Utilizing SQL with Python: SQLAlchemy and Pandas
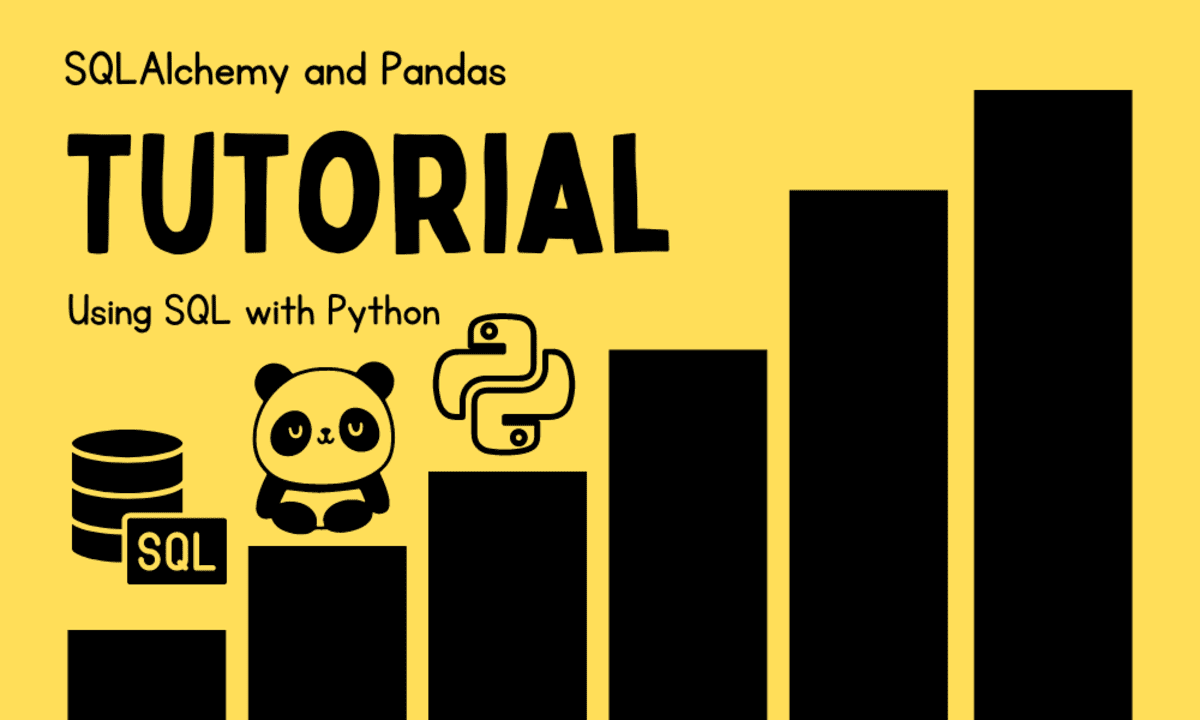
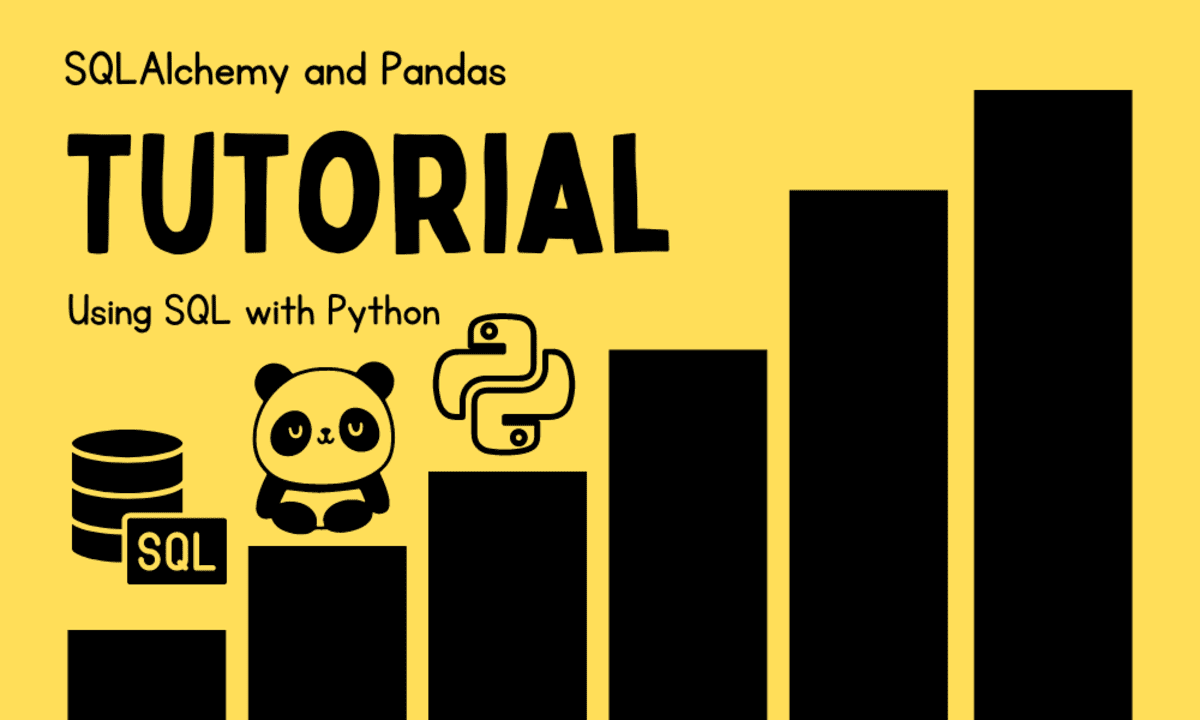
Picture by Creator
As a knowledge scientist, you want Python for detailed knowledge evaluation, knowledge visualization, and modeling. Nonetheless, when your knowledge is saved in a relational database, it’s good to use SQL (Structured Question Language) to extract and manipulate the info. However how do you combine SQL with Python to unlock the complete potential of your knowledge?
