[ad_1]
Introduction
This text gives an in depth information on automating e-mail sending utilizing Python‘s `smtplib` library. It covers establishing an setting, producing pattern information, and creating primary line plots. It additionally discusses superior customization choices like line types, colours, and markers for visually interesting visualizations. The information additionally teaches the right way to annotate plots and save them as picture recordsdata. This text is appropriate for these new to Python or seeking to improve information visualization expertise.
Overview
- Learn to set up needed libraries and arrange Python for e-mail automation.
- Perceive the right way to use smtplib to ship emails to a single recipient, together with authentication and session administration.
- Discover strategies for sending emails to a number of recipients utilizing Python and smtplib.
- Discover customization choices for e-mail content material and formatting, and learn to deal with exceptions and errors in e-mail sending.
Why Use Python for Sending Emails?
Python is straightforward and its readability makes it a wonderful selection for automation which incorporates sending emails. When we have to ship common updates, notification or advertising and marketing emails, Python could make this course of time saving.
For sending emails with Python we can be utilizing SMTP which additionally stands for Easy Mail Switch Protocol. This protocol is used to ship emails throughout the Web. This Library “smtplib” creates a shopper session object which can be utilized to ship to any legitimate E mail ID . The SMTP server operates on port 25, however for safe transmission, port 587 is used.
Earlier than beginning, you will need to have Python already put in in your system. And also you want entry to an e-mail account with SMTP credentials. Many of the e-mail suppliers give these credentials equivalent to Gmail or Outlook.
Let’s see the steps of this now:
- First, you have to import the smtplib library.
- Now create a session.
- On this, you have to go the parameter of the server location and the port the primary parameter must be the server location after which port. For Gmail , we use port quantity 587.
- for safety objective, now put the SMTP connection inTSL(Transport Layer Safety) mode. After this , for safety and authentication, you have to go your Gmail account credentials within the login occasion.
- Now retailer the message you have to ship in variable, message and utilizing the sendmail() occasion ship your message with the three parameters within the sequence.
Ship E mail Single Recipient
Ship e-mail out of your to single Recipient’s account utilizing Python.
With Python, you might use the smtplib bundle to ship an e-mail to a single recipient. This library lets you ship emails by way of the SMTP. This methodology works nicely for automating sending alerts, notifications, or custom-made messages. To ship an e-mail to a single recipient, authenticate, and arrange an SMTP session, see the next snippet of code.
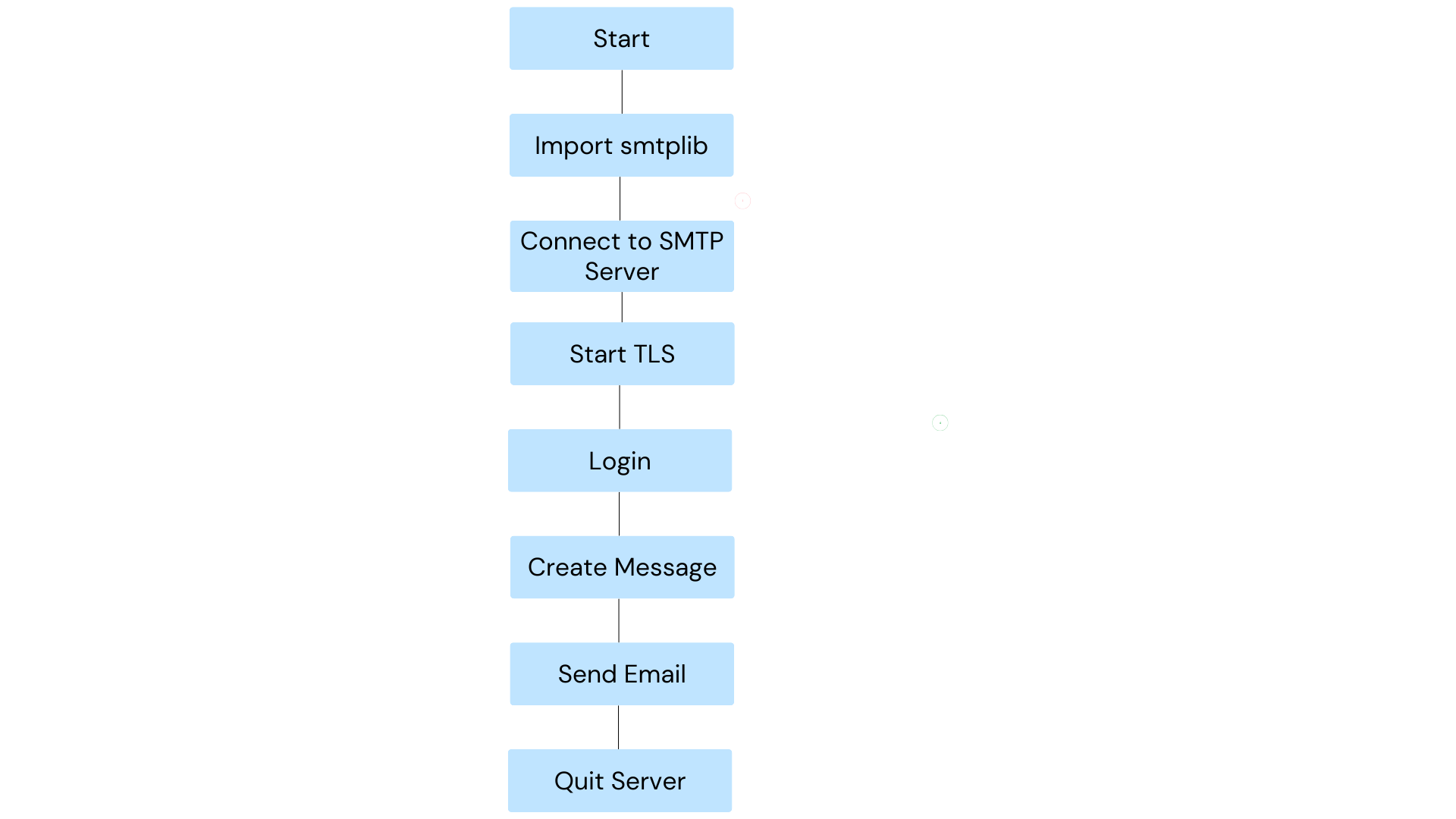
#import csvimport smtplib
server= smtplib.SMTP(‘smtp.gmail.com’,587)
server.starttls()
server.login(‘sender_email_id’,’sender_email_password”)
message=”Message to be despatched”
server.sendmail(‘sender_email_id”,”receiver_email”,message)
server.stop()
Ship E mail to A number of Recipients
Now, Let’s see the right way to Ship e-mail to A number of Recipients utilizing Python.
