Take a look at-Driving HTML Templates
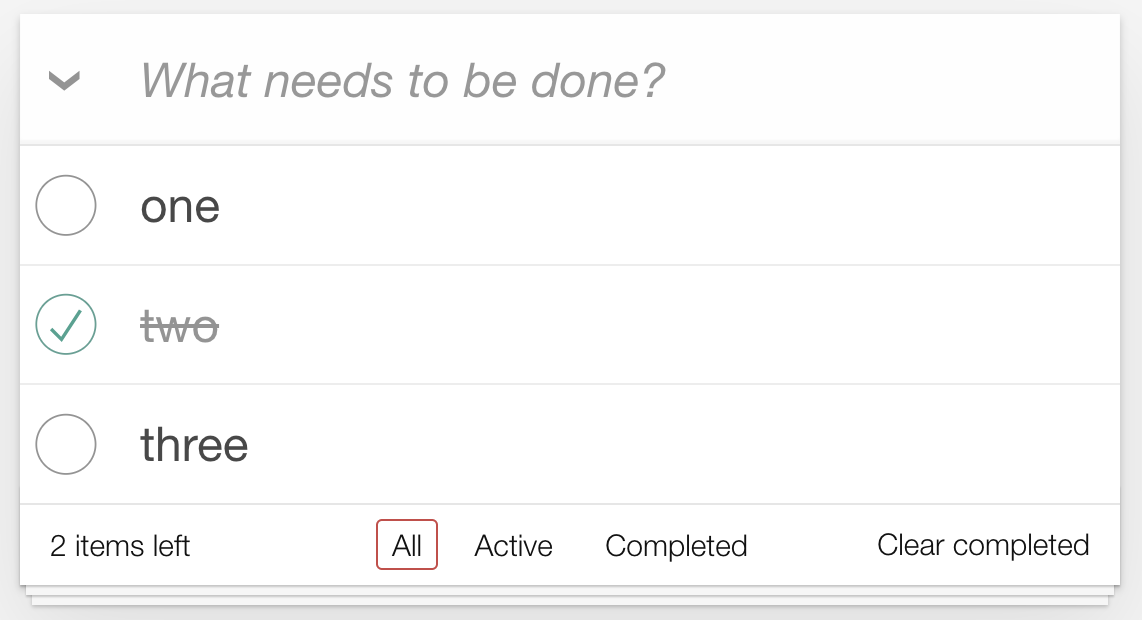
After a decade or extra the place Single-Web page-Functions generated by
JavaScript frameworks have
develop into the norm, we see that server-side rendered HTML is turning into
common once more, additionally because of libraries akin to HTMX or Turbo. Writing a wealthy internet UI in a
historically server-side language like Go or Java is no longer simply potential,
however a really engaging proposition.
We then face the issue of how you can write automated assessments for the HTML
components of our internet purposes. Whereas the JavaScript world has advanced highly effective and refined methods to check the UI,
ranging in dimension from unit-level to integration to end-to-end, in different
languages we would not have such a richness of instruments obtainable.
When writing an online utility in Go or Java, HTML is usually generated
by way of templates, which include small fragments of logic. It’s definitely
potential to check them not directly by way of end-to-end assessments, however these assessments
are gradual and costly.
We will as an alternative write unit assessments that use CSS selectors to probe the
presence and proper content material of particular HTML components inside a doc.
Parameterizing these assessments makes it straightforward so as to add new assessments and to obviously
point out what particulars every check is verifying. This strategy works with any
language that has entry to an HTML parsing library that helps CSS
selectors; examples are supplied in Go and Java.
Stage 1: checking for sound HTML
The primary factor we need to verify is that the HTML we produce is
principally sound. I do not imply to verify that HTML is legitimate based on the
W3C; it might be cool to do it, but it surely’s higher to start out with a lot less complicated and quicker checks.
For example, we would like our assessments to
break if the template generates one thing like
<div>foo</p>
Let’s have a look at how you can do it in phases: we begin with the next check that
tries to compile the template. In Go we use the usual html/template
package deal.
Go
func Test_wellFormedHtml(t *testing.T) { templ := template.Should(template.ParseFiles("index.tmpl")) _ = templ }
In Java, we use jmustache
as a result of it is quite simple to make use of; Freemarker or
Velocity are different widespread selections.