On this tutorial, we are going to be taught to mix the ability of SQL with the pliability of Python utilizing SQLAlchemy and Pandas. We’ll discover ways to connect with databases, execute SQL queries utilizing SQLAlchemy, and analyze and visualize knowledge utilizing Pandas.
Set up Pandas and SQLAlchemy utilizing:
pip set up pandas sqlalchemy
1. Saving the Pandas DataFrame as an SQL Desk
To create the SQL desk utilizing the CSV dataset, we are going to:
- Create a SQLite database utilizing the SQLAlchemy.
- Load the CSV dataset utilizing the Pandas. The countries_poluation dataset consists of the Air High quality Index (AQI) for all nations on this planet from 2017 to 2023.
- Convert all of the AQI columns from object to numerical and drop row with lacking values.
# Import needed packages
import pandas as pd
import psycopg2
from sqlalchemy import create_engine
# creating the brand new db
engine = create_engine(
"sqlite:///kdnuggets.db")
# learn the CSV dataset
knowledge = pd.read_csv("/work/air_pollution new.csv")
col = ['2017', '2018', '2019', '2020', '2021', '2022', '2023']
for s in col:
knowledge[s] = pd.to_numeric(knowledge[s], errors="coerce")
knowledge = knowledge.dropna(subset=[s])
- Save the Pandas dataframe as a SQL desk. The `to_sql` perform requires a desk title and the engine object.
# save the dataframe as a SQLite desk
knowledge.to_sql('countries_poluation', engine, if_exists="substitute")
Because of this, your SQLite database is saved in your file listing.
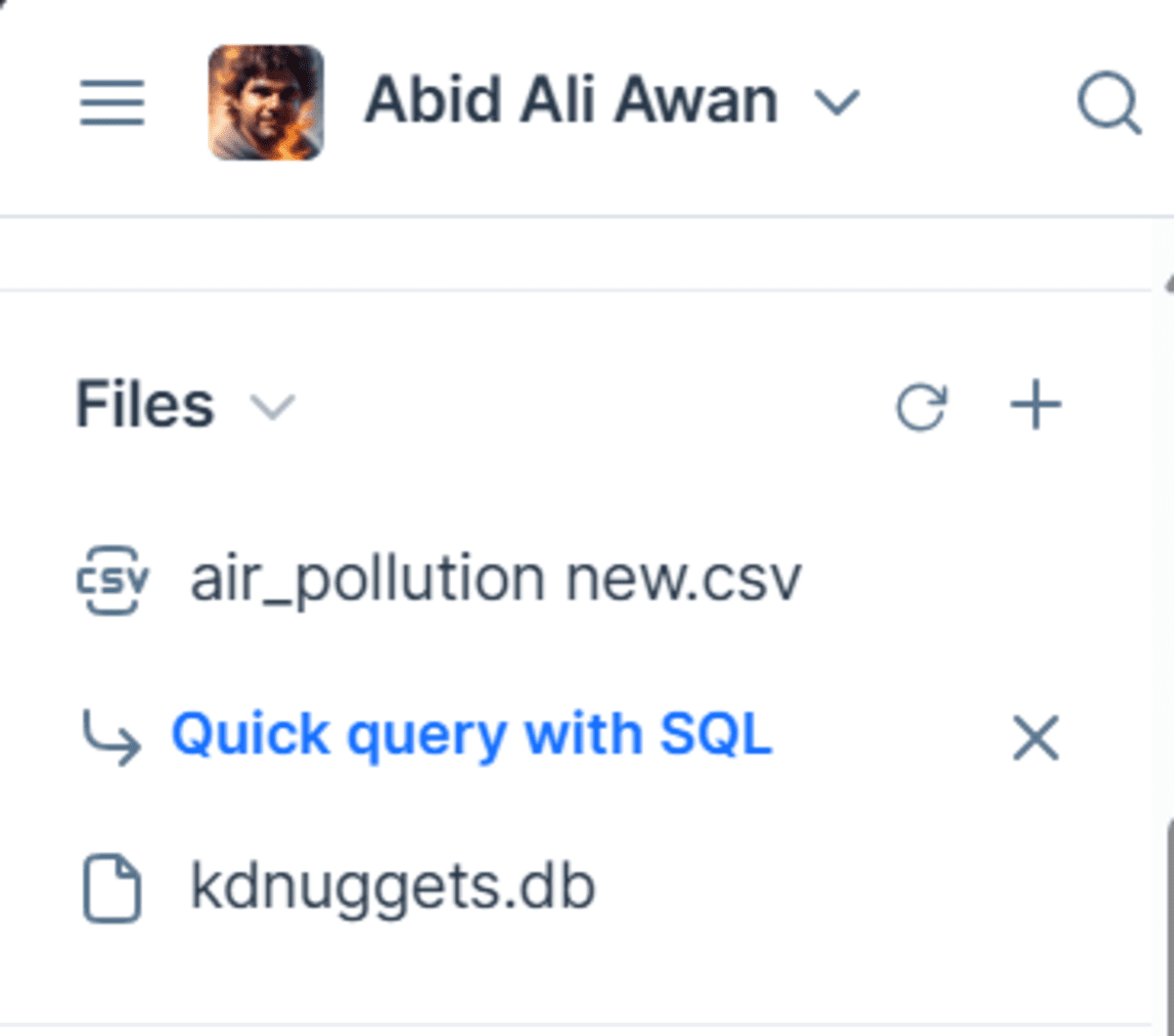
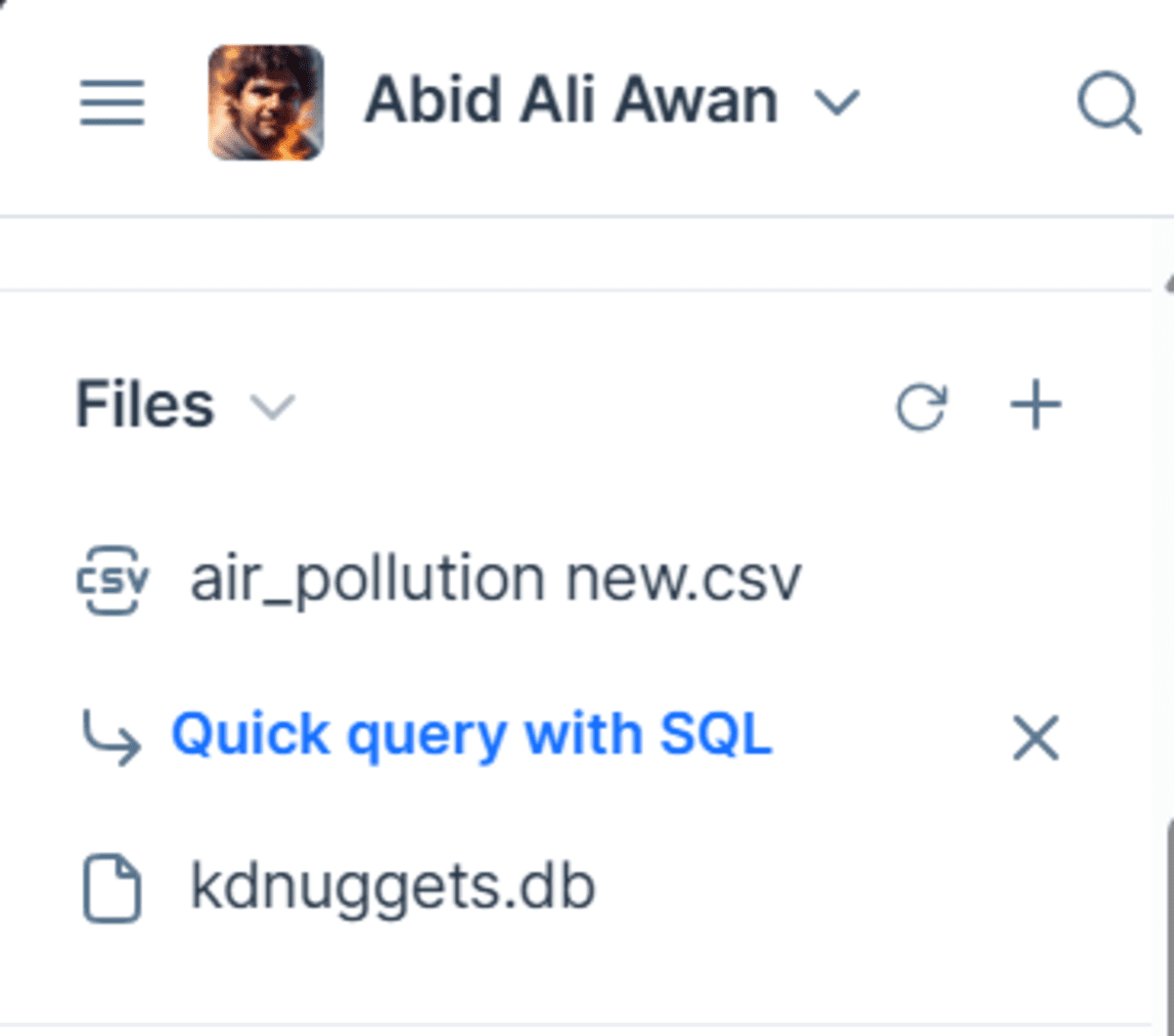
Observe: I’m utilizing Deepnote for this tutorial to run the Python code seamlessly. Deepnote is a free AI Cloud Pocket book that may allow you to rapidly run any knowledge science code.
