[ad_1]
Introduction
The best way to get the shortest path? A intelligent problem-solver, nevertheless, if you happen to use the Grasping Greatest-First Search (GBFS) algorithm, you’re prepared to assist. Consider it as that one buddy who all the time places the most effective foot ahead. On this sequence of articles, I’ll clarify Grasping Greatest-First Search and present examples utilizing Python code. On this weblog put up, Allow us to see the wonders of Grasping Greatest-First Search whereas it makes good decisions and when it’s apt for the job.
Studying Outcomes
- Perceive the essential ideas of the Grasping Greatest-First Search (GBFS) algorithm.
- Discover ways to implement the GBFS algorithm in Python.
- Discover the usage of Euclidean distance as a heuristic for GBFS.
- Analyze the benefits and drawbacks of utilizing GBFS for pathfinding.
- Apply GBFS to unravel pathfinding issues in grid-based eventualities.
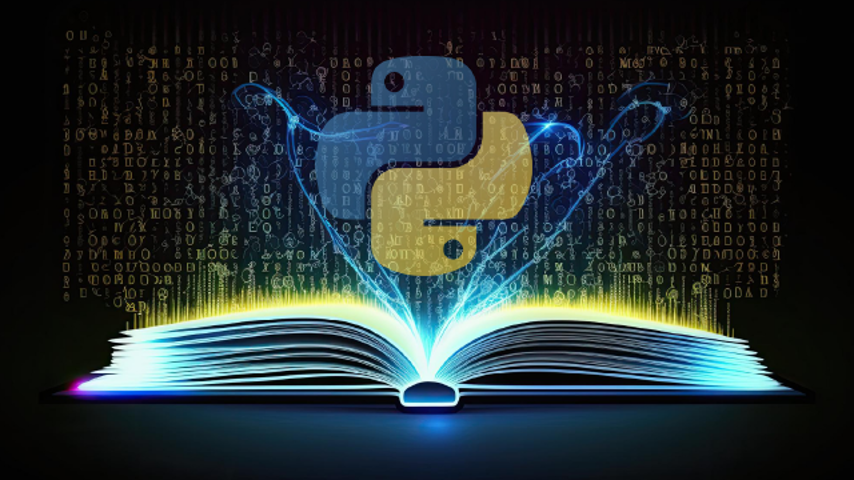
How does GBFS Work?
Right here’s a easy solution to perceive the GBFS algorithm:
- Begin in the beginning: You begin on the preliminary place or node.
- Consider choices: Have a look at all of the locations you may go subsequent.
- Select the best choice: Decide the place that appears closest to the objective.
- Repeat: Hold shifting to the best-looking subsequent place till you attain the objective.
Sounds easy, proper? However there’s a catch! The GBFS algorithm doesn’t all the time discover the shortest path as a result of it solely seems at what appears greatest proper now, not contemplating the entire journey.
Step-by-Step Instance
Let’s see an instance utilizing a easy grid. Think about we’ve got a 4×4 grid, and we wish to go from the top-left nook (0, 0) to the bottom-right nook (3, 3). Right here’s the grid with some obstacles:
[ [0, 1, 1, 1]
[1, 0, 1, 1]
[1, 0, 0, 1]
[1, 1, 0, 0] ]
On this grid, 1 means you may’t undergo that cell, and 0 means you may. We’ll use the Euclidean distance as our heuristic, which is only a fancy method of claiming the straight-line distance to the objective.
Writing the GBFS Algorithm in Python
Right here’s how we are able to write the Grasping Greatest-First Search algorithm in Python.
Python Code:
import heapq
import math
class Node:
def __init__(self, x, y, price):
self.x = x
self.y = y
self.price = price
def __lt__(self, different):
return self.price < different.price
def euclidean_distance(x1, y1, x2, y2):
return math.sqrt((x1 - x2)**2 + (y1 - y2)**2)
def greedy_best_first_search(grid, begin, objective):
rows = len(grid)
cols = len(grid[0])
pq = []
heapq.heappush(pq, Node(begin[0], begin[1], 0))
visited = set()
visited.add((begin[0], begin[1]))
instructions = [(-1, 0), (1, 0), (0, -1), (0, 1)]
whereas pq:
present = heapq.heappop(pq)
if (present.x, present.y) == objective:
print(f"Objective reached at ({present.x}, {present.y})")
return
for d in instructions:
new_x, new_y = present.x + d[0], present.y + d[1]
if 0 <= new_x < rows and 0 <= new_y < cols and grid[new_x][new_y] == 0 and (new_x, new_y) not in visited:
price = euclidean_distance(new_x, new_y, objective[0], objective[1])
heapq.heappush(pq, Node(new_x, new_y, price))
visited.add((new_x, new_y))
print("Objective not reachable")
# Instance grid
grid = [
[0, 1, 1, 1],
[1, 0, 1, 1],
[1, 0, 0, 1],
[1, 1, 0, 0]
]
begin = (0, 0)
objective = (3, 3)
greedy_best_first_search(grid, begin, objective)
Clarification of the Code
- Node Class: This class represents some extent within the grid. It shops the x and y coordinates and the associated fee to succeed in that node.
- Euclidean Distance: This perform calculates the straight-line distance between two factors, which we use as our heuristic.
- Precedence Queue: We use Python’s `heapq` to handle our precedence queue. This helps us all the time choose the following node with the smallest price.
- Visited Set: To maintain monitor of the nodes we’ve got already checked, we use a set known as `visited`.
- Instructions: These are the potential strikes (up, down, left, proper) we are able to make from any level.
Operating the Algorithm
While you run this code, it begins from the top-left nook (0, 0) and tries to maneuver to the bottom-right nook (3, 3). It picks the following step primarily based on which one seems closest to the objective utilizing the Euclidean distance.
Benefits and Disadvantages
Benefits:
- Easy and Simple to Implement: The GBFS algorithm is simple to know.
- Quick: It will possibly shortly discover a path to the objective if the heuristic is sweet.
Disadvantages:
- Not All the time Optimum: It doesn’t assure the shortest path.
- Can Get Caught: Generally, it’d get caught in a loop or go down a dead-end path if the heuristic is deceptive.
Conclusion
The Grasping Greatest-First Search algorithm supplies a precious method for tackling pathfinding issues in grids or graphs. Its energy lies in quickly figuring out promising routes towards the objective by leveraging a well-designed heuristic perform. Nevertheless, it’s essential to know that the GBFS strategy doesn’t assure discovering the optimum, shortest path. Its grasping nature could typically lead it astray if the heuristic is imperfect or deceptive.
Regardless of this limitation, the algorithm’s simplicity, effectivity, and skill to supply fairly good options shortly make it a precious instrument for programmers, significantly in time-sensitive conditions the place a near-optimal resolution is preferable to an exhaustive however computationally costly seek for absolutely the shortest path. Cautious implementation and heuristic design may also help harness the facility of GBFS for a variety of pathfinding challenges.
Ceaselessly Requested Questions
A. The Grasping Greatest-First Search algorithm is a pathfinding method that selects the following transfer primarily based on which possibility seems closest to the objective, utilizing a heuristic to information its choices.
A. In contrast to algorithms like A* that think about each the present price and the estimated price to the objective, GBFS focuses solely on the heuristic estimate to the objective, making it quicker however not all the time optimum.
A. No, GBFS doesn’t assure the shortest path as a result of it solely considers the heuristic estimate and never the general price from the begin to the objective.
[ad_2]