Java
@Take a look at void indexIsSoundHtml() { var template = Mustache.compiler().compile( new InputStreamReader( getClass().getResourceAsStream("/index.tmpl"))); }
If we run this check, it would fail, as a result of the index.tmpl
file does
not exist. So we create it, with the above damaged HTML. Now the check ought to go.
Then we create a mannequin for the template to make use of. The appliance manages a todo-list, and
we will create a minimal mannequin for demonstration functions.
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Should(template.ParseFiles("index.tmpl"))
mannequin := todo.NewList()
_ = templ
_ = mannequin
}
Java
@Take a look at
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var mannequin = new TodoList();
}
Now we render the template, saving the leads to a bytes buffer (Go) or as a String
(Java).
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Should(template.ParseFiles("index.tmpl"))
mannequin := todo.NewList()
var buf bytes.Buffer
err := templ.Execute(&buf, mannequin)
if err != nil {
panic(err)
}
}
Java
@Take a look at
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var mannequin = new TodoList();
var html = template.execute(mannequin);
}
At this level, we need to parse the HTML and we anticipate to see an
error, as a result of in our damaged HTML there’s a div
factor that
is closed by a p
factor. There may be an HTML parser within the Go
customary library, however it’s too lenient: if we run it on our damaged HTML, we do not get an
error. Fortunately, the Go customary library additionally has an XML parser that may be
configured to parse HTML (because of this Stack Overflow reply)
Go
func Test_wellFormedHtml(t *testing.T) {
templ := template.Should(template.ParseFiles("index.tmpl"))
mannequin := todo.NewList()
// render the template right into a buffer
var buf bytes.Buffer
err := templ.Execute(&buf, mannequin)
if err != nil {
panic(err)
}
// verify that the template may be parsed as (lenient) XML
decoder := xml.NewDecoder(bytes.NewReader(buf.Bytes()))
decoder.Strict = false
decoder.AutoClose = xml.HTMLAutoClose
decoder.Entity = xml.HTMLEntity
for {
_, err := decoder.Token()
swap err {
case io.EOF:
return // We're achieved, it is legitimate!
case nil:
// do nothing
default:
t.Fatalf("Error parsing html: %s", err)
}
}
}
This code configures the HTML parser to have the appropriate degree of leniency
for HTML, after which parses the HTML token by token. Certainly, we see the error
message we wished:
--- FAIL: Test_wellFormedHtml (0.00s) index_template_test.go:61: Error parsing html: XML syntax error on line 4: sudden finish factor </p>
In Java, a flexible library to make use of is jsoup:
Java
@Take a look at
void indexIsSoundHtml() {
var template = Mustache.compiler().compile(
new InputStreamReader(
getClass().getResourceAsStream("/index.tmpl")));
var mannequin = new TodoList();
var html = template.execute(mannequin);
var parser = Parser.htmlParser().setTrackErrors(10);
Jsoup.parse(html, "", parser);
assertThat(parser.getErrors()).isEmpty();
}
And we see it fail:
java.lang.AssertionError: Anticipating empty however was:<[<1:13>: Unexpected EndTag token [</p>] when in state [InBody],
Success! Now if we copy over the contents of the TodoMVC
template to our index.tmpl
file, the check passes.
The check, nevertheless, is simply too verbose: we extract two helper capabilities, in
order to make the intention of the check clearer, and we get
Go
func Test_wellFormedHtml(t *testing.T) { mannequin := todo.NewList() buf := renderTemplate("index.tmpl", mannequin) assertWellFormedHtml(t, buf) }
Java
@Take a look at void indexIsSoundHtml() { var mannequin = new TodoList(); var html = renderTemplate("/index.tmpl", mannequin); assertSoundHtml(html); }
Stage 2: testing HTML construction
What else ought to we check?
We all know that the appears to be like of a web page can solely be examined, in the end, by a
human taking a look at how it’s rendered in a browser. Nevertheless, there’s typically
logic in templates, and we would like to have the ability to check that logic.
One could be tempted to check the rendered HTML with string equality,
however this system fails in observe, as a result of templates include a variety of
particulars that make string equality assertions impractical. The assertions
develop into very verbose, and when studying the assertion, it turns into tough
to know what it’s that we’re attempting to show.
