[ad_1]
We all know that numerous types of written communication, like social media and emails, generate huge volumes of unstructured textual knowledge. This knowledge incorporates useful insights and data. Nevertheless, manually extracting related insights from massive quantities of uncooked textual content is very labor-intensive and time-consuming. Textual content mining addresses this problem. Utilizing laptop methods it refers to mechanically analyzing and reworking unstructured textual content knowledge to find patterns, traits, and important data. Computer systems have the flexibility to course of textual content written in human languages because of textual content mining. To seek out, extract, and measure related data from massive textual content collections, it makes use of pure language processing methods.
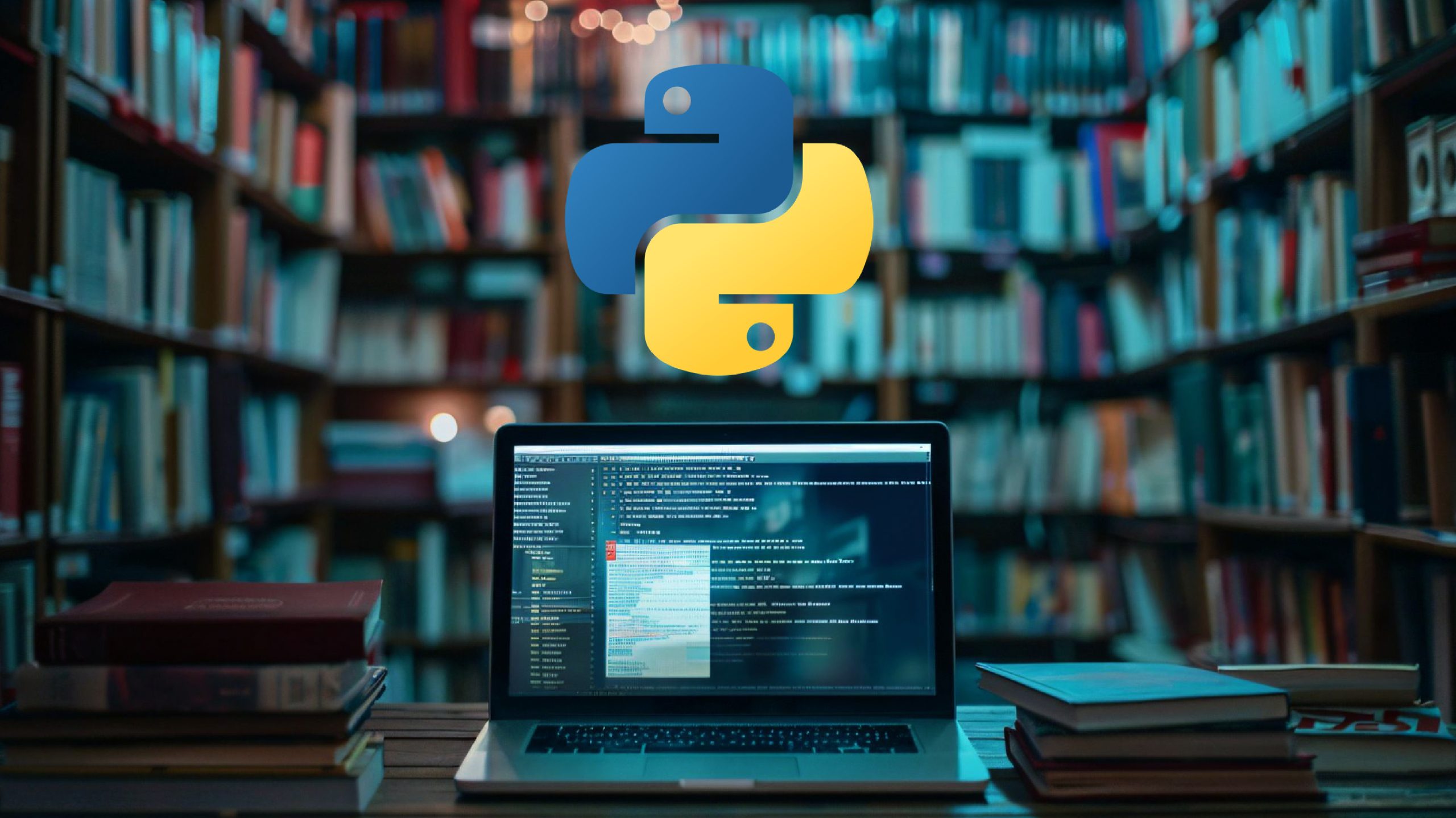
Overview
- Perceive textual content mining and its significance in numerous fields.
- Study primary textual content mining methods like tokenization, cease phrases removing and POS tagging.
- Discover real-world purposes of textual content mining in sentiment evaluation and named entity recognition.
