[ad_1]
Introduction
Key-value pairs will be saved in dictionaries, that are dependable information constructions. Extraordinary dictionaries, nevertheless, didn’t protect the order of things as they had been launched earlier than to model 3.7. That is the use case for OrderedDict. A dictionary subclass known as OrderedDict retains monitor of the order by which keys are added. This text will go into nice element on OrderedDict, together with its options, functions, and variations from customary dictionaries.
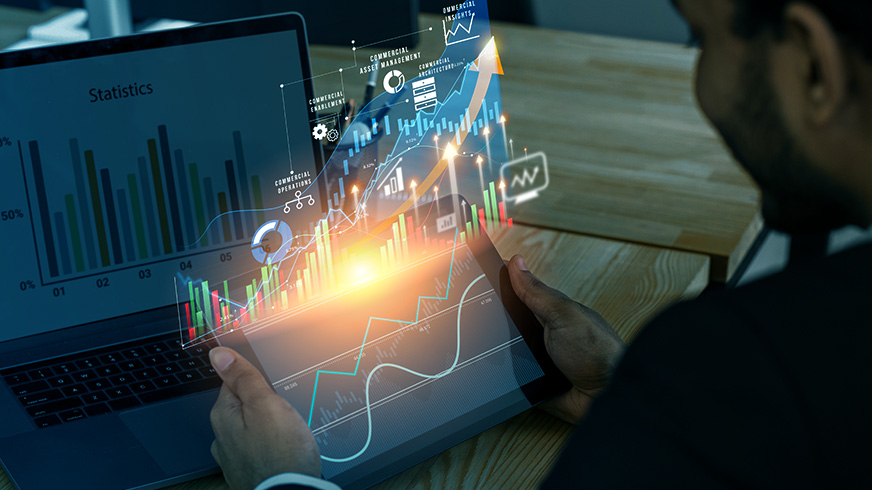
Overview
- Acknowledge the idea behind and performance of Python’s OrderedDict.
- Purchase the understanding wanted to create and work with OrderedDict objects.
- Verify the primary traits that set OrderedDict aside from typical dictionaries.
- Analyse helpful functions of OrderedDict in real-world contexts.
- In Python 3.7+, evaluate OrderedDict with regular dictionaries.
What’s OrderedDict?
An ordered dictionary is supplied within the collections module through the OrderedDict class. It retains the weather within the order that they had been initially put. This may be particularly useful in case your software’s elemental order is vital.
Let’s begin with a primary instance:
from collections import OrderedDict
# Creating an OrderedDict
od = OrderedDict()
od['apple'] = 1
od['banana'] = 2
od['cherry'] = 3
print(od)
Output:
OrderedDict([('apple', 1), ('banana', 2), ('cherry', 3)])
Creating an OrderedDict
There are a number of methods to create an OrderedDict:
from collections import OrderedDict
# Methodology 1: Creating an empty OrderedDict and including objects
od1 = OrderedDict()
od1['a'] = 1
od1['b'] = 2
# Methodology 2: Creating from a listing of tuples
od2 = OrderedDict([('x', 10), ('y', 20), ('z', 30)])
# Methodology 3: Creating from one other dictionary
regular_dict = {'p': 100, 'q': 200, 'r': 300}
od3 = OrderedDict(regular_dict)
print("od1:", od1)
print("od2:", od2)
print("od3:", od3)
Output:
#od1: OrderedDict([('a', 1), ('b', 2)])
#od2: OrderedDict([('x', 10), ('y', 20), ('z', 30)])
#od3: OrderedDict([('p', 100), ('q', 200), ('r', 300)])
Key Options of OrderedDict
Allow us to now discover key options of OrderedDict with instance.
Sustaining Insertion Order
Probably the most vital characteristic of OrderedDict is its capacity to take care of the order of insertion.
od = OrderedDict()
od['first'] = 1
od['second'] = 2
od['third'] = 3
for key, worth in od.objects():
print(f"{key}: {worth}")
Output:
#first: 1
#second: 2
#third: 3
Reordering
OrderedDict supplies strategies to maneuver objects to the start or finish of the dictionary.
od = OrderedDict([('a', 1), ('b', 2), ('c', 3)])
print("Unique:", od)
# Transfer 'c' to the start
od.move_to_end('c', final=False)
print("After transferring 'c' to the start:", od)
# Transfer 'a' to the tip
od.move_to_end('a')
print("After transferring 'a' to the tip:", od)
Output :
#Unique: OrderedDict([('a', 1), ('b', 2), ('c', 3)])
#After transferring 'c' to the start: OrderedDict([('c', 3), ('a', 1), ('b', 2)])
#After transferring 'a' to the tip: OrderedDict([('c', 3), ('b', 2), ('a', 1)])
Equality Comparability
OrderedDict considers the order when evaluating for equality.