What we want
is a way to say that some components of the rendered HTML
correspond to what we anticipate, and to ignore all the main points we do not
care about. A method to do that is by working queries with the CSS selector language:
it’s a highly effective language that permits us to pick out the
components that we care about from the entire HTML doc. As soon as we’ve
chosen these components, we (1) depend that the variety of factor returned
is what we anticipate, and (2) that they include the textual content or different content material
that we anticipate.
The UI that we’re imagined to generate appears to be like like this:
There are a number of particulars which are rendered dynamically:
- The variety of gadgets and their textual content content material change, clearly
- The type of the todo-item modifications when it is accomplished (e.g., the
second) - The “2 gadgets left” textual content will change with the variety of non-completed
gadgets - One of many three buttons “All”, “Energetic”, “Accomplished” shall be
highlighted, relying on the present url; as an example if we resolve that the
url that reveals solely the “Energetic” gadgets is/energetic
, then when the present url
is/energetic
, the “Energetic” button ought to be surrounded by a skinny pink
rectangle - The “Clear accomplished” button ought to solely be seen if any merchandise is
accomplished
Every of this issues may be examined with the assistance of CSS selectors.
It is a snippet from the TodoMVC template (barely simplified). I
haven’t but added the dynamic bits, so what we see right here is static
content material, supplied for instance:
index.tmpl
<part class="todoapp"> <ul class="todo-list"> <!-- These are right here simply to indicate the construction of the checklist gadgets --> <!-- Checklist gadgets ought to get the category `accomplished` when marked as accomplished --> <li class="accomplished"> ② <div class="view"> <enter class="toggle" sort="checkbox" checked> <label>Style JavaScript</label> ① <button class="destroy"></button> </div> </li> <li> <div class="view"> <enter class="toggle" sort="checkbox"> <label>Purchase a unicorn</label> ① <button class="destroy"></button> </div> </li> </ul> <footer class="footer"> <!-- This ought to be `0 gadgets left` by default --> <span class="todo-count"><robust>0</robust> merchandise left</span> ⓷ <ul class="filters"> <li> <a class="chosen" href="#/">All</a> ④ </li> <li> <a href="#/energetic">Energetic</a> </li> <li> <a href="#/accomplished">Accomplished</a> </li> </ul> <!-- Hidden if no accomplished gadgets are left ↓ --> <button class="clear-completed">Clear accomplished</button> ⑤ </footer> </part>
By trying on the static model of the template, we will deduce which
CSS selectors can be utilized to establish the related components for the 5 dynamic
options listed above:
function | CSS selector | |
---|---|---|
① | All of the gadgets | ul.todo-list li |
② | Accomplished gadgets | ul.todo-list li.accomplished |
⓷ | Objects left | span.todo-count |
④ | Highlighted navigation hyperlink | ul.filters a.chosen |
⑤ | Clear accomplished button | button.clear-completed |
We will use these selectors to focus our assessments on simply the issues we need to check.
Testing HTML content material
The primary check will search for all of the gadgets, and show that the info
arrange by the check is rendered appropriately.
func Test_todoItemsAreShown(t *testing.T) { mannequin := todo.NewList() mannequin.Add("Foo") mannequin.Add("Bar") buf := renderTemplate(mannequin) // assert there are two <li> components contained in the <ul class="todo-list"> // assert the primary <li> textual content is "Foo" // assert the second <li> textual content is "Bar" }
We’d like a strategy to question the HTML doc with our CSS selector;
library for Go is goquery, that implements an API impressed by jQuery.