Significance of Textual content Mining within the Fashionable World
Textual content mining is vital in lots of areas. It helps companies perceive what prospects really feel and enhance advertising. In healthcare, it’s used to have a look at affected person data and analysis papers. It additionally helps the police by checking authorized paperwork and social media for threats. Textual content mining is essential for pulling helpful data from textual content in several industries.
Understanding Pure Language Processing
Pure Language Processing is a kind of synthetic intelligence. It helps computer systems perceive and use human language to speak with folks. NLP permits computer systems to interpret and reply to what we are saying in a approach that is smart.
Key Ideas in NLP
- Stemming and Lemmatization: Cut back phrases to their primary type.
- Cease Phrases: Take away widespread phrases like “the,” “is,” and “at” that don’t add a lot which means.
- Half-of-Speech Tagging: Assign elements of speech, like nouns, verbs, and adjectives, to every phrase.
- Named Entity Recognition (NER): Determine correct names in textual content, corresponding to folks, organizations, and areas.
Getting Began with Textual content Mining in Python
Allow us to now look into the steps with which we will get began with textual content mining in Python.
Step1: Setting Up the Setting
To begin textual content mining in Python, you want an acceptable atmosphere. Python gives numerous libraries that simplify textual content mining duties.
Be sure to have Python put in. You possibly can obtain it from python.org.
Set Up a Digital Setting by typing the next code. It’s observe to create a digital atmosphere. This retains your mission dependencies remoted.
python -m venv textmining_env
supply textmining_env/bin/activate # On Home windows use `textmining_envScriptsactivate`
Step2: Putting in Mandatory Libraries
Python has a number of libraries for textual content mining. Listed below are the important ones:
- NLTK (Pure Language Toolkit): A robust library for NLP.
pip set up nltk
- Pandas: For knowledge manipulation and evaluation.
pip set up pandas
- NumPy: For numerical computations.
pip set up numpy
With these libraries, you’re prepared to begin textual content mining in Python.
Primary Terminologies in NLP
Allow us to discover primary terminologies in NLP.
Tokenization
Tokenization is step one in NLP. It entails breaking down textual content into smaller items referred to as tokens, often phrases or phrases. This course of is important for textual content evaluation as a result of it helps computer systems perceive and course of the textual content.
Instance Code and Output:
import nltk
from nltk.tokenize import word_tokenize
# Obtain the punkt tokenizer mannequin
nltk.obtain('punkt')
# Pattern textual content
textual content = "In Brazil, they drive on the right-hand facet of the highway."
# Tokenize the textual content
tokens = word_tokenize(textual content)
print(tokens)
Output:
['In', 'Brazil', ',', 'they', 'drive', 'on', 'the', 'right-hand', 'side', 'of', 'the', 'road', '.']
Stemming
Stemming reduces phrases to their root type. It removes suffixes to supply the stem of a phrase. There are two widespread varieties of stemmers: Porter and Lancaster.
- Porter Stemmer: Much less aggressive and broadly used.
- Lancaster Stemmer: Extra aggressive, generally eradicating greater than needed.
Instance Code and Output:
from nltk.stem import PorterStemmer, LancasterStemmer
# Pattern phrases
phrases = ["waited", "waiting", "waits"]
# Porter Stemmer
porter = PorterStemmer()
for phrase in phrases:
print(f"{phrase}: {porter.stem(phrase)}")
# Lancaster Stemmer
lancaster = LancasterStemmer()
for phrase in phrases:
print(f"{phrase}: {lancaster.stem(phrase)}")
Output:
waited: wait
ready: wait
waits: wait
waited: wait
ready: wait
waits: wait
Lemmatization
Lemmatization is much like stemming however considers the context. It converts phrases to their base or dictionary type. Not like stemming, lemmatization ensures that the bottom type is a significant phrase.
Instance Code and Output:
import nltk
from nltk.stem import WordNetLemmatizer
# Obtain the wordnet corpus
nltk.obtain('wordnet')
# Pattern phrases
phrases = ["rocks", "corpora"]
# Lemmatizer
lemmatizer = WordNetLemmatizer()
for phrase in phrases:
print(f"{phrase}: {lemmatizer.lemmatize(phrase)}")
Output:
rocks: rock
corpora: corpus
Cease Phrases
Cease phrases are widespread phrases that add little worth to textual content evaluation. Phrases like “the”, “is”, and “at” are thought-about cease phrases. Eradicating them helps give attention to the vital phrases within the textual content.
Instance Code and Output:
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# Pattern textual content
textual content = "Cristiano Ronaldo was born on February 5, 1985, in Funchal, Madeira, Portugal."
