Understanding Reminiscence Consistency in Java Threads
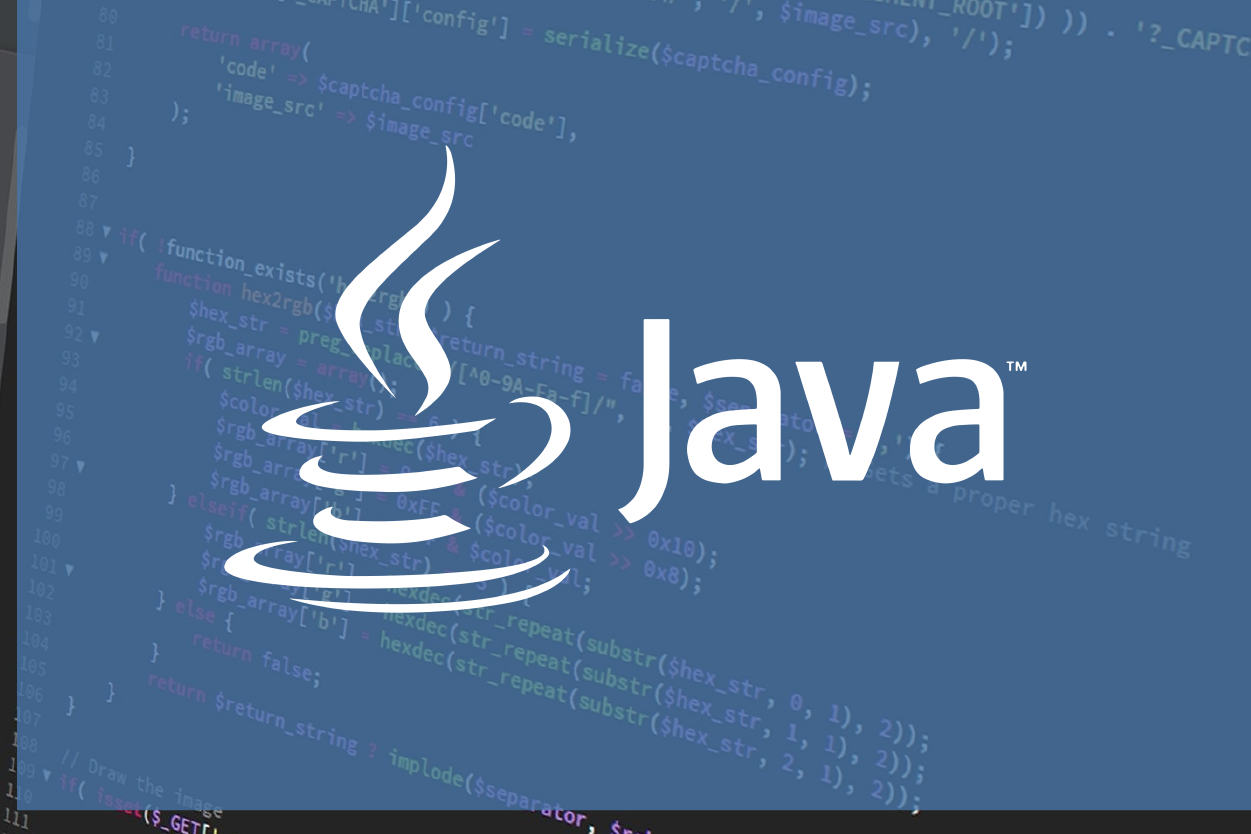
Java, as a flexible and widely-used programming language, offers help for multithreading, permitting builders to create concurrent functions that may execute a number of duties concurrently. Nonetheless, with the advantages of concurrency come challenges, and one of many vital features to think about is reminiscence consistency in Java threads.
In a multithreaded setting, a number of threads share the identical reminiscence house, resulting in potential points associated to information visibility and consistency. Reminiscence consistency refers back to the order and visibility of reminiscence operations throughout a number of threads. In Java, the Java Reminiscence Mannequin (JMM) defines the foundations and ensures for a way threads work together with reminiscence, making certain a degree of consistency that permits for dependable and predictable conduct.