In Java, we hold utilizing the identical library we used to check for sound HTML, particularly
jsoup. Our check turns into:
Go
func Test_todoItemsAreShown(t *testing.T) { mannequin := todo.NewList() mannequin.Add("Foo") mannequin.Add("Bar") buf := renderTemplate("index.tmpl", mannequin) // parse the HTML with goquery doc, err := goquery.NewDocumentFromReader(bytes.NewReader(buf.Bytes())) if err != nil { // if parsing fails, we cease the check right here with t.FatalF t.Fatalf("Error rendering template %s", err) } // assert there are two <li> components contained in the <ul class="todo-list"> choice := doc.Discover("ul.todo-list li") assert.Equal(t, 2, choice.Size()) // assert the primary <li> textual content is "Foo" assert.Equal(t, "Foo", textual content(choice.Nodes[0])) // assert the second <li> textual content is "Bar" assert.Equal(t, "Bar", textual content(choice.Nodes[1])) } func textual content(node *html.Node) string { // Just a little mess as a result of the truth that goquery has // a .Textual content() methodology on Choice however not on html.Node sel := goquery.Choice{Nodes: []*html.Node{node}} return strings.TrimSpace(sel.Textual content()) }
Java
@Take a look at void todoItemsAreShown() throws IOException { var mannequin = new TodoList(); mannequin.add("Foo"); mannequin.add("Bar"); var html = renderTemplate("/index.tmpl", mannequin); // parse the HTML with jsoup Doc doc = Jsoup.parse(html, ""); // assert there are two <li> components contained in the <ul class="todo-list"> var choice = doc.choose("ul.todo-list li"); assertThat(choice).hasSize(2); // assert the primary <li> textual content is "Foo" assertThat(choice.get(0).textual content()).isEqualTo("Foo"); // assert the second <li> textual content is "Bar" assertThat(choice.get(1).textual content()).isEqualTo("Bar"); }
If we nonetheless have not modified the template to populate the checklist from the
mannequin, this check will fail, as a result of the static template
todo gadgets have completely different textual content:
Go
--- FAIL: Test_todoItemsAreShown (0.00s) index_template_test.go:44: First checklist merchandise: need Foo, acquired Style JavaScript index_template_test.go:49: Second checklist merchandise: need Bar, acquired Purchase a unicorn
Java
IndexTemplateTest > todoItemsAreShown() FAILED org.opentest4j.AssertionFailedError: Anticipating: <"Style JavaScript"> to be equal to: <"Foo"> however was not.
We repair it by making the template use the mannequin knowledge:
Go
<ul class="todo-list"> {{ vary .Objects }} <li> <div class="view"> <enter class="toggle" sort="checkbox"> <label>{{ .Title }}</label> <button class="destroy"></button> </div> </li> {{ finish }} </ul>
Java – jmustache
<ul class="todo-list"> {{ #allItems }} <li> <div class="view"> <enter class="toggle" sort="checkbox"> <label>{{ title }}</label> <button class="destroy"></button> </div> </li> {{ /allItems }} </ul>
Take a look at each content material and soundness on the identical time
Our check works, however it’s a bit verbose, particularly the Go model. If we’ll have extra
assessments, they’ll develop into repetitive and tough to learn, so we make it extra concise by extracting a helper operate for parsing the html. We additionally take away the
feedback, because the code ought to be clear sufficient
Go
func Test_todoItemsAreShown(t *testing.T) { mannequin := todo.NewList() mannequin.Add("Foo") mannequin.Add("Bar") buf := renderTemplate("index.tmpl", mannequin) doc := parseHtml(t, buf) choice := doc.Discover("ul.todo-list li") assert.Equal(t, 2, choice.Size()) assert.Equal(t, "Foo", textual content(choice.Nodes[0])) assert.Equal(t, "Bar", textual content(choice.Nodes[1])) } func parseHtml(t *testing.T, buf bytes.Buffer) *goquery.Doc { doc, err := goquery.NewDocumentFromReader(bytes.NewReader(buf.Bytes())) if err != nil { // if parsing fails, we cease the check right here with t.FatalF t.Fatalf("Error rendering template %s", err) } return doc }
Java
@Take a look at void todoItemsAreShown() throws IOException { var mannequin = new TodoList(); mannequin.add("Foo"); mannequin.add("Bar"); var html = renderTemplate("/index.tmpl", mannequin); var doc = parseHtml(html); var choice = doc.choose("ul.todo-list li"); assertThat(choice).hasSize(2); assertThat(choice.get(0).textual content()).isEqualTo("Foo"); assertThat(choice.get(1).textual content()).isEqualTo("Bar"); } non-public static Doc parseHtml(String html) { return Jsoup.parse(html, ""); }
Significantly better! No less than in my view. Now that we extracted the parseHtml
helper, it is
a good suggestion to verify for sound HTML within the helper:
Go
func parseHtml(t *testing.T, buf bytes.Buffer) *goquery.Doc {
assertWellFormedHtml(t, buf)
doc, err := goquery.NewDocumentFromReader(bytes.NewReader(buf.Bytes()))
if err != nil {
// if parsing fails, we cease the check right here with t.FatalF
t.Fatalf("Error rendering template %s", err)
}
return doc
}
Java
non-public static Doc parseHtml(String html) { var parser = Parser.htmlParser().setTrackErrors(10); var doc = Jsoup.parse(html, "", parser); assertThat(parser.getErrors()).isEmpty(); return doc; }
And with this, we will eliminate the primary check that we wrote, as we are actually testing for sound HTML on a regular basis.
