[ad_1]
Introduction
Think about you can make your Python applications run a lot quicker with out a lot effort. That’s what caching can do for you. Consider python caching as a method to save the solutions to exhausting issues so that you don’t have to resolve them once more. By holding these solutions helpful, your applications can skip the exhausting work and get outcomes shortly.
If you use pcaching, you retailer the outcomes of time-consuming calculations. The subsequent time your program wants that outcome, it might probably simply seize it from storage as a substitute of doing the calculation another time. This not solely hurries up your code but in addition makes it simpler to deal with advanced duties.
On this article, we’ll learn to use this highly effective method to turbocharge your code and obtain smoother, quicker Python experiences.
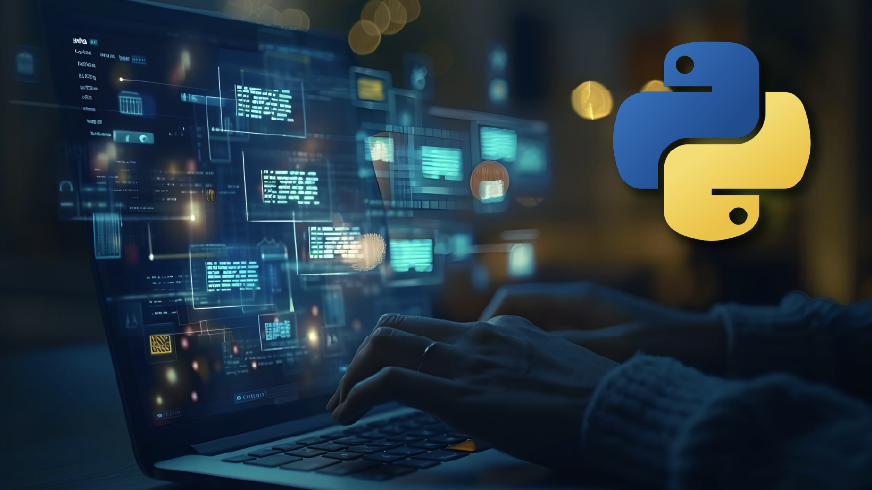
Overview
- Perceive the idea and advantages of caching in Python purposes.
- Implement caching utilizing Python’s built-in functools.lru_cache decorator.
- Create customized caching options utilizing dictionaries and exterior libraries like cachetools.
- Apply caching methods to optimize database queries and API requires improved efficiency.
What’s Caching?
Caching includes saving the outcomes of pricy or continuously executed operations in order that subsequent calls with the identical parameters can return the cached outcomes as a substitute of recomputing them. This reduces the time complexity, particularly for features with excessive computational prices or these which can be referred to as repeatedly with the identical inputs.
When to Use Caching
Caching is useful in situations the place:
- You’ve got features with costly computations.
- Capabilities are referred to as a number of instances with the identical arguments.
- The operate outcomes are immutable and deterministic.
Implementing Caching in Python
Python’s functools module supplies a built-in caching decorator referred to as lru_cache, which stands for Least Not too long ago Used cache. It’s simple to make use of and extremely efficient for a lot of use instances.
Utilizing functools.lru_cache
Right here’s how you should utilize lru_cache to cache operate outcomes:
Import the Decorator
from functools import lru_cache
Apply the Decorator
You apply lru_cache to a operate to cache its return values.
@lru_cache(maxsize=128)
def expensive_function(x):
# Simulate an costly computation
outcome = x * x
return outcome
maxsize specifies the variety of outcomes to cache. As soon as this restrict is reached, the least just lately used result’s discarded. Setting maxsize=None permits the cache to develop indefinitely.
Instance Utilization
import time
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
start_time = time.time()
print(fibonacci(35)) # First name will take longer
print("First name took", time.time() - start_time, "seconds")
start_time = time.time()
print(fibonacci(35)) # Subsequent calls are a lot quicker
print("Second name took", time.time() - start_time, "seconds")
Customized Caching Options
For extra advanced situations, you may want customized caching options. Python presents numerous libraries and methods for creating customized caches:
Utilizing a Dictionary
cache = {}
def expensive_function(x):
if x not in cache:
cache[x] = x * x # Simulate an costly computation
return cache[x]
Utilizing cachetools
The cachetools library supplies quite a lot of cache varieties and is extra versatile than lru_cache.
from cachetools import cached, LRUCache
cache = LRUCache(maxsize=128)
@cached(cache)
def expensive_function(x):
return x * x # Simulate an costly computation
Sensible Software
- Database Queries: Caching outcomes of database queries can considerably cut back the load in your database and enhance response instances.
query_cache = {}
def get_user_data(user_id):
if user_id not in query_cache:
# Simulate a database question
query_cache[user_id] = {"title": "John Doe", "age": 30}
return query_cache[user_id]
- API Calls: Cache the outcomes of API calls to keep away from hitting fee limits and cut back latency.
import requests
api_cache = {}
def get_weather(metropolis):
if metropolis not in api_cache:
response = requests.get(f'http://api.climate.com/{metropolis}')
api_cache[city] = response.json()
return api_cache[city]
Conclusion
Caching is a mechanism to optimise python code, particularly in terms of costly computations and performance calls which aren’t recurring. We will use this to construct our cache simply utilizing instruments already accessible in Python itself like functools.lry_cache or different customized methods to cache, enormous efficiency wins of the applying will be attained. Cache is an efficient instrument to avoid wasting time and assets, whether or not you might be optimizing database queries or API calls (as we’ll on this instance), computational features and so forth.
Steadily Requested Questions
A. It shops the outcomes of pricy operate calls and reuses them for a similar inputs to enhance efficiency.
A. Use caching for features with costly computations, frequent calls with the identical arguments, and immutable, deterministic outcomes.
A. Caching is helpful for optimizing database queries and API calls, decreasing load and enhancing response instances.
[ad_2]