The way to use Non-compulsory in Java
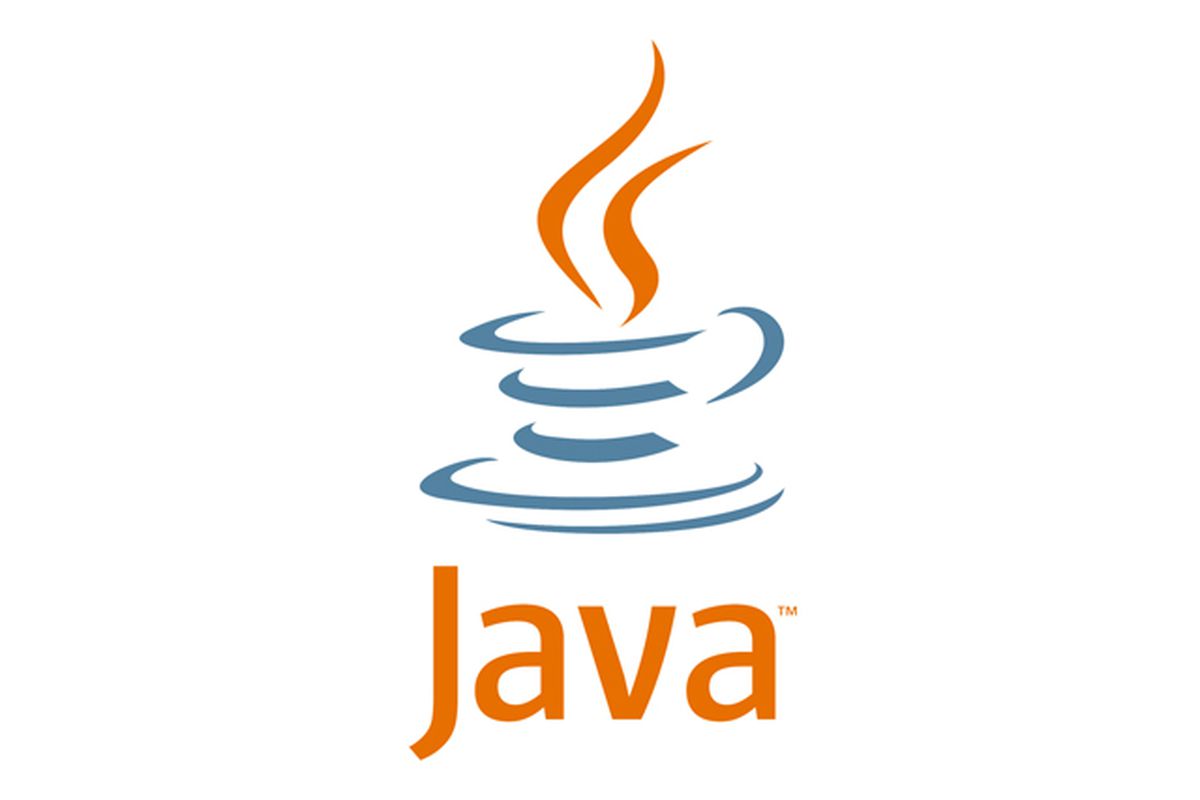
An Non-compulsory object in Java is a container object that may maintain each empty and a non-null values. If an Non-compulsory object does comprise a price, we are saying that it’s current; if it doesn’t comprise a price, we are saying that it’s empty. Right here, we’ll check out the Non-compulsory class in Java and the way it may be used to assist enhance your code. We will even have a look at a few of the drawbacks of utilizing the Non-compulsory key phrase in Java and a few finest practices.
Soar to:
What’s the Non-compulsory Kind in Java?
Non-compulsory is a brand new kind launched in Java 8. It’s used to signify a price which will or might not be current. In different phrases, an Non-compulsory object can both comprise a non-null worth (through which case it’s thought of current) or it could comprise no worth in any respect (through which case it’s thought of empty).
An Non-compulsory object can have one of many following potential states:
- Current: The Non-compulsory object doesn’t signify absence. A price is within the Non-compulsory object and it may be accessed by invoking the get() technique.
- Absent: The Non-compulsory object does signify the absence of a price; you can’t entry its content material with the get() technique.
Why Do Builders Want Non-compulsory in Java?
Non-compulsory is mostly used as a return kind for strategies that may not at all times have a outcome to return. For instance, a way that appears up a person by ID won’t discover a match, through which case it will return an empty Non-compulsory object.
Non-compulsory may help cut back the variety of null pointer exceptions in your code as nicely. It’s not supposed as a alternative for present reference sorts, akin to String or Checklist, however, fairly, as an addition to the Java kind system.
The way to Create an Non-compulsory Object in Java
There are a number of methods to create an Non-compulsory object in Java, together with the static manufacturing unit strategies empty() and of(), which pertain to the Non-compulsory class. You’ll be able to create an Non-compulsory object utilizing the of() technique, which can return an Non-compulsory object containing the given worth if the worth is non-null, or an empty Non-compulsory object if the worth is null.
Programmers also can use the ofNullable() technique, which can return an empty Non-compulsory object if the worth is null, or an Non-compulsory object containing the given worth whether it is non-null. Lastly, you possibly can create an empty Non-compulsory object utilizing the empty() technique.
