeError: __init__() takes 2 positional arguments however 3 got.
Conclusion
`Self` is important for accessing occasion variables and strategies inside a category. It permits for object-oriented programming practices and ensures that strategies function on the proper cases. This provides you the facility to construct complicated, reusable, and modular code. Whilst you can technically use one other identify as an alternative of `self`, it isn’t really useful as a result of conventions and readability. Additionally do not forget that not utilizing `self` the place required will end in errors, disrupting the sleek operation of your code. Embrace ‘self’, and also you’ll discover your Python programming turns into extra intuitive and efficient.
Regularly Requested Questions
A. In Python, ‘self` is a reference to the occasion of the category. Python makes use of the time period `’self’` to confer with the occasion that’s calling the category. And in a category, it’s used to name up the occasion variables and strategies.
A. In Python, ‘self
‘ refers back to the occasion of the category and is used to entry its attributes and strategies. In the meantime, the __init__
technique is a particular constructor technique that initializes the article’s attributes when a brand new occasion of the category is created.
A. The ‘self’ key in Python is used to characterize the occasion of a category. With this key phrase, you may entry the attributes and strategies of a specific Python class.
[ad_2]