If the identical e-mail must be ship to totally different particular person. For loop can be utilized for that. Let’s see this with an instance
import smtplib
list_of_email=[‘[email protected]’,’[email protected]’]
for i in list_of_email:
server= smtplib.SMTP(‘smtp.gmail.com’,587)
server.starttls()
server.login(‘sender_email_id’,’sender_email_password”)
message=”Message to be despatched”
server.sendmail(‘sender_email_id”,i,message)
server.stop()
Sending e-mail with Attachment from Gmail Account
Now we are going to discover the code on how we will ship e-mail with attachment from Gmail account.
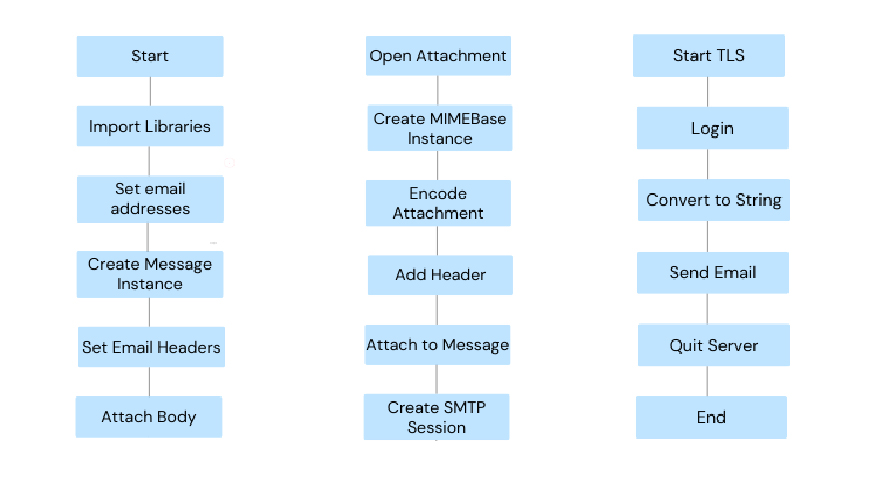
#Libraries to import
import smtplib
from e-mail.mime.textual content import MIMEText
from e-mail.mime.multipart import MIMEMultipart
from e-mail.mime.base import MIMEBase
from e-mail import encoders
from_email_add= “E mail ID of sender”
to_email_add=”E mail ID of receiver”
#occasion of MIMEMultipart
msg= MIMEMultipart()
msg[‘from’]=from_email_add
msg[‘to’]=to_email_add
msg[‘subject’]=”Topic of the mail”
physique=”Physique of the mail”
#connect the physique with the msg occasion
msg.connect(MIMEText(physique,’plain’))
#open the file to be despatched
filename=”file_with_the_extension”
attachment=open(“Path of the file”,”rb”)
#occasion of MIMEBase and named as server
q=MIMEBase(‘software’,’octet-stream’)
#To alter the payload into encoded type
q.set_payload((attachment).learn())
#encode into base64
encoders.encode_base64(server)
q.add_header(‘Content material-Disposition’,’attachment; filename=%s” % filename)
#connect the occasion ‘server’ to occasion ‘msg’
msg.connect(q)
#creates SMTP session
server= smtplib.SMTP(‘smtp.gmail.com’,587)
server.starttls()
server.login(from_email_add,”Password of the sender”)
#Changing the Multipart msg right into a string
textual content=msg.as_string()
#sending the mail
server.sendmail(from_email_add,to_email_add,textual content)
#terminate the session
server.stop()
On this as nicely you need to use loop for sending it to a number of individuals. This code won’t work it two step verification on you Gmail account is enabled
Conclusion
Automating e-mail sending duties is straightforward and environment friendly with Python’s smtplib bundle. Python’s SMTP protocol and ease of use make it a versatile choice for sending messages to a number of recipients, in addition to together with attachments. Python is a good instrument for a wide range of purposes, from advertising and marketing campaigns to notifications, because it streamlines communication procedures and saves time when automating e-mail actions.
Continuously Requested Questions
smtplib
in Python?
A. The smtplib Python library is used to ship emails utilizing SMTP. It gives a handy solution to ship emails programmatically out of your Python software.
A. SMTP credentials are the username and password used to authenticate and hook up with an SMTP server. You want these credentials to ship emails by way of SMTP.
smtplib
?
A. It’s attainable to ship emails with attachments utilizing smtplib. You’ll be able to connect recordsdata to e-mail messages by attaching them to MIMEMultipart messages after encoding them as Base64 strings.
[ad_2]