od1 = OrderedDict([('a', 1), ('b', 2)])
od2 = OrderedDict([('b', 2), ('a', 1)])
regular_dict1 = {'a': 1, 'b': 2}
regular_dict2 = {'b': 2, 'a': 1}
print("od1 == od2:", od1 == od2)
print("regular_dict1 == regular_dict2:", regular_dict1 == regular_dict2)
Output :
#od1 == od2: False
#regular_dict1 == regular_dict2: True
Use Circumstances for OrderedDict
Allow us to now discover use circumstances of OrderedDict.
LRU (Least Not too long ago Used) Cache
OrderedDict is ideal for implementing an LRU cache.
from collections import OrderedDict
class LRUCache:
def __init__(self, capability):
self.cache = OrderedDict()
self.capability = capability
def get(self, key):
if key not in self.cache:
return -1
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, worth):
if key in self.cache:
self.cache.move_to_end(key)
self.cache[key] = worth
if len(self.cache) > self.capability:
self.cache.popitem(final=False)
# Utilization
lru = LRUCache(3)
lru.put(1, 1)
lru.put(2, 2)
lru.put(3, 3)
print(lru.cache)
lru.put(4, 4) # This can take away the least lately used merchandise (1)
print(lru.cache)
Output:
#OrderedDict([(1, 1), (2, 2), (3, 3)])
#OrderedDict([(2, 2), (3, 3), (4, 4)])
Sustaining Order in JSON
When working with JSON information the place order issues, OrderedDict will be helpful.
import json
from collections import OrderedDict
information = OrderedDict([
("name", "John Doe"),
("age", 30),
("city", "New York")
])
json_str = json.dumps(information)
print("JSON string:", json_str)
# Parsing again to OrderedDict
parsed_data = json.masses(json_str, object_pairs_hook=OrderedDict)
print("Parsed information:", parsed_data)
Output:
#JSON string: {"title": "John Doe", "age": 30, "metropolis": "New York"}
#Parsed information: OrderedDict([('name', 'John Doe'), ('age', 30), ('city', 'New York')])
OrderedDict vs. Common Dict ( 3.7+)
Since 3.7, common dictionaries additionally preserve insertion order. Nevertheless, there are nonetheless some variations:
# Common dict in 3.7+
regular_dict = {}
regular_dict['a'] = 1
regular_dict['b'] = 2
regular_dict['c'] = 3
# OrderedDict
from collections import OrderedDict
od = OrderedDict()
od['a'] = 1
od['b'] = 2
od['c'] = 3
print("Common dict:", regular_dict)
print("OrderedDict:", od)
# Equality comparability
print("regular_dict == od:", regular_dict == od)
print("sort(regular_dict) == sort(od):", sort(regular_dict) == sort(od))
Output:
Common dict: {‘a’: 1, ‘b’: 2, ‘c’: 3}
OrderedDict: OrderedDict([(‘a’, 1), (‘b’, 2), (‘c’, 3)])
regular_dict == od: True
sort(regular_dict) == sort(od): False
Whereas each preserve order, OrderedDict supplies extra strategies like `move_to_end()` and considers order in equality comparisons.
Conclusion
One efficient instrument for conserving dictionary objects of their right order is OrderedDict. Even when customary dictionaries in 3.7+ additionally hold issues organised, OrderedDict nonetheless has particular capabilities that come in useful typically. You could write simpler and organised code by understanding when and utilise OrderedDict, notably when working with order-sensitive information or developing specialised information constructions like LRU caches.
Ceaselessly Requested Questions
A. The primary distinction is that OrderedDict ensures the order of merchandise insertion and supplies extra strategies like move_to_end(). In Python variations prior to three.7, common dictionaries didn’t preserve order.
A. Use OrderedDict when the order of things is essential to your software, while you want operations like transferring objects to the start or finish, or when implementing order-sensitive information constructions like LRU caches.
A. OrderedDict could have barely larger reminiscence utilization and will be marginally slower for sure operations because of sustaining order. Nevertheless, for many functions, the distinction is negligible.
A. Sure, you possibly can create an OrderedDict from an everyday dictionary utilizing OrderedDict(regular_dict). Nevertheless, if the unique dictionary is unordered (Python < 3.7), the ensuing OrderedDict’s order could not match the insertion order.
A. Sure, you should use OrderedDict with JSON by utilizing the object_pairs_hook parameter in json.masses() to parse JSON into an OrderedDict, sustaining the order of keys.
[ad_2]