2. Loading the SQL Desk utilizing Pandas
To load all the desk from the SQL database as a Pandas dataframe, we are going to:
- Set up the reference to our database by offering the database URL.
- Use the `pd.read_sql_table` perform to load all the desk and convert it right into a Pandas dataframe. The perform requires desk anime, engine objects, and column names.
- Show the highest 5 rows.
import pandas as pd
import psycopg2
from sqlalchemy import create_engine
# set up a reference to the database
engine = create_engine("sqlite:///kdnuggets.db")
# learn the sqlite desk
table_df = pd.read_sql_table(
"countries_poluation",
con=engine,
columns=['city', 'country', '2017', '2018', '2019', '2020', '2021', '2022',
'2023']
)
table_df.head()
The SQL desk has been efficiently loaded as a dataframe. This implies that you could now use it to carry out knowledge evaluation and visualization utilizing in style Python packages equivalent to Seaborn, Matplotlib, Scipy, Numpy, and extra.


3. Operating the SQL Question utilizing Pandas
As a substitute of limiting ourselves to 1 desk, we are able to entry all the database through the use of the `pd.read_sql` perform. Simply write a easy SQL question and supply it with the engine object.
The SQL question will show two columns from the “countries_population” desk, type it by the “2023” column, and show the highest 5 outcomes.
# learn desk knowledge utilizing sql question
sql_df = pd.read_sql(
"SELECT metropolis,[2023] FROM countries_poluation ORDER BY [2023] DESC LIMIT 5",
con=engine
)
print(sql_df)
We received to the highest 5 cities on this planet with the worst air high quality.
metropolis 2023
0 Lahore 97.4
1 Hotan 95.0
2 Bhiwadi 93.3