The second check
Now we’re in place for testing extra rendering logic. The
second dynamic function in our checklist is “Checklist gadgets ought to get the category
accomplished
when marked as accomplished”. We will write a check for this:
Go
func Test_completedItemsGetCompletedClass(t *testing.T) { mannequin := todo.NewList() mannequin.Add("Foo") mannequin.AddCompleted("Bar") buf := renderTemplate("index.tmpl", mannequin) doc := parseHtml(t, buf) choice := doc.Discover("ul.todo-list li.accomplished") assert.Equal(t, 1, choice.Measurement()) assert.Equal(t, "Bar", textual content(choice.Nodes[0])) }
Java
@Take a look at void completedItemsGetCompletedClass() { var mannequin = new TodoList(); mannequin.add("Foo"); mannequin.addCompleted("Bar"); var html = renderTemplate("/index.tmpl", mannequin); Doc doc = Jsoup.parse(html, ""); var choice = doc.choose("ul.todo-list li.accomplished"); assertThat(choice).hasSize(1); assertThat(choice.textual content()).isEqualTo("Bar"); }
And this check may be made inexperienced by including this little bit of logic to the
template:
Go
<ul class="todo-list">
{{ vary .Objects }}
<li class="{{ if .IsCompleted }}accomplished{{ finish }}">
<div class="view">
<enter class="toggle" sort="checkbox">
<label>{{ .Title }}</label>
<button class="destroy"></button>
</div>
</li>
{{ finish }}
</ul>
Java – jmustache
<ul class="todo-list">
{{ #allItems }}
<li class="{{ #isCompleted }}accomplished{{ /isCompleted }}">
<div class="view">
<enter class="toggle" sort="checkbox">
<label>{{ title }}</label>
<button class="destroy"></button>
</div>
</li>
{{ /allItems }}
</ul>
So little by little, we will check and add the varied dynamic options
that our template ought to have.
Make it straightforward so as to add new assessments
The primary of the 20 suggestions from the wonderful speak by Russ Cox on Go
Testing is “Make it straightforward so as to add new check circumstances“. Certainly, in Go there
is a bent to make most assessments parameterized, for this very motive.
Then again, whereas Java has
good help
for parameterized assessments with JUnit 5, they aren’t used as a lot.
Since our present two assessments have the identical construction, we
may issue them right into a single parameterized check.
A check case for us will include:
- A reputation (in order that we will produce clear error messages when the check
fails) - A mannequin (in our case a
todo.Checklist
) - A CSS selector
- An inventory of textual content matches that we anticipate finding once we run the CSS
selector on the rendered HTML.
So that is the info construction for our check circumstances:
Go
var testCases = []struct { identify string mannequin *todo.Checklist selector string matches []string }{ { identify: "all todo gadgets are proven", mannequin: todo.NewList(). Add("Foo"). Add("Bar"), selector: "ul.todo-list li", matches: []string{"Foo", "Bar"}, }, { identify: "accomplished gadgets get the 'accomplished' class", mannequin: todo.NewList(). Add("Foo"). AddCompleted("Bar"), selector: "ul.todo-list li.accomplished", matches: []string{"Bar"}, }, }
Java
document TestCase(String identify, TodoList mannequin, String selector, Checklist<String> matches) { @Override public String toString() { return identify; } } public static TestCase[] indexTestCases() { return new TestCase[]{ new TestCase( "all todo gadgets are proven", new TodoList() .add("Foo") .add("Bar"), "ul.todo-list li", Checklist.of("Foo", "Bar")), new TestCase( "accomplished gadgets get the 'accomplished' class", new TodoList() .add("Foo") .addCompleted("Bar"), "ul.todo-list li.accomplished", Checklist.of("Bar")), }; }
And that is our parameterized check:
Go