After getting created an Non-compulsory object, you should utilize the isPresent() technique to examine if it incorporates a non-null worth. If it does, you should utilize the get() technique to retrieve the worth. Builders also can use the getOrElse() technique, which can return the worth whether it is current, or a default worth if it’s not.
Learn: Introduction to Interior Lessons in Java
The Java isPresent and ifPresent Strategies
Builders can make the most of the isPresent technique to examine if an Non-compulsory object is empty or non-empty. The ifPresent technique, in the meantime, can examine if a specific Non-compulsory object is non-empty. The next code instance illustrates how one can work with the ifPresent and isPresent strategies in Java:
import java.util.Non-compulsory; public class OptionalDemo { public static void foremost(String[] args) { Non-compulsory obj1 = Non-compulsory.of ("This can be a pattern textual content"); Non-compulsory obj2 = Non-compulsory.empty(); if (obj1.isPresent()) { System.out.println ("isPresent technique known as on obj1 returned true"); } obj1.ifPresent(s -> System.out.println ("ifPresent technique known as on obj1")); obj2.ifPresent(s -> System.out.println ("ifPresent technique known as on obj2 ")); } }
Within the above code instance, we first examine to see if two Non-compulsory object exists, utilizing the isPresent() technique. We assigned a price to obj1, so it should print out the string “This can be a pattern textual content”. obj2, nevertheless, was assigned an empty worth, so it should print out nothing. We then print some extra textual content to alert us that ifPresent was known as on each of our Non-compulsory objects.
The way to use Non-compulsory Objects in Java
There are a variety of how to create Non-compulsory objects. The commonest approach is to make use of the static manufacturing unit technique Non-compulsory.of(T), which creates an Non-compulsory object that’s current and incorporates the given non-null worth, as proven within the code snippet beneath:
Non-compulsory elective = Non-compulsory.of("worth");
Moreover, we will create an empty Non-compulsory object utilizing the static manufacturing unit technique Non-compulsory.empty, as proven within the code instance beneath:
Non-compulsory elective = Non-compulsory.empty();
If we’ve a price that may be null, we will use the static manufacturing unit technique Non-compulsory.ofNullable(T) to create an Non-compulsory object which will or might not be current:
Non-compulsory elective = Non-compulsory.ofNullable(null);
Programmers also can use strategies like ifPresent() and orElse() if you might want to carry out some motion based mostly on whether or not the elective has been set (if it incorporates a sure worth) or if not, respectively:
Non-compulsory optionalString = Non-compulsory.of("worth"); optionalString.ifPresent(s -> System.out.println(s));
Execs and Cons of utilizing Non-compulsory Objects in Java
There are a couple of key execs to utilizing Non-compulsory that Java builders ought to concentrate on, together with:
- Non-compulsory may help to forestall NullPointerException errors by making it specific when a variable might or might not comprise a price. This could result in cleaner and extra readable code.
- Non-compulsory offers a number of strategies that can be utilized to soundly work with knowledge which will or might not be current.
- Non-compulsory can be utilized as an peculiar class, which implies that there isn’t any want for particular syntax for invoking strategies or accessing fields.
Regardless of these advantages, there are a couple of potential downsides to utilizing Non-compulsory as nicely:
- Non-compulsory can add important overhead to code execution time, because the Non-compulsory wrapper have to be created and checked every time a variable is accessed.
- Some builders discover Non-compulsory complicated and tough to work with, which may result in extra errors as a substitute of fewer, and extra growth effort and time than common consequently.
Learn: Greatest Mission Administration Instruments for Builders
Options to Utilizing Non-compulsory Objects in Java
There are a couple of alternate options to utilizing Non-compulsory, akin to utilizing the null examine operator (?.), utilizing an if-else assertion, or utilizing a ternary operator.
The null examine operator can be utilized to examine if a price is null earlier than accessing it. This may be executed through the use of the ?. operator earlier than the variable identify. For instance, the next Java code will examine if the variable abc is null earlier than accessing it:
if (abc != null) { //Write your code right here }
If the variable abc isn’t null, the code contained in the if assertion shall be executed. The if-else assertion within the above code checks if the worth is null earlier than accessing it.
Greatest Practices for Utilizing Non-compulsory
Beneath are some finest practices to think about when utilizing Non-compulsory in your Java code:
- Use Non-compulsory to decrease the quantity of null pointer exceptions and account for instances when returned values are empty or lacking.
- Don’t use Non-compulsory as a stop-all for each kind of null pointers. Coders nonetheless have to account technique and constructor parameters which will additionally comprise empty values.
- Contemplate the context of your Non-compulsory objects; absent Non-compulsory values can imply various things, akin to a specific worth not being discovered versus no worth in any respect being discovered. Account for these prospects.
- Use Non-compulsory as a return kind after which retrieve its worth whether it is current or present a unique consequence if not.
- Don’t use Non-compulsory a parameter for strategies or constructors. Utilizing it in such method ends in sloppy, onerous to learn, and tough to take care of code.
Closing Ideas on Utilizing Non-compulsory Objects in Java
Non-compulsory is a brand new function in Java 8 that gives a solution to deal with null values in a extra elegant approach. The java.util.Non-compulsory class was launched in Java 8 as a solution to handle the widespread downside of null pointer exceptions. By utilizing Non-compulsory, programmers can keep away from NullPointerExceptions and write cleaner code.
Wish to study extra about objects and courses in Java? We suggest studying our tutorial What’s an Summary Class in Java as a subsequent step.