3 Delhi (NCT) 92.7
4 Peshawar 91.9
4. Utilizing the SQL Question Consequence with Pandas
We are able to additionally use the outcomes from SQL question and carry out additional evaluation. For instance, calculate the typical of the highest 5 cities utilizing Pandas.
average_air = sql_df['2023'].imply()
print(f"The typical of high 5 cities: {average_air:.2f}")
Output:
The typical of high 5 cities: 94.06
Or, create a bar plot by specifying the x and y arguments and the kind of plot.
sql_df.plot(x="metropolis",y="2023",sort = "barh");


Conclusion
The chances of utilizing SQLAlchemy with Pandas are limitless. You may carry out easy knowledge evaluation utilizing the SQL question, however to visualise the outcomes and even practice the machine studying mannequin, it’s a must to convert it right into a Pandas dataframe.
On this tutorial, now we have realized easy methods to load a SQL database into Python, carry out knowledge evaluation, and create visualizations. If you happen to loved this information, additionally, you will admire ‘A Information to Working with SQLite Databases in Python‘, which supplies an in-depth exploration of utilizing Python’s built-in sqlite3 module.
Abid Ali Awan (@1abidaliawan) is a licensed knowledge scientist skilled who loves constructing machine studying fashions. At present, he’s specializing in content material creation and writing technical blogs on machine studying and knowledge science applied sciences. Abid holds a Grasp’s diploma in expertise administration and a bachelor’s diploma in telecommunication engineering. His imaginative and prescient is to construct an AI product utilizing a graph neural community for college kids fighting psychological sickness.