func Test_indexTemplate(t *testing.T) { for _, check := vary testCases { t.Run(check.identify, func(t *testing.T) { buf := renderTemplate("index.tmpl", check.mannequin) assertWellFormedHtml(t, buf) doc := parseHtml(t, buf) choice := doc.Discover(check.selector) require.Equal(t, len(check.matches), len(choice.Nodes), "sudden # of matches") for i, node := vary choice.Nodes { assert.Equal(t, check.matches[i], textual content(node)) } }) } }
Java
@ParameterizedTest @MethodSource("indexTestCases") void testIndexTemplate(TestCase check) { var html = renderTemplate("/index.tmpl", check.mannequin); var doc = parseHtml(html); var choice = doc.choose(check.selector); assertThat(choice).hasSize(check.matches.dimension()); for (int i = 0; i < check.matches.dimension(); i++) { assertThat(choice.get(i).textual content()).isEqualTo(check.matches.get(i)); } }
We will now run our parameterized check and see it go:
Go
$ go check -v === RUN Test_indexTemplate === RUN Test_indexTemplate/all_todo_items_are_shown === RUN Test_indexTemplate/completed_items_get_the_'accomplished'_class --- PASS: Test_indexTemplate (0.00s) --- PASS: Test_indexTemplate/all_todo_items_are_shown (0.00s) --- PASS: Test_indexTemplate/completed_items_get_the_'accomplished'_class (0.00s) PASS okay tdd-html-templates 0.608s
Java
$ ./gradlew check > Job :check IndexTemplateTest > testIndexTemplate(TestCase) > [1] all todo gadgets are proven PASSED IndexTemplateTest > testIndexTemplate(TestCase) > [2] accomplished gadgets get the 'accomplished' class PASSED
Be aware how, by giving a reputation to our check circumstances, we get very readable check output, each on the terminal and within the IDE:
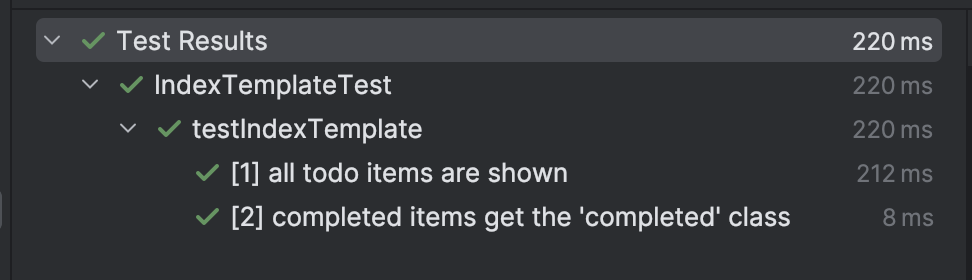
Having rewritten our two outdated assessments in desk kind, it is now tremendous straightforward so as to add
one other. That is the check for the “x gadgets left” textual content:
Go
{ identify: "gadgets left", mannequin: todo.NewList(). Add("One"). Add("Two"). AddCompleted("Three"), selector: "span.todo-count", matches: []string{"2 gadgets left"}, },
Java
new TestCase( "gadgets left", new TodoList() .add("One") .add("Two") .addCompleted("Three"), "span.todo-count", Checklist.of("2 gadgets left")),
And the corresponding change within the html template is:
Go
<span class="todo-count"><robust>{{len .ActiveItems}}</robust> gadgets left</span>
Java – jmustache
<span class="todo-count"><robust>{{activeItemsCount}}</robust> gadgets left</span>
The above change within the template requires a supporting methodology within the mannequin:
Go
sort Merchandise struct {
Title string
IsCompleted bool
}
sort Checklist struct {
Objects []*Merchandise
}
func (l *Checklist) ActiveItems() []*Merchandise {
var consequence []*Merchandise
for _, merchandise := vary l.Objects {
if !merchandise.IsCompleted {
consequence = append(consequence, merchandise)
}
}
return consequence
}
Java
public class TodoList {
non-public remaining Checklist<TodoItem> gadgets = new ArrayList<>();
// ...
public lengthy activeItemsCount() {
return gadgets.stream().filter(TodoItem::isActive).depend();
}
}
We have invested a bit effort in our testing infrastructure, in order that including new
check circumstances is less complicated. Within the subsequent part, we’ll see that the necessities
for the subsequent check circumstances will push us to refine our check infrastructure additional.
Making the desk extra expressive, on the expense of the check code
We’ll now check the “All”, “Energetic” and “Accomplished” navigation hyperlinks at
the underside of the UI (see the image above),
and these rely on which url we’re visiting, which is
one thing that our template has no strategy to discover out.
At the moment, all we go to our template is our mannequin, which is a todo-list.