Learn: Prime On-line Programs for Java
How Does Reminiscence Consistency in Java Work?
Understanding reminiscence consistency includes greedy ideas like atomicity, visibility, and ordering of operations. Let’s delve into these features to get a clearer image.
Atomicity
Within the context of multithreading, atomicity refers back to the indivisibility of an operation. An atomic operation is one which seems to happen instantaneously, with none interleaved operations from different threads. In Java, sure operations, akin to studying or writing to primitive variables (besides lengthy and double), are assured to be atomic. Nonetheless, compound actions, like incrementing a non-volatile lengthy, are usually not atomic.
Here’s a code instance demonstrating atomicity:
public class AtomicityExample { non-public int counter = 0; public void increment() { counter++; // Not atomic for lengthy or double } public int getCounter() { return counter; // Atomic for int (and different primitive varieties besides lengthy and double) } }
For atomic operations on lengthy and double, Java offers the java.util.concurrent.atomic package deal with lessons like AtomicLong and AtomicDouble, as proven under:
import java.util.concurrent.atomic.AtomicLong; public class AtomicExample { non-public AtomicLong atomicCounter = new AtomicLong(0); public void increment() { atomicCounter.incrementAndGet(); // Atomic operation } public lengthy getCounter() { return atomicCounter.get(); // Atomic operation } }
Visibility
Visibility refers as to if modifications made by one thread to shared variables are seen to different threads. In a multithreaded setting, threads could cache variables regionally, resulting in conditions the place modifications made by one thread are usually not instantly seen to others. To deal with this, Java offers the unstable key phrase.
public class VisibilityExample { non-public unstable boolean flag = false; public void setFlag() { flag = true; // Seen to different threads instantly } public boolean isFlag() { return flag; // At all times reads the newest worth from reminiscence } }
Utilizing unstable ensures that any thread studying the variable sees the newest write.
Ordering
Ordering pertains to the sequence by which operations seem like executed. In a multithreaded setting, the order by which statements are executed by completely different threads could not all the time match the order by which they have been written within the code. The Java Reminiscence Mannequin defines guidelines for establishing a happens-before relationship, making certain a constant order of operations.
public class OrderingExample { non-public int x = 0; non-public boolean prepared = false; public void write() { x = 42; prepared = true; } public int learn() { whereas (!prepared) { // Spin till prepared } return x; // Assured to see the write due to happens-before relationship } }
By understanding these primary ideas of atomicity, visibility, and ordering, builders can write thread-safe code and keep away from frequent pitfalls associated to reminiscence consistency.
Learn: Greatest Practices for Multithreading in Java
Thread Synchronization
Java offers synchronization mechanisms to regulate entry to shared sources and guarantee reminiscence consistency. The 2 essential synchronization mechanisms are synchronized strategies/blocks and the java.util.concurrent package deal.
Synchronized Strategies and Blocks
The synchronized key phrase ensures that just one thread can execute a synchronized methodology or block at a time, stopping concurrent entry and sustaining reminiscence consistency. Right here is an quick code instance demonstrating how one can use the synchronized key phrase in Java:
public class SynchronizationExample { non-public int sharedData = 0; public synchronized void synchronizedMethod() { // Entry and modify sharedData safely } public void nonSynchronizedMethod() { synchronized (this) { // Entry and modify sharedData safely } } }
Whereas synchronized offers a simple technique to obtain synchronization, it may result in efficiency points in sure conditions as a result of its inherent locking mechanism.
java.util.concurrent Package deal
The java.util.concurrent package deal introduces extra versatile and granular synchronization mechanisms, akin to Locks, Semaphores, and CountDownLatch. These lessons supply higher management over concurrency and could be extra environment friendly than conventional synchronization.
import java.util.concurrent.locks.Lock; import java.util.concurrent.locks.ReentrantLock; public class LockExample { non-public int sharedData = 0; non-public Lock lock = new ReentrantLock(); public void performOperation() { lock.lock(); attempt { // Entry and modify sharedData safely } lastly { lock.unlock(); } } }
Utilizing locks permits for extra fine-grained management over synchronization and might result in improved efficiency in conditions the place conventional synchronization is perhaps too coarse.
