[ad_1]
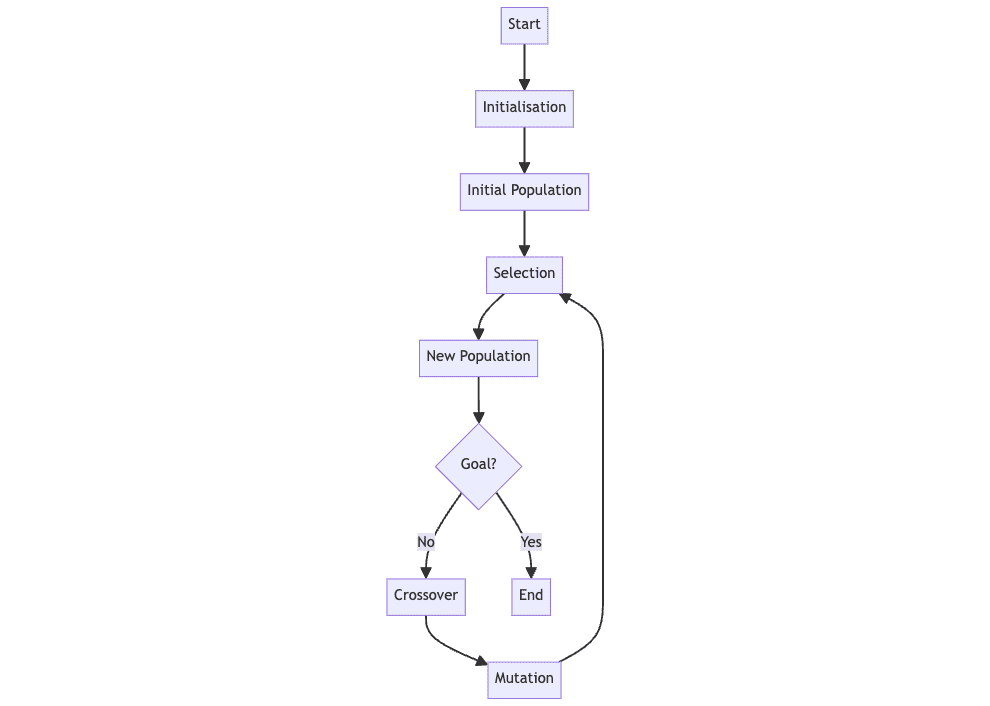
Picture by Writer
Genetic algorithms are methods based mostly on pure choice used to resolve advanced issues. They’re used to reach at cheap options to the issue quite than different strategies as a result of the issues are difficult. On this article, we are going to cowl the fundamentals of genetic algorithms and the way they are often applied in Python.
Genetic Elements
Health Perform
The health perform gauges the proximity of a thought-about answer to the absolute best answer to the issue. It gives a health stage for every individual within the inhabitants, which describes the standard or effectivity of the present era. This rating defines the selection whereas the upper health worth suggests an improved answer.
As an example, suppose we’re concerned within the technique of coping with an precise perform, f(x) during which x is a set of parameters. The optimum worth to seek out is x in order that f(x) assumes the most important worth.
Choice
This can be a course of that defines which people throughout the current era are to be favored and thus reproduce and contribute to the subsequent era. It’s attainable to determine many choice strategies, and every of them has its personal options and appropriate contexts.
- Roulette Wheel Choice:
Relying on the health stage of the person, the likelihood of selecting the person can also be maximal. - Match Choice:
A gaggle is randomly chosen and the very best of them is taken. - Rank-Primarily based Choice:
Individuals are sorted in keeping with health and choice chances are high proportionally allotted in keeping with the health scores.
Crossover
Crossover is a fundamental idea of genetic algorithm that goals on the alternate of genetic data of two father or mother people to kind a number of progeny. This course of is carefully just like the crossover and recombination of the biology taking place in nature. Making use of the essential rules of heredity, crossover makes an attempt to supply offspring that may embody fascinating traits of the mother and father and, thus, possess higher adaptation within the subsequent generations. Crossover is a comparatively broad idea which might be divided into a number of varieties every of which has their peculiarities and the sphere the place they are often utilized successfully.
- Single-Level Crossover: A crossover level is chosen on the father or mother chromosomes and just one crossover really occurs. Previous to this place all genes are taken from the primary father or mother, and all genes since this place are taken from the second father or mother.
- Two-Level Crossover: Two breakpoints are chosen and the half between them is swapped between the 2 father or mother chromosomes. It additionally favors interchanging of genetic data versus single level crossover.
Mutation
In Genetic Algorithms, mutation is of paramount significance as a result of it gives range which is an important issue when avoiding convergence instantly in the direction of the realm of the optimum options. Subsequently, getting random adjustments within the string of a person mutation permits the algorithm to enter different areas of the answer area that it can’t attain by way of crossover operations alone. This stochastic course of ensures that it doesn’t matter what, the inhabitants will evolve or shift its place within the areas of the search area which have been recognized as optimum by the genetic algorithm.
Steps To Implement A Genetic Algorithm
Let’s attempt to implement the genetic algorithm in Python.
Drawback Definition
Drawback: Compute on the precise perform; f(x) = x^2f(x) = x^2; solely integer values of x.
Health Perform: For the case of a chromosome that’s binary being x, an instance of the health perform could possibly be f(x)= x^2.
def health(chromosome):
x = int(''.be part of(map(str, chromosome)), 2)
return x ** 2
Inhabitants Initialization
Generate a random chromosome of a given size.
def generate_chromosome(size):
return [random.randint(0, 1) for _ in range(length)]
def generate_population(measurement, chromosome_length):
return [generate_chromosome(chromosome_length) for _ in range(size)]
population_size = 10
chromosome_length = 5
inhabitants = generate_population(population_size, chromosome_length)
Health Analysis
Consider the health of every chromosome within the inhabitants.
fitnesses = [fitness(chromosome) for chromosome in population]
Choice
Use roulette wheel choice to pick father or mother chromosomes based mostly on their health.
def select_pair(inhabitants, fitnesses):
total_fitness = sum(fitnesses)
selection_probs = [f / total_fitness for f in fitnesses]
parent1 = inhabitants[random.choices(range(len(population)), selection_probs)[0]]
parent2 = inhabitants[random.choices(range(len(population)), selection_probs)[0]]
return parent1, parent2
Crossover
Use single-point crossover by selecting a random cross-over place in a mother and father’ string and swapping all of the gene values after this location between the 2 strings.
def crossover(parent1, parent2):
level = random.randint(1, len(parent1) - 1)
offspring1 = parent1[:point] + parent2[point:]
offspring2 = parent2[:point] + parent1[point:]
return offspring1, offspring2
Mutation
Implement mutation by flipping bits with a sure likelihood.
def mutate(chromosome, mutation_rate):
return [gene if random.random() > mutation_rate else 1 - gene for gene in chromosome]
mutation_rate = 0.01
Wrapping Up
To sum up, genetic algorithms are constant and environment friendly for fixing optimization issues that can’t be solved instantly as they mimic the evolution of species. Thus, when you grasp the necessities of GAs and perceive methods to put them into follow in Python, the answer to advanced duties might be a lot simpler. Choice, crossover, and mutation keys allow you to make modifications in options and get the very best or almost finest solutions always. Having learn this text, you’re ready to use the genetic algorithms to your individual duties and thereby enhance in several duties and drawback fixing.
Jayita Gulati is a machine studying fanatic and technical author pushed by her ardour for constructing machine studying fashions. She holds a Grasp’s diploma in Laptop Science from the College of Liverpool.
[ad_2]