It isn’t appropriate so as to add the at the moment visited url to the mannequin, as a result of that’s
person navigation state, not utility state.
So we have to go extra info to the template past the mannequin. A straightforward approach
is to go a map, which we assemble in our
renderTemplate
operate:
Go
func renderTemplate(mannequin *todo.Checklist, path string) bytes.Buffer { templ := template.Should(template.ParseFiles("index.tmpl")) var buf bytes.Buffer knowledge := map[string]any{ "mannequin": mannequin, "path": path, } err := templ.Execute(&buf, knowledge) if err != nil { panic(err) } return buf }
Java
non-public String renderTemplate(String templateName, TodoList mannequin, String path) { var template = Mustache.compiler().compile( new InputStreamReader( getClass().getResourceAsStream(templateName))); var knowledge = Map.of( "mannequin", mannequin, "path", path ); return template.execute(knowledge); }
And correspondingly our check circumstances desk has yet one more discipline:
Go
var testCases = []struct { identify string mannequin *todo.Checklist path string selector string matches []string }{ { identify: "all todo gadgets are proven", mannequin: todo.NewList(). Add("Foo"). Add("Bar"), selector: "ul.todo-list li", matches: []string{"Foo", "Bar"}, }, // ... the opposite circumstances { identify: "highlighted navigation hyperlink: All", path: "/", selector: "ul.filters a.chosen", matches: []string{"All"}, }, { identify: "highlighted navigation hyperlink: Energetic", path: "/energetic", selector: "ul.filters a.chosen", matches: []string{"Energetic"}, }, { identify: "highlighted navigation hyperlink: Accomplished", path: "/accomplished", selector: "ul.filters a.chosen", matches: []string{"Accomplished"}, }, }
Java
document TestCase(String identify, TodoList mannequin, String path, String selector, Checklist<String> matches) { @Override public String toString() { return identify; } } public static TestCase[] indexTestCases() { return new TestCase[]{ new TestCase( "all todo gadgets are proven", new TodoList() .add("Foo") .add("Bar"), "/", "ul.todo-list li", Checklist.of("Foo", "Bar")), // ... the earlier circumstances new TestCase( "highlighted navigation hyperlink: All", new TodoList(), "/", "ul.filters a.chosen", Checklist.of("All")), new TestCase( "highlighted navigation hyperlink: Energetic", new TodoList(), "/energetic", "ul.filters a.chosen", Checklist.of("Energetic")), new TestCase( "highlighted navigation hyperlink: Accomplished", new TodoList(), "/accomplished", "ul.filters a.chosen", Checklist.of("Accomplished")), }; }
We discover that for the three new circumstances, the mannequin is irrelevant;
whereas for the earlier circumstances, the trail is irrelevant. The Go syntax permits us
to initialize a struct with simply the fields we’re fascinated with, however Java doesn’t have
an analogous function, so we’re pushed to go additional info, and this makes the check circumstances
desk more durable to know.
A developer may take a look at the primary check case and marvel if the anticipated habits relies upon
on the trail being set to "/"
, and could be tempted so as to add extra circumstances with
a distinct path. In the identical approach, when studying the
highlighted navigation hyperlink check circumstances, the developer may marvel if the
anticipated habits depends upon the mannequin being set to an empty todo checklist. If that’s the case, one may
be led so as to add irrelevant check circumstances for the highlighted hyperlink with non-empty todo-lists.
We need to optimize for the time of the builders, so it is worthwhile to keep away from including irrelevant
knowledge to our check case. In Java we would go null
for the
irrelevant fields, however there’s a greater approach: we will use
the builder sample,
popularized by Joshua Bloch.
We will rapidly write one for the Java TestCase
document this fashion:
Java
document TestCase(String identify,
TodoList mannequin,
String path,
String selector,
Checklist<String> matches) {
@Override
public String toString() {
return identify;
}
public static remaining class Builder {
String identify;
TodoList mannequin;
String path;
String selector;
Checklist<String> matches;
public Builder identify(String identify) {
this.identify = identify;
return this;
}
public Builder mannequin(TodoList mannequin) {
this.mannequin = mannequin;
return this;
}
public Builder path(String path) {
this.path = path;
return this;
}
public Builder selector(String selector) {
this.selector = selector;
return this;
}
public Builder matches(String ... matches) {
this.matches = Arrays.asList(matches);
return this;
}
public TestCase construct() {
return new TestCase(identify, mannequin, path, selector, matches);
}
}
}
Hand-coding builders is a bit tedious, however doable, although there are
automated methods to put in writing them.