Reminiscence Consistency Ensures
The Java Reminiscence Mannequin offers a number of ensures to make sure reminiscence consistency and a constant and predictable order of execution for operations in multithreaded applications:
- Program Order Rule: Every motion in a thread happens-before each motion in that thread that comes later in this system order.
- Monitor Lock Rule: An unlock on a monitor happens-before each subsequent lock on that monitor.
- Unstable Variable Rule: A write to a unstable area happens-before each subsequent learn of that area.
- Thread Begin Rule: A name to Thread.begin on a thread happens-before any motion within the began thread.
- Thread Termination Rule: Any motion in a thread happens-before another thread detects that thread has terminated.
Sensible Suggestions for Managing Reminiscence Consistency
Now that we’ve lined the basics, let’s discover some sensible suggestions for managing reminiscence consistency in Java threads.
1. Use unstable Correctly
Whereas unstable ensures visibility, it doesn’t present atomicity for compound actions. Use unstable judiciously for easy flags or variables the place atomicity will not be a priority.
public class VolatileExample { non-public unstable boolean flag = false; public void setFlag() { flag = true; // Seen to different threads instantly, however not atomic } public boolean isFlag() { return flag; // At all times reads the newest worth from reminiscence } }
2. Make use of Thread-Secure Collections
Java offers thread-safe implementations of frequent assortment lessons within the java.util.concurrent package deal, akin to ConcurrentHashMap and CopyOnWriteArrayList. Utilizing these lessons can eradicate the necessity for specific synchronization in lots of instances.
import java.util.Map; import java.util.concurrent.ConcurrentHashMap; public class ConcurrentHashMapExample { non-public Map<String, Integer> concurrentMap = new ConcurrentHashMap<>(); public void addToMap(String key, int worth) { concurrentMap.put(key, worth); // Thread-safe operation } public int getValue(String key) { return concurrentMap.getOrDefault(key, 0); // Thread-safe operation } }
You may study extra about thread-safe operations in our tutorial: Java Thread Security.
3. Atomic Courses for Atomic Operations
For atomic operations on variables like int and lengthy, think about using lessons from the java.util.concurrent.atomic package deal, akin to AtomicInteger and AtomicLong.
import java.util.concurrent.atomic.AtomicInteger; public class AtomicIntegerExample { non-public AtomicInteger atomicCounter = new AtomicInteger(0); public void increment() { atomicCounter.incrementAndGet(); // Atomic operation } public int getCounter() { return atomicCounter.get(); // Atomic operation } }
4. Wonderful-Grained Locking
As an alternative of utilizing coarse-grained synchronization with synchronized strategies, think about using finer-grained locks to enhance concurrency and efficiency.
import java.util.concurrent.locks.Lock; import java.util.concurrent.locks.ReentrantLock; public class FineGrainedLockingExample { non-public int sharedData = 0; non-public Lock lock = new ReentrantLock(); public void performOperation() { lock.lock(); attempt { // Entry and modify sharedData safely } lastly { lock.unlock(); } } }
5. Perceive the Occurs-Earlier than Relationship
Pay attention to the happens-before relationship outlined by the Java Reminiscence Mannequin (see the Reminiscence Consistency Ensures part above.) Understanding these relationships helps in writing right and predictable multithreaded code.
Last Ideas on Reminiscence Consistency in Java Threads
Reminiscence consistency in Java threads is a vital side of multithreaded programming. Builders want to pay attention to the Java Reminiscence Mannequin, perceive the ensures it offers, and make use of synchronization mechanisms judiciously. Through the use of strategies like unstable for visibility, locks for fine-grained management, and atomic lessons for particular operations, builders can guarantee reminiscence consistency of their concurrent Java functions.