# Tokenize the textual content
tokens = word_tokenize(textual content.decrease())
# Take away cease phrases
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
# Obtain the stopwords corpus
nltk.obtain('stopwords')
# Take away cease phrases
stop_words = set(stopwords.phrases('english'))
filtered_tokens = [word for word in tokens if word not in stop_words]
print(filtered_tokens)
Output:
['cristiano', 'ronaldo', 'born', 'february', '5', ',', '1985', ',', 'funchal', ',', 'madeira', ',', 'portugal', '.']
Superior NLP Methods
Allow us to discover superior NLP methods.
A part of Speech Tagging (POS)
A part of Speech Tagging means marking every phrase in a textual content as a noun, verb, adjective, or adverb. It’s key for understanding how sentences are constructed. This helps break down sentences and see how phrases join, which is vital for duties like recognizing names, understanding feelings, and translating between languages.
Instance Code and Output:
import nltk
from nltk.tokenize import word_tokenize
from nltk import ne_chunk
# Pattern textual content
textual content = "Google's CEO Sundar Pichai launched the brand new Pixel at Minnesota Roi Centre Occasion."
# Tokenize the textual content
tokens = word_tokenize(textual content)
# POS tagging
pos_tags = nltk.pos_tag(tokens)
# NER
ner_tags = ne_chunk(pos_tags)
print(ner_tags)
Output:
(S
(GPE Google/NNP)
's/POS
(ORGANIZATION CEO/NNP Sundar/NNP Pichai/NNP)
launched/VBD
the/DT
new/JJ
Pixel/NNP
at/IN
(ORGANIZATION Minnesota/NNP Roi/NNP Centre/NNP)
Occasion/NNP
./.)
Chunking
Chunking teams small items, like phrases, into greater, significant items, like phrases. In NLP, chunking finds phrases in sentences, corresponding to noun or verb phrases. This helps perceive sentences higher than simply phrases. It’s vital for analyzing sentence construction and pulling out data.
Instance Code and Output:
import nltk
from nltk.tokenize import word_tokenize
# Pattern textual content
textual content = "We noticed the yellow canine."
# Tokenize the textual content
tokens = word_tokenize(textual content)
# POS tagging
pos_tags = nltk.pos_tag(tokens)
# Chunking
grammar = "NP: {<DT>?<JJ>*<NN>}"
chunk_parser = nltk.RegexpParser(grammar)
tree = chunk_parser.parse(pos_tags)
print(tree)
Output:
(S (NP We/PRP) noticed/VBD (NP the/DT yellow/JJ canine/NN) ./.)
Chunking helps in extracting significant phrases from textual content, which can be utilized in numerous NLP duties corresponding to parsing, data retrieval, and query answering.
Sensible Examples of Textual content Mining
Allow us to now discover sensible examples of textual content mining.
Sentiment Evaluation
Sentiment evaluation identifies feelings in textual content, like whether or not it’s constructive, detrimental, or impartial. It helps perceive folks’s emotions. Companies use it to study buyer opinions, monitor their fame, and enhance merchandise. It’s generally used to trace social media, analyze buyer suggestions, and conduct market analysis.
Textual content Classification
Textual content classification is about sorting textual content into set classes. It’s used so much find spam, analyzing emotions, and grouping matters. By mechanically tagging textual content, companies can higher arrange and deal with plenty of data.
Named Entity Extraction finds and types particular issues in textual content, like names of individuals, locations, organizations, and dates. It’s used to get data, pull out vital details, and enhance search engines like google and yahoo. NER turns messy textual content into organized knowledge by figuring out key parts.
Textual content mining is utilized in many areas:
- Buyer Service: It helps mechanically analyze buyer suggestions to make service higher.
- Healthcare: It pulls out vital particulars from medical notes and analysis papers to assist in medical research.
- Finance: It appears to be like at monetary stories and information articles to assist make smarter funding selections.
- Authorized: It accelerates the overview of authorized paperwork to seek out vital data rapidly.
Conclusion
Textual content mining in Python cleans up messy textual content and finds helpful insights. It makes use of methods like breaking textual content into phrases (tokenization), simplifying phrases (stemming and lemmatization), and labeling elements of speech (POS tagging). Superior steps like figuring out names (named entity recognition) and grouping phrases (chunking) enhance knowledge extraction. Sensible makes use of embrace analyzing feelings (sentiment evaluation) and sorting texts (textual content classification). Case research in e-commerce, healthcare, finance, and authorized present how textual content mining results in smarter selections and new concepts. As textual content mining evolves, it turns into important in at this time’s digital world.
Incessantly Requested Questions
A. Textual content mining is the method of using computational methods to extract significant patterns and traits from massive volumes of unstructured textual knowledge.
A. Textual content mining performs a vital function in unlocking useful insights which are usually embedded inside huge quantities of textual data.
A. Textual content mining finds purposes in numerous domains, together with sentiment evaluation of buyer evaluations and named entity recognition inside authorized paperwork.
[ad_2]