Now we will rewrite our Java check circumstances with the Builder
, to
obtain higher readability:
Java
public static TestCase[] indexTestCases() { return new TestCase[]{ new TestCase.Builder() .identify("all todo gadgets are proven") .mannequin(new TodoList() .add("Foo") .add("Bar")) .selector("ul.todo-list li") .matches("Foo", "Bar") .construct(), // ... different circumstances new TestCase.Builder() .identify("highlighted navigation hyperlink: Accomplished") .path("/accomplished") .selector("ul.filters a.chosen") .matches("Accomplished") .construct(), }; }
So, the place are we with our assessments? At current, they fail for the mistaken motive: null-pointer exceptions
as a result of lacking mannequin
and path
values.
With the intention to get our new check circumstances to fail for the appropriate motive, particularly that the template does
not but have logic to focus on the right hyperlink, we should
present default values for mannequin
and path
. In Go, we will do that
within the check methodology:
Go
func Test_indexTemplate(t *testing.T) {
for _, check := vary testCases {
t.Run(check.identify, func(t *testing.T) {
if check.mannequin == nil {
check.mannequin = todo.NewList()
}
buf := renderTemplate(check.mannequin, check.path)
// ... identical as earlier than
})
}
}
In Java, we will present default values within the builder:
Java
public static remaining class Builder { String identify; TodoList mannequin = new TodoList(); String path = "/"; String selector; Checklist<String> matches; // ... }
With these modifications, we see that the final two check circumstances, those for the highlighted hyperlink Energetic
and Accomplished fail, for the anticipated motive that the highlighted hyperlink doesn’t change:
Go
=== RUN Test_indexTemplate/highlighted_navigation_link:_Active index_template_test.go:82: Error Hint: .../tdd-templates/go/index_template_test.go:82 Error: Not equal: anticipated: "Energetic" precise : "All" === RUN Test_indexTemplate/highlighted_navigation_link:_Completed index_template_test.go:82: Error Hint: .../tdd-templates/go/index_template_test.go:82 Error: Not equal: anticipated: "Accomplished" precise : "All"
Java
IndexTemplateTest > testIndexTemplate(TestCase) > [5] highlighted navigation hyperlink: Energetic FAILED org.opentest4j.AssertionFailedError: Anticipating: <"All"> to be equal to: <"Energetic"> however was not. IndexTemplateTest > testIndexTemplate(TestCase) > [6] highlighted navigation hyperlink: Accomplished FAILED org.opentest4j.AssertionFailedError: Anticipating: <"All"> to be equal to: <"Accomplished"> however was not.
To make the assessments go, we make these modifications to the template:
Go
<ul class="filters"> <li> <a class="{{ if eq .path "/" }}chosen{{ finish }}" href="#/">All</a> </li> <li> <a class="{{ if eq .path "/energetic" }}chosen{{ finish }}" href="#/energetic">Energetic</a> </li> <li> <a class="{{ if eq .path "/accomplished" }}chosen{{ finish }}" href="#/accomplished">Accomplished</a> </li> </ul>
Java – jmustache
<ul class="filters"> <li> <a class="{{ #pathRoot }}chosen{{ /pathRoot }}" href="#/">All</a> </li> <li> <a class="{{ #pathActive }}chosen{{ /pathActive }}" href="#/energetic">Energetic</a> </li> <li> <a class="{{ #pathCompleted }}chosen{{ /pathCompleted }}" href="#/accomplished">Accomplished</a> </li> </ul>
For the reason that Mustache template language doesn’t permit for equality testing, we should change the
knowledge handed to the template in order that we execute the equality assessments earlier than rendering the template:
Java
non-public String renderTemplate(String templateName, TodoList mannequin, String path) { var template = Mustache.compiler().compile( new InputStreamReader( getClass().getResourceAsStream(templateName))); var knowledge = Map.of( "mannequin", mannequin, "pathRoot", path.equals("/"), "pathActive", path.equals("/energetic"), "pathCompleted", path.equals("/accomplished") ); return template.execute(knowledge); }
And with these modifications, all of our assessments now go.
To recap this part, we made the check code a bit bit extra difficult, in order that the check
circumstances are clearer: this can be